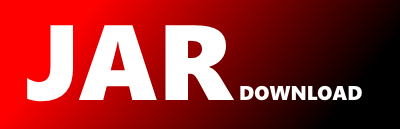
software.amazon.awssdk.services.protocoljsonrpccustomized.DefaultProtocolJsonRpcCustomizedClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protocol-tests Show documentation
Show all versions of protocol-tests Show documentation
Contains functional tests for all supported protocols.
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocoljsonrpccustomized;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.protocoljsonrpccustomized.model.ProtocolJsonRpcCustomizedException;
import software.amazon.awssdk.services.protocoljsonrpccustomized.model.SimpleRequest;
import software.amazon.awssdk.services.protocoljsonrpccustomized.model.SimpleResponse;
import software.amazon.awssdk.services.protocoljsonrpccustomized.transform.SimpleRequestMarshaller;
import software.amazon.awssdk.services.protocoljsonrpccustomized.transform.SimpleResponseUnmarshaller;
/**
* Internal implementation of {@link ProtocolJsonRpcCustomizedClient}.
*
* @see ProtocolJsonRpcCustomizedClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultProtocolJsonRpcCustomizedClient implements ProtocolJsonRpcCustomizedClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultProtocolJsonRpcCustomizedClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(false);
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Invokes the Simple operation.
*
* @param simpleRequest
* @return Result of the Simple operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolJsonRpcCustomizedException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolJsonRpcCustomizedClient.Simple
*/
@Override
public SimpleResponse simple(SimpleRequest simpleRequest) throws AwsServiceException, SdkClientException,
ProtocolJsonRpcCustomizedException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new SimpleResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory);
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(simpleRequest)
.withMarshaller(new SimpleRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler(AwsJsonProtocolFactory protocolFactory) {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init(boolean supportsCbor) {
return new AwsJsonProtocolFactory(
new JsonClientMetadata()
.withSupportsCbor(supportsCbor)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.protocoljsonrpccustomized.model.ProtocolJsonRpcCustomizedException.class),
AwsJsonProtocolMetadata.builder().protocolVersion("1.1").protocol(AwsJsonProtocol.AWS_JSON).build());
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy