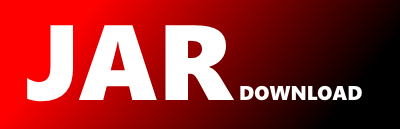
software.amazon.awssdk.services.outposts.model.RackPhysicalProperties Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the physical and logistical details for racks at sites. For more information about hardware
* requirements for racks, see Network readiness
* checklist in the Amazon Web Services Outposts User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RackPhysicalProperties implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField POWER_DRAW_KVA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerDrawKva").getter(getter(RackPhysicalProperties::powerDrawKvaAsString))
.setter(setter(Builder::powerDrawKva))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerDrawKva").build()).build();
private static final SdkField POWER_PHASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerPhase").getter(getter(RackPhysicalProperties::powerPhaseAsString))
.setter(setter(Builder::powerPhase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerPhase").build()).build();
private static final SdkField POWER_CONNECTOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerConnector").getter(getter(RackPhysicalProperties::powerConnectorAsString))
.setter(setter(Builder::powerConnector))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerConnector").build()).build();
private static final SdkField POWER_FEED_DROP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerFeedDrop").getter(getter(RackPhysicalProperties::powerFeedDropAsString))
.setter(setter(Builder::powerFeedDrop))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerFeedDrop").build()).build();
private static final SdkField UPLINK_GBPS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UplinkGbps").getter(getter(RackPhysicalProperties::uplinkGbpsAsString))
.setter(setter(Builder::uplinkGbps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UplinkGbps").build()).build();
private static final SdkField UPLINK_COUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UplinkCount").getter(getter(RackPhysicalProperties::uplinkCountAsString))
.setter(setter(Builder::uplinkCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UplinkCount").build()).build();
private static final SdkField FIBER_OPTIC_CABLE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FiberOpticCableType").getter(getter(RackPhysicalProperties::fiberOpticCableTypeAsString))
.setter(setter(Builder::fiberOpticCableType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FiberOpticCableType").build())
.build();
private static final SdkField OPTICAL_STANDARD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OpticalStandard").getter(getter(RackPhysicalProperties::opticalStandardAsString))
.setter(setter(Builder::opticalStandard))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OpticalStandard").build()).build();
private static final SdkField MAXIMUM_SUPPORTED_WEIGHT_LBS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaximumSupportedWeightLbs").getter(getter(RackPhysicalProperties::maximumSupportedWeightLbsAsString))
.setter(setter(Builder::maximumSupportedWeightLbs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumSupportedWeightLbs").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POWER_DRAW_KVA_FIELD,
POWER_PHASE_FIELD, POWER_CONNECTOR_FIELD, POWER_FEED_DROP_FIELD, UPLINK_GBPS_FIELD, UPLINK_COUNT_FIELD,
FIBER_OPTIC_CABLE_TYPE_FIELD, OPTICAL_STANDARD_FIELD, MAXIMUM_SUPPORTED_WEIGHT_LBS_FIELD));
private static final long serialVersionUID = 1L;
private final String powerDrawKva;
private final String powerPhase;
private final String powerConnector;
private final String powerFeedDrop;
private final String uplinkGbps;
private final String uplinkCount;
private final String fiberOpticCableType;
private final String opticalStandard;
private final String maximumSupportedWeightLbs;
private RackPhysicalProperties(BuilderImpl builder) {
this.powerDrawKva = builder.powerDrawKva;
this.powerPhase = builder.powerPhase;
this.powerConnector = builder.powerConnector;
this.powerFeedDrop = builder.powerFeedDrop;
this.uplinkGbps = builder.uplinkGbps;
this.uplinkCount = builder.uplinkCount;
this.fiberOpticCableType = builder.fiberOpticCableType;
this.opticalStandard = builder.opticalStandard;
this.maximumSupportedWeightLbs = builder.maximumSupportedWeightLbs;
}
/**
*
* The power draw available at the hardware placement position for the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerDrawKva} will
* return {@link PowerDrawKva#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerDrawKvaAsString}.
*
*
* @return The power draw available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public final PowerDrawKva powerDrawKva() {
return PowerDrawKva.fromValue(powerDrawKva);
}
/**
*
* The power draw available at the hardware placement position for the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerDrawKva} will
* return {@link PowerDrawKva#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerDrawKvaAsString}.
*
*
* @return The power draw available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public final String powerDrawKvaAsString() {
return powerDrawKva;
}
/**
*
* The power option that you can provide for hardware.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerPhase} will
* return {@link PowerPhase#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerPhaseAsString}.
*
*
* @return The power option that you can provide for hardware.
* @see PowerPhase
*/
public final PowerPhase powerPhase() {
return PowerPhase.fromValue(powerPhase);
}
/**
*
* The power option that you can provide for hardware.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerPhase} will
* return {@link PowerPhase#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerPhaseAsString}.
*
*
* @return The power option that you can provide for hardware.
* @see PowerPhase
*/
public final String powerPhaseAsString() {
return powerPhase;
}
/**
*
* The power connector for the hardware.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerConnector}
* will return {@link PowerConnector#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #powerConnectorAsString}.
*
*
* @return The power connector for the hardware.
* @see PowerConnector
*/
public final PowerConnector powerConnector() {
return PowerConnector.fromValue(powerConnector);
}
/**
*
* The power connector for the hardware.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerConnector}
* will return {@link PowerConnector#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #powerConnectorAsString}.
*
*
* @return The power connector for the hardware.
* @see PowerConnector
*/
public final String powerConnectorAsString() {
return powerConnector;
}
/**
*
* The position of the power feed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerFeedDrop}
* will return {@link PowerFeedDrop#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerFeedDropAsString}.
*
*
* @return The position of the power feed.
* @see PowerFeedDrop
*/
public final PowerFeedDrop powerFeedDrop() {
return PowerFeedDrop.fromValue(powerFeedDrop);
}
/**
*
* The position of the power feed.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerFeedDrop}
* will return {@link PowerFeedDrop#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerFeedDropAsString}.
*
*
* @return The position of the power feed.
* @see PowerFeedDrop
*/
public final String powerFeedDropAsString() {
return powerFeedDrop;
}
/**
*
* The uplink speed the rack supports for the connection to the Region.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkGbps} will
* return {@link UplinkGbps#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkGbpsAsString}.
*
*
* @return The uplink speed the rack supports for the connection to the Region.
* @see UplinkGbps
*/
public final UplinkGbps uplinkGbps() {
return UplinkGbps.fromValue(uplinkGbps);
}
/**
*
* The uplink speed the rack supports for the connection to the Region.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkGbps} will
* return {@link UplinkGbps#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkGbpsAsString}.
*
*
* @return The uplink speed the rack supports for the connection to the Region.
* @see UplinkGbps
*/
public final String uplinkGbpsAsString() {
return uplinkGbps;
}
/**
*
* The number of uplinks each Outpost network device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkCount} will
* return {@link UplinkCount#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkCountAsString}.
*
*
* @return The number of uplinks each Outpost network device.
* @see UplinkCount
*/
public final UplinkCount uplinkCount() {
return UplinkCount.fromValue(uplinkCount);
}
/**
*
* The number of uplinks each Outpost network device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkCount} will
* return {@link UplinkCount#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkCountAsString}.
*
*
* @return The number of uplinks each Outpost network device.
* @see UplinkCount
*/
public final String uplinkCountAsString() {
return uplinkCount;
}
/**
*
* The type of fiber used to attach the Outpost to the network.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #fiberOpticCableType} will return {@link FiberOpticCableType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #fiberOpticCableTypeAsString}.
*
*
* @return The type of fiber used to attach the Outpost to the network.
* @see FiberOpticCableType
*/
public final FiberOpticCableType fiberOpticCableType() {
return FiberOpticCableType.fromValue(fiberOpticCableType);
}
/**
*
* The type of fiber used to attach the Outpost to the network.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #fiberOpticCableType} will return {@link FiberOpticCableType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #fiberOpticCableTypeAsString}.
*
*
* @return The type of fiber used to attach the Outpost to the network.
* @see FiberOpticCableType
*/
public final String fiberOpticCableTypeAsString() {
return fiberOpticCableType;
}
/**
*
* The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink speed,
* fiber type, and distance to the upstream device. For more information about networking requirements for racks,
* see Network in the Amazon Web Services Outposts User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #opticalStandard}
* will return {@link OpticalStandard#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #opticalStandardAsString}.
*
*
* @return The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink
* speed, fiber type, and distance to the upstream device. For more information about networking
* requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
* @see OpticalStandard
*/
public final OpticalStandard opticalStandard() {
return OpticalStandard.fromValue(opticalStandard);
}
/**
*
* The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink speed,
* fiber type, and distance to the upstream device. For more information about networking requirements for racks,
* see Network in the Amazon Web Services Outposts User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #opticalStandard}
* will return {@link OpticalStandard#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #opticalStandardAsString}.
*
*
* @return The type of optical standard used to attach the Outpost to the network. This field is dependent on uplink
* speed, fiber type, and distance to the upstream device. For more information about networking
* requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
* @see OpticalStandard
*/
public final String opticalStandardAsString() {
return opticalStandard;
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000 lbs (907 kg).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #maximumSupportedWeightLbs} will return {@link MaximumSupportedWeightLbs#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #maximumSupportedWeightLbsAsString}.
*
*
* @return The maximum rack weight that this site can support. NO_LIMIT
is over 2000 lbs (907 kg).
* @see MaximumSupportedWeightLbs
*/
public final MaximumSupportedWeightLbs maximumSupportedWeightLbs() {
return MaximumSupportedWeightLbs.fromValue(maximumSupportedWeightLbs);
}
/**
*
* The maximum rack weight that this site can support. NO_LIMIT
is over 2000 lbs (907 kg).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #maximumSupportedWeightLbs} will return {@link MaximumSupportedWeightLbs#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #maximumSupportedWeightLbsAsString}.
*
*
* @return The maximum rack weight that this site can support. NO_LIMIT
is over 2000 lbs (907 kg).
* @see MaximumSupportedWeightLbs
*/
public final String maximumSupportedWeightLbsAsString() {
return maximumSupportedWeightLbs;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(powerDrawKvaAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerPhaseAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerConnectorAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerFeedDropAsString());
hashCode = 31 * hashCode + Objects.hashCode(uplinkGbpsAsString());
hashCode = 31 * hashCode + Objects.hashCode(uplinkCountAsString());
hashCode = 31 * hashCode + Objects.hashCode(fiberOpticCableTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(opticalStandardAsString());
hashCode = 31 * hashCode + Objects.hashCode(maximumSupportedWeightLbsAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RackPhysicalProperties)) {
return false;
}
RackPhysicalProperties other = (RackPhysicalProperties) obj;
return Objects.equals(powerDrawKvaAsString(), other.powerDrawKvaAsString())
&& Objects.equals(powerPhaseAsString(), other.powerPhaseAsString())
&& Objects.equals(powerConnectorAsString(), other.powerConnectorAsString())
&& Objects.equals(powerFeedDropAsString(), other.powerFeedDropAsString())
&& Objects.equals(uplinkGbpsAsString(), other.uplinkGbpsAsString())
&& Objects.equals(uplinkCountAsString(), other.uplinkCountAsString())
&& Objects.equals(fiberOpticCableTypeAsString(), other.fiberOpticCableTypeAsString())
&& Objects.equals(opticalStandardAsString(), other.opticalStandardAsString())
&& Objects.equals(maximumSupportedWeightLbsAsString(), other.maximumSupportedWeightLbsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RackPhysicalProperties").add("PowerDrawKva", powerDrawKvaAsString())
.add("PowerPhase", powerPhaseAsString()).add("PowerConnector", powerConnectorAsString())
.add("PowerFeedDrop", powerFeedDropAsString()).add("UplinkGbps", uplinkGbpsAsString())
.add("UplinkCount", uplinkCountAsString()).add("FiberOpticCableType", fiberOpticCableTypeAsString())
.add("OpticalStandard", opticalStandardAsString())
.add("MaximumSupportedWeightLbs", maximumSupportedWeightLbsAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PowerDrawKva":
return Optional.ofNullable(clazz.cast(powerDrawKvaAsString()));
case "PowerPhase":
return Optional.ofNullable(clazz.cast(powerPhaseAsString()));
case "PowerConnector":
return Optional.ofNullable(clazz.cast(powerConnectorAsString()));
case "PowerFeedDrop":
return Optional.ofNullable(clazz.cast(powerFeedDropAsString()));
case "UplinkGbps":
return Optional.ofNullable(clazz.cast(uplinkGbpsAsString()));
case "UplinkCount":
return Optional.ofNullable(clazz.cast(uplinkCountAsString()));
case "FiberOpticCableType":
return Optional.ofNullable(clazz.cast(fiberOpticCableTypeAsString()));
case "OpticalStandard":
return Optional.ofNullable(clazz.cast(opticalStandardAsString()));
case "MaximumSupportedWeightLbs":
return Optional.ofNullable(clazz.cast(maximumSupportedWeightLbsAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function