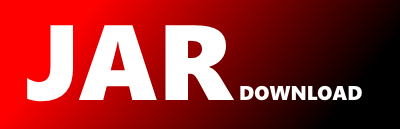
software.amazon.awssdk.services.partnercentralselling.model.LifeCycleSummary Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object that contains a LifeCycle
object's subset of fields.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LifeCycleSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CLOSED_LOST_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClosedLostReason").getter(getter(LifeCycleSummary::closedLostReasonAsString))
.setter(setter(Builder::closedLostReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClosedLostReason").build()).build();
private static final SdkField NEXT_STEPS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextSteps").getter(getter(LifeCycleSummary::nextSteps)).setter(setter(Builder::nextSteps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextSteps").build()).build();
private static final SdkField REVIEW_COMMENTS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReviewComments").getter(getter(LifeCycleSummary::reviewComments))
.setter(setter(Builder::reviewComments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReviewComments").build()).build();
private static final SdkField REVIEW_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReviewStatus").getter(getter(LifeCycleSummary::reviewStatusAsString))
.setter(setter(Builder::reviewStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReviewStatus").build()).build();
private static final SdkField REVIEW_STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReviewStatusReason").getter(getter(LifeCycleSummary::reviewStatusReason))
.setter(setter(Builder::reviewStatusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReviewStatusReason").build())
.build();
private static final SdkField STAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Stage")
.getter(getter(LifeCycleSummary::stageAsString)).setter(setter(Builder::stage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Stage").build()).build();
private static final SdkField TARGET_CLOSE_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetCloseDate").getter(getter(LifeCycleSummary::targetCloseDate))
.setter(setter(Builder::targetCloseDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetCloseDate").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLOSED_LOST_REASON_FIELD,
NEXT_STEPS_FIELD, REVIEW_COMMENTS_FIELD, REVIEW_STATUS_FIELD, REVIEW_STATUS_REASON_FIELD, STAGE_FIELD,
TARGET_CLOSE_DATE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String closedLostReason;
private final String nextSteps;
private final String reviewComments;
private final String reviewStatus;
private final String reviewStatusReason;
private final String stage;
private final String targetCloseDate;
private LifeCycleSummary(BuilderImpl builder) {
this.closedLostReason = builder.closedLostReason;
this.nextSteps = builder.nextSteps;
this.reviewComments = builder.reviewComments;
this.reviewStatus = builder.reviewStatus;
this.reviewStatusReason = builder.reviewStatusReason;
this.stage = builder.stage;
this.targetCloseDate = builder.targetCloseDate;
}
/**
*
* Specifies the reason code when an opportunity is marked as Closed Lost. When you select an appropriate
* reason code, you communicate the context for closing the Opportunity
, and aid in accurate reports
* and analysis of opportunity outcomes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #closedLostReason}
* will return {@link ClosedLostReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #closedLostReasonAsString}.
*
*
* @return Specifies the reason code when an opportunity is marked as Closed Lost. When you select an
* appropriate reason code, you communicate the context for closing the Opportunity
, and aid in
* accurate reports and analysis of opportunity outcomes.
* @see ClosedLostReason
*/
public final ClosedLostReason closedLostReason() {
return ClosedLostReason.fromValue(closedLostReason);
}
/**
*
* Specifies the reason code when an opportunity is marked as Closed Lost. When you select an appropriate
* reason code, you communicate the context for closing the Opportunity
, and aid in accurate reports
* and analysis of opportunity outcomes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #closedLostReason}
* will return {@link ClosedLostReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #closedLostReasonAsString}.
*
*
* @return Specifies the reason code when an opportunity is marked as Closed Lost. When you select an
* appropriate reason code, you communicate the context for closing the Opportunity
, and aid in
* accurate reports and analysis of opportunity outcomes.
* @see ClosedLostReason
*/
public final String closedLostReasonAsString() {
return closedLostReason;
}
/**
*
* Specifies the upcoming actions or tasks for the Opportunity
. This field is utilized to communicate
* to Amazon Web Services the next actions required for the Opportunity
.
*
*
* @return Specifies the upcoming actions or tasks for the Opportunity
. This field is utilized to
* communicate to Amazon Web Services the next actions required for the Opportunity
.
*/
public final String nextSteps() {
return nextSteps;
}
/**
*
* Indicates why an opportunity was sent back for further details. Partners must take corrective action based on the
* ReviewComments
.
*
*
* @return Indicates why an opportunity was sent back for further details. Partners must take corrective action
* based on the ReviewComments
.
*/
public final String reviewComments() {
return reviewComments;
}
/**
*
* Indicates the review status of a partner referred opportunity. This field is read-only and only applicable for
* partner referrals. Valid values:
*
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the UpdateOpportunity
API
* action to update the opportunity, and ensure you make all required changes. Only these fields are editable when
* the Lifecycle.ReviewStatus
is Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues are
* resolved, and the opportunity's Lifecycle.ReviewStatus
is set to Approved
or
* Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reviewStatus} will
* return {@link ReviewStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reviewStatusAsString}.
*
*
* @return Indicates the review status of a partner referred opportunity. This field is read-only and only
* applicable for partner referrals. Valid values:
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the
* UpdateOpportunity
API action to update the opportunity, and ensure you make all required
* changes. Only these fields are editable when the Lifecycle.ReviewStatus
is
* Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues are
* resolved, and the opportunity's Lifecycle.ReviewStatus
is set to Approved
or
* Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
* @see ReviewStatus
*/
public final ReviewStatus reviewStatus() {
return ReviewStatus.fromValue(reviewStatus);
}
/**
*
* Indicates the review status of a partner referred opportunity. This field is read-only and only applicable for
* partner referrals. Valid values:
*
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the UpdateOpportunity
API
* action to update the opportunity, and ensure you make all required changes. Only these fields are editable when
* the Lifecycle.ReviewStatus
is Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues are
* resolved, and the opportunity's Lifecycle.ReviewStatus
is set to Approved
or
* Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #reviewStatus} will
* return {@link ReviewStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #reviewStatusAsString}.
*
*
* @return Indicates the review status of a partner referred opportunity. This field is read-only and only
* applicable for partner referrals. Valid values:
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the
* UpdateOpportunity
API action to update the opportunity, and ensure you make all required
* changes. Only these fields are editable when the Lifecycle.ReviewStatus
is
* Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues are
* resolved, and the opportunity's Lifecycle.ReviewStatus
is set to Approved
or
* Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
* @see ReviewStatus
*/
public final String reviewStatusAsString() {
return reviewStatus;
}
/**
*
* Indicates the reason a specific decision was taken during the opportunity review process. This field combines the
* reasons for both disqualified and action required statuses, and provides clarity for why an opportunity was
* disqualified or required further action.
*
*
* @return Indicates the reason a specific decision was taken during the opportunity review process. This field
* combines the reasons for both disqualified and action required statuses, and provides clarity for why an
* opportunity was disqualified or required further action.
*/
public final String reviewStatusReason() {
return reviewStatusReason;
}
/**
*
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services stages
* from the current stage in the partner CRM. This field provides a translated value of the stage, and offers
* insight into the Opportunity
's progression in the sales cycle, according to Amazon Web Services
* definitions.
*
*
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end customer
* through a lead) or latent (Your account team believes it exists based on research, account plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements. The
* customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stage} will return
* {@link Stage#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stageAsString}.
*
*
* @return Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services
* stages from the current stage in the partner CRM. This field provides a translated value of the stage,
* and offers insight into the Opportunity
's progression in the sales cycle, according to
* Amazon Web Services definitions.
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end
* customer through a lead) or latent (Your account team believes it exists based on research, account
* plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements.
* The customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
* @see Stage
*/
public final Stage stage() {
return Stage.fromValue(stage);
}
/**
*
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services stages
* from the current stage in the partner CRM. This field provides a translated value of the stage, and offers
* insight into the Opportunity
's progression in the sales cycle, according to Amazon Web Services
* definitions.
*
*
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end customer
* through a lead) or latent (Your account team believes it exists based on research, account plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements. The
* customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stage} will return
* {@link Stage#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stageAsString}.
*
*
* @return Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services
* stages from the current stage in the partner CRM. This field provides a translated value of the stage,
* and offers insight into the Opportunity
's progression in the sales cycle, according to
* Amazon Web Services definitions.
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end
* customer through a lead) or latent (Your account team believes it exists based on research, account
* plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements.
* The customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
* @see Stage
*/
public final String stageAsString() {
return stage;
}
/**
*
* Specifies the date when Amazon Web Services expects to start significant billing, when the project finishes, and
* when it moves into production. This field informs the Amazon Web Services seller about when the opportunity
* launches and starts to incur Amazon Web Services usage.
*
*
* Ensure the Target Close Date
isn't in the past.
*
*
* @return Specifies the date when Amazon Web Services expects to start significant billing, when the project
* finishes, and when it moves into production. This field informs the Amazon Web Services seller about when
* the opportunity launches and starts to incur Amazon Web Services usage.
*
* Ensure the Target Close Date
isn't in the past.
*/
public final String targetCloseDate() {
return targetCloseDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(closedLostReasonAsString());
hashCode = 31 * hashCode + Objects.hashCode(nextSteps());
hashCode = 31 * hashCode + Objects.hashCode(reviewComments());
hashCode = 31 * hashCode + Objects.hashCode(reviewStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(reviewStatusReason());
hashCode = 31 * hashCode + Objects.hashCode(stageAsString());
hashCode = 31 * hashCode + Objects.hashCode(targetCloseDate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LifeCycleSummary)) {
return false;
}
LifeCycleSummary other = (LifeCycleSummary) obj;
return Objects.equals(closedLostReasonAsString(), other.closedLostReasonAsString())
&& Objects.equals(nextSteps(), other.nextSteps()) && Objects.equals(reviewComments(), other.reviewComments())
&& Objects.equals(reviewStatusAsString(), other.reviewStatusAsString())
&& Objects.equals(reviewStatusReason(), other.reviewStatusReason())
&& Objects.equals(stageAsString(), other.stageAsString())
&& Objects.equals(targetCloseDate(), other.targetCloseDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LifeCycleSummary").add("ClosedLostReason", closedLostReasonAsString())
.add("NextSteps", nextSteps() == null ? null : "*** Sensitive Data Redacted ***")
.add("ReviewComments", reviewComments()).add("ReviewStatus", reviewStatusAsString())
.add("ReviewStatusReason", reviewStatusReason()).add("Stage", stageAsString())
.add("TargetCloseDate", targetCloseDate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClosedLostReason":
return Optional.ofNullable(clazz.cast(closedLostReasonAsString()));
case "NextSteps":
return Optional.ofNullable(clazz.cast(nextSteps()));
case "ReviewComments":
return Optional.ofNullable(clazz.cast(reviewComments()));
case "ReviewStatus":
return Optional.ofNullable(clazz.cast(reviewStatusAsString()));
case "ReviewStatusReason":
return Optional.ofNullable(clazz.cast(reviewStatusReason()));
case "Stage":
return Optional.ofNullable(clazz.cast(stageAsString()));
case "TargetCloseDate":
return Optional.ofNullable(clazz.cast(targetCloseDate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ClosedLostReason", CLOSED_LOST_REASON_FIELD);
map.put("NextSteps", NEXT_STEPS_FIELD);
map.put("ReviewComments", REVIEW_COMMENTS_FIELD);
map.put("ReviewStatus", REVIEW_STATUS_FIELD);
map.put("ReviewStatusReason", REVIEW_STATUS_REASON_FIELD);
map.put("Stage", STAGE_FIELD);
map.put("TargetCloseDate", TARGET_CLOSE_DATE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the
* UpdateOpportunity
API action to update the opportunity, and ensure you make all required
* changes. Only these fields are editable when the Lifecycle.ReviewStatus
is
* Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues
* are resolved, and the opportunity's Lifecycle.ReviewStatus
is set to
* Approved
or Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
* @see ReviewStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewStatus
*/
Builder reviewStatus(String reviewStatus);
/**
*
* Indicates the review status of a partner referred opportunity. This field is read-only and only applicable
* for partner referrals. Valid values:
*
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the UpdateOpportunity
* API action to update the opportunity, and ensure you make all required changes. Only these fields are
* editable when the Lifecycle.ReviewStatus
is Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues are
* resolved, and the opportunity's Lifecycle.ReviewStatus
is set to Approved
or
* Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
*
*
* @param reviewStatus
* Indicates the review status of a partner referred opportunity. This field is read-only and only
* applicable for partner referrals. Valid values:
*
* -
*
* Pending Submission: Not submitted for validation (editable).
*
*
* -
*
* Submitted: Submitted for validation and not yet Amazon Web Services reviewed (read-only).
*
*
* -
*
* In Review: Undergoing Amazon Web Services validation (read-only).
*
*
* -
*
* Action Required: Address any issues Amazon Web Services highlights. Use the
* UpdateOpportunity
API action to update the opportunity, and ensure you make all required
* changes. Only these fields are editable when the Lifecycle.ReviewStatus
is
* Action Required
:
*
*
* -
*
* Customer.Account.Address.City
*
*
* -
*
* Customer.Account.Address.CountryCode
*
*
* -
*
* Customer.Account.Address.PostalCode
*
*
* -
*
* Customer.Account.Address.StateOrRegion
*
*
* -
*
* Customer.Account.Address.StreetAddress
*
*
* -
*
* Customer.Account.WebsiteUrl
*
*
* -
*
* LifeCycle.TargetCloseDate
*
*
* -
*
* Project.ExpectedCustomerSpend.Amount
*
*
* -
*
* Project.ExpectedCustomerSpend.CurrencyCode
*
*
* -
*
* Project.CustomerBusinessProblem
*
*
* -
*
* PartnerOpportunityIdentifier
*
*
*
*
* After updates, the opportunity re-enters the validation phase. This process repeats until all issues
* are resolved, and the opportunity's Lifecycle.ReviewStatus
is set to
* Approved
or Rejected
.
*
*
* -
*
* Approved: Validated and converted into the Amazon Web Services seller's pipeline (editable).
*
*
* -
*
* Rejected: Disqualified (read-only).
*
*
* @see ReviewStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewStatus
*/
Builder reviewStatus(ReviewStatus reviewStatus);
/**
*
* Indicates the reason a specific decision was taken during the opportunity review process. This field combines
* the reasons for both disqualified and action required statuses, and provides clarity for why an opportunity
* was disqualified or required further action.
*
*
* @param reviewStatusReason
* Indicates the reason a specific decision was taken during the opportunity review process. This field
* combines the reasons for both disqualified and action required statuses, and provides clarity for why
* an opportunity was disqualified or required further action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder reviewStatusReason(String reviewStatusReason);
/**
*
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services
* stages from the current stage in the partner CRM. This field provides a translated value of the stage, and
* offers insight into the Opportunity
's progression in the sales cycle, according to Amazon Web
* Services definitions.
*
*
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end
* customer through a lead) or latent (Your account team believes it exists based on research, account plans,
* sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements. The
* customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
*
*
* @param stage
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web
* Services stages from the current stage in the partner CRM. This field provides a translated value of
* the stage, and offers insight into the Opportunity
's progression in the sales cycle,
* according to Amazon Web Services definitions.
*
* A lead and a prospect must be further matured to a Qualified
opportunity before
* submission. Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the
* end customer through a lead) or latent (Your account team believes it exists based on research,
* account plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand
* requirements. The customer agreed that the opportunity is real, of interest, and may solve
* business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
* @see Stage
* @return Returns a reference to this object so that method calls can be chained together.
* @see Stage
*/
Builder stage(String stage);
/**
*
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web Services
* stages from the current stage in the partner CRM. This field provides a translated value of the stage, and
* offers insight into the Opportunity
's progression in the sales cycle, according to Amazon Web
* Services definitions.
*
*
*
* A lead and a prospect must be further matured to a Qualified
opportunity before submission.
* Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the end
* customer through a lead) or latent (Your account team believes it exists based on research, account plans,
* sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand requirements. The
* customer agreed that the opportunity is real, of interest, and may solve business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
*
*
* @param stage
* Specifies the current stage of the Opportunity
's lifecycle as it maps to Amazon Web
* Services stages from the current stage in the partner CRM. This field provides a translated value of
* the stage, and offers insight into the Opportunity
's progression in the sales cycle,
* according to Amazon Web Services definitions.
*
* A lead and a prospect must be further matured to a Qualified
opportunity before
* submission. Opportunities that were closed/lost before submission aren't suitable for submission.
*
*
*
* The descriptions of each sales stage are:
*
*
* -
*
* Prospect: Amazon Web Services identifies the opportunity. It can be active (Comes directly from the
* end customer through a lead) or latent (Your account team believes it exists based on research,
* account plans, sales plays).
*
*
* -
*
* Qualified: Your account team engaged with the customer to discuss viability and understand
* requirements. The customer agreed that the opportunity is real, of interest, and may solve
* business/technical needs.
