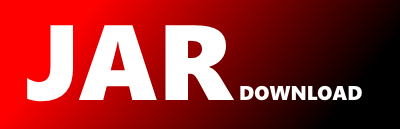
software.amazon.awssdk.services.rds.model.DomainMembership Maven / Gradle / Ivy
Show all versions of rds Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An Active Directory Domain membership record associated with the DB instance or cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DomainMembership implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Domain")
.getter(getter(DomainMembership::domain)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Domain").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DomainMembership::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField FQDN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("FQDN")
.getter(getter(DomainMembership::fqdn)).setter(setter(Builder::fqdn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FQDN").build()).build();
private static final SdkField IAM_ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IAMRoleName").getter(getter(DomainMembership::iamRoleName)).setter(setter(Builder::iamRoleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IAMRoleName").build()).build();
private static final SdkField OU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("OU")
.getter(getter(DomainMembership::ou)).setter(setter(Builder::ou))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OU").build()).build();
private static final SdkField AUTH_SECRET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthSecretArn").getter(getter(DomainMembership::authSecretArn)).setter(setter(Builder::authSecretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthSecretArn").build()).build();
private static final SdkField> DNS_IPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DnsIps")
.getter(getter(DomainMembership::dnsIps))
.setter(setter(Builder::dnsIps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DnsIps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DOMAIN_FIELD, STATUS_FIELD,
FQDN_FIELD, IAM_ROLE_NAME_FIELD, OU_FIELD, AUTH_SECRET_ARN_FIELD, DNS_IPS_FIELD));
private static final long serialVersionUID = 1L;
private final String domain;
private final String status;
private final String fqdn;
private final String iamRoleName;
private final String ou;
private final String authSecretArn;
private final List dnsIps;
private DomainMembership(BuilderImpl builder) {
this.domain = builder.domain;
this.status = builder.status;
this.fqdn = builder.fqdn;
this.iamRoleName = builder.iamRoleName;
this.ou = builder.ou;
this.authSecretArn = builder.authSecretArn;
this.dnsIps = builder.dnsIps;
}
/**
*
* The identifier of the Active Directory Domain.
*
*
* @return The identifier of the Active Directory Domain.
*/
public final String domain() {
return domain;
}
/**
*
* The status of the Active Directory Domain membership for the DB instance or cluster. Values include
* joined
, pending-join
, failed
, and so on.
*
*
* @return The status of the Active Directory Domain membership for the DB instance or cluster. Values include
* joined
, pending-join
, failed
, and so on.
*/
public final String status() {
return status;
}
/**
*
* The fully qualified domain name (FQDN) of the Active Directory Domain.
*
*
* @return The fully qualified domain name (FQDN) of the Active Directory Domain.
*/
public final String fqdn() {
return fqdn;
}
/**
*
* The name of the IAM role used when making API calls to the Directory Service.
*
*
* @return The name of the IAM role used when making API calls to the Directory Service.
*/
public final String iamRoleName() {
return iamRoleName;
}
/**
*
* The Active Directory organizational unit for the DB instance or cluster.
*
*
* @return The Active Directory organizational unit for the DB instance or cluster.
*/
public final String ou() {
return ou;
}
/**
*
* The ARN for the Secrets Manager secret with the credentials for the user that's a member of the domain.
*
*
* @return The ARN for the Secrets Manager secret with the credentials for the user that's a member of the domain.
*/
public final String authSecretArn() {
return authSecretArn;
}
/**
* For responses, this returns true if the service returned a value for the DnsIps property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDnsIps() {
return dnsIps != null && !(dnsIps instanceof SdkAutoConstructList);
}
/**
*
* The IPv4 DNS IP addresses of the primary and secondary Active Directory domain controllers.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDnsIps} method.
*
*
* @return The IPv4 DNS IP addresses of the primary and secondary Active Directory domain controllers.
*/
public final List dnsIps() {
return dnsIps;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(domain());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(fqdn());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleName());
hashCode = 31 * hashCode + Objects.hashCode(ou());
hashCode = 31 * hashCode + Objects.hashCode(authSecretArn());
hashCode = 31 * hashCode + Objects.hashCode(hasDnsIps() ? dnsIps() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DomainMembership)) {
return false;
}
DomainMembership other = (DomainMembership) obj;
return Objects.equals(domain(), other.domain()) && Objects.equals(status(), other.status())
&& Objects.equals(fqdn(), other.fqdn()) && Objects.equals(iamRoleName(), other.iamRoleName())
&& Objects.equals(ou(), other.ou()) && Objects.equals(authSecretArn(), other.authSecretArn())
&& hasDnsIps() == other.hasDnsIps() && Objects.equals(dnsIps(), other.dnsIps());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DomainMembership").add("Domain", domain()).add("Status", status()).add("FQDN", fqdn())
.add("IAMRoleName", iamRoleName()).add("OU", ou()).add("AuthSecretArn", authSecretArn())
.add("DnsIps", hasDnsIps() ? dnsIps() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Domain":
return Optional.ofNullable(clazz.cast(domain()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "FQDN":
return Optional.ofNullable(clazz.cast(fqdn()));
case "IAMRoleName":
return Optional.ofNullable(clazz.cast(iamRoleName()));
case "OU":
return Optional.ofNullable(clazz.cast(ou()));
case "AuthSecretArn":
return Optional.ofNullable(clazz.cast(authSecretArn()));
case "DnsIps":
return Optional.ofNullable(clazz.cast(dnsIps()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function