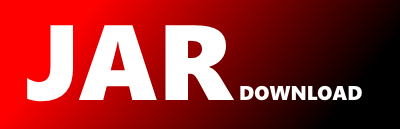
software.amazon.awssdk.services.rds.RdsAsyncClient Maven / Gradle / Ivy
Show all versions of rds Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.rds.model.AddRoleToDbClusterRequest;
import software.amazon.awssdk.services.rds.model.AddRoleToDbClusterResponse;
import software.amazon.awssdk.services.rds.model.AddRoleToDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.AddRoleToDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.AddSourceIdentifierToSubscriptionRequest;
import software.amazon.awssdk.services.rds.model.AddSourceIdentifierToSubscriptionResponse;
import software.amazon.awssdk.services.rds.model.AddTagsToResourceRequest;
import software.amazon.awssdk.services.rds.model.AddTagsToResourceResponse;
import software.amazon.awssdk.services.rds.model.ApplyPendingMaintenanceActionRequest;
import software.amazon.awssdk.services.rds.model.ApplyPendingMaintenanceActionResponse;
import software.amazon.awssdk.services.rds.model.AuthorizeDbSecurityGroupIngressRequest;
import software.amazon.awssdk.services.rds.model.AuthorizeDbSecurityGroupIngressResponse;
import software.amazon.awssdk.services.rds.model.BacktrackDbClusterRequest;
import software.amazon.awssdk.services.rds.model.BacktrackDbClusterResponse;
import software.amazon.awssdk.services.rds.model.CancelExportTaskRequest;
import software.amazon.awssdk.services.rds.model.CancelExportTaskResponse;
import software.amazon.awssdk.services.rds.model.CopyDbClusterParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.CopyDbClusterParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.CopyDbClusterSnapshotRequest;
import software.amazon.awssdk.services.rds.model.CopyDbClusterSnapshotResponse;
import software.amazon.awssdk.services.rds.model.CopyDbParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.CopyDbParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.CopyDbSnapshotRequest;
import software.amazon.awssdk.services.rds.model.CopyDbSnapshotResponse;
import software.amazon.awssdk.services.rds.model.CopyOptionGroupRequest;
import software.amazon.awssdk.services.rds.model.CopyOptionGroupResponse;
import software.amazon.awssdk.services.rds.model.CreateCustomAvailabilityZoneRequest;
import software.amazon.awssdk.services.rds.model.CreateCustomAvailabilityZoneResponse;
import software.amazon.awssdk.services.rds.model.CreateDbClusterEndpointRequest;
import software.amazon.awssdk.services.rds.model.CreateDbClusterEndpointResponse;
import software.amazon.awssdk.services.rds.model.CreateDbClusterParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.CreateDbClusterParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.CreateDbClusterRequest;
import software.amazon.awssdk.services.rds.model.CreateDbClusterResponse;
import software.amazon.awssdk.services.rds.model.CreateDbClusterSnapshotRequest;
import software.amazon.awssdk.services.rds.model.CreateDbClusterSnapshotResponse;
import software.amazon.awssdk.services.rds.model.CreateDbInstanceReadReplicaRequest;
import software.amazon.awssdk.services.rds.model.CreateDbInstanceReadReplicaResponse;
import software.amazon.awssdk.services.rds.model.CreateDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.CreateDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.CreateDbParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.CreateDbParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.CreateDbProxyRequest;
import software.amazon.awssdk.services.rds.model.CreateDbProxyResponse;
import software.amazon.awssdk.services.rds.model.CreateDbSecurityGroupRequest;
import software.amazon.awssdk.services.rds.model.CreateDbSecurityGroupResponse;
import software.amazon.awssdk.services.rds.model.CreateDbSnapshotRequest;
import software.amazon.awssdk.services.rds.model.CreateDbSnapshotResponse;
import software.amazon.awssdk.services.rds.model.CreateDbSubnetGroupRequest;
import software.amazon.awssdk.services.rds.model.CreateDbSubnetGroupResponse;
import software.amazon.awssdk.services.rds.model.CreateEventSubscriptionRequest;
import software.amazon.awssdk.services.rds.model.CreateEventSubscriptionResponse;
import software.amazon.awssdk.services.rds.model.CreateGlobalClusterRequest;
import software.amazon.awssdk.services.rds.model.CreateGlobalClusterResponse;
import software.amazon.awssdk.services.rds.model.CreateOptionGroupRequest;
import software.amazon.awssdk.services.rds.model.CreateOptionGroupResponse;
import software.amazon.awssdk.services.rds.model.DeleteCustomAvailabilityZoneRequest;
import software.amazon.awssdk.services.rds.model.DeleteCustomAvailabilityZoneResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterEndpointRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterEndpointResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterSnapshotRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbClusterSnapshotResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbInstanceAutomatedBackupRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbInstanceAutomatedBackupResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbProxyRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbProxyResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbSecurityGroupRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbSecurityGroupResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbSnapshotRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbSnapshotResponse;
import software.amazon.awssdk.services.rds.model.DeleteDbSubnetGroupRequest;
import software.amazon.awssdk.services.rds.model.DeleteDbSubnetGroupResponse;
import software.amazon.awssdk.services.rds.model.DeleteEventSubscriptionRequest;
import software.amazon.awssdk.services.rds.model.DeleteEventSubscriptionResponse;
import software.amazon.awssdk.services.rds.model.DeleteGlobalClusterRequest;
import software.amazon.awssdk.services.rds.model.DeleteGlobalClusterResponse;
import software.amazon.awssdk.services.rds.model.DeleteInstallationMediaRequest;
import software.amazon.awssdk.services.rds.model.DeleteInstallationMediaResponse;
import software.amazon.awssdk.services.rds.model.DeleteOptionGroupRequest;
import software.amazon.awssdk.services.rds.model.DeleteOptionGroupResponse;
import software.amazon.awssdk.services.rds.model.DeregisterDbProxyTargetsRequest;
import software.amazon.awssdk.services.rds.model.DeregisterDbProxyTargetsResponse;
import software.amazon.awssdk.services.rds.model.DescribeAccountAttributesRequest;
import software.amazon.awssdk.services.rds.model.DescribeAccountAttributesResponse;
import software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest;
import software.amazon.awssdk.services.rds.model.DescribeCertificatesResponse;
import software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesRequest;
import software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotAttributesRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotAttributesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbClustersResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbInstancesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbLogFilesRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbLogFilesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbParametersRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbParametersResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbProxiesRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbProxiesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbSnapshotAttributesRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbSnapshotAttributesResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsResponse;
import software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeEngineDefaultClusterParametersRequest;
import software.amazon.awssdk.services.rds.model.DescribeEngineDefaultClusterParametersResponse;
import software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersRequest;
import software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersResponse;
import software.amazon.awssdk.services.rds.model.DescribeEventCategoriesRequest;
import software.amazon.awssdk.services.rds.model.DescribeEventCategoriesResponse;
import software.amazon.awssdk.services.rds.model.DescribeEventSubscriptionsRequest;
import software.amazon.awssdk.services.rds.model.DescribeEventSubscriptionsResponse;
import software.amazon.awssdk.services.rds.model.DescribeEventsRequest;
import software.amazon.awssdk.services.rds.model.DescribeEventsResponse;
import software.amazon.awssdk.services.rds.model.DescribeExportTasksRequest;
import software.amazon.awssdk.services.rds.model.DescribeExportTasksResponse;
import software.amazon.awssdk.services.rds.model.DescribeGlobalClustersRequest;
import software.amazon.awssdk.services.rds.model.DescribeGlobalClustersResponse;
import software.amazon.awssdk.services.rds.model.DescribeInstallationMediaRequest;
import software.amazon.awssdk.services.rds.model.DescribeInstallationMediaResponse;
import software.amazon.awssdk.services.rds.model.DescribeOptionGroupOptionsRequest;
import software.amazon.awssdk.services.rds.model.DescribeOptionGroupOptionsResponse;
import software.amazon.awssdk.services.rds.model.DescribeOptionGroupsRequest;
import software.amazon.awssdk.services.rds.model.DescribeOptionGroupsResponse;
import software.amazon.awssdk.services.rds.model.DescribeOrderableDbInstanceOptionsRequest;
import software.amazon.awssdk.services.rds.model.DescribeOrderableDbInstanceOptionsResponse;
import software.amazon.awssdk.services.rds.model.DescribePendingMaintenanceActionsRequest;
import software.amazon.awssdk.services.rds.model.DescribePendingMaintenanceActionsResponse;
import software.amazon.awssdk.services.rds.model.DescribeReservedDbInstancesOfferingsRequest;
import software.amazon.awssdk.services.rds.model.DescribeReservedDbInstancesOfferingsResponse;
import software.amazon.awssdk.services.rds.model.DescribeReservedDbInstancesRequest;
import software.amazon.awssdk.services.rds.model.DescribeReservedDbInstancesResponse;
import software.amazon.awssdk.services.rds.model.DescribeSourceRegionsRequest;
import software.amazon.awssdk.services.rds.model.DescribeSourceRegionsResponse;
import software.amazon.awssdk.services.rds.model.DescribeValidDbInstanceModificationsRequest;
import software.amazon.awssdk.services.rds.model.DescribeValidDbInstanceModificationsResponse;
import software.amazon.awssdk.services.rds.model.DownloadDbLogFilePortionRequest;
import software.amazon.awssdk.services.rds.model.DownloadDbLogFilePortionResponse;
import software.amazon.awssdk.services.rds.model.FailoverDbClusterRequest;
import software.amazon.awssdk.services.rds.model.FailoverDbClusterResponse;
import software.amazon.awssdk.services.rds.model.ImportInstallationMediaRequest;
import software.amazon.awssdk.services.rds.model.ImportInstallationMediaResponse;
import software.amazon.awssdk.services.rds.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.rds.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.rds.model.ModifyCertificatesRequest;
import software.amazon.awssdk.services.rds.model.ModifyCertificatesResponse;
import software.amazon.awssdk.services.rds.model.ModifyCurrentDbClusterCapacityRequest;
import software.amazon.awssdk.services.rds.model.ModifyCurrentDbClusterCapacityResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterEndpointRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterEndpointResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterSnapshotAttributeRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbClusterSnapshotAttributeResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbProxyRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbProxyResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbProxyTargetGroupRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbProxyTargetGroupResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbSnapshotAttributeRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbSnapshotAttributeResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbSnapshotRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbSnapshotResponse;
import software.amazon.awssdk.services.rds.model.ModifyDbSubnetGroupRequest;
import software.amazon.awssdk.services.rds.model.ModifyDbSubnetGroupResponse;
import software.amazon.awssdk.services.rds.model.ModifyEventSubscriptionRequest;
import software.amazon.awssdk.services.rds.model.ModifyEventSubscriptionResponse;
import software.amazon.awssdk.services.rds.model.ModifyGlobalClusterRequest;
import software.amazon.awssdk.services.rds.model.ModifyGlobalClusterResponse;
import software.amazon.awssdk.services.rds.model.ModifyOptionGroupRequest;
import software.amazon.awssdk.services.rds.model.ModifyOptionGroupResponse;
import software.amazon.awssdk.services.rds.model.PromoteReadReplicaDbClusterRequest;
import software.amazon.awssdk.services.rds.model.PromoteReadReplicaDbClusterResponse;
import software.amazon.awssdk.services.rds.model.PromoteReadReplicaRequest;
import software.amazon.awssdk.services.rds.model.PromoteReadReplicaResponse;
import software.amazon.awssdk.services.rds.model.PurchaseReservedDbInstancesOfferingRequest;
import software.amazon.awssdk.services.rds.model.PurchaseReservedDbInstancesOfferingResponse;
import software.amazon.awssdk.services.rds.model.RebootDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.RebootDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.RegisterDbProxyTargetsRequest;
import software.amazon.awssdk.services.rds.model.RegisterDbProxyTargetsResponse;
import software.amazon.awssdk.services.rds.model.RemoveFromGlobalClusterRequest;
import software.amazon.awssdk.services.rds.model.RemoveFromGlobalClusterResponse;
import software.amazon.awssdk.services.rds.model.RemoveRoleFromDbClusterRequest;
import software.amazon.awssdk.services.rds.model.RemoveRoleFromDbClusterResponse;
import software.amazon.awssdk.services.rds.model.RemoveRoleFromDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.RemoveRoleFromDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.RemoveSourceIdentifierFromSubscriptionRequest;
import software.amazon.awssdk.services.rds.model.RemoveSourceIdentifierFromSubscriptionResponse;
import software.amazon.awssdk.services.rds.model.RemoveTagsFromResourceRequest;
import software.amazon.awssdk.services.rds.model.RemoveTagsFromResourceResponse;
import software.amazon.awssdk.services.rds.model.ResetDbClusterParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.ResetDbClusterParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.ResetDbParameterGroupRequest;
import software.amazon.awssdk.services.rds.model.ResetDbParameterGroupResponse;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterFromS3Request;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterFromS3Response;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterFromSnapshotRequest;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterFromSnapshotResponse;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterToPointInTimeRequest;
import software.amazon.awssdk.services.rds.model.RestoreDbClusterToPointInTimeResponse;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceFromDbSnapshotRequest;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceFromDbSnapshotResponse;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceFromS3Request;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceFromS3Response;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceToPointInTimeRequest;
import software.amazon.awssdk.services.rds.model.RestoreDbInstanceToPointInTimeResponse;
import software.amazon.awssdk.services.rds.model.RevokeDbSecurityGroupIngressRequest;
import software.amazon.awssdk.services.rds.model.RevokeDbSecurityGroupIngressResponse;
import software.amazon.awssdk.services.rds.model.StartActivityStreamRequest;
import software.amazon.awssdk.services.rds.model.StartActivityStreamResponse;
import software.amazon.awssdk.services.rds.model.StartDbClusterRequest;
import software.amazon.awssdk.services.rds.model.StartDbClusterResponse;
import software.amazon.awssdk.services.rds.model.StartDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.StartDbInstanceResponse;
import software.amazon.awssdk.services.rds.model.StartExportTaskRequest;
import software.amazon.awssdk.services.rds.model.StartExportTaskResponse;
import software.amazon.awssdk.services.rds.model.StopActivityStreamRequest;
import software.amazon.awssdk.services.rds.model.StopActivityStreamResponse;
import software.amazon.awssdk.services.rds.model.StopDbClusterRequest;
import software.amazon.awssdk.services.rds.model.StopDbClusterResponse;
import software.amazon.awssdk.services.rds.model.StopDbInstanceRequest;
import software.amazon.awssdk.services.rds.model.StopDbInstanceResponse;
import software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeCustomAvailabilityZonesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClusterBacktracksPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParametersPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBLogFilesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBParametersPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBProxiesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeEngineDefaultParametersPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeEventSubscriptionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeEventsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeExportTasksPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeGlobalClustersPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeInstallationMediaPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeOptionGroupOptionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeOptionGroupsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeOrderableDBInstanceOptionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribePendingMaintenanceActionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeReservedDBInstancesOfferingsPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeReservedDBInstancesPublisher;
import software.amazon.awssdk.services.rds.paginators.DescribeSourceRegionsPublisher;
import software.amazon.awssdk.services.rds.paginators.DownloadDBLogFilePortionPublisher;
/**
* Service client for accessing Amazon RDS asynchronously. This can be created using the static {@link #builder()}
* method.
*
* Amazon Relational Database Service
*
*
*
* Amazon Relational Database Service (Amazon RDS) is a web service that makes it easier to set up, operate, and scale a
* relational database in the cloud. It provides cost-efficient, resizeable capacity for an industry-standard relational
* database and manages common database administration tasks, freeing up developers to focus on what makes their
* applications and businesses unique.
*
*
* Amazon RDS gives you access to the capabilities of a MySQL, MariaDB, PostgreSQL, Microsoft SQL Server, Oracle, or
* Amazon Aurora database server. These capabilities mean that the code, applications, and tools you already use today
* with your existing databases work with Amazon RDS without modification. Amazon RDS automatically backs up your
* database and maintains the database software that powers your DB instance. Amazon RDS is flexible: you can scale your
* DB instance's compute resources and storage capacity to meet your application's demand. As with all Amazon Web
* Services, there are no up-front investments, and you pay only for the resources you use.
*
*
* This interface reference for Amazon RDS contains documentation for a programming or command line interface you can
* use to manage Amazon RDS. Amazon RDS is asynchronous, which means that some interfaces might require techniques such
* as polling or callback functions to determine when a command has been applied. In this reference, the parameter
* descriptions indicate whether a command is applied immediately, on the next instance reboot, or during the
* maintenance window. The reference structure is as follows, and we list following some related topics from the user
* guide.
*
*
* Amazon RDS API Reference
*
*
* -
*
* For the alphabetical list of API actions, see API Actions.
*
*
* -
*
* For the alphabetical list of data types, see Data Types.
*
*
* -
*
* For a list of common query parameters, see Common Parameters.
*
*
* -
*
* For descriptions of the error codes, see Common Errors.
*
*
*
*
* Amazon RDS User Guide
*
*
* -
*
* For a summary of the Amazon RDS interfaces, see Available RDS
* Interfaces.
*
*
* -
*
* For more information about how to use the Query API, see Using the Query API.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface RdsAsyncClient extends SdkClient {
String SERVICE_NAME = "rds";
/**
* Create a {@link RdsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static RdsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link RdsAsyncClient}.
*/
static RdsAsyncClientBuilder builder() {
return new DefaultRdsAsyncClientBuilder();
}
/**
*
* Associates an Identity and Access Management (IAM) role from an Amazon Aurora DB cluster. For more information,
* see Authorizing Amazon Aurora MySQL to Access Other AWS Services on Your Behalf in the Amazon Aurora User
* Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param addRoleToDbClusterRequest
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterRoleAlreadyExistsException The specified IAM role Amazon Resource Name (ARN) is already
* associated with the specified DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterRoleQuotaExceededException You have exceeded the maximum number of IAM roles that can be
* associated with the specified DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture addRoleToDBCluster(AddRoleToDbClusterRequest addRoleToDbClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates an Identity and Access Management (IAM) role from an Amazon Aurora DB cluster. For more information,
* see Authorizing Amazon Aurora MySQL to Access Other AWS Services on Your Behalf in the Amazon Aurora User
* Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link AddRoleToDbClusterRequest.Builder} avoiding the
* need to create one manually via {@link AddRoleToDbClusterRequest#builder()}
*
*
* @param addRoleToDbClusterRequest
* A {@link Consumer} that will call methods on {@link AddRoleToDBClusterMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterRoleAlreadyExistsException The specified IAM role Amazon Resource Name (ARN) is already
* associated with the specified DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterRoleQuotaExceededException You have exceeded the maximum number of IAM roles that can be
* associated with the specified DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture addRoleToDBCluster(
Consumer addRoleToDbClusterRequest) {
return addRoleToDBCluster(AddRoleToDbClusterRequest.builder().applyMutation(addRoleToDbClusterRequest).build());
}
/**
*
* Associates an AWS Identity and Access Management (IAM) role with a DB instance.
*
*
*
* To add a role to a DB instance, the status of the DB instance must be available
.
*
*
*
* @param addRoleToDbInstanceRequest
* @return A Java Future containing the result of the AddRoleToDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbInstanceRoleAlreadyExistsException The specified
RoleArn
or FeatureName
* value is already associated with the DB instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbInstanceRoleQuotaExceededException You can't associate any more AWS Identity and Access Management
* (IAM) roles with the DB instance because the quota has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddRoleToDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture addRoleToDBInstance(
AddRoleToDbInstanceRequest addRoleToDbInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates an AWS Identity and Access Management (IAM) role with a DB instance.
*
*
*
* To add a role to a DB instance, the status of the DB instance must be available
.
*
*
*
* This is a convenience which creates an instance of the {@link AddRoleToDbInstanceRequest.Builder} avoiding the
* need to create one manually via {@link AddRoleToDbInstanceRequest#builder()}
*
*
* @param addRoleToDbInstanceRequest
* A {@link Consumer} that will call methods on {@link AddRoleToDBInstanceMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the AddRoleToDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbInstanceRoleAlreadyExistsException The specified
RoleArn
or FeatureName
* value is already associated with the DB instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbInstanceRoleQuotaExceededException You can't associate any more AWS Identity and Access Management
* (IAM) roles with the DB instance because the quota has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddRoleToDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture addRoleToDBInstance(
Consumer addRoleToDbInstanceRequest) {
return addRoleToDBInstance(AddRoleToDbInstanceRequest.builder().applyMutation(addRoleToDbInstanceRequest).build());
}
/**
*
* Adds a source identifier to an existing RDS event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SubscriptionNotFoundException The subscription name does not exist.
* - SourceNotFoundException The requested source could not be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
default CompletableFuture addSourceIdentifierToSubscription(
AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a source identifier to an existing RDS event notification subscription.
*
*
*
* This is a convenience which creates an instance of the {@link AddSourceIdentifierToSubscriptionRequest.Builder}
* avoiding the need to create one manually via {@link AddSourceIdentifierToSubscriptionRequest#builder()}
*
*
* @param addSourceIdentifierToSubscriptionRequest
* A {@link Consumer} that will call methods on {@link AddSourceIdentifierToSubscriptionMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SubscriptionNotFoundException The subscription name does not exist.
* - SourceNotFoundException The requested source could not be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
default CompletableFuture addSourceIdentifierToSubscription(
Consumer addSourceIdentifierToSubscriptionRequest) {
return addSourceIdentifierToSubscription(AddSourceIdentifierToSubscriptionRequest.builder()
.applyMutation(addSourceIdentifierToSubscriptionRequest).build());
}
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be used with cost allocation reporting to track
* cost associated with Amazon RDS resources, or used in a Condition statement in an IAM policy for Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see Tagging Amazon RDS
* Resources.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddTagsToResource
* @see AWS API
* Documentation
*/
default CompletableFuture addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be used with cost allocation reporting to track
* cost associated with Amazon RDS resources, or used in a Condition statement in an IAM policy for Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see Tagging Amazon RDS
* Resources.
*
*
*
* This is a convenience which creates an instance of the {@link AddTagsToResourceRequest.Builder} avoiding the need
* to create one manually via {@link AddTagsToResourceRequest#builder()}
*
*
* @param addTagsToResourceRequest
* A {@link Consumer} that will call methods on {@link AddTagsToResourceMessage.Builder} to create a request.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AddTagsToResource
* @see AWS API
* Documentation
*/
default CompletableFuture addTagsToResource(
Consumer addTagsToResourceRequest) {
return addTagsToResource(AddTagsToResourceRequest.builder().applyMutation(addTagsToResourceRequest).build());
}
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource ID was not found.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
default CompletableFuture applyPendingMaintenanceAction(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
*
* This is a convenience which creates an instance of the {@link ApplyPendingMaintenanceActionRequest.Builder}
* avoiding the need to create one manually via {@link ApplyPendingMaintenanceActionRequest#builder()}
*
*
* @param applyPendingMaintenanceActionRequest
* A {@link Consumer} that will call methods on {@link ApplyPendingMaintenanceActionMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource ID was not found.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
default CompletableFuture applyPendingMaintenanceAction(
Consumer applyPendingMaintenanceActionRequest) {
return applyPendingMaintenanceAction(ApplyPendingMaintenanceActionRequest.builder()
.applyMutation(applyPendingMaintenanceActionRequest).build());
}
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of authorization. First, EC2 or VPC security groups
* can be added to the DBSecurityGroup if the application using the database is running on EC2 or VPC instances.
* Second, IP ranges are available if the application accessing your database is running on the Internet. Required
* parameters for this API are one of CIDR range, EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
*
* You can't authorize ingress from an EC2 security group in one AWS Region to an Amazon RDS DB instance in another.
* You can't authorize ingress from a VPC security group in one VPC to an Amazon RDS DB instance in another.
*
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param authorizeDbSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeDBSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InvalidDbSecurityGroupStateException The state of the DB security group doesn't allow deletion.
* - AuthorizationAlreadyExistsException The specified CIDR IP range or Amazon EC2 security group is
* already authorized for the specified DB security group.
* - AuthorizationQuotaExceededException The DB security group authorization quota has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AuthorizeDBSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture authorizeDBSecurityGroupIngress(
AuthorizeDbSecurityGroupIngressRequest authorizeDbSecurityGroupIngressRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of authorization. First, EC2 or VPC security groups
* can be added to the DBSecurityGroup if the application using the database is running on EC2 or VPC instances.
* Second, IP ranges are available if the application accessing your database is running on the Internet. Required
* parameters for this API are one of CIDR range, EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
*
* You can't authorize ingress from an EC2 security group in one AWS Region to an Amazon RDS DB instance in another.
* You can't authorize ingress from a VPC security group in one VPC to an Amazon RDS DB instance in another.
*
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
*
* This is a convenience which creates an instance of the {@link AuthorizeDbSecurityGroupIngressRequest.Builder}
* avoiding the need to create one manually via {@link AuthorizeDbSecurityGroupIngressRequest#builder()}
*
*
* @param authorizeDbSecurityGroupIngressRequest
* A {@link Consumer} that will call methods on {@link AuthorizeDBSecurityGroupIngressMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the AuthorizeDBSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InvalidDbSecurityGroupStateException The state of the DB security group doesn't allow deletion.
* - AuthorizationAlreadyExistsException The specified CIDR IP range or Amazon EC2 security group is
* already authorized for the specified DB security group.
* - AuthorizationQuotaExceededException The DB security group authorization quota has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.AuthorizeDBSecurityGroupIngress
* @see AWS API Documentation
*/
default CompletableFuture authorizeDBSecurityGroupIngress(
Consumer authorizeDbSecurityGroupIngressRequest) {
return authorizeDBSecurityGroupIngress(AuthorizeDbSecurityGroupIngressRequest.builder()
.applyMutation(authorizeDbSecurityGroupIngressRequest).build());
}
/**
*
* Backtracks a DB cluster to a specific time, without creating a new DB cluster.
*
*
* For more information on backtracking, see
* Backtracking an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param backtrackDbClusterRequest
* @return A Java Future containing the result of the BacktrackDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.BacktrackDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture backtrackDBCluster(BacktrackDbClusterRequest backtrackDbClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Backtracks a DB cluster to a specific time, without creating a new DB cluster.
*
*
* For more information on backtracking, see
* Backtracking an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link BacktrackDbClusterRequest.Builder} avoiding the
* need to create one manually via {@link BacktrackDbClusterRequest#builder()}
*
*
* @param backtrackDbClusterRequest
* A {@link Consumer} that will call methods on {@link BacktrackDBClusterMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the BacktrackDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.BacktrackDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture backtrackDBCluster(
Consumer backtrackDbClusterRequest) {
return backtrackDBCluster(BacktrackDbClusterRequest.builder().applyMutation(backtrackDbClusterRequest).build());
}
/**
*
* Cancels an export task in progress that is exporting a snapshot to Amazon S3. Any data that has already been
* written to the S3 bucket isn't removed.
*
*
* @param cancelExportTaskRequest
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ExportTaskNotFoundException The export task doesn't exist.
* - InvalidExportTaskStateException You can't cancel an export task that has completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CancelExportTask
* @see AWS API
* Documentation
*/
default CompletableFuture cancelExportTask(CancelExportTaskRequest cancelExportTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels an export task in progress that is exporting a snapshot to Amazon S3. Any data that has already been
* written to the S3 bucket isn't removed.
*
*
*
* This is a convenience which creates an instance of the {@link CancelExportTaskRequest.Builder} avoiding the need
* to create one manually via {@link CancelExportTaskRequest#builder()}
*
*
* @param cancelExportTaskRequest
* A {@link Consumer} that will call methods on {@link CancelExportTaskMessage.Builder} to create a request.
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ExportTaskNotFoundException The export task doesn't exist.
* - InvalidExportTaskStateException You can't cancel an export task that has completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CancelExportTask
* @see AWS API
* Documentation
*/
default CompletableFuture cancelExportTask(
Consumer cancelExportTaskRequest) {
return cancelExportTask(CancelExportTaskRequest.builder().applyMutation(cancelExportTaskRequest).build());
}
/**
*
* Copies the specified DB cluster parameter group.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDbClusterParameterGroupRequest
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture copyDBClusterParameterGroup(
CopyDbClusterParameterGroupRequest copyDbClusterParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies the specified DB cluster parameter group.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CopyDbClusterParameterGroupRequest.Builder}
* avoiding the need to create one manually via {@link CopyDbClusterParameterGroupRequest#builder()}
*
*
* @param copyDbClusterParameterGroupRequest
* A {@link Consumer} that will call methods on {@link CopyDBClusterParameterGroupMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture copyDBClusterParameterGroup(
Consumer copyDbClusterParameterGroupRequest) {
return copyDBClusterParameterGroup(CopyDbClusterParameterGroupRequest.builder()
.applyMutation(copyDbClusterParameterGroupRequest).build());
}
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* You can copy an encrypted DB cluster snapshot from another AWS Region. In that case, the AWS Region where you
* call the CopyDBClusterSnapshot
action is the destination AWS Region for the encrypted DB cluster
* snapshot to be copied to. To copy an encrypted DB cluster snapshot from another AWS Region, you must provide the
* following values:
*
*
* -
*
* KmsKeyId
- The AWS Key Management System (AWS KMS) key identifier for the key to use to encrypt the
* copy of the DB cluster snapshot in the destination AWS Region.
*
*
* -
*
* PreSignedUrl
- A URL that contains a Signature Version 4 signed request for the
* CopyDBClusterSnapshot
action to be called in the source AWS Region where the DB cluster snapshot is
* copied from. The pre-signed URL must be a valid request for the CopyDBClusterSnapshot
API action
* that can be executed in the source AWS Region that contains the encrypted DB cluster snapshot to be copied.
*
*
* The pre-signed URL request must contain the following parameter values:
*
*
* -
*
* KmsKeyId
- The KMS key identifier for the key to use to encrypt the copy of the DB cluster snapshot
* in the destination AWS Region. This is the same identifier for both the CopyDBClusterSnapshot
action
* that is called in the destination AWS Region, and the action contained in the pre-signed URL.
*
*
* -
*
* DestinationRegion
- The name of the AWS Region that the DB cluster snapshot is to be created in.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the Amazon Resource Name (ARN) format for the source AWS
* Region. For example, if you are copying an encrypted DB cluster snapshot from the us-west-2 AWS Region, then your
* SourceDBClusterSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:cluster-snapshot:aurora-cluster1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (AWS Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an AWS SDK tool or the AWS CLI, you can specify SourceRegion
(or
* --source-region
for the AWS CLI) instead of specifying PreSignedUrl
manually.
* Specifying SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation
* that can be executed in the source AWS Region.
*
*
* -
*
* TargetDBClusterSnapshotIdentifier
- The identifier for the new copy of the DB cluster snapshot in
* the destination AWS Region.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the ARN format for the source AWS Region and is the same value
* as the SourceDBClusterSnapshotIdentifier
in the pre-signed URL.
*
*
*
*
* To cancel the copy operation once it is in progress, delete the target DB cluster snapshot identified by
* TargetDBClusterSnapshotIdentifier
while that DB cluster snapshot is in "copying" status.
*
*
* For more information on copying encrypted DB cluster snapshots from one AWS Region to another, see Copying a
* Snapshot in the Amazon Aurora User Guide.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDbClusterSnapshotRequest
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBClusterSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBClusterSnapshot(
CopyDbClusterSnapshotRequest copyDbClusterSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* You can copy an encrypted DB cluster snapshot from another AWS Region. In that case, the AWS Region where you
* call the CopyDBClusterSnapshot
action is the destination AWS Region for the encrypted DB cluster
* snapshot to be copied to. To copy an encrypted DB cluster snapshot from another AWS Region, you must provide the
* following values:
*
*
* -
*
* KmsKeyId
- The AWS Key Management System (AWS KMS) key identifier for the key to use to encrypt the
* copy of the DB cluster snapshot in the destination AWS Region.
*
*
* -
*
* PreSignedUrl
- A URL that contains a Signature Version 4 signed request for the
* CopyDBClusterSnapshot
action to be called in the source AWS Region where the DB cluster snapshot is
* copied from. The pre-signed URL must be a valid request for the CopyDBClusterSnapshot
API action
* that can be executed in the source AWS Region that contains the encrypted DB cluster snapshot to be copied.
*
*
* The pre-signed URL request must contain the following parameter values:
*
*
* -
*
* KmsKeyId
- The KMS key identifier for the key to use to encrypt the copy of the DB cluster snapshot
* in the destination AWS Region. This is the same identifier for both the CopyDBClusterSnapshot
action
* that is called in the destination AWS Region, and the action contained in the pre-signed URL.
*
*
* -
*
* DestinationRegion
- The name of the AWS Region that the DB cluster snapshot is to be created in.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the Amazon Resource Name (ARN) format for the source AWS
* Region. For example, if you are copying an encrypted DB cluster snapshot from the us-west-2 AWS Region, then your
* SourceDBClusterSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:cluster-snapshot:aurora-cluster1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (AWS Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an AWS SDK tool or the AWS CLI, you can specify SourceRegion
(or
* --source-region
for the AWS CLI) instead of specifying PreSignedUrl
manually.
* Specifying SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation
* that can be executed in the source AWS Region.
*
*
* -
*
* TargetDBClusterSnapshotIdentifier
- The identifier for the new copy of the DB cluster snapshot in
* the destination AWS Region.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the ARN format for the source AWS Region and is the same value
* as the SourceDBClusterSnapshotIdentifier
in the pre-signed URL.
*
*
*
*
* To cancel the copy operation once it is in progress, delete the target DB cluster snapshot identified by
* TargetDBClusterSnapshotIdentifier
while that DB cluster snapshot is in "copying" status.
*
*
* For more information on copying encrypted DB cluster snapshots from one AWS Region to another, see Copying a
* Snapshot in the Amazon Aurora User Guide.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CopyDbClusterSnapshotRequest.Builder} avoiding the
* need to create one manually via {@link CopyDbClusterSnapshotRequest#builder()}
*
*
* @param copyDbClusterSnapshotRequest
* A {@link Consumer} that will call methods on {@link CopyDBClusterSnapshotMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBClusterSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBClusterSnapshot(
Consumer copyDbClusterSnapshotRequest) {
return copyDBClusterSnapshot(CopyDbClusterSnapshotRequest.builder().applyMutation(copyDbClusterSnapshotRequest).build());
}
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDbParameterGroupRequest
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBParameterGroup(
CopyDbParameterGroupRequest copyDbParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies the specified DB parameter group.
*
*
*
* This is a convenience which creates an instance of the {@link CopyDbParameterGroupRequest.Builder} avoiding the
* need to create one manually via {@link CopyDbParameterGroupRequest#builder()}
*
*
* @param copyDbParameterGroupRequest
* A {@link Consumer} that will call methods on {@link CopyDBParameterGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBParameterGroup(
Consumer copyDbParameterGroupRequest) {
return copyDBParameterGroup(CopyDbParameterGroupRequest.builder().applyMutation(copyDbParameterGroupRequest).build());
}
/**
*
* Copies the specified DB snapshot. The source DB snapshot must be in the "available" state.
*
*
* You can copy a snapshot from one AWS Region to another. In that case, the AWS Region where you call the
* CopyDBSnapshot
action is the destination AWS Region for the DB snapshot copy.
*
*
* For more information about copying snapshots, see Copying
* a DB Snapshot in the Amazon RDS User Guide.
*
*
* @param copyDbSnapshotRequest
* @return A Java Future containing the result of the CopyDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - InvalidDbSnapshotStateException The state of the DB snapshot doesn't allow deletion.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBSnapshot(CopyDbSnapshotRequest copyDbSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies the specified DB snapshot. The source DB snapshot must be in the "available" state.
*
*
* You can copy a snapshot from one AWS Region to another. In that case, the AWS Region where you call the
* CopyDBSnapshot
action is the destination AWS Region for the DB snapshot copy.
*
*
* For more information about copying snapshots, see Copying
* a DB Snapshot in the Amazon RDS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CopyDbSnapshotRequest.Builder} avoiding the need to
* create one manually via {@link CopyDbSnapshotRequest#builder()}
*
*
* @param copyDbSnapshotRequest
* A {@link Consumer} that will call methods on {@link CopyDBSnapshotMessage.Builder} to create a request.
* @return A Java Future containing the result of the CopyDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - InvalidDbSnapshotStateException The state of the DB snapshot doesn't allow deletion.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture copyDBSnapshot(Consumer copyDbSnapshotRequest) {
return copyDBSnapshot(CopyDbSnapshotRequest.builder().applyMutation(copyDbSnapshotRequest).build());
}
/**
*
* Copies the specified option group.
*
*
* @param copyOptionGroupRequest
* @return A Java Future containing the result of the CopyOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupAlreadyExistsException The option group you are trying to create already exists.
* - OptionGroupNotFoundException The specified option group could not be found.
* - OptionGroupQuotaExceededException The quota of 20 option groups was exceeded for this AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture copyOptionGroup(CopyOptionGroupRequest copyOptionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Copies the specified option group.
*
*
*
* This is a convenience which creates an instance of the {@link CopyOptionGroupRequest.Builder} avoiding the need
* to create one manually via {@link CopyOptionGroupRequest#builder()}
*
*
* @param copyOptionGroupRequest
* A {@link Consumer} that will call methods on {@link CopyOptionGroupMessage.Builder} to create a request.
* @return A Java Future containing the result of the CopyOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupAlreadyExistsException The option group you are trying to create already exists.
* - OptionGroupNotFoundException The specified option group could not be found.
* - OptionGroupQuotaExceededException The quota of 20 option groups was exceeded for this AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CopyOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture copyOptionGroup(
Consumer copyOptionGroupRequest) {
return copyOptionGroup(CopyOptionGroupRequest.builder().applyMutation(copyOptionGroupRequest).build());
}
/**
*
* Creates a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
* @param createCustomAvailabilityZoneRequest
* @return A Java Future containing the result of the CreateCustomAvailabilityZone operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneAlreadyExistsException
CustomAvailabilityZoneName
is already used
* by an existing custom Availability Zone.
* - CustomAvailabilityZoneQuotaExceededException You have exceeded the maximum number of custom
* Availability Zones.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateCustomAvailabilityZone
* @see AWS API Documentation
*/
default CompletableFuture createCustomAvailabilityZone(
CreateCustomAvailabilityZoneRequest createCustomAvailabilityZoneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCustomAvailabilityZoneRequest.Builder}
* avoiding the need to create one manually via {@link CreateCustomAvailabilityZoneRequest#builder()}
*
*
* @param createCustomAvailabilityZoneRequest
* A {@link Consumer} that will call methods on {@link CreateCustomAvailabilityZoneMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateCustomAvailabilityZone operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneAlreadyExistsException
CustomAvailabilityZoneName
is already used
* by an existing custom Availability Zone.
* - CustomAvailabilityZoneQuotaExceededException You have exceeded the maximum number of custom
* Availability Zones.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateCustomAvailabilityZone
* @see AWS API Documentation
*/
default CompletableFuture createCustomAvailabilityZone(
Consumer createCustomAvailabilityZoneRequest) {
return createCustomAvailabilityZone(CreateCustomAvailabilityZoneRequest.builder()
.applyMutation(createCustomAvailabilityZoneRequest).build());
}
/**
*
* Creates a new Amazon Aurora DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a read replica of
* another DB cluster or Amazon RDS MySQL DB instance. For cross-region replication where the DB cluster identified
* by ReplicationSourceIdentifier
is encrypted, you must also specify the PreSignedUrl
* parameter.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDbClusterRequest
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterAlreadyExistsException The user already has a DB cluster with the given identifier.
* - InsufficientStorageClusterCapacityException There is insufficient storage available for the current
* action. You might be able to resolve this error by updating your subnet group to use different
* Availability Zones that have more storage available.
* - DbClusterQuotaExceededException The user attempted to create a new DB cluster and the user has
* already reached the maximum allowed DB cluster quota.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbSubnetGroupStateException The DB subnet group cannot be deleted because it's in use.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbClusterParameterGroupNotFoundException
DBClusterParameterGroupName
doesn't refer to an
* existing DB cluster parameter group.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - GlobalClusterNotFoundException
* - InvalidGlobalClusterStateException
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createDBCluster(CreateDbClusterRequest createDbClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon Aurora DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a read replica of
* another DB cluster or Amazon RDS MySQL DB instance. For cross-region replication where the DB cluster identified
* by ReplicationSourceIdentifier
is encrypted, you must also specify the PreSignedUrl
* parameter.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbClusterRequest.Builder} avoiding the need
* to create one manually via {@link CreateDbClusterRequest#builder()}
*
*
* @param createDbClusterRequest
* A {@link Consumer} that will call methods on {@link CreateDBClusterMessage.Builder} to create a request.
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterAlreadyExistsException The user already has a DB cluster with the given identifier.
* - InsufficientStorageClusterCapacityException There is insufficient storage available for the current
* action. You might be able to resolve this error by updating your subnet group to use different
* Availability Zones that have more storage available.
* - DbClusterQuotaExceededException The user attempted to create a new DB cluster and the user has
* already reached the maximum allowed DB cluster quota.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidDbSubnetGroupStateException The DB subnet group cannot be deleted because it's in use.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbClusterParameterGroupNotFoundException
DBClusterParameterGroupName
doesn't refer to an
* existing DB cluster parameter group.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - GlobalClusterNotFoundException
* - InvalidGlobalClusterStateException
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createDBCluster(
Consumer createDbClusterRequest) {
return createDBCluster(CreateDbClusterRequest.builder().applyMutation(createDbClusterRequest).build());
}
/**
*
* Creates a new custom endpoint and associates it with an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDbClusterEndpointRequest
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterEndpointQuotaExceededException The cluster already has the maximum number of custom
* endpoints.
* - DbClusterEndpointAlreadyExistsException The specified custom endpoint can't be created because it
* already exists.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture createDBClusterEndpoint(
CreateDbClusterEndpointRequest createDbClusterEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new custom endpoint and associates it with an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbClusterEndpointRequest.Builder} avoiding
* the need to create one manually via {@link CreateDbClusterEndpointRequest#builder()}
*
*
* @param createDbClusterEndpointRequest
* A {@link Consumer} that will call methods on {@link CreateDBClusterEndpointMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterEndpointQuotaExceededException The cluster already has the maximum number of custom
* endpoints.
* - DbClusterEndpointAlreadyExistsException The specified custom endpoint can't be created because it
* already exists.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture createDBClusterEndpoint(
Consumer createDbClusterEndpointRequest) {
return createDBClusterEndpoint(CreateDbClusterEndpointRequest.builder().applyMutation(createDbClusterEndpointRequest)
.build());
}
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup
. Once you've created a DB cluster parameter group,
* you need to associate it with your DB cluster using ModifyDBCluster
. When you associate a new DB
* cluster parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without
* failover for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon RDS to fully
* complete the create action before the DB cluster parameter group is used as the default for a new DB cluster.
* This is especially important for parameters that are critical when creating the default database for a DB
* cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon RDS console or the DescribeDBClusterParameters
* action to verify that your DB cluster parameter group has been created or modified.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDbClusterParameterGroupRequest
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture createDBClusterParameterGroup(
CreateDbClusterParameterGroupRequest createDbClusterParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup
. Once you've created a DB cluster parameter group,
* you need to associate it with your DB cluster using ModifyDBCluster
. When you associate a new DB
* cluster parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without
* failover for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon RDS to fully
* complete the create action before the DB cluster parameter group is used as the default for a new DB cluster.
* This is especially important for parameters that are critical when creating the default database for a DB
* cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon RDS console or the DescribeDBClusterParameters
* action to verify that your DB cluster parameter group has been created or modified.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbClusterParameterGroupRequest.Builder}
* avoiding the need to create one manually via {@link CreateDbClusterParameterGroupRequest#builder()}
*
*
* @param createDbClusterParameterGroupRequest
* A {@link Consumer} that will call methods on {@link CreateDBClusterParameterGroupMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture createDBClusterParameterGroup(
Consumer createDbClusterParameterGroupRequest) {
return createDBClusterParameterGroup(CreateDbClusterParameterGroupRequest.builder()
.applyMutation(createDbClusterParameterGroupRequest).build());
}
/**
*
* Creates a snapshot of a DB cluster. For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDbClusterSnapshotRequest
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture createDBClusterSnapshot(
CreateDbClusterSnapshotRequest createDbClusterSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a snapshot of a DB cluster. For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbClusterSnapshotRequest.Builder} avoiding
* the need to create one manually via {@link CreateDbClusterSnapshotRequest#builder()}
*
*
* @param createDbClusterSnapshotRequest
* A {@link Consumer} that will call methods on {@link CreateDBClusterSnapshotMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBClusterSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture createDBClusterSnapshot(
Consumer createDbClusterSnapshotRequest) {
return createDBClusterSnapshot(CreateDbClusterSnapshotRequest.builder().applyMutation(createDbClusterSnapshotRequest)
.build());
}
/**
*
* Creates a new DB instance.
*
*
* @param createDbInstanceRequest
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAlreadyExistsException The user already has a DB instance with the given identifier.
* - InsufficientDbInstanceCapacityException The specified DB instance class isn't available in the
* specified Availability Zone.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InstanceQuotaExceededException The request would result in the user exceeding the allowed number of
* DB instances.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - ProvisionedIopsNotAvailableInAzException Provisioned IOPS not available in the specified Availability
* Zone.
* - OptionGroupNotFoundException The specified option group could not be found.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - StorageTypeNotSupportedException Storage of the
StorageType
specified can't be
* associated with the DB instance.
* - AuthorizationNotFoundException The specified CIDR IP range or Amazon EC2 security group might not be
* authorized for the specified DB security group.
*
* Or, RDS might not be authorized to perform necessary actions using IAM on your behalf.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture createDBInstance(CreateDbInstanceRequest createDbInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbInstanceRequest.Builder} avoiding the need
* to create one manually via {@link CreateDbInstanceRequest#builder()}
*
*
* @param createDbInstanceRequest
* A {@link Consumer} that will call methods on {@link CreateDBInstanceMessage.Builder} to create a request.
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAlreadyExistsException The user already has a DB instance with the given identifier.
* - InsufficientDbInstanceCapacityException The specified DB instance class isn't available in the
* specified Availability Zone.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InstanceQuotaExceededException The request would result in the user exceeding the allowed number of
* DB instances.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - ProvisionedIopsNotAvailableInAzException Provisioned IOPS not available in the specified Availability
* Zone.
* - OptionGroupNotFoundException The specified option group could not be found.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - StorageTypeNotSupportedException Storage of the
StorageType
specified can't be
* associated with the DB instance.
* - AuthorizationNotFoundException The specified CIDR IP range or Amazon EC2 security group might not be
* authorized for the specified DB security group.
*
* Or, RDS might not be authorized to perform necessary actions using IAM on your behalf.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture createDBInstance(
Consumer createDbInstanceRequest) {
return createDBInstance(CreateDbInstanceRequest.builder().applyMutation(createDbInstanceRequest).build());
}
/**
*
* Creates a new DB instance that acts as a read replica for an existing source DB instance. You can create a read
* replica for a DB instance running MySQL, MariaDB, Oracle, PostgreSQL, or SQL Server. For more information, see Working with Read Replicas
* in the Amazon RDS User Guide.
*
*
* Amazon Aurora doesn't support this action. Call the CreateDBInstance
action to create a DB instance
* for an Aurora DB cluster.
*
*
* All read replica DB instances are created with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source DB instance, except as specified.
*
*
*
* Your source DB instance must have backup retention enabled.
*
*
*
* @param createDbInstanceReadReplicaRequest
* @return A Java Future containing the result of the CreateDBInstanceReadReplica operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAlreadyExistsException The user already has a DB instance with the given identifier.
* - InsufficientDbInstanceCapacityException The specified DB instance class isn't available in the
* specified Availability Zone.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InstanceQuotaExceededException The request would result in the user exceeding the allowed number of
* DB instances.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - ProvisionedIopsNotAvailableInAzException Provisioned IOPS not available in the specified Availability
* Zone.
* - OptionGroupNotFoundException The specified option group could not be found.
* - DbSubnetGroupNotAllowedException The DBSubnetGroup shouldn't be specified while creating read
* replicas that lie in the same region as the source instance.
* - InvalidDbSubnetGroupException The DBSubnetGroup doesn't belong to the same VPC as that of an existing
* cross-region read replica of the same source instance.
* - StorageTypeNotSupportedException Storage of the
StorageType
specified can't be
* associated with the DB instance.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBInstanceReadReplica
* @see AWS API Documentation
*/
default CompletableFuture createDBInstanceReadReplica(
CreateDbInstanceReadReplicaRequest createDbInstanceReadReplicaRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB instance that acts as a read replica for an existing source DB instance. You can create a read
* replica for a DB instance running MySQL, MariaDB, Oracle, PostgreSQL, or SQL Server. For more information, see Working with Read Replicas
* in the Amazon RDS User Guide.
*
*
* Amazon Aurora doesn't support this action. Call the CreateDBInstance
action to create a DB instance
* for an Aurora DB cluster.
*
*
* All read replica DB instances are created with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source DB instance, except as specified.
*
*
*
* Your source DB instance must have backup retention enabled.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbInstanceReadReplicaRequest.Builder}
* avoiding the need to create one manually via {@link CreateDbInstanceReadReplicaRequest#builder()}
*
*
* @param createDbInstanceReadReplicaRequest
* A {@link Consumer} that will call methods on {@link CreateDBInstanceReadReplicaMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateDBInstanceReadReplica operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAlreadyExistsException The user already has a DB instance with the given identifier.
* - InsufficientDbInstanceCapacityException The specified DB instance class isn't available in the
* specified Availability Zone.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - InstanceQuotaExceededException The request would result in the user exceeding the allowed number of
* DB instances.
* - StorageQuotaExceededException The request would result in the user exceeding the allowed amount of
* storage available across all DB instances.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - InvalidVpcNetworkStateException The DB subnet group doesn't cover all Availability Zones after it's
* created because of users' change.
* - ProvisionedIopsNotAvailableInAzException Provisioned IOPS not available in the specified Availability
* Zone.
* - OptionGroupNotFoundException The specified option group could not be found.
* - DbSubnetGroupNotAllowedException The DBSubnetGroup shouldn't be specified while creating read
* replicas that lie in the same region as the source instance.
* - InvalidDbSubnetGroupException The DBSubnetGroup doesn't belong to the same VPC as that of an existing
* cross-region read replica of the same source instance.
* - StorageTypeNotSupportedException Storage of the
StorageType
specified can't be
* associated with the DB instance.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - DomainNotFoundException
Domain
doesn't refer to an existing Active Directory domain.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBInstanceReadReplica
* @see AWS API Documentation
*/
default CompletableFuture createDBInstanceReadReplica(
Consumer createDbInstanceReadReplicaRequest) {
return createDBInstanceReadReplica(CreateDbInstanceReadReplicaRequest.builder()
.applyMutation(createDbInstanceReadReplicaRequest).build());
}
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup. Once you've created a DB parameter group, you need to associate it with your DB
* instance using ModifyDBInstance. When you associate a new DB parameter group with a running DB instance,
* you need to reboot the DB instance without failover for the new DB parameter group and associated settings to
* take effect.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon RDS to fully complete the
* create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter group has been created or modified.
*
*
*
* @param createDbParameterGroupRequest
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBParameterGroup(
CreateDbParameterGroupRequest createDbParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup. Once you've created a DB parameter group, you need to associate it with your DB
* instance using ModifyDBInstance. When you associate a new DB parameter group with a running DB instance,
* you need to reboot the DB instance without failover for the new DB parameter group and associated settings to
* take effect.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon RDS to fully complete the
* create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter group has been created or modified.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbParameterGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateDbParameterGroupRequest#builder()}
*
*
* @param createDbParameterGroupRequest
* A {@link Consumer} that will call methods on {@link CreateDBParameterGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB parameter groups.
* - DbParameterGroupAlreadyExistsException A DB parameter group with the same name exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBParameterGroup(
Consumer createDbParameterGroupRequest) {
return createDBParameterGroup(CreateDbParameterGroupRequest.builder().applyMutation(createDbParameterGroupRequest)
.build());
}
/**
*
* Creates a new DB proxy.
*
*
* @param createDbProxyRequest
* @return A Java Future containing the result of the CreateDBProxy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - DbProxyAlreadyExistsException The specified proxy name must be unique for all proxies owned by your
* AWS account in the specified AWS Region.
* - DbProxyQuotaExceededException Your AWS account already has the maximum number of proxies in the
* specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBProxy
* @see AWS API
* Documentation
*/
default CompletableFuture createDBProxy(CreateDbProxyRequest createDbProxyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB proxy.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbProxyRequest.Builder} avoiding the need to
* create one manually via {@link CreateDbProxyRequest#builder()}
*
*
* @param createDbProxyRequest
* A {@link Consumer} that will call methods on {@link CreateDBProxyRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateDBProxy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - DbProxyAlreadyExistsException The specified proxy name must be unique for all proxies owned by your
* AWS account in the specified AWS Region.
* - DbProxyQuotaExceededException Your AWS account already has the maximum number of proxies in the
* specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBProxy
* @see AWS API
* Documentation
*/
default CompletableFuture createDBProxy(Consumer createDbProxyRequest) {
return createDBProxy(CreateDbProxyRequest.builder().applyMutation(createDbProxyRequest).build());
}
/**
*
* Creates a new DB security group. DB security groups control access to a DB instance.
*
*
*
* A DB security group controls access to EC2-Classic DB instances that are not in a VPC.
*
*
*
* @param createDbSecurityGroupRequest
* @return A Java Future containing the result of the CreateDBSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupAlreadyExistsException A DB security group with the name specified in
*
DBSecurityGroupName
already exists.
* - DbSecurityGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB security groups.
* - DbSecurityGroupNotSupportedException A DB security group isn't allowed for this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSecurityGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSecurityGroup(
CreateDbSecurityGroupRequest createDbSecurityGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB security group. DB security groups control access to a DB instance.
*
*
*
* A DB security group controls access to EC2-Classic DB instances that are not in a VPC.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbSecurityGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateDbSecurityGroupRequest#builder()}
*
*
* @param createDbSecurityGroupRequest
* A {@link Consumer} that will call methods on {@link CreateDBSecurityGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDBSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupAlreadyExistsException A DB security group with the name specified in
*
DBSecurityGroupName
already exists.
* - DbSecurityGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of DB security groups.
* - DbSecurityGroupNotSupportedException A DB security group isn't allowed for this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSecurityGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSecurityGroup(
Consumer createDbSecurityGroupRequest) {
return createDBSecurityGroup(CreateDbSecurityGroupRequest.builder().applyMutation(createDbSecurityGroupRequest).build());
}
/**
*
* Creates a DBSnapshot. The source DBInstance must be in "available" state.
*
*
* @param createDbSnapshotRequest
* @return A Java Future containing the result of the CreateDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSnapshot(CreateDbSnapshotRequest createDbSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a DBSnapshot. The source DBInstance must be in "available" state.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbSnapshotRequest.Builder} avoiding the need
* to create one manually via {@link CreateDbSnapshotRequest#builder()}
*
*
* @param createDbSnapshotRequest
* A {@link Consumer} that will call methods on {@link CreateDBSnapshotMessage.Builder} to create a request.
* @return A Java Future containing the result of the CreateDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSnapshot(
Consumer createDbSnapshotRequest) {
return createDBSnapshot(CreateDbSnapshotRequest.builder().applyMutation(createDbSnapshotRequest).build());
}
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the AWS
* Region.
*
*
* @param createDbSubnetGroupRequest
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupAlreadyExistsException
DBSubnetGroupName
is already used by an existing DB
* subnet group.
* - DbSubnetGroupQuotaExceededException The request would result in the user exceeding the allowed number
* of DB subnet groups.
* - DbSubnetQuotaExceededException The request would result in the user exceeding the allowed number of
* subnets in a DB subnet groups.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSubnetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSubnetGroup(
CreateDbSubnetGroupRequest createDbSubnetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the AWS
* Region.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDbSubnetGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateDbSubnetGroupRequest#builder()}
*
*
* @param createDbSubnetGroupRequest
* A {@link Consumer} that will call methods on {@link CreateDBSubnetGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupAlreadyExistsException
DBSubnetGroupName
is already used by an existing DB
* subnet group.
* - DbSubnetGroupQuotaExceededException The request would result in the user exceeding the allowed number
* of DB subnet groups.
* - DbSubnetQuotaExceededException The request would result in the user exceeding the allowed number of
* subnets in a DB subnet groups.
* - DbSubnetGroupDoesNotCoverEnoughAZsException Subnets in the DB subnet group should cover at least two
* Availability Zones unless there is only one Availability Zone.
* - InvalidSubnetException The requested subnet is invalid, or multiple subnets were requested that are
* not all in a common VPC.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateDBSubnetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createDBSubnetGroup(
Consumer createDbSubnetGroupRequest) {
return createDBSubnetGroup(CreateDbSubnetGroupRequest.builder().applyMutation(createDbSubnetGroupRequest).build());
}
/**
*
* Creates an RDS event notification subscription. This action requires a topic Amazon Resource Name (ARN) created
* by either the RDS console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType
) that you want to be notified of and provide a list
* of RDS sources (SourceIds
) that triggers the events. You can also provide a list of event categories
* (EventCategories
) for events that you want to be notified of. For example, you can specify
* SourceType
= db-instance
, SourceIds
= mydbinstance1
,
* mydbinstance2
and EventCategories
= Availability
, Backup
.
*
*
* If you specify both the SourceType
and SourceIds
, such as SourceType
=
* db-instance
and SourceIdentifier
= myDBInstance1
, you are notified of all
* the db-instance
events for the specified source. If you specify a SourceType
but do not
* specify a SourceIdentifier
, you receive notice of the events for that source type for all your RDS
* sources. If you don't specify either the SourceType or the SourceIdentifier
, you are notified of
* events generated from all RDS sources belonging to your customer account.
*
*
*
* RDS event notification is only available for unencrypted SNS topics. If you specify an encrypted SNS topic, event
* notifications aren't sent for the topic.
*
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EventSubscriptionQuotaExceededException You have reached the maximum number of event subscriptions.
* - SubscriptionAlreadyExistException The supplied subscription name already exists.
* - SnsInvalidTopicException SNS has responded that there is a problem with the SND topic specified.
* - SnsNoAuthorizationException You do not have permission to publish to the SNS topic ARN.
* - SnsTopicArnNotFoundException The SNS topic ARN does not exist.
* - SubscriptionCategoryNotFoundException The supplied category does not exist.
* - SourceNotFoundException The requested source could not be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateEventSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture createEventSubscription(
CreateEventSubscriptionRequest createEventSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an RDS event notification subscription. This action requires a topic Amazon Resource Name (ARN) created
* by either the RDS console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType
) that you want to be notified of and provide a list
* of RDS sources (SourceIds
) that triggers the events. You can also provide a list of event categories
* (EventCategories
) for events that you want to be notified of. For example, you can specify
* SourceType
= db-instance
, SourceIds
= mydbinstance1
,
* mydbinstance2
and EventCategories
= Availability
, Backup
.
*
*
* If you specify both the SourceType
and SourceIds
, such as SourceType
=
* db-instance
and SourceIdentifier
= myDBInstance1
, you are notified of all
* the db-instance
events for the specified source. If you specify a SourceType
but do not
* specify a SourceIdentifier
, you receive notice of the events for that source type for all your RDS
* sources. If you don't specify either the SourceType or the SourceIdentifier
, you are notified of
* events generated from all RDS sources belonging to your customer account.
*
*
*
* RDS event notification is only available for unencrypted SNS topics. If you specify an encrypted SNS topic, event
* notifications aren't sent for the topic.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEventSubscriptionRequest.Builder} avoiding
* the need to create one manually via {@link CreateEventSubscriptionRequest#builder()}
*
*
* @param createEventSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateEventSubscriptionMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - EventSubscriptionQuotaExceededException You have reached the maximum number of event subscriptions.
* - SubscriptionAlreadyExistException The supplied subscription name already exists.
* - SnsInvalidTopicException SNS has responded that there is a problem with the SND topic specified.
* - SnsNoAuthorizationException You do not have permission to publish to the SNS topic ARN.
* - SnsTopicArnNotFoundException The SNS topic ARN does not exist.
* - SubscriptionCategoryNotFoundException The supplied category does not exist.
* - SourceNotFoundException The requested source could not be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateEventSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture createEventSubscription(
Consumer createEventSubscriptionRequest) {
return createEventSubscription(CreateEventSubscriptionRequest.builder().applyMutation(createEventSubscriptionRequest)
.build());
}
/**
*
* Creates an Aurora global database spread across multiple regions. The global database contains a single primary
* cluster with read-write capability, and a read-only secondary cluster that receives data from the primary cluster
* through high-speed replication performed by the Aurora storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and a secondary cluster
* to it. Or you can specify an existing Aurora cluster during the create operation, and this cluster becomes the
* primary cluster of the global database.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createGlobalClusterRequest
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - GlobalClusterAlreadyExistsException
* - GlobalClusterQuotaExceededException
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateGlobalCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createGlobalCluster(
CreateGlobalClusterRequest createGlobalClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Aurora global database spread across multiple regions. The global database contains a single primary
* cluster with read-write capability, and a read-only secondary cluster that receives data from the primary cluster
* through high-speed replication performed by the Aurora storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and a secondary cluster
* to it. Or you can specify an existing Aurora cluster during the create operation, and this cluster becomes the
* primary cluster of the global database.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGlobalClusterRequest.Builder} avoiding the
* need to create one manually via {@link CreateGlobalClusterRequest#builder()}
*
*
* @param createGlobalClusterRequest
* A {@link Consumer} that will call methods on {@link CreateGlobalClusterMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - GlobalClusterAlreadyExistsException
* - GlobalClusterQuotaExceededException
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateGlobalCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createGlobalCluster(
Consumer createGlobalClusterRequest) {
return createGlobalCluster(CreateGlobalClusterRequest.builder().applyMutation(createGlobalClusterRequest).build());
}
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
* @param createOptionGroupRequest
* @return A Java Future containing the result of the CreateOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupAlreadyExistsException The option group you are trying to create already exists.
* - OptionGroupQuotaExceededException The quota of 20 option groups was exceeded for this AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createOptionGroup(CreateOptionGroupRequest createOptionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOptionGroupRequest.Builder} avoiding the need
* to create one manually via {@link CreateOptionGroupRequest#builder()}
*
*
* @param createOptionGroupRequest
* A {@link Consumer} that will call methods on {@link CreateOptionGroupMessage.Builder} to create a request.
* @return A Java Future containing the result of the CreateOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupAlreadyExistsException The option group you are trying to create already exists.
* - OptionGroupQuotaExceededException The quota of 20 option groups was exceeded for this AWS account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.CreateOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture createOptionGroup(
Consumer createOptionGroupRequest) {
return createOptionGroup(CreateOptionGroupRequest.builder().applyMutation(createOptionGroupRequest).build());
}
/**
*
* Deletes a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
* @param deleteCustomAvailabilityZoneRequest
* @return A Java Future containing the result of the DeleteCustomAvailabilityZone operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteCustomAvailabilityZone
* @see AWS API Documentation
*/
default CompletableFuture deleteCustomAvailabilityZone(
DeleteCustomAvailabilityZoneRequest deleteCustomAvailabilityZoneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCustomAvailabilityZoneRequest.Builder}
* avoiding the need to create one manually via {@link DeleteCustomAvailabilityZoneRequest#builder()}
*
*
* @param deleteCustomAvailabilityZoneRequest
* A {@link Consumer} that will call methods on {@link DeleteCustomAvailabilityZoneMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteCustomAvailabilityZone operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - KmsKeyNotAccessibleException An error occurred accessing an AWS KMS key.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteCustomAvailabilityZone
* @see AWS API Documentation
*/
default CompletableFuture deleteCustomAvailabilityZone(
Consumer deleteCustomAvailabilityZoneRequest) {
return deleteCustomAvailabilityZone(DeleteCustomAvailabilityZoneRequest.builder()
.applyMutation(deleteCustomAvailabilityZoneRequest).build());
}
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDbClusterRequest
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBCluster(DeleteDbClusterRequest deleteDbClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbClusterRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDbClusterRequest#builder()}
*
*
* @param deleteDbClusterRequest
* A {@link Consumer} that will call methods on {@link DeleteDBClusterMessage.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbClusterSnapshotAlreadyExistsException The user already has a DB cluster snapshot with the given
* identifier.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBCluster(
Consumer deleteDbClusterRequest) {
return deleteDBCluster(DeleteDbClusterRequest.builder().applyMutation(deleteDbClusterRequest).build());
}
/**
*
* Deletes a custom endpoint and removes it from an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDbClusterEndpointRequest
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbClusterEndpointStateException The requested operation can't be performed on the endpoint
* while the endpoint is in this state.
* - DbClusterEndpointNotFoundException The specified custom endpoint doesn't exist.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDBClusterEndpoint(
DeleteDbClusterEndpointRequest deleteDbClusterEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a custom endpoint and removes it from an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbClusterEndpointRequest.Builder} avoiding
* the need to create one manually via {@link DeleteDbClusterEndpointRequest#builder()}
*
*
* @param deleteDbClusterEndpointRequest
* A {@link Consumer} that will call methods on {@link DeleteDBClusterEndpointMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbClusterEndpointStateException The requested operation can't be performed on the endpoint
* while the endpoint is in this state.
* - DbClusterEndpointNotFoundException The specified custom endpoint doesn't exist.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDBClusterEndpoint(
Consumer deleteDbClusterEndpointRequest) {
return deleteDBClusterEndpoint(DeleteDbClusterEndpointRequest.builder().applyMutation(deleteDbClusterEndpointRequest)
.build());
}
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDbClusterParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbParameterGroupStateException The DB parameter group is in use or is in an invalid state. If
* you are attempting to delete the parameter group, you can't delete it when the parameter group is in this
* state.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteDBClusterParameterGroup(
DeleteDbClusterParameterGroupRequest deleteDbClusterParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbClusterParameterGroupRequest.Builder}
* avoiding the need to create one manually via {@link DeleteDbClusterParameterGroupRequest#builder()}
*
*
* @param deleteDbClusterParameterGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteDBClusterParameterGroupMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbParameterGroupStateException The DB parameter group is in use or is in an invalid state. If
* you are attempting to delete the parameter group, you can't delete it when the parameter group is in this
* state.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteDBClusterParameterGroup(
Consumer deleteDbClusterParameterGroupRequest) {
return deleteDBClusterParameterGroup(DeleteDbClusterParameterGroupRequest.builder()
.applyMutation(deleteDbClusterParameterGroupRequest).build());
}
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDbClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDBClusterSnapshot(
DeleteDbClusterSnapshotRequest deleteDbClusterSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbClusterSnapshotRequest.Builder} avoiding
* the need to create one manually via {@link DeleteDbClusterSnapshotRequest#builder()}
*
*
* @param deleteDbClusterSnapshotRequest
* A {@link Consumer} that will call methods on {@link DeleteDBClusterSnapshotMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbClusterSnapshotStateException The supplied value isn't a valid DB cluster snapshot state.
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBClusterSnapshot
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDBClusterSnapshot(
Consumer deleteDbClusterSnapshotRequest) {
return deleteDBClusterSnapshot(DeleteDbClusterSnapshotRequest.builder().applyMutation(deleteDbClusterSnapshotRequest)
.build());
}
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon RDS DB instance is deleting
until the DB
* snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* When a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when you skip
* creation of the final snapshot with the SkipFinalSnapshot
parameter.
*
*
* If the specified DB instance is part of an Amazon Aurora DB cluster, you can't delete the DB instance if both of
* the following conditions are true:
*
*
* -
*
* The DB cluster is a read replica of another Amazon Aurora DB cluster.
*
*
* -
*
* The DB instance is the only instance in the DB cluster.
*
*
*
*
* To delete a DB instance in this case, first call the PromoteReadReplicaDBCluster
API action to
* promote the DB cluster so it's no longer a read replica. After the promotion completes, then call the
* DeleteDBInstance
API action to delete the final instance in the DB cluster.
*
*
* @param deleteDbInstanceRequest
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbInstanceAutomatedBackupQuotaExceededException The quota for retained automated backups was
* exceeded. This prevents you from retaining any additional automated backups. The retained automated
* backups quota is the same as your DB Instance quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBInstance(DeleteDbInstanceRequest deleteDbInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon RDS DB instance is deleting
until the DB
* snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* When a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when you skip
* creation of the final snapshot with the SkipFinalSnapshot
parameter.
*
*
* If the specified DB instance is part of an Amazon Aurora DB cluster, you can't delete the DB instance if both of
* the following conditions are true:
*
*
* -
*
* The DB cluster is a read replica of another Amazon Aurora DB cluster.
*
*
* -
*
* The DB instance is the only instance in the DB cluster.
*
*
*
*
* To delete a DB instance in this case, first call the PromoteReadReplicaDBCluster
API action to
* promote the DB cluster so it's no longer a read replica. After the promotion completes, then call the
* DeleteDBInstance
API action to delete the final instance in the DB cluster.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbInstanceRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDbInstanceRequest#builder()}
*
*
* @param deleteDbInstanceRequest
* A {@link Consumer} that will call methods on {@link DeleteDBInstanceMessage.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - InvalidDbInstanceStateException The DB instance isn't in a valid state.
* - DbSnapshotAlreadyExistsException
DBSnapshotIdentifier
is already used by an existing
* snapshot.
* - SnapshotQuotaExceededException The request would result in the user exceeding the allowed number of
* DB snapshots.
* - InvalidDbClusterStateException The requested operation can't be performed while the cluster is in
* this state.
* - DbInstanceAutomatedBackupQuotaExceededException The quota for retained automated backups was
* exceeded. This prevents you from retaining any additional automated backups. The retained automated
* backups quota is the same as your DB Instance quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBInstance
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBInstance(
Consumer deleteDbInstanceRequest) {
return deleteDBInstance(DeleteDbInstanceRequest.builder().applyMutation(deleteDbInstanceRequest).build());
}
/**
*
* Deletes automated backups based on the source instance's DbiResourceId
value or the restorable
* instance's resource ID.
*
*
* @param deleteDbInstanceAutomatedBackupRequest
* Parameter input for the DeleteDBInstanceAutomatedBackup
operation.
* @return A Java Future containing the result of the DeleteDBInstanceAutomatedBackup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbInstanceAutomatedBackupStateException The automated backup is in an invalid state. For
* example, this automated backup is associated with an active instance.
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBInstanceAutomatedBackup
* @see AWS API Documentation
*/
default CompletableFuture deleteDBInstanceAutomatedBackup(
DeleteDbInstanceAutomatedBackupRequest deleteDbInstanceAutomatedBackupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes automated backups based on the source instance's DbiResourceId
value or the restorable
* instance's resource ID.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbInstanceAutomatedBackupRequest.Builder}
* avoiding the need to create one manually via {@link DeleteDbInstanceAutomatedBackupRequest#builder()}
*
*
* @param deleteDbInstanceAutomatedBackupRequest
* A {@link Consumer} that will call methods on {@link DeleteDBInstanceAutomatedBackupMessage.Builder} to
* create a request. Parameter input for the DeleteDBInstanceAutomatedBackup
operation.
* @return A Java Future containing the result of the DeleteDBInstanceAutomatedBackup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbInstanceAutomatedBackupStateException The automated backup is in an invalid state. For
* example, this automated backup is associated with an active instance.
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBInstanceAutomatedBackup
* @see AWS API Documentation
*/
default CompletableFuture deleteDBInstanceAutomatedBackup(
Consumer deleteDbInstanceAutomatedBackupRequest) {
return deleteDBInstanceAutomatedBackup(DeleteDbInstanceAutomatedBackupRequest.builder()
.applyMutation(deleteDbInstanceAutomatedBackupRequest).build());
}
/**
*
* Deletes a specified DB parameter group. The DB parameter group to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDbParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbParameterGroupStateException The DB parameter group is in use or is in an invalid state. If
* you are attempting to delete the parameter group, you can't delete it when the parameter group is in this
* state.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBParameterGroup(
DeleteDbParameterGroupRequest deleteDbParameterGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified DB parameter group. The DB parameter group to be deleted can't be associated with any DB
* instances.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbParameterGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteDbParameterGroupRequest#builder()}
*
*
* @param deleteDbParameterGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteDBParameterGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbParameterGroupStateException The DB parameter group is in use or is in an invalid state. If
* you are attempting to delete the parameter group, you can't delete it when the parameter group is in this
* state.
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBParameterGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBParameterGroup(
Consumer deleteDbParameterGroupRequest) {
return deleteDBParameterGroup(DeleteDbParameterGroupRequest.builder().applyMutation(deleteDbParameterGroupRequest)
.build());
}
/**
*
* Deletes an existing proxy.
*
*
* @param deleteDbProxyRequest
* @return A Java Future containing the result of the DeleteDBProxy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBProxy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBProxy(DeleteDbProxyRequest deleteDbProxyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing proxy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbProxyRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDbProxyRequest#builder()}
*
*
* @param deleteDbProxyRequest
* A {@link Consumer} that will call methods on {@link DeleteDBProxyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDBProxy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBProxy
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBProxy(Consumer deleteDbProxyRequest) {
return deleteDBProxy(DeleteDbProxyRequest.builder().applyMutation(deleteDbProxyRequest).build());
}
/**
*
* Deletes a DB security group.
*
*
*
* The specified DB security group must not be associated with any DB instances.
*
*
*
* @param deleteDbSecurityGroupRequest
* @return A Java Future containing the result of the DeleteDBSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSecurityGroupStateException The state of the DB security group doesn't allow deletion.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSecurityGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSecurityGroup(
DeleteDbSecurityGroupRequest deleteDbSecurityGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a DB security group.
*
*
*
* The specified DB security group must not be associated with any DB instances.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbSecurityGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteDbSecurityGroupRequest#builder()}
*
*
* @param deleteDbSecurityGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteDBSecurityGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDBSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSecurityGroupStateException The state of the DB security group doesn't allow deletion.
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSecurityGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSecurityGroup(
Consumer deleteDbSecurityGroupRequest) {
return deleteDBSecurityGroup(DeleteDbSecurityGroupRequest.builder().applyMutation(deleteDbSecurityGroupRequest).build());
}
/**
*
* Deletes a DB snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDbSnapshotRequest
* @return A Java Future containing the result of the DeleteDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSnapshotStateException The state of the DB snapshot doesn't allow deletion.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSnapshot(DeleteDbSnapshotRequest deleteDbSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a DB snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB snapshot must be in the available
state to be deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbSnapshotRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDbSnapshotRequest#builder()}
*
*
* @param deleteDbSnapshotRequest
* A {@link Consumer} that will call methods on {@link DeleteDBSnapshotMessage.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDBSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSnapshotStateException The state of the DB snapshot doesn't allow deletion.
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSnapshot(
Consumer deleteDbSnapshotRequest) {
return deleteDBSnapshot(DeleteDbSnapshotRequest.builder().applyMutation(deleteDbSnapshotRequest).build());
}
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDbSubnetGroupRequest
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSubnetGroupStateException The DB subnet group cannot be deleted because it's in use.
* - InvalidDbSubnetStateException The DB subnet isn't in the available state.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSubnetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSubnetGroup(
DeleteDbSubnetGroupRequest deleteDbSubnetGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDbSubnetGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteDbSubnetGroupRequest#builder()}
*
*
* @param deleteDbSubnetGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteDBSubnetGroupMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidDbSubnetGroupStateException The DB subnet group cannot be deleted because it's in use.
* - InvalidDbSubnetStateException The DB subnet isn't in the available state.
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteDBSubnetGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDBSubnetGroup(
Consumer deleteDbSubnetGroupRequest) {
return deleteDBSubnetGroup(DeleteDbSubnetGroupRequest.builder().applyMutation(deleteDbSubnetGroupRequest).build());
}
/**
*
* Deletes an RDS event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SubscriptionNotFoundException The subscription name does not exist.
* - InvalidEventSubscriptionStateException This error can occur if someone else is modifying a
* subscription. You should retry the action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteEventSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture deleteEventSubscription(
DeleteEventSubscriptionRequest deleteEventSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an RDS event notification subscription.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEventSubscriptionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteEventSubscriptionRequest#builder()}
*
*
* @param deleteEventSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DeleteEventSubscriptionMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SubscriptionNotFoundException The subscription name does not exist.
* - InvalidEventSubscriptionStateException This error can occur if someone else is modifying a
* subscription. You should retry the action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteEventSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture deleteEventSubscription(
Consumer deleteEventSubscriptionRequest) {
return deleteEventSubscription(DeleteEventSubscriptionRequest.builder().applyMutation(deleteEventSubscriptionRequest)
.build());
}
/**
*
* Deletes a global database cluster. The primary and secondary clusters must already be detached or destroyed
* first.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteGlobalClusterRequest
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - GlobalClusterNotFoundException
* - InvalidGlobalClusterStateException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteGlobalCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteGlobalCluster(
DeleteGlobalClusterRequest deleteGlobalClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a global database cluster. The primary and secondary clusters must already be detached or destroyed
* first.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGlobalClusterRequest.Builder} avoiding the
* need to create one manually via {@link DeleteGlobalClusterRequest#builder()}
*
*
* @param deleteGlobalClusterRequest
* A {@link Consumer} that will call methods on {@link DeleteGlobalClusterMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - GlobalClusterNotFoundException
* - InvalidGlobalClusterStateException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteGlobalCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteGlobalCluster(
Consumer deleteGlobalClusterRequest) {
return deleteGlobalCluster(DeleteGlobalClusterRequest.builder().applyMutation(deleteGlobalClusterRequest).build());
}
/**
*
* Deletes the installation medium for a DB engine that requires an on-premises customer provided license, such as
* Microsoft SQL Server.
*
*
* @param deleteInstallationMediaRequest
* @return A Java Future containing the result of the DeleteInstallationMedia operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InstallationMediaNotFoundException
InstallationMediaID
doesn't refer to an existing
* installation medium.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteInstallationMedia
* @see AWS
* API Documentation
*/
default CompletableFuture deleteInstallationMedia(
DeleteInstallationMediaRequest deleteInstallationMediaRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the installation medium for a DB engine that requires an on-premises customer provided license, such as
* Microsoft SQL Server.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInstallationMediaRequest.Builder} avoiding
* the need to create one manually via {@link DeleteInstallationMediaRequest#builder()}
*
*
* @param deleteInstallationMediaRequest
* A {@link Consumer} that will call methods on {@link DeleteInstallationMediaMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteInstallationMedia operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InstallationMediaNotFoundException
InstallationMediaID
doesn't refer to an existing
* installation medium.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteInstallationMedia
* @see AWS
* API Documentation
*/
default CompletableFuture deleteInstallationMedia(
Consumer deleteInstallationMediaRequest) {
return deleteInstallationMedia(DeleteInstallationMediaRequest.builder().applyMutation(deleteInstallationMediaRequest)
.build());
}
/**
*
* Deletes an existing option group.
*
*
* @param deleteOptionGroupRequest
* @return A Java Future containing the result of the DeleteOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupNotFoundException The specified option group could not be found.
* - InvalidOptionGroupStateException The option group isn't in the available state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOptionGroup(DeleteOptionGroupRequest deleteOptionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing option group.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteOptionGroupRequest.Builder} avoiding the need
* to create one manually via {@link DeleteOptionGroupRequest#builder()}
*
*
* @param deleteOptionGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteOptionGroupMessage.Builder} to create a request.
* @return A Java Future containing the result of the DeleteOptionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - OptionGroupNotFoundException The specified option group could not be found.
* - InvalidOptionGroupStateException The option group isn't in the available state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeleteOptionGroup
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOptionGroup(
Consumer deleteOptionGroupRequest) {
return deleteOptionGroup(DeleteOptionGroupRequest.builder().applyMutation(deleteOptionGroupRequest).build());
}
/**
*
* Remove the association between one or more DBProxyTarget
data structures and a
* DBProxyTargetGroup
.
*
*
* @param deregisterDbProxyTargetsRequest
* @return A Java Future containing the result of the DeregisterDBProxyTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeregisterDBProxyTargets
* @see AWS
* API Documentation
*/
default CompletableFuture deregisterDBProxyTargets(
DeregisterDbProxyTargetsRequest deregisterDbProxyTargetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Remove the association between one or more DBProxyTarget
data structures and a
* DBProxyTargetGroup
.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterDbProxyTargetsRequest.Builder} avoiding
* the need to create one manually via {@link DeregisterDbProxyTargetsRequest#builder()}
*
*
* @param deregisterDbProxyTargetsRequest
* A {@link Consumer} that will call methods on {@link DeregisterDBProxyTargetsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeregisterDBProxyTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DeregisterDBProxyTargets
* @see AWS
* API Documentation
*/
default CompletableFuture deregisterDBProxyTargets(
Consumer deregisterDbProxyTargetsRequest) {
return deregisterDBProxyTargets(DeregisterDbProxyTargetsRequest.builder().applyMutation(deregisterDbProxyTargetsRequest)
.build());
}
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
default CompletableFuture describeAccountAttributes(
DescribeAccountAttributesRequest describeAccountAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAccountAttributesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeAccountAttributesRequest#builder()}
*
*
* @param describeAccountAttributesRequest
* A {@link Consumer} that will call methods on {@link DescribeAccountAttributesMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
default CompletableFuture describeAccountAttributes(
Consumer describeAccountAttributesRequest) {
return describeAccountAttributes(DescribeAccountAttributesRequest.builder()
.applyMutation(describeAccountAttributesRequest).build());
}
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
default CompletableFuture describeAccountAttributes() {
return describeAccountAttributes(DescribeAccountAttributesRequest.builder().build());
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
* @param describeCertificatesRequest
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default CompletableFuture describeCertificates(
DescribeCertificatesRequest describeCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCertificatesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCertificatesRequest#builder()}
*
*
* @param describeCertificatesRequest
* A {@link Consumer} that will call methods on {@link DescribeCertificatesMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default CompletableFuture describeCertificates(
Consumer describeCertificatesRequest) {
return describeCertificates(DescribeCertificatesRequest.builder().applyMutation(describeCertificatesRequest).build());
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default CompletableFuture describeCertificates() {
return describeCertificates(DescribeCertificatesRequest.builder().build());
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
*
* This is a variant of
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default DescribeCertificatesPublisher describeCertificatesPaginator() {
return describeCertificatesPaginator(DescribeCertificatesRequest.builder().build());
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
*
* This is a variant of
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)}
* operation.
*
*
* @param describeCertificatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default DescribeCertificatesPublisher describeCertificatesPaginator(DescribeCertificatesRequest describeCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this AWS account.
*
*
*
* This is a variant of
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCertificatesPublisher publisher = client.describeCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCertificates(software.amazon.awssdk.services.rds.model.DescribeCertificatesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeCertificatesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeCertificatesRequest#builder()}
*
*
* @param describeCertificatesRequest
* A {@link Consumer} that will call methods on {@link DescribeCertificatesMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CertificateNotFoundException
CertificateIdentifier
doesn't refer to an existing
* certificate.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCertificates
* @see AWS API
* Documentation
*/
default DescribeCertificatesPublisher describeCertificatesPaginator(
Consumer describeCertificatesRequest) {
return describeCertificatesPaginator(DescribeCertificatesRequest.builder().applyMutation(describeCertificatesRequest)
.build());
}
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
* @param describeCustomAvailabilityZonesRequest
* @return A Java Future containing the result of the DescribeCustomAvailabilityZones operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
default CompletableFuture describeCustomAvailabilityZones(
DescribeCustomAvailabilityZonesRequest describeCustomAvailabilityZonesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCustomAvailabilityZonesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCustomAvailabilityZonesRequest#builder()}
*
*
* @param describeCustomAvailabilityZonesRequest
* A {@link Consumer} that will call methods on {@link DescribeCustomAvailabilityZonesMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeCustomAvailabilityZones operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
default CompletableFuture describeCustomAvailabilityZones(
Consumer describeCustomAvailabilityZonesRequest) {
return describeCustomAvailabilityZones(DescribeCustomAvailabilityZonesRequest.builder()
.applyMutation(describeCustomAvailabilityZonesRequest).build());
}
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
*
* This is a variant of
* {@link #describeCustomAvailabilityZones(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCustomAvailabilityZonesPublisher publisher = client.describeCustomAvailabilityZonesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCustomAvailabilityZonesPublisher publisher = client.describeCustomAvailabilityZonesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCustomAvailabilityZones(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesRequest)}
* operation.
*
*
* @param describeCustomAvailabilityZonesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
default DescribeCustomAvailabilityZonesPublisher describeCustomAvailabilityZonesPaginator(
DescribeCustomAvailabilityZonesRequest describeCustomAvailabilityZonesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware
* User Guide.
*
*
*
* This is a variant of
* {@link #describeCustomAvailabilityZones(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCustomAvailabilityZonesPublisher publisher = client.describeCustomAvailabilityZonesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeCustomAvailabilityZonesPublisher publisher = client.describeCustomAvailabilityZonesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeCustomAvailabilityZones(software.amazon.awssdk.services.rds.model.DescribeCustomAvailabilityZonesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeCustomAvailabilityZonesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCustomAvailabilityZonesRequest#builder()}
*
*
* @param describeCustomAvailabilityZonesRequest
* A {@link Consumer} that will call methods on {@link DescribeCustomAvailabilityZonesMessage.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CustomAvailabilityZoneNotFoundException
CustomAvailabilityZoneId
doesn't refer to an
* existing custom Availability Zone identifier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
default DescribeCustomAvailabilityZonesPublisher describeCustomAvailabilityZonesPaginator(
Consumer describeCustomAvailabilityZonesRequest) {
return describeCustomAvailabilityZonesPaginator(DescribeCustomAvailabilityZonesRequest.builder()
.applyMutation(describeCustomAvailabilityZonesRequest).build());
}
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param describeDbClusterBacktracksRequest
* @return A Java Future containing the result of the DescribeDBClusterBacktracks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterBacktrackNotFoundException
BacktrackIdentifier
doesn't refer to an existing
* backtrack.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterBacktracks(
DescribeDbClusterBacktracksRequest describeDbClusterBacktracksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterBacktracksRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterBacktracksRequest#builder()}
*
*
* @param describeDbClusterBacktracksRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterBacktracksMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeDBClusterBacktracks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterBacktrackNotFoundException
BacktrackIdentifier
doesn't refer to an existing
* backtrack.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterBacktracks(
Consumer describeDbClusterBacktracksRequest) {
return describeDBClusterBacktracks(DescribeDbClusterBacktracksRequest.builder()
.applyMutation(describeDbClusterBacktracksRequest).build());
}
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterBacktracks(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterBacktracksPublisher publisher = client.describeDBClusterBacktracksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterBacktracksPublisher publisher = client.describeDBClusterBacktracksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterBacktracks(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksRequest)}
* operation.
*
*
* @param describeDbClusterBacktracksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterBacktrackNotFoundException
BacktrackIdentifier
doesn't refer to an existing
* backtrack.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
default DescribeDBClusterBacktracksPublisher describeDBClusterBacktracksPaginator(
DescribeDbClusterBacktracksRequest describeDbClusterBacktracksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterBacktracks(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterBacktracksPublisher publisher = client.describeDBClusterBacktracksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterBacktracksPublisher publisher = client.describeDBClusterBacktracksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterBacktracks(software.amazon.awssdk.services.rds.model.DescribeDbClusterBacktracksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterBacktracksRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterBacktracksRequest#builder()}
*
*
* @param describeDbClusterBacktracksRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterBacktracksMessage.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - DbClusterBacktrackNotFoundException
BacktrackIdentifier
doesn't refer to an existing
* backtrack.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
default DescribeDBClusterBacktracksPublisher describeDBClusterBacktracksPaginator(
Consumer describeDbClusterBacktracksRequest) {
return describeDBClusterBacktracksPaginator(DescribeDbClusterBacktracksRequest.builder()
.applyMutation(describeDbClusterBacktracksRequest).build());
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDbClusterEndpointsRequest
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterEndpoints(
DescribeDbClusterEndpointsRequest describeDbClusterEndpointsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterEndpointsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbClusterEndpointsRequest#builder()}
*
*
* @param describeDbClusterEndpointsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterEndpointsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterEndpoints(
Consumer describeDbClusterEndpointsRequest) {
return describeDBClusterEndpoints(DescribeDbClusterEndpointsRequest.builder()
.applyMutation(describeDbClusterEndpointsRequest).build());
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterEndpoints() {
return describeDBClusterEndpoints(DescribeDbClusterEndpointsRequest.builder().build());
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default DescribeDBClusterEndpointsPublisher describeDBClusterEndpointsPaginator() {
return describeDBClusterEndpointsPaginator(DescribeDbClusterEndpointsRequest.builder().build());
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation.
*
*
* @param describeDbClusterEndpointsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default DescribeDBClusterEndpointsPublisher describeDBClusterEndpointsPaginator(
DescribeDbClusterEndpointsRequest describeDbClusterEndpointsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterEndpointsPublisher publisher = client.describeDBClusterEndpointsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterEndpoints(software.amazon.awssdk.services.rds.model.DescribeDbClusterEndpointsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterEndpointsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbClusterEndpointsRequest#builder()}
*
*
* @param describeDbClusterEndpointsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterEndpointsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
default DescribeDBClusterEndpointsPublisher describeDBClusterEndpointsPaginator(
Consumer describeDbClusterEndpointsRequest) {
return describeDBClusterEndpointsPaginator(DescribeDbClusterEndpointsRequest.builder()
.applyMutation(describeDbClusterEndpointsRequest).build());
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDbClusterParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterParameterGroups(
DescribeDbClusterParameterGroupsRequest describeDbClusterParameterGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterParameterGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterParameterGroupsRequest#builder()}
*
*
* @param describeDbClusterParameterGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterParameterGroupsMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterParameterGroups(
Consumer describeDbClusterParameterGroupsRequest) {
return describeDBClusterParameterGroups(DescribeDbClusterParameterGroupsRequest.builder()
.applyMutation(describeDbClusterParameterGroupsRequest).build());
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterParameterGroups() {
return describeDBClusterParameterGroups(DescribeDbClusterParameterGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default DescribeDBClusterParameterGroupsPublisher describeDBClusterParameterGroupsPaginator() {
return describeDBClusterParameterGroupsPaginator(DescribeDbClusterParameterGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation.
*
*
* @param describeDbClusterParameterGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default DescribeDBClusterParameterGroupsPublisher describeDBClusterParameterGroupsPaginator(
DescribeDbClusterParameterGroupsRequest describeDbClusterParameterGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParameterGroupsPublisher publisher = client.describeDBClusterParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbClusterParameterGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterParameterGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterParameterGroupsRequest#builder()}
*
*
* @param describeDbClusterParameterGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterParameterGroupsMessage.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
default DescribeDBClusterParameterGroupsPublisher describeDBClusterParameterGroupsPaginator(
Consumer describeDbClusterParameterGroupsRequest) {
return describeDBClusterParameterGroupsPaginator(DescribeDbClusterParameterGroupsRequest.builder()
.applyMutation(describeDbClusterParameterGroupsRequest).build());
}
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDbClusterParametersRequest
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameters
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterParameters(
DescribeDbClusterParametersRequest describeDbClusterParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterParametersRequest#builder()}
*
*
* @param describeDbClusterParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterParametersMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameters
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterParameters(
Consumer describeDbClusterParametersRequest) {
return describeDBClusterParameters(DescribeDbClusterParametersRequest.builder()
.applyMutation(describeDbClusterParametersRequest).build());
}
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterParameters(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParametersPublisher publisher = client.describeDBClusterParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParametersPublisher publisher = client.describeDBClusterParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterParameters(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersRequest)}
* operation.
*
*
* @param describeDbClusterParametersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameters
* @see AWS API Documentation
*/
default DescribeDBClusterParametersPublisher describeDBClusterParametersPaginator(
DescribeDbClusterParametersRequest describeDbClusterParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterParameters(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParametersPublisher publisher = client.describeDBClusterParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterParametersPublisher publisher = client.describeDBClusterParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterParameters(software.amazon.awssdk.services.rds.model.DescribeDbClusterParametersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterParametersRequest#builder()}
*
*
* @param describeDbClusterParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterParametersMessage.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterParameters
* @see AWS API Documentation
*/
default DescribeDBClusterParametersPublisher describeDBClusterParametersPaginator(
Consumer describeDbClusterParametersRequest) {
return describeDBClusterParametersPaginator(DescribeDbClusterParametersRequest.builder()
.applyMutation(describeDbClusterParametersRequest).build());
}
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other AWS accounts, DescribeDBClusterSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the AWS accounts that are authorized to copy or restore the
* manual DB cluster snapshot. If all
is included in the list of values for the restore
* attribute, then the manual DB cluster snapshot is public and can be copied or restored by all AWS accounts.
*
*
* To add or remove access for an AWS account to copy or restore a manual DB cluster snapshot, or to make the manual
* DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute
API action.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDbClusterSnapshotAttributesRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterSnapshotAttributes(
DescribeDbClusterSnapshotAttributesRequest describeDbClusterSnapshotAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other AWS accounts, DescribeDBClusterSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the AWS accounts that are authorized to copy or restore the
* manual DB cluster snapshot. If all
is included in the list of values for the restore
* attribute, then the manual DB cluster snapshot is public and can be copied or restored by all AWS accounts.
*
*
* To add or remove access for an AWS account to copy or restore a manual DB cluster snapshot, or to make the manual
* DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute
API action.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterSnapshotAttributesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbClusterSnapshotAttributesRequest#builder()}
*
*
* @param describeDbClusterSnapshotAttributesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterSnapshotAttributesMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeDBClusterSnapshotAttributes(
Consumer describeDbClusterSnapshotAttributesRequest) {
return describeDBClusterSnapshotAttributes(DescribeDbClusterSnapshotAttributesRequest.builder()
.applyMutation(describeDbClusterSnapshotAttributesRequest).build());
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDbClusterSnapshotsRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterSnapshots(
DescribeDbClusterSnapshotsRequest describeDbClusterSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterSnapshotsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbClusterSnapshotsRequest#builder()}
*
*
* @param describeDbClusterSnapshotsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterSnapshotsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterSnapshots(
Consumer describeDbClusterSnapshotsRequest) {
return describeDBClusterSnapshots(DescribeDbClusterSnapshotsRequest.builder()
.applyMutation(describeDbClusterSnapshotsRequest).build());
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBClusterSnapshots() {
return describeDBClusterSnapshots(DescribeDbClusterSnapshotsRequest.builder().build());
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default DescribeDBClusterSnapshotsPublisher describeDBClusterSnapshotsPaginator() {
return describeDBClusterSnapshotsPaginator(DescribeDbClusterSnapshotsRequest.builder().build());
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation.
*
*
* @param describeDbClusterSnapshotsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default DescribeDBClusterSnapshotsPublisher describeDBClusterSnapshotsPaginator(
DescribeDbClusterSnapshotsRequest describeDbClusterSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* This is a variant of
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClusterSnapshotsPublisher publisher = client.describeDBClusterSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusterSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbClusterSnapshotsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClusterSnapshotsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbClusterSnapshotsRequest#builder()}
*
*
* @param describeDbClusterSnapshotsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClusterSnapshotsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterSnapshotNotFoundException
DBClusterSnapshotIdentifier
doesn't refer to an
* existing DB cluster snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
default DescribeDBClusterSnapshotsPublisher describeDBClusterSnapshotsPaginator(
Consumer describeDbClusterSnapshotsRequest) {
return describeDBClusterSnapshotsPaginator(DescribeDbClusterSnapshotsRequest.builder()
.applyMutation(describeDbClusterSnapshotsRequest).build());
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDbClustersRequest
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBClusters(DescribeDbClustersRequest describeDbClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbClustersRequest#builder()}
*
*
* @param describeDbClustersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClustersMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBClusters(
Consumer describeDbClustersRequest) {
return describeDBClusters(DescribeDbClustersRequest.builder().applyMutation(describeDbClustersRequest).build());
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBClusters() {
return describeDBClusters(DescribeDbClustersRequest.builder().build());
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default DescribeDBClustersPublisher describeDBClustersPaginator() {
return describeDBClustersPaginator(DescribeDbClustersRequest.builder().build());
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation.
*
*
* @param describeDbClustersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default DescribeDBClustersPublisher describeDBClustersPaginator(DescribeDbClustersRequest describeDbClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBClustersPublisher publisher = client.describeDBClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBClusters(software.amazon.awssdk.services.rds.model.DescribeDbClustersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbClustersRequest#builder()}
*
*
* @param describeDbClustersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBClustersMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbClusterNotFoundException
DBClusterIdentifier
doesn't refer to an existing DB cluster.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBClusters
* @see AWS API
* Documentation
*/
default DescribeDBClustersPublisher describeDBClustersPaginator(
Consumer describeDbClustersRequest) {
return describeDBClustersPaginator(DescribeDbClustersRequest.builder().applyMutation(describeDbClustersRequest).build());
}
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDbEngineVersionsRequest
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBEngineVersions(
DescribeDbEngineVersionsRequest describeDbEngineVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the available DB engines.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbEngineVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbEngineVersionsRequest#builder()}
*
*
* @param describeDbEngineVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBEngineVersionsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBEngineVersions(
Consumer describeDbEngineVersionsRequest) {
return describeDBEngineVersions(DescribeDbEngineVersionsRequest.builder().applyMutation(describeDbEngineVersionsRequest)
.build());
}
/**
*
* Returns a list of the available DB engines.
*
*
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBEngineVersions() {
return describeDBEngineVersions(DescribeDbEngineVersionsRequest.builder().build());
}
/**
*
* Returns a list of the available DB engines.
*
*
*
* This is a variant of
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default DescribeDBEngineVersionsPublisher describeDBEngineVersionsPaginator() {
return describeDBEngineVersionsPaginator(DescribeDbEngineVersionsRequest.builder().build());
}
/**
*
* Returns a list of the available DB engines.
*
*
*
* This is a variant of
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation.
*
*
* @param describeDbEngineVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default DescribeDBEngineVersionsPublisher describeDBEngineVersionsPaginator(
DescribeDbEngineVersionsRequest describeDbEngineVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the available DB engines.
*
*
*
* This is a variant of
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBEngineVersionsPublisher publisher = client.describeDBEngineVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBEngineVersions(software.amazon.awssdk.services.rds.model.DescribeDbEngineVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbEngineVersionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbEngineVersionsRequest#builder()}
*
*
* @param describeDbEngineVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBEngineVersionsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
default DescribeDBEngineVersionsPublisher describeDBEngineVersionsPaginator(
Consumer describeDbEngineVersionsRequest) {
return describeDBEngineVersionsPaginator(DescribeDbEngineVersionsRequest.builder()
.applyMutation(describeDbEngineVersionsRequest).build());
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
* @param describeDbInstanceAutomatedBackupsRequest
* Parameter input for DescribeDBInstanceAutomatedBackups.
* @return A Java Future containing the result of the DescribeDBInstanceAutomatedBackups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default CompletableFuture describeDBInstanceAutomatedBackups(
DescribeDbInstanceAutomatedBackupsRequest describeDbInstanceAutomatedBackupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbInstanceAutomatedBackupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbInstanceAutomatedBackupsRequest#builder()}
*
*
* @param describeDbInstanceAutomatedBackupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBInstanceAutomatedBackupsMessage.Builder} to
* create a request. Parameter input for DescribeDBInstanceAutomatedBackups.
* @return A Java Future containing the result of the DescribeDBInstanceAutomatedBackups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default CompletableFuture describeDBInstanceAutomatedBackups(
Consumer describeDbInstanceAutomatedBackupsRequest) {
return describeDBInstanceAutomatedBackups(DescribeDbInstanceAutomatedBackupsRequest.builder()
.applyMutation(describeDbInstanceAutomatedBackupsRequest).build());
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
* @return A Java Future containing the result of the DescribeDBInstanceAutomatedBackups operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default CompletableFuture describeDBInstanceAutomatedBackups() {
return describeDBInstanceAutomatedBackups(DescribeDbInstanceAutomatedBackupsRequest.builder().build());
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
*
* This is a variant of
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default DescribeDBInstanceAutomatedBackupsPublisher describeDBInstanceAutomatedBackupsPaginator() {
return describeDBInstanceAutomatedBackupsPaginator(DescribeDbInstanceAutomatedBackupsRequest.builder().build());
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
*
* This is a variant of
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation.
*
*
* @param describeDbInstanceAutomatedBackupsRequest
* Parameter input for DescribeDBInstanceAutomatedBackups.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default DescribeDBInstanceAutomatedBackupsPublisher describeDBInstanceAutomatedBackupsPaginator(
DescribeDbInstanceAutomatedBackupsRequest describeDbInstanceAutomatedBackupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
*
* This is a variant of
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstanceAutomatedBackupsPublisher publisher = client.describeDBInstanceAutomatedBackupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstanceAutomatedBackups(software.amazon.awssdk.services.rds.model.DescribeDbInstanceAutomatedBackupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbInstanceAutomatedBackupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbInstanceAutomatedBackupsRequest#builder()}
*
*
* @param describeDbInstanceAutomatedBackupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBInstanceAutomatedBackupsMessage.Builder} to
* create a request. Parameter input for DescribeDBInstanceAutomatedBackups.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceAutomatedBackupNotFoundException No automated backup for this DB instance was found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
default DescribeDBInstanceAutomatedBackupsPublisher describeDBInstanceAutomatedBackupsPaginator(
Consumer describeDbInstanceAutomatedBackupsRequest) {
return describeDBInstanceAutomatedBackupsPaginator(DescribeDbInstanceAutomatedBackupsRequest.builder()
.applyMutation(describeDbInstanceAutomatedBackupsRequest).build());
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDbInstancesRequest
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBInstances(
DescribeDbInstancesRequest describeDbInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbInstancesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbInstancesRequest#builder()}
*
*
* @param describeDbInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBInstancesMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBInstances(
Consumer describeDbInstancesRequest) {
return describeDBInstances(DescribeDbInstancesRequest.builder().applyMutation(describeDbInstancesRequest).build());
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBInstances() {
return describeDBInstances(DescribeDbInstancesRequest.builder().build());
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default DescribeDBInstancesPublisher describeDBInstancesPaginator() {
return describeDBInstancesPaginator(DescribeDbInstancesRequest.builder().build());
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation.
*
*
* @param describeDbInstancesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default DescribeDBInstancesPublisher describeDBInstancesPaginator(DescribeDbInstancesRequest describeDbInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* This is a variant of
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBInstancesPublisher publisher = client.describeDBInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBInstances(software.amazon.awssdk.services.rds.model.DescribeDbInstancesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbInstancesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbInstancesRequest#builder()}
*
*
* @param describeDbInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBInstancesMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBInstances
* @see AWS API
* Documentation
*/
default DescribeDBInstancesPublisher describeDBInstancesPaginator(
Consumer describeDbInstancesRequest) {
return describeDBInstancesPaginator(DescribeDbInstancesRequest.builder().applyMutation(describeDbInstancesRequest)
.build());
}
/**
*
* Returns a list of DB log files for the DB instance.
*
*
* @param describeDbLogFilesRequest
* @return A Java Future containing the result of the DescribeDBLogFiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBLogFiles(DescribeDbLogFilesRequest describeDbLogFilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DB log files for the DB instance.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbLogFilesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbLogFilesRequest#builder()}
*
*
* @param describeDbLogFilesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBLogFilesMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBLogFiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBLogFiles(
Consumer describeDbLogFilesRequest) {
return describeDBLogFiles(DescribeDbLogFilesRequest.builder().applyMutation(describeDbLogFilesRequest).build());
}
/**
*
* Returns a list of DB log files for the DB instance.
*
*
*
* This is a variant of
* {@link #describeDBLogFiles(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBLogFilesPublisher publisher = client.describeDBLogFilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBLogFilesPublisher publisher = client.describeDBLogFilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBLogFiles(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesRequest)} operation.
*
*
* @param describeDbLogFilesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
default DescribeDBLogFilesPublisher describeDBLogFilesPaginator(DescribeDbLogFilesRequest describeDbLogFilesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DB log files for the DB instance.
*
*
*
* This is a variant of
* {@link #describeDBLogFiles(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBLogFilesPublisher publisher = client.describeDBLogFilesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBLogFilesPublisher publisher = client.describeDBLogFilesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBLogFiles(software.amazon.awssdk.services.rds.model.DescribeDbLogFilesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbLogFilesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbLogFilesRequest#builder()}
*
*
* @param describeDbLogFilesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBLogFilesMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbInstanceNotFoundException
DBInstanceIdentifier
doesn't refer to an existing DB
* instance.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
default DescribeDBLogFilesPublisher describeDBLogFilesPaginator(
Consumer describeDbLogFilesRequest) {
return describeDBLogFilesPaginator(DescribeDbLogFilesRequest.builder().applyMutation(describeDbLogFilesRequest).build());
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @param describeDbParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBParameterGroups(
DescribeDbParameterGroupsRequest describeDbParameterGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbParameterGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbParameterGroupsRequest#builder()}
*
*
* @param describeDbParameterGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBParameterGroupsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBParameterGroups(
Consumer describeDbParameterGroupsRequest) {
return describeDBParameterGroups(DescribeDbParameterGroupsRequest.builder()
.applyMutation(describeDbParameterGroupsRequest).build());
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBParameterGroups() {
return describeDBParameterGroups(DescribeDbParameterGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
*
* This is a variant of
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default DescribeDBParameterGroupsPublisher describeDBParameterGroupsPaginator() {
return describeDBParameterGroupsPaginator(DescribeDbParameterGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
*
* This is a variant of
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation.
*
*
* @param describeDbParameterGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default DescribeDBParameterGroupsPublisher describeDBParameterGroupsPaginator(
DescribeDbParameterGroupsRequest describeDbParameterGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
*
* This is a variant of
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParameterGroupsPublisher publisher = client.describeDBParameterGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBParameterGroups(software.amazon.awssdk.services.rds.model.DescribeDbParameterGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbParameterGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbParameterGroupsRequest#builder()}
*
*
* @param describeDbParameterGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBParameterGroupsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
default DescribeDBParameterGroupsPublisher describeDBParameterGroupsPaginator(
Consumer describeDbParameterGroupsRequest) {
return describeDBParameterGroupsPaginator(DescribeDbParameterGroupsRequest.builder()
.applyMutation(describeDbParameterGroupsRequest).build());
}
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
* @param describeDbParametersRequest
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameters
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBParameters(
DescribeDbParametersRequest describeDbParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbParametersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbParametersRequest#builder()}
*
*
* @param describeDbParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBParametersMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameters
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBParameters(
Consumer describeDbParametersRequest) {
return describeDBParameters(DescribeDbParametersRequest.builder().applyMutation(describeDbParametersRequest).build());
}
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
*
* This is a variant of
* {@link #describeDBParameters(software.amazon.awssdk.services.rds.model.DescribeDbParametersRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParametersPublisher publisher = client.describeDBParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParametersPublisher publisher = client.describeDBParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBParameters(software.amazon.awssdk.services.rds.model.DescribeDbParametersRequest)}
* operation.
*
*
* @param describeDbParametersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameters
* @see AWS API
* Documentation
*/
default DescribeDBParametersPublisher describeDBParametersPaginator(DescribeDbParametersRequest describeDbParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
*
* This is a variant of
* {@link #describeDBParameters(software.amazon.awssdk.services.rds.model.DescribeDbParametersRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParametersPublisher publisher = client.describeDBParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBParametersPublisher publisher = client.describeDBParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBParameters(software.amazon.awssdk.services.rds.model.DescribeDbParametersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbParametersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbParametersRequest#builder()}
*
*
* @param describeDbParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeDBParametersMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbParameterGroupNotFoundException
DBParameterGroupName
doesn't refer to an existing DB
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBParameters
* @see AWS API
* Documentation
*/
default DescribeDBParametersPublisher describeDBParametersPaginator(
Consumer describeDbParametersRequest) {
return describeDBParametersPaginator(DescribeDbParametersRequest.builder().applyMutation(describeDbParametersRequest)
.build());
}
/**
*
* Returns information about DB proxies.
*
*
* @param describeDbProxiesRequest
* @return A Java Future containing the result of the DescribeDBProxies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxies
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBProxies(DescribeDbProxiesRequest describeDbProxiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB proxies.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxiesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDbProxiesRequest#builder()}
*
*
* @param describeDbProxiesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxiesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeDBProxies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxies
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBProxies(
Consumer describeDbProxiesRequest) {
return describeDBProxies(DescribeDbProxiesRequest.builder().applyMutation(describeDbProxiesRequest).build());
}
/**
*
* Returns information about DB proxies.
*
*
*
* This is a variant of
* {@link #describeDBProxies(software.amazon.awssdk.services.rds.model.DescribeDbProxiesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxiesPublisher publisher = client.describeDBProxiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxiesPublisher publisher = client.describeDBProxiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxies(software.amazon.awssdk.services.rds.model.DescribeDbProxiesRequest)} operation.
*
*
* @param describeDbProxiesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxies
* @see AWS API
* Documentation
*/
default DescribeDBProxiesPublisher describeDBProxiesPaginator(DescribeDbProxiesRequest describeDbProxiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB proxies.
*
*
*
* This is a variant of
* {@link #describeDBProxies(software.amazon.awssdk.services.rds.model.DescribeDbProxiesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxiesPublisher publisher = client.describeDBProxiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxiesPublisher publisher = client.describeDBProxiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxies(software.amazon.awssdk.services.rds.model.DescribeDbProxiesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxiesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDbProxiesRequest#builder()}
*
*
* @param describeDbProxiesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxiesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxies
* @see AWS API
* Documentation
*/
default DescribeDBProxiesPublisher describeDBProxiesPaginator(
Consumer describeDbProxiesRequest) {
return describeDBProxiesPaginator(DescribeDbProxiesRequest.builder().applyMutation(describeDbProxiesRequest).build());
}
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
* @param describeDbProxyTargetGroupsRequest
* @return A Java Future containing the result of the DescribeDBProxyTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeDBProxyTargetGroups(
DescribeDbProxyTargetGroupsRequest describeDbProxyTargetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxyTargetGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbProxyTargetGroupsRequest#builder()}
*
*
* @param describeDbProxyTargetGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxyTargetGroupsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeDBProxyTargetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
default CompletableFuture describeDBProxyTargetGroups(
Consumer describeDbProxyTargetGroupsRequest) {
return describeDBProxyTargetGroups(DescribeDbProxyTargetGroupsRequest.builder()
.applyMutation(describeDbProxyTargetGroupsRequest).build());
}
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
*
* This is a variant of
* {@link #describeDBProxyTargetGroups(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetGroupsPublisher publisher = client.describeDBProxyTargetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetGroupsPublisher publisher = client.describeDBProxyTargetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxyTargetGroups(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsRequest)}
* operation.
*
*
* @param describeDbProxyTargetGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
default DescribeDBProxyTargetGroupsPublisher describeDBProxyTargetGroupsPaginator(
DescribeDbProxyTargetGroupsRequest describeDbProxyTargetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
*
* This is a variant of
* {@link #describeDBProxyTargetGroups(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetGroupsPublisher publisher = client.describeDBProxyTargetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetGroupsPublisher publisher = client.describeDBProxyTargetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxyTargetGroups(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxyTargetGroupsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbProxyTargetGroupsRequest#builder()}
*
*
* @param describeDbProxyTargetGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxyTargetGroupsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
default DescribeDBProxyTargetGroupsPublisher describeDBProxyTargetGroupsPaginator(
Consumer describeDbProxyTargetGroupsRequest) {
return describeDBProxyTargetGroupsPaginator(DescribeDbProxyTargetGroupsRequest.builder()
.applyMutation(describeDbProxyTargetGroupsRequest).build());
}
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
* @param describeDbProxyTargetsRequest
* @return A Java Future containing the result of the DescribeDBProxyTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBProxyTargets(
DescribeDbProxyTargetsRequest describeDbProxyTargetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxyTargetsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbProxyTargetsRequest#builder()}
*
*
* @param describeDbProxyTargetsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxyTargetsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBProxyTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBProxyTargets(
Consumer describeDbProxyTargetsRequest) {
return describeDBProxyTargets(DescribeDbProxyTargetsRequest.builder().applyMutation(describeDbProxyTargetsRequest)
.build());
}
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
*
* This is a variant of
* {@link #describeDBProxyTargets(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetsPublisher publisher = client.describeDBProxyTargetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetsPublisher publisher = client.describeDBProxyTargetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxyTargets(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsRequest)}
* operation.
*
*
* @param describeDbProxyTargetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
default DescribeDBProxyTargetsPublisher describeDBProxyTargetsPaginator(
DescribeDbProxyTargetsRequest describeDbProxyTargetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
*
* This is a variant of
* {@link #describeDBProxyTargets(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetsPublisher publisher = client.describeDBProxyTargetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBProxyTargetsPublisher publisher = client.describeDBProxyTargetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBProxyTargets(software.amazon.awssdk.services.rds.model.DescribeDbProxyTargetsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbProxyTargetsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbProxyTargetsRequest#builder()}
*
*
* @param describeDbProxyTargetsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBProxyTargetsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbProxyNotFoundException The specified proxy name doesn't correspond to a proxy owned by your AWS
* accoutn in the specified AWS Region.
* - DbProxyTargetNotFoundException The specified RDS DB instance or Aurora DB cluster isn't available for
* a proxy owned by your AWS account in the specified AWS Region.
* - DbProxyTargetGroupNotFoundException The specified target group isn't available for a proxy owned by
* your AWS account in the specified AWS Region.
* - InvalidDbProxyStateException The requested operation can't be performed while the proxy is in this
* state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
default DescribeDBProxyTargetsPublisher describeDBProxyTargetsPaginator(
Consumer describeDbProxyTargetsRequest) {
return describeDBProxyTargetsPaginator(DescribeDbProxyTargetsRequest.builder()
.applyMutation(describeDbProxyTargetsRequest).build());
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
* @param describeDbSecurityGroupsRequest
* @return A Java Future containing the result of the DescribeDBSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBSecurityGroups(
DescribeDbSecurityGroupsRequest describeDbSecurityGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSecurityGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbSecurityGroupsRequest#builder()}
*
*
* @param describeDbSecurityGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSecurityGroupsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBSecurityGroups(
Consumer describeDbSecurityGroupsRequest) {
return describeDBSecurityGroups(DescribeDbSecurityGroupsRequest.builder().applyMutation(describeDbSecurityGroupsRequest)
.build());
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
* @return A Java Future containing the result of the DescribeDBSecurityGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default CompletableFuture describeDBSecurityGroups() {
return describeDBSecurityGroups(DescribeDbSecurityGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
*
* This is a variant of
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default DescribeDBSecurityGroupsPublisher describeDBSecurityGroupsPaginator() {
return describeDBSecurityGroupsPaginator(DescribeDbSecurityGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
*
* This is a variant of
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation.
*
*
* @param describeDbSecurityGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default DescribeDBSecurityGroupsPublisher describeDBSecurityGroupsPaginator(
DescribeDbSecurityGroupsRequest describeDbSecurityGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
*
* This is a variant of
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSecurityGroupsPublisher publisher = client.describeDBSecurityGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSecurityGroups(software.amazon.awssdk.services.rds.model.DescribeDbSecurityGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSecurityGroupsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDbSecurityGroupsRequest#builder()}
*
*
* @param describeDbSecurityGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSecurityGroupsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSecurityGroupNotFoundException
DBSecurityGroupName
doesn't refer to an existing DB
* security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
default DescribeDBSecurityGroupsPublisher describeDBSecurityGroupsPaginator(
Consumer describeDbSecurityGroupsRequest) {
return describeDBSecurityGroupsPaginator(DescribeDbSecurityGroupsRequest.builder()
.applyMutation(describeDbSecurityGroupsRequest).build());
}
/**
*
* Returns a list of DB snapshot attribute names and values for a manual DB snapshot.
*
*
* When sharing snapshots with other AWS accounts, DescribeDBSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the AWS accounts that are authorized to copy or restore the
* manual DB snapshot. If all
is included in the list of values for the restore
attribute,
* then the manual DB snapshot is public and can be copied or restored by all AWS accounts.
*
*
* To add or remove access for an AWS account to copy or restore a manual DB snapshot, or to make the manual DB
* snapshot public or private, use the ModifyDBSnapshotAttribute
API action.
*
*
* @param describeDbSnapshotAttributesRequest
* @return A Java Future containing the result of the DescribeDBSnapshotAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshotAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeDBSnapshotAttributes(
DescribeDbSnapshotAttributesRequest describeDbSnapshotAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DB snapshot attribute names and values for a manual DB snapshot.
*
*
* When sharing snapshots with other AWS accounts, DescribeDBSnapshotAttributes
returns the
* restore
attribute and a list of IDs for the AWS accounts that are authorized to copy or restore the
* manual DB snapshot. If all
is included in the list of values for the restore
attribute,
* then the manual DB snapshot is public and can be copied or restored by all AWS accounts.
*
*
* To add or remove access for an AWS account to copy or restore a manual DB snapshot, or to make the manual DB
* snapshot public or private, use the ModifyDBSnapshotAttribute
API action.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSnapshotAttributesRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDbSnapshotAttributesRequest#builder()}
*
*
* @param describeDbSnapshotAttributesRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSnapshotAttributesMessage.Builder} to create
* a request.
* @return A Java Future containing the result of the DescribeDBSnapshotAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshotAttributes
* @see AWS API Documentation
*/
default CompletableFuture describeDBSnapshotAttributes(
Consumer describeDbSnapshotAttributesRequest) {
return describeDBSnapshotAttributes(DescribeDbSnapshotAttributesRequest.builder()
.applyMutation(describeDbSnapshotAttributesRequest).build());
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
* @param describeDbSnapshotsRequest
* @return A Java Future containing the result of the DescribeDBSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSnapshots(
DescribeDbSnapshotsRequest describeDbSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSnapshotsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbSnapshotsRequest#builder()}
*
*
* @param describeDbSnapshotsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSnapshotsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSnapshots(
Consumer describeDbSnapshotsRequest) {
return describeDBSnapshots(DescribeDbSnapshotsRequest.builder().applyMutation(describeDbSnapshotsRequest).build());
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
* @return A Java Future containing the result of the DescribeDBSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSnapshots() {
return describeDBSnapshots(DescribeDbSnapshotsRequest.builder().build());
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
*
* This is a variant of
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default DescribeDBSnapshotsPublisher describeDBSnapshotsPaginator() {
return describeDBSnapshotsPaginator(DescribeDbSnapshotsRequest.builder().build());
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
*
* This is a variant of
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation.
*
*
* @param describeDbSnapshotsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default DescribeDBSnapshotsPublisher describeDBSnapshotsPaginator(DescribeDbSnapshotsRequest describeDbSnapshotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
*
* This is a variant of
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSnapshotsPublisher publisher = client.describeDBSnapshotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSnapshots(software.amazon.awssdk.services.rds.model.DescribeDbSnapshotsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSnapshotsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbSnapshotsRequest#builder()}
*
*
* @param describeDbSnapshotsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSnapshotsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSnapshotNotFoundException
DBSnapshotIdentifier
doesn't refer to an existing DB
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
default DescribeDBSnapshotsPublisher describeDBSnapshotsPaginator(
Consumer describeDbSnapshotsRequest) {
return describeDBSnapshotsPaginator(DescribeDbSnapshotsRequest.builder().applyMutation(describeDbSnapshotsRequest)
.build());
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param describeDbSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeDBSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSubnetGroups(
DescribeDbSubnetGroupsRequest describeDbSubnetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSubnetGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbSubnetGroupsRequest#builder()}
*
*
* @param describeDbSubnetGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSubnetGroupsMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeDBSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSubnetGroups(
Consumer describeDbSubnetGroupsRequest) {
return describeDBSubnetGroups(DescribeDbSubnetGroupsRequest.builder().applyMutation(describeDbSubnetGroupsRequest)
.build());
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @return A Java Future containing the result of the DescribeDBSubnetGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default CompletableFuture describeDBSubnetGroups() {
return describeDBSubnetGroups(DescribeDbSubnetGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
*
* This is a variant of
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default DescribeDBSubnetGroupsPublisher describeDBSubnetGroupsPaginator() {
return describeDBSubnetGroupsPaginator(DescribeDbSubnetGroupsRequest.builder().build());
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
*
* This is a variant of
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation.
*
*
* @param describeDbSubnetGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default DescribeDBSubnetGroupsPublisher describeDBSubnetGroupsPaginator(
DescribeDbSubnetGroupsRequest describeDbSubnetGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
*
* This is a variant of
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeDBSubnetGroupsPublisher publisher = client.describeDBSubnetGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDBSubnetGroups(software.amazon.awssdk.services.rds.model.DescribeDbSubnetGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDbSubnetGroupsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDbSubnetGroupsRequest#builder()}
*
*
* @param describeDbSubnetGroupsRequest
* A {@link Consumer} that will call methods on {@link DescribeDBSubnetGroupsMessage.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DbSubnetGroupNotFoundException
DBSubnetGroupName
doesn't refer to an existing DB subnet
* group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeDBSubnetGroups
* @see AWS API
* Documentation
*/
default DescribeDBSubnetGroupsPublisher describeDBSubnetGroupsPaginator(
Consumer describeDbSubnetGroupsRequest) {
return describeDBSubnetGroupsPaginator(DescribeDbSubnetGroupsRequest.builder()
.applyMutation(describeDbSubnetGroupsRequest).build());
}
/**
*
* Returns the default engine and system parameter information for the cluster database engine.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
* @param describeEngineDefaultClusterParametersRequest
* @return A Java Future containing the result of the DescribeEngineDefaultClusterParameters operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultClusterParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultClusterParameters(
DescribeEngineDefaultClusterParametersRequest describeEngineDefaultClusterParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the default engine and system parameter information for the cluster database engine.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This is a convenience which creates an instance of the
* {@link DescribeEngineDefaultClusterParametersRequest.Builder} avoiding the need to create one manually via
* {@link DescribeEngineDefaultClusterParametersRequest#builder()}
*
*
* @param describeEngineDefaultClusterParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeEngineDefaultClusterParametersMessage.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeEngineDefaultClusterParameters operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultClusterParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultClusterParameters(
Consumer describeEngineDefaultClusterParametersRequest) {
return describeEngineDefaultClusterParameters(DescribeEngineDefaultClusterParametersRequest.builder()
.applyMutation(describeEngineDefaultClusterParametersRequest).build());
}
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
* @param describeEngineDefaultParametersRequest
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultParameters(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEngineDefaultParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEngineDefaultParametersRequest#builder()}
*
*
* @param describeEngineDefaultParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeEngineDefaultParametersMessage.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeEngineDefaultParameters operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default CompletableFuture describeEngineDefaultParameters(
Consumer describeEngineDefaultParametersRequest) {
return describeEngineDefaultParameters(DescribeEngineDefaultParametersRequest.builder()
.applyMutation(describeEngineDefaultParametersRequest).build());
}
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
*
* This is a variant of
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersRequest)}
* operation.
*
*
* @param describeEngineDefaultParametersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default DescribeEngineDefaultParametersPublisher describeEngineDefaultParametersPaginator(
DescribeEngineDefaultParametersRequest describeEngineDefaultParametersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the default engine and system parameter information for the specified database engine.
*
*
*
* This is a variant of
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.rds.paginators.DescribeEngineDefaultParametersPublisher publisher = client.describeEngineDefaultParametersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxRecords won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEngineDefaultParameters(software.amazon.awssdk.services.rds.model.DescribeEngineDefaultParametersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeEngineDefaultParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEngineDefaultParametersRequest#builder()}
*
*
* @param describeEngineDefaultParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeEngineDefaultParametersMessage.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEngineDefaultParameters
* @see AWS API Documentation
*/
default DescribeEngineDefaultParametersPublisher describeEngineDefaultParametersPaginator(
Consumer describeEngineDefaultParametersRequest) {
return describeEngineDefaultParametersPaginator(DescribeEngineDefaultParametersRequest.builder()
.applyMutation(describeEngineDefaultParametersRequest).build());
}
/**
*
* Displays a list of categories for all event source types, or, if specified, for a specified source type. You can
* see a list of the event categories and source types in Events in the Amazon RDS
* User Guide.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEventCategories
* @see AWS
* API Documentation
*/
default CompletableFuture describeEventCategories(
DescribeEventCategoriesRequest describeEventCategoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Displays a list of categories for all event source types, or, if specified, for a specified source type. You can
* see a list of the event categories and source types in Events in the Amazon RDS
* User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEventCategoriesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeEventCategoriesRequest#builder()}
*
*
* @param describeEventCategoriesRequest
* A {@link Consumer} that will call methods on {@link DescribeEventCategoriesMessage.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEventCategories
* @see AWS
* API Documentation
*/
default CompletableFuture describeEventCategories(
Consumer describeEventCategoriesRequest) {
return describeEventCategories(DescribeEventCategoriesRequest.builder().applyMutation(describeEventCategoriesRequest)
.build());
}
/**
*
* Displays a list of categories for all event source types, or, if specified, for a specified source type. You can
* see a list of the event categories and source types in Events in the Amazon RDS
* User Guide.
*
*
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEventCategories
* @see AWS
* API Documentation
*/
default CompletableFuture describeEventCategories() {
return describeEventCategories(DescribeEventCategoriesRequest.builder().build());
}
/**
*
* Lists all the subscription descriptions for a customer account. The description for a subscription includes
* SubscriptionName
, SNSTopicARN
, CustomerID
, SourceType
,
* SourceID
, CreationTime
, and Status
.
*
*
* If you specify a SubscriptionName
, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SubscriptionNotFoundException The subscription name does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RdsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample RdsAsyncClient.DescribeEventSubscriptions
* @see AWS
* API Documentation
*/
default CompletableFuture describeEventSubscriptions(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the subscription descriptions for a customer account. The description for a subscription includes
*