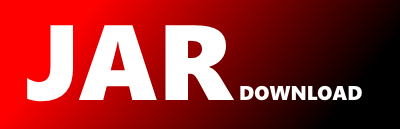
software.amazon.awssdk.services.rds.model.BlueGreenDeployment Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about a blue/green deployment.
*
*
* For more information, see Using Amazon RDS Blue/Green
* Deployments for database updates in the Amazon RDS User Guide and Using Amazon RDS
* Blue/Green Deployments for database updates in the Amazon Aurora User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BlueGreenDeployment implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BLUE_GREEN_DEPLOYMENT_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("BlueGreenDeploymentIdentifier")
.getter(getter(BlueGreenDeployment::blueGreenDeploymentIdentifier))
.setter(setter(Builder::blueGreenDeploymentIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlueGreenDeploymentIdentifier")
.build()).build();
private static final SdkField BLUE_GREEN_DEPLOYMENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BlueGreenDeploymentName").getter(getter(BlueGreenDeployment::blueGreenDeploymentName))
.setter(setter(Builder::blueGreenDeploymentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlueGreenDeploymentName").build())
.build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Source")
.getter(getter(BlueGreenDeployment::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField TARGET_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Target")
.getter(getter(BlueGreenDeployment::target)).setter(setter(Builder::target))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Target").build()).build();
private static final SdkField> SWITCHOVER_DETAILS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SwitchoverDetails")
.getter(getter(BlueGreenDeployment::switchoverDetails))
.setter(setter(Builder::switchoverDetails))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SwitchoverDetails").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SwitchoverDetail::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TASKS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tasks")
.getter(getter(BlueGreenDeployment::tasks))
.setter(setter(Builder::tasks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tasks").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BlueGreenDeploymentTask::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(BlueGreenDeployment::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField STATUS_DETAILS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StatusDetails").getter(getter(BlueGreenDeployment::statusDetails))
.setter(setter(Builder::statusDetails))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatusDetails").build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTime").getter(getter(BlueGreenDeployment::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTime").build()).build();
private static final SdkField DELETE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DeleteTime").getter(getter(BlueGreenDeployment::deleteTime)).setter(setter(Builder::deleteTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeleteTime").build()).build();
private static final SdkField> TAG_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagList")
.getter(getter(BlueGreenDeployment::tagList))
.setter(setter(Builder::tagList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagList").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
BLUE_GREEN_DEPLOYMENT_IDENTIFIER_FIELD, BLUE_GREEN_DEPLOYMENT_NAME_FIELD, SOURCE_FIELD, TARGET_FIELD,
SWITCHOVER_DETAILS_FIELD, TASKS_FIELD, STATUS_FIELD, STATUS_DETAILS_FIELD, CREATE_TIME_FIELD, DELETE_TIME_FIELD,
TAG_LIST_FIELD));
private static final long serialVersionUID = 1L;
private final String blueGreenDeploymentIdentifier;
private final String blueGreenDeploymentName;
private final String source;
private final String target;
private final List switchoverDetails;
private final List tasks;
private final String status;
private final String statusDetails;
private final Instant createTime;
private final Instant deleteTime;
private final List tagList;
private BlueGreenDeployment(BuilderImpl builder) {
this.blueGreenDeploymentIdentifier = builder.blueGreenDeploymentIdentifier;
this.blueGreenDeploymentName = builder.blueGreenDeploymentName;
this.source = builder.source;
this.target = builder.target;
this.switchoverDetails = builder.switchoverDetails;
this.tasks = builder.tasks;
this.status = builder.status;
this.statusDetails = builder.statusDetails;
this.createTime = builder.createTime;
this.deleteTime = builder.deleteTime;
this.tagList = builder.tagList;
}
/**
*
* The unique identifier of the blue/green deployment.
*
*
* @return The unique identifier of the blue/green deployment.
*/
public final String blueGreenDeploymentIdentifier() {
return blueGreenDeploymentIdentifier;
}
/**
*
* The user-supplied name of the blue/green deployment.
*
*
* @return The user-supplied name of the blue/green deployment.
*/
public final String blueGreenDeploymentName() {
return blueGreenDeploymentName;
}
/**
*
* The source database for the blue/green deployment.
*
*
* Before switchover, the source database is the production database in the blue environment.
*
*
* @return The source database for the blue/green deployment.
*
* Before switchover, the source database is the production database in the blue environment.
*/
public final String source() {
return source;
}
/**
*
* The target database for the blue/green deployment.
*
*
* Before switchover, the target database is the clone database in the green environment.
*
*
* @return The target database for the blue/green deployment.
*
* Before switchover, the target database is the clone database in the green environment.
*/
public final String target() {
return target;
}
/**
* For responses, this returns true if the service returned a value for the SwitchoverDetails property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSwitchoverDetails() {
return switchoverDetails != null && !(switchoverDetails instanceof SdkAutoConstructList);
}
/**
*
* The details about each source and target resource in the blue/green deployment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSwitchoverDetails} method.
*
*
* @return The details about each source and target resource in the blue/green deployment.
*/
public final List switchoverDetails() {
return switchoverDetails;
}
/**
* For responses, this returns true if the service returned a value for the Tasks property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTasks() {
return tasks != null && !(tasks instanceof SdkAutoConstructList);
}
/**
*
* Either tasks to be performed or tasks that have been completed on the target database before switchover.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTasks} method.
*
*
* @return Either tasks to be performed or tasks that have been completed on the target database before switchover.
*/
public final List tasks() {
return tasks;
}
/**
*
* The status of the blue/green deployment.
*
*
* Valid Values:
*
*
* -
*
* PROVISIONING
- Resources are being created in the green environment.
*
*
* -
*
* AVAILABLE
- Resources are available in the green environment.
*
*
* -
*
* SWITCHOVER_IN_PROGRESS
- The deployment is being switched from the blue environment to the green
* environment.
*
*
* -
*
* SWITCHOVER_COMPLETED
- Switchover from the blue environment to the green environment is complete.
*
*
* -
*
* INVALID_CONFIGURATION
- Resources in the green environment are invalid, so switchover isn't
* possible.
*
*
* -
*
* SWITCHOVER_FAILED
- Switchover was attempted but failed.
*
*
* -
*
* DELETING
- The blue/green deployment is being deleted.
*
*
*
*
* @return The status of the blue/green deployment.
*
* Valid Values:
*
*
* -
*
* PROVISIONING
- Resources are being created in the green environment.
*
*
* -
*
* AVAILABLE
- Resources are available in the green environment.
*
*
* -
*
* SWITCHOVER_IN_PROGRESS
- The deployment is being switched from the blue environment to the
* green environment.
*
*
* -
*
* SWITCHOVER_COMPLETED
- Switchover from the blue environment to the green environment is
* complete.
*
*
* -
*
* INVALID_CONFIGURATION
- Resources in the green environment are invalid, so switchover isn't
* possible.
*
*
* -
*
* SWITCHOVER_FAILED
- Switchover was attempted but failed.
*
*
* -
*
* DELETING
- The blue/green deployment is being deleted.
*
*
*/
public final String status() {
return status;
}
/**
*
* Additional information about the status of the blue/green deployment.
*
*
* @return Additional information about the status of the blue/green deployment.
*/
public final String statusDetails() {
return statusDetails;
}
/**
*
* The time when the blue/green deployment was created, in Universal Coordinated Time (UTC).
*
*
* @return The time when the blue/green deployment was created, in Universal Coordinated Time (UTC).
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The time when the blue/green deployment was deleted, in Universal Coordinated Time (UTC).
*
*
* @return The time when the blue/green deployment was deleted, in Universal Coordinated Time (UTC).
*/
public final Instant deleteTime() {
return deleteTime;
}
/**
* For responses, this returns true if the service returned a value for the TagList property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTagList() {
return tagList != null && !(tagList instanceof SdkAutoConstructList);
}
/**
* Returns the value of the TagList property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagList} method.
*
*
* @return The value of the TagList property for this object.
*/
public final List tagList() {
return tagList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(blueGreenDeploymentIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(blueGreenDeploymentName());
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(target());
hashCode = 31 * hashCode + Objects.hashCode(hasSwitchoverDetails() ? switchoverDetails() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTasks() ? tasks() : null);
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(statusDetails());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(deleteTime());
hashCode = 31 * hashCode + Objects.hashCode(hasTagList() ? tagList() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BlueGreenDeployment)) {
return false;
}
BlueGreenDeployment other = (BlueGreenDeployment) obj;
return Objects.equals(blueGreenDeploymentIdentifier(), other.blueGreenDeploymentIdentifier())
&& Objects.equals(blueGreenDeploymentName(), other.blueGreenDeploymentName())
&& Objects.equals(source(), other.source()) && Objects.equals(target(), other.target())
&& hasSwitchoverDetails() == other.hasSwitchoverDetails()
&& Objects.equals(switchoverDetails(), other.switchoverDetails()) && hasTasks() == other.hasTasks()
&& Objects.equals(tasks(), other.tasks()) && Objects.equals(status(), other.status())
&& Objects.equals(statusDetails(), other.statusDetails()) && Objects.equals(createTime(), other.createTime())
&& Objects.equals(deleteTime(), other.deleteTime()) && hasTagList() == other.hasTagList()
&& Objects.equals(tagList(), other.tagList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BlueGreenDeployment").add("BlueGreenDeploymentIdentifier", blueGreenDeploymentIdentifier())
.add("BlueGreenDeploymentName", blueGreenDeploymentName()).add("Source", source()).add("Target", target())
.add("SwitchoverDetails", hasSwitchoverDetails() ? switchoverDetails() : null)
.add("Tasks", hasTasks() ? tasks() : null).add("Status", status()).add("StatusDetails", statusDetails())
.add("CreateTime", createTime()).add("DeleteTime", deleteTime()).add("TagList", hasTagList() ? tagList() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BlueGreenDeploymentIdentifier":
return Optional.ofNullable(clazz.cast(blueGreenDeploymentIdentifier()));
case "BlueGreenDeploymentName":
return Optional.ofNullable(clazz.cast(blueGreenDeploymentName()));
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "Target":
return Optional.ofNullable(clazz.cast(target()));
case "SwitchoverDetails":
return Optional.ofNullable(clazz.cast(switchoverDetails()));
case "Tasks":
return Optional.ofNullable(clazz.cast(tasks()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "StatusDetails":
return Optional.ofNullable(clazz.cast(statusDetails()));
case "CreateTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "DeleteTime":
return Optional.ofNullable(clazz.cast(deleteTime()));
case "TagList":
return Optional.ofNullable(clazz.cast(tagList()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function