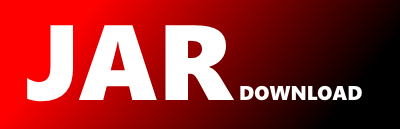
software.amazon.awssdk.services.pinpoint.DefaultPinpointAsyncClient Maven / Gradle / Ivy
Show all versions of pinpoint Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpoint;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.pinpoint.model.BadRequestException;
import software.amazon.awssdk.services.pinpoint.model.ConflictException;
import software.amazon.awssdk.services.pinpoint.model.CreateAppRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateAppResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateCampaignRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateCampaignResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateEmailTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateEmailTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateExportJobRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateExportJobResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateImportJobRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateImportJobResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateInAppTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateInAppTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateJourneyRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateJourneyResponse;
import software.amazon.awssdk.services.pinpoint.model.CreatePushTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.CreatePushTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateRecommenderConfigurationRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateRecommenderConfigurationResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateSegmentRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateSegmentResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateSmsTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateSmsTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.CreateVoiceTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.CreateVoiceTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteAdmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteAdmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsVoipChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsVoipChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsVoipSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteApnsVoipSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteAppRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteAppResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteBaiduChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteBaiduChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteCampaignRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteCampaignResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteEmailChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteEmailChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteEmailTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteEmailTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteEndpointRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteEndpointResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteEventStreamRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteEventStreamResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteGcmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteGcmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteInAppTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteInAppTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteJourneyRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteJourneyResponse;
import software.amazon.awssdk.services.pinpoint.model.DeletePushTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.DeletePushTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteRecommenderConfigurationRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteRecommenderConfigurationResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteSegmentRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteSegmentResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteSmsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteSmsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteSmsTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteSmsTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteUserEndpointsRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteUserEndpointsResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteVoiceChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteVoiceChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.DeleteVoiceTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.DeleteVoiceTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.ForbiddenException;
import software.amazon.awssdk.services.pinpoint.model.GetAdmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetAdmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApnsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApnsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApnsSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApnsSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApnsVoipChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApnsVoipChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApnsVoipSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApnsVoipSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetAppRequest;
import software.amazon.awssdk.services.pinpoint.model.GetAppResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApplicationDateRangeKpiRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApplicationDateRangeKpiResponse;
import software.amazon.awssdk.services.pinpoint.model.GetApplicationSettingsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetApplicationSettingsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetAppsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetAppsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetBaiduChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetBaiduChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignActivitiesRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignActivitiesResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignDateRangeKpiRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignDateRangeKpiResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignVersionRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignVersionResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignVersionsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignVersionsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetCampaignsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetChannelsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetChannelsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetEmailChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetEmailChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetEmailTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.GetEmailTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.GetEndpointRequest;
import software.amazon.awssdk.services.pinpoint.model.GetEndpointResponse;
import software.amazon.awssdk.services.pinpoint.model.GetEventStreamRequest;
import software.amazon.awssdk.services.pinpoint.model.GetEventStreamResponse;
import software.amazon.awssdk.services.pinpoint.model.GetExportJobRequest;
import software.amazon.awssdk.services.pinpoint.model.GetExportJobResponse;
import software.amazon.awssdk.services.pinpoint.model.GetExportJobsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetExportJobsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetGcmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetGcmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetImportJobRequest;
import software.amazon.awssdk.services.pinpoint.model.GetImportJobResponse;
import software.amazon.awssdk.services.pinpoint.model.GetImportJobsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetImportJobsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetInAppMessagesRequest;
import software.amazon.awssdk.services.pinpoint.model.GetInAppMessagesResponse;
import software.amazon.awssdk.services.pinpoint.model.GetInAppTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.GetInAppTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyDateRangeKpiRequest;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyDateRangeKpiResponse;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyExecutionActivityMetricsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyExecutionActivityMetricsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyExecutionMetricsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyExecutionMetricsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyRequest;
import software.amazon.awssdk.services.pinpoint.model.GetJourneyResponse;
import software.amazon.awssdk.services.pinpoint.model.GetPushTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.GetPushTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.GetRecommenderConfigurationRequest;
import software.amazon.awssdk.services.pinpoint.model.GetRecommenderConfigurationResponse;
import software.amazon.awssdk.services.pinpoint.model.GetRecommenderConfigurationsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetRecommenderConfigurationsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentExportJobsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentExportJobsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentImportJobsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentImportJobsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentVersionRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentVersionResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentVersionsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentVersionsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSegmentsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSmsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSmsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetSmsTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.GetSmsTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.GetUserEndpointsRequest;
import software.amazon.awssdk.services.pinpoint.model.GetUserEndpointsResponse;
import software.amazon.awssdk.services.pinpoint.model.GetVoiceChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.GetVoiceChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.GetVoiceTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.GetVoiceTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.InternalServerErrorException;
import software.amazon.awssdk.services.pinpoint.model.ListJourneysRequest;
import software.amazon.awssdk.services.pinpoint.model.ListJourneysResponse;
import software.amazon.awssdk.services.pinpoint.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.pinpoint.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.pinpoint.model.ListTemplateVersionsRequest;
import software.amazon.awssdk.services.pinpoint.model.ListTemplateVersionsResponse;
import software.amazon.awssdk.services.pinpoint.model.ListTemplatesRequest;
import software.amazon.awssdk.services.pinpoint.model.ListTemplatesResponse;
import software.amazon.awssdk.services.pinpoint.model.MethodNotAllowedException;
import software.amazon.awssdk.services.pinpoint.model.NotFoundException;
import software.amazon.awssdk.services.pinpoint.model.PayloadTooLargeException;
import software.amazon.awssdk.services.pinpoint.model.PhoneNumberValidateRequest;
import software.amazon.awssdk.services.pinpoint.model.PhoneNumberValidateResponse;
import software.amazon.awssdk.services.pinpoint.model.PinpointException;
import software.amazon.awssdk.services.pinpoint.model.PutEventStreamRequest;
import software.amazon.awssdk.services.pinpoint.model.PutEventStreamResponse;
import software.amazon.awssdk.services.pinpoint.model.PutEventsRequest;
import software.amazon.awssdk.services.pinpoint.model.PutEventsResponse;
import software.amazon.awssdk.services.pinpoint.model.RemoveAttributesRequest;
import software.amazon.awssdk.services.pinpoint.model.RemoveAttributesResponse;
import software.amazon.awssdk.services.pinpoint.model.SendMessagesRequest;
import software.amazon.awssdk.services.pinpoint.model.SendMessagesResponse;
import software.amazon.awssdk.services.pinpoint.model.SendUsersMessagesRequest;
import software.amazon.awssdk.services.pinpoint.model.SendUsersMessagesResponse;
import software.amazon.awssdk.services.pinpoint.model.TagResourceRequest;
import software.amazon.awssdk.services.pinpoint.model.TagResourceResponse;
import software.amazon.awssdk.services.pinpoint.model.TooManyRequestsException;
import software.amazon.awssdk.services.pinpoint.model.UntagResourceRequest;
import software.amazon.awssdk.services.pinpoint.model.UntagResourceResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateAdmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateAdmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsVoipChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsVoipChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsVoipSandboxChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateApnsVoipSandboxChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateApplicationSettingsRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateApplicationSettingsResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateBaiduChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateBaiduChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateCampaignRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateCampaignResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateEmailChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateEmailChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateEmailTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateEmailTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateEndpointRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateEndpointResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateEndpointsBatchRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateEndpointsBatchResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateGcmChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateGcmChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateInAppTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateInAppTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateJourneyRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateJourneyResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateJourneyStateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateJourneyStateResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdatePushTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdatePushTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateRecommenderConfigurationRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateRecommenderConfigurationResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateSegmentRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateSegmentResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateSmsChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateSmsChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateSmsTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateSmsTemplateResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateTemplateActiveVersionRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateTemplateActiveVersionResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateVoiceChannelRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateVoiceChannelResponse;
import software.amazon.awssdk.services.pinpoint.model.UpdateVoiceTemplateRequest;
import software.amazon.awssdk.services.pinpoint.model.UpdateVoiceTemplateResponse;
import software.amazon.awssdk.services.pinpoint.transform.CreateAppRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateCampaignRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateExportJobRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateImportJobRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateInAppTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateJourneyRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreatePushTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateRecommenderConfigurationRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateSegmentRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateSmsTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.CreateVoiceTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteAdmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteApnsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteApnsSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteApnsVoipChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteApnsVoipSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteAppRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteBaiduChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteCampaignRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteEmailChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteEndpointRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteEventStreamRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteGcmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteInAppTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteJourneyRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeletePushTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteRecommenderConfigurationRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteSegmentRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteSmsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteSmsTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteUserEndpointsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteVoiceChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.DeleteVoiceTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetAdmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApnsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApnsSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApnsVoipChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApnsVoipSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetAppRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApplicationDateRangeKpiRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetApplicationSettingsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetAppsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetBaiduChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignActivitiesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignDateRangeKpiRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignVersionRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignVersionsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetCampaignsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetChannelsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetEmailChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetEndpointRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetEventStreamRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetExportJobRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetExportJobsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetGcmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetImportJobRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetImportJobsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetInAppMessagesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetInAppTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetJourneyDateRangeKpiRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetJourneyExecutionActivityMetricsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetJourneyExecutionMetricsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetJourneyRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetPushTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetRecommenderConfigurationRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetRecommenderConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentExportJobsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentImportJobsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentVersionRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentVersionsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSegmentsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSmsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetSmsTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetUserEndpointsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetVoiceChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.GetVoiceTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.ListJourneysRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.ListTemplateVersionsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.ListTemplatesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.PhoneNumberValidateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.PutEventStreamRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.PutEventsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.RemoveAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.SendMessagesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.SendUsersMessagesRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateAdmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateApnsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateApnsSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateApnsVoipChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateApnsVoipSandboxChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateApplicationSettingsRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateBaiduChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateCampaignRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateEmailChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateEndpointRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateEndpointsBatchRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateGcmChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateInAppTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateJourneyRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateJourneyStateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdatePushTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateRecommenderConfigurationRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateSegmentRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateSmsChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateSmsTemplateRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateTemplateActiveVersionRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateVoiceChannelRequestMarshaller;
import software.amazon.awssdk.services.pinpoint.transform.UpdateVoiceTemplateRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link PinpointAsyncClient}.
*
* @see PinpointAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultPinpointAsyncClient implements PinpointAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultPinpointAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultPinpointAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates an application.
*
*
* @param createAppRequest
* @return A Java Future containing the result of the CreateApp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateApp
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createApp(CreateAppRequest createAppRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateApp")
.withMarshaller(new CreateAppRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(createAppRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createAppRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new campaign for an application or updates the settings of an existing campaign for an application.
*
*
* @param createCampaignRequest
* @return A Java Future containing the result of the CreateCampaign operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateCampaign
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createCampaign(CreateCampaignRequest createCampaignRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCampaign");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCampaign")
.withMarshaller(new CreateCampaignRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createCampaignRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createCampaignRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a message template for messages that are sent through the email channel.
*
*
* @param createEmailTemplateRequest
* @return A Java Future containing the result of the CreateEmailTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateEmailTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createEmailTemplate(
CreateEmailTemplateRequest createEmailTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEmailTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEmailTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEmailTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEmailTemplate")
.withMarshaller(new CreateEmailTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createEmailTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createEmailTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an export job for an application.
*
*
* @param createExportJobRequest
* @return A Java Future containing the result of the CreateExportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateExportJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createExportJob(CreateExportJobRequest createExportJobRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createExportJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateExportJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateExportJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateExportJob")
.withMarshaller(new CreateExportJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createExportJobRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createExportJobRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an import job for an application.
*
*
* @param createImportJobRequest
* @return A Java Future containing the result of the CreateImportJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateImportJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createImportJob(CreateImportJobRequest createImportJobRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createImportJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateImportJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateImportJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateImportJob")
.withMarshaller(new CreateImportJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createImportJobRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createImportJobRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new message template for messages using the in-app message channel.
*
*
* @param createInAppTemplateRequest
* @return A Java Future containing the result of the CreateInAppTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateInAppTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createInAppTemplate(
CreateInAppTemplateRequest createInAppTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInAppTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInAppTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateInAppTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateInAppTemplate")
.withMarshaller(new CreateInAppTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createInAppTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createInAppTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a journey for an application.
*
*
* @param createJourneyRequest
* @return A Java Future containing the result of the CreateJourney operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateJourney
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createJourney(CreateJourneyRequest createJourneyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createJourneyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateJourney");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateJourneyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateJourney")
.withMarshaller(new CreateJourneyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createJourneyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createJourneyRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a message template for messages that are sent through a push notification channel.
*
*
* @param createPushTemplateRequest
* @return A Java Future containing the result of the CreatePushTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreatePushTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createPushTemplate(CreatePushTemplateRequest createPushTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPushTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePushTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreatePushTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePushTemplate")
.withMarshaller(new CreatePushTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createPushTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createPushTemplateRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon Pinpoint configuration for a recommender model.
*
*
* @param createRecommenderConfigurationRequest
* @return A Java Future containing the result of the CreateRecommenderConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateRecommenderConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createRecommenderConfiguration(
CreateRecommenderConfigurationRequest createRecommenderConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createRecommenderConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRecommenderConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRecommenderConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateRecommenderConfiguration")
.withMarshaller(new CreateRecommenderConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createRecommenderConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createRecommenderConfigurationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new segment for an application or updates the configuration, dimension, and other settings for an
* existing segment that's associated with an application.
*
*
* @param createSegmentRequest
* @return A Java Future containing the result of the CreateSegment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateSegment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createSegment(CreateSegmentRequest createSegmentRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSegmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSegment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateSegmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSegment")
.withMarshaller(new CreateSegmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createSegmentRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createSegmentRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a message template for messages that are sent through the SMS channel.
*
*
* @param createSmsTemplateRequest
* @return A Java Future containing the result of the CreateSmsTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateSmsTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createSmsTemplate(CreateSmsTemplateRequest createSmsTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSmsTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSmsTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSmsTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSmsTemplate")
.withMarshaller(new CreateSmsTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createSmsTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createSmsTemplateRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a message template for messages that are sent through the voice channel.
*
*
* @param createVoiceTemplateRequest
* @return A Java Future containing the result of the CreateVoiceTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.CreateVoiceTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createVoiceTemplate(
CreateVoiceTemplateRequest createVoiceTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVoiceTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVoiceTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVoiceTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVoiceTemplate")
.withMarshaller(new CreateVoiceTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createVoiceTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createVoiceTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the ADM channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteAdmChannelRequest
* @return A Java Future containing the result of the DeleteAdmChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteAdmChannel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAdmChannel(DeleteAdmChannelRequest deleteAdmChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAdmChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAdmChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAdmChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAdmChannel")
.withMarshaller(new DeleteAdmChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteAdmChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteAdmChannelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the APNs channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteApnsChannelRequest
* @return A Java Future containing the result of the DeleteApnsChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteApnsChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteApnsChannel(DeleteApnsChannelRequest deleteApnsChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApnsChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApnsChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApnsChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApnsChannel")
.withMarshaller(new DeleteApnsChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteApnsChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteApnsChannelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the APNs sandbox channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteApnsSandboxChannelRequest
* @return A Java Future containing the result of the DeleteApnsSandboxChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteApnsSandboxChannel
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteApnsSandboxChannel(
DeleteApnsSandboxChannelRequest deleteApnsSandboxChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApnsSandboxChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApnsSandboxChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApnsSandboxChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApnsSandboxChannel")
.withMarshaller(new DeleteApnsSandboxChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteApnsSandboxChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteApnsSandboxChannelRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the APNs VoIP channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteApnsVoipChannelRequest
* @return A Java Future containing the result of the DeleteApnsVoipChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteApnsVoipChannel
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteApnsVoipChannel(
DeleteApnsVoipChannelRequest deleteApnsVoipChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApnsVoipChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApnsVoipChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApnsVoipChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApnsVoipChannel")
.withMarshaller(new DeleteApnsVoipChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteApnsVoipChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteApnsVoipChannelRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the APNs VoIP sandbox channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteApnsVoipSandboxChannelRequest
* @return A Java Future containing the result of the DeleteApnsVoipSandboxChannel operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteApnsVoipSandboxChannel
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteApnsVoipSandboxChannel(
DeleteApnsVoipSandboxChannelRequest deleteApnsVoipSandboxChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApnsVoipSandboxChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApnsVoipSandboxChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApnsVoipSandboxChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApnsVoipSandboxChannel")
.withMarshaller(new DeleteApnsVoipSandboxChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteApnsVoipSandboxChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteApnsVoipSandboxChannelRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an application.
*
*
* @param deleteAppRequest
* @return A Java Future containing the result of the DeleteApp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteApp
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteApp(DeleteAppRequest deleteAppRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteApp")
.withMarshaller(new DeleteAppRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAppRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteAppRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the Baidu channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteBaiduChannelRequest
* @return A Java Future containing the result of the DeleteBaiduChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteBaiduChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteBaiduChannel(DeleteBaiduChannelRequest deleteBaiduChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteBaiduChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteBaiduChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteBaiduChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteBaiduChannel")
.withMarshaller(new DeleteBaiduChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteBaiduChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteBaiduChannelRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a campaign from an application.
*
*
* @param deleteCampaignRequest
* @return A Java Future containing the result of the DeleteCampaign operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteCampaign
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteCampaign(DeleteCampaignRequest deleteCampaignRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCampaign");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCampaign")
.withMarshaller(new DeleteCampaignRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteCampaignRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteCampaignRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the email channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteEmailChannelRequest
* @return A Java Future containing the result of the DeleteEmailChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteEmailChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteEmailChannel(DeleteEmailChannelRequest deleteEmailChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEmailChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEmailChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEmailChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEmailChannel")
.withMarshaller(new DeleteEmailChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteEmailChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteEmailChannelRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a message template for messages that were sent through the email channel.
*
*
* @param deleteEmailTemplateRequest
* @return A Java Future containing the result of the DeleteEmailTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteEmailTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteEmailTemplate(
DeleteEmailTemplateRequest deleteEmailTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEmailTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEmailTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEmailTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEmailTemplate")
.withMarshaller(new DeleteEmailTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteEmailTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteEmailTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an endpoint from an application.
*
*
* @param deleteEndpointRequest
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteEndpoint
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteEndpoint(DeleteEndpointRequest deleteEndpointRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEndpoint")
.withMarshaller(new DeleteEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteEndpointRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteEndpointRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the event stream for an application.
*
*
* @param deleteEventStreamRequest
* @return A Java Future containing the result of the DeleteEventStream operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteEventStream
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteEventStream(DeleteEventStreamRequest deleteEventStreamRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEventStreamRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEventStream");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEventStreamResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEventStream")
.withMarshaller(new DeleteEventStreamRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteEventStreamRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteEventStreamRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the GCM channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteGcmChannelRequest
* @return A Java Future containing the result of the DeleteGcmChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteGcmChannel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteGcmChannel(DeleteGcmChannelRequest deleteGcmChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGcmChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGcmChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteGcmChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteGcmChannel")
.withMarshaller(new DeleteGcmChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteGcmChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteGcmChannelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a message template for messages sent using the in-app message channel.
*
*
* @param deleteInAppTemplateRequest
* @return A Java Future containing the result of the DeleteInAppTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteInAppTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteInAppTemplate(
DeleteInAppTemplateRequest deleteInAppTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInAppTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInAppTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteInAppTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInAppTemplate")
.withMarshaller(new DeleteInAppTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteInAppTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteInAppTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a journey from an application.
*
*
* @param deleteJourneyRequest
* @return A Java Future containing the result of the DeleteJourney operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteJourney
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteJourney(DeleteJourneyRequest deleteJourneyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteJourneyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteJourney");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteJourneyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteJourney")
.withMarshaller(new DeleteJourneyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteJourneyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteJourneyRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a message template for messages that were sent through a push notification channel.
