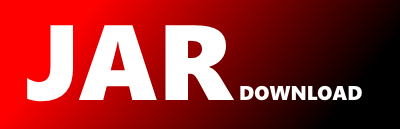
software.amazon.awssdk.services.pinpointemail.DefaultPinpointEmailAsyncClient Maven / Gradle / Ivy
Show all versions of pinpointemail Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.pinpointemail;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.pinpointemail.internal.PinpointEmailServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.pinpointemail.model.AccountSuspendedException;
import software.amazon.awssdk.services.pinpointemail.model.AlreadyExistsException;
import software.amazon.awssdk.services.pinpointemail.model.BadRequestException;
import software.amazon.awssdk.services.pinpointemail.model.ConcurrentModificationException;
import software.amazon.awssdk.services.pinpointemail.model.CreateConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.pinpointemail.model.CreateConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.pinpointemail.model.CreateConfigurationSetRequest;
import software.amazon.awssdk.services.pinpointemail.model.CreateConfigurationSetResponse;
import software.amazon.awssdk.services.pinpointemail.model.CreateDedicatedIpPoolRequest;
import software.amazon.awssdk.services.pinpointemail.model.CreateDedicatedIpPoolResponse;
import software.amazon.awssdk.services.pinpointemail.model.CreateDeliverabilityTestReportRequest;
import software.amazon.awssdk.services.pinpointemail.model.CreateDeliverabilityTestReportResponse;
import software.amazon.awssdk.services.pinpointemail.model.CreateEmailIdentityRequest;
import software.amazon.awssdk.services.pinpointemail.model.CreateEmailIdentityResponse;
import software.amazon.awssdk.services.pinpointemail.model.DeleteConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.pinpointemail.model.DeleteConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.pinpointemail.model.DeleteConfigurationSetRequest;
import software.amazon.awssdk.services.pinpointemail.model.DeleteConfigurationSetResponse;
import software.amazon.awssdk.services.pinpointemail.model.DeleteDedicatedIpPoolRequest;
import software.amazon.awssdk.services.pinpointemail.model.DeleteDedicatedIpPoolResponse;
import software.amazon.awssdk.services.pinpointemail.model.DeleteEmailIdentityRequest;
import software.amazon.awssdk.services.pinpointemail.model.DeleteEmailIdentityResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetAccountRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetAccountResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetBlacklistReportsRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetBlacklistReportsResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetConfigurationSetEventDestinationsRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetConfigurationSetEventDestinationsResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetConfigurationSetRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetConfigurationSetResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDedicatedIpRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDedicatedIpResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDedicatedIpsRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDedicatedIpsResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDeliverabilityDashboardOptionsRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDeliverabilityDashboardOptionsResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDeliverabilityTestReportRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDeliverabilityTestReportResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDomainDeliverabilityCampaignRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDomainDeliverabilityCampaignResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetDomainStatisticsReportRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetDomainStatisticsReportResponse;
import software.amazon.awssdk.services.pinpointemail.model.GetEmailIdentityRequest;
import software.amazon.awssdk.services.pinpointemail.model.GetEmailIdentityResponse;
import software.amazon.awssdk.services.pinpointemail.model.LimitExceededException;
import software.amazon.awssdk.services.pinpointemail.model.ListConfigurationSetsRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListConfigurationSetsResponse;
import software.amazon.awssdk.services.pinpointemail.model.ListDedicatedIpPoolsRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListDedicatedIpPoolsResponse;
import software.amazon.awssdk.services.pinpointemail.model.ListDeliverabilityTestReportsRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListDeliverabilityTestReportsResponse;
import software.amazon.awssdk.services.pinpointemail.model.ListDomainDeliverabilityCampaignsRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListDomainDeliverabilityCampaignsResponse;
import software.amazon.awssdk.services.pinpointemail.model.ListEmailIdentitiesRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListEmailIdentitiesResponse;
import software.amazon.awssdk.services.pinpointemail.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.pinpointemail.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.pinpointemail.model.MailFromDomainNotVerifiedException;
import software.amazon.awssdk.services.pinpointemail.model.MessageRejectedException;
import software.amazon.awssdk.services.pinpointemail.model.NotFoundException;
import software.amazon.awssdk.services.pinpointemail.model.PinpointEmailException;
import software.amazon.awssdk.services.pinpointemail.model.PutAccountDedicatedIpWarmupAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutAccountDedicatedIpWarmupAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutAccountSendingAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutAccountSendingAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetDeliveryOptionsRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetDeliveryOptionsResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetReputationOptionsRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetReputationOptionsResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetSendingOptionsRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetSendingOptionsResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetTrackingOptionsRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutConfigurationSetTrackingOptionsResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutDedicatedIpInPoolRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutDedicatedIpInPoolResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutDedicatedIpWarmupAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutDedicatedIpWarmupAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutDeliverabilityDashboardOptionRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutDeliverabilityDashboardOptionResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityDkimAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityDkimAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityFeedbackAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityFeedbackAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityMailFromAttributesRequest;
import software.amazon.awssdk.services.pinpointemail.model.PutEmailIdentityMailFromAttributesResponse;
import software.amazon.awssdk.services.pinpointemail.model.SendEmailRequest;
import software.amazon.awssdk.services.pinpointemail.model.SendEmailResponse;
import software.amazon.awssdk.services.pinpointemail.model.SendingPausedException;
import software.amazon.awssdk.services.pinpointemail.model.TagResourceRequest;
import software.amazon.awssdk.services.pinpointemail.model.TagResourceResponse;
import software.amazon.awssdk.services.pinpointemail.model.TooManyRequestsException;
import software.amazon.awssdk.services.pinpointemail.model.UntagResourceRequest;
import software.amazon.awssdk.services.pinpointemail.model.UntagResourceResponse;
import software.amazon.awssdk.services.pinpointemail.model.UpdateConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.pinpointemail.model.UpdateConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.pinpointemail.transform.CreateConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.CreateConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.CreateDedicatedIpPoolRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.CreateDeliverabilityTestReportRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.CreateEmailIdentityRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.DeleteConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.DeleteConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.DeleteDedicatedIpPoolRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.DeleteEmailIdentityRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetAccountRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetBlacklistReportsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetConfigurationSetEventDestinationsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDedicatedIpRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDedicatedIpsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDeliverabilityDashboardOptionsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDeliverabilityTestReportRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDomainDeliverabilityCampaignRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetDomainStatisticsReportRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.GetEmailIdentityRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListConfigurationSetsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListDedicatedIpPoolsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListDeliverabilityTestReportsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListDomainDeliverabilityCampaignsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListEmailIdentitiesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutAccountDedicatedIpWarmupAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutAccountSendingAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutConfigurationSetDeliveryOptionsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutConfigurationSetReputationOptionsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutConfigurationSetSendingOptionsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutConfigurationSetTrackingOptionsRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutDedicatedIpInPoolRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutDedicatedIpWarmupAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutDeliverabilityDashboardOptionRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutEmailIdentityDkimAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutEmailIdentityFeedbackAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.PutEmailIdentityMailFromAttributesRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.SendEmailRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.pinpointemail.transform.UpdateConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link PinpointEmailAsyncClient}.
*
* @see PinpointEmailAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultPinpointEmailAsyncClient implements PinpointEmailAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultPinpointEmailAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultPinpointEmailAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails you
* send using Amazon Pinpoint. You apply a configuration set to an email by including a reference to the
* configuration set in the headers of the email. When you apply a configuration set to an email, all of the rules
* in that configuration set are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException The resource specified in your request already exists.
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - LimitExceededException There are too many instances of the specified resource type.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.CreateConfigurationSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture createConfigurationSet(
CreateConfigurationSetRequest createConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConfigurationSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConfigurationSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create an event destination. In Amazon Pinpoint, events include message sends, deliveries, opens, clicks,
* bounces, and complaints. Event destinations are places that you can send information about these events
* to. For example, you can send event data to Amazon SNS to receive notifications when you receive bounces or
* complaints, or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - AlreadyExistsException The resource specified in your request already exists.
* - LimitExceededException There are too many instances of the specified resource type.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture createConfigurationSetEventDestination(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConfigurationSetEventDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createConfigurationSetEventDestinationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConfigurationSetEventDestination");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CreateConfigurationSetEventDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConfigurationSetEventDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConfigurationSetEventDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConfigurationSetEventDestinationRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Pinpoint account. You can associate a pool with a configuration set. When you send an
* email that uses that configuration set, Amazon Pinpoint sends it using only the IP addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @return A Java Future containing the result of the CreateDedicatedIpPool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException The resource specified in your request already exists.
* - LimitExceededException There are too many instances of the specified resource type.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.CreateDedicatedIpPool
* @see AWS API Documentation
*/
@Override
public CompletableFuture createDedicatedIpPool(
CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDedicatedIpPoolRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDedicatedIpPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDedicatedIpPool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDedicatedIpPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDedicatedIpPool").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDedicatedIpPoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDedicatedIpPoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon Pinpoint then sends that message to special email addresses spread across several major email providers.
* After about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
* operation to view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. Amazon Pinpoint then sends that message to special email addresses spread across
* several major email providers. After about 24 hours, the test is complete, and you can use the
* GetDeliverabilityTestReport
operation to view the results of the test.
* @return A Java Future containing the result of the CreateDeliverabilityTestReport operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccountSuspendedException The message can't be sent because the account's ability to send email has
* been permanently restricted.
* - SendingPausedException The message can't be sent because the account's ability to send email is
* currently paused.
* - MessageRejectedException The message can't be sent because it contains invalid content.
* - MailFromDomainNotVerifiedException The message can't be sent because the sending domain isn't
* verified.
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - LimitExceededException There are too many instances of the specified resource type.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
@Override
public CompletableFuture createDeliverabilityTestReport(
CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeliverabilityTestReportRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createDeliverabilityTestReportRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeliverabilityTestReport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDeliverabilityTestReportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDeliverabilityTestReport").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDeliverabilityTestReportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDeliverabilityTestReportRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Verifies an email identity for use with Amazon Pinpoint. In Amazon Pinpoint, an identity is an email address or
* domain that you use when you send email. Before you can use an identity to send email with Amazon Pinpoint, you
* first have to verify it. By verifying an address, you demonstrate that you're the owner of the address, and that
* you've given Amazon Pinpoint permission to send email from the address.
*
*
* When you verify an email address, Amazon Pinpoint sends an email to the address. Your email address is verified
* as soon as you follow the link in the verification email.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon Pinpoint
* detects these records in the DNS configuration for your domain. It usually takes around 72 hours to complete the
* domain verification process.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @return A Java Future containing the result of the CreateEmailIdentity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException There are too many instances of the specified resource type.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.CreateEmailIdentity
* @see AWS API Documentation
*/
@Override
public CompletableFuture createEmailIdentity(
CreateEmailIdentityRequest createEmailIdentityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEmailIdentityRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEmailIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEmailIdentity");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEmailIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEmailIdentity").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEmailIdentityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEmailIdentityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delete an existing configuration set.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.DeleteConfigurationSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteConfigurationSet(
DeleteConfigurationSetRequest deleteConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConfigurationSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConfigurationSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delete an event destination.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteConfigurationSetEventDestination(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConfigurationSetEventDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteConfigurationSetEventDestinationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConfigurationSetEventDestination");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DeleteConfigurationSetEventDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConfigurationSetEventDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConfigurationSetEventDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConfigurationSetEventDestinationRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @return A Java Future containing the result of the DeleteDedicatedIpPool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.DeleteDedicatedIpPool
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteDedicatedIpPool(
DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDedicatedIpPoolRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDedicatedIpPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDedicatedIpPool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDedicatedIpPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDedicatedIpPool").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteDedicatedIpPoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteDedicatedIpPoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an email identity that you previously verified for use with Amazon Pinpoint. An identity can be either an
* email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to use
* Amazon Pinpoint to send email from that identity. You can restore your ability to send email by completing
* the verification process for the identity again.
* @return A Java Future containing the result of the DeleteEmailIdentity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - ConcurrentModificationException The resource is being modified by another operation or thread.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.DeleteEmailIdentity
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteEmailIdentity(
DeleteEmailIdentityRequest deleteEmailIdentityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteEmailIdentityRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEmailIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEmailIdentity");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEmailIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEmailIdentity").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteEmailIdentityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteEmailIdentityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon Pinpoint account in the current
* AWS Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon Pinpoint account.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetAccount
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getAccount(GetAccountRequest getAccountRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAccountRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccount");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetAccount")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAccountRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @return A Java Future containing the result of the GetBlacklistReports operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetBlacklistReports
* @see AWS API Documentation
*/
@Override
public CompletableFuture getBlacklistReports(
GetBlacklistReportsRequest getBlacklistReportsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getBlacklistReportsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getBlacklistReportsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBlacklistReports");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetBlacklistReportsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBlacklistReports").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetBlacklistReportsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getBlacklistReportsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @return A Java Future containing the result of the GetConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetConfigurationSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture getConfigurationSet(
GetConfigurationSetRequest getConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetConfigurationSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getConfigurationSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* In Amazon Pinpoint, events include message sends, deliveries, opens, clicks, bounces, and complaints.
* Event destinations are places that you can send information about these events to. For example, you can
* send event data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use
* Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @return A Java Future containing the result of the GetConfigurationSetEventDestinations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
@Override
public CompletableFuture getConfigurationSetEventDestinations(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getConfigurationSetEventDestinationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getConfigurationSetEventDestinationsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetConfigurationSetEventDestinations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetConfigurationSetEventDestinationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetConfigurationSetEventDestinations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetConfigurationSetEventDestinationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getConfigurationSetEventDestinationsRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @return A Java Future containing the result of the GetDedicatedIp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDedicatedIp
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getDedicatedIp(GetDedicatedIpRequest getDedicatedIpRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDedicatedIpRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDedicatedIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDedicatedIp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDedicatedIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDedicatedIp").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDedicatedIpRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDedicatedIpRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the dedicated IP addresses that are associated with your Amazon Pinpoint account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @return A Java Future containing the result of the GetDedicatedIps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDedicatedIps
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDedicatedIps(GetDedicatedIpsRequest getDedicatedIpsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDedicatedIpsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDedicatedIpsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDedicatedIps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDedicatedIpsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDedicatedIps").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDedicatedIpsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDedicatedIpsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account. When the
* Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the
* domains that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Pinpoint account.
* When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and other
* metrics for the domains that you use to send email using Amazon Pinpoint. You also gain the ability to
* perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return A Java Future containing the result of the GetDeliverabilityDashboardOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
-
* LimitExceededException There are too many instances of the specified resource type.
-
* BadRequestException The input you provided is invalid.
- SdkException Base class for all
* exceptions that can be thrown by the SDK (both service and client). Can be used for catch all scenarios.
*
- SdkClientException If any client side error occurs such as an IO related failure, failure to
* get credentials, etc.
- PinpointEmailException Base class for all service exceptions. Unknown
* exceptions will be thrown as an instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDeliverabilityDashboardOptions(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeliverabilityDashboardOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getDeliverabilityDashboardOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeliverabilityDashboardOptions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetDeliverabilityDashboardOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDeliverabilityDashboardOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDeliverabilityDashboardOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDeliverabilityDashboardOptionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @return A Java Future containing the result of the GetDeliverabilityTestReport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDeliverabilityTestReport(
GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDeliverabilityTestReportRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeliverabilityTestReportRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeliverabilityTestReport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeliverabilityTestReportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDeliverabilityTestReport").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDeliverabilityTestReportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDeliverabilityTestReportRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @return A Java Future containing the result of the GetDomainDeliverabilityCampaign operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - NotFoundException The resource you attempted to access doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDomainDeliverabilityCampaign(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDomainDeliverabilityCampaignRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getDomainDeliverabilityCampaignRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDomainDeliverabilityCampaign");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDomainDeliverabilityCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDomainDeliverabilityCampaign").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDomainDeliverabilityCampaignRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDomainDeliverabilityCampaignRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @return A Java Future containing the result of the GetDomainStatisticsReport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetDomainStatisticsReport
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDomainStatisticsReport(
GetDomainStatisticsReportRequest getDomainStatisticsReportRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDomainStatisticsReportRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDomainStatisticsReportRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDomainStatisticsReport");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDomainStatisticsReportResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDomainStatisticsReport").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDomainStatisticsReportRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDomainStatisticsReportRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about a specific identity associated with your Amazon Pinpoint account, including the
* identity's verification status, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @return A Java Future containing the result of the GetEmailIdentity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.GetEmailIdentity
* @see AWS API Documentation
*/
@Override
public CompletableFuture getEmailIdentity(GetEmailIdentityRequest getEmailIdentityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getEmailIdentityRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getEmailIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetEmailIdentity");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetEmailIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetEmailIdentity").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetEmailIdentityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getEmailIdentityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List all of the configuration sets associated with your Amazon Pinpoint account in the current region.
*
*
* In Amazon Pinpoint, configuration sets are groups of rules that you can apply to the emails you send. You
* apply a configuration set to an email by including a reference to the configuration set in the headers of the
* email. When you apply a configuration set to an email, all of the rules in that configuration set are applied to
* the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon Pinpoint account in the current AWS
* Region.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListConfigurationSets
* @see AWS API Documentation
*/
@Override
public CompletableFuture listConfigurationSets(
ListConfigurationSetsRequest listConfigurationSetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConfigurationSetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConfigurationSetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConfigurationSets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListConfigurationSetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListConfigurationSets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListConfigurationSetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listConfigurationSetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List all of the dedicated IP pools that exist in your Amazon Pinpoint account in the current AWS Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @return A Java Future containing the result of the ListDedicatedIpPools operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListDedicatedIpPools
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDedicatedIpPools(
ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDedicatedIpPoolsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDedicatedIpPoolsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDedicatedIpPools");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDedicatedIpPoolsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDedicatedIpPools").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDedicatedIpPoolsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDedicatedIpPoolsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @return A Java Future containing the result of the ListDeliverabilityTestReports operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - NotFoundException The resource you attempted to access doesn't exist.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDeliverabilityTestReports(
ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeliverabilityTestReportsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listDeliverabilityTestReportsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeliverabilityTestReports");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeliverabilityTestReportsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDeliverabilityTestReports").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDeliverabilityTestReportsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDeliverabilityTestReportsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard (
* PutDeliverabilityDashboardOption
operation) for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability dashboard
* (PutDeliverabilityDashboardOption
operation) for the domain.
* @return A Java Future containing the result of the ListDomainDeliverabilityCampaigns operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - NotFoundException The resource you attempted to access doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDomainDeliverabilityCampaigns(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDomainDeliverabilityCampaignsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listDomainDeliverabilityCampaignsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDomainDeliverabilityCampaigns");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListDomainDeliverabilityCampaignsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDomainDeliverabilityCampaigns").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDomainDeliverabilityCampaignsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDomainDeliverabilityCampaignsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Pinpoint account. An identity
* can be either an email address or a domain. This operation returns identities that are verified as well as those
* that aren't.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Pinpoint account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @return A Java Future containing the result of the ListEmailIdentities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListEmailIdentities
* @see AWS API Documentation
*/
@Override
public CompletableFuture listEmailIdentities(
ListEmailIdentitiesRequest listEmailIdentitiesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEmailIdentitiesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEmailIdentitiesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEmailIdentities");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEmailIdentitiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEmailIdentities").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEmailIdentitiesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEmailIdentitiesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource in Amazon Pinpoint. Each tag consists of a
* required tag key and an optional associated tag value. A tag key is a general label that acts as a
* category for more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BadRequestException The input you provided is invalid.
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @return A Java Future containing the result of the PutAccountDedicatedIpWarmupAttributes operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture putAccountDedicatedIpWarmupAttributes(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAccountDedicatedIpWarmupAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putAccountDedicatedIpWarmupAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccountDedicatedIpWarmupAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutAccountDedicatedIpWarmupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccountDedicatedIpWarmupAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutAccountDedicatedIpWarmupAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putAccountDedicatedIpWarmupAttributesRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @return A Java Future containing the result of the PutAccountSendingAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutAccountSendingAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture putAccountSendingAttributes(
PutAccountSendingAttributesRequest putAccountSendingAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAccountSendingAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAccountSendingAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccountSendingAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAccountSendingAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccountSendingAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutAccountSendingAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putAccountSendingAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @return A Java Future containing the result of the PutConfigurationSetDeliveryOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture putConfigurationSetDeliveryOptions(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putConfigurationSetDeliveryOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putConfigurationSetDeliveryOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutConfigurationSetDeliveryOptions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutConfigurationSetDeliveryOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutConfigurationSetDeliveryOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutConfigurationSetDeliveryOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putConfigurationSetDeliveryOptionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific AWS Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetReputationOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture putConfigurationSetReputationOptions(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putConfigurationSetReputationOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putConfigurationSetReputationOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutConfigurationSetReputationOptions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutConfigurationSetReputationOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutConfigurationSetReputationOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutConfigurationSetReputationOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putConfigurationSetReputationOptionsRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific AWS Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon Pinpoint to send emails that use a specific
* configuration set.
* @return A Java Future containing the result of the PutConfigurationSetSendingOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture putConfigurationSetSendingOptions(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putConfigurationSetSendingOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putConfigurationSetSendingOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutConfigurationSetSendingOptions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutConfigurationSetSendingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutConfigurationSetSendingOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutConfigurationSetSendingOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putConfigurationSetSendingOptionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send using Amazon Pinpoint.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetTrackingOptions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture putConfigurationSetTrackingOptions(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putConfigurationSetTrackingOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putConfigurationSetTrackingOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutConfigurationSetTrackingOptions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutConfigurationSetTrackingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutConfigurationSetTrackingOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutConfigurationSetTrackingOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putConfigurationSetTrackingOptionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Pinpoint
* account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @return A Java Future containing the result of the PutDedicatedIpInPool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutDedicatedIpInPool
* @see AWS API Documentation
*/
@Override
public CompletableFuture putDedicatedIpInPool(
PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putDedicatedIpInPoolRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putDedicatedIpInPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutDedicatedIpInPool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutDedicatedIpInPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutDedicatedIpInPool").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutDedicatedIpInPoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putDedicatedIpInPoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @return A Java Future containing the result of the PutDedicatedIpWarmupAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
-
* TooManyRequestsException Too many requests have been made to the operation.
- BadRequestException
* The input you provided is invalid.
- SdkException Base class for all exceptions that can be thrown
* by the SDK (both service and client). Can be used for catch all scenarios.
- SdkClientException If
* any client side error occurs such as an IO related failure, failure to get credentials, etc.
-
* PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture putDedicatedIpWarmupAttributes(
PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putDedicatedIpWarmupAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putDedicatedIpWarmupAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutDedicatedIpWarmupAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutDedicatedIpWarmupAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutDedicatedIpWarmupAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutDedicatedIpWarmupAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putDedicatedIpWarmupAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains that
* you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox placement
* tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon Pinpoint. For more information about the features and cost of a Deliverability
* dashboard subscription, see Amazon Pinpoint Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard for your Amazon Pinpoint account. When you enable the
* Deliverability dashboard, you gain access to reputation, deliverability, and other metrics for the domains
* that you use to send email using Amazon Pinpoint. You also gain the ability to perform predictive inbox
* placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon Pinpoint. For more information about the features and cost of a
* Deliverability dashboard subscription, see Amazon
* Pinpoint Pricing.
* @return A Java Future containing the result of the PutDeliverabilityDashboardOption operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException The resource specified in your request already exists.
-
* NotFoundException The resource you attempted to access doesn't exist.
- TooManyRequestsException
* Too many requests have been made to the operation.
- LimitExceededException There are too many
* instances of the specified resource type.
- BadRequestException The input you provided is invalid.
*
- SdkException Base class for all exceptions that can be thrown by the SDK (both service and
* client). Can be used for catch all scenarios.
- SdkClientException If any client side error occurs
* such as an IO related failure, failure to get credentials, etc.
- PinpointEmailException Base
* class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
*
* @sample PinpointEmailAsyncClient.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
@Override
public CompletableFuture putDeliverabilityDashboardOption(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putDeliverabilityDashboardOptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putDeliverabilityDashboardOptionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutDeliverabilityDashboardOption");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, PutDeliverabilityDashboardOptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutDeliverabilityDashboardOption").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutDeliverabilityDashboardOptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putDeliverabilityDashboardOptionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @return A Java Future containing the result of the PutEmailIdentityDkimAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource you attempted to access doesn't exist.
* - TooManyRequestsException Too many requests have been made to the operation.
* - BadRequestException The input you provided is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - PinpointEmailException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample PinpointEmailAsyncClient.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture putEmailIdentityDkimAttributes(
PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putEmailIdentityDkimAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putEmailIdentityDkimAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Pinpoint Email");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutEmailIdentityDkimAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutEmailIdentityDkimAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams