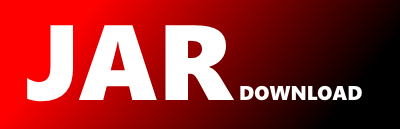
software.amazon.awssdk.services.partnercentralselling.model.AssigneeContact Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the contact details of the individual assigned to manage the opportunity within the partner organization.
* This helps to ensure that there is a point of contact for the opportunity's progress.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AssigneeContact implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BUSINESS_TITLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BusinessTitle").getter(getter(AssigneeContact::businessTitle)).setter(setter(Builder::businessTitle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BusinessTitle").build()).build();
private static final SdkField EMAIL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Email")
.getter(getter(AssigneeContact::email)).setter(setter(Builder::email))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Email").build()).build();
private static final SdkField FIRST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FirstName").getter(getter(AssigneeContact::firstName)).setter(setter(Builder::firstName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirstName").build()).build();
private static final SdkField LAST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastName").getter(getter(AssigneeContact::lastName)).setter(setter(Builder::lastName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUSINESS_TITLE_FIELD,
EMAIL_FIELD, FIRST_NAME_FIELD, LAST_NAME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String businessTitle;
private final String email;
private final String firstName;
private final String lastName;
private AssigneeContact(BuilderImpl builder) {
this.businessTitle = builder.businessTitle;
this.email = builder.email;
this.firstName = builder.firstName;
this.lastName = builder.lastName;
}
/**
*
* Specifies the business title of the assignee managing the opportunity. This helps clarify the individual's role
* and responsibilities within the organization. Use the value PartnerAccountManager
to update details
* of the opportunity owner.
*
*
* @return Specifies the business title of the assignee managing the opportunity. This helps clarify the
* individual's role and responsibilities within the organization. Use the value
* PartnerAccountManager
to update details of the opportunity owner.
*/
public final String businessTitle() {
return businessTitle;
}
/**
*
* Provides the email address of the assignee. This email is used for communications and notifications related to
* the opportunity.
*
*
* @return Provides the email address of the assignee. This email is used for communications and notifications
* related to the opportunity.
*/
public final String email() {
return email;
}
/**
*
* Specifies the first name of the assignee managing the opportunity. The system automatically retrieves this value
* from the user profile by referencing the associated email address.
*
*
* @return Specifies the first name of the assignee managing the opportunity. The system automatically retrieves
* this value from the user profile by referencing the associated email address.
*/
public final String firstName() {
return firstName;
}
/**
*
* Specifies the last name of the assignee managing the opportunity. The system automatically retrieves this value
* from the user profile by referencing the associated email address.
*
*
* @return Specifies the last name of the assignee managing the opportunity. The system automatically retrieves this
* value from the user profile by referencing the associated email address.
*/
public final String lastName() {
return lastName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(businessTitle());
hashCode = 31 * hashCode + Objects.hashCode(email());
hashCode = 31 * hashCode + Objects.hashCode(firstName());
hashCode = 31 * hashCode + Objects.hashCode(lastName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AssigneeContact)) {
return false;
}
AssigneeContact other = (AssigneeContact) obj;
return Objects.equals(businessTitle(), other.businessTitle()) && Objects.equals(email(), other.email())
&& Objects.equals(firstName(), other.firstName()) && Objects.equals(lastName(), other.lastName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AssigneeContact")
.add("BusinessTitle", businessTitle() == null ? null : "*** Sensitive Data Redacted ***")
.add("Email", email() == null ? null : "*** Sensitive Data Redacted ***")
.add("FirstName", firstName() == null ? null : "*** Sensitive Data Redacted ***")
.add("LastName", lastName() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BusinessTitle":
return Optional.ofNullable(clazz.cast(businessTitle()));
case "Email":
return Optional.ofNullable(clazz.cast(email()));
case "FirstName":
return Optional.ofNullable(clazz.cast(firstName()));
case "LastName":
return Optional.ofNullable(clazz.cast(lastName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("BusinessTitle", BUSINESS_TITLE_FIELD);
map.put("Email", EMAIL_FIELD);
map.put("FirstName", FIRST_NAME_FIELD);
map.put("LastName", LAST_NAME_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function