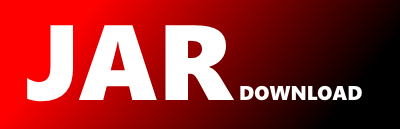
software.amazon.awssdk.services.partnercentralselling.model.StartEngagementByAcceptingInvitationTaskResponse Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartEngagementByAcceptingInvitationTaskResponse extends PartnerCentralSellingResponse
implements
ToCopyableBuilder {
private static final SdkField ENGAGEMENT_INVITATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngagementInvitationId")
.getter(getter(StartEngagementByAcceptingInvitationTaskResponse::engagementInvitationId))
.setter(setter(Builder::engagementInvitationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngagementInvitationId").build())
.build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Message")
.getter(getter(StartEngagementByAcceptingInvitationTaskResponse::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField OPPORTUNITY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OpportunityId").getter(getter(StartEngagementByAcceptingInvitationTaskResponse::opportunityId))
.setter(setter(Builder::opportunityId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OpportunityId").build()).build();
private static final SdkField REASON_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReasonCode").getter(getter(StartEngagementByAcceptingInvitationTaskResponse::reasonCodeAsString))
.setter(setter(Builder::reasonCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReasonCode").build()).build();
private static final SdkField START_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StartTime")
.getter(getter(StartEngagementByAcceptingInvitationTaskResponse::startTime))
.setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField TASK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskArn").getter(getter(StartEngagementByAcceptingInvitationTaskResponse::taskArn))
.setter(setter(Builder::taskArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskArn").build()).build();
private static final SdkField TASK_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("TaskId")
.getter(getter(StartEngagementByAcceptingInvitationTaskResponse::taskId)).setter(setter(Builder::taskId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskId").build()).build();
private static final SdkField TASK_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskStatus").getter(getter(StartEngagementByAcceptingInvitationTaskResponse::taskStatusAsString))
.setter(setter(Builder::taskStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
ENGAGEMENT_INVITATION_ID_FIELD, MESSAGE_FIELD, OPPORTUNITY_ID_FIELD, REASON_CODE_FIELD, START_TIME_FIELD,
TASK_ARN_FIELD, TASK_ID_FIELD, TASK_STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("EngagementInvitationId", ENGAGEMENT_INVITATION_ID_FIELD);
put("Message", MESSAGE_FIELD);
put("OpportunityId", OPPORTUNITY_ID_FIELD);
put("ReasonCode", REASON_CODE_FIELD);
put("StartTime", START_TIME_FIELD);
put("TaskArn", TASK_ARN_FIELD);
put("TaskId", TASK_ID_FIELD);
put("TaskStatus", TASK_STATUS_FIELD);
}
});
private final String engagementInvitationId;
private final String message;
private final String opportunityId;
private final String reasonCode;
private final Instant startTime;
private final String taskArn;
private final String taskId;
private final String taskStatus;
private StartEngagementByAcceptingInvitationTaskResponse(BuilderImpl builder) {
super(builder);
this.engagementInvitationId = builder.engagementInvitationId;
this.message = builder.message;
this.opportunityId = builder.opportunityId;
this.reasonCode = builder.reasonCode;
this.startTime = builder.startTime;
this.taskArn = builder.taskArn;
this.taskId = builder.taskId;
this.taskStatus = builder.taskStatus;
}
/**
*
* Returns the identifier of the engagement invitation that was accepted and used to create the opportunity.
*
*
* @return Returns the identifier of the engagement invitation that was accepted and used to create the opportunity.
*/
public final String engagementInvitationId() {
return engagementInvitationId;
}
/**
*
* If the task fails, this field contains a detailed message describing the failure and possible recovery steps.
*
*
* @return If the task fails, this field contains a detailed message describing the failure and possible recovery
* steps.
*/
public final String message() {
return message;
}
/**
*
* Returns the original opportunity identifier passed in the request. This is the unique identifier for the
* opportunity.
*
*
* @return Returns the original opportunity identifier passed in the request. This is the unique identifier for the
* opportunity.
*/
public final String opportunityId() {
return opportunityId;
}
/**
*
* Indicates the reason for task failure using an enumerated code.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reasonCode} will
* return {@link ReasonCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reasonCodeAsString}.
*
*
* @return Indicates the reason for task failure using an enumerated code.
* @see ReasonCode
*/
public final ReasonCode reasonCode() {
return ReasonCode.fromValue(reasonCode);
}
/**
*
* Indicates the reason for task failure using an enumerated code.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reasonCode} will
* return {@link ReasonCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reasonCodeAsString}.
*
*
* @return Indicates the reason for task failure using an enumerated code.
* @see ReasonCode
*/
public final String reasonCodeAsString() {
return reasonCode;
}
/**
*
* The timestamp indicating when the task was initiated. The format follows RFC 3339 section 5.6.
*
*
* @return The timestamp indicating when the task was initiated. The format follows RFC 3339 section 5.6.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The Amazon Resource Name (ARN) of the task, used for tracking and managing the task within AWS.
*
*
* @return The Amazon Resource Name (ARN) of the task, used for tracking and managing the task within AWS.
*/
public final String taskArn() {
return taskArn;
}
/**
*
* The unique identifier of the task, used to track the task’s progress.
*
*
* @return The unique identifier of the task, used to track the task’s progress.
*/
public final String taskId() {
return taskId;
}
/**
*
* Indicates the current status of the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #taskStatus} will
* return {@link TaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #taskStatusAsString}.
*
*
* @return Indicates the current status of the task.
* @see TaskStatus
*/
public final TaskStatus taskStatus() {
return TaskStatus.fromValue(taskStatus);
}
/**
*
* Indicates the current status of the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #taskStatus} will
* return {@link TaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #taskStatusAsString}.
*
*
* @return Indicates the current status of the task.
* @see TaskStatus
*/
public final String taskStatusAsString() {
return taskStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(engagementInvitationId());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(opportunityId());
hashCode = 31 * hashCode + Objects.hashCode(reasonCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(taskArn());
hashCode = 31 * hashCode + Objects.hashCode(taskId());
hashCode = 31 * hashCode + Objects.hashCode(taskStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartEngagementByAcceptingInvitationTaskResponse)) {
return false;
}
StartEngagementByAcceptingInvitationTaskResponse other = (StartEngagementByAcceptingInvitationTaskResponse) obj;
return Objects.equals(engagementInvitationId(), other.engagementInvitationId())
&& Objects.equals(message(), other.message()) && Objects.equals(opportunityId(), other.opportunityId())
&& Objects.equals(reasonCodeAsString(), other.reasonCodeAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(taskArn(), other.taskArn())
&& Objects.equals(taskId(), other.taskId()) && Objects.equals(taskStatusAsString(), other.taskStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartEngagementByAcceptingInvitationTaskResponse")
.add("EngagementInvitationId", engagementInvitationId()).add("Message", message())
.add("OpportunityId", opportunityId()).add("ReasonCode", reasonCodeAsString()).add("StartTime", startTime())
.add("TaskArn", taskArn()).add("TaskId", taskId()).add("TaskStatus", taskStatusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EngagementInvitationId":
return Optional.ofNullable(clazz.cast(engagementInvitationId()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "OpportunityId":
return Optional.ofNullable(clazz.cast(opportunityId()));
case "ReasonCode":
return Optional.ofNullable(clazz.cast(reasonCodeAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "TaskArn":
return Optional.ofNullable(clazz.cast(taskArn()));
case "TaskId":
return Optional.ofNullable(clazz.cast(taskId()));
case "TaskStatus":
return Optional.ofNullable(clazz.cast(taskStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function