*
*
* -
*
* Technical Validation: All parties understand the implementation plan.
*
*
* -
*
* Business Validation: Pricing was proposed, and all parties agree to the steps to close.
*
*
* -
*
* Committed: The customer signed the contract, but Amazon Web Services hasn't started billing.
*
*
* -
*
* Launched: The workload is complete, and Amazon Web Services has started billing.
*
*
* -
*
* Closed Lost: The opportunity is lost, and there are no steps to move forward.
*
*
* @see Stage
* @return Returns a reference to this object so that method calls can be chained together.
* @see Stage
*/
Builder stage(Stage stage);
/**
*
* Specifies the date when Amazon Web Services expects to start significant billing, when the project finishes,
* and when it moves into production. This field informs the Amazon Web Services seller about when the
* opportunity launches and starts to incur Amazon Web Services usage.
*
*
* Ensure the Target Close Date
isn't in the past.
*
*
* @param targetCloseDate
* Specifies the date when Amazon Web Services expects to start significant billing, when the project
* finishes, and when it moves into production. This field informs the Amazon Web Services seller about
* when the opportunity launches and starts to incur Amazon Web Services usage.
*
* Ensure the Target Close Date
isn't in the past.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder targetCloseDate(String targetCloseDate);
}
static final class BuilderImpl implements Builder {
private String closedLostReason;
private String nextSteps;
private String reviewComments;
private String reviewStatus;
private String reviewStatusReason;
private String stage;
private String targetCloseDate;
private BuilderImpl() {
}
private BuilderImpl(LifeCycleSummary model) {
closedLostReason(model.closedLostReason);
nextSteps(model.nextSteps);
reviewComments(model.reviewComments);
reviewStatus(model.reviewStatus);
reviewStatusReason(model.reviewStatusReason);
stage(model.stage);
targetCloseDate(model.targetCloseDate);
}
public final String getClosedLostReason() {
return closedLostReason;
}
public final void setClosedLostReason(String closedLostReason) {
this.closedLostReason = closedLostReason;
}
@Override
public final Builder closedLostReason(String closedLostReason) {
this.closedLostReason = closedLostReason;
return this;
}
@Override
public final Builder closedLostReason(ClosedLostReason closedLostReason) {
this.closedLostReason(closedLostReason == null ? null : closedLostReason.toString());
return this;
}
public final String getNextSteps() {
return nextSteps;
}
public final void setNextSteps(String nextSteps) {
this.nextSteps = nextSteps;
}
@Override
public final Builder nextSteps(String nextSteps) {
this.nextSteps = nextSteps;
return this;
}
public final String getReviewComments() {
return reviewComments;
}
public final void setReviewComments(String reviewComments) {
this.reviewComments = reviewComments;
}
@Override
public final Builder reviewComments(String reviewComments) {
this.reviewComments = reviewComments;
return this;
}
public final String getReviewStatus() {
return reviewStatus;
}
public final void setReviewStatus(String reviewStatus) {
this.reviewStatus = reviewStatus;
}
@Override
public final Builder reviewStatus(String reviewStatus) {
this.reviewStatus = reviewStatus;
return this;
}
@Override
public final Builder reviewStatus(ReviewStatus reviewStatus) {
this.reviewStatus(reviewStatus == null ? null : reviewStatus.toString());
return this;
}
public final String getReviewStatusReason() {
return reviewStatusReason;
}
public final void setReviewStatusReason(String reviewStatusReason) {
this.reviewStatusReason = reviewStatusReason;
}
@Override
public final Builder reviewStatusReason(String reviewStatusReason) {
this.reviewStatusReason = reviewStatusReason;
return this;
}
public final String getStage() {
return stage;
}
public final void setStage(String stage) {
this.stage = stage;
}
@Override
public final Builder stage(String stage) {
this.stage = stage;
return this;
}
@Override
public final Builder stage(Stage stage) {
this.stage(stage == null ? null : stage.toString());
return this;
}
public final String getTargetCloseDate() {
return targetCloseDate;
}
public final void setTargetCloseDate(String targetCloseDate) {
this.targetCloseDate = targetCloseDate;
}
@Override
public final Builder targetCloseDate(String targetCloseDate) {
this.targetCloseDate = targetCloseDate;
return this;
}
@Override
public LifeCycleSummary build() {
return new LifeCycleSummary(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}