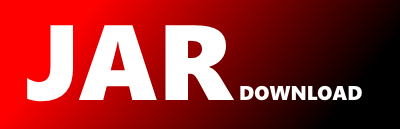
software.amazon.awssdk.services.protocolrestxml.model.MembersInQueryParamsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protocol-tests Show documentation
Show all versions of protocol-tests Show documentation
Contains functional tests for all supported protocols.
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.protocolrestxml.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class MembersInQueryParamsRequest extends ProtocolRestXmlRequest implements
ToCopyableBuilder {
private final String stringQueryParam;
private final Boolean booleanQueryParam;
private final Integer integerQueryParam;
private final Long longQueryParam;
private final Float floatQueryParam;
private final Double doubleQueryParam;
private final Instant timestampQueryParam;
private final List listOfStrings;
private final Map mapOfStringToString;
private MembersInQueryParamsRequest(BuilderImpl builder) {
super(builder);
this.stringQueryParam = builder.stringQueryParam;
this.booleanQueryParam = builder.booleanQueryParam;
this.integerQueryParam = builder.integerQueryParam;
this.longQueryParam = builder.longQueryParam;
this.floatQueryParam = builder.floatQueryParam;
this.doubleQueryParam = builder.doubleQueryParam;
this.timestampQueryParam = builder.timestampQueryParam;
this.listOfStrings = builder.listOfStrings;
this.mapOfStringToString = builder.mapOfStringToString;
}
/**
* Returns the value of the StringQueryParam property for this object.
*
* @return The value of the StringQueryParam property for this object.
*/
public String stringQueryParam() {
return stringQueryParam;
}
/**
* Returns the value of the BooleanQueryParam property for this object.
*
* @return The value of the BooleanQueryParam property for this object.
*/
public Boolean booleanQueryParam() {
return booleanQueryParam;
}
/**
* Returns the value of the IntegerQueryParam property for this object.
*
* @return The value of the IntegerQueryParam property for this object.
*/
public Integer integerQueryParam() {
return integerQueryParam;
}
/**
* Returns the value of the LongQueryParam property for this object.
*
* @return The value of the LongQueryParam property for this object.
*/
public Long longQueryParam() {
return longQueryParam;
}
/**
* Returns the value of the FloatQueryParam property for this object.
*
* @return The value of the FloatQueryParam property for this object.
*/
public Float floatQueryParam() {
return floatQueryParam;
}
/**
* Returns the value of the DoubleQueryParam property for this object.
*
* @return The value of the DoubleQueryParam property for this object.
*/
public Double doubleQueryParam() {
return doubleQueryParam;
}
/**
* Returns the value of the TimestampQueryParam property for this object.
*
* @return The value of the TimestampQueryParam property for this object.
*/
public Instant timestampQueryParam() {
return timestampQueryParam;
}
/**
* Returns the value of the ListOfStrings property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the ListOfStrings property for this object.
*/
public List listOfStrings() {
return listOfStrings;
}
/**
* Returns the value of the MapOfStringToString property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the MapOfStringToString property for this object.
*/
public Map mapOfStringToString() {
return mapOfStringToString;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(stringQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(booleanQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(integerQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(longQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(floatQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(doubleQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(timestampQueryParam());
hashCode = 31 * hashCode + Objects.hashCode(listOfStrings());
hashCode = 31 * hashCode + Objects.hashCode(mapOfStringToString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MembersInQueryParamsRequest)) {
return false;
}
MembersInQueryParamsRequest other = (MembersInQueryParamsRequest) obj;
return Objects.equals(stringQueryParam(), other.stringQueryParam())
&& Objects.equals(booleanQueryParam(), other.booleanQueryParam())
&& Objects.equals(integerQueryParam(), other.integerQueryParam())
&& Objects.equals(longQueryParam(), other.longQueryParam())
&& Objects.equals(floatQueryParam(), other.floatQueryParam())
&& Objects.equals(doubleQueryParam(), other.doubleQueryParam())
&& Objects.equals(timestampQueryParam(), other.timestampQueryParam())
&& Objects.equals(listOfStrings(), other.listOfStrings())
&& Objects.equals(mapOfStringToString(), other.mapOfStringToString());
}
@Override
public String toString() {
return ToString.builder("MembersInQueryParamsRequest").add("StringQueryParam", stringQueryParam())
.add("BooleanQueryParam", booleanQueryParam()).add("IntegerQueryParam", integerQueryParam())
.add("LongQueryParam", longQueryParam()).add("FloatQueryParam", floatQueryParam())
.add("DoubleQueryParam", doubleQueryParam()).add("TimestampQueryParam", timestampQueryParam())
.add("ListOfStrings", listOfStrings()).add("MapOfStringToString", mapOfStringToString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StringQueryParam":
return Optional.ofNullable(clazz.cast(stringQueryParam()));
case "BooleanQueryParam":
return Optional.ofNullable(clazz.cast(booleanQueryParam()));
case "IntegerQueryParam":
return Optional.ofNullable(clazz.cast(integerQueryParam()));
case "LongQueryParam":
return Optional.ofNullable(clazz.cast(longQueryParam()));
case "FloatQueryParam":
return Optional.ofNullable(clazz.cast(floatQueryParam()));
case "DoubleQueryParam":
return Optional.ofNullable(clazz.cast(doubleQueryParam()));
case "TimestampQueryParam":
return Optional.ofNullable(clazz.cast(timestampQueryParam()));
case "ListOfStrings":
return Optional.ofNullable(clazz.cast(listOfStrings()));
case "MapOfStringToString":
return Optional.ofNullable(clazz.cast(mapOfStringToString()));
default:
return Optional.empty();
}
}
public interface Builder extends ProtocolRestXmlRequest.Builder, CopyableBuilder {
/**
* Sets the value of the StringQueryParam property for this object.
*
* @param stringQueryParam
* The new value for the StringQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stringQueryParam(String stringQueryParam);
/**
* Sets the value of the BooleanQueryParam property for this object.
*
* @param booleanQueryParam
* The new value for the BooleanQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder booleanQueryParam(Boolean booleanQueryParam);
/**
* Sets the value of the IntegerQueryParam property for this object.
*
* @param integerQueryParam
* The new value for the IntegerQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder integerQueryParam(Integer integerQueryParam);
/**
* Sets the value of the LongQueryParam property for this object.
*
* @param longQueryParam
* The new value for the LongQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder longQueryParam(Long longQueryParam);
/**
* Sets the value of the FloatQueryParam property for this object.
*
* @param floatQueryParam
* The new value for the FloatQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder floatQueryParam(Float floatQueryParam);
/**
* Sets the value of the DoubleQueryParam property for this object.
*
* @param doubleQueryParam
* The new value for the DoubleQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder doubleQueryParam(Double doubleQueryParam);
/**
* Sets the value of the TimestampQueryParam property for this object.
*
* @param timestampQueryParam
* The new value for the TimestampQueryParam property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timestampQueryParam(Instant timestampQueryParam);
/**
* Sets the value of the ListOfStrings property for this object.
*
* @param listOfStrings
* The new value for the ListOfStrings property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder listOfStrings(Collection listOfStrings);
/**
* Sets the value of the ListOfStrings property for this object.
*
* @param listOfStrings
* The new value for the ListOfStrings property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder listOfStrings(String... listOfStrings);
/**
* Sets the value of the MapOfStringToString property for this object.
*
* @param mapOfStringToString
* The new value for the MapOfStringToString property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mapOfStringToString(Map mapOfStringToString);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ProtocolRestXmlRequest.BuilderImpl implements Builder {
private String stringQueryParam;
private Boolean booleanQueryParam;
private Integer integerQueryParam;
private Long longQueryParam;
private Float floatQueryParam;
private Double doubleQueryParam;
private Instant timestampQueryParam;
private List listOfStrings = DefaultSdkAutoConstructList.getInstance();
private Map mapOfStringToString = DefaultSdkAutoConstructMap.getInstance();
private BuilderImpl() {
}
private BuilderImpl(MembersInQueryParamsRequest model) {
super(model);
stringQueryParam(model.stringQueryParam);
booleanQueryParam(model.booleanQueryParam);
integerQueryParam(model.integerQueryParam);
longQueryParam(model.longQueryParam);
floatQueryParam(model.floatQueryParam);
doubleQueryParam(model.doubleQueryParam);
timestampQueryParam(model.timestampQueryParam);
listOfStrings(model.listOfStrings);
mapOfStringToString(model.mapOfStringToString);
}
public final String getStringQueryParam() {
return stringQueryParam;
}
@Override
public final Builder stringQueryParam(String stringQueryParam) {
this.stringQueryParam = stringQueryParam;
return this;
}
public final void setStringQueryParam(String stringQueryParam) {
this.stringQueryParam = stringQueryParam;
}
public final Boolean getBooleanQueryParam() {
return booleanQueryParam;
}
@Override
public final Builder booleanQueryParam(Boolean booleanQueryParam) {
this.booleanQueryParam = booleanQueryParam;
return this;
}
public final void setBooleanQueryParam(Boolean booleanQueryParam) {
this.booleanQueryParam = booleanQueryParam;
}
public final Integer getIntegerQueryParam() {
return integerQueryParam;
}
@Override
public final Builder integerQueryParam(Integer integerQueryParam) {
this.integerQueryParam = integerQueryParam;
return this;
}
public final void setIntegerQueryParam(Integer integerQueryParam) {
this.integerQueryParam = integerQueryParam;
}
public final Long getLongQueryParam() {
return longQueryParam;
}
@Override
public final Builder longQueryParam(Long longQueryParam) {
this.longQueryParam = longQueryParam;
return this;
}
public final void setLongQueryParam(Long longQueryParam) {
this.longQueryParam = longQueryParam;
}
public final Float getFloatQueryParam() {
return floatQueryParam;
}
@Override
public final Builder floatQueryParam(Float floatQueryParam) {
this.floatQueryParam = floatQueryParam;
return this;
}
public final void setFloatQueryParam(Float floatQueryParam) {
this.floatQueryParam = floatQueryParam;
}
public final Double getDoubleQueryParam() {
return doubleQueryParam;
}
@Override
public final Builder doubleQueryParam(Double doubleQueryParam) {
this.doubleQueryParam = doubleQueryParam;
return this;
}
public final void setDoubleQueryParam(Double doubleQueryParam) {
this.doubleQueryParam = doubleQueryParam;
}
public final Instant getTimestampQueryParam() {
return timestampQueryParam;
}
@Override
public final Builder timestampQueryParam(Instant timestampQueryParam) {
this.timestampQueryParam = timestampQueryParam;
return this;
}
public final void setTimestampQueryParam(Instant timestampQueryParam) {
this.timestampQueryParam = timestampQueryParam;
}
public final Collection getListOfStrings() {
return listOfStrings;
}
@Override
public final Builder listOfStrings(Collection listOfStrings) {
this.listOfStrings = ListOfStringsCopier.copy(listOfStrings);
return this;
}
@Override
@SafeVarargs
public final Builder listOfStrings(String... listOfStrings) {
listOfStrings(Arrays.asList(listOfStrings));
return this;
}
public final void setListOfStrings(Collection listOfStrings) {
this.listOfStrings = ListOfStringsCopier.copy(listOfStrings);
}
public final Map getMapOfStringToString() {
return mapOfStringToString;
}
@Override
public final Builder mapOfStringToString(Map mapOfStringToString) {
this.mapOfStringToString = MapOfStringToStringCopier.copy(mapOfStringToString);
return this;
}
public final void setMapOfStringToString(Map mapOfStringToString) {
this.mapOfStringToString = MapOfStringToStringCopier.copy(mapOfStringToString);
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public MembersInQueryParamsRequest build() {
return new MembersInQueryParamsRequest(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy