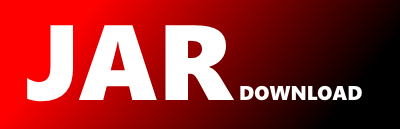
software.amazon.awssdk.services.partnercentralselling.model.SoftwareRevenue Maven / Gradle / Ivy
Show all versions of partnercentralselling Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.partnercentralselling.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies a customer's procurement terms details. Required only for partners in eligible programs.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SoftwareRevenue implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DELIVERY_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DeliveryModel").getter(getter(SoftwareRevenue::deliveryModelAsString))
.setter(setter(Builder::deliveryModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeliveryModel").build()).build();
private static final SdkField EFFECTIVE_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EffectiveDate").getter(getter(SoftwareRevenue::effectiveDate)).setter(setter(Builder::effectiveDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EffectiveDate").build()).build();
private static final SdkField EXPIRATION_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExpirationDate").getter(getter(SoftwareRevenue::expirationDate)).setter(setter(Builder::expirationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpirationDate").build()).build();
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Value").getter(getter(SoftwareRevenue::value)).setter(setter(Builder::value))
.constructor(MonetaryValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Value").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DELIVERY_MODEL_FIELD,
EFFECTIVE_DATE_FIELD, EXPIRATION_DATE_FIELD, VALUE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String deliveryModel;
private final String effectiveDate;
private final String expirationDate;
private final MonetaryValue value;
private SoftwareRevenue(BuilderImpl builder) {
this.deliveryModel = builder.deliveryModel;
this.effectiveDate = builder.effectiveDate;
this.expirationDate = builder.expirationDate;
this.value = builder.value;
}
/**
*
* Specifies the customer's intended payment type agreement or procurement method to acquire the solution or service
* outlined in the Opportunity
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #deliveryModel}
* will return {@link RevenueModel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #deliveryModelAsString}.
*
*
* @return Specifies the customer's intended payment type agreement or procurement method to acquire the solution or
* service outlined in the Opportunity
.
* @see RevenueModel
*/
public final RevenueModel deliveryModel() {
return RevenueModel.fromValue(deliveryModel);
}
/**
*
* Specifies the customer's intended payment type agreement or procurement method to acquire the solution or service
* outlined in the Opportunity
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #deliveryModel}
* will return {@link RevenueModel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #deliveryModelAsString}.
*
*
* @return Specifies the customer's intended payment type agreement or procurement method to acquire the solution or
* service outlined in the Opportunity
.
* @see RevenueModel
*/
public final String deliveryModelAsString() {
return deliveryModel;
}
/**
*
* Specifies the Opportunity
's customer engagement start date for the contract's effectiveness.
*
*
* @return Specifies the Opportunity
's customer engagement start date for the contract's effectiveness.
*/
public final String effectiveDate() {
return effectiveDate;
}
/**
*
* Specifies the expiration date for the contract between the customer and Amazon Web Services partner. It signifies
* the termination date of the agreed-upon engagement period between both parties.
*
*
* @return Specifies the expiration date for the contract between the customer and Amazon Web Services partner. It
* signifies the termination date of the agreed-upon engagement period between both parties.
*/
public final String expirationDate() {
return expirationDate;
}
/**
*
* Specifies the payment value (amount and currency).
*
*
* @return Specifies the payment value (amount and currency).
*/
public final MonetaryValue value() {
return value;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(deliveryModelAsString());
hashCode = 31 * hashCode + Objects.hashCode(effectiveDate());
hashCode = 31 * hashCode + Objects.hashCode(expirationDate());
hashCode = 31 * hashCode + Objects.hashCode(value());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SoftwareRevenue)) {
return false;
}
SoftwareRevenue other = (SoftwareRevenue) obj;
return Objects.equals(deliveryModelAsString(), other.deliveryModelAsString())
&& Objects.equals(effectiveDate(), other.effectiveDate())
&& Objects.equals(expirationDate(), other.expirationDate()) && Objects.equals(value(), other.value());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SoftwareRevenue").add("DeliveryModel", deliveryModelAsString())
.add("EffectiveDate", effectiveDate()).add("ExpirationDate", expirationDate()).add("Value", value()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DeliveryModel":
return Optional.ofNullable(clazz.cast(deliveryModelAsString()));
case "EffectiveDate":
return Optional.ofNullable(clazz.cast(effectiveDate()));
case "ExpirationDate":
return Optional.ofNullable(clazz.cast(expirationDate()));
case "Value":
return Optional.ofNullable(clazz.cast(value()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("DeliveryModel", DELIVERY_MODEL_FIELD);
map.put("EffectiveDate", EFFECTIVE_DATE_FIELD);
map.put("ExpirationDate", EXPIRATION_DATE_FIELD);
map.put("Value", VALUE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function