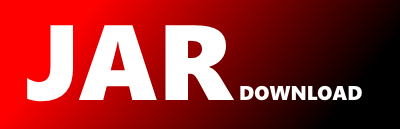
software.amazon.awssdk.services.ram.model.ResourceSharePermissionDetail Maven / Gradle / Ivy
Show all versions of ram Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ram.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a RAM managed permission.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResourceSharePermissionDetail implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(ResourceSharePermissionDetail::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("version")
.getter(getter(ResourceSharePermissionDetail::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField DEFAULT_VERSION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("defaultVersion").getter(getter(ResourceSharePermissionDetail::defaultVersion))
.setter(setter(Builder::defaultVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("defaultVersion").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(ResourceSharePermissionDetail::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceType").getter(getter(ResourceSharePermissionDetail::resourceType))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceType").build()).build();
private static final SdkField PERMISSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("permission").getter(getter(ResourceSharePermissionDetail::permission))
.setter(setter(Builder::permission))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("permission").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("creationTime").getter(getter(ResourceSharePermissionDetail::creationTime))
.setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationTime").build()).build();
private static final SdkField LAST_UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastUpdatedTime").getter(getter(ResourceSharePermissionDetail::lastUpdatedTime))
.setter(setter(Builder::lastUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedTime").build()).build();
private static final SdkField IS_RESOURCE_TYPE_DEFAULT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isResourceTypeDefault").getter(getter(ResourceSharePermissionDetail::isResourceTypeDefault))
.setter(setter(Builder::isResourceTypeDefault))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isResourceTypeDefault").build())
.build();
private static final SdkField PERMISSION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("permissionType").getter(getter(ResourceSharePermissionDetail::permissionTypeAsString))
.setter(setter(Builder::permissionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("permissionType").build()).build();
private static final SdkField FEATURE_SET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("featureSet").getter(getter(ResourceSharePermissionDetail::featureSetAsString))
.setter(setter(Builder::featureSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("featureSet").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ResourceSharePermissionDetail::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(ResourceSharePermissionDetail::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, VERSION_FIELD,
DEFAULT_VERSION_FIELD, NAME_FIELD, RESOURCE_TYPE_FIELD, PERMISSION_FIELD, CREATION_TIME_FIELD,
LAST_UPDATED_TIME_FIELD, IS_RESOURCE_TYPE_DEFAULT_FIELD, PERMISSION_TYPE_FIELD, FEATURE_SET_FIELD, STATUS_FIELD,
TAGS_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String version;
private final Boolean defaultVersion;
private final String name;
private final String resourceType;
private final String permission;
private final Instant creationTime;
private final Instant lastUpdatedTime;
private final Boolean isResourceTypeDefault;
private final String permissionType;
private final String featureSet;
private final String status;
private final List tags;
private ResourceSharePermissionDetail(BuilderImpl builder) {
this.arn = builder.arn;
this.version = builder.version;
this.defaultVersion = builder.defaultVersion;
this.name = builder.name;
this.resourceType = builder.resourceType;
this.permission = builder.permission;
this.creationTime = builder.creationTime;
this.lastUpdatedTime = builder.lastUpdatedTime;
this.isResourceTypeDefault = builder.isResourceTypeDefault;
this.permissionType = builder.permissionType;
this.featureSet = builder.featureSet;
this.status = builder.status;
this.tags = builder.tags;
}
/**
*
* The Amazon Resource Name
* (ARN) of this RAM managed permission.
*
*
* @return The Amazon Resource
* Name (ARN) of this RAM managed permission.
*/
public final String arn() {
return arn;
}
/**
*
* The version of the permission described in this response.
*
*
* @return The version of the permission described in this response.
*/
public final String version() {
return version;
}
/**
*
* Specifies whether the version of the permission represented in this response is the default version for this
* permission.
*
*
* @return Specifies whether the version of the permission represented in this response is the default version for
* this permission.
*/
public final Boolean defaultVersion() {
return defaultVersion;
}
/**
*
* The name of this permission.
*
*
* @return The name of this permission.
*/
public final String name() {
return name;
}
/**
*
* The resource type to which this permission applies.
*
*
* @return The resource type to which this permission applies.
*/
public final String resourceType() {
return resourceType;
}
/**
*
* The permission's effect and actions in JSON format. The effect
indicates whether the specified
* actions are allowed or denied. The actions
list the operations to which the principal is granted or
* denied access.
*
*
* @return The permission's effect and actions in JSON format. The effect
indicates whether the
* specified actions are allowed or denied. The actions
list the operations to which the
* principal is granted or denied access.
*/
public final String permission() {
return permission;
}
/**
*
* The date and time when the permission was created.
*
*
* @return The date and time when the permission was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The date and time when the permission was last updated.
*
*
* @return The date and time when the permission was last updated.
*/
public final Instant lastUpdatedTime() {
return lastUpdatedTime;
}
/**
*
* Specifies whether the version of the permission represented in this response is the default version for all
* resources of this resource type.
*
*
* @return Specifies whether the version of the permission represented in this response is the default version for
* all resources of this resource type.
*/
public final Boolean isResourceTypeDefault() {
return isResourceTypeDefault;
}
/**
*
* The type of managed permission. This can be one of the following values:
*
*
* -
*
* AWS_MANAGED
– Amazon Web Services created and manages this managed permission. You can associate it
* with your resource shares, but you can't modify it.
*
*
* -
*
* CUSTOMER_MANAGED
– You, or another principal in your account created this managed permission. You
* can associate it with your resource shares and create new versions that have different permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionType}
* will return {@link PermissionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionTypeAsString}.
*
*
* @return The type of managed permission. This can be one of the following values:
*
* -
*
* AWS_MANAGED
– Amazon Web Services created and manages this managed permission. You can
* associate it with your resource shares, but you can't modify it.
*
*
* -
*
* CUSTOMER_MANAGED
– You, or another principal in your account created this managed
* permission. You can associate it with your resource shares and create new versions that have different
* permissions.
*
*
* @see PermissionType
*/
public final PermissionType permissionType() {
return PermissionType.fromValue(permissionType);
}
/**
*
* The type of managed permission. This can be one of the following values:
*
*
* -
*
* AWS_MANAGED
– Amazon Web Services created and manages this managed permission. You can associate it
* with your resource shares, but you can't modify it.
*
*
* -
*
* CUSTOMER_MANAGED
– You, or another principal in your account created this managed permission. You
* can associate it with your resource shares and create new versions that have different permissions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #permissionType}
* will return {@link PermissionType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #permissionTypeAsString}.
*
*
* @return The type of managed permission. This can be one of the following values:
*
* -
*
* AWS_MANAGED
– Amazon Web Services created and manages this managed permission. You can
* associate it with your resource shares, but you can't modify it.
*
*
* -
*
* CUSTOMER_MANAGED
– You, or another principal in your account created this managed
* permission. You can associate it with your resource shares and create new versions that have different
* permissions.
*
*
* @see PermissionType
*/
public final String permissionTypeAsString() {
return permissionType;
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureSet} will
* return {@link PermissionFeatureSet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureSetAsString}.
*
*
* @return Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based
* policy. That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in
* progress. This value changes to STANDARD
when complete.
*
*
* @see PermissionFeatureSet
*/
public final PermissionFeatureSet featureSet() {
return PermissionFeatureSet.fromValue(featureSet);
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureSet} will
* return {@link PermissionFeatureSet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureSetAsString}.
*
*
* @return Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based
* policy. That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in
* progress. This value changes to STANDARD
when complete.
*
*
* @see PermissionFeatureSet
*/
public final String featureSetAsString() {
return featureSet;
}
/**
*
* The current status of the association between the permission and the resource share. The following are the
* possible values:
*
*
* -
*
* ATTACHABLE
– This permission or version can be associated with resource shares.
*
*
* -
*
* UNATTACHABLE
– This permission or version can't currently be associated with resource shares.
*
*
* -
*
* DELETING
– This permission or version is in the process of being deleted.
*
*
* -
*
* DELETED
– This permission or version is deleted.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link PermissionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the association between the permission and the resource share. The following are
* the possible values:
*
* -
*
* ATTACHABLE
– This permission or version can be associated with resource shares.
*
*
* -
*
* UNATTACHABLE
– This permission or version can't currently be associated with resource
* shares.
*
*
* -
*
* DELETING
– This permission or version is in the process of being deleted.
*
*
* -
*
* DELETED
– This permission or version is deleted.
*
*
* @see PermissionStatus
*/
public final PermissionStatus status() {
return PermissionStatus.fromValue(status);
}
/**
*
* The current status of the association between the permission and the resource share. The following are the
* possible values:
*
*
* -
*
* ATTACHABLE
– This permission or version can be associated with resource shares.
*
*
* -
*
* UNATTACHABLE
– This permission or version can't currently be associated with resource shares.
*
*
* -
*
* DELETING
– This permission or version is in the process of being deleted.
*
*
* -
*
* DELETED
– This permission or version is deleted.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link PermissionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the association between the permission and the resource share. The following are
* the possible values:
*
* -
*
* ATTACHABLE
– This permission or version can be associated with resource shares.
*
*
* -
*
* UNATTACHABLE
– This permission or version can't currently be associated with resource
* shares.
*
*
* -
*
* DELETING
– This permission or version is in the process of being deleted.
*
*
* -
*
* DELETED
– This permission or version is deleted.
*
*
* @see PermissionStatus
*/
public final String statusAsString() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tag key and value pairs attached to the resource share.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tag key and value pairs attached to the resource share.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(defaultVersion());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(permission());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(isResourceTypeDefault());
hashCode = 31 * hashCode + Objects.hashCode(permissionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(featureSetAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResourceSharePermissionDetail)) {
return false;
}
ResourceSharePermissionDetail other = (ResourceSharePermissionDetail) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(version(), other.version())
&& Objects.equals(defaultVersion(), other.defaultVersion()) && Objects.equals(name(), other.name())
&& Objects.equals(resourceType(), other.resourceType()) && Objects.equals(permission(), other.permission())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastUpdatedTime(), other.lastUpdatedTime())
&& Objects.equals(isResourceTypeDefault(), other.isResourceTypeDefault())
&& Objects.equals(permissionTypeAsString(), other.permissionTypeAsString())
&& Objects.equals(featureSetAsString(), other.featureSetAsString())
&& Objects.equals(statusAsString(), other.statusAsString()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ResourceSharePermissionDetail").add("Arn", arn()).add("Version", version())
.add("DefaultVersion", defaultVersion()).add("Name", name()).add("ResourceType", resourceType())
.add("Permission", permission()).add("CreationTime", creationTime()).add("LastUpdatedTime", lastUpdatedTime())
.add("IsResourceTypeDefault", isResourceTypeDefault()).add("PermissionType", permissionTypeAsString())
.add("FeatureSet", featureSetAsString()).add("Status", statusAsString()).add("Tags", hasTags() ? tags() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "defaultVersion":
return Optional.ofNullable(clazz.cast(defaultVersion()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "resourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "permission":
return Optional.ofNullable(clazz.cast(permission()));
case "creationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "lastUpdatedTime":
return Optional.ofNullable(clazz.cast(lastUpdatedTime()));
case "isResourceTypeDefault":
return Optional.ofNullable(clazz.cast(isResourceTypeDefault()));
case "permissionType":
return Optional.ofNullable(clazz.cast(permissionTypeAsString()));
case "featureSet":
return Optional.ofNullable(clazz.cast(featureSetAsString()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function