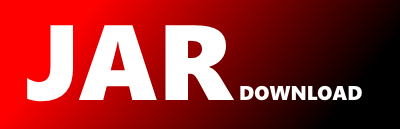
software.amazon.awssdk.services.pinpoint.model.EndpointDemographic Maven / Gradle / Ivy
Show all versions of pinpoint Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpoint.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies demographic information about an endpoint, such as the applicable time zone and platform.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EndpointDemographic implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField APP_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AppVersion").getter(getter(EndpointDemographic::appVersion)).setter(setter(Builder::appVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AppVersion").build()).build();
private static final SdkField LOCALE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Locale")
.getter(getter(EndpointDemographic::locale)).setter(setter(Builder::locale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Locale").build()).build();
private static final SdkField MAKE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Make")
.getter(getter(EndpointDemographic::make)).setter(setter(Builder::make))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Make").build()).build();
private static final SdkField MODEL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Model")
.getter(getter(EndpointDemographic::model)).setter(setter(Builder::model))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Model").build()).build();
private static final SdkField MODEL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ModelVersion").getter(getter(EndpointDemographic::modelVersion)).setter(setter(Builder::modelVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ModelVersion").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(EndpointDemographic::platform)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformVersion").getter(getter(EndpointDemographic::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformVersion").build()).build();
private static final SdkField TIMEZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Timezone").getter(getter(EndpointDemographic::timezone)).setter(setter(Builder::timezone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timezone").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APP_VERSION_FIELD,
LOCALE_FIELD, MAKE_FIELD, MODEL_FIELD, MODEL_VERSION_FIELD, PLATFORM_FIELD, PLATFORM_VERSION_FIELD, TIMEZONE_FIELD));
private static final long serialVersionUID = 1L;
private final String appVersion;
private final String locale;
private final String make;
private final String model;
private final String modelVersion;
private final String platform;
private final String platformVersion;
private final String timezone;
private EndpointDemographic(BuilderImpl builder) {
this.appVersion = builder.appVersion;
this.locale = builder.locale;
this.make = builder.make;
this.model = builder.model;
this.modelVersion = builder.modelVersion;
this.platform = builder.platform;
this.platformVersion = builder.platformVersion;
this.timezone = builder.timezone;
}
/**
*
* The version of the app that's associated with the endpoint.
*
*
* @return The version of the app that's associated with the endpoint.
*/
public final String appVersion() {
return appVersion;
}
/**
*
* The locale of the endpoint, in the following format: the ISO 639-1 alpha-2 code, followed by an underscore (_),
* followed by an ISO 3166-1 alpha-2 value.
*
*
* @return The locale of the endpoint, in the following format: the ISO 639-1 alpha-2 code, followed by an
* underscore (_), followed by an ISO 3166-1 alpha-2 value.
*/
public final String locale() {
return locale;
}
/**
*
* The manufacturer of the endpoint device, such as apple or samsung.
*
*
* @return The manufacturer of the endpoint device, such as apple or samsung.
*/
public final String make() {
return make;
}
/**
*
* The model name or number of the endpoint device, such as iPhone or SM-G900F.
*
*
* @return The model name or number of the endpoint device, such as iPhone or SM-G900F.
*/
public final String model() {
return model;
}
/**
*
* The model version of the endpoint device.
*
*
* @return The model version of the endpoint device.
*/
public final String modelVersion() {
return modelVersion;
}
/**
*
* The platform of the endpoint device, such as ios.
*
*
* @return The platform of the endpoint device, such as ios.
*/
public final String platform() {
return platform;
}
/**
*
* The platform version of the endpoint device.
*
*
* @return The platform version of the endpoint device.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The time zone of the endpoint, specified as a tz database name value, such as America/Los_Angeles.
*
*
* @return The time zone of the endpoint, specified as a tz database name value, such as America/Los_Angeles.
*/
public final String timezone() {
return timezone;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(appVersion());
hashCode = 31 * hashCode + Objects.hashCode(locale());
hashCode = 31 * hashCode + Objects.hashCode(make());
hashCode = 31 * hashCode + Objects.hashCode(model());
hashCode = 31 * hashCode + Objects.hashCode(modelVersion());
hashCode = 31 * hashCode + Objects.hashCode(platform());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(timezone());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EndpointDemographic)) {
return false;
}
EndpointDemographic other = (EndpointDemographic) obj;
return Objects.equals(appVersion(), other.appVersion()) && Objects.equals(locale(), other.locale())
&& Objects.equals(make(), other.make()) && Objects.equals(model(), other.model())
&& Objects.equals(modelVersion(), other.modelVersion()) && Objects.equals(platform(), other.platform())
&& Objects.equals(platformVersion(), other.platformVersion()) && Objects.equals(timezone(), other.timezone());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EndpointDemographic").add("AppVersion", appVersion()).add("Locale", locale())
.add("Make", make()).add("Model", model()).add("ModelVersion", modelVersion()).add("Platform", platform())
.add("PlatformVersion", platformVersion()).add("Timezone", timezone()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AppVersion":
return Optional.ofNullable(clazz.cast(appVersion()));
case "Locale":
return Optional.ofNullable(clazz.cast(locale()));
case "Make":
return Optional.ofNullable(clazz.cast(make()));
case "Model":
return Optional.ofNullable(clazz.cast(model()));
case "ModelVersion":
return Optional.ofNullable(clazz.cast(modelVersion()));
case "Platform":
return Optional.ofNullable(clazz.cast(platform()));
case "PlatformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "Timezone":
return Optional.ofNullable(clazz.cast(timezone()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function