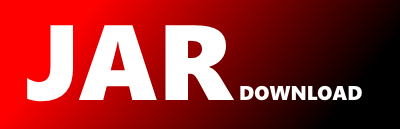
software.amazon.awssdk.services.partnercentralselling.model.GetResourceSnapshotJobResponse Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetResourceSnapshotJobResponse extends PartnerCentralSellingResponse implements
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(GetResourceSnapshotJobResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField CATALOG_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Catalog")
.getter(getter(GetResourceSnapshotJobResponse::catalog)).setter(setter(Builder::catalog))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Catalog").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CreatedAt")
.getter(getter(GetResourceSnapshotJobResponse::createdAt))
.setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField ENGAGEMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngagementId").getter(getter(GetResourceSnapshotJobResponse::engagementId))
.setter(setter(Builder::engagementId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngagementId").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(GetResourceSnapshotJobResponse::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField LAST_FAILURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastFailure").getter(getter(GetResourceSnapshotJobResponse::lastFailure))
.setter(setter(Builder::lastFailure))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastFailure").build()).build();
private static final SdkField LAST_SUCCESSFUL_EXECUTION_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("LastSuccessfulExecutionDate")
.getter(getter(GetResourceSnapshotJobResponse::lastSuccessfulExecutionDate))
.setter(setter(Builder::lastSuccessfulExecutionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastSuccessfulExecutionDate")
.build(), TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceArn").getter(getter(GetResourceSnapshotJobResponse::resourceArn))
.setter(setter(Builder::resourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceArn").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceId").getter(getter(GetResourceSnapshotJobResponse::resourceId))
.setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceId").build()).build();
private static final SdkField RESOURCE_SNAPSHOT_TEMPLATE_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ResourceSnapshotTemplateName")
.getter(getter(GetResourceSnapshotJobResponse::resourceSnapshotTemplateName))
.setter(setter(Builder::resourceSnapshotTemplateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceSnapshotTemplateName")
.build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceType").getter(getter(GetResourceSnapshotJobResponse::resourceTypeAsString))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(GetResourceSnapshotJobResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, CATALOG_FIELD,
CREATED_AT_FIELD, ENGAGEMENT_ID_FIELD, ID_FIELD, LAST_FAILURE_FIELD, LAST_SUCCESSFUL_EXECUTION_DATE_FIELD,
RESOURCE_ARN_FIELD, RESOURCE_ID_FIELD, RESOURCE_SNAPSHOT_TEMPLATE_NAME_FIELD, RESOURCE_TYPE_FIELD, STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String arn;
private final String catalog;
private final Instant createdAt;
private final String engagementId;
private final String id;
private final String lastFailure;
private final Instant lastSuccessfulExecutionDate;
private final String resourceArn;
private final String resourceId;
private final String resourceSnapshotTemplateName;
private final String resourceType;
private final String status;
private GetResourceSnapshotJobResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.catalog = builder.catalog;
this.createdAt = builder.createdAt;
this.engagementId = builder.engagementId;
this.id = builder.id;
this.lastFailure = builder.lastFailure;
this.lastSuccessfulExecutionDate = builder.lastSuccessfulExecutionDate;
this.resourceArn = builder.resourceArn;
this.resourceId = builder.resourceId;
this.resourceSnapshotTemplateName = builder.resourceSnapshotTemplateName;
this.resourceType = builder.resourceType;
this.status = builder.status;
}
/**
*
* he Amazon Resource Name (ARN) of the snapshot job. This globally unique identifier can be used for
* resource-specific operations across AWS services.
*
*
* @return he Amazon Resource Name (ARN) of the snapshot job. This globally unique identifier can be used for
* resource-specific operations across AWS services.
*/
public final String arn() {
return arn;
}
/**
*
* The catalog in which the snapshot job was created. This will match the catalog specified in the request.
*
*
* @return The catalog in which the snapshot job was created. This will match the catalog specified in the request.
*/
public final String catalog() {
return catalog;
}
/**
*
* The date and time when the snapshot job was created, in ISO 8601 format (UTC). Example: "2023-05-01T20:37:46Z"
*
*
* @return The date and time when the snapshot job was created, in ISO 8601 format (UTC). Example:
* "2023-05-01T20:37:46Z"
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The identifier of the engagement associated with this snapshot job. This links the job to a specific engagement
* context.
*
*
* @return The identifier of the engagement associated with this snapshot job. This links the job to a specific
* engagement context.
*/
public final String engagementId() {
return engagementId;
}
/**
*
* The unique identifier of the snapshot job. This matches the ResourceSnapshotJobIdentifier
provided
* in the request.
*
*
* @return The unique identifier of the snapshot job. This matches the ResourceSnapshotJobIdentifier
* provided in the request.
*/
public final String id() {
return id;
}
/**
*
* If the job has encountered any failures, this field contains the error message from the most recent failure. This
* can be useful for troubleshooting issues with the job.
*
*
* @return If the job has encountered any failures, this field contains the error message from the most recent
* failure. This can be useful for troubleshooting issues with the job.
*/
public final String lastFailure() {
return lastFailure;
}
/**
*
* The date and time of the last successful execution of the job, in ISO 8601 format (UTC). Example:
* "2023-05-01T20:37:46Z"
*
*
* @return The date and time of the last successful execution of the job, in ISO 8601 format (UTC). Example:
* "2023-05-01T20:37:46Z"
*/
public final Instant lastSuccessfulExecutionDate() {
return lastSuccessfulExecutionDate;
}
/**
*
* The Amazon Resource Name (ARN) of the resource being snapshotted. This provides a globally unique identifier for
* the resource across AWS.
*
*
* @return The Amazon Resource Name (ARN) of the resource being snapshotted. This provides a globally unique
* identifier for the resource across AWS.
*/
public final String resourceArn() {
return resourceArn;
}
/**
*
* The identifier of the specific resource being snapshotted. The format may vary depending on the
* ResourceType
.
*
*
* @return The identifier of the specific resource being snapshotted. The format may vary depending on the
* ResourceType
.
*/
public final String resourceId() {
return resourceId;
}
/**
*
* The name of the template used for creating the snapshot. This is the same as the template name. It defines the
* structure and content of the snapshot.
*
*
* @return The name of the template used for creating the snapshot. This is the same as the template name. It
* defines the structure and content of the snapshot.
*/
public final String resourceSnapshotTemplateName() {
return resourceSnapshotTemplateName;
}
/**
*
* The type of resource being snapshotted. This would have Opportunity
as a value as it is dependent on
* the supported resource type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of resource being snapshotted. This would have Opportunity
as a value as it is
* dependent on the supported resource type.
* @see ResourceType
*/
public final ResourceType resourceType() {
return ResourceType.fromValue(resourceType);
}
/**
*
* The type of resource being snapshotted. This would have Opportunity
as a value as it is dependent on
* the supported resource type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of resource being snapshotted. This would have Opportunity
as a value as it is
* dependent on the supported resource type.
* @see ResourceType
*/
public final String resourceTypeAsString() {
return resourceType;
}
/**
*
* The current status of the snapshot job. Valid values:
*
*
* -
*
* STOPPED: The job is not currently running.
*
*
* -
*
* RUNNING: The job is actively executing.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ResourceSnapshotJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The current status of the snapshot job. Valid values:
*
* -
*
* STOPPED: The job is not currently running.
*
*
* -
*
* RUNNING: The job is actively executing.
*
*
* @see ResourceSnapshotJobStatus
*/
public final ResourceSnapshotJobStatus status() {
return ResourceSnapshotJobStatus.fromValue(status);
}
/**
*
* The current status of the snapshot job. Valid values:
*
*
* -
*
* STOPPED: The job is not currently running.
*
*
* -
*
* RUNNING: The job is actively executing.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ResourceSnapshotJobStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The current status of the snapshot job. Valid values:
*
* -
*
* STOPPED: The job is not currently running.
*
*
* -
*
* RUNNING: The job is actively executing.
*
*
* @see ResourceSnapshotJobStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(catalog());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(engagementId());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(lastFailure());
hashCode = 31 * hashCode + Objects.hashCode(lastSuccessfulExecutionDate());
hashCode = 31 * hashCode + Objects.hashCode(resourceArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(resourceSnapshotTemplateName());
hashCode = 31 * hashCode + Objects.hashCode(resourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetResourceSnapshotJobResponse)) {
return false;
}
GetResourceSnapshotJobResponse other = (GetResourceSnapshotJobResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(catalog(), other.catalog())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(engagementId(), other.engagementId())
&& Objects.equals(id(), other.id()) && Objects.equals(lastFailure(), other.lastFailure())
&& Objects.equals(lastSuccessfulExecutionDate(), other.lastSuccessfulExecutionDate())
&& Objects.equals(resourceArn(), other.resourceArn()) && Objects.equals(resourceId(), other.resourceId())
&& Objects.equals(resourceSnapshotTemplateName(), other.resourceSnapshotTemplateName())
&& Objects.equals(resourceTypeAsString(), other.resourceTypeAsString())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetResourceSnapshotJobResponse").add("Arn", arn()).add("Catalog", catalog())
.add("CreatedAt", createdAt()).add("EngagementId", engagementId()).add("Id", id())
.add("LastFailure", lastFailure()).add("LastSuccessfulExecutionDate", lastSuccessfulExecutionDate())
.add("ResourceArn", resourceArn()).add("ResourceId", resourceId())
.add("ResourceSnapshotTemplateName", resourceSnapshotTemplateName()).add("ResourceType", resourceTypeAsString())
.add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Catalog":
return Optional.ofNullable(clazz.cast(catalog()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "EngagementId":
return Optional.ofNullable(clazz.cast(engagementId()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "LastFailure":
return Optional.ofNullable(clazz.cast(lastFailure()));
case "LastSuccessfulExecutionDate":
return Optional.ofNullable(clazz.cast(lastSuccessfulExecutionDate()));
case "ResourceArn":
return Optional.ofNullable(clazz.cast(resourceArn()));
case "ResourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "ResourceSnapshotTemplateName":
return Optional.ofNullable(clazz.cast(resourceSnapshotTemplateName()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceTypeAsString()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Arn", ARN_FIELD);
map.put("Catalog", CATALOG_FIELD);
map.put("CreatedAt", CREATED_AT_FIELD);
map.put("EngagementId", ENGAGEMENT_ID_FIELD);
map.put("Id", ID_FIELD);
map.put("LastFailure", LAST_FAILURE_FIELD);
map.put("LastSuccessfulExecutionDate", LAST_SUCCESSFUL_EXECUTION_DATE_FIELD);
map.put("ResourceArn", RESOURCE_ARN_FIELD);
map.put("ResourceId", RESOURCE_ID_FIELD);
map.put("ResourceSnapshotTemplateName", RESOURCE_SNAPSHOT_TEMPLATE_NAME_FIELD);
map.put("ResourceType", RESOURCE_TYPE_FIELD);
map.put("Status", STATUS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function