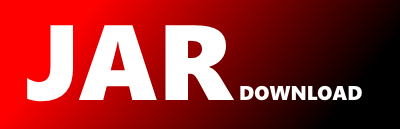
software.amazon.awssdk.services.protocolrestjson.ProtocolRestJsonAsyncClient Maven / Gradle / Ivy
Show all versions of protocol-tests Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocolrestjson;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.protocolrestjson.model.AllTypesRequest;
import software.amazon.awssdk.services.protocolrestjson.model.AllTypesResponse;
import software.amazon.awssdk.services.protocolrestjson.model.DeleteOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.DeleteOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.HeadOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.HeadOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.IdempotentOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.IdempotentOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.JsonValuesOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.JsonValuesOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.MapOfStringToListOfStringInQueryParamsRequest;
import software.amazon.awssdk.services.protocolrestjson.model.MapOfStringToListOfStringInQueryParamsResponse;
import software.amazon.awssdk.services.protocolrestjson.model.MembersInHeadersRequest;
import software.amazon.awssdk.services.protocolrestjson.model.MembersInHeadersResponse;
import software.amazon.awssdk.services.protocolrestjson.model.MembersInQueryParamsRequest;
import software.amazon.awssdk.services.protocolrestjson.model.MembersInQueryParamsResponse;
import software.amazon.awssdk.services.protocolrestjson.model.MultiLocationOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.MultiLocationOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.NestedContainersRequest;
import software.amazon.awssdk.services.protocolrestjson.model.NestedContainersResponse;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithExplicitPayloadBlobRequest;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithExplicitPayloadBlobResponse;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithExplicitPayloadStructureRequest;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithExplicitPayloadStructureResponse;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithGreedyLabelRequest;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithGreedyLabelResponse;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithModeledContentTypeRequest;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithModeledContentTypeResponse;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithNoInputOrOutputRequest;
import software.amazon.awssdk.services.protocolrestjson.model.OperationWithNoInputOrOutputResponse;
import software.amazon.awssdk.services.protocolrestjson.model.QueryParamWithoutValueRequest;
import software.amazon.awssdk.services.protocolrestjson.model.QueryParamWithoutValueResponse;
import software.amazon.awssdk.services.protocolrestjson.model.StreamingInputOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.StreamingInputOperationResponse;
import software.amazon.awssdk.services.protocolrestjson.model.StreamingOutputOperationRequest;
import software.amazon.awssdk.services.protocolrestjson.model.StreamingOutputOperationResponse;
/**
* Service client for accessing AmazonProtocolRestJson asynchronously. This can be created using the static
* {@link #builder()} method.
*
* null
*/
@Generated("software.amazon.awssdk:codegen")
public interface ProtocolRestJsonAsyncClient extends SdkClient {
String SERVICE_NAME = "restjson";
/**
* Create a {@link ProtocolRestJsonAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ProtocolRestJsonAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ProtocolRestJsonAsyncClient}.
*/
static ProtocolRestJsonAsyncClientBuilder builder() {
return new DefaultProtocolRestJsonAsyncClientBuilder();
}
/**
* Invokes the AllTypes operation asynchronously.
*
* @param allTypesRequest
* @return A Java Future containing the result of the AllTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.AllTypes
* @see AWS API
* Documentation
*/
default CompletableFuture allTypes(AllTypesRequest allTypesRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the AllTypes operation asynchronously.
*
* This is a convenience which creates an instance of the {@link AllTypesRequest.Builder} avoiding the need to
* create one manually via {@link AllTypesRequest#builder()}
*
*
* @param allTypesRequest
* A {@link Consumer} that will call methods on {@link AllTypesStructure.Builder} to create a request.
* @return A Java Future containing the result of the AllTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.AllTypes
* @see AWS API
* Documentation
*/
default CompletableFuture allTypes(Consumer allTypesRequest) {
return allTypes(AllTypesRequest.builder().applyMutation(allTypesRequest).build());
}
/**
* Invokes the AllTypes operation asynchronously.
*
* @return A Java Future containing the result of the AllTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.AllTypes
* @see AWS API
* Documentation
*/
default CompletableFuture allTypes() {
return allTypes(AllTypesRequest.builder().build());
}
/**
* Invokes the DeleteOperation operation asynchronously.
*
* @param deleteOperationRequest
* @return A Java Future containing the result of the DeleteOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.DeleteOperation
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOperation(DeleteOperationRequest deleteOperationRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the DeleteOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link DeleteOperationRequest.Builder} avoiding the need
* to create one manually via {@link DeleteOperationRequest#builder()}
*
*
* @param deleteOperationRequest
* A {@link Consumer} that will call methods on {@link DeleteOperationRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.DeleteOperation
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOperation(
Consumer deleteOperationRequest) {
return deleteOperation(DeleteOperationRequest.builder().applyMutation(deleteOperationRequest).build());
}
/**
* Invokes the DeleteOperation operation asynchronously.
*
* @return A Java Future containing the result of the DeleteOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.DeleteOperation
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOperation() {
return deleteOperation(DeleteOperationRequest.builder().build());
}
/**
* Invokes the HeadOperation operation asynchronously.
*
* @param headOperationRequest
* @return A Java Future containing the result of the HeadOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.HeadOperation
* @see AWS API
* Documentation
*/
default CompletableFuture headOperation(HeadOperationRequest headOperationRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the HeadOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link HeadOperationRequest.Builder} avoiding the need to
* create one manually via {@link HeadOperationRequest#builder()}
*
*
* @param headOperationRequest
* A {@link Consumer} that will call methods on {@link HeadOperationRequest.Builder} to create a request.
* @return A Java Future containing the result of the HeadOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.HeadOperation
* @see AWS API
* Documentation
*/
default CompletableFuture headOperation(Consumer headOperationRequest) {
return headOperation(HeadOperationRequest.builder().applyMutation(headOperationRequest).build());
}
/**
* Invokes the HeadOperation operation asynchronously.
*
* @return A Java Future containing the result of the HeadOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.HeadOperation
* @see AWS API
* Documentation
*/
default CompletableFuture headOperation() {
return headOperation(HeadOperationRequest.builder().build());
}
/**
* Invokes the IdempotentOperation operation asynchronously.
*
* @param idempotentOperationRequest
* @return A Java Future containing the result of the IdempotentOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.IdempotentOperation
* @see AWS
* API Documentation
*/
default CompletableFuture idempotentOperation(
IdempotentOperationRequest idempotentOperationRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the IdempotentOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link IdempotentOperationRequest.Builder} avoiding the
* need to create one manually via {@link IdempotentOperationRequest#builder()}
*
*
* @param idempotentOperationRequest
* A {@link Consumer} that will call methods on {@link IdempotentOperationStructure.Builder} to create a
* request.
* @return A Java Future containing the result of the IdempotentOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.IdempotentOperation
* @see AWS
* API Documentation
*/
default CompletableFuture idempotentOperation(
Consumer idempotentOperationRequest) {
return idempotentOperation(IdempotentOperationRequest.builder().applyMutation(idempotentOperationRequest).build());
}
/**
* Invokes the JsonValuesOperation operation asynchronously.
*
* @param jsonValuesOperationRequest
* @return A Java Future containing the result of the JsonValuesOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.JsonValuesOperation
* @see AWS
* API Documentation
*/
default CompletableFuture jsonValuesOperation(
JsonValuesOperationRequest jsonValuesOperationRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the JsonValuesOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link JsonValuesOperationRequest.Builder} avoiding the
* need to create one manually via {@link JsonValuesOperationRequest#builder()}
*
*
* @param jsonValuesOperationRequest
* A {@link Consumer} that will call methods on {@link JsonValuesStructure.Builder} to create a request.
* @return A Java Future containing the result of the JsonValuesOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.JsonValuesOperation
* @see AWS
* API Documentation
*/
default CompletableFuture jsonValuesOperation(
Consumer jsonValuesOperationRequest) {
return jsonValuesOperation(JsonValuesOperationRequest.builder().applyMutation(jsonValuesOperationRequest).build());
}
/**
* Invokes the JsonValuesOperation operation asynchronously.
