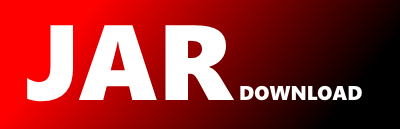
software.amazon.awssdk.services.partnercentralselling.model.AccountSummary Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object that contains an Account
's subset of fields.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AccountSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ADDRESS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Address").getter(getter(AccountSummary::address)).setter(setter(Builder::address))
.constructor(AddressSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Address").build()).build();
private static final SdkField COMPANY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompanyName").getter(getter(AccountSummary::companyName)).setter(setter(Builder::companyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompanyName").build()).build();
private static final SdkField INDUSTRY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Industry").getter(getter(AccountSummary::industryAsString)).setter(setter(Builder::industry))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Industry").build()).build();
private static final SdkField OTHER_INDUSTRY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OtherIndustry").getter(getter(AccountSummary::otherIndustry)).setter(setter(Builder::otherIndustry))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OtherIndustry").build()).build();
private static final SdkField WEBSITE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WebsiteUrl").getter(getter(AccountSummary::websiteUrl)).setter(setter(Builder::websiteUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WebsiteUrl").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ADDRESS_FIELD,
COMPANY_NAME_FIELD, INDUSTRY_FIELD, OTHER_INDUSTRY_FIELD, WEBSITE_URL_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Address", ADDRESS_FIELD);
put("CompanyName", COMPANY_NAME_FIELD);
put("Industry", INDUSTRY_FIELD);
put("OtherIndustry", OTHER_INDUSTRY_FIELD);
put("WebsiteUrl", WEBSITE_URL_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final AddressSummary address;
private final String companyName;
private final String industry;
private final String otherIndustry;
private final String websiteUrl;
private AccountSummary(BuilderImpl builder) {
this.address = builder.address;
this.companyName = builder.companyName;
this.industry = builder.industry;
this.otherIndustry = builder.otherIndustry;
this.websiteUrl = builder.websiteUrl;
}
/**
*
* Specifies the end Customer
's address details associated with the Opportunity
.
*
*
* @return Specifies the end Customer
's address details associated with the Opportunity
.
*/
public final AddressSummary address() {
return address;
}
/**
*
* Specifies the end Customer
's company name associated with the Opportunity
.
*
*
* @return Specifies the end Customer
's company name associated with the Opportunity
.
*/
public final String companyName() {
return companyName;
}
/**
*
* Specifies which industry the end Customer
belongs to associated with the Opportunity
.
* It refers to the category or sector that the customer's business operates in.
*
*
* To submit a value outside the picklist, use Other
.
*
*
* Conditionally mandatory if Other
is selected for Industry Vertical in LOVs.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #industry} will
* return {@link Industry#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #industryAsString}.
*
*
* @return Specifies which industry the end Customer
belongs to associated with the
* Opportunity
. It refers to the category or sector that the customer's business operates in.
*
*
* To submit a value outside the picklist, use Other
.
*
*
* Conditionally mandatory if Other
is selected for Industry Vertical in LOVs.
* @see Industry
*/
public final Industry industry() {
return Industry.fromValue(industry);
}
/**
*
* Specifies which industry the end Customer
belongs to associated with the Opportunity
.
* It refers to the category or sector that the customer's business operates in.
*
*
* To submit a value outside the picklist, use Other
.
*
*
* Conditionally mandatory if Other
is selected for Industry Vertical in LOVs.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #industry} will
* return {@link Industry#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #industryAsString}.
*
*
* @return Specifies which industry the end Customer
belongs to associated with the
* Opportunity
. It refers to the category or sector that the customer's business operates in.
*
*
* To submit a value outside the picklist, use Other
.
*
*
* Conditionally mandatory if Other
is selected for Industry Vertical in LOVs.
* @see Industry
*/
public final String industryAsString() {
return industry;
}
/**
*
* Specifies the end Customer
's industry associated with the Opportunity
, when the
* selected value in the Industry
field is Other
. This field is relevant when the
* customer's industry doesn't fall under the predefined picklist values and requires a custom description.
*
*
* @return Specifies the end Customer
's industry associated with the Opportunity
, when
* the selected value in the Industry
field is Other
. This field is relevant when
* the customer's industry doesn't fall under the predefined picklist values and requires a custom
* description.
*/
public final String otherIndustry() {
return otherIndustry;
}
/**
*
* Specifies the end customer's company website URL associated with the Opportunity
. This value is
* crucial to map the customer within the Amazon Web Services CRM system.
*
*
* @return Specifies the end customer's company website URL associated with the Opportunity
. This value
* is crucial to map the customer within the Amazon Web Services CRM system.
*/
public final String websiteUrl() {
return websiteUrl;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(address());
hashCode = 31 * hashCode + Objects.hashCode(companyName());
hashCode = 31 * hashCode + Objects.hashCode(industryAsString());
hashCode = 31 * hashCode + Objects.hashCode(otherIndustry());
hashCode = 31 * hashCode + Objects.hashCode(websiteUrl());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccountSummary)) {
return false;
}
AccountSummary other = (AccountSummary) obj;
return Objects.equals(address(), other.address()) && Objects.equals(companyName(), other.companyName())
&& Objects.equals(industryAsString(), other.industryAsString())
&& Objects.equals(otherIndustry(), other.otherIndustry()) && Objects.equals(websiteUrl(), other.websiteUrl());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AccountSummary").add("Address", address())
.add("CompanyName", companyName() == null ? null : "*** Sensitive Data Redacted ***")
.add("Industry", industryAsString()).add("OtherIndustry", otherIndustry())
.add("WebsiteUrl", websiteUrl() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Address":
return Optional.ofNullable(clazz.cast(address()));
case "CompanyName":
return Optional.ofNullable(clazz.cast(companyName()));
case "Industry":
return Optional.ofNullable(clazz.cast(industryAsString()));
case "OtherIndustry":
return Optional.ofNullable(clazz.cast(otherIndustry()));
case "WebsiteUrl":
return Optional.ofNullable(clazz.cast(websiteUrl()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function