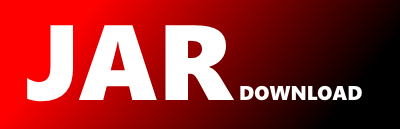
software.amazon.awssdk.services.pinpointemail.model.DomainDeliverabilityCampaign Maven / Gradle / Ivy
Show all versions of pinpointemail Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpointemail.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object that contains the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DomainDeliverabilityCampaign implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CAMPAIGN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CampaignId").getter(getter(DomainDeliverabilityCampaign::campaignId))
.setter(setter(Builder::campaignId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CampaignId").build()).build();
private static final SdkField IMAGE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImageUrl").getter(getter(DomainDeliverabilityCampaign::imageUrl)).setter(setter(Builder::imageUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImageUrl").build()).build();
private static final SdkField SUBJECT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Subject")
.getter(getter(DomainDeliverabilityCampaign::subject)).setter(setter(Builder::subject))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Subject").build()).build();
private static final SdkField FROM_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FromAddress").getter(getter(DomainDeliverabilityCampaign::fromAddress))
.setter(setter(Builder::fromAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FromAddress").build()).build();
private static final SdkField> SENDING_IPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SendingIps")
.getter(getter(DomainDeliverabilityCampaign::sendingIps))
.setter(setter(Builder::sendingIps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SendingIps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FIRST_SEEN_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("FirstSeenDateTime").getter(getter(DomainDeliverabilityCampaign::firstSeenDateTime))
.setter(setter(Builder::firstSeenDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirstSeenDateTime").build()).build();
private static final SdkField LAST_SEEN_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastSeenDateTime").getter(getter(DomainDeliverabilityCampaign::lastSeenDateTime))
.setter(setter(Builder::lastSeenDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastSeenDateTime").build()).build();
private static final SdkField INBOX_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("InboxCount").getter(getter(DomainDeliverabilityCampaign::inboxCount))
.setter(setter(Builder::inboxCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InboxCount").build()).build();
private static final SdkField SPAM_COUNT_FIELD = SdkField. builder(MarshallingType.LONG).memberName("SpamCount")
.getter(getter(DomainDeliverabilityCampaign::spamCount)).setter(setter(Builder::spamCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpamCount").build()).build();
private static final SdkField READ_RATE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("ReadRate").getter(getter(DomainDeliverabilityCampaign::readRate)).setter(setter(Builder::readRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReadRate").build()).build();
private static final SdkField DELETE_RATE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("DeleteRate").getter(getter(DomainDeliverabilityCampaign::deleteRate))
.setter(setter(Builder::deleteRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteRate").build()).build();
private static final SdkField READ_DELETE_RATE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("ReadDeleteRate").getter(getter(DomainDeliverabilityCampaign::readDeleteRate))
.setter(setter(Builder::readDeleteRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReadDeleteRate").build()).build();
private static final SdkField PROJECTED_VOLUME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ProjectedVolume").getter(getter(DomainDeliverabilityCampaign::projectedVolume))
.setter(setter(Builder::projectedVolume))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProjectedVolume").build()).build();
private static final SdkField> ESPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Esps")
.getter(getter(DomainDeliverabilityCampaign::esps))
.setter(setter(Builder::esps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Esps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CAMPAIGN_ID_FIELD,
IMAGE_URL_FIELD, SUBJECT_FIELD, FROM_ADDRESS_FIELD, SENDING_IPS_FIELD, FIRST_SEEN_DATE_TIME_FIELD,
LAST_SEEN_DATE_TIME_FIELD, INBOX_COUNT_FIELD, SPAM_COUNT_FIELD, READ_RATE_FIELD, DELETE_RATE_FIELD,
READ_DELETE_RATE_FIELD, PROJECTED_VOLUME_FIELD, ESPS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("CampaignId", CAMPAIGN_ID_FIELD);
put("ImageUrl", IMAGE_URL_FIELD);
put("Subject", SUBJECT_FIELD);
put("FromAddress", FROM_ADDRESS_FIELD);
put("SendingIps", SENDING_IPS_FIELD);
put("FirstSeenDateTime", FIRST_SEEN_DATE_TIME_FIELD);
put("LastSeenDateTime", LAST_SEEN_DATE_TIME_FIELD);
put("InboxCount", INBOX_COUNT_FIELD);
put("SpamCount", SPAM_COUNT_FIELD);
put("ReadRate", READ_RATE_FIELD);
put("DeleteRate", DELETE_RATE_FIELD);
put("ReadDeleteRate", READ_DELETE_RATE_FIELD);
put("ProjectedVolume", PROJECTED_VOLUME_FIELD);
put("Esps", ESPS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String campaignId;
private final String imageUrl;
private final String subject;
private final String fromAddress;
private final List sendingIps;
private final Instant firstSeenDateTime;
private final Instant lastSeenDateTime;
private final Long inboxCount;
private final Long spamCount;
private final Double readRate;
private final Double deleteRate;
private final Double readDeleteRate;
private final Long projectedVolume;
private final List esps;
private DomainDeliverabilityCampaign(BuilderImpl builder) {
this.campaignId = builder.campaignId;
this.imageUrl = builder.imageUrl;
this.subject = builder.subject;
this.fromAddress = builder.fromAddress;
this.sendingIps = builder.sendingIps;
this.firstSeenDateTime = builder.firstSeenDateTime;
this.lastSeenDateTime = builder.lastSeenDateTime;
this.inboxCount = builder.inboxCount;
this.spamCount = builder.spamCount;
this.readRate = builder.readRate;
this.deleteRate = builder.deleteRate;
this.readDeleteRate = builder.readDeleteRate;
this.projectedVolume = builder.projectedVolume;
this.esps = builder.esps;
}
/**
*
* The unique identifier for the campaign. Amazon Pinpoint automatically generates and assigns this identifier to a
* campaign. This value is not the same as the campaign identifier that Amazon Pinpoint assigns to campaigns that
* you create and manage by using the Amazon Pinpoint API or the Amazon Pinpoint console.
*
*
* @return The unique identifier for the campaign. Amazon Pinpoint automatically generates and assigns this
* identifier to a campaign. This value is not the same as the campaign identifier that Amazon Pinpoint
* assigns to campaigns that you create and manage by using the Amazon Pinpoint API or the Amazon Pinpoint
* console.
*/
public final String campaignId() {
return campaignId;
}
/**
*
* The URL of an image that contains a snapshot of the email message that was sent.
*
*
* @return The URL of an image that contains a snapshot of the email message that was sent.
*/
public final String imageUrl() {
return imageUrl;
}
/**
*
* The subject line, or title, of the email message.
*
*
* @return The subject line, or title, of the email message.
*/
public final String subject() {
return subject;
}
/**
*
* The verified email address that the email message was sent from.
*
*
* @return The verified email address that the email message was sent from.
*/
public final String fromAddress() {
return fromAddress;
}
/**
* For responses, this returns true if the service returned a value for the SendingIps property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSendingIps() {
return sendingIps != null && !(sendingIps instanceof SdkAutoConstructList);
}
/**
*
* The IP addresses that were used to send the email message.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSendingIps} method.
*
*
* @return The IP addresses that were used to send the email message.
*/
public final List sendingIps() {
return sendingIps;
}
/**
*
* The first time, in Unix time format, when the email message was delivered to any recipient's inbox. This value
* can help you determine how long it took for a campaign to deliver an email message.
*
*
* @return The first time, in Unix time format, when the email message was delivered to any recipient's inbox. This
* value can help you determine how long it took for a campaign to deliver an email message.
*/
public final Instant firstSeenDateTime() {
return firstSeenDateTime;
}
/**
*
* The last time, in Unix time format, when the email message was delivered to any recipient's inbox. This value can
* help you determine how long it took for a campaign to deliver an email message.
*
*
* @return The last time, in Unix time format, when the email message was delivered to any recipient's inbox. This
* value can help you determine how long it took for a campaign to deliver an email message.
*/
public final Instant lastSeenDateTime() {
return lastSeenDateTime;
}
/**
*
* The number of email messages that were delivered to recipients’ inboxes.
*
*
* @return The number of email messages that were delivered to recipients’ inboxes.
*/
public final Long inboxCount() {
return inboxCount;
}
/**
*
* The number of email messages that were delivered to recipients' spam or junk mail folders.
*
*
* @return The number of email messages that were delivered to recipients' spam or junk mail folders.
*/
public final Long spamCount() {
return spamCount;
}
/**
*
* The percentage of email messages that were opened by recipients. Due to technical limitations, this value only
* includes recipients who opened the message by using an email client that supports images.
*
*
* @return The percentage of email messages that were opened by recipients. Due to technical limitations, this value
* only includes recipients who opened the message by using an email client that supports images.
*/
public final Double readRate() {
return readRate;
}
/**
*
* The percentage of email messages that were deleted by recipients, without being opened first. Due to technical
* limitations, this value only includes recipients who opened the message by using an email client that supports
* images.
*
*
* @return The percentage of email messages that were deleted by recipients, without being opened first. Due to
* technical limitations, this value only includes recipients who opened the message by using an email
* client that supports images.
*/
public final Double deleteRate() {
return deleteRate;
}
/**
*
* The percentage of email messages that were opened and then deleted by recipients. Due to technical limitations,
* this value only includes recipients who opened the message by using an email client that supports images.