*
* @return A Java Future containing the result of the JsonValuesOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EmptyModeledException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.JsonValuesOperation
* @see AWS
* API Documentation
*/
default CompletableFuture jsonValuesOperation() {
return jsonValuesOperation(JsonValuesOperationRequest.builder().build());
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation asynchronously.
*
* @param mapOfStringToListOfStringInQueryParamsRequest
* @return A Java Future containing the result of the MapOfStringToListOfStringInQueryParams operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MapOfStringToListOfStringInQueryParams
* @see AWS API Documentation
*/
default CompletableFuture mapOfStringToListOfStringInQueryParams(
MapOfStringToListOfStringInQueryParamsRequest mapOfStringToListOfStringInQueryParamsRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation asynchronously.
*
* This is a convenience which creates an instance of the
* {@link MapOfStringToListOfStringInQueryParamsRequest.Builder} avoiding the need to create one manually via
* {@link MapOfStringToListOfStringInQueryParamsRequest#builder()}
*
*
* @param mapOfStringToListOfStringInQueryParamsRequest
* A {@link Consumer} that will call methods on {@link MapOfStringToListOfStringInQueryParamsInput.Builder}
* to create a request.
* @return A Java Future containing the result of the MapOfStringToListOfStringInQueryParams operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MapOfStringToListOfStringInQueryParams
* @see AWS API Documentation
*/
default CompletableFuture mapOfStringToListOfStringInQueryParams(
Consumer mapOfStringToListOfStringInQueryParamsRequest) {
return mapOfStringToListOfStringInQueryParams(MapOfStringToListOfStringInQueryParamsRequest.builder()
.applyMutation(mapOfStringToListOfStringInQueryParamsRequest).build());
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation asynchronously.
*
* @return A Java Future containing the result of the MapOfStringToListOfStringInQueryParams operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MapOfStringToListOfStringInQueryParams
* @see AWS API Documentation
*/
default CompletableFuture mapOfStringToListOfStringInQueryParams() {
return mapOfStringToListOfStringInQueryParams(MapOfStringToListOfStringInQueryParamsRequest.builder().build());
}
/**
* Invokes the MembersInHeaders operation asynchronously.
*
* @param membersInHeadersRequest
* @return A Java Future containing the result of the MembersInHeaders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInHeaders
* @see AWS API
* Documentation
*/
default CompletableFuture membersInHeaders(MembersInHeadersRequest membersInHeadersRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the MembersInHeaders operation asynchronously.
*
* This is a convenience which creates an instance of the {@link MembersInHeadersRequest.Builder} avoiding the need
* to create one manually via {@link MembersInHeadersRequest#builder()}
*
*
* @param membersInHeadersRequest
* A {@link Consumer} that will call methods on {@link MembersInHeadersInput.Builder} to create a request.
* @return A Java Future containing the result of the MembersInHeaders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInHeaders
* @see AWS API
* Documentation
*/
default CompletableFuture membersInHeaders(
Consumer membersInHeadersRequest) {
return membersInHeaders(MembersInHeadersRequest.builder().applyMutation(membersInHeadersRequest).build());
}
/**
* Invokes the MembersInHeaders operation asynchronously.
*
* @return A Java Future containing the result of the MembersInHeaders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInHeaders
* @see AWS API
* Documentation
*/
default CompletableFuture membersInHeaders() {
return membersInHeaders(MembersInHeadersRequest.builder().build());
}
/**
* Invokes the MembersInQueryParams operation asynchronously.
*
* @param membersInQueryParamsRequest
* @return A Java Future containing the result of the MembersInQueryParams operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInQueryParams
* @see AWS
* API Documentation
*/
default CompletableFuture membersInQueryParams(
MembersInQueryParamsRequest membersInQueryParamsRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the MembersInQueryParams operation asynchronously.
*
* This is a convenience which creates an instance of the {@link MembersInQueryParamsRequest.Builder} avoiding the
* need to create one manually via {@link MembersInQueryParamsRequest#builder()}
*
*
* @param membersInQueryParamsRequest
* A {@link Consumer} that will call methods on {@link MembersInQueryParamsInput.Builder} to create a
* request.
* @return A Java Future containing the result of the MembersInQueryParams operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInQueryParams
* @see AWS
* API Documentation
*/
default CompletableFuture membersInQueryParams(
Consumer membersInQueryParamsRequest) {
return membersInQueryParams(MembersInQueryParamsRequest.builder().applyMutation(membersInQueryParamsRequest).build());
}
/**
* Invokes the MembersInQueryParams operation asynchronously.
*
* @return A Java Future containing the result of the MembersInQueryParams operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MembersInQueryParams
* @see AWS
* API Documentation
*/
default CompletableFuture membersInQueryParams() {
return membersInQueryParams(MembersInQueryParamsRequest.builder().build());
}
/**
* Invokes the MultiLocationOperation operation asynchronously.
*
* @param multiLocationOperationRequest
* @return A Java Future containing the result of the MultiLocationOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MultiLocationOperation
* @see AWS API Documentation
*/
default CompletableFuture multiLocationOperation(
MultiLocationOperationRequest multiLocationOperationRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the MultiLocationOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link MultiLocationOperationRequest.Builder} avoiding the
* need to create one manually via {@link MultiLocationOperationRequest#builder()}
*
*
* @param multiLocationOperationRequest
* A {@link Consumer} that will call methods on {@link MultiLocationOperationInput.Builder} to create a
* request.
* @return A Java Future containing the result of the MultiLocationOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.MultiLocationOperation
* @see AWS API Documentation
*/
default CompletableFuture multiLocationOperation(
Consumer multiLocationOperationRequest) {
return multiLocationOperation(MultiLocationOperationRequest.builder().applyMutation(multiLocationOperationRequest)
.build());
}
/**
* Invokes the NestedContainers operation asynchronously.
*
* @param nestedContainersRequest
* @return A Java Future containing the result of the NestedContainers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.NestedContainers
* @see AWS API
* Documentation
*/
default CompletableFuture nestedContainers(NestedContainersRequest nestedContainersRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the NestedContainers operation asynchronously.
*
* This is a convenience which creates an instance of the {@link NestedContainersRequest.Builder} avoiding the need
* to create one manually via {@link NestedContainersRequest#builder()}
*
*
* @param nestedContainersRequest
* A {@link Consumer} that will call methods on {@link NestedContainersStructure.Builder} to create a
* request.
* @return A Java Future containing the result of the NestedContainers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.NestedContainers
* @see AWS API
* Documentation
*/
default CompletableFuture nestedContainers(
Consumer nestedContainersRequest) {
return nestedContainers(NestedContainersRequest.builder().applyMutation(nestedContainersRequest).build());
}
/**
* Invokes the NestedContainers operation asynchronously.
*
* @return A Java Future containing the result of the NestedContainers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.NestedContainers
* @see AWS API
* Documentation
*/
default CompletableFuture nestedContainers() {
return nestedContainers(NestedContainersRequest.builder().build());
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation asynchronously.
*
* @param operationWithExplicitPayloadBlobRequest
* @return A Java Future containing the result of the OperationWithExplicitPayloadBlob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadBlob
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadBlob(
OperationWithExplicitPayloadBlobRequest operationWithExplicitPayloadBlobRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation asynchronously.
*
* This is a convenience which creates an instance of the {@link OperationWithExplicitPayloadBlobRequest.Builder}
* avoiding the need to create one manually via {@link OperationWithExplicitPayloadBlobRequest#builder()}
*
*
* @param operationWithExplicitPayloadBlobRequest
* A {@link Consumer} that will call methods on {@link OperationWithExplicitPayloadBlobInput.Builder} to
* create a request.
* @return A Java Future containing the result of the OperationWithExplicitPayloadBlob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadBlob
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadBlob(
Consumer operationWithExplicitPayloadBlobRequest) {
return operationWithExplicitPayloadBlob(OperationWithExplicitPayloadBlobRequest.builder()
.applyMutation(operationWithExplicitPayloadBlobRequest).build());
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation asynchronously.
*
* @return A Java Future containing the result of the OperationWithExplicitPayloadBlob operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadBlob
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadBlob() {
return operationWithExplicitPayloadBlob(OperationWithExplicitPayloadBlobRequest.builder().build());
}
/**
* Invokes the OperationWithExplicitPayloadStructure operation asynchronously.
*
* @param operationWithExplicitPayloadStructureRequest
* @return A Java Future containing the result of the OperationWithExplicitPayloadStructure operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadStructure
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadStructure(
OperationWithExplicitPayloadStructureRequest operationWithExplicitPayloadStructureRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithExplicitPayloadStructure operation asynchronously.
*
* This is a convenience which creates an instance of the
* {@link OperationWithExplicitPayloadStructureRequest.Builder} avoiding the need to create one manually via
* {@link OperationWithExplicitPayloadStructureRequest#builder()}
*
*
* @param operationWithExplicitPayloadStructureRequest
* A {@link Consumer} that will call methods on {@link OperationWithExplicitPayloadStructureInput.Builder} to
* create a request.
* @return A Java Future containing the result of the OperationWithExplicitPayloadStructure operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadStructure
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadStructure(
Consumer operationWithExplicitPayloadStructureRequest) {
return operationWithExplicitPayloadStructure(OperationWithExplicitPayloadStructureRequest.builder()
.applyMutation(operationWithExplicitPayloadStructureRequest).build());
}
/**
* Invokes the OperationWithExplicitPayloadStructure operation asynchronously.
*
* @return A Java Future containing the result of the OperationWithExplicitPayloadStructure operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithExplicitPayloadStructure
* @see AWS API Documentation
*/
default CompletableFuture operationWithExplicitPayloadStructure() {
return operationWithExplicitPayloadStructure(OperationWithExplicitPayloadStructureRequest.builder().build());
}
/**
* Invokes the OperationWithGreedyLabel operation asynchronously.
*
* @param operationWithGreedyLabelRequest
* @return A Java Future containing the result of the OperationWithGreedyLabel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithGreedyLabel
* @see AWS API Documentation
*/
default CompletableFuture operationWithGreedyLabel(
OperationWithGreedyLabelRequest operationWithGreedyLabelRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithGreedyLabel operation asynchronously.
*
* This is a convenience which creates an instance of the {@link OperationWithGreedyLabelRequest.Builder} avoiding
* the need to create one manually via {@link OperationWithGreedyLabelRequest#builder()}
*
*
* @param operationWithGreedyLabelRequest
* A {@link Consumer} that will call methods on {@link OperationWithGreedyLabelInput.Builder} to create a
* request.
* @return A Java Future containing the result of the OperationWithGreedyLabel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithGreedyLabel
* @see AWS API Documentation
*/
default CompletableFuture operationWithGreedyLabel(
Consumer operationWithGreedyLabelRequest) {
return operationWithGreedyLabel(OperationWithGreedyLabelRequest.builder().applyMutation(operationWithGreedyLabelRequest)
.build());
}
/**
* Invokes the OperationWithModeledContentType operation asynchronously.
*
* @param operationWithModeledContentTypeRequest
* @return A Java Future containing the result of the OperationWithModeledContentType operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithModeledContentType
* @see AWS API Documentation
*/
default CompletableFuture operationWithModeledContentType(
OperationWithModeledContentTypeRequest operationWithModeledContentTypeRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithModeledContentType operation asynchronously.
*
* This is a convenience which creates an instance of the {@link OperationWithModeledContentTypeRequest.Builder}
* avoiding the need to create one manually via {@link OperationWithModeledContentTypeRequest#builder()}
*
*
* @param operationWithModeledContentTypeRequest
* A {@link Consumer} that will call methods on {@link OperationWithModeledContentTypeInput.Builder} to
* create a request.
* @return A Java Future containing the result of the OperationWithModeledContentType operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithModeledContentType
* @see AWS API Documentation
*/
default CompletableFuture operationWithModeledContentType(
Consumer operationWithModeledContentTypeRequest) {
return operationWithModeledContentType(OperationWithModeledContentTypeRequest.builder()
.applyMutation(operationWithModeledContentTypeRequest).build());
}
/**
* Invokes the OperationWithModeledContentType operation asynchronously.
*
* @return A Java Future containing the result of the OperationWithModeledContentType operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithModeledContentType
* @see AWS API Documentation
*/
default CompletableFuture operationWithModeledContentType() {
return operationWithModeledContentType(OperationWithModeledContentTypeRequest.builder().build());
}
/**
* Invokes the OperationWithNoInputOrOutput operation asynchronously.
*
* @param operationWithNoInputOrOutputRequest
* @return A Java Future containing the result of the OperationWithNoInputOrOutput operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithNoInputOrOutput
* @see AWS API Documentation
*/
default CompletableFuture operationWithNoInputOrOutput(
OperationWithNoInputOrOutputRequest operationWithNoInputOrOutputRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithNoInputOrOutput operation asynchronously.
*
* This is a convenience which creates an instance of the {@link OperationWithNoInputOrOutputRequest.Builder}
* avoiding the need to create one manually via {@link OperationWithNoInputOrOutputRequest#builder()}
*
*
* @param operationWithNoInputOrOutputRequest
* A {@link Consumer} that will call methods on {@link OperationWithNoInputOrOutputRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the OperationWithNoInputOrOutput operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithNoInputOrOutput
* @see AWS API Documentation
*/
default CompletableFuture operationWithNoInputOrOutput(
Consumer operationWithNoInputOrOutputRequest) {
return operationWithNoInputOrOutput(OperationWithNoInputOrOutputRequest.builder()
.applyMutation(operationWithNoInputOrOutputRequest).build());
}
/**
* Invokes the OperationWithNoInputOrOutput operation asynchronously.
*
* @return A Java Future containing the result of the OperationWithNoInputOrOutput operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.OperationWithNoInputOrOutput
* @see AWS API Documentation
*/
default CompletableFuture operationWithNoInputOrOutput() {
return operationWithNoInputOrOutput(OperationWithNoInputOrOutputRequest.builder().build());
}
/**
* Invokes the QueryParamWithoutValue operation asynchronously.
*
* @param queryParamWithoutValueRequest
* @return A Java Future containing the result of the QueryParamWithoutValue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.QueryParamWithoutValue
* @see AWS API Documentation
*/
default CompletableFuture queryParamWithoutValue(
QueryParamWithoutValueRequest queryParamWithoutValueRequest) {
throw new UnsupportedOperationException();
}
/**
* Invokes the QueryParamWithoutValue operation asynchronously.
*
* This is a convenience which creates an instance of the {@link QueryParamWithoutValueRequest.Builder} avoiding the
* need to create one manually via {@link QueryParamWithoutValueRequest#builder()}
*
*
* @param queryParamWithoutValueRequest
* A {@link Consumer} that will call methods on {@link QueryParamWithoutValueInput.Builder} to create a
* request.
* @return A Java Future containing the result of the QueryParamWithoutValue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.QueryParamWithoutValue
* @see AWS API Documentation
*/
default CompletableFuture queryParamWithoutValue(
Consumer queryParamWithoutValueRequest) {
return queryParamWithoutValue(QueryParamWithoutValueRequest.builder().applyMutation(queryParamWithoutValueRequest)
.build());
}
/**
* Invokes the QueryParamWithoutValue operation asynchronously.
*
* @return A Java Future containing the result of the QueryParamWithoutValue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.QueryParamWithoutValue
* @see AWS API Documentation
*/
default CompletableFuture queryParamWithoutValue() {
return queryParamWithoutValue(QueryParamWithoutValueRequest.builder().build());
}
/**
* Invokes the StreamingInputOperation operation asynchronously.
*
* @param streamingInputOperationRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows ''
* @return A Java Future containing the result of the StreamingInputOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingInputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingInputOperation(
StreamingInputOperationRequest streamingInputOperationRequest, AsyncRequestBody requestBody) {
throw new UnsupportedOperationException();
}
/**
* Invokes the StreamingInputOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link StreamingInputOperationRequest.Builder} avoiding
* the need to create one manually via {@link StreamingInputOperationRequest#builder()}
*
*
* @param streamingInputOperationRequest
* A {@link Consumer} that will call methods on {@link StructureWithStreamingMember.Builder} to create a
* request.
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows ''
* @return A Java Future containing the result of the StreamingInputOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingInputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingInputOperation(
Consumer streamingInputOperationRequest, AsyncRequestBody requestBody) {
return streamingInputOperation(StreamingInputOperationRequest.builder().applyMutation(streamingInputOperationRequest)
.build(), requestBody);
}
/**
* Invokes the StreamingInputOperation operation asynchronously.
*
* @param streamingInputOperationRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows ''
* @return A Java Future containing the result of the StreamingInputOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingInputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingInputOperation(
StreamingInputOperationRequest streamingInputOperationRequest, Path sourcePath) {
return streamingInputOperation(streamingInputOperationRequest, AsyncRequestBody.fromFile(sourcePath));
}
/**
* Invokes the StreamingInputOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link StreamingInputOperationRequest.Builder} avoiding
* the need to create one manually via {@link StreamingInputOperationRequest#builder()}
*
*
* @param streamingInputOperationRequest
* A {@link Consumer} that will call methods on {@link StructureWithStreamingMember.Builder} to create a
* request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows ''
* @return A Java Future containing the result of the StreamingInputOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingInputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingInputOperation(
Consumer streamingInputOperationRequest, Path sourcePath) {
return streamingInputOperation(StreamingInputOperationRequest.builder().applyMutation(streamingInputOperationRequest)
.build(), sourcePath);
}
/**
* Invokes the StreamingOutputOperation operation asynchronously.
*
* @param streamingOutputOperationRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows ''.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingOutputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingOutputOperation(
StreamingOutputOperationRequest streamingOutputOperationRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
* Invokes the StreamingOutputOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link StreamingOutputOperationRequest.Builder} avoiding
* the need to create one manually via {@link StreamingOutputOperationRequest#builder()}
*
*
* @param streamingOutputOperationRequest
* A {@link Consumer} that will call methods on {@link StreamingOutputOperationRequest.Builder} to create a
* request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows ''.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingOutputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingOutputOperation(
Consumer streamingOutputOperationRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return streamingOutputOperation(StreamingOutputOperationRequest.builder().applyMutation(streamingOutputOperationRequest)
.build(), asyncResponseTransformer);
}
/**
* Invokes the StreamingOutputOperation operation asynchronously.
*
* @param streamingOutputOperationRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows ''.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingOutputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingOutputOperation(
StreamingOutputOperationRequest streamingOutputOperationRequest, Path destinationPath) {
return streamingOutputOperation(streamingOutputOperationRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
* Invokes the StreamingOutputOperation operation asynchronously.
*
* This is a convenience which creates an instance of the {@link StreamingOutputOperationRequest.Builder} avoiding
* the need to create one manually via {@link StreamingOutputOperationRequest#builder()}
*
*
* @param streamingOutputOperationRequest
* A {@link Consumer} that will call methods on {@link StreamingOutputOperationRequest.Builder} to create a
* request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows ''.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ProtocolRestJsonException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample ProtocolRestJsonAsyncClient.StreamingOutputOperation
* @see AWS API Documentation
*/
default CompletableFuture streamingOutputOperation(
Consumer streamingOutputOperationRequest, Path destinationPath) {
return streamingOutputOperation(StreamingOutputOperationRequest.builder().applyMutation(streamingOutputOperationRequest)
.build(), destinationPath);
}
}