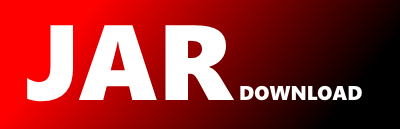
software.amazon.awssdk.services.partnercentralselling.model.ProjectDetails Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains details about the project associated with the Engagement Invitation, including the business problem and
* expected outcomes.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProjectDetails implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BUSINESS_PROBLEM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BusinessProblem").getter(getter(ProjectDetails::businessProblem))
.setter(setter(Builder::businessProblem))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BusinessProblem").build()).build();
private static final SdkField> EXPECTED_CUSTOMER_SPEND_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExpectedCustomerSpend")
.getter(getter(ProjectDetails::expectedCustomerSpend))
.setter(setter(Builder::expectedCustomerSpend))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedCustomerSpend").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExpectedCustomerSpend::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TARGET_COMPLETION_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetCompletionDate").getter(getter(ProjectDetails::targetCompletionDate))
.setter(setter(Builder::targetCompletionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetCompletionDate").build())
.build();
private static final SdkField TITLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Title")
.getter(getter(ProjectDetails::title)).setter(setter(Builder::title))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Title").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUSINESS_PROBLEM_FIELD,
EXPECTED_CUSTOMER_SPEND_FIELD, TARGET_COMPLETION_DATE_FIELD, TITLE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("BusinessProblem", BUSINESS_PROBLEM_FIELD);
put("ExpectedCustomerSpend", EXPECTED_CUSTOMER_SPEND_FIELD);
put("TargetCompletionDate", TARGET_COMPLETION_DATE_FIELD);
put("Title", TITLE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String businessProblem;
private final List expectedCustomerSpend;
private final String targetCompletionDate;
private final String title;
private ProjectDetails(BuilderImpl builder) {
this.businessProblem = builder.businessProblem;
this.expectedCustomerSpend = builder.expectedCustomerSpend;
this.targetCompletionDate = builder.targetCompletionDate;
this.title = builder.title;
}
/**
*
* Describes the business problem that the project aims to solve. This information is crucial for understanding the
* project’s goals and objectives.
*
*
* @return Describes the business problem that the project aims to solve. This information is crucial for
* understanding the project’s goals and objectives.
*/
public final String businessProblem() {
return businessProblem;
}
/**
* For responses, this returns true if the service returned a value for the ExpectedCustomerSpend property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExpectedCustomerSpend() {
return expectedCustomerSpend != null && !(expectedCustomerSpend instanceof SdkAutoConstructList);
}
/**
*
* Contains revenue estimates for the partner related to the project. This field provides an idea of the financial
* potential of the opportunity for the partner.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExpectedCustomerSpend} method.
*
*
* @return Contains revenue estimates for the partner related to the project. This field provides an idea of the
* financial potential of the opportunity for the partner.
*/
public final List expectedCustomerSpend() {
return expectedCustomerSpend;
}
/**
*
* Specifies the estimated date of project completion. This field helps track the project timeline and manage
* expectations.
*
*
* @return Specifies the estimated date of project completion. This field helps track the project timeline and
* manage expectations.
*/
public final String targetCompletionDate() {
return targetCompletionDate;
}
/**
*
* Specifies the title of the project. This title helps partners quickly identify and understand the focus of the
* project.
*
*
* @return Specifies the title of the project. This title helps partners quickly identify and understand the focus
* of the project.
*/
public final String title() {
return title;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(businessProblem());
hashCode = 31 * hashCode + Objects.hashCode(hasExpectedCustomerSpend() ? expectedCustomerSpend() : null);
hashCode = 31 * hashCode + Objects.hashCode(targetCompletionDate());
hashCode = 31 * hashCode + Objects.hashCode(title());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProjectDetails)) {
return false;
}
ProjectDetails other = (ProjectDetails) obj;
return Objects.equals(businessProblem(), other.businessProblem())
&& hasExpectedCustomerSpend() == other.hasExpectedCustomerSpend()
&& Objects.equals(expectedCustomerSpend(), other.expectedCustomerSpend())
&& Objects.equals(targetCompletionDate(), other.targetCompletionDate()) && Objects.equals(title(), other.title());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProjectDetails")
.add("BusinessProblem", businessProblem() == null ? null : "*** Sensitive Data Redacted ***")
.add("ExpectedCustomerSpend", hasExpectedCustomerSpend() ? expectedCustomerSpend() : null)
.add("TargetCompletionDate", targetCompletionDate()).add("Title", title()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BusinessProblem":
return Optional.ofNullable(clazz.cast(businessProblem()));
case "ExpectedCustomerSpend":
return Optional.ofNullable(clazz.cast(expectedCustomerSpend()));
case "TargetCompletionDate":
return Optional.ofNullable(clazz.cast(targetCompletionDate()));
case "Title":
return Optional.ofNullable(clazz.cast(title()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function