*
*
* @return The percentage of email messages that were opened and then deleted by recipients. Due to technical
* limitations, this value only includes recipients who opened the message by using an email client that
* supports images.
*/
public final Double readDeleteRate() {
return readDeleteRate;
}
/**
*
* The projected number of recipients that the email message was sent to.
*
*
* @return The projected number of recipients that the email message was sent to.
*/
public final Long projectedVolume() {
return projectedVolume;
}
/**
* For responses, this returns true if the service returned a value for the Esps property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasEsps() {
return esps != null && !(esps instanceof SdkAutoConstructList);
}
/**
*
* The major email providers who handled the email message.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEsps} method.
*
*
* @return The major email providers who handled the email message.
*/
public final List esps() {
return esps;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(campaignId());
hashCode = 31 * hashCode + Objects.hashCode(imageUrl());
hashCode = 31 * hashCode + Objects.hashCode(subject());
hashCode = 31 * hashCode + Objects.hashCode(fromAddress());
hashCode = 31 * hashCode + Objects.hashCode(hasSendingIps() ? sendingIps() : null);
hashCode = 31 * hashCode + Objects.hashCode(firstSeenDateTime());
hashCode = 31 * hashCode + Objects.hashCode(lastSeenDateTime());
hashCode = 31 * hashCode + Objects.hashCode(inboxCount());
hashCode = 31 * hashCode + Objects.hashCode(spamCount());
hashCode = 31 * hashCode + Objects.hashCode(readRate());
hashCode = 31 * hashCode + Objects.hashCode(deleteRate());
hashCode = 31 * hashCode + Objects.hashCode(readDeleteRate());
hashCode = 31 * hashCode + Objects.hashCode(projectedVolume());
hashCode = 31 * hashCode + Objects.hashCode(hasEsps() ? esps() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DomainDeliverabilityCampaign)) {
return false;
}
DomainDeliverabilityCampaign other = (DomainDeliverabilityCampaign) obj;
return Objects.equals(campaignId(), other.campaignId()) && Objects.equals(imageUrl(), other.imageUrl())
&& Objects.equals(subject(), other.subject()) && Objects.equals(fromAddress(), other.fromAddress())
&& hasSendingIps() == other.hasSendingIps() && Objects.equals(sendingIps(), other.sendingIps())
&& Objects.equals(firstSeenDateTime(), other.firstSeenDateTime())
&& Objects.equals(lastSeenDateTime(), other.lastSeenDateTime())
&& Objects.equals(inboxCount(), other.inboxCount()) && Objects.equals(spamCount(), other.spamCount())
&& Objects.equals(readRate(), other.readRate()) && Objects.equals(deleteRate(), other.deleteRate())
&& Objects.equals(readDeleteRate(), other.readDeleteRate())
&& Objects.equals(projectedVolume(), other.projectedVolume()) && hasEsps() == other.hasEsps()
&& Objects.equals(esps(), other.esps());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DomainDeliverabilityCampaign").add("CampaignId", campaignId()).add("ImageUrl", imageUrl())
.add("Subject", subject()).add("FromAddress", fromAddress())
.add("SendingIps", hasSendingIps() ? sendingIps() : null).add("FirstSeenDateTime", firstSeenDateTime())
.add("LastSeenDateTime", lastSeenDateTime()).add("InboxCount", inboxCount()).add("SpamCount", spamCount())
.add("ReadRate", readRate()).add("DeleteRate", deleteRate()).add("ReadDeleteRate", readDeleteRate())
.add("ProjectedVolume", projectedVolume()).add("Esps", hasEsps() ? esps() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CampaignId":
return Optional.ofNullable(clazz.cast(campaignId()));
case "ImageUrl":
return Optional.ofNullable(clazz.cast(imageUrl()));
case "Subject":
return Optional.ofNullable(clazz.cast(subject()));
case "FromAddress":
return Optional.ofNullable(clazz.cast(fromAddress()));
case "SendingIps":
return Optional.ofNullable(clazz.cast(sendingIps()));
case "FirstSeenDateTime":
return Optional.ofNullable(clazz.cast(firstSeenDateTime()));
case "LastSeenDateTime":
return Optional.ofNullable(clazz.cast(lastSeenDateTime()));
case "InboxCount":
return Optional.ofNullable(clazz.cast(inboxCount()));
case "SpamCount":
return Optional.ofNullable(clazz.cast(spamCount()));
case "ReadRate":
return Optional.ofNullable(clazz.cast(readRate()));
case "DeleteRate":
return Optional.ofNullable(clazz.cast(deleteRate()));
case "ReadDeleteRate":
return Optional.ofNullable(clazz.cast(readDeleteRate()));
case "ProjectedVolume":
return Optional.ofNullable(clazz.cast(projectedVolume()));
case "Esps":
return Optional.ofNullable(clazz.cast(esps()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function