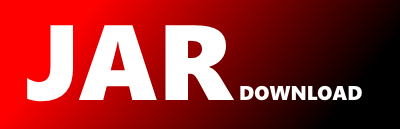
software.amazon.awssdk.services.pi.PiClient Maven / Gradle / Ivy
Show all versions of pi Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pi;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.pi.model.CreatePerformanceAnalysisReportRequest;
import software.amazon.awssdk.services.pi.model.CreatePerformanceAnalysisReportResponse;
import software.amazon.awssdk.services.pi.model.DeletePerformanceAnalysisReportRequest;
import software.amazon.awssdk.services.pi.model.DeletePerformanceAnalysisReportResponse;
import software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest;
import software.amazon.awssdk.services.pi.model.DescribeDimensionKeysResponse;
import software.amazon.awssdk.services.pi.model.GetDimensionKeyDetailsRequest;
import software.amazon.awssdk.services.pi.model.GetDimensionKeyDetailsResponse;
import software.amazon.awssdk.services.pi.model.GetPerformanceAnalysisReportRequest;
import software.amazon.awssdk.services.pi.model.GetPerformanceAnalysisReportResponse;
import software.amazon.awssdk.services.pi.model.GetResourceMetadataRequest;
import software.amazon.awssdk.services.pi.model.GetResourceMetadataResponse;
import software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest;
import software.amazon.awssdk.services.pi.model.GetResourceMetricsResponse;
import software.amazon.awssdk.services.pi.model.InternalServiceErrorException;
import software.amazon.awssdk.services.pi.model.InvalidArgumentException;
import software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest;
import software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsResponse;
import software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest;
import software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsResponse;
import software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest;
import software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsResponse;
import software.amazon.awssdk.services.pi.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.pi.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.pi.model.NotAuthorizedException;
import software.amazon.awssdk.services.pi.model.PiException;
import software.amazon.awssdk.services.pi.model.TagResourceRequest;
import software.amazon.awssdk.services.pi.model.TagResourceResponse;
import software.amazon.awssdk.services.pi.model.UntagResourceRequest;
import software.amazon.awssdk.services.pi.model.UntagResourceResponse;
import software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable;
import software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable;
import software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable;
import software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable;
import software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable;
/**
* Service client for accessing AWS PI. This can be created using the static {@link #builder()} method.
*
* Amazon RDS Performance Insights
*
* Amazon RDS Performance Insights enables you to monitor and explore different dimensions of database load based on
* data captured from a running DB instance. The guide provides detailed information about Performance Insights data
* types, parameters and errors.
*
*
* When Performance Insights is enabled, the Amazon RDS Performance Insights API provides visibility into the
* performance of your DB instance. Amazon CloudWatch provides the authoritative source for Amazon Web Services
* service-vended monitoring metrics. Performance Insights offers a domain-specific view of DB load.
*
*
* DB load is measured as average active sessions. Performance Insights provides the data to API consumers as a
* two-dimensional time-series dataset. The time dimension provides DB load data for each time point in the queried time
* range. Each time point decomposes overall load in relation to the requested dimensions, measured at that time point.
* Examples include SQL, Wait event, User, and Host.
*
*
* -
*
* To learn more about Performance Insights and Amazon Aurora DB instances, go to the Amazon Aurora User
* Guide .
*
*
* -
*
* To learn more about Performance Insights and Amazon RDS DB instances, go to the Amazon RDS User Guide .
*
*
* -
*
* To learn more about Performance Insights and Amazon DocumentDB clusters, go to the Amazon DocumentDB
* Developer Guide .
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface PiClient extends AwsClient {
String SERVICE_NAME = "pi";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "pi";
/**
*
* Creates a new performance analysis report for a specific time period for the DB instance.
*
*
* @param createPerformanceAnalysisReportRequest
* @return Result of the CreatePerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.CreatePerformanceAnalysisReport
* @see AWS API Documentation
*/
default CreatePerformanceAnalysisReportResponse createPerformanceAnalysisReport(
CreatePerformanceAnalysisReportRequest createPerformanceAnalysisReportRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new performance analysis report for a specific time period for the DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePerformanceAnalysisReportRequest.Builder}
* avoiding the need to create one manually via {@link CreatePerformanceAnalysisReportRequest#builder()}
*
*
* @param createPerformanceAnalysisReportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.CreatePerformanceAnalysisReportRequest.Builder} to create
* a request.
* @return Result of the CreatePerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.CreatePerformanceAnalysisReport
* @see AWS API Documentation
*/
default CreatePerformanceAnalysisReportResponse createPerformanceAnalysisReport(
Consumer createPerformanceAnalysisReportRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return createPerformanceAnalysisReport(CreatePerformanceAnalysisReportRequest.builder()
.applyMutation(createPerformanceAnalysisReportRequest).build());
}
/**
*
* Deletes a performance analysis report.
*
*
* @param deletePerformanceAnalysisReportRequest
* @return Result of the DeletePerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DeletePerformanceAnalysisReport
* @see AWS API Documentation
*/
default DeletePerformanceAnalysisReportResponse deletePerformanceAnalysisReport(
DeletePerformanceAnalysisReportRequest deletePerformanceAnalysisReportRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a performance analysis report.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePerformanceAnalysisReportRequest.Builder}
* avoiding the need to create one manually via {@link DeletePerformanceAnalysisReportRequest#builder()}
*
*
* @param deletePerformanceAnalysisReportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.DeletePerformanceAnalysisReportRequest.Builder} to create
* a request.
* @return Result of the DeletePerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DeletePerformanceAnalysisReport
* @see AWS API Documentation
*/
default DeletePerformanceAnalysisReportResponse deletePerformanceAnalysisReport(
Consumer deletePerformanceAnalysisReportRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return deletePerformanceAnalysisReport(DeletePerformanceAnalysisReportRequest.builder()
.applyMutation(deletePerformanceAnalysisReportRequest).build());
}
/**
*
* For a specific time period, retrieve the top N
dimension keys for a metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param describeDimensionKeysRequest
* @return Result of the DescribeDimensionKeys operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
default DescribeDimensionKeysResponse describeDimensionKeys(DescribeDimensionKeysRequest describeDimensionKeysRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* For a specific time period, retrieve the top N
dimension keys for a metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDimensionKeysRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDimensionKeysRequest#builder()}
*
*
* @param describeDimensionKeysRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest.Builder} to create a request.
* @return Result of the DescribeDimensionKeys operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
default DescribeDimensionKeysResponse describeDimensionKeys(
Consumer describeDimensionKeysRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return describeDimensionKeys(DescribeDimensionKeysRequest.builder().applyMutation(describeDimensionKeysRequest).build());
}
/**
*
* This is a variant of
* {@link #describeDimensionKeys(software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client.describeDimensionKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client
* .describeDimensionKeysPaginator(request);
* for (software.amazon.awssdk.services.pi.model.DescribeDimensionKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client.describeDimensionKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDimensionKeys(software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest)}
* operation.
*
*
* @param describeDimensionKeysRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
default DescribeDimensionKeysIterable describeDimensionKeysPaginator(DescribeDimensionKeysRequest describeDimensionKeysRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return new DescribeDimensionKeysIterable(this, describeDimensionKeysRequest);
}
/**
*
* This is a variant of
* {@link #describeDimensionKeys(software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client.describeDimensionKeysPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client
* .describeDimensionKeysPaginator(request);
* for (software.amazon.awssdk.services.pi.model.DescribeDimensionKeysResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.DescribeDimensionKeysIterable responses = client.describeDimensionKeysPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDimensionKeys(software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDimensionKeysRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDimensionKeysRequest#builder()}
*
*
* @param describeDimensionKeysRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.DescribeDimensionKeysRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.DescribeDimensionKeys
* @see AWS API
* Documentation
*/
default DescribeDimensionKeysIterable describeDimensionKeysPaginator(
Consumer describeDimensionKeysRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return describeDimensionKeysPaginator(DescribeDimensionKeysRequest.builder().applyMutation(describeDimensionKeysRequest)
.build());
}
/**
*
* Get the attributes of the specified dimension group for a DB instance or data source. For example, if you specify
* a SQL ID, GetDimensionKeyDetails
retrieves the full text of the dimension
* db.sql.statement
associated with this ID. This operation is useful because
* GetResourceMetrics
and DescribeDimensionKeys
don't support retrieval of large SQL
* statement text.
*
*
* @param getDimensionKeyDetailsRequest
* @return Result of the GetDimensionKeyDetails operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetDimensionKeyDetails
* @see AWS API
* Documentation
*/
default GetDimensionKeyDetailsResponse getDimensionKeyDetails(GetDimensionKeyDetailsRequest getDimensionKeyDetailsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Get the attributes of the specified dimension group for a DB instance or data source. For example, if you specify
* a SQL ID, GetDimensionKeyDetails
retrieves the full text of the dimension
* db.sql.statement
associated with this ID. This operation is useful because
* GetResourceMetrics
and DescribeDimensionKeys
don't support retrieval of large SQL
* statement text.
*
*
*
* This is a convenience which creates an instance of the {@link GetDimensionKeyDetailsRequest.Builder} avoiding the
* need to create one manually via {@link GetDimensionKeyDetailsRequest#builder()}
*
*
* @param getDimensionKeyDetailsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.GetDimensionKeyDetailsRequest.Builder} to create a
* request.
* @return Result of the GetDimensionKeyDetails operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetDimensionKeyDetails
* @see AWS API
* Documentation
*/
default GetDimensionKeyDetailsResponse getDimensionKeyDetails(
Consumer getDimensionKeyDetailsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return getDimensionKeyDetails(GetDimensionKeyDetailsRequest.builder().applyMutation(getDimensionKeyDetailsRequest)
.build());
}
/**
*
* Retrieves the report including the report ID, status, time details, and the insights with recommendations. The
* report status can be RUNNING
, SUCCEEDED
, or FAILED
. The insights include
* the description
and recommendation
fields.
*
*
* @param getPerformanceAnalysisReportRequest
* @return Result of the GetPerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetPerformanceAnalysisReport
* @see AWS API Documentation
*/
default GetPerformanceAnalysisReportResponse getPerformanceAnalysisReport(
GetPerformanceAnalysisReportRequest getPerformanceAnalysisReportRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the report including the report ID, status, time details, and the insights with recommendations. The
* report status can be RUNNING
, SUCCEEDED
, or FAILED
. The insights include
* the description
and recommendation
fields.
*
*
*
* This is a convenience which creates an instance of the {@link GetPerformanceAnalysisReportRequest.Builder}
* avoiding the need to create one manually via {@link GetPerformanceAnalysisReportRequest#builder()}
*
*
* @param getPerformanceAnalysisReportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.GetPerformanceAnalysisReportRequest.Builder} to create a
* request.
* @return Result of the GetPerformanceAnalysisReport operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetPerformanceAnalysisReport
* @see AWS API Documentation
*/
default GetPerformanceAnalysisReportResponse getPerformanceAnalysisReport(
Consumer getPerformanceAnalysisReportRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return getPerformanceAnalysisReport(GetPerformanceAnalysisReportRequest.builder()
.applyMutation(getPerformanceAnalysisReportRequest).build());
}
/**
*
* Retrieve the metadata for different features. For example, the metadata might indicate that a feature is turned
* on or off on a specific DB instance.
*
*
* @param getResourceMetadataRequest
* @return Result of the GetResourceMetadata operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetadata
* @see AWS API
* Documentation
*/
default GetResourceMetadataResponse getResourceMetadata(GetResourceMetadataRequest getResourceMetadataRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve the metadata for different features. For example, the metadata might indicate that a feature is turned
* on or off on a specific DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceMetadataRequest.Builder} avoiding the
* need to create one manually via {@link GetResourceMetadataRequest#builder()}
*
*
* @param getResourceMetadataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.GetResourceMetadataRequest.Builder} to create a request.
* @return Result of the GetResourceMetadata operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetadata
* @see AWS API
* Documentation
*/
default GetResourceMetadataResponse getResourceMetadata(
Consumer getResourceMetadataRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return getResourceMetadata(GetResourceMetadataRequest.builder().applyMutation(getResourceMetadataRequest).build());
}
/**
*
* Retrieve Performance Insights metrics for a set of data sources over a time period. You can provide specific
* dimension groups and dimensions, and provide filtering criteria for each group. You must specify an aggregate
* function for each metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* @param getResourceMetricsRequest
* @return Result of the GetResourceMetrics operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetrics
* @see AWS API
* Documentation
*/
default GetResourceMetricsResponse getResourceMetrics(GetResourceMetricsRequest getResourceMetricsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve Performance Insights metrics for a set of data sources over a time period. You can provide specific
* dimension groups and dimensions, and provide filtering criteria for each group. You must specify an aggregate
* function for each metric.
*
*
*
* Each response element returns a maximum of 500 bytes. For larger elements, such as SQL statements, only the first
* 500 bytes are returned.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceMetricsRequest.Builder} avoiding the
* need to create one manually via {@link GetResourceMetricsRequest#builder()}
*
*
* @param getResourceMetricsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest.Builder} to create a request.
* @return Result of the GetResourceMetrics operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetrics
* @see AWS API
* Documentation
*/
default GetResourceMetricsResponse getResourceMetrics(Consumer getResourceMetricsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return getResourceMetrics(GetResourceMetricsRequest.builder().applyMutation(getResourceMetricsRequest).build());
}
/**
*
* This is a variant of
* {@link #getResourceMetrics(software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client.getResourceMetricsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client
* .getResourceMetricsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.GetResourceMetricsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client.getResourceMetricsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceMetrics(software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest)} operation.
*
*
* @param getResourceMetricsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetrics
* @see AWS API
* Documentation
*/
default GetResourceMetricsIterable getResourceMetricsPaginator(GetResourceMetricsRequest getResourceMetricsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return new GetResourceMetricsIterable(this, getResourceMetricsRequest);
}
/**
*
* This is a variant of
* {@link #getResourceMetrics(software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client.getResourceMetricsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client
* .getResourceMetricsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.GetResourceMetricsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.GetResourceMetricsIterable responses = client.getResourceMetricsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceMetrics(software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceMetricsRequest.Builder} avoiding the
* need to create one manually via {@link GetResourceMetricsRequest#builder()}
*
*
* @param getResourceMetricsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.GetResourceMetricsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.GetResourceMetrics
* @see AWS API
* Documentation
*/
default GetResourceMetricsIterable getResourceMetricsPaginator(
Consumer getResourceMetricsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return getResourceMetricsPaginator(GetResourceMetricsRequest.builder().applyMutation(getResourceMetricsRequest).build());
}
/**
*
* Retrieve the dimensions that can be queried for each specified metric type on a specified DB instance.
*
*
* @param listAvailableResourceDimensionsRequest
* @return Result of the ListAvailableResourceDimensions operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
default ListAvailableResourceDimensionsResponse listAvailableResourceDimensions(
ListAvailableResourceDimensionsRequest listAvailableResourceDimensionsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve the dimensions that can be queried for each specified metric type on a specified DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link ListAvailableResourceDimensionsRequest.Builder}
* avoiding the need to create one manually via {@link ListAvailableResourceDimensionsRequest#builder()}
*
*
* @param listAvailableResourceDimensionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest.Builder} to create
* a request.
* @return Result of the ListAvailableResourceDimensions operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
default ListAvailableResourceDimensionsResponse listAvailableResourceDimensions(
Consumer listAvailableResourceDimensionsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listAvailableResourceDimensions(ListAvailableResourceDimensionsRequest.builder()
.applyMutation(listAvailableResourceDimensionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listAvailableResourceDimensions(software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client.listAvailableResourceDimensionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client
* .listAvailableResourceDimensionsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client.listAvailableResourceDimensionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAvailableResourceDimensions(software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest)}
* operation.
*
*
* @param listAvailableResourceDimensionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
default ListAvailableResourceDimensionsIterable listAvailableResourceDimensionsPaginator(
ListAvailableResourceDimensionsRequest listAvailableResourceDimensionsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return new ListAvailableResourceDimensionsIterable(this, listAvailableResourceDimensionsRequest);
}
/**
*
* This is a variant of
* {@link #listAvailableResourceDimensions(software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client.listAvailableResourceDimensionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client
* .listAvailableResourceDimensionsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceDimensionsIterable responses = client.listAvailableResourceDimensionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAvailableResourceDimensions(software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAvailableResourceDimensionsRequest.Builder}
* avoiding the need to create one manually via {@link ListAvailableResourceDimensionsRequest#builder()}
*
*
* @param listAvailableResourceDimensionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListAvailableResourceDimensionsRequest.Builder} to create
* a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceDimensions
* @see AWS API Documentation
*/
default ListAvailableResourceDimensionsIterable listAvailableResourceDimensionsPaginator(
Consumer listAvailableResourceDimensionsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listAvailableResourceDimensionsPaginator(ListAvailableResourceDimensionsRequest.builder()
.applyMutation(listAvailableResourceDimensionsRequest).build());
}
/**
*
* Retrieve metrics of the specified types that can be queried for a specified DB instance.
*
*
* @param listAvailableResourceMetricsRequest
* @return Result of the ListAvailableResourceMetrics operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
default ListAvailableResourceMetricsResponse listAvailableResourceMetrics(
ListAvailableResourceMetricsRequest listAvailableResourceMetricsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve metrics of the specified types that can be queried for a specified DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link ListAvailableResourceMetricsRequest.Builder}
* avoiding the need to create one manually via {@link ListAvailableResourceMetricsRequest#builder()}
*
*
* @param listAvailableResourceMetricsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest.Builder} to create a
* request.
* @return Result of the ListAvailableResourceMetrics operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
default ListAvailableResourceMetricsResponse listAvailableResourceMetrics(
Consumer listAvailableResourceMetricsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listAvailableResourceMetrics(ListAvailableResourceMetricsRequest.builder()
.applyMutation(listAvailableResourceMetricsRequest).build());
}
/**
*
* This is a variant of
* {@link #listAvailableResourceMetrics(software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client.listAvailableResourceMetricsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client
* .listAvailableResourceMetricsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client.listAvailableResourceMetricsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAvailableResourceMetrics(software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest)}
* operation.
*
*
* @param listAvailableResourceMetricsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
default ListAvailableResourceMetricsIterable listAvailableResourceMetricsPaginator(
ListAvailableResourceMetricsRequest listAvailableResourceMetricsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return new ListAvailableResourceMetricsIterable(this, listAvailableResourceMetricsRequest);
}
/**
*
* This is a variant of
* {@link #listAvailableResourceMetrics(software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client.listAvailableResourceMetricsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client
* .listAvailableResourceMetricsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListAvailableResourceMetricsIterable responses = client.listAvailableResourceMetricsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAvailableResourceMetrics(software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListAvailableResourceMetricsRequest.Builder}
* avoiding the need to create one manually via {@link ListAvailableResourceMetricsRequest#builder()}
*
*
* @param listAvailableResourceMetricsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListAvailableResourceMetricsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListAvailableResourceMetrics
* @see AWS API Documentation
*/
default ListAvailableResourceMetricsIterable listAvailableResourceMetricsPaginator(
Consumer listAvailableResourceMetricsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listAvailableResourceMetricsPaginator(ListAvailableResourceMetricsRequest.builder()
.applyMutation(listAvailableResourceMetricsRequest).build());
}
/**
*
* Lists all the analysis reports created for the DB instance. The reports are sorted based on the start time of
* each report.
*
*
* @param listPerformanceAnalysisReportsRequest
* @return Result of the ListPerformanceAnalysisReports operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
default ListPerformanceAnalysisReportsResponse listPerformanceAnalysisReports(
ListPerformanceAnalysisReportsRequest listPerformanceAnalysisReportsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the analysis reports created for the DB instance. The reports are sorted based on the start time of
* each report.
*
*
*
* This is a convenience which creates an instance of the {@link ListPerformanceAnalysisReportsRequest.Builder}
* avoiding the need to create one manually via {@link ListPerformanceAnalysisReportsRequest#builder()}
*
*
* @param listPerformanceAnalysisReportsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest.Builder} to create a
* request.
* @return Result of the ListPerformanceAnalysisReports operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
default ListPerformanceAnalysisReportsResponse listPerformanceAnalysisReports(
Consumer listPerformanceAnalysisReportsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listPerformanceAnalysisReports(ListPerformanceAnalysisReportsRequest.builder()
.applyMutation(listPerformanceAnalysisReportsRequest).build());
}
/**
*
* This is a variant of
* {@link #listPerformanceAnalysisReports(software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client.listPerformanceAnalysisReportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client
* .listPerformanceAnalysisReportsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client.listPerformanceAnalysisReportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPerformanceAnalysisReports(software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest)}
* operation.
*
*
* @param listPerformanceAnalysisReportsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
default ListPerformanceAnalysisReportsIterable listPerformanceAnalysisReportsPaginator(
ListPerformanceAnalysisReportsRequest listPerformanceAnalysisReportsRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return new ListPerformanceAnalysisReportsIterable(this, listPerformanceAnalysisReportsRequest);
}
/**
*
* This is a variant of
* {@link #listPerformanceAnalysisReports(software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client.listPerformanceAnalysisReportsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client
* .listPerformanceAnalysisReportsPaginator(request);
* for (software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.pi.paginators.ListPerformanceAnalysisReportsIterable responses = client.listPerformanceAnalysisReportsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPerformanceAnalysisReports(software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPerformanceAnalysisReportsRequest.Builder}
* avoiding the need to create one manually via {@link ListPerformanceAnalysisReportsRequest#builder()}
*
*
* @param listPerformanceAnalysisReportsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListPerformanceAnalysisReportsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListPerformanceAnalysisReports
* @see AWS API Documentation
*/
default ListPerformanceAnalysisReportsIterable listPerformanceAnalysisReportsPaginator(
Consumer listPerformanceAnalysisReportsRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return listPerformanceAnalysisReportsPaginator(ListPerformanceAnalysisReportsRequest.builder()
.applyMutation(listPerformanceAnalysisReportsRequest).build());
}
/**
*
* Retrieves all the metadata tags associated with Amazon RDS Performance Insights resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all the metadata tags associated with Amazon RDS Performance Insights resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.ListTagsForResourceRequest.Builder} to create a request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds metadata tags to the Amazon RDS Performance Insights resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Adds metadata tags to the Amazon RDS Performance Insights resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes the metadata tags from the Amazon RDS Performance Insights resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InvalidArgumentException,
InternalServiceErrorException, NotAuthorizedException, AwsServiceException, SdkClientException, PiException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the metadata tags from the Amazon RDS Performance Insights resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.pi.model.UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidArgumentException
* One of the arguments provided is invalid for this request.
* @throws InternalServiceErrorException
* The request failed due to an unknown error.
* @throws NotAuthorizedException
* The user is not authorized to perform this request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws PiException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample PiClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws InvalidArgumentException, InternalServiceErrorException, NotAuthorizedException, AwsServiceException,
SdkClientException, PiException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
* Create a {@link PiClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static PiClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link PiClient}.
*/
static PiClientBuilder builder() {
return new DefaultPiClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default PiServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}