*
*
* @param deletePushTemplateRequest
* @return A Java Future containing the result of the DeletePushTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeletePushTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deletePushTemplate(DeletePushTemplateRequest deletePushTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePushTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePushTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePushTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePushTemplate")
.withMarshaller(new DeletePushTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deletePushTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deletePushTemplateRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon Pinpoint configuration for a recommender model.
*
*
* @param deleteRecommenderConfigurationRequest
* @return A Java Future containing the result of the DeleteRecommenderConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteRecommenderConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteRecommenderConfiguration(
DeleteRecommenderConfigurationRequest deleteRecommenderConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteRecommenderConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRecommenderConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRecommenderConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRecommenderConfiguration")
.withMarshaller(new DeleteRecommenderConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteRecommenderConfigurationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteRecommenderConfigurationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a segment from an application.
*
*
* @param deleteSegmentRequest
* @return A Java Future containing the result of the DeleteSegment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteSegment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteSegment(DeleteSegmentRequest deleteSegmentRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSegmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSegment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteSegmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSegment")
.withMarshaller(new DeleteSegmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSegmentRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteSegmentRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the SMS channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteSmsChannelRequest
* @return A Java Future containing the result of the DeleteSmsChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteSmsChannel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteSmsChannel(DeleteSmsChannelRequest deleteSmsChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSmsChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSmsChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSmsChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSmsChannel")
.withMarshaller(new DeleteSmsChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSmsChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteSmsChannelRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a message template for messages that were sent through the SMS channel.
*
*
* @param deleteSmsTemplateRequest
* @return A Java Future containing the result of the DeleteSmsTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteSmsTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteSmsTemplate(DeleteSmsTemplateRequest deleteSmsTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSmsTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSmsTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSmsTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSmsTemplate")
.withMarshaller(new DeleteSmsTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSmsTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteSmsTemplateRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes all the endpoints that are associated with a specific user ID.
*
*
* @param deleteUserEndpointsRequest
* @return A Java Future containing the result of the DeleteUserEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteUserEndpoints
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteUserEndpoints(
DeleteUserEndpointsRequest deleteUserEndpointsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserEndpointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserEndpoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteUserEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUserEndpoints")
.withMarshaller(new DeleteUserEndpointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUserEndpointsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteUserEndpointsRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the voice channel for an application and deletes any existing settings for the channel.
*
*
* @param deleteVoiceChannelRequest
* @return A Java Future containing the result of the DeleteVoiceChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteVoiceChannel
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteVoiceChannel(DeleteVoiceChannelRequest deleteVoiceChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVoiceChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVoiceChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVoiceChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVoiceChannel")
.withMarshaller(new DeleteVoiceChannelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteVoiceChannelRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteVoiceChannelRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a message template for messages that were sent through the voice channel.
*
*
* @param deleteVoiceTemplateRequest
* @return A Java Future containing the result of the DeleteVoiceTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.DeleteVoiceTemplate
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteVoiceTemplate(
DeleteVoiceTemplateRequest deleteVoiceTemplateRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVoiceTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVoiceTemplate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVoiceTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVoiceTemplate")
.withMarshaller(new DeleteVoiceTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteVoiceTemplateRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteVoiceTemplateRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about the status and settings of the ADM channel for an application.
*
*
* @param getAdmChannelRequest
* @return A Java Future containing the result of the GetAdmChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException The request contains a syntax error (BadRequestException).
* - InternalServerErrorException The request failed due to an unknown internal server error, exception,
* or failure (InternalServerErrorException).
* - PayloadTooLargeException The request failed because the payload for the body of the request is too
* large (RequestEntityTooLargeException).
* - ForbiddenException The request was denied because access to the specified resource is forbidden
* (ForbiddenException).
* - NotFoundException The request failed because the specified resource was not found
* (NotFoundException).
* - MethodNotAllowedException The request failed because the method is not allowed for the specified
* resource (MethodNotAllowedException).
* - TooManyRequestsException The request failed because too many requests were sent during a certain
* amount of time (TooManyRequestsException).
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointAsyncClient.GetAdmChannel
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAdmChannel(GetAdmChannelRequest getAdmChannelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAdmChannelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAdmChannel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAdmChannelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams