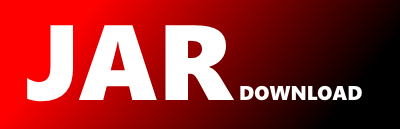
software.amazon.awssdk.services.pinpoint.model.SimpleCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pinpoint Show documentation
Show all versions of pinpoint Show documentation
The AWS Java SDK for Amazon Pinpoint module holds the client classes that are used for communicating
with Amazon Pinpoint Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpoint.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies a condition to evaluate for an activity in a journey.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SimpleCondition implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField EVENT_CONDITION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("EventCondition")
.getter(getter(SimpleCondition::eventCondition)).setter(setter(Builder::eventCondition))
.constructor(EventCondition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventCondition").build()).build();
private static final SdkField SEGMENT_CONDITION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SegmentCondition")
.getter(getter(SimpleCondition::segmentCondition)).setter(setter(Builder::segmentCondition))
.constructor(SegmentCondition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SegmentCondition").build()).build();
private static final SdkField SEGMENT_DIMENSIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SegmentDimensions")
.getter(getter(SimpleCondition::segmentDimensions)).setter(setter(Builder::segmentDimensions))
.constructor(SegmentDimensions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("segmentDimensions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EVENT_CONDITION_FIELD,
SEGMENT_CONDITION_FIELD, SEGMENT_DIMENSIONS_FIELD));
private static final long serialVersionUID = 1L;
private final EventCondition eventCondition;
private final SegmentCondition segmentCondition;
private final SegmentDimensions segmentDimensions;
private SimpleCondition(BuilderImpl builder) {
this.eventCondition = builder.eventCondition;
this.segmentCondition = builder.segmentCondition;
this.segmentDimensions = builder.segmentDimensions;
}
/**
*
* The dimension settings for the event that's associated with the activity.
*
*
* @return The dimension settings for the event that's associated with the activity.
*/
public final EventCondition eventCondition() {
return eventCondition;
}
/**
*
* The segment that's associated with the activity.
*
*
* @return The segment that's associated with the activity.
*/
public final SegmentCondition segmentCondition() {
return segmentCondition;
}
/**
*
* The dimension settings for the segment that's associated with the activity.
*
*
* @return The dimension settings for the segment that's associated with the activity.
*/
public final SegmentDimensions segmentDimensions() {
return segmentDimensions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eventCondition());
hashCode = 31 * hashCode + Objects.hashCode(segmentCondition());
hashCode = 31 * hashCode + Objects.hashCode(segmentDimensions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SimpleCondition)) {
return false;
}
SimpleCondition other = (SimpleCondition) obj;
return Objects.equals(eventCondition(), other.eventCondition())
&& Objects.equals(segmentCondition(), other.segmentCondition())
&& Objects.equals(segmentDimensions(), other.segmentDimensions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SimpleCondition").add("EventCondition", eventCondition())
.add("SegmentCondition", segmentCondition()).add("SegmentDimensions", segmentDimensions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EventCondition":
return Optional.ofNullable(clazz.cast(eventCondition()));
case "SegmentCondition":
return Optional.ofNullable(clazz.cast(segmentCondition()));
case "SegmentDimensions":
return Optional.ofNullable(clazz.cast(segmentDimensions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy