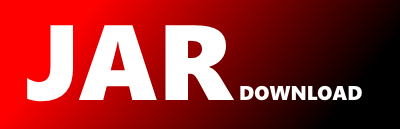
software.amazon.awssdk.services.quicksight.model.StartAssetBundleExportJobRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.quicksight.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartAssetBundleExportJobRequest extends QuickSightRequest implements
ToCopyableBuilder {
private static final SdkField AWS_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AwsAccountId").getter(getter(StartAssetBundleExportJobRequest::awsAccountId))
.setter(setter(Builder::awsAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("AwsAccountId").build()).build();
private static final SdkField ASSET_BUNDLE_EXPORT_JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AssetBundleExportJobId").getter(getter(StartAssetBundleExportJobRequest::assetBundleExportJobId))
.setter(setter(Builder::assetBundleExportJobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssetBundleExportJobId").build())
.build();
private static final SdkField> RESOURCE_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceArns")
.getter(getter(StartAssetBundleExportJobRequest::resourceArns))
.setter(setter(Builder::resourceArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INCLUDE_ALL_DEPENDENCIES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeAllDependencies").getter(getter(StartAssetBundleExportJobRequest::includeAllDependencies))
.setter(setter(Builder::includeAllDependencies))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeAllDependencies").build())
.build();
private static final SdkField EXPORT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportFormat").getter(getter(StartAssetBundleExportJobRequest::exportFormatAsString))
.setter(setter(Builder::exportFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportFormat").build()).build();
private static final SdkField CLOUD_FORMATION_OVERRIDE_PROPERTY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("CloudFormationOverridePropertyConfiguration")
.getter(getter(StartAssetBundleExportJobRequest::cloudFormationOverridePropertyConfiguration))
.setter(setter(Builder::cloudFormationOverridePropertyConfiguration))
.constructor(AssetBundleCloudFormationOverridePropertyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CloudFormationOverridePropertyConfiguration").build()).build();
private static final SdkField INCLUDE_PERMISSIONS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludePermissions").getter(getter(StartAssetBundleExportJobRequest::includePermissions))
.setter(setter(Builder::includePermissions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludePermissions").build())
.build();
private static final SdkField INCLUDE_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeTags").getter(getter(StartAssetBundleExportJobRequest::includeTags))
.setter(setter(Builder::includeTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeTags").build()).build();
private static final SdkField VALIDATION_STRATEGY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ValidationStrategy")
.getter(getter(StartAssetBundleExportJobRequest::validationStrategy)).setter(setter(Builder::validationStrategy))
.constructor(AssetBundleExportJobValidationStrategy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidationStrategy").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AWS_ACCOUNT_ID_FIELD,
ASSET_BUNDLE_EXPORT_JOB_ID_FIELD, RESOURCE_ARNS_FIELD, INCLUDE_ALL_DEPENDENCIES_FIELD, EXPORT_FORMAT_FIELD,
CLOUD_FORMATION_OVERRIDE_PROPERTY_CONFIGURATION_FIELD, INCLUDE_PERMISSIONS_FIELD, INCLUDE_TAGS_FIELD,
VALIDATION_STRATEGY_FIELD));
private final String awsAccountId;
private final String assetBundleExportJobId;
private final List resourceArns;
private final Boolean includeAllDependencies;
private final String exportFormat;
private final AssetBundleCloudFormationOverridePropertyConfiguration cloudFormationOverridePropertyConfiguration;
private final Boolean includePermissions;
private final Boolean includeTags;
private final AssetBundleExportJobValidationStrategy validationStrategy;
private StartAssetBundleExportJobRequest(BuilderImpl builder) {
super(builder);
this.awsAccountId = builder.awsAccountId;
this.assetBundleExportJobId = builder.assetBundleExportJobId;
this.resourceArns = builder.resourceArns;
this.includeAllDependencies = builder.includeAllDependencies;
this.exportFormat = builder.exportFormat;
this.cloudFormationOverridePropertyConfiguration = builder.cloudFormationOverridePropertyConfiguration;
this.includePermissions = builder.includePermissions;
this.includeTags = builder.includeTags;
this.validationStrategy = builder.validationStrategy;
}
/**
*
* The ID of the Amazon Web Services account to export assets from.
*
*
* @return The ID of the Amazon Web Services account to export assets from.
*/
public final String awsAccountId() {
return awsAccountId;
}
/**
*
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse this ID
* for another job.
*
*
* @return The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse
* this ID for another job.
*/
public final String assetBundleExportJobId() {
return assetBundleExportJobId;
}
/**
* For responses, this returns true if the service returned a value for the ResourceArns property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceArns() {
return resourceArns != null && !(resourceArns instanceof SdkAutoConstructList);
}
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceArns} method.
*
*
* @return An array of resource ARNs to export. The following resources are supported.
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the
* resources can be exported.
*/
public final List resourceArns() {
return resourceArns;
}
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*
* @return A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the
* job. For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource
* that is a dependency of the dashboard is also exported.
*/
public final Boolean includeAllDependencies() {
return includeAllDependencies;
}
/**
*
* The export data format.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportFormat} will
* return {@link AssetBundleExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #exportFormatAsString}.
*
*
* @return The export data format.
* @see AssetBundleExportFormat
*/
public final AssetBundleExportFormat exportFormat() {
return AssetBundleExportFormat.fromValue(exportFormat);
}
/**
*
* The export data format.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportFormat} will
* return {@link AssetBundleExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #exportFormatAsString}.
*
*
* @return The export data format.
* @see AssetBundleExportFormat
*/
public final String exportFormatAsString() {
return exportFormat;
}
/**
*
* An optional collection of structures that generate CloudFormation parameters to override the existing resource
* property values when the resource is exported to a new CloudFormation template.
*
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
API call
* is set to CLOUDFORMATION_JSON
.
*
*
* @return An optional collection of structures that generate CloudFormation parameters to override the existing
* resource property values when the resource is exported to a new CloudFormation template.
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
* API call is set to CLOUDFORMATION_JSON
.
*/
public final AssetBundleCloudFormationOverridePropertyConfiguration cloudFormationOverridePropertyConfiguration() {
return cloudFormationOverridePropertyConfiguration;
}
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*
* @return A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you
* set IncludePermissions
to TRUE
, any permissions associated with each resource
* are exported.
*/
public final Boolean includePermissions() {
return includePermissions;
}
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*
* @return A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*/
public final Boolean includeTags() {
return includeTags;
}
/**
*
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown as
* warnings. The default value for StrictModeForAllResources
is FALSE
.
*
*
* @return An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown
* as warnings. The default value for StrictModeForAllResources
is FALSE
.
*/
public final AssetBundleExportJobValidationStrategy validationStrategy() {
return validationStrategy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(awsAccountId());
hashCode = 31 * hashCode + Objects.hashCode(assetBundleExportJobId());
hashCode = 31 * hashCode + Objects.hashCode(hasResourceArns() ? resourceArns() : null);
hashCode = 31 * hashCode + Objects.hashCode(includeAllDependencies());
hashCode = 31 * hashCode + Objects.hashCode(exportFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(cloudFormationOverridePropertyConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(includePermissions());
hashCode = 31 * hashCode + Objects.hashCode(includeTags());
hashCode = 31 * hashCode + Objects.hashCode(validationStrategy());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartAssetBundleExportJobRequest)) {
return false;
}
StartAssetBundleExportJobRequest other = (StartAssetBundleExportJobRequest) obj;
return Objects.equals(awsAccountId(), other.awsAccountId())
&& Objects.equals(assetBundleExportJobId(), other.assetBundleExportJobId())
&& hasResourceArns() == other.hasResourceArns()
&& Objects.equals(resourceArns(), other.resourceArns())
&& Objects.equals(includeAllDependencies(), other.includeAllDependencies())
&& Objects.equals(exportFormatAsString(), other.exportFormatAsString())
&& Objects.equals(cloudFormationOverridePropertyConfiguration(),
other.cloudFormationOverridePropertyConfiguration())
&& Objects.equals(includePermissions(), other.includePermissions())
&& Objects.equals(includeTags(), other.includeTags())
&& Objects.equals(validationStrategy(), other.validationStrategy());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartAssetBundleExportJobRequest").add("AwsAccountId", awsAccountId())
.add("AssetBundleExportJobId", assetBundleExportJobId())
.add("ResourceArns", hasResourceArns() ? resourceArns() : null)
.add("IncludeAllDependencies", includeAllDependencies()).add("ExportFormat", exportFormatAsString())
.add("CloudFormationOverridePropertyConfiguration", cloudFormationOverridePropertyConfiguration())
.add("IncludePermissions", includePermissions()).add("IncludeTags", includeTags())
.add("ValidationStrategy", validationStrategy()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AwsAccountId":
return Optional.ofNullable(clazz.cast(awsAccountId()));
case "AssetBundleExportJobId":
return Optional.ofNullable(clazz.cast(assetBundleExportJobId()));
case "ResourceArns":
return Optional.ofNullable(clazz.cast(resourceArns()));
case "IncludeAllDependencies":
return Optional.ofNullable(clazz.cast(includeAllDependencies()));
case "ExportFormat":
return Optional.ofNullable(clazz.cast(exportFormatAsString()));
case "CloudFormationOverridePropertyConfiguration":
return Optional.ofNullable(clazz.cast(cloudFormationOverridePropertyConfiguration()));
case "IncludePermissions":
return Optional.ofNullable(clazz.cast(includePermissions()));
case "IncludeTags":
return Optional.ofNullable(clazz.cast(includeTags()));
case "ValidationStrategy":
return Optional.ofNullable(clazz.cast(validationStrategy()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function