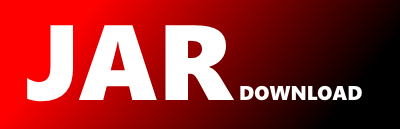
software.amazon.awssdk.services.ram.RamAsyncClient Maven / Gradle / Ivy
Show all versions of ram Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ram;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.ram.model.AcceptResourceShareInvitationRequest;
import software.amazon.awssdk.services.ram.model.AcceptResourceShareInvitationResponse;
import software.amazon.awssdk.services.ram.model.AssociateResourceSharePermissionRequest;
import software.amazon.awssdk.services.ram.model.AssociateResourceSharePermissionResponse;
import software.amazon.awssdk.services.ram.model.AssociateResourceShareRequest;
import software.amazon.awssdk.services.ram.model.AssociateResourceShareResponse;
import software.amazon.awssdk.services.ram.model.CreateResourceShareRequest;
import software.amazon.awssdk.services.ram.model.CreateResourceShareResponse;
import software.amazon.awssdk.services.ram.model.DeleteResourceShareRequest;
import software.amazon.awssdk.services.ram.model.DeleteResourceShareResponse;
import software.amazon.awssdk.services.ram.model.DisassociateResourceSharePermissionRequest;
import software.amazon.awssdk.services.ram.model.DisassociateResourceSharePermissionResponse;
import software.amazon.awssdk.services.ram.model.DisassociateResourceShareRequest;
import software.amazon.awssdk.services.ram.model.DisassociateResourceShareResponse;
import software.amazon.awssdk.services.ram.model.EnableSharingWithAwsOrganizationRequest;
import software.amazon.awssdk.services.ram.model.EnableSharingWithAwsOrganizationResponse;
import software.amazon.awssdk.services.ram.model.GetPermissionRequest;
import software.amazon.awssdk.services.ram.model.GetPermissionResponse;
import software.amazon.awssdk.services.ram.model.GetResourcePoliciesRequest;
import software.amazon.awssdk.services.ram.model.GetResourcePoliciesResponse;
import software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsRequest;
import software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsResponse;
import software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest;
import software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsResponse;
import software.amazon.awssdk.services.ram.model.GetResourceSharesRequest;
import software.amazon.awssdk.services.ram.model.GetResourceSharesResponse;
import software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesRequest;
import software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesResponse;
import software.amazon.awssdk.services.ram.model.ListPermissionVersionsRequest;
import software.amazon.awssdk.services.ram.model.ListPermissionVersionsResponse;
import software.amazon.awssdk.services.ram.model.ListPermissionsRequest;
import software.amazon.awssdk.services.ram.model.ListPermissionsResponse;
import software.amazon.awssdk.services.ram.model.ListPrincipalsRequest;
import software.amazon.awssdk.services.ram.model.ListPrincipalsResponse;
import software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsRequest;
import software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsResponse;
import software.amazon.awssdk.services.ram.model.ListResourceTypesRequest;
import software.amazon.awssdk.services.ram.model.ListResourceTypesResponse;
import software.amazon.awssdk.services.ram.model.ListResourcesRequest;
import software.amazon.awssdk.services.ram.model.ListResourcesResponse;
import software.amazon.awssdk.services.ram.model.PromoteResourceShareCreatedFromPolicyRequest;
import software.amazon.awssdk.services.ram.model.PromoteResourceShareCreatedFromPolicyResponse;
import software.amazon.awssdk.services.ram.model.RejectResourceShareInvitationRequest;
import software.amazon.awssdk.services.ram.model.RejectResourceShareInvitationResponse;
import software.amazon.awssdk.services.ram.model.TagResourceRequest;
import software.amazon.awssdk.services.ram.model.TagResourceResponse;
import software.amazon.awssdk.services.ram.model.UntagResourceRequest;
import software.amazon.awssdk.services.ram.model.UntagResourceResponse;
import software.amazon.awssdk.services.ram.model.UpdateResourceShareRequest;
import software.amazon.awssdk.services.ram.model.UpdateResourceShareResponse;
import software.amazon.awssdk.services.ram.paginators.GetResourcePoliciesPublisher;
import software.amazon.awssdk.services.ram.paginators.GetResourceShareAssociationsPublisher;
import software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher;
import software.amazon.awssdk.services.ram.paginators.GetResourceSharesPublisher;
import software.amazon.awssdk.services.ram.paginators.ListPendingInvitationResourcesPublisher;
import software.amazon.awssdk.services.ram.paginators.ListPermissionVersionsPublisher;
import software.amazon.awssdk.services.ram.paginators.ListPermissionsPublisher;
import software.amazon.awssdk.services.ram.paginators.ListPrincipalsPublisher;
import software.amazon.awssdk.services.ram.paginators.ListResourceSharePermissionsPublisher;
import software.amazon.awssdk.services.ram.paginators.ListResourceTypesPublisher;
import software.amazon.awssdk.services.ram.paginators.ListResourcesPublisher;
/**
* Service client for accessing RAM asynchronously. This can be created using the static {@link #builder()} method.
*
*
* This is the Resource Access Manager API Reference. This documentation provides descriptions and syntax for
* each of the actions and data types in RAM. RAM is a service that helps you securely share your Amazon Web Services
* resources across Amazon Web Services accounts. If you have multiple Amazon Web Services accounts, you can use RAM to
* share those resources with other accounts. If you use Organizations to manage your accounts, then you share your
* resources with your organization or organizational units (OUs). For supported resource types, you can also share
* resources with individual Identity and Access Management (IAM) roles an users.
*
*
* To learn more about RAM, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface RamAsyncClient extends SdkClient {
String SERVICE_NAME = "ram";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ram";
/**
* Create a {@link RamAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static RamAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link RamAsyncClient}.
*/
static RamAsyncClientBuilder builder() {
return new DefaultRamAsyncClientBuilder();
}
/**
*
* Accepts an invitation to a resource share from another Amazon Web Services account. After you accept the
* invitation, the resources included in the resource share are available to interact with in the relevant Amazon
* Web Services Management Consoles and tools.
*
*
* @param acceptResourceShareInvitationRequest
* @return A Java Future containing the result of the AcceptResourceShareInvitation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - ResourceShareInvitationAlreadyAcceptedException The specified invitation was already accepted.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - InvalidClientTokenException The client token is not valid.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AcceptResourceShareInvitation
* @see AWS API Documentation
*/
default CompletableFuture acceptResourceShareInvitation(
AcceptResourceShareInvitationRequest acceptResourceShareInvitationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Accepts an invitation to a resource share from another Amazon Web Services account. After you accept the
* invitation, the resources included in the resource share are available to interact with in the relevant Amazon
* Web Services Management Consoles and tools.
*
*
*
* This is a convenience which creates an instance of the {@link AcceptResourceShareInvitationRequest.Builder}
* avoiding the need to create one manually via {@link AcceptResourceShareInvitationRequest#builder()}
*
*
* @param acceptResourceShareInvitationRequest
* A {@link Consumer} that will call methods on {@link AcceptResourceShareInvitationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AcceptResourceShareInvitation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - ResourceShareInvitationAlreadyAcceptedException The specified invitation was already accepted.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - InvalidClientTokenException The client token is not valid.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AcceptResourceShareInvitation
* @see AWS API Documentation
*/
default CompletableFuture acceptResourceShareInvitation(
Consumer acceptResourceShareInvitationRequest) {
return acceptResourceShareInvitation(AcceptResourceShareInvitationRequest.builder()
.applyMutation(acceptResourceShareInvitationRequest).build());
}
/**
*
* Adds the specified list of principals and list of resources to a resource share. Principals that already have
* access to this resource share immediately receive access to the added resources. Newly added principals
* immediately receive access to the resources shared in this resource share.
*
*
* @param associateResourceShareRequest
* @return A Java Future containing the result of the AssociateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - UnknownResourceException A specified resource was not found.
* - InvalidStateTransitionException The requested state transition is not valid.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidStateTransitionException The requested state transition is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - ThrottlingException You exceeded the rate at which you are allowed to perform this operation. Please
* try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AssociateResourceShare
* @see AWS
* API Documentation
*/
default CompletableFuture associateResourceShare(
AssociateResourceShareRequest associateResourceShareRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified list of principals and list of resources to a resource share. Principals that already have
* access to this resource share immediately receive access to the added resources. Newly added principals
* immediately receive access to the resources shared in this resource share.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateResourceShareRequest.Builder} avoiding the
* need to create one manually via {@link AssociateResourceShareRequest#builder()}
*
*
* @param associateResourceShareRequest
* A {@link Consumer} that will call methods on {@link AssociateResourceShareRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - UnknownResourceException A specified resource was not found.
* - InvalidStateTransitionException The requested state transition is not valid.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidStateTransitionException The requested state transition is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - ThrottlingException You exceeded the rate at which you are allowed to perform this operation. Please
* try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AssociateResourceShare
* @see AWS
* API Documentation
*/
default CompletableFuture associateResourceShare(
Consumer associateResourceShareRequest) {
return associateResourceShare(AssociateResourceShareRequest.builder().applyMutation(associateResourceShareRequest)
.build());
}
/**
*
* Adds or replaces the RAM permission for a resource type included in a resource share. You can have exactly one
* permission associated with each resource type in the resource share. You can add a new RAM permission only if
* there are currently no resources of that resource type currently in the resource share.
*
*
* @param associateResourceSharePermissionRequest
* @return A Java Future containing the result of the AssociateResourceSharePermission operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidParameterException A parameter is not valid.
* - InvalidClientTokenException The client token is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AssociateResourceSharePermission
* @see AWS API Documentation
*/
default CompletableFuture associateResourceSharePermission(
AssociateResourceSharePermissionRequest associateResourceSharePermissionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or replaces the RAM permission for a resource type included in a resource share. You can have exactly one
* permission associated with each resource type in the resource share. You can add a new RAM permission only if
* there are currently no resources of that resource type currently in the resource share.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateResourceSharePermissionRequest.Builder}
* avoiding the need to create one manually via {@link AssociateResourceSharePermissionRequest#builder()}
*
*
* @param associateResourceSharePermissionRequest
* A {@link Consumer} that will call methods on {@link AssociateResourceSharePermissionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateResourceSharePermission operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidParameterException A parameter is not valid.
* - InvalidClientTokenException The client token is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.AssociateResourceSharePermission
* @see AWS API Documentation
*/
default CompletableFuture associateResourceSharePermission(
Consumer associateResourceSharePermissionRequest) {
return associateResourceSharePermission(AssociateResourceSharePermissionRequest.builder()
.applyMutation(associateResourceSharePermissionRequest).build());
}
/**
*
* Creates a resource share. You can provide a list of the Amazon Resource Names
* (ARNs) for the resources that you want to share, a list of principals you want to share the resources with,
* and the permissions to grant those principals.
*
*
*
* Sharing a resource makes it available for use by principals outside of the Amazon Web Services account that
* created the resource. Sharing doesn't change any permissions or quotas that apply to the resource in the account
* that created it.
*
*
*
* @param createResourceShareRequest
* @return A Java Future containing the result of the CreateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - InvalidStateTransitionException The requested state transition is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - TagPolicyViolationException The specified tag key is a reserved word and can't be used.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.CreateResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture createResourceShare(
CreateResourceShareRequest createResourceShareRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a resource share. You can provide a list of the Amazon Resource Names
* (ARNs) for the resources that you want to share, a list of principals you want to share the resources with,
* and the permissions to grant those principals.
*
*
*
* Sharing a resource makes it available for use by principals outside of the Amazon Web Services account that
* created the resource. Sharing doesn't change any permissions or quotas that apply to the resource in the account
* that created it.
*
*
*
* This is a convenience which creates an instance of the {@link CreateResourceShareRequest.Builder} avoiding the
* need to create one manually via {@link CreateResourceShareRequest#builder()}
*
*
* @param createResourceShareRequest
* A {@link Consumer} that will call methods on {@link CreateResourceShareRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - InvalidStateTransitionException The requested state transition is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - TagPolicyViolationException The specified tag key is a reserved word and can't be used.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.CreateResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture createResourceShare(
Consumer createResourceShareRequest) {
return createResourceShare(CreateResourceShareRequest.builder().applyMutation(createResourceShareRequest).build());
}
/**
*
* Deletes the specified resource share. This doesn't delete any of the resources that were associated with the
* resource share; it only stops the sharing of those resources outside of the Amazon Web Services account that
* created them.
*
*
* @param deleteResourceShareRequest
* @return A Java Future containing the result of the DeleteResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The requested operation is not permitted.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - InvalidStateTransitionException The requested state transition is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DeleteResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture deleteResourceShare(
DeleteResourceShareRequest deleteResourceShareRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified resource share. This doesn't delete any of the resources that were associated with the
* resource share; it only stops the sharing of those resources outside of the Amazon Web Services account that
* created them.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteResourceShareRequest.Builder} avoiding the
* need to create one manually via {@link DeleteResourceShareRequest#builder()}
*
*
* @param deleteResourceShareRequest
* A {@link Consumer} that will call methods on {@link DeleteResourceShareRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The requested operation is not permitted.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - InvalidStateTransitionException The requested state transition is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DeleteResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture deleteResourceShare(
Consumer deleteResourceShareRequest) {
return deleteResourceShare(DeleteResourceShareRequest.builder().applyMutation(deleteResourceShareRequest).build());
}
/**
*
* Disassociates the specified principals or resources from the specified resource share.
*
*
* @param disassociateResourceShareRequest
* @return A Java Future containing the result of the DisassociateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidStateTransitionException The requested state transition is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DisassociateResourceShare
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateResourceShare(
DisassociateResourceShareRequest disassociateResourceShareRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the specified principals or resources from the specified resource share.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateResourceShareRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateResourceShareRequest#builder()}
*
*
* @param disassociateResourceShareRequest
* A {@link Consumer} that will call methods on {@link DisassociateResourceShareRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidStateTransitionException The requested state transition is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DisassociateResourceShare
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateResourceShare(
Consumer disassociateResourceShareRequest) {
return disassociateResourceShare(DisassociateResourceShareRequest.builder()
.applyMutation(disassociateResourceShareRequest).build());
}
/**
*
* Disassociates an RAM permission from a resource share. Permission changes take effect immediately. You can remove
* a RAM permission from a resource share only if there are currently no resources of the relevant resource type
* currently attached to the resource share.
*
*
* @param disassociateResourceSharePermissionRequest
* @return A Java Future containing the result of the DisassociateResourceSharePermission operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidParameterException A parameter is not valid.
* - InvalidClientTokenException The client token is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidStateTransitionException The requested state transition is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DisassociateResourceSharePermission
* @see AWS API Documentation
*/
default CompletableFuture disassociateResourceSharePermission(
DisassociateResourceSharePermissionRequest disassociateResourceSharePermissionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an RAM permission from a resource share. Permission changes take effect immediately. You can remove
* a RAM permission from a resource share only if there are currently no resources of the relevant resource type
* currently attached to the resource share.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateResourceSharePermissionRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateResourceSharePermissionRequest#builder()}
*
*
* @param disassociateResourceSharePermissionRequest
* A {@link Consumer} that will call methods on {@link DisassociateResourceSharePermissionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateResourceSharePermission operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidParameterException A parameter is not valid.
* - InvalidClientTokenException The client token is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidStateTransitionException The requested state transition is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.DisassociateResourceSharePermission
* @see AWS API Documentation
*/
default CompletableFuture disassociateResourceSharePermission(
Consumer disassociateResourceSharePermissionRequest) {
return disassociateResourceSharePermission(DisassociateResourceSharePermissionRequest.builder()
.applyMutation(disassociateResourceSharePermissionRequest).build());
}
/**
*
* Enables resource sharing within your organization in Organizations. Calling this operation enables RAM to
* retrieve information about the organization and its structure. This lets you share resources with all of the
* accounts in an organization by specifying the organization's ID, or all of the accounts in an organizational unit
* (OU) by specifying the OU's ID. Until you enable sharing within the organization, you can specify only individual
* Amazon Web Services accounts, or for supported resource types, IAM users and roles.
*
*
* You must call this operation from an IAM user or role in the organization's management account.
*
*
* @param enableSharingWithAwsOrganizationRequest
* @return A Java Future containing the result of the EnableSharingWithAwsOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.EnableSharingWithAwsOrganization
* @see AWS API Documentation
*/
default CompletableFuture enableSharingWithAwsOrganization(
EnableSharingWithAwsOrganizationRequest enableSharingWithAwsOrganizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enables resource sharing within your organization in Organizations. Calling this operation enables RAM to
* retrieve information about the organization and its structure. This lets you share resources with all of the
* accounts in an organization by specifying the organization's ID, or all of the accounts in an organizational unit
* (OU) by specifying the OU's ID. Until you enable sharing within the organization, you can specify only individual
* Amazon Web Services accounts, or for supported resource types, IAM users and roles.
*
*
* You must call this operation from an IAM user or role in the organization's management account.
*
*
*
* This is a convenience which creates an instance of the {@link EnableSharingWithAwsOrganizationRequest.Builder}
* avoiding the need to create one manually via {@link EnableSharingWithAwsOrganizationRequest#builder()}
*
*
* @param enableSharingWithAwsOrganizationRequest
* A {@link Consumer} that will call methods on {@link EnableSharingWithAwsOrganizationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the EnableSharingWithAwsOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.EnableSharingWithAwsOrganization
* @see AWS API Documentation
*/
default CompletableFuture enableSharingWithAwsOrganization(
Consumer enableSharingWithAwsOrganizationRequest) {
return enableSharingWithAwsOrganization(EnableSharingWithAwsOrganizationRequest.builder()
.applyMutation(enableSharingWithAwsOrganizationRequest).build());
}
/**
*
* Enables resource sharing within your organization in Organizations. Calling this operation enables RAM to
* retrieve information about the organization and its structure. This lets you share resources with all of the
* accounts in an organization by specifying the organization's ID, or all of the accounts in an organizational unit
* (OU) by specifying the OU's ID. Until you enable sharing within the organization, you can specify only individual
* Amazon Web Services accounts, or for supported resource types, IAM users and roles.
*
*
* You must call this operation from an IAM user or role in the organization's management account.
*
*
* @return A Java Future containing the result of the EnableSharingWithAwsOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.EnableSharingWithAwsOrganization
* @see AWS API Documentation
*/
default CompletableFuture enableSharingWithAwsOrganization() {
return enableSharingWithAwsOrganization(EnableSharingWithAwsOrganizationRequest.builder().build());
}
/**
*
* Gets the contents of an RAM permission in JSON format.
*
*
* @param getPermissionRequest
* @return A Java Future containing the result of the GetPermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetPermission
* @see AWS API
* Documentation
*/
default CompletableFuture getPermission(GetPermissionRequest getPermissionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the contents of an RAM permission in JSON format.
*
*
*
* This is a convenience which creates an instance of the {@link GetPermissionRequest.Builder} avoiding the need to
* create one manually via {@link GetPermissionRequest#builder()}
*
*
* @param getPermissionRequest
* A {@link Consumer} that will call methods on {@link GetPermissionRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetPermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetPermission
* @see AWS API
* Documentation
*/
default CompletableFuture getPermission(Consumer getPermissionRequest) {
return getPermission(GetPermissionRequest.builder().applyMutation(getPermissionRequest).build());
}
/**
*
* Retrieves the resource policies for the specified resources that you own and have shared.
*
*
* @param getResourcePoliciesRequest
* @return A Java Future containing the result of the GetResourcePolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourcePolicies
* @see AWS API
* Documentation
*/
default CompletableFuture getResourcePolicies(
GetResourcePoliciesRequest getResourcePoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource policies for the specified resources that you own and have shared.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcePoliciesRequest.Builder} avoiding the
* need to create one manually via {@link GetResourcePoliciesRequest#builder()}
*
*
* @param getResourcePoliciesRequest
* A {@link Consumer} that will call methods on {@link GetResourcePoliciesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetResourcePolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourcePolicies
* @see AWS API
* Documentation
*/
default CompletableFuture getResourcePolicies(
Consumer getResourcePoliciesRequest) {
return getResourcePolicies(GetResourcePoliciesRequest.builder().applyMutation(getResourcePoliciesRequest).build());
}
/**
*
* Retrieves the resource policies for the specified resources that you own and have shared.
*
*
*
* This is a variant of
* {@link #getResourcePolicies(software.amazon.awssdk.services.ram.model.GetResourcePoliciesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourcePoliciesPublisher publisher = client.getResourcePoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourcePoliciesPublisher publisher = client.getResourcePoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourcePoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourcePolicies(software.amazon.awssdk.services.ram.model.GetResourcePoliciesRequest)} operation.
*
*
* @param getResourcePoliciesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourcePolicies
* @see AWS API
* Documentation
*/
default GetResourcePoliciesPublisher getResourcePoliciesPaginator(GetResourcePoliciesRequest getResourcePoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource policies for the specified resources that you own and have shared.
*
*
*
* This is a variant of
* {@link #getResourcePolicies(software.amazon.awssdk.services.ram.model.GetResourcePoliciesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourcePoliciesPublisher publisher = client.getResourcePoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourcePoliciesPublisher publisher = client.getResourcePoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourcePoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourcePolicies(software.amazon.awssdk.services.ram.model.GetResourcePoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetResourcePoliciesRequest.Builder} avoiding the
* need to create one manually via {@link GetResourcePoliciesRequest#builder()}
*
*
* @param getResourcePoliciesRequest
* A {@link Consumer} that will call methods on {@link GetResourcePoliciesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourcePolicies
* @see AWS API
* Documentation
*/
default GetResourcePoliciesPublisher getResourcePoliciesPaginator(
Consumer getResourcePoliciesRequest) {
return getResourcePoliciesPaginator(GetResourcePoliciesRequest.builder().applyMutation(getResourcePoliciesRequest)
.build());
}
/**
*
* Retrieves the resource and principal associations for resource shares that you own.
*
*
* @param getResourceShareAssociationsRequest
* @return A Java Future containing the result of the GetResourceShareAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareAssociations
* @see AWS API Documentation
*/
default CompletableFuture getResourceShareAssociations(
GetResourceShareAssociationsRequest getResourceShareAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource and principal associations for resource shares that you own.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceShareAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetResourceShareAssociationsRequest#builder()}
*
*
* @param getResourceShareAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetResourceShareAssociationsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetResourceShareAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareAssociations
* @see AWS API Documentation
*/
default CompletableFuture getResourceShareAssociations(
Consumer getResourceShareAssociationsRequest) {
return getResourceShareAssociations(GetResourceShareAssociationsRequest.builder()
.applyMutation(getResourceShareAssociationsRequest).build());
}
/**
*
* Retrieves the resource and principal associations for resource shares that you own.
*
*
*
* This is a variant of
* {@link #getResourceShareAssociations(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareAssociationsPublisher publisher = client.getResourceShareAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareAssociationsPublisher publisher = client.getResourceShareAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShareAssociations(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsRequest)}
* operation.
*
*
* @param getResourceShareAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareAssociations
* @see AWS API Documentation
*/
default GetResourceShareAssociationsPublisher getResourceShareAssociationsPaginator(
GetResourceShareAssociationsRequest getResourceShareAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resource and principal associations for resource shares that you own.
*
*
*
* This is a variant of
* {@link #getResourceShareAssociations(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareAssociationsPublisher publisher = client.getResourceShareAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareAssociationsPublisher publisher = client.getResourceShareAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShareAssociations(software.amazon.awssdk.services.ram.model.GetResourceShareAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetResourceShareAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetResourceShareAssociationsRequest#builder()}
*
*
* @param getResourceShareAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetResourceShareAssociationsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareAssociations
* @see AWS API Documentation
*/
default GetResourceShareAssociationsPublisher getResourceShareAssociationsPaginator(
Consumer getResourceShareAssociationsRequest) {
return getResourceShareAssociationsPaginator(GetResourceShareAssociationsRequest.builder()
.applyMutation(getResourceShareAssociationsRequest).build());
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
* @param getResourceShareInvitationsRequest
* @return A Java Future containing the result of the GetResourceShareInvitations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default CompletableFuture getResourceShareInvitations(
GetResourceShareInvitationsRequest getResourceShareInvitationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceShareInvitationsRequest.Builder}
* avoiding the need to create one manually via {@link GetResourceShareInvitationsRequest#builder()}
*
*
* @param getResourceShareInvitationsRequest
* A {@link Consumer} that will call methods on {@link GetResourceShareInvitationsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetResourceShareInvitations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default CompletableFuture getResourceShareInvitations(
Consumer getResourceShareInvitationsRequest) {
return getResourceShareInvitations(GetResourceShareInvitationsRequest.builder()
.applyMutation(getResourceShareInvitationsRequest).build());
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
* @return A Java Future containing the result of the GetResourceShareInvitations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default CompletableFuture getResourceShareInvitations() {
return getResourceShareInvitations(GetResourceShareInvitationsRequest.builder().build());
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
*
* This is a variant of
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default GetResourceShareInvitationsPublisher getResourceShareInvitationsPaginator() {
return getResourceShareInvitationsPaginator(GetResourceShareInvitationsRequest.builder().build());
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
*
* This is a variant of
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation.
*
*
* @param getResourceShareInvitationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default GetResourceShareInvitationsPublisher getResourceShareInvitationsPaginator(
GetResourceShareInvitationsRequest getResourceShareInvitationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
*
* This is a variant of
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceShareInvitationsPublisher publisher = client.getResourceShareInvitationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShareInvitations(software.amazon.awssdk.services.ram.model.GetResourceShareInvitationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetResourceShareInvitationsRequest.Builder}
* avoiding the need to create one manually via {@link GetResourceShareInvitationsRequest#builder()}
*
*
* @param getResourceShareInvitationsRequest
* A {@link Consumer} that will call methods on {@link GetResourceShareInvitationsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - InvalidMaxResultsException The specified value for
MaxResults
is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShareInvitations
* @see AWS API Documentation
*/
default GetResourceShareInvitationsPublisher getResourceShareInvitationsPaginator(
Consumer getResourceShareInvitationsRequest) {
return getResourceShareInvitationsPaginator(GetResourceShareInvitationsRequest.builder()
.applyMutation(getResourceShareInvitationsRequest).build());
}
/**
*
* Retrieves details about the resource shares that you own or that are shared with you.
*
*
* @param getResourceSharesRequest
* @return A Java Future containing the result of the GetResourceShares operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShares
* @see AWS API
* Documentation
*/
default CompletableFuture getResourceShares(GetResourceSharesRequest getResourceSharesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details about the resource shares that you own or that are shared with you.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourceSharesRequest.Builder} avoiding the need
* to create one manually via {@link GetResourceSharesRequest#builder()}
*
*
* @param getResourceSharesRequest
* A {@link Consumer} that will call methods on {@link GetResourceSharesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetResourceShares operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShares
* @see AWS API
* Documentation
*/
default CompletableFuture getResourceShares(
Consumer getResourceSharesRequest) {
return getResourceShares(GetResourceSharesRequest.builder().applyMutation(getResourceSharesRequest).build());
}
/**
*
* Retrieves details about the resource shares that you own or that are shared with you.
*
*
*
* This is a variant of
* {@link #getResourceShares(software.amazon.awssdk.services.ram.model.GetResourceSharesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceSharesPublisher publisher = client.getResourceSharesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceSharesPublisher publisher = client.getResourceSharesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceSharesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShares(software.amazon.awssdk.services.ram.model.GetResourceSharesRequest)} operation.
*
*
* @param getResourceSharesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShares
* @see AWS API
* Documentation
*/
default GetResourceSharesPublisher getResourceSharesPaginator(GetResourceSharesRequest getResourceSharesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details about the resource shares that you own or that are shared with you.
*
*
*
* This is a variant of
* {@link #getResourceShares(software.amazon.awssdk.services.ram.model.GetResourceSharesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceSharesPublisher publisher = client.getResourceSharesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.GetResourceSharesPublisher publisher = client.getResourceSharesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.GetResourceSharesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getResourceShares(software.amazon.awssdk.services.ram.model.GetResourceSharesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetResourceSharesRequest.Builder} avoiding the need
* to create one manually via {@link GetResourceSharesRequest#builder()}
*
*
* @param getResourceSharesRequest
* A {@link Consumer} that will call methods on {@link GetResourceSharesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.GetResourceShares
* @see AWS API
* Documentation
*/
default GetResourceSharesPublisher getResourceSharesPaginator(
Consumer getResourceSharesRequest) {
return getResourceSharesPaginator(GetResourceSharesRequest.builder().applyMutation(getResourceSharesRequest).build());
}
/**
*
* Lists the resources in a resource share that is shared with you but for which the invitation is still
* PENDING
. That means that you haven't accepted or rejected the invitation and the invitation hasn't
* expired.
*
*
* @param listPendingInvitationResourcesRequest
* @return A Java Future containing the result of the ListPendingInvitationResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - MissingRequiredParameterException A required input parameter is missing.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPendingInvitationResources
* @see AWS API Documentation
*/
default CompletableFuture listPendingInvitationResources(
ListPendingInvitationResourcesRequest listPendingInvitationResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resources in a resource share that is shared with you but for which the invitation is still
* PENDING
. That means that you haven't accepted or rejected the invitation and the invitation hasn't
* expired.
*
*
*
* This is a convenience which creates an instance of the {@link ListPendingInvitationResourcesRequest.Builder}
* avoiding the need to create one manually via {@link ListPendingInvitationResourcesRequest#builder()}
*
*
* @param listPendingInvitationResourcesRequest
* A {@link Consumer} that will call methods on {@link ListPendingInvitationResourcesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListPendingInvitationResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - MissingRequiredParameterException A required input parameter is missing.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPendingInvitationResources
* @see AWS API Documentation
*/
default CompletableFuture listPendingInvitationResources(
Consumer listPendingInvitationResourcesRequest) {
return listPendingInvitationResources(ListPendingInvitationResourcesRequest.builder()
.applyMutation(listPendingInvitationResourcesRequest).build());
}
/**
*
* Lists the resources in a resource share that is shared with you but for which the invitation is still
* PENDING
. That means that you haven't accepted or rejected the invitation and the invitation hasn't
* expired.
*
*
*
* This is a variant of
* {@link #listPendingInvitationResources(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPendingInvitationResourcesPublisher publisher = client.listPendingInvitationResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPendingInvitationResourcesPublisher publisher = client.listPendingInvitationResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPendingInvitationResources(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesRequest)}
* operation.
*
*
* @param listPendingInvitationResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - MissingRequiredParameterException A required input parameter is missing.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPendingInvitationResources
* @see AWS API Documentation
*/
default ListPendingInvitationResourcesPublisher listPendingInvitationResourcesPaginator(
ListPendingInvitationResourcesRequest listPendingInvitationResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resources in a resource share that is shared with you but for which the invitation is still
* PENDING
. That means that you haven't accepted or rejected the invitation and the invitation hasn't
* expired.
*
*
*
* This is a variant of
* {@link #listPendingInvitationResources(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPendingInvitationResourcesPublisher publisher = client.listPendingInvitationResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPendingInvitationResourcesPublisher publisher = client.listPendingInvitationResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPendingInvitationResources(software.amazon.awssdk.services.ram.model.ListPendingInvitationResourcesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPendingInvitationResourcesRequest.Builder}
* avoiding the need to create one manually via {@link ListPendingInvitationResourcesRequest#builder()}
*
*
* @param listPendingInvitationResourcesRequest
* A {@link Consumer} that will call methods on {@link ListPendingInvitationResourcesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - MissingRequiredParameterException A required input parameter is missing.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPendingInvitationResources
* @see AWS API Documentation
*/
default ListPendingInvitationResourcesPublisher listPendingInvitationResourcesPaginator(
Consumer listPendingInvitationResourcesRequest) {
return listPendingInvitationResourcesPaginator(ListPendingInvitationResourcesRequest.builder()
.applyMutation(listPendingInvitationResourcesRequest).build());
}
/**
*
* Lists the available versions of the specified RAM permission.
*
*
* @param listPermissionVersionsRequest
* @return A Java Future containing the result of the ListPermissionVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissionVersions
* @see AWS
* API Documentation
*/
default CompletableFuture listPermissionVersions(
ListPermissionVersionsRequest listPermissionVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available versions of the specified RAM permission.
*
*
*
* This is a convenience which creates an instance of the {@link ListPermissionVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPermissionVersionsRequest#builder()}
*
*
* @param listPermissionVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPermissionVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissionVersions
* @see AWS
* API Documentation
*/
default CompletableFuture listPermissionVersions(
Consumer listPermissionVersionsRequest) {
return listPermissionVersions(ListPermissionVersionsRequest.builder().applyMutation(listPermissionVersionsRequest)
.build());
}
/**
*
* Lists the available versions of the specified RAM permission.
*
*
*
* This is a variant of
* {@link #listPermissionVersions(software.amazon.awssdk.services.ram.model.ListPermissionVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionVersionsPublisher publisher = client.listPermissionVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionVersionsPublisher publisher = client.listPermissionVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPermissionVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissionVersions(software.amazon.awssdk.services.ram.model.ListPermissionVersionsRequest)}
* operation.
*
*
* @param listPermissionVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissionVersions
* @see AWS
* API Documentation
*/
default ListPermissionVersionsPublisher listPermissionVersionsPaginator(
ListPermissionVersionsRequest listPermissionVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available versions of the specified RAM permission.
*
*
*
* This is a variant of
* {@link #listPermissionVersions(software.amazon.awssdk.services.ram.model.ListPermissionVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionVersionsPublisher publisher = client.listPermissionVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionVersionsPublisher publisher = client.listPermissionVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPermissionVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissionVersions(software.amazon.awssdk.services.ram.model.ListPermissionVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPermissionVersionsRequest.Builder} avoiding the
* need to create one manually via {@link ListPermissionVersionsRequest#builder()}
*
*
* @param listPermissionVersionsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionVersionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissionVersions
* @see AWS
* API Documentation
*/
default ListPermissionVersionsPublisher listPermissionVersionsPaginator(
Consumer listPermissionVersionsRequest) {
return listPermissionVersionsPaginator(ListPermissionVersionsRequest.builder()
.applyMutation(listPermissionVersionsRequest).build());
}
/**
*
* Retrieves a list of available RAM permissions that you can use for the supported resource types.
*
*
* @param listPermissionsRequest
* @return A Java Future containing the result of the ListPermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissions
* @see AWS API
* Documentation
*/
default CompletableFuture listPermissions(ListPermissionsRequest listPermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of available RAM permissions that you can use for the supported resource types.
*
*
*
* This is a convenience which creates an instance of the {@link ListPermissionsRequest.Builder} avoiding the need
* to create one manually via {@link ListPermissionsRequest#builder()}
*
*
* @param listPermissionsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListPermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissions
* @see AWS API
* Documentation
*/
default CompletableFuture listPermissions(
Consumer listPermissionsRequest) {
return listPermissions(ListPermissionsRequest.builder().applyMutation(listPermissionsRequest).build());
}
/**
*
* Retrieves a list of available RAM permissions that you can use for the supported resource types.
*
*
*
* This is a variant of {@link #listPermissions(software.amazon.awssdk.services.ram.model.ListPermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionsPublisher publisher = client.listPermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionsPublisher publisher = client.listPermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissions(software.amazon.awssdk.services.ram.model.ListPermissionsRequest)} operation.
*
*
* @param listPermissionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissions
* @see AWS API
* Documentation
*/
default ListPermissionsPublisher listPermissionsPaginator(ListPermissionsRequest listPermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of available RAM permissions that you can use for the supported resource types.
*
*
*
* This is a variant of {@link #listPermissions(software.amazon.awssdk.services.ram.model.ListPermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionsPublisher publisher = client.listPermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPermissionsPublisher publisher = client.listPermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissions(software.amazon.awssdk.services.ram.model.ListPermissionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPermissionsRequest.Builder} avoiding the need
* to create one manually via {@link ListPermissionsRequest#builder()}
*
*
* @param listPermissionsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPermissions
* @see AWS API
* Documentation
*/
default ListPermissionsPublisher listPermissionsPaginator(Consumer listPermissionsRequest) {
return listPermissionsPaginator(ListPermissionsRequest.builder().applyMutation(listPermissionsRequest).build());
}
/**
*
* Lists the principals that you are sharing resources with or that are sharing resources with you.
*
*
* @param listPrincipalsRequest
* @return A Java Future containing the result of the ListPrincipals operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPrincipals
* @see AWS API
* Documentation
*/
default CompletableFuture listPrincipals(ListPrincipalsRequest listPrincipalsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the principals that you are sharing resources with or that are sharing resources with you.
*
*
*
* This is a convenience which creates an instance of the {@link ListPrincipalsRequest.Builder} avoiding the need to
* create one manually via {@link ListPrincipalsRequest#builder()}
*
*
* @param listPrincipalsRequest
* A {@link Consumer} that will call methods on {@link ListPrincipalsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListPrincipals operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPrincipals
* @see AWS API
* Documentation
*/
default CompletableFuture listPrincipals(Consumer listPrincipalsRequest) {
return listPrincipals(ListPrincipalsRequest.builder().applyMutation(listPrincipalsRequest).build());
}
/**
*
* Lists the principals that you are sharing resources with or that are sharing resources with you.
*
*
*
* This is a variant of {@link #listPrincipals(software.amazon.awssdk.services.ram.model.ListPrincipalsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPrincipalsPublisher publisher = client.listPrincipalsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPrincipalsPublisher publisher = client.listPrincipalsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPrincipalsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPrincipals(software.amazon.awssdk.services.ram.model.ListPrincipalsRequest)} operation.
*
*
* @param listPrincipalsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPrincipals
* @see AWS API
* Documentation
*/
default ListPrincipalsPublisher listPrincipalsPaginator(ListPrincipalsRequest listPrincipalsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the principals that you are sharing resources with or that are sharing resources with you.
*
*
*
* This is a variant of {@link #listPrincipals(software.amazon.awssdk.services.ram.model.ListPrincipalsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPrincipalsPublisher publisher = client.listPrincipalsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListPrincipalsPublisher publisher = client.listPrincipalsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListPrincipalsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPrincipals(software.amazon.awssdk.services.ram.model.ListPrincipalsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPrincipalsRequest.Builder} avoiding the need to
* create one manually via {@link ListPrincipalsRequest#builder()}
*
*
* @param listPrincipalsRequest
* A {@link Consumer} that will call methods on {@link ListPrincipalsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListPrincipals
* @see AWS API
* Documentation
*/
default ListPrincipalsPublisher listPrincipalsPaginator(Consumer listPrincipalsRequest) {
return listPrincipalsPaginator(ListPrincipalsRequest.builder().applyMutation(listPrincipalsRequest).build());
}
/**
*
* Lists the RAM permissions that are associated with a resource share.
*
*
* @param listResourceSharePermissionsRequest
* @return A Java Future containing the result of the ListResourceSharePermissions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceSharePermissions
* @see AWS API Documentation
*/
default CompletableFuture listResourceSharePermissions(
ListResourceSharePermissionsRequest listResourceSharePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the RAM permissions that are associated with a resource share.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourceSharePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link ListResourceSharePermissionsRequest#builder()}
*
*
* @param listResourceSharePermissionsRequest
* A {@link Consumer} that will call methods on {@link ListResourceSharePermissionsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListResourceSharePermissions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceSharePermissions
* @see AWS API Documentation
*/
default CompletableFuture listResourceSharePermissions(
Consumer listResourceSharePermissionsRequest) {
return listResourceSharePermissions(ListResourceSharePermissionsRequest.builder()
.applyMutation(listResourceSharePermissionsRequest).build());
}
/**
*
* Lists the RAM permissions that are associated with a resource share.
*
*
*
* This is a variant of
* {@link #listResourceSharePermissions(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceSharePermissionsPublisher publisher = client.listResourceSharePermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceSharePermissionsPublisher publisher = client.listResourceSharePermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourceSharePermissions(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsRequest)}
* operation.
*
*
* @param listResourceSharePermissionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceSharePermissions
* @see AWS API Documentation
*/
default ListResourceSharePermissionsPublisher listResourceSharePermissionsPaginator(
ListResourceSharePermissionsRequest listResourceSharePermissionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the RAM permissions that are associated with a resource share.
*
*
*
* This is a variant of
* {@link #listResourceSharePermissions(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceSharePermissionsPublisher publisher = client.listResourceSharePermissionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceSharePermissionsPublisher publisher = client.listResourceSharePermissionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourceSharePermissions(software.amazon.awssdk.services.ram.model.ListResourceSharePermissionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListResourceSharePermissionsRequest.Builder}
* avoiding the need to create one manually via {@link ListResourceSharePermissionsRequest#builder()}
*
*
* @param listResourceSharePermissionsRequest
* A {@link Consumer} that will call methods on {@link ListResourceSharePermissionsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - OperationNotPermittedException The requested operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceSharePermissions
* @see AWS API Documentation
*/
default ListResourceSharePermissionsPublisher listResourceSharePermissionsPaginator(
Consumer listResourceSharePermissionsRequest) {
return listResourceSharePermissionsPaginator(ListResourceSharePermissionsRequest.builder()
.applyMutation(listResourceSharePermissionsRequest).build());
}
/**
*
* Lists the resource types that can be shared by RAM.
*
*
* @param listResourceTypesRequest
* @return A Java Future containing the result of the ListResourceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceTypes
* @see AWS API
* Documentation
*/
default CompletableFuture listResourceTypes(ListResourceTypesRequest listResourceTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resource types that can be shared by RAM.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourceTypesRequest.Builder} avoiding the need
* to create one manually via {@link ListResourceTypesRequest#builder()}
*
*
* @param listResourceTypesRequest
* A {@link Consumer} that will call methods on {@link ListResourceTypesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListResourceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceTypes
* @see AWS API
* Documentation
*/
default CompletableFuture listResourceTypes(
Consumer listResourceTypesRequest) {
return listResourceTypes(ListResourceTypesRequest.builder().applyMutation(listResourceTypesRequest).build());
}
/**
*
* Lists the resource types that can be shared by RAM.
*
*
*
* This is a variant of
* {@link #listResourceTypes(software.amazon.awssdk.services.ram.model.ListResourceTypesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceTypesPublisher publisher = client.listResourceTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceTypesPublisher publisher = client.listResourceTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourceTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourceTypes(software.amazon.awssdk.services.ram.model.ListResourceTypesRequest)} operation.
*
*
* @param listResourceTypesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceTypes
* @see AWS API
* Documentation
*/
default ListResourceTypesPublisher listResourceTypesPaginator(ListResourceTypesRequest listResourceTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resource types that can be shared by RAM.
*
*
*
* This is a variant of
* {@link #listResourceTypes(software.amazon.awssdk.services.ram.model.ListResourceTypesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceTypesPublisher publisher = client.listResourceTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourceTypesPublisher publisher = client.listResourceTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourceTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourceTypes(software.amazon.awssdk.services.ram.model.ListResourceTypesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListResourceTypesRequest.Builder} avoiding the need
* to create one manually via {@link ListResourceTypesRequest#builder()}
*
*
* @param listResourceTypesRequest
* A {@link Consumer} that will call methods on {@link ListResourceTypesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResourceTypes
* @see AWS API
* Documentation
*/
default ListResourceTypesPublisher listResourceTypesPaginator(
Consumer listResourceTypesRequest) {
return listResourceTypesPaginator(ListResourceTypesRequest.builder().applyMutation(listResourceTypesRequest).build());
}
/**
*
* Lists the resources that you added to a resource share or the resources that are shared with you.
*
*
* @param listResourcesRequest
* @return A Java Future containing the result of the ListResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidResourceTypeException The specified resource type is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default CompletableFuture listResources(ListResourcesRequest listResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resources that you added to a resource share or the resources that are shared with you.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourcesRequest.Builder} avoiding the need to
* create one manually via {@link ListResourcesRequest#builder()}
*
*
* @param listResourcesRequest
* A {@link Consumer} that will call methods on {@link ListResourcesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidResourceTypeException The specified resource type is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default CompletableFuture listResources(Consumer listResourcesRequest) {
return listResources(ListResourcesRequest.builder().applyMutation(listResourcesRequest).build());
}
/**
*
* Lists the resources that you added to a resource share or the resources that are shared with you.
*
*
*
* This is a variant of {@link #listResources(software.amazon.awssdk.services.ram.model.ListResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourcesPublisher publisher = client.listResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourcesPublisher publisher = client.listResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResources(software.amazon.awssdk.services.ram.model.ListResourcesRequest)} operation.
*
*
* @param listResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidResourceTypeException The specified resource type is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default ListResourcesPublisher listResourcesPaginator(ListResourcesRequest listResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the resources that you added to a resource share or the resources that are shared with you.
*
*
*
* This is a variant of {@link #listResources(software.amazon.awssdk.services.ram.model.ListResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourcesPublisher publisher = client.listResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ram.paginators.ListResourcesPublisher publisher = client.listResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ram.model.ListResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResources(software.amazon.awssdk.services.ram.model.ListResourcesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListResourcesRequest.Builder} avoiding the need to
* create one manually via {@link ListResourcesRequest#builder()}
*
*
* @param listResourcesRequest
* A {@link Consumer} that will call methods on {@link ListResourcesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidResourceTypeException The specified resource type is not valid.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidNextTokenException The specified value for
NextToken
is not valid.
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.ListResources
* @see AWS API
* Documentation
*/
default ListResourcesPublisher listResourcesPaginator(Consumer listResourcesRequest) {
return listResourcesPaginator(ListResourcesRequest.builder().applyMutation(listResourcesRequest).build());
}
/**
*
* When you attach a resource-based permission policy to a resource, it automatically creates a resource share.
* However, resource shares created this way are visible only to the resource share owner, and the resource share
* can't be modified in RAM.
*
*
* You can use this operation to promote the resource share to a full RAM resource share. When you promote a
* resource share, you can then manage the resource share in RAM and it becomes visible to all of the principals you
* shared it with.
*
*
* @param promoteResourceShareCreatedFromPolicyRequest
* @return A Java Future containing the result of the PromoteResourceShareCreatedFromPolicy operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - MissingRequiredParameterException A required input parameter is missing.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.PromoteResourceShareCreatedFromPolicy
* @see AWS API Documentation
*/
default CompletableFuture promoteResourceShareCreatedFromPolicy(
PromoteResourceShareCreatedFromPolicyRequest promoteResourceShareCreatedFromPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* When you attach a resource-based permission policy to a resource, it automatically creates a resource share.
* However, resource shares created this way are visible only to the resource share owner, and the resource share
* can't be modified in RAM.
*
*
* You can use this operation to promote the resource share to a full RAM resource share. When you promote a
* resource share, you can then manage the resource share in RAM and it becomes visible to all of the principals you
* shared it with.
*
*
*
* This is a convenience which creates an instance of the
* {@link PromoteResourceShareCreatedFromPolicyRequest.Builder} avoiding the need to create one manually via
* {@link PromoteResourceShareCreatedFromPolicyRequest#builder()}
*
*
* @param promoteResourceShareCreatedFromPolicyRequest
* A {@link Consumer} that will call methods on {@link PromoteResourceShareCreatedFromPolicyRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the PromoteResourceShareCreatedFromPolicy operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - ResourceShareLimitExceededException This request would exceed the limit for resource shares for your
* account.
* - OperationNotPermittedException The requested operation is not permitted.
* - InvalidParameterException A parameter is not valid.
* - MissingRequiredParameterException A required input parameter is missing.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - UnknownResourceException A specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.PromoteResourceShareCreatedFromPolicy
* @see AWS API Documentation
*/
default CompletableFuture promoteResourceShareCreatedFromPolicy(
Consumer promoteResourceShareCreatedFromPolicyRequest) {
return promoteResourceShareCreatedFromPolicy(PromoteResourceShareCreatedFromPolicyRequest.builder()
.applyMutation(promoteResourceShareCreatedFromPolicyRequest).build());
}
/**
*
* Rejects an invitation to a resource share from another Amazon Web Services account.
*
*
* @param rejectResourceShareInvitationRequest
* @return A Java Future containing the result of the RejectResourceShareInvitation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - ResourceShareInvitationAlreadyAcceptedException The specified invitation was already accepted.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - InvalidClientTokenException The client token is not valid.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.RejectResourceShareInvitation
* @see AWS API Documentation
*/
default CompletableFuture rejectResourceShareInvitation(
RejectResourceShareInvitationRequest rejectResourceShareInvitationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Rejects an invitation to a resource share from another Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link RejectResourceShareInvitationRequest.Builder}
* avoiding the need to create one manually via {@link RejectResourceShareInvitationRequest#builder()}
*
*
* @param rejectResourceShareInvitationRequest
* A {@link Consumer} that will call methods on {@link RejectResourceShareInvitationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the RejectResourceShareInvitation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ResourceShareInvitationArnNotFoundException The specified Amazon Resource Name (ARN) for an
* invitation was not found.
* - ResourceShareInvitationAlreadyAcceptedException The specified invitation was already accepted.
* - ResourceShareInvitationAlreadyRejectedException The specified invitation was already rejected.
* - ResourceShareInvitationExpiredException The specified invitation is expired.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - InvalidClientTokenException The client token is not valid.
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.RejectResourceShareInvitation
* @see AWS API Documentation
*/
default CompletableFuture rejectResourceShareInvitation(
Consumer rejectResourceShareInvitationRequest) {
return rejectResourceShareInvitation(RejectResourceShareInvitationRequest.builder()
.applyMutation(rejectResourceShareInvitationRequest).build());
}
/**
*
* Adds the specified tag keys and values to the specified resource share. The tags are attached only to the
* resource share, not to the resources that are in the resource share.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - TagLimitExceededException This request would exceed the limit for tags for your account.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - TagPolicyViolationException The specified tag key is a reserved word and can't be used.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds the specified tag keys and values to the specified resource share. The tags are attached only to the
* resource share, not to the resources that are in the resource share.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - UnknownResourceException A specified resource was not found.
* - TagLimitExceededException This request would exceed the limit for tags for your account.
* - ResourceArnNotFoundException The specified Amazon Resource Name (ARN) was not found.
* - TagPolicyViolationException The specified tag key is a reserved word and can't be used.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes the specified tag key and value pairs from the specified resource share.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tag key and value pairs from the specified resource share.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter is not valid.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Modifies some of the properties of the specified resource share.
*
*
* @param updateResourceShareRequest
* @return A Java Future containing the result of the UpdateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - MissingRequiredParameterException A required input parameter is missing.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.UpdateResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture updateResourceShare(
UpdateResourceShareRequest updateResourceShareRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies some of the properties of the specified resource share.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateResourceShareRequest.Builder} avoiding the
* need to create one manually via {@link UpdateResourceShareRequest#builder()}
*
*
* @param updateResourceShareRequest
* A {@link Consumer} that will call methods on {@link UpdateResourceShareRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateResourceShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - IdempotentParameterMismatchException The client token input parameter was matched one used with a
* previous call to the operation, but at least one of the other input parameters is different from the
* previous call.
* - MissingRequiredParameterException A required input parameter is missing.
* - UnknownResourceException A specified resource was not found.
* - MalformedArnException The format of an Amazon Resource Name (ARN) is not valid.
* - InvalidClientTokenException The client token is not valid.
* - InvalidParameterException A parameter is not valid.
* - OperationNotPermittedException The requested operation is not permitted.
* - ServerInternalException The service could not respond to the request due to an internal problem.
* - ServiceUnavailableException The service is not available.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RamException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RamAsyncClient.UpdateResourceShare
* @see AWS API
* Documentation
*/
default CompletableFuture updateResourceShare(
Consumer updateResourceShareRequest) {
return updateResourceShare(UpdateResourceShareRequest.builder().applyMutation(updateResourceShareRequest).build());
}
}