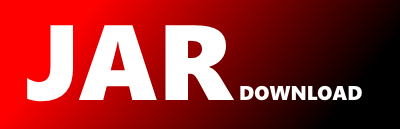
software.amazon.awssdk.services.rds.model.DBRecommendation Maven / Gradle / Ivy
Show all versions of rds Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The recommendation for your DB instances, DB clusters, and DB parameter groups.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DBRecommendation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RECOMMENDATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecommendationId").getter(getter(DBRecommendation::recommendationId))
.setter(setter(Builder::recommendationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendationId").build()).build();
private static final SdkField TYPE_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("TypeId")
.getter(getter(DBRecommendation::typeId)).setter(setter(Builder::typeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeId").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Severity").getter(getter(DBRecommendation::severity)).setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Severity").build()).build();
private static final SdkField RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceArn").getter(getter(DBRecommendation::resourceArn)).setter(setter(Builder::resourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceArn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DBRecommendation::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField CREATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedTime").getter(getter(DBRecommendation::createdTime)).setter(setter(Builder::createdTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedTime").build()).build();
private static final SdkField UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("UpdatedTime").getter(getter(DBRecommendation::updatedTime)).setter(setter(Builder::updatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedTime").build()).build();
private static final SdkField DETECTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Detection").getter(getter(DBRecommendation::detection)).setter(setter(Builder::detection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Detection").build()).build();
private static final SdkField RECOMMENDATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Recommendation").getter(getter(DBRecommendation::recommendation))
.setter(setter(Builder::recommendation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Recommendation").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(DBRecommendation::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Reason")
.getter(getter(DBRecommendation::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Reason").build()).build();
private static final SdkField> RECOMMENDED_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RecommendedActions")
.getter(getter(DBRecommendation::recommendedActions))
.setter(setter(Builder::recommendedActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendedActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RecommendedAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CATEGORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Category").getter(getter(DBRecommendation::category)).setter(setter(Builder::category))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Category").build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Source")
.getter(getter(DBRecommendation::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField TYPE_DETECTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TypeDetection").getter(getter(DBRecommendation::typeDetection)).setter(setter(Builder::typeDetection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeDetection").build()).build();
private static final SdkField TYPE_RECOMMENDATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TypeRecommendation").getter(getter(DBRecommendation::typeRecommendation))
.setter(setter(Builder::typeRecommendation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TypeRecommendation").build())
.build();
private static final SdkField IMPACT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Impact")
.getter(getter(DBRecommendation::impact)).setter(setter(Builder::impact))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Impact").build()).build();
private static final SdkField ADDITIONAL_INFO_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdditionalInfo").getter(getter(DBRecommendation::additionalInfo))
.setter(setter(Builder::additionalInfo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalInfo").build()).build();
private static final SdkField> LINKS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Links")
.getter(getter(DBRecommendation::links))
.setter(setter(Builder::links))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Links").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DocLink::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ISSUE_DETAILS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("IssueDetails").getter(getter(DBRecommendation::issueDetails)).setter(setter(Builder::issueDetails))
.constructor(IssueDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IssueDetails").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(RECOMMENDATION_ID_FIELD,
TYPE_ID_FIELD, SEVERITY_FIELD, RESOURCE_ARN_FIELD, STATUS_FIELD, CREATED_TIME_FIELD, UPDATED_TIME_FIELD,
DETECTION_FIELD, RECOMMENDATION_FIELD, DESCRIPTION_FIELD, REASON_FIELD, RECOMMENDED_ACTIONS_FIELD, CATEGORY_FIELD,
SOURCE_FIELD, TYPE_DETECTION_FIELD, TYPE_RECOMMENDATION_FIELD, IMPACT_FIELD, ADDITIONAL_INFO_FIELD, LINKS_FIELD,
ISSUE_DETAILS_FIELD));
private static final long serialVersionUID = 1L;
private final String recommendationId;
private final String typeId;
private final String severity;
private final String resourceArn;
private final String status;
private final Instant createdTime;
private final Instant updatedTime;
private final String detection;
private final String recommendation;
private final String description;
private final String reason;
private final List recommendedActions;
private final String category;
private final String source;
private final String typeDetection;
private final String typeRecommendation;
private final String impact;
private final String additionalInfo;
private final List links;
private final IssueDetails issueDetails;
private DBRecommendation(BuilderImpl builder) {
this.recommendationId = builder.recommendationId;
this.typeId = builder.typeId;
this.severity = builder.severity;
this.resourceArn = builder.resourceArn;
this.status = builder.status;
this.createdTime = builder.createdTime;
this.updatedTime = builder.updatedTime;
this.detection = builder.detection;
this.recommendation = builder.recommendation;
this.description = builder.description;
this.reason = builder.reason;
this.recommendedActions = builder.recommendedActions;
this.category = builder.category;
this.source = builder.source;
this.typeDetection = builder.typeDetection;
this.typeRecommendation = builder.typeRecommendation;
this.impact = builder.impact;
this.additionalInfo = builder.additionalInfo;
this.links = builder.links;
this.issueDetails = builder.issueDetails;
}
/**
*
* The unique identifier of the recommendation.
*
*
* @return The unique identifier of the recommendation.
*/
public final String recommendationId() {
return recommendationId;
}
/**
*
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*
*
* @return A value that indicates the type of recommendation. This value determines how the description is rendered.
*/
public final String typeId() {
return typeId;
}
/**
*
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*
*
* @return The severity level of the recommendation. The severity level can help you decide the urgency with which
* to address the recommendation.
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*/
public final String severity() {
return severity;
}
/**
*
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*
*
* @return The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*/
public final String resourceArn() {
return resourceArn;
}
/**
*
* The current status of the recommendation.
*
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*
*
* @return The current status of the recommendation.
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*/
public final String status() {
return status;
}
/**
*
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*
*
* @return The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*/
public final Instant createdTime() {
return createdTime;
}
/**
*
* The time when the recommendation was last updated.
*
*
* @return The time when the recommendation was last updated.
*/
public final Instant updatedTime() {
return updatedTime;
}
/**
*
* A short description of the issue identified for this recommendation. The description might contain markdown.
*
*
* @return A short description of the issue identified for this recommendation. The description might contain
* markdown.
*/
public final String detection() {
return detection;
}
/**
*
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*
*
* @return A short description of the recommendation to resolve an issue. The description might contain markdown.
*/
public final String recommendation() {
return recommendation;
}
/**
*
* A detailed description of the recommendation. The description might contain markdown.
*
*
* @return A detailed description of the recommendation. The description might contain markdown.
*/
public final String description() {
return description;
}
/**
*
* The reason why this recommendation was created. The information might contain markdown.
*
*
* @return The reason why this recommendation was created. The information might contain markdown.
*/
public final String reason() {
return reason;
}
/**
* For responses, this returns true if the service returned a value for the RecommendedActions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRecommendedActions() {
return recommendedActions != null && !(recommendedActions instanceof SdkAutoConstructList);
}
/**
*
* A list of recommended actions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRecommendedActions} method.
*
*
* @return A list of recommended actions.
*/
public final List recommendedActions() {
return recommendedActions;
}
/**
*
* The category of the recommendation.
*
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*
*
* @return The category of the recommendation.
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*/
public final String category() {
return category;
}
/**
*
* The Amazon Web Services service that generated the recommendations.
*
*
* @return The Amazon Web Services service that generated the recommendations.
*/
public final String source() {
return source;
}
/**
*
* A short description of the recommendation type. The description might contain markdown.
*
*
* @return A short description of the recommendation type. The description might contain markdown.
*/
public final String typeDetection() {
return typeDetection;
}
/**
*
* A short description that summarizes the recommendation to fix all the issues of the recommendation type. The
* description might contain markdown.
*
*
* @return A short description that summarizes the recommendation to fix all the issues of the recommendation type.
* The description might contain markdown.
*/
public final String typeRecommendation() {
return typeRecommendation;
}
/**
*
* A short description that explains the possible impact of an issue.
*
*
* @return A short description that explains the possible impact of an issue.
*/
public final String impact() {
return impact;
}
/**
*
* Additional information about the recommendation. The information might contain markdown.
*
*
* @return Additional information about the recommendation. The information might contain markdown.
*/
public final String additionalInfo() {
return additionalInfo;
}
/**
* For responses, this returns true if the service returned a value for the Links property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasLinks() {
return links != null && !(links instanceof SdkAutoConstructList);
}
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLinks} method.
*
*
* @return A link to documentation that provides additional information about the recommendation.
*/
public final List links() {
return links;
}
/**
*
* Details of the issue that caused the recommendation.
*
*
* @return Details of the issue that caused the recommendation.
*/
public final IssueDetails issueDetails() {
return issueDetails;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(recommendationId());
hashCode = 31 * hashCode + Objects.hashCode(typeId());
hashCode = 31 * hashCode + Objects.hashCode(severity());
hashCode = 31 * hashCode + Objects.hashCode(resourceArn());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(createdTime());
hashCode = 31 * hashCode + Objects.hashCode(updatedTime());
hashCode = 31 * hashCode + Objects.hashCode(detection());
hashCode = 31 * hashCode + Objects.hashCode(recommendation());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(reason());
hashCode = 31 * hashCode + Objects.hashCode(hasRecommendedActions() ? recommendedActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(category());
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(typeDetection());
hashCode = 31 * hashCode + Objects.hashCode(typeRecommendation());
hashCode = 31 * hashCode + Objects.hashCode(impact());
hashCode = 31 * hashCode + Objects.hashCode(additionalInfo());
hashCode = 31 * hashCode + Objects.hashCode(hasLinks() ? links() : null);
hashCode = 31 * hashCode + Objects.hashCode(issueDetails());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DBRecommendation)) {
return false;
}
DBRecommendation other = (DBRecommendation) obj;
return Objects.equals(recommendationId(), other.recommendationId()) && Objects.equals(typeId(), other.typeId())
&& Objects.equals(severity(), other.severity()) && Objects.equals(resourceArn(), other.resourceArn())
&& Objects.equals(status(), other.status()) && Objects.equals(createdTime(), other.createdTime())
&& Objects.equals(updatedTime(), other.updatedTime()) && Objects.equals(detection(), other.detection())
&& Objects.equals(recommendation(), other.recommendation()) && Objects.equals(description(), other.description())
&& Objects.equals(reason(), other.reason()) && hasRecommendedActions() == other.hasRecommendedActions()
&& Objects.equals(recommendedActions(), other.recommendedActions())
&& Objects.equals(category(), other.category()) && Objects.equals(source(), other.source())
&& Objects.equals(typeDetection(), other.typeDetection())
&& Objects.equals(typeRecommendation(), other.typeRecommendation()) && Objects.equals(impact(), other.impact())
&& Objects.equals(additionalInfo(), other.additionalInfo()) && hasLinks() == other.hasLinks()
&& Objects.equals(links(), other.links()) && Objects.equals(issueDetails(), other.issueDetails());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DBRecommendation").add("RecommendationId", recommendationId()).add("TypeId", typeId())
.add("Severity", severity()).add("ResourceArn", resourceArn()).add("Status", status())
.add("CreatedTime", createdTime()).add("UpdatedTime", updatedTime()).add("Detection", detection())
.add("Recommendation", recommendation()).add("Description", description()).add("Reason", reason())
.add("RecommendedActions", hasRecommendedActions() ? recommendedActions() : null).add("Category", category())
.add("Source", source()).add("TypeDetection", typeDetection()).add("TypeRecommendation", typeRecommendation())
.add("Impact", impact()).add("AdditionalInfo", additionalInfo()).add("Links", hasLinks() ? links() : null)
.add("IssueDetails", issueDetails()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RecommendationId":
return Optional.ofNullable(clazz.cast(recommendationId()));
case "TypeId":
return Optional.ofNullable(clazz.cast(typeId()));
case "Severity":
return Optional.ofNullable(clazz.cast(severity()));
case "ResourceArn":
return Optional.ofNullable(clazz.cast(resourceArn()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "CreatedTime":
return Optional.ofNullable(clazz.cast(createdTime()));
case "UpdatedTime":
return Optional.ofNullable(clazz.cast(updatedTime()));
case "Detection":
return Optional.ofNullable(clazz.cast(detection()));
case "Recommendation":
return Optional.ofNullable(clazz.cast(recommendation()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Reason":
return Optional.ofNullable(clazz.cast(reason()));
case "RecommendedActions":
return Optional.ofNullable(clazz.cast(recommendedActions()));
case "Category":
return Optional.ofNullable(clazz.cast(category()));
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "TypeDetection":
return Optional.ofNullable(clazz.cast(typeDetection()));
case "TypeRecommendation":
return Optional.ofNullable(clazz.cast(typeRecommendation()));
case "Impact":
return Optional.ofNullable(clazz.cast(impact()));
case "AdditionalInfo":
return Optional.ofNullable(clazz.cast(additionalInfo()));
case "Links":
return Optional.ofNullable(clazz.cast(links()));
case "IssueDetails":
return Optional.ofNullable(clazz.cast(issueDetails()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function