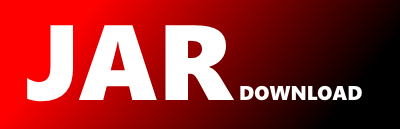
software.amazon.awssdk.services.protocolrestxml.ProtocolRestXmlClient Maven / Gradle / Ivy
Show all versions of protocol-tests Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocolrestxml;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.protocolrestxml.model.AllTypesRequest;
import software.amazon.awssdk.services.protocolrestxml.model.AllTypesResponse;
import software.amazon.awssdk.services.protocolrestxml.model.DeleteOperationRequest;
import software.amazon.awssdk.services.protocolrestxml.model.DeleteOperationResponse;
import software.amazon.awssdk.services.protocolrestxml.model.EmptyModeledException;
import software.amazon.awssdk.services.protocolrestxml.model.IdempotentOperationRequest;
import software.amazon.awssdk.services.protocolrestxml.model.IdempotentOperationResponse;
import software.amazon.awssdk.services.protocolrestxml.model.MapOfStringToListOfStringInQueryParamsRequest;
import software.amazon.awssdk.services.protocolrestxml.model.MapOfStringToListOfStringInQueryParamsResponse;
import software.amazon.awssdk.services.protocolrestxml.model.MembersInHeadersRequest;
import software.amazon.awssdk.services.protocolrestxml.model.MembersInHeadersResponse;
import software.amazon.awssdk.services.protocolrestxml.model.MembersInQueryParamsRequest;
import software.amazon.awssdk.services.protocolrestxml.model.MembersInQueryParamsResponse;
import software.amazon.awssdk.services.protocolrestxml.model.MultiLocationOperationRequest;
import software.amazon.awssdk.services.protocolrestxml.model.MultiLocationOperationResponse;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithExplicitPayloadBlobRequest;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithExplicitPayloadBlobResponse;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithGreedyLabelRequest;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithGreedyLabelResponse;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithModeledContentTypeRequest;
import software.amazon.awssdk.services.protocolrestxml.model.OperationWithModeledContentTypeResponse;
import software.amazon.awssdk.services.protocolrestxml.model.ProtocolRestXmlException;
import software.amazon.awssdk.services.protocolrestxml.model.QueryParamWithoutValueRequest;
import software.amazon.awssdk.services.protocolrestxml.model.QueryParamWithoutValueResponse;
import software.amazon.awssdk.services.protocolrestxml.model.RestXmlTypesRequest;
import software.amazon.awssdk.services.protocolrestxml.model.RestXmlTypesResponse;
/**
* Service client for accessing AmazonProtocolRestXml. This can be created using the static {@link #builder()} method.
*
* null
*/
@Generated("software.amazon.awssdk:codegen")
public interface ProtocolRestXmlClient extends SdkClient {
String SERVICE_NAME = "restxml";
/**
* Create a {@link ProtocolRestXmlClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ProtocolRestXmlClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ProtocolRestXmlClient}.
*/
static ProtocolRestXmlClientBuilder builder() {
return new DefaultProtocolRestXmlClientBuilder();
}
/**
* Invokes the AllTypes operation.
*
* @return Result of the AllTypes operation returned by the service.
* @throws EmptyModeledException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.AllTypes
* @see #allTypes(AllTypesRequest)
*/
default AllTypesResponse allTypes() throws EmptyModeledException, AwsServiceException, SdkClientException,
ProtocolRestXmlException {
return allTypes(AllTypesRequest.builder().build());
}
/**
* Invokes the AllTypes operation.
*
* @param allTypesRequest
* @return Result of the AllTypes operation returned by the service.
* @throws EmptyModeledException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.AllTypes
*/
default AllTypesResponse allTypes(AllTypesRequest allTypesRequest) throws EmptyModeledException, AwsServiceException,
SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the AllTypes operation.
*
* This is a convenience which creates an instance of the {@link AllTypesRequest.Builder} avoiding the need to
* create one manually via {@link AllTypesRequest#builder()}
*
*
* @param allTypesRequest
* A {@link Consumer} that will call methods on {@link AllTypesStructure.Builder} to create a request.
* @return Result of the AllTypes operation returned by the service.
* @throws EmptyModeledException
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.AllTypes
*/
default AllTypesResponse allTypes(Consumer allTypesRequest) throws EmptyModeledException,
AwsServiceException, SdkClientException, ProtocolRestXmlException {
return allTypes(AllTypesRequest.builder().applyMutation(allTypesRequest).build());
}
/**
* Invokes the DeleteOperation operation.
*
* @return Result of the DeleteOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.DeleteOperation
* @see #deleteOperation(DeleteOperationRequest)
*/
default DeleteOperationResponse deleteOperation() throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return deleteOperation(DeleteOperationRequest.builder().build());
}
/**
* Invokes the DeleteOperation operation.
*
* @param deleteOperationRequest
* @return Result of the DeleteOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.DeleteOperation
*/
default DeleteOperationResponse deleteOperation(DeleteOperationRequest deleteOperationRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the DeleteOperation operation.
*
* This is a convenience which creates an instance of the {@link DeleteOperationRequest.Builder} avoiding the need
* to create one manually via {@link DeleteOperationRequest#builder()}
*
*
* @param deleteOperationRequest
* A {@link Consumer} that will call methods on {@link DeleteOperationRequest.Builder} to create a request.
* @return Result of the DeleteOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.DeleteOperation
*/
default DeleteOperationResponse deleteOperation(Consumer deleteOperationRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return deleteOperation(DeleteOperationRequest.builder().applyMutation(deleteOperationRequest).build());
}
/**
* Invokes the IdempotentOperation operation.
*
* @param idempotentOperationRequest
* @return Result of the IdempotentOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.IdempotentOperation
*/
default IdempotentOperationResponse idempotentOperation(IdempotentOperationRequest idempotentOperationRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the IdempotentOperation operation.
*
* This is a convenience which creates an instance of the {@link IdempotentOperationRequest.Builder} avoiding the
* need to create one manually via {@link IdempotentOperationRequest#builder()}
*
*
* @param idempotentOperationRequest
* A {@link Consumer} that will call methods on {@link IdempotentOperationStructure.Builder} to create a
* request.
* @return Result of the IdempotentOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.IdempotentOperation
*/
default IdempotentOperationResponse idempotentOperation(
Consumer idempotentOperationRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return idempotentOperation(IdempotentOperationRequest.builder().applyMutation(idempotentOperationRequest).build());
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation.
*
* @return Result of the MapOfStringToListOfStringInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MapOfStringToListOfStringInQueryParams
* @see #mapOfStringToListOfStringInQueryParams(MapOfStringToListOfStringInQueryParamsRequest)
*/
default MapOfStringToListOfStringInQueryParamsResponse mapOfStringToListOfStringInQueryParams() throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return mapOfStringToListOfStringInQueryParams(MapOfStringToListOfStringInQueryParamsRequest.builder().build());
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation.
*
* @param mapOfStringToListOfStringInQueryParamsRequest
* @return Result of the MapOfStringToListOfStringInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MapOfStringToListOfStringInQueryParams
*/
default MapOfStringToListOfStringInQueryParamsResponse mapOfStringToListOfStringInQueryParams(
MapOfStringToListOfStringInQueryParamsRequest mapOfStringToListOfStringInQueryParamsRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the MapOfStringToListOfStringInQueryParams operation.
*
* This is a convenience which creates an instance of the
* {@link MapOfStringToListOfStringInQueryParamsRequest.Builder} avoiding the need to create one manually via
* {@link MapOfStringToListOfStringInQueryParamsRequest#builder()}
*
*
* @param mapOfStringToListOfStringInQueryParamsRequest
* A {@link Consumer} that will call methods on {@link MapOfStringToListOfStringInQueryParamsInput.Builder}
* to create a request.
* @return Result of the MapOfStringToListOfStringInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MapOfStringToListOfStringInQueryParams
*/
default MapOfStringToListOfStringInQueryParamsResponse mapOfStringToListOfStringInQueryParams(
Consumer mapOfStringToListOfStringInQueryParamsRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return mapOfStringToListOfStringInQueryParams(MapOfStringToListOfStringInQueryParamsRequest.builder()
.applyMutation(mapOfStringToListOfStringInQueryParamsRequest).build());
}
/**
* Invokes the MembersInHeaders operation.
*
* @return Result of the MembersInHeaders operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInHeaders
* @see #membersInHeaders(MembersInHeadersRequest)
*/
default MembersInHeadersResponse membersInHeaders() throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return membersInHeaders(MembersInHeadersRequest.builder().build());
}
/**
* Invokes the MembersInHeaders operation.
*
* @param membersInHeadersRequest
* @return Result of the MembersInHeaders operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInHeaders
*/
default MembersInHeadersResponse membersInHeaders(MembersInHeadersRequest membersInHeadersRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the MembersInHeaders operation.
*
* This is a convenience which creates an instance of the {@link MembersInHeadersRequest.Builder} avoiding the need
* to create one manually via {@link MembersInHeadersRequest#builder()}
*
*
* @param membersInHeadersRequest
* A {@link Consumer} that will call methods on {@link MembersInHeadersInput.Builder} to create a request.
* @return Result of the MembersInHeaders operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInHeaders
*/
default MembersInHeadersResponse membersInHeaders(Consumer membersInHeadersRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return membersInHeaders(MembersInHeadersRequest.builder().applyMutation(membersInHeadersRequest).build());
}
/**
* Invokes the MembersInQueryParams operation.
*
* @return Result of the MembersInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInQueryParams
* @see #membersInQueryParams(MembersInQueryParamsRequest)
*/
default MembersInQueryParamsResponse membersInQueryParams() throws AwsServiceException, SdkClientException,
ProtocolRestXmlException {
return membersInQueryParams(MembersInQueryParamsRequest.builder().build());
}
/**
* Invokes the MembersInQueryParams operation.
*
* @param membersInQueryParamsRequest
* @return Result of the MembersInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInQueryParams
*/
default MembersInQueryParamsResponse membersInQueryParams(MembersInQueryParamsRequest membersInQueryParamsRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the MembersInQueryParams operation.
*
* This is a convenience which creates an instance of the {@link MembersInQueryParamsRequest.Builder} avoiding the
* need to create one manually via {@link MembersInQueryParamsRequest#builder()}
*
*
* @param membersInQueryParamsRequest
* A {@link Consumer} that will call methods on {@link MembersInQueryParamsInput.Builder} to create a
* request.
* @return Result of the MembersInQueryParams operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MembersInQueryParams
*/
default MembersInQueryParamsResponse membersInQueryParams(
Consumer membersInQueryParamsRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return membersInQueryParams(MembersInQueryParamsRequest.builder().applyMutation(membersInQueryParamsRequest).build());
}
/**
* Invokes the MultiLocationOperation operation.
*
* @param multiLocationOperationRequest
* @return Result of the MultiLocationOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MultiLocationOperation
*/
default MultiLocationOperationResponse multiLocationOperation(MultiLocationOperationRequest multiLocationOperationRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the MultiLocationOperation operation.
*
* This is a convenience which creates an instance of the {@link MultiLocationOperationRequest.Builder} avoiding the
* need to create one manually via {@link MultiLocationOperationRequest#builder()}
*
*
* @param multiLocationOperationRequest
* A {@link Consumer} that will call methods on {@link MultiLocationOperationInput.Builder} to create a
* request.
* @return Result of the MultiLocationOperation operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.MultiLocationOperation
*/
default MultiLocationOperationResponse multiLocationOperation(
Consumer multiLocationOperationRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return multiLocationOperation(MultiLocationOperationRequest.builder().applyMutation(multiLocationOperationRequest)
.build());
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation.
*
* @return Result of the OperationWithExplicitPayloadBlob operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithExplicitPayloadBlob
* @see #operationWithExplicitPayloadBlob(OperationWithExplicitPayloadBlobRequest)
*/
default OperationWithExplicitPayloadBlobResponse operationWithExplicitPayloadBlob() throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return operationWithExplicitPayloadBlob(OperationWithExplicitPayloadBlobRequest.builder().build());
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation.
*
* @param operationWithExplicitPayloadBlobRequest
* @return Result of the OperationWithExplicitPayloadBlob operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithExplicitPayloadBlob
*/
default OperationWithExplicitPayloadBlobResponse operationWithExplicitPayloadBlob(
OperationWithExplicitPayloadBlobRequest operationWithExplicitPayloadBlobRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithExplicitPayloadBlob operation.
*
* This is a convenience which creates an instance of the {@link OperationWithExplicitPayloadBlobRequest.Builder}
* avoiding the need to create one manually via {@link OperationWithExplicitPayloadBlobRequest#builder()}
*
*
* @param operationWithExplicitPayloadBlobRequest
* A {@link Consumer} that will call methods on {@link OperationWithExplicitPayloadBlobInput.Builder} to
* create a request.
* @return Result of the OperationWithExplicitPayloadBlob operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithExplicitPayloadBlob
*/
default OperationWithExplicitPayloadBlobResponse operationWithExplicitPayloadBlob(
Consumer operationWithExplicitPayloadBlobRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return operationWithExplicitPayloadBlob(OperationWithExplicitPayloadBlobRequest.builder()
.applyMutation(operationWithExplicitPayloadBlobRequest).build());
}
/**
* Invokes the OperationWithGreedyLabel operation.
*
* @param operationWithGreedyLabelRequest
* @return Result of the OperationWithGreedyLabel operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithGreedyLabel
*/
default OperationWithGreedyLabelResponse operationWithGreedyLabel(
OperationWithGreedyLabelRequest operationWithGreedyLabelRequest) throws AwsServiceException, SdkClientException,
ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithGreedyLabel operation.
*
* This is a convenience which creates an instance of the {@link OperationWithGreedyLabelRequest.Builder} avoiding
* the need to create one manually via {@link OperationWithGreedyLabelRequest#builder()}
*
*
* @param operationWithGreedyLabelRequest
* A {@link Consumer} that will call methods on {@link OperationWithGreedyLabelInput.Builder} to create a
* request.
* @return Result of the OperationWithGreedyLabel operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithGreedyLabel
*/
default OperationWithGreedyLabelResponse operationWithGreedyLabel(
Consumer operationWithGreedyLabelRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return operationWithGreedyLabel(OperationWithGreedyLabelRequest.builder().applyMutation(operationWithGreedyLabelRequest)
.build());
}
/**
* Invokes the OperationWithModeledContentType operation.
*
* @return Result of the OperationWithModeledContentType operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithModeledContentType
* @see #operationWithModeledContentType(OperationWithModeledContentTypeRequest)
*/
default OperationWithModeledContentTypeResponse operationWithModeledContentType() throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return operationWithModeledContentType(OperationWithModeledContentTypeRequest.builder().build());
}
/**
* Invokes the OperationWithModeledContentType operation.
*
* @param operationWithModeledContentTypeRequest
* @return Result of the OperationWithModeledContentType operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithModeledContentType
*/
default OperationWithModeledContentTypeResponse operationWithModeledContentType(
OperationWithModeledContentTypeRequest operationWithModeledContentTypeRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the OperationWithModeledContentType operation.
*
* This is a convenience which creates an instance of the {@link OperationWithModeledContentTypeRequest.Builder}
* avoiding the need to create one manually via {@link OperationWithModeledContentTypeRequest#builder()}
*
*
* @param operationWithModeledContentTypeRequest
* A {@link Consumer} that will call methods on {@link OperationWithModeledContentTypeInput.Builder} to
* create a request.
* @return Result of the OperationWithModeledContentType operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.OperationWithModeledContentType
*/
default OperationWithModeledContentTypeResponse operationWithModeledContentType(
Consumer operationWithModeledContentTypeRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return operationWithModeledContentType(OperationWithModeledContentTypeRequest.builder()
.applyMutation(operationWithModeledContentTypeRequest).build());
}
/**
* Invokes the QueryParamWithoutValue operation.
*
* @return Result of the QueryParamWithoutValue operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.QueryParamWithoutValue
* @see #queryParamWithoutValue(QueryParamWithoutValueRequest)
*/
default QueryParamWithoutValueResponse queryParamWithoutValue() throws AwsServiceException, SdkClientException,
ProtocolRestXmlException {
return queryParamWithoutValue(QueryParamWithoutValueRequest.builder().build());
}
/**
* Invokes the QueryParamWithoutValue operation.
*
* @param queryParamWithoutValueRequest
* @return Result of the QueryParamWithoutValue operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.QueryParamWithoutValue
*/
default QueryParamWithoutValueResponse queryParamWithoutValue(QueryParamWithoutValueRequest queryParamWithoutValueRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the QueryParamWithoutValue operation.
*
* This is a convenience which creates an instance of the {@link QueryParamWithoutValueRequest.Builder} avoiding the
* need to create one manually via {@link QueryParamWithoutValueRequest#builder()}
*
*
* @param queryParamWithoutValueRequest
* A {@link Consumer} that will call methods on {@link QueryParamWithoutValueInput.Builder} to create a
* request.
* @return Result of the QueryParamWithoutValue operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.QueryParamWithoutValue
*/
default QueryParamWithoutValueResponse queryParamWithoutValue(
Consumer queryParamWithoutValueRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
return queryParamWithoutValue(QueryParamWithoutValueRequest.builder().applyMutation(queryParamWithoutValueRequest)
.build());
}
/**
* Invokes the RestXmlTypes operation.
*
* @return Result of the RestXmlTypes operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.RestXmlTypes
* @see #restXmlTypes(RestXmlTypesRequest)
*/
default RestXmlTypesResponse restXmlTypes() throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return restXmlTypes(RestXmlTypesRequest.builder().build());
}
/**
* Invokes the RestXmlTypes operation.
*
* @param restXmlTypesRequest
* @return Result of the RestXmlTypes operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.RestXmlTypes
*/
default RestXmlTypesResponse restXmlTypes(RestXmlTypesRequest restXmlTypesRequest) throws AwsServiceException,
SdkClientException, ProtocolRestXmlException {
throw new UnsupportedOperationException();
}
/**
* Invokes the RestXmlTypes operation.
*
* This is a convenience which creates an instance of the {@link RestXmlTypesRequest.Builder} avoiding the need to
* create one manually via {@link RestXmlTypesRequest#builder()}
*
*
* @param restXmlTypesRequest
* A {@link Consumer} that will call methods on {@link RestXmlTypesStructure.Builder} to create a request.
* @return Result of the RestXmlTypes operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ProtocolRestXmlException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ProtocolRestXmlClient.RestXmlTypes
*/
default RestXmlTypesResponse restXmlTypes(Consumer restXmlTypesRequest)
throws AwsServiceException, SdkClientException, ProtocolRestXmlException {
return restXmlTypes(RestXmlTypesRequest.builder().applyMutation(restXmlTypesRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("restxml");
}
}