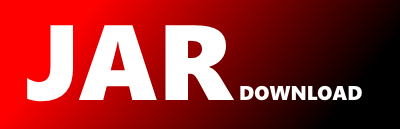
software.amazon.awssdk.services.protocolec2.DefaultProtocolEc2AsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protocol-tests Show documentation
Show all versions of protocol-tests Show documentation
Contains functional tests for all supported protocols.
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocolec2;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Node;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.http.response.DefaultErrorResponseHandler;
import software.amazon.awssdk.awscore.http.response.StaxResponseHandler;
import software.amazon.awssdk.awscore.protocol.xml.LegacyErrorUnmarshaller;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.runtime.transform.Unmarshaller;
import software.amazon.awssdk.services.protocolec2.model.AllTypesRequest;
import software.amazon.awssdk.services.protocolec2.model.AllTypesResponse;
import software.amazon.awssdk.services.protocolec2.model.Ec2TypesRequest;
import software.amazon.awssdk.services.protocolec2.model.Ec2TypesResponse;
import software.amazon.awssdk.services.protocolec2.model.IdempotentOperationRequest;
import software.amazon.awssdk.services.protocolec2.model.IdempotentOperationResponse;
import software.amazon.awssdk.services.protocolec2.model.ProtocolEc2Exception;
import software.amazon.awssdk.services.protocolec2.transform.AllTypesRequestMarshaller;
import software.amazon.awssdk.services.protocolec2.transform.AllTypesResponseUnmarshaller;
import software.amazon.awssdk.services.protocolec2.transform.Ec2TypesRequestMarshaller;
import software.amazon.awssdk.services.protocolec2.transform.Ec2TypesResponseUnmarshaller;
import software.amazon.awssdk.services.protocolec2.transform.IdempotentOperationRequestMarshaller;
import software.amazon.awssdk.services.protocolec2.transform.IdempotentOperationResponseUnmarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ProtocolEc2AsyncClient}.
*
* @see ProtocolEc2AsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultProtocolEc2AsyncClient implements ProtocolEc2AsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultProtocolEc2AsyncClient.class);
private final AsyncClientHandler clientHandler;
private final List> exceptionUnmarshallers;
protected DefaultProtocolEc2AsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.exceptionUnmarshallers = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Invokes the AllTypes operation asynchronously.
*
* @param allTypesRequest
* @return A Java Future containing the result of the AllTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolEc2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ProtocolEc2AsyncClient.AllTypes
*/
@Override
public CompletableFuture allTypes(AllTypesRequest allTypesRequest) {
try {
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AllTypesResponseUnmarshaller());
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new AllTypesRequestMarshaller()).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(allTypesRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the Ec2Types operation asynchronously.
*
* @param ec2TypesRequest
* @return A Java Future containing the result of the Ec2Types operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolEc2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ProtocolEc2AsyncClient.Ec2Types
*/
@Override
public CompletableFuture ec2Types(Ec2TypesRequest ec2TypesRequest) {
try {
StaxResponseHandler responseHandler = new StaxResponseHandler(
new Ec2TypesResponseUnmarshaller());
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new Ec2TypesRequestMarshaller()).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(ec2TypesRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the IdempotentOperation operation asynchronously.
*
* @param idempotentOperationRequest
* @return A Java Future containing the result of the IdempotentOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolEc2Exception Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ProtocolEc2AsyncClient.IdempotentOperation
*/
@Override
public CompletableFuture idempotentOperation(
IdempotentOperationRequest idempotentOperationRequest) {
try {
StaxResponseHandler responseHandler = new StaxResponseHandler(
new IdempotentOperationResponseUnmarshaller());
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new IdempotentOperationRequestMarshaller()).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(idempotentOperationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private List> init() {
List> unmarshallers = new ArrayList<>();
unmarshallers.add(new LegacyErrorUnmarshaller(ProtocolEc2Exception.class));
return unmarshallers;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy