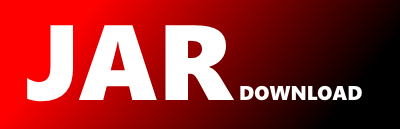
software.amazon.awssdk.services.outposts.model.CatalogItem Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a catalog item.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CatalogItem implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CATALOG_ITEM_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CatalogItemId").getter(getter(CatalogItem::catalogItemId)).setter(setter(Builder::catalogItemId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CatalogItemId").build()).build();
private static final SdkField ITEM_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ItemStatus").getter(getter(CatalogItem::itemStatusAsString)).setter(setter(Builder::itemStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ItemStatus").build()).build();
private static final SdkField> EC2_CAPACITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EC2Capacities")
.getter(getter(CatalogItem::ec2Capacities))
.setter(setter(Builder::ec2Capacities))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EC2Capacities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EC2Capacity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField POWER_KVA_FIELD = SdkField. builder(MarshallingType.FLOAT).memberName("PowerKva")
.getter(getter(CatalogItem::powerKva)).setter(setter(Builder::powerKva))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerKva").build()).build();
private static final SdkField WEIGHT_LBS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("WeightLbs").getter(getter(CatalogItem::weightLbs)).setter(setter(Builder::weightLbs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WeightLbs").build()).build();
private static final SdkField> SUPPORTED_UPLINK_GBPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedUplinkGbps")
.getter(getter(CatalogItem::supportedUplinkGbps))
.setter(setter(Builder::supportedUplinkGbps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedUplinkGbps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.INTEGER)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SUPPORTED_STORAGE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedStorage")
.getter(getter(CatalogItem::supportedStorageAsStrings))
.setter(setter(Builder::supportedStorageWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedStorage").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CATALOG_ITEM_ID_FIELD,
ITEM_STATUS_FIELD, EC2_CAPACITIES_FIELD, POWER_KVA_FIELD, WEIGHT_LBS_FIELD, SUPPORTED_UPLINK_GBPS_FIELD,
SUPPORTED_STORAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String catalogItemId;
private final String itemStatus;
private final List ec2Capacities;
private final Float powerKva;
private final Integer weightLbs;
private final List supportedUplinkGbps;
private final List supportedStorage;
private CatalogItem(BuilderImpl builder) {
this.catalogItemId = builder.catalogItemId;
this.itemStatus = builder.itemStatus;
this.ec2Capacities = builder.ec2Capacities;
this.powerKva = builder.powerKva;
this.weightLbs = builder.weightLbs;
this.supportedUplinkGbps = builder.supportedUplinkGbps;
this.supportedStorage = builder.supportedStorage;
}
/**
*
* The ID of the catalog item.
*
*
* @return The ID of the catalog item.
*/
public final String catalogItemId() {
return catalogItemId;
}
/**
*
* The status of a catalog item.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #itemStatus} will
* return {@link CatalogItemStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #itemStatusAsString}.
*
*
* @return The status of a catalog item.
* @see CatalogItemStatus
*/
public final CatalogItemStatus itemStatus() {
return CatalogItemStatus.fromValue(itemStatus);
}
/**
*
* The status of a catalog item.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #itemStatus} will
* return {@link CatalogItemStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #itemStatusAsString}.
*
*
* @return The status of a catalog item.
* @see CatalogItemStatus
*/
public final String itemStatusAsString() {
return itemStatus;
}
/**
* For responses, this returns true if the service returned a value for the EC2Capacities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEc2Capacities() {
return ec2Capacities != null && !(ec2Capacities instanceof SdkAutoConstructList);
}
/**
*
* Information about the EC2 capacity of an item.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEc2Capacities} method.
*
*
* @return Information about the EC2 capacity of an item.
*/
public final List ec2Capacities() {
return ec2Capacities;
}
/**
*
* Information about the power draw of an item.
*
*
* @return Information about the power draw of an item.
*/
public final Float powerKva() {
return powerKva;
}
/**
*
* The weight of the item in pounds.
*
*
* @return The weight of the item in pounds.
*/
public final Integer weightLbs() {
return weightLbs;
}
/**
* For responses, this returns true if the service returned a value for the SupportedUplinkGbps property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSupportedUplinkGbps() {
return supportedUplinkGbps != null && !(supportedUplinkGbps instanceof SdkAutoConstructList);
}
/**
*
* The uplink speed this catalog item requires for the connection to the Region.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedUplinkGbps} method.
*
*
* @return The uplink speed this catalog item requires for the connection to the Region.
*/
public final List supportedUplinkGbps() {
return supportedUplinkGbps;
}
/**
*
* The supported storage options for the catalog item.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedStorage} method.
*
*
* @return The supported storage options for the catalog item.
*/
public final List supportedStorage() {
return SupportedStorageListCopier.copyStringToEnum(supportedStorage);
}
/**
* For responses, this returns true if the service returned a value for the SupportedStorage property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSupportedStorage() {
return supportedStorage != null && !(supportedStorage instanceof SdkAutoConstructList);
}
/**
*
* The supported storage options for the catalog item.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedStorage} method.
*
*
* @return The supported storage options for the catalog item.
*/
public final List supportedStorageAsStrings() {
return supportedStorage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(catalogItemId());
hashCode = 31 * hashCode + Objects.hashCode(itemStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasEc2Capacities() ? ec2Capacities() : null);
hashCode = 31 * hashCode + Objects.hashCode(powerKva());
hashCode = 31 * hashCode + Objects.hashCode(weightLbs());
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedUplinkGbps() ? supportedUplinkGbps() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedStorage() ? supportedStorageAsStrings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CatalogItem)) {
return false;
}
CatalogItem other = (CatalogItem) obj;
return Objects.equals(catalogItemId(), other.catalogItemId())
&& Objects.equals(itemStatusAsString(), other.itemStatusAsString())
&& hasEc2Capacities() == other.hasEc2Capacities() && Objects.equals(ec2Capacities(), other.ec2Capacities())
&& Objects.equals(powerKva(), other.powerKva()) && Objects.equals(weightLbs(), other.weightLbs())
&& hasSupportedUplinkGbps() == other.hasSupportedUplinkGbps()
&& Objects.equals(supportedUplinkGbps(), other.supportedUplinkGbps())
&& hasSupportedStorage() == other.hasSupportedStorage()
&& Objects.equals(supportedStorageAsStrings(), other.supportedStorageAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CatalogItem").add("CatalogItemId", catalogItemId()).add("ItemStatus", itemStatusAsString())
.add("EC2Capacities", hasEc2Capacities() ? ec2Capacities() : null).add("PowerKva", powerKva())
.add("WeightLbs", weightLbs())
.add("SupportedUplinkGbps", hasSupportedUplinkGbps() ? supportedUplinkGbps() : null)
.add("SupportedStorage", hasSupportedStorage() ? supportedStorageAsStrings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CatalogItemId":
return Optional.ofNullable(clazz.cast(catalogItemId()));
case "ItemStatus":
return Optional.ofNullable(clazz.cast(itemStatusAsString()));
case "EC2Capacities":
return Optional.ofNullable(clazz.cast(ec2Capacities()));
case "PowerKva":
return Optional.ofNullable(clazz.cast(powerKva()));
case "WeightLbs":
return Optional.ofNullable(clazz.cast(weightLbs()));
case "SupportedUplinkGbps":
return Optional.ofNullable(clazz.cast(supportedUplinkGbps()));
case "SupportedStorage":
return Optional.ofNullable(clazz.cast(supportedStorageAsStrings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function