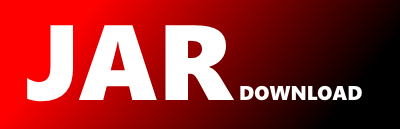
software.amazon.awssdk.services.protocolrestjson.model.MultiLocationOperationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protocol-tests Show documentation
Show all versions of protocol-tests Show documentation
Contains functional tests for all supported protocols.
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocolrestjson.model;
import java.time.Instant;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class MultiLocationOperationRequest extends ProtocolRestJsonRequest implements
ToCopyableBuilder {
private final String pathParam;
private final String queryParamOne;
private final String queryParamTwo;
private final String stringHeaderMember;
private final Instant timestampHeaderMember;
private final PayloadStructType payloadStructParam;
private MultiLocationOperationRequest(BuilderImpl builder) {
super(builder);
this.pathParam = builder.pathParam;
this.queryParamOne = builder.queryParamOne;
this.queryParamTwo = builder.queryParamTwo;
this.stringHeaderMember = builder.stringHeaderMember;
this.timestampHeaderMember = builder.timestampHeaderMember;
this.payloadStructParam = builder.payloadStructParam;
}
/**
* Returns the value of the PathParam property for this object.
*
* @return The value of the PathParam property for this object.
*/
public String pathParam() {
return pathParam;
}
/**
* Returns the value of the QueryParamOne property for this object.
*
* @return The value of the QueryParamOne property for this object.
*/
public String queryParamOne() {
return queryParamOne;
}
/**
* Returns the value of the QueryParamTwo property for this object.
*
* @return The value of the QueryParamTwo property for this object.
*/
public String queryParamTwo() {
return queryParamTwo;
}
/**
* Returns the value of the StringHeaderMember property for this object.
*
* @return The value of the StringHeaderMember property for this object.
*/
public String stringHeaderMember() {
return stringHeaderMember;
}
/**
* Returns the value of the TimestampHeaderMember property for this object.
*
* @return The value of the TimestampHeaderMember property for this object.
*/
public Instant timestampHeaderMember() {
return timestampHeaderMember;
}
/**
* Returns the value of the PayloadStructParam property for this object.
*
* @return The value of the PayloadStructParam property for this object.
*/
public PayloadStructType payloadStructParam() {
return payloadStructParam;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(pathParam());
hashCode = 31 * hashCode + Objects.hashCode(queryParamOne());
hashCode = 31 * hashCode + Objects.hashCode(queryParamTwo());
hashCode = 31 * hashCode + Objects.hashCode(stringHeaderMember());
hashCode = 31 * hashCode + Objects.hashCode(timestampHeaderMember());
hashCode = 31 * hashCode + Objects.hashCode(payloadStructParam());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MultiLocationOperationRequest)) {
return false;
}
MultiLocationOperationRequest other = (MultiLocationOperationRequest) obj;
return Objects.equals(pathParam(), other.pathParam()) && Objects.equals(queryParamOne(), other.queryParamOne())
&& Objects.equals(queryParamTwo(), other.queryParamTwo())
&& Objects.equals(stringHeaderMember(), other.stringHeaderMember())
&& Objects.equals(timestampHeaderMember(), other.timestampHeaderMember())
&& Objects.equals(payloadStructParam(), other.payloadStructParam());
}
@Override
public String toString() {
return ToString.builder("MultiLocationOperationRequest").add("PathParam", pathParam())
.add("QueryParamOne", queryParamOne()).add("QueryParamTwo", queryParamTwo())
.add("StringHeaderMember", stringHeaderMember()).add("TimestampHeaderMember", timestampHeaderMember())
.add("PayloadStructParam", payloadStructParam()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PathParam":
return Optional.ofNullable(clazz.cast(pathParam()));
case "QueryParamOne":
return Optional.ofNullable(clazz.cast(queryParamOne()));
case "QueryParamTwo":
return Optional.ofNullable(clazz.cast(queryParamTwo()));
case "StringHeaderMember":
return Optional.ofNullable(clazz.cast(stringHeaderMember()));
case "TimestampHeaderMember":
return Optional.ofNullable(clazz.cast(timestampHeaderMember()));
case "PayloadStructParam":
return Optional.ofNullable(clazz.cast(payloadStructParam()));
default:
return Optional.empty();
}
}
public interface Builder extends ProtocolRestJsonRequest.Builder, CopyableBuilder {
/**
* Sets the value of the PathParam property for this object.
*
* @param pathParam
* The new value for the PathParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder pathParam(String pathParam);
/**
* Sets the value of the QueryParamOne property for this object.
*
* @param queryParamOne
* The new value for the QueryParamOne property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queryParamOne(String queryParamOne);
/**
* Sets the value of the QueryParamTwo property for this object.
*
* @param queryParamTwo
* The new value for the QueryParamTwo property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queryParamTwo(String queryParamTwo);
/**
* Sets the value of the StringHeaderMember property for this object.
*
* @param stringHeaderMember
* The new value for the StringHeaderMember property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stringHeaderMember(String stringHeaderMember);
/**
* Sets the value of the TimestampHeaderMember property for this object.
*
* @param timestampHeaderMember
* The new value for the TimestampHeaderMember property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timestampHeaderMember(Instant timestampHeaderMember);
/**
* Sets the value of the PayloadStructParam property for this object.
*
* @param payloadStructParam
* The new value for the PayloadStructParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder payloadStructParam(PayloadStructType payloadStructParam);
/**
* Sets the value of the PayloadStructParam property for this object.
*
* This is a convenience that creates an instance of the {@link PayloadStructType.Builder} avoiding the need to
* create one manually via {@link PayloadStructType#builder()}.
*
* When the {@link Consumer} completes, {@link PayloadStructType.Builder#build()} is called immediately and its
* result is passed to {@link #payloadStructParam(PayloadStructType)}.
*
* @param payloadStructParam
* a consumer that will call methods on {@link PayloadStructType.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #payloadStructParam(PayloadStructType)
*/
default Builder payloadStructParam(Consumer payloadStructParam) {
return payloadStructParam(PayloadStructType.builder().applyMutation(payloadStructParam).build());
}
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ProtocolRestJsonRequest.BuilderImpl implements Builder {
private String pathParam;
private String queryParamOne;
private String queryParamTwo;
private String stringHeaderMember;
private Instant timestampHeaderMember;
private PayloadStructType payloadStructParam;
private BuilderImpl() {
}
private BuilderImpl(MultiLocationOperationRequest model) {
super(model);
pathParam(model.pathParam);
queryParamOne(model.queryParamOne);
queryParamTwo(model.queryParamTwo);
stringHeaderMember(model.stringHeaderMember);
timestampHeaderMember(model.timestampHeaderMember);
payloadStructParam(model.payloadStructParam);
}
public final String getPathParam() {
return pathParam;
}
@Override
public final Builder pathParam(String pathParam) {
this.pathParam = pathParam;
return this;
}
public final void setPathParam(String pathParam) {
this.pathParam = pathParam;
}
public final String getQueryParamOne() {
return queryParamOne;
}
@Override
public final Builder queryParamOne(String queryParamOne) {
this.queryParamOne = queryParamOne;
return this;
}
public final void setQueryParamOne(String queryParamOne) {
this.queryParamOne = queryParamOne;
}
public final String getQueryParamTwo() {
return queryParamTwo;
}
@Override
public final Builder queryParamTwo(String queryParamTwo) {
this.queryParamTwo = queryParamTwo;
return this;
}
public final void setQueryParamTwo(String queryParamTwo) {
this.queryParamTwo = queryParamTwo;
}
public final String getStringHeaderMember() {
return stringHeaderMember;
}
@Override
public final Builder stringHeaderMember(String stringHeaderMember) {
this.stringHeaderMember = stringHeaderMember;
return this;
}
public final void setStringHeaderMember(String stringHeaderMember) {
this.stringHeaderMember = stringHeaderMember;
}
public final Instant getTimestampHeaderMember() {
return timestampHeaderMember;
}
@Override
public final Builder timestampHeaderMember(Instant timestampHeaderMember) {
this.timestampHeaderMember = timestampHeaderMember;
return this;
}
public final void setTimestampHeaderMember(Instant timestampHeaderMember) {
this.timestampHeaderMember = timestampHeaderMember;
}
public final PayloadStructType.Builder getPayloadStructParam() {
return payloadStructParam != null ? payloadStructParam.toBuilder() : null;
}
@Override
public final Builder payloadStructParam(PayloadStructType payloadStructParam) {
this.payloadStructParam = payloadStructParam;
return this;
}
public final void setPayloadStructParam(PayloadStructType.BuilderImpl payloadStructParam) {
this.payloadStructParam = payloadStructParam != null ? payloadStructParam.build() : null;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public MultiLocationOperationRequest build() {
return new MultiLocationOperationRequest(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy