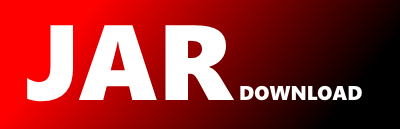
software.amazon.awssdk.services.ram.model.ResourceShareAssociation Maven / Gradle / Ivy
Show all versions of ram Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ram.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an association with a resource share and either a principal or a resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResourceShareAssociation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RESOURCE_SHARE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceShareArn").getter(getter(ResourceShareAssociation::resourceShareArn))
.setter(setter(Builder::resourceShareArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceShareArn").build()).build();
private static final SdkField RESOURCE_SHARE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceShareName").getter(getter(ResourceShareAssociation::resourceShareName))
.setter(setter(Builder::resourceShareName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceShareName").build()).build();
private static final SdkField ASSOCIATED_ENTITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("associatedEntity").getter(getter(ResourceShareAssociation::associatedEntity))
.setter(setter(Builder::associatedEntity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("associatedEntity").build()).build();
private static final SdkField ASSOCIATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("associationType").getter(getter(ResourceShareAssociation::associationTypeAsString))
.setter(setter(Builder::associationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("associationType").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ResourceShareAssociation::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField STATUS_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("statusMessage").getter(getter(ResourceShareAssociation::statusMessage))
.setter(setter(Builder::statusMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("statusMessage").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("creationTime").getter(getter(ResourceShareAssociation::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationTime").build()).build();
private static final SdkField LAST_UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastUpdatedTime").getter(getter(ResourceShareAssociation::lastUpdatedTime))
.setter(setter(Builder::lastUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedTime").build()).build();
private static final SdkField EXTERNAL_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("external").getter(getter(ResourceShareAssociation::external)).setter(setter(Builder::external))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("external").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RESOURCE_SHARE_ARN_FIELD,
RESOURCE_SHARE_NAME_FIELD, ASSOCIATED_ENTITY_FIELD, ASSOCIATION_TYPE_FIELD, STATUS_FIELD, STATUS_MESSAGE_FIELD,
CREATION_TIME_FIELD, LAST_UPDATED_TIME_FIELD, EXTERNAL_FIELD));
private static final long serialVersionUID = 1L;
private final String resourceShareArn;
private final String resourceShareName;
private final String associatedEntity;
private final String associationType;
private final String status;
private final String statusMessage;
private final Instant creationTime;
private final Instant lastUpdatedTime;
private final Boolean external;
private ResourceShareAssociation(BuilderImpl builder) {
this.resourceShareArn = builder.resourceShareArn;
this.resourceShareName = builder.resourceShareName;
this.associatedEntity = builder.associatedEntity;
this.associationType = builder.associationType;
this.status = builder.status;
this.statusMessage = builder.statusMessage;
this.creationTime = builder.creationTime;
this.lastUpdatedTime = builder.lastUpdatedTime;
this.external = builder.external;
}
/**
*
* The Amazon Resoure Name
* (ARN) of the resource share.
*
*
* @return The Amazon Resoure
* Name (ARN) of the resource share.
*/
public final String resourceShareArn() {
return resourceShareArn;
}
/**
*
* The name of the resource share.
*
*
* @return The name of the resource share.
*/
public final String resourceShareName() {
return resourceShareName;
}
/**
*
* The associated entity. This can be either of the following:
*
*
* -
*
* For a resource association, this is the Amazon Resoure Name (ARN)
* of the resource.
*
*
* -
*
* For principal associations, this is one of the following:
*
*
* -
*
* The ID of an Amazon Web Services account
*
*
* -
*
* The Amazon Resoure Name
* (ARN) of an organization in Organizations
*
*
* -
*
* The ARN of an organizational unit (OU) in Organizations
*
*
* -
*
* The ARN of an IAM role
*
*
* -
*
* The ARN of an IAM user
*
*
*
*
*
*
* @return The associated entity. This can be either of the following:
*
* -
*
* For a resource association, this is the Amazon Resoure Name
* (ARN) of the resource.
*
*
* -
*
* For principal associations, this is one of the following:
*
*
* -
*
* The ID of an Amazon Web Services account
*
*
* -
*
* The Amazon Resoure
* Name (ARN) of an organization in Organizations
*
*
* -
*
* The ARN of an organizational unit (OU) in Organizations
*
*
* -
*
* The ARN of an IAM role
*
*
* -
*
* The ARN of an IAM user
*
*
*
*
*/
public final String associatedEntity() {
return associatedEntity;
}
/**
*
* The type of entity included in this association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #associationType}
* will return {@link ResourceShareAssociationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #associationTypeAsString}.
*
*
* @return The type of entity included in this association.
* @see ResourceShareAssociationType
*/
public final ResourceShareAssociationType associationType() {
return ResourceShareAssociationType.fromValue(associationType);
}
/**
*
* The type of entity included in this association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #associationType}
* will return {@link ResourceShareAssociationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #associationTypeAsString}.
*
*
* @return The type of entity included in this association.
* @see ResourceShareAssociationType
*/
public final String associationTypeAsString() {
return associationType;
}
/**
*
* The current status of the association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ResourceShareAssociationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The current status of the association.
* @see ResourceShareAssociationStatus
*/
public final ResourceShareAssociationStatus status() {
return ResourceShareAssociationStatus.fromValue(status);
}
/**
*
* The current status of the association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ResourceShareAssociationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #statusAsString}.
*
*
* @return The current status of the association.
* @see ResourceShareAssociationStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* A message about the status of the association.
*
*
* @return A message about the status of the association.
*/
public final String statusMessage() {
return statusMessage;
}
/**
*
* The date and time when the association was created.
*
*
* @return The date and time when the association was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The date and time when the association was last updated.
*
*
* @return The date and time when the association was last updated.
*/
public final Instant lastUpdatedTime() {
return lastUpdatedTime;
}
/**
*
* Indicates whether the principal belongs to the same organization in Organizations as the Amazon Web Services
* account that owns the resource share.
*
*
* @return Indicates whether the principal belongs to the same organization in Organizations as the Amazon Web
* Services account that owns the resource share.
*/
public final Boolean external() {
return external;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(resourceShareArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceShareName());
hashCode = 31 * hashCode + Objects.hashCode(associatedEntity());
hashCode = 31 * hashCode + Objects.hashCode(associationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(external());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResourceShareAssociation)) {
return false;
}
ResourceShareAssociation other = (ResourceShareAssociation) obj;
return Objects.equals(resourceShareArn(), other.resourceShareArn())
&& Objects.equals(resourceShareName(), other.resourceShareName())
&& Objects.equals(associatedEntity(), other.associatedEntity())
&& Objects.equals(associationTypeAsString(), other.associationTypeAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusMessage(), other.statusMessage()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastUpdatedTime(), other.lastUpdatedTime()) && Objects.equals(external(), other.external());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ResourceShareAssociation").add("ResourceShareArn", resourceShareArn())
.add("ResourceShareName", resourceShareName()).add("AssociatedEntity", associatedEntity())
.add("AssociationType", associationTypeAsString()).add("Status", statusAsString())
.add("StatusMessage", statusMessage()).add("CreationTime", creationTime())
.add("LastUpdatedTime", lastUpdatedTime()).add("External", external()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "resourceShareArn":
return Optional.ofNullable(clazz.cast(resourceShareArn()));
case "resourceShareName":
return Optional.ofNullable(clazz.cast(resourceShareName()));
case "associatedEntity":
return Optional.ofNullable(clazz.cast(associatedEntity()));
case "associationType":
return Optional.ofNullable(clazz.cast(associationTypeAsString()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "statusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "creationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "lastUpdatedTime":
return Optional.ofNullable(clazz.cast(lastUpdatedTime()));
case "external":
return Optional.ofNullable(clazz.cast(external()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function