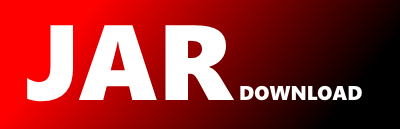
software.amazon.awssdk.services.protocoljsonrpc.DefaultProtocolJsonRpcAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protocol-tests Show documentation
Show all versions of protocol-tests Show documentation
Contains functional tests for all supported protocols.
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocoljsonrpc;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.protocoljsonrpc.model.AllTypesRequest;
import software.amazon.awssdk.services.protocoljsonrpc.model.AllTypesResponse;
import software.amazon.awssdk.services.protocoljsonrpc.model.EmptyModeledException;
import software.amazon.awssdk.services.protocoljsonrpc.model.IdempotentOperationRequest;
import software.amazon.awssdk.services.protocoljsonrpc.model.IdempotentOperationResponse;
import software.amazon.awssdk.services.protocoljsonrpc.model.NestedContainersRequest;
import software.amazon.awssdk.services.protocoljsonrpc.model.NestedContainersResponse;
import software.amazon.awssdk.services.protocoljsonrpc.model.OperationWithNoInputOrOutputRequest;
import software.amazon.awssdk.services.protocoljsonrpc.model.OperationWithNoInputOrOutputResponse;
import software.amazon.awssdk.services.protocoljsonrpc.transform.AllTypesRequestMarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.AllTypesResponseUnmarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.IdempotentOperationRequestMarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.IdempotentOperationResponseUnmarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.NestedContainersRequestMarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.NestedContainersResponseUnmarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.OperationWithNoInputOrOutputRequestMarshaller;
import software.amazon.awssdk.services.protocoljsonrpc.transform.OperationWithNoInputOrOutputResponseUnmarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ProtocolJsonRpcAsyncClient}.
*
* @see ProtocolJsonRpcAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultProtocolJsonRpcAsyncClient implements ProtocolJsonRpcAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultProtocolJsonRpcAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
protected DefaultProtocolJsonRpcAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.protocolFactory = init(false);
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Invokes the AllTypes operation asynchronously.
*
* @param allTypesRequest
* @return A Java Future containing the result of the AllTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolJsonRpcException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolJsonRpcAsyncClient.AllTypes
*/
@Override
public CompletableFuture allTypes(AllTypesRequest allTypesRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AllTypesResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new AllTypesRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(allTypesRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the IdempotentOperation operation asynchronously.
*
* @param idempotentOperationRequest
* @return A Java Future containing the result of the IdempotentOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolJsonRpcException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolJsonRpcAsyncClient.IdempotentOperation
*/
@Override
public CompletableFuture idempotentOperation(
IdempotentOperationRequest idempotentOperationRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new IdempotentOperationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new IdempotentOperationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(idempotentOperationRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the NestedContainers operation asynchronously.
*
* @param nestedContainersRequest
* @return A Java Future containing the result of the NestedContainers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolJsonRpcException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolJsonRpcAsyncClient.NestedContainers
*/
@Override
public CompletableFuture nestedContainers(NestedContainersRequest nestedContainersRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new NestedContainersResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory);
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new NestedContainersRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(nestedContainersRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
* Invokes the OperationWithNoInputOrOutput operation asynchronously.
*
* @param operationWithNoInputOrOutputRequest
* @return A Java Future containing the result of the OperationWithNoInputOrOutput operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolJsonRpcException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolJsonRpcAsyncClient.OperationWithNoInputOrOutput
*/
@Override
public CompletableFuture operationWithNoInputOrOutput(
OperationWithNoInputOrOutputRequest operationWithNoInputOrOutputRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new OperationWithNoInputOrOutputResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory);
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new OperationWithNoInputOrOutputRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(operationWithNoInputOrOutputRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init(boolean supportsCbor) {
return new AwsJsonProtocolFactory(new JsonClientMetadata()
.withSupportsCbor(supportsCbor)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.protocoljsonrpc.model.ProtocolJsonRpcException.class)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EmptyModeledException").withModeledClass(
EmptyModeledException.class)), AwsJsonProtocolMetadata.builder().protocolVersion("1.1")
.protocol(AwsJsonProtocol.AWS_JSON).build());
}
private HttpResponseHandler createErrorResponseHandler(AwsJsonProtocolFactory protocolFactory) {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy