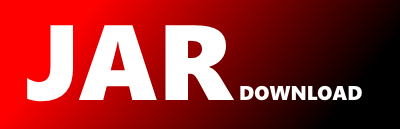
software.amazon.awssdk.services.rds.model.DBInstanceAutomatedBackup Maven / Gradle / Ivy
Show all versions of rds Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An automated backup of a DB instance. It consists of system backups, transaction logs, and the database instance
* properties that existed at the time you deleted the source instance.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DBInstanceAutomatedBackup implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DB_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceArn").getter(getter(DBInstanceAutomatedBackup::dbInstanceArn))
.setter(setter(Builder::dbInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceArn").build()).build();
private static final SdkField DBI_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DbiResourceId").getter(getter(DBInstanceAutomatedBackup::dbiResourceId))
.setter(setter(Builder::dbiResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbiResourceId").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Region")
.getter(getter(DBInstanceAutomatedBackup::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region").build()).build();
private static final SdkField DB_INSTANCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceIdentifier").getter(getter(DBInstanceAutomatedBackup::dbInstanceIdentifier))
.setter(setter(Builder::dbInstanceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceIdentifier").build())
.build();
private static final SdkField RESTORE_WINDOW_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RestoreWindow")
.getter(getter(DBInstanceAutomatedBackup::restoreWindow)).setter(setter(Builder::restoreWindow))
.constructor(RestoreWindow::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RestoreWindow").build()).build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(DBInstanceAutomatedBackup::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DBInstanceAutomatedBackup::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(DBInstanceAutomatedBackup::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(DBInstanceAutomatedBackup::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(DBInstanceAutomatedBackup::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField INSTANCE_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("InstanceCreateTime").getter(getter(DBInstanceAutomatedBackup::instanceCreateTime))
.setter(setter(Builder::instanceCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCreateTime").build())
.build();
private static final SdkField MASTER_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUsername").getter(getter(DBInstanceAutomatedBackup::masterUsername))
.setter(setter(Builder::masterUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUsername").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(DBInstanceAutomatedBackup::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(DBInstanceAutomatedBackup::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField LICENSE_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LicenseModel").getter(getter(DBInstanceAutomatedBackup::licenseModel))
.setter(setter(Builder::licenseModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LicenseModel").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(DBInstanceAutomatedBackup::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final SdkField OPTION_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OptionGroupName").getter(getter(DBInstanceAutomatedBackup::optionGroupName))
.setter(setter(Builder::optionGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionGroupName").build()).build();
private static final SdkField TDE_CREDENTIAL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TdeCredentialArn").getter(getter(DBInstanceAutomatedBackup::tdeCredentialArn))
.setter(setter(Builder::tdeCredentialArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TdeCredentialArn").build()).build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted").getter(getter(DBInstanceAutomatedBackup::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted").build()).build();
private static final SdkField STORAGE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StorageType").getter(getter(DBInstanceAutomatedBackup::storageType))
.setter(setter(Builder::storageType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageType").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(DBInstanceAutomatedBackup::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField TIMEZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Timezone").getter(getter(DBInstanceAutomatedBackup::timezone)).setter(setter(Builder::timezone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Timezone").build()).build();
private static final SdkField IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IAMDatabaseAuthenticationEnabled")
.getter(getter(DBInstanceAutomatedBackup::iamDatabaseAuthenticationEnabled))
.setter(setter(Builder::iamDatabaseAuthenticationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IAMDatabaseAuthenticationEnabled")
.build()).build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(DBInstanceAutomatedBackup::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField DB_INSTANCE_AUTOMATED_BACKUPS_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DBInstanceAutomatedBackupsArn")
.getter(getter(DBInstanceAutomatedBackup::dbInstanceAutomatedBackupsArn))
.setter(setter(Builder::dbInstanceAutomatedBackupsArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceAutomatedBackupsArn")
.build()).build();
private static final SdkField> DB_INSTANCE_AUTOMATED_BACKUPS_REPLICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DBInstanceAutomatedBackupsReplications")
.getter(getter(DBInstanceAutomatedBackup::dbInstanceAutomatedBackupsReplications))
.setter(setter(Builder::dbInstanceAutomatedBackupsReplications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBInstanceAutomatedBackupsReplications").build(),
ListTrait
.builder()
.memberLocationName("DBInstanceAutomatedBackupsReplication")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DBInstanceAutomatedBackupsReplication::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBInstanceAutomatedBackupsReplication").build()).build())
.build()).build();
private static final SdkField BACKUP_TARGET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BackupTarget").getter(getter(DBInstanceAutomatedBackup::backupTarget))
.setter(setter(Builder::backupTarget))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupTarget").build()).build();
private static final SdkField STORAGE_THROUGHPUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StorageThroughput").getter(getter(DBInstanceAutomatedBackup::storageThroughput))
.setter(setter(Builder::storageThroughput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageThroughput").build()).build();
private static final SdkField AWS_BACKUP_RECOVERY_POINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AwsBackupRecoveryPointArn").getter(getter(DBInstanceAutomatedBackup::awsBackupRecoveryPointArn))
.setter(setter(Builder::awsBackupRecoveryPointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AwsBackupRecoveryPointArn").build())
.build();
private static final SdkField DEDICATED_LOG_VOLUME_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DedicatedLogVolume").getter(getter(DBInstanceAutomatedBackup::dedicatedLogVolume))
.setter(setter(Builder::dedicatedLogVolume))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DedicatedLogVolume").build())
.build();
private static final SdkField MULTI_TENANT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiTenant").getter(getter(DBInstanceAutomatedBackup::multiTenant))
.setter(setter(Builder::multiTenant))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiTenant").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_INSTANCE_ARN_FIELD,
DBI_RESOURCE_ID_FIELD, REGION_FIELD, DB_INSTANCE_IDENTIFIER_FIELD, RESTORE_WINDOW_FIELD, ALLOCATED_STORAGE_FIELD,
STATUS_FIELD, PORT_FIELD, AVAILABILITY_ZONE_FIELD, VPC_ID_FIELD, INSTANCE_CREATE_TIME_FIELD, MASTER_USERNAME_FIELD,
ENGINE_FIELD, ENGINE_VERSION_FIELD, LICENSE_MODEL_FIELD, IOPS_FIELD, OPTION_GROUP_NAME_FIELD,
TDE_CREDENTIAL_ARN_FIELD, ENCRYPTED_FIELD, STORAGE_TYPE_FIELD, KMS_KEY_ID_FIELD, TIMEZONE_FIELD,
IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD, BACKUP_RETENTION_PERIOD_FIELD, DB_INSTANCE_AUTOMATED_BACKUPS_ARN_FIELD,
DB_INSTANCE_AUTOMATED_BACKUPS_REPLICATIONS_FIELD, BACKUP_TARGET_FIELD, STORAGE_THROUGHPUT_FIELD,
AWS_BACKUP_RECOVERY_POINT_ARN_FIELD, DEDICATED_LOG_VOLUME_FIELD, MULTI_TENANT_FIELD));
private static final long serialVersionUID = 1L;
private final String dbInstanceArn;
private final String dbiResourceId;
private final String region;
private final String dbInstanceIdentifier;
private final RestoreWindow restoreWindow;
private final Integer allocatedStorage;
private final String status;
private final Integer port;
private final String availabilityZone;
private final String vpcId;
private final Instant instanceCreateTime;
private final String masterUsername;
private final String engine;
private final String engineVersion;
private final String licenseModel;
private final Integer iops;
private final String optionGroupName;
private final String tdeCredentialArn;
private final Boolean encrypted;
private final String storageType;
private final String kmsKeyId;
private final String timezone;
private final Boolean iamDatabaseAuthenticationEnabled;
private final Integer backupRetentionPeriod;
private final String dbInstanceAutomatedBackupsArn;
private final List dbInstanceAutomatedBackupsReplications;
private final String backupTarget;
private final Integer storageThroughput;
private final String awsBackupRecoveryPointArn;
private final Boolean dedicatedLogVolume;
private final Boolean multiTenant;
private DBInstanceAutomatedBackup(BuilderImpl builder) {
this.dbInstanceArn = builder.dbInstanceArn;
this.dbiResourceId = builder.dbiResourceId;
this.region = builder.region;
this.dbInstanceIdentifier = builder.dbInstanceIdentifier;
this.restoreWindow = builder.restoreWindow;
this.allocatedStorage = builder.allocatedStorage;
this.status = builder.status;
this.port = builder.port;
this.availabilityZone = builder.availabilityZone;
this.vpcId = builder.vpcId;
this.instanceCreateTime = builder.instanceCreateTime;
this.masterUsername = builder.masterUsername;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.licenseModel = builder.licenseModel;
this.iops = builder.iops;
this.optionGroupName = builder.optionGroupName;
this.tdeCredentialArn = builder.tdeCredentialArn;
this.encrypted = builder.encrypted;
this.storageType = builder.storageType;
this.kmsKeyId = builder.kmsKeyId;
this.timezone = builder.timezone;
this.iamDatabaseAuthenticationEnabled = builder.iamDatabaseAuthenticationEnabled;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.dbInstanceAutomatedBackupsArn = builder.dbInstanceAutomatedBackupsArn;
this.dbInstanceAutomatedBackupsReplications = builder.dbInstanceAutomatedBackupsReplications;
this.backupTarget = builder.backupTarget;
this.storageThroughput = builder.storageThroughput;
this.awsBackupRecoveryPointArn = builder.awsBackupRecoveryPointArn;
this.dedicatedLogVolume = builder.dedicatedLogVolume;
this.multiTenant = builder.multiTenant;
}
/**
*
* The Amazon Resource Name (ARN) for the automated backups.
*
*
* @return The Amazon Resource Name (ARN) for the automated backups.
*/
public final String dbInstanceArn() {
return dbInstanceArn;
}
/**
*
* The resource ID for the source DB instance, which can't be changed and which is unique to an Amazon Web Services
* Region.
*
*
* @return The resource ID for the source DB instance, which can't be changed and which is unique to an Amazon Web
* Services Region.
*/
public final String dbiResourceId() {
return dbiResourceId;
}
/**
*
* The Amazon Web Services Region associated with the automated backup.
*
*
* @return The Amazon Web Services Region associated with the automated backup.
*/
public final String region() {
return region;
}
/**
*
* The identifier for the source DB instance, which can't be changed and which is unique to an Amazon Web Services
* Region.
*
*
* @return The identifier for the source DB instance, which can't be changed and which is unique to an Amazon Web
* Services Region.
*/
public final String dbInstanceIdentifier() {
return dbInstanceIdentifier;
}
/**
*
* The earliest and latest time a DB instance can be restored to.
*
*
* @return The earliest and latest time a DB instance can be restored to.
*/
public final RestoreWindow restoreWindow() {
return restoreWindow;
}
/**
*
* The allocated storage size for the the automated backup in gibibytes (GiB).
*
*
* @return The allocated storage size for the the automated backup in gibibytes (GiB).
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* A list of status information for an automated backup:
*
*
* -
*
* active
- Automated backups for current instances.
*
*
* -
*
* retained
- Automated backups for deleted instances.
*
*
* -
*
* creating
- Automated backups that are waiting for the first automated snapshot to be available.
*
*
*
*
* @return A list of status information for an automated backup:
*
* -
*
* active
- Automated backups for current instances.
*
*
* -
*
* retained
- Automated backups for deleted instances.
*
*
* -
*
* creating
- Automated backups that are waiting for the first automated snapshot to be
* available.
*
*
*/
public final String status() {
return status;
}
/**
*
* The port number that the automated backup used for connections.
*
*
* Default: Inherits from the source DB instance
*
*
* Valid Values: 1150-65535
*
*
* @return The port number that the automated backup used for connections.
*
* Default: Inherits from the source DB instance
*
*
* Valid Values: 1150-65535
*/
public final Integer port() {
return port;
}
/**
*
* The Availability Zone that the automated backup was created in. For information on Amazon Web Services Regions
* and Availability Zones, see Regions
* and Availability Zones.
*
*
* @return The Availability Zone that the automated backup was created in. For information on Amazon Web Services
* Regions and Availability Zones, see Regions and Availability Zones.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The VPC ID associated with the DB instance.
*
*
* @return The VPC ID associated with the DB instance.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* The date and time when the DB instance was created.
*
*
* @return The date and time when the DB instance was created.
*/
public final Instant instanceCreateTime() {
return instanceCreateTime;
}
/**
*
* The master user name of an automated backup.
*
*
* @return The master user name of an automated backup.
*/
public final String masterUsername() {
return masterUsername;
}
/**
*
* The name of the database engine for this automated backup.
*
*
* @return The name of the database engine for this automated backup.
*/
public final String engine() {
return engine;
}
/**
*
* The version of the database engine for the automated backup.
*
*
* @return The version of the database engine for the automated backup.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The license model information for the automated backup.
*
*
* @return The license model information for the automated backup.
*/
public final String licenseModel() {
return licenseModel;
}
/**
*
* The IOPS (I/O operations per second) value for the automated backup.
*
*
* @return The IOPS (I/O operations per second) value for the automated backup.
*/
public final Integer iops() {
return iops;
}
/**
*
* The option group the automated backup is associated with. If omitted, the default option group for the engine
* specified is used.
*
*
* @return The option group the automated backup is associated with. If omitted, the default option group for the
* engine specified is used.
*/
public final String optionGroupName() {
return optionGroupName;
}
/**
*
* The ARN from the key store with which the automated backup is associated for TDE encryption.
*
*
* @return The ARN from the key store with which the automated backup is associated for TDE encryption.
*/
public final String tdeCredentialArn() {
return tdeCredentialArn;
}
/**
*
* Indicates whether the automated backup is encrypted.
*
*
* @return Indicates whether the automated backup is encrypted.
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* The storage type associated with the automated backup.
*
*
* @return The storage type associated with the automated backup.
*/
public final String storageType() {
return storageType;
}
/**
*
* The Amazon Web Services KMS key ID for an automated backup.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @return The Amazon Web Services KMS key ID for an automated backup.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The time zone of the automated backup. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a time
* zone specified.
*
*
* @return The time zone of the automated backup. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a
* time zone specified.
*/
public final String timezone() {
return timezone;
}
/**
*
* True if mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database accounts is
* enabled, and otherwise false.
*
*
* @return True if mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database accounts
* is enabled, and otherwise false.
*/
public final Boolean iamDatabaseAuthenticationEnabled() {
return iamDatabaseAuthenticationEnabled;
}
/**
*
* The retention period for the automated backups.
*
*
* @return The retention period for the automated backups.
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* The Amazon Resource Name (ARN) for the replicated automated backups.
*
*
* @return The Amazon Resource Name (ARN) for the replicated automated backups.
*/
public final String dbInstanceAutomatedBackupsArn() {
return dbInstanceAutomatedBackupsArn;
}
/**
* For responses, this returns true if the service returned a value for the DBInstanceAutomatedBackupsReplications
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasDbInstanceAutomatedBackupsReplications() {
return dbInstanceAutomatedBackupsReplications != null
&& !(dbInstanceAutomatedBackupsReplications instanceof SdkAutoConstructList);
}
/**
*
* The list of replications to different Amazon Web Services Regions associated with the automated backup.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDbInstanceAutomatedBackupsReplications}
* method.
*
*
* @return The list of replications to different Amazon Web Services Regions associated with the automated backup.
*/
public final List dbInstanceAutomatedBackupsReplications() {
return dbInstanceAutomatedBackupsReplications;
}
/**
*
* The location where automated backups are stored: Amazon Web Services Outposts or the Amazon Web Services Region.
*
*
* @return The location where automated backups are stored: Amazon Web Services Outposts or the Amazon Web Services
* Region.
*/
public final String backupTarget() {
return backupTarget;
}
/**
*
* The storage throughput for the automated backup.
*
*
* @return The storage throughput for the automated backup.
*/
public final Integer storageThroughput() {
return storageThroughput;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @return The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*/
public final String awsBackupRecoveryPointArn() {
return awsBackupRecoveryPointArn;
}
/**
*
* Indicates whether the DB instance has a dedicated log volume (DLV) enabled.
*
*
* @return Indicates whether the DB instance has a dedicated log volume (DLV) enabled.
*/
public final Boolean dedicatedLogVolume() {
return dedicatedLogVolume;
}
/**
*
* Specifies whether the automatic backup is for a DB instance in the multi-tenant configuration (TRUE) or the
* single-tenant configuration (FALSE).
*
*
* @return Specifies whether the automatic backup is for a DB instance in the multi-tenant configuration (TRUE) or
* the single-tenant configuration (FALSE).
*/
public final Boolean multiTenant() {
return multiTenant;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(dbiResourceId());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(restoreWindow());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(instanceCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(masterUsername());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(licenseModel());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(optionGroupName());
hashCode = 31 * hashCode + Objects.hashCode(tdeCredentialArn());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(storageType());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(timezone());
hashCode = 31 * hashCode + Objects.hashCode(iamDatabaseAuthenticationEnabled());
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceAutomatedBackupsArn());
hashCode = 31 * hashCode
+ Objects.hashCode(hasDbInstanceAutomatedBackupsReplications() ? dbInstanceAutomatedBackupsReplications() : null);
hashCode = 31 * hashCode + Objects.hashCode(backupTarget());
hashCode = 31 * hashCode + Objects.hashCode(storageThroughput());
hashCode = 31 * hashCode + Objects.hashCode(awsBackupRecoveryPointArn());
hashCode = 31 * hashCode + Objects.hashCode(dedicatedLogVolume());
hashCode = 31 * hashCode + Objects.hashCode(multiTenant());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DBInstanceAutomatedBackup)) {
return false;
}
DBInstanceAutomatedBackup other = (DBInstanceAutomatedBackup) obj;
return Objects.equals(dbInstanceArn(), other.dbInstanceArn()) && Objects.equals(dbiResourceId(), other.dbiResourceId())
&& Objects.equals(region(), other.region())
&& Objects.equals(dbInstanceIdentifier(), other.dbInstanceIdentifier())
&& Objects.equals(restoreWindow(), other.restoreWindow())
&& Objects.equals(allocatedStorage(), other.allocatedStorage()) && Objects.equals(status(), other.status())
&& Objects.equals(port(), other.port()) && Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(vpcId(), other.vpcId()) && Objects.equals(instanceCreateTime(), other.instanceCreateTime())
&& Objects.equals(masterUsername(), other.masterUsername()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion()) && Objects.equals(licenseModel(), other.licenseModel())
&& Objects.equals(iops(), other.iops()) && Objects.equals(optionGroupName(), other.optionGroupName())
&& Objects.equals(tdeCredentialArn(), other.tdeCredentialArn()) && Objects.equals(encrypted(), other.encrypted())
&& Objects.equals(storageType(), other.storageType()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(timezone(), other.timezone())
&& Objects.equals(iamDatabaseAuthenticationEnabled(), other.iamDatabaseAuthenticationEnabled())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(dbInstanceAutomatedBackupsArn(), other.dbInstanceAutomatedBackupsArn())
&& hasDbInstanceAutomatedBackupsReplications() == other.hasDbInstanceAutomatedBackupsReplications()
&& Objects.equals(dbInstanceAutomatedBackupsReplications(), other.dbInstanceAutomatedBackupsReplications())
&& Objects.equals(backupTarget(), other.backupTarget())
&& Objects.equals(storageThroughput(), other.storageThroughput())
&& Objects.equals(awsBackupRecoveryPointArn(), other.awsBackupRecoveryPointArn())
&& Objects.equals(dedicatedLogVolume(), other.dedicatedLogVolume())
&& Objects.equals(multiTenant(), other.multiTenant());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("DBInstanceAutomatedBackup")
.add("DBInstanceArn", dbInstanceArn())
.add("DbiResourceId", dbiResourceId())
.add("Region", region())
.add("DBInstanceIdentifier", dbInstanceIdentifier())
.add("RestoreWindow", restoreWindow())
.add("AllocatedStorage", allocatedStorage())
.add("Status", status())
.add("Port", port())
.add("AvailabilityZone", availabilityZone())
.add("VpcId", vpcId())
.add("InstanceCreateTime", instanceCreateTime())
.add("MasterUsername", masterUsername())
.add("Engine", engine())
.add("EngineVersion", engineVersion())
.add("LicenseModel", licenseModel())
.add("Iops", iops())
.add("OptionGroupName", optionGroupName())
.add("TdeCredentialArn", tdeCredentialArn())
.add("Encrypted", encrypted())
.add("StorageType", storageType())
.add("KmsKeyId", kmsKeyId())
.add("Timezone", timezone())
.add("IAMDatabaseAuthenticationEnabled", iamDatabaseAuthenticationEnabled())
.add("BackupRetentionPeriod", backupRetentionPeriod())
.add("DBInstanceAutomatedBackupsArn", dbInstanceAutomatedBackupsArn())
.add("DBInstanceAutomatedBackupsReplications",
hasDbInstanceAutomatedBackupsReplications() ? dbInstanceAutomatedBackupsReplications() : null)
.add("BackupTarget", backupTarget()).add("StorageThroughput", storageThroughput())
.add("AwsBackupRecoveryPointArn", awsBackupRecoveryPointArn()).add("DedicatedLogVolume", dedicatedLogVolume())
.add("MultiTenant", multiTenant()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBInstanceArn":
return Optional.ofNullable(clazz.cast(dbInstanceArn()));
case "DbiResourceId":
return Optional.ofNullable(clazz.cast(dbiResourceId()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "DBInstanceIdentifier":
return Optional.ofNullable(clazz.cast(dbInstanceIdentifier()));
case "RestoreWindow":
return Optional.ofNullable(clazz.cast(restoreWindow()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "InstanceCreateTime":
return Optional.ofNullable(clazz.cast(instanceCreateTime()));
case "MasterUsername":
return Optional.ofNullable(clazz.cast(masterUsername()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "LicenseModel":
return Optional.ofNullable(clazz.cast(licenseModel()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "OptionGroupName":
return Optional.ofNullable(clazz.cast(optionGroupName()));
case "TdeCredentialArn":
return Optional.ofNullable(clazz.cast(tdeCredentialArn()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "StorageType":
return Optional.ofNullable(clazz.cast(storageType()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "Timezone":
return Optional.ofNullable(clazz.cast(timezone()));
case "IAMDatabaseAuthenticationEnabled":
return Optional.ofNullable(clazz.cast(iamDatabaseAuthenticationEnabled()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "DBInstanceAutomatedBackupsArn":
return Optional.ofNullable(clazz.cast(dbInstanceAutomatedBackupsArn()));
case "DBInstanceAutomatedBackupsReplications":
return Optional.ofNullable(clazz.cast(dbInstanceAutomatedBackupsReplications()));
case "BackupTarget":
return Optional.ofNullable(clazz.cast(backupTarget()));
case "StorageThroughput":
return Optional.ofNullable(clazz.cast(storageThroughput()));
case "AwsBackupRecoveryPointArn":
return Optional.ofNullable(clazz.cast(awsBackupRecoveryPointArn()));
case "DedicatedLogVolume":
return Optional.ofNullable(clazz.cast(dedicatedLogVolume()));
case "MultiTenant":
return Optional.ofNullable(clazz.cast(multiTenant()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function