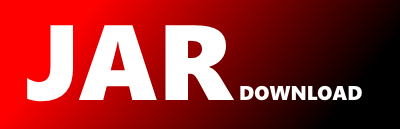
software.amazon.awssdk.services.rds.model.CancelExportTaskResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the details of a snapshot or cluster export to Amazon S3.
*
*
* This data type is used as a response element in the DescribeExportTasks
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CancelExportTaskResponse extends RdsResponse implements
ToCopyableBuilder {
private static final SdkField EXPORT_TASK_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportTaskIdentifier").getter(getter(CancelExportTaskResponse::exportTaskIdentifier))
.setter(setter(Builder::exportTaskIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportTaskIdentifier").build())
.build();
private static final SdkField SOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceArn").getter(getter(CancelExportTaskResponse::sourceArn)).setter(setter(Builder::sourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceArn").build()).build();
private static final SdkField> EXPORT_ONLY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExportOnly")
.getter(getter(CancelExportTaskResponse::exportOnly))
.setter(setter(Builder::exportOnly))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportOnly").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SNAPSHOT_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("SnapshotTime").getter(getter(CancelExportTaskResponse::snapshotTime))
.setter(setter(Builder::snapshotTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotTime").build()).build();
private static final SdkField TASK_START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("TaskStartTime").getter(getter(CancelExportTaskResponse::taskStartTime))
.setter(setter(Builder::taskStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskStartTime").build()).build();
private static final SdkField TASK_END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("TaskEndTime").getter(getter(CancelExportTaskResponse::taskEndTime)).setter(setter(Builder::taskEndTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskEndTime").build()).build();
private static final SdkField S3_BUCKET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3Bucket").getter(getter(CancelExportTaskResponse::s3Bucket)).setter(setter(Builder::s3Bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Bucket").build()).build();
private static final SdkField S3_PREFIX_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3Prefix").getter(getter(CancelExportTaskResponse::s3Prefix)).setter(setter(Builder::s3Prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Prefix").build()).build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IamRoleArn").getter(getter(CancelExportTaskResponse::iamRoleArn)).setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(CancelExportTaskResponse::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(CancelExportTaskResponse::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PERCENT_PROGRESS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PercentProgress").getter(getter(CancelExportTaskResponse::percentProgress))
.setter(setter(Builder::percentProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentProgress").build()).build();
private static final SdkField TOTAL_EXTRACTED_DATA_IN_GB_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TotalExtractedDataInGB").getter(getter(CancelExportTaskResponse::totalExtractedDataInGB))
.setter(setter(Builder::totalExtractedDataInGB))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalExtractedDataInGB").build())
.build();
private static final SdkField FAILURE_CAUSE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureCause").getter(getter(CancelExportTaskResponse::failureCause))
.setter(setter(Builder::failureCause))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureCause").build()).build();
private static final SdkField WARNING_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WarningMessage").getter(getter(CancelExportTaskResponse::warningMessage))
.setter(setter(Builder::warningMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WarningMessage").build()).build();
private static final SdkField SOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceType").getter(getter(CancelExportTaskResponse::sourceTypeAsString))
.setter(setter(Builder::sourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EXPORT_TASK_IDENTIFIER_FIELD,
SOURCE_ARN_FIELD, EXPORT_ONLY_FIELD, SNAPSHOT_TIME_FIELD, TASK_START_TIME_FIELD, TASK_END_TIME_FIELD,
S3_BUCKET_FIELD, S3_PREFIX_FIELD, IAM_ROLE_ARN_FIELD, KMS_KEY_ID_FIELD, STATUS_FIELD, PERCENT_PROGRESS_FIELD,
TOTAL_EXTRACTED_DATA_IN_GB_FIELD, FAILURE_CAUSE_FIELD, WARNING_MESSAGE_FIELD, SOURCE_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String exportTaskIdentifier;
private final String sourceArn;
private final List exportOnly;
private final Instant snapshotTime;
private final Instant taskStartTime;
private final Instant taskEndTime;
private final String s3Bucket;
private final String s3Prefix;
private final String iamRoleArn;
private final String kmsKeyId;
private final String status;
private final Integer percentProgress;
private final Integer totalExtractedDataInGB;
private final String failureCause;
private final String warningMessage;
private final String sourceType;
private CancelExportTaskResponse(BuilderImpl builder) {
super(builder);
this.exportTaskIdentifier = builder.exportTaskIdentifier;
this.sourceArn = builder.sourceArn;
this.exportOnly = builder.exportOnly;
this.snapshotTime = builder.snapshotTime;
this.taskStartTime = builder.taskStartTime;
this.taskEndTime = builder.taskEndTime;
this.s3Bucket = builder.s3Bucket;
this.s3Prefix = builder.s3Prefix;
this.iamRoleArn = builder.iamRoleArn;
this.kmsKeyId = builder.kmsKeyId;
this.status = builder.status;
this.percentProgress = builder.percentProgress;
this.totalExtractedDataInGB = builder.totalExtractedDataInGB;
this.failureCause = builder.failureCause;
this.warningMessage = builder.warningMessage;
this.sourceType = builder.sourceType;
}
/**
*
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3 bucket
* where the data is exported.
*
*
* @return A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon
* S3 bucket where the data is exported.
*/
public final String exportTaskIdentifier() {
return exportTaskIdentifier;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*
*
* @return The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*/
public final String sourceArn() {
return sourceArn;
}
/**
* For responses, this returns true if the service returned a value for the ExportOnly property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasExportOnly() {
return exportOnly != null && !(exportOnly instanceof SdkAutoConstructList);
}
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExportOnly} method.
*
*
* @return The data exported from the snapshot or cluster.
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is
* valid only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster.
* This format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*/
public final List exportOnly() {
return exportOnly;
}
/**
*
* The time when the snapshot was created.
*
*
* @return The time when the snapshot was created.
*/
public final Instant snapshotTime() {
return snapshotTime;
}
/**
*
* The time when the snapshot or cluster export task started.
*
*
* @return The time when the snapshot or cluster export task started.
*/
public final Instant taskStartTime() {
return taskStartTime;
}
/**
*
* The time when the snapshot or cluster export task ended.
*
*
* @return The time when the snapshot or cluster export task ended.
*/
public final Instant taskEndTime() {
return taskEndTime;
}
/**
*
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*
*
* @return The Amazon S3 bucket where the snapshot or cluster is exported to.
*/
public final String s3Bucket() {
return s3Bucket;
}
/**
*
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*
*
* @return The Amazon S3 bucket prefix that is the file name and path of the exported data.
*/
public final String s3Prefix() {
return s3Prefix;
}
/**
*
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*
*
* @return The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*/
public final String iamRoleArn() {
return iamRoleArn;
}
/**
*
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported to
* Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used for the
* export must have encryption and decryption permissions to use this KMS key.
*
*
* @return The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported
* to Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used
* for the export must have encryption and decryption permissions to use this KMS key.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The progress status of the export task. The status can be one of the following:
*
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*
*
* @return The progress status of the export task. The status can be one of the following:
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*/
public final String status() {
return status;
}
/**
*
* The progress of the snapshot or cluster export task as a percentage.
*
*
* @return The progress of the snapshot or cluster export task as a percentage.
*/
public final Integer percentProgress() {
return percentProgress;
}
/**
*
* The total amount of data exported, in gigabytes.
*
*
* @return The total amount of data exported, in gigabytes.
*/
public final Integer totalExtractedDataInGB() {
return totalExtractedDataInGB;
}
/**
*
* The reason the export failed, if it failed.
*
*
* @return The reason the export failed, if it failed.
*/
public final String failureCause() {
return failureCause;
}
/**
*
* A warning about the snapshot or cluster export task.
*
*
* @return A warning about the snapshot or cluster export task.
*/
public final String warningMessage() {
return warningMessage;
}
/**
*
* The type of source for the export.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link ExportSourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The type of source for the export.
* @see ExportSourceType
