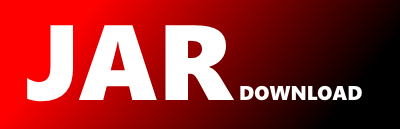
software.amazon.awssdk.services.pinpoint.model.NumberValidateResponse Maven / Gradle / Ivy
Show all versions of pinpoint Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpoint.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information about a phone number.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NumberValidateResponse implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CARRIER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Carrier")
.getter(getter(NumberValidateResponse::carrier)).setter(setter(Builder::carrier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Carrier").build()).build();
private static final SdkField CITY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("City")
.getter(getter(NumberValidateResponse::city)).setter(setter(Builder::city))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("City").build()).build();
private static final SdkField CLEANSED_PHONE_NUMBER_E164_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CleansedPhoneNumberE164").getter(getter(NumberValidateResponse::cleansedPhoneNumberE164))
.setter(setter(Builder::cleansedPhoneNumberE164))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CleansedPhoneNumberE164").build())
.build();
private static final SdkField CLEANSED_PHONE_NUMBER_NATIONAL_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CleansedPhoneNumberNational")
.getter(getter(NumberValidateResponse::cleansedPhoneNumberNational))
.setter(setter(Builder::cleansedPhoneNumberNational))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CleansedPhoneNumberNational")
.build()).build();
private static final SdkField COUNTRY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Country")
.getter(getter(NumberValidateResponse::country)).setter(setter(Builder::country))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Country").build()).build();
private static final SdkField COUNTRY_CODE_ISO2_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CountryCodeIso2").getter(getter(NumberValidateResponse::countryCodeIso2))
.setter(setter(Builder::countryCodeIso2))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CountryCodeIso2").build()).build();
private static final SdkField COUNTRY_CODE_NUMERIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CountryCodeNumeric").getter(getter(NumberValidateResponse::countryCodeNumeric))
.setter(setter(Builder::countryCodeNumeric))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CountryCodeNumeric").build())
.build();
private static final SdkField COUNTY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("County")
.getter(getter(NumberValidateResponse::county)).setter(setter(Builder::county))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("County").build()).build();
private static final SdkField ORIGINAL_COUNTRY_CODE_ISO2_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OriginalCountryCodeIso2").getter(getter(NumberValidateResponse::originalCountryCodeIso2))
.setter(setter(Builder::originalCountryCodeIso2))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OriginalCountryCodeIso2").build())
.build();
private static final SdkField ORIGINAL_PHONE_NUMBER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OriginalPhoneNumber").getter(getter(NumberValidateResponse::originalPhoneNumber))
.setter(setter(Builder::originalPhoneNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OriginalPhoneNumber").build())
.build();
private static final SdkField PHONE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PhoneType").getter(getter(NumberValidateResponse::phoneType)).setter(setter(Builder::phoneType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhoneType").build()).build();
private static final SdkField PHONE_TYPE_CODE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PhoneTypeCode").getter(getter(NumberValidateResponse::phoneTypeCode))
.setter(setter(Builder::phoneTypeCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PhoneTypeCode").build()).build();
private static final SdkField TIMEZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Timezone").getter(getter(NumberValidateResponse::timezone)).setter(setter(Builder::timezone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timezone").build()).build();
private static final SdkField ZIP_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ZipCode").getter(getter(NumberValidateResponse::zipCode)).setter(setter(Builder::zipCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ZipCode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CARRIER_FIELD, CITY_FIELD,
CLEANSED_PHONE_NUMBER_E164_FIELD, CLEANSED_PHONE_NUMBER_NATIONAL_FIELD, COUNTRY_FIELD, COUNTRY_CODE_ISO2_FIELD,
COUNTRY_CODE_NUMERIC_FIELD, COUNTY_FIELD, ORIGINAL_COUNTRY_CODE_ISO2_FIELD, ORIGINAL_PHONE_NUMBER_FIELD,
PHONE_TYPE_FIELD, PHONE_TYPE_CODE_FIELD, TIMEZONE_FIELD, ZIP_CODE_FIELD));
private static final long serialVersionUID = 1L;
private final String carrier;
private final String city;
private final String cleansedPhoneNumberE164;
private final String cleansedPhoneNumberNational;
private final String country;
private final String countryCodeIso2;
private final String countryCodeNumeric;
private final String county;
private final String originalCountryCodeIso2;
private final String originalPhoneNumber;
private final String phoneType;
private final Integer phoneTypeCode;
private final String timezone;
private final String zipCode;
private NumberValidateResponse(BuilderImpl builder) {
this.carrier = builder.carrier;
this.city = builder.city;
this.cleansedPhoneNumberE164 = builder.cleansedPhoneNumberE164;
this.cleansedPhoneNumberNational = builder.cleansedPhoneNumberNational;
this.country = builder.country;
this.countryCodeIso2 = builder.countryCodeIso2;
this.countryCodeNumeric = builder.countryCodeNumeric;
this.county = builder.county;
this.originalCountryCodeIso2 = builder.originalCountryCodeIso2;
this.originalPhoneNumber = builder.originalPhoneNumber;
this.phoneType = builder.phoneType;
this.phoneTypeCode = builder.phoneTypeCode;
this.timezone = builder.timezone;
this.zipCode = builder.zipCode;
}
/**
*
* The carrier or service provider that the phone number is currently registered with. In some countries and
* regions, this value may be the carrier or service provider that the phone number was originally registered with.
*
*
* @return The carrier or service provider that the phone number is currently registered with. In some countries and
* regions, this value may be the carrier or service provider that the phone number was originally
* registered with.
*/
public final String carrier() {
return carrier;
}
/**
*
* The name of the city where the phone number was originally registered.
*
*
* @return The name of the city where the phone number was originally registered.
*/
public final String city() {
return city;
}
/**
*
* The cleansed phone number, in E.164 format, for the location where the phone number was originally registered.
*
*
* @return The cleansed phone number, in E.164 format, for the location where the phone number was originally
* registered.
*/
public final String cleansedPhoneNumberE164() {
return cleansedPhoneNumberE164;
}
/**
*
* The cleansed phone number, in the format for the location where the phone number was originally registered.
*
*
* @return The cleansed phone number, in the format for the location where the phone number was originally
* registered.
*/
public final String cleansedPhoneNumberNational() {
return cleansedPhoneNumberNational;
}
/**
*
* The name of the country or region where the phone number was originally registered.
*
*
* @return The name of the country or region where the phone number was originally registered.
*/
public final String country() {
return country;
}
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region where the phone number was
* originally registered.
*
*
* @return The two-character code, in ISO 3166-1 alpha-2 format, for the country or region where the phone number
* was originally registered.
*/
public final String countryCodeIso2() {
return countryCodeIso2;
}
/**
*
* The numeric code for the country or region where the phone number was originally registered.
*
*
* @return The numeric code for the country or region where the phone number was originally registered.
*/
public final String countryCodeNumeric() {
return countryCodeNumeric;
}
/**
*
* The name of the county where the phone number was originally registered.
*
*
* @return The name of the county where the phone number was originally registered.
*/
public final String county() {
return county;
}
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, that was sent in the request body.
*
*
* @return The two-character code, in ISO 3166-1 alpha-2 format, that was sent in the request body.
*/
public final String originalCountryCodeIso2() {
return originalCountryCodeIso2;
}
/**
*
* The phone number that was sent in the request body.
*
*
* @return The phone number that was sent in the request body.
*/
public final String originalPhoneNumber() {
return originalPhoneNumber;
}
/**
*
* The description of the phone type. Valid values are: MOBILE, LANDLINE, VOIP, INVALID, PREPAID, and OTHER.
*
*
* @return The description of the phone type. Valid values are: MOBILE, LANDLINE, VOIP, INVALID, PREPAID, and OTHER.
*/
public final String phoneType() {
return phoneType;
}
/**
*
* The phone type, represented by an integer. Valid values are: 0 (mobile), 1 (landline), 2 (VoIP), 3 (invalid), 4
* (other), and 5 (prepaid).
*
*
* @return The phone type, represented by an integer. Valid values are: 0 (mobile), 1 (landline), 2 (VoIP), 3
* (invalid), 4 (other), and 5 (prepaid).
*/
public final Integer phoneTypeCode() {
return phoneTypeCode;
}
/**
*
* The time zone for the location where the phone number was originally registered.
*
*
* @return The time zone for the location where the phone number was originally registered.
*/
public final String timezone() {
return timezone;
}
/**
*
* The postal or ZIP code for the location where the phone number was originally registered.
*
*
* @return The postal or ZIP code for the location where the phone number was originally registered.
*/
public final String zipCode() {
return zipCode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(carrier());
hashCode = 31 * hashCode + Objects.hashCode(city());
hashCode = 31 * hashCode + Objects.hashCode(cleansedPhoneNumberE164());
hashCode = 31 * hashCode + Objects.hashCode(cleansedPhoneNumberNational());
hashCode = 31 * hashCode + Objects.hashCode(country());
hashCode = 31 * hashCode + Objects.hashCode(countryCodeIso2());
hashCode = 31 * hashCode + Objects.hashCode(countryCodeNumeric());
hashCode = 31 * hashCode + Objects.hashCode(county());
hashCode = 31 * hashCode + Objects.hashCode(originalCountryCodeIso2());
hashCode = 31 * hashCode + Objects.hashCode(originalPhoneNumber());
hashCode = 31 * hashCode + Objects.hashCode(phoneType());
hashCode = 31 * hashCode + Objects.hashCode(phoneTypeCode());
hashCode = 31 * hashCode + Objects.hashCode(timezone());
hashCode = 31 * hashCode + Objects.hashCode(zipCode());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NumberValidateResponse)) {
return false;
}
NumberValidateResponse other = (NumberValidateResponse) obj;
return Objects.equals(carrier(), other.carrier()) && Objects.equals(city(), other.city())
&& Objects.equals(cleansedPhoneNumberE164(), other.cleansedPhoneNumberE164())
&& Objects.equals(cleansedPhoneNumberNational(), other.cleansedPhoneNumberNational())
&& Objects.equals(country(), other.country()) && Objects.equals(countryCodeIso2(), other.countryCodeIso2())
&& Objects.equals(countryCodeNumeric(), other.countryCodeNumeric()) && Objects.equals(county(), other.county())
&& Objects.equals(originalCountryCodeIso2(), other.originalCountryCodeIso2())
&& Objects.equals(originalPhoneNumber(), other.originalPhoneNumber())
&& Objects.equals(phoneType(), other.phoneType()) && Objects.equals(phoneTypeCode(), other.phoneTypeCode())
&& Objects.equals(timezone(), other.timezone()) && Objects.equals(zipCode(), other.zipCode());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NumberValidateResponse").add("Carrier", carrier()).add("City", city())
.add("CleansedPhoneNumberE164", cleansedPhoneNumberE164())
.add("CleansedPhoneNumberNational", cleansedPhoneNumberNational()).add("Country", country())
.add("CountryCodeIso2", countryCodeIso2()).add("CountryCodeNumeric", countryCodeNumeric())
.add("County", county()).add("OriginalCountryCodeIso2", originalCountryCodeIso2())
.add("OriginalPhoneNumber", originalPhoneNumber()).add("PhoneType", phoneType())
.add("PhoneTypeCode", phoneTypeCode()).add("Timezone", timezone()).add("ZipCode", zipCode()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Carrier":
return Optional.ofNullable(clazz.cast(carrier()));
case "City":
return Optional.ofNullable(clazz.cast(city()));
case "CleansedPhoneNumberE164":
return Optional.ofNullable(clazz.cast(cleansedPhoneNumberE164()));
case "CleansedPhoneNumberNational":
return Optional.ofNullable(clazz.cast(cleansedPhoneNumberNational()));
case "Country":
return Optional.ofNullable(clazz.cast(country()));
case "CountryCodeIso2":
return Optional.ofNullable(clazz.cast(countryCodeIso2()));
case "CountryCodeNumeric":
return Optional.ofNullable(clazz.cast(countryCodeNumeric()));
case "County":
return Optional.ofNullable(clazz.cast(county()));
case "OriginalCountryCodeIso2":
return Optional.ofNullable(clazz.cast(originalCountryCodeIso2()));
case "OriginalPhoneNumber":
return Optional.ofNullable(clazz.cast(originalPhoneNumber()));
case "PhoneType":
return Optional.ofNullable(clazz.cast(phoneType()));
case "PhoneTypeCode":
return Optional.ofNullable(clazz.cast(phoneTypeCode()));
case "Timezone":
return Optional.ofNullable(clazz.cast(timezone()));
case "ZipCode":
return Optional.ofNullable(clazz.cast(zipCode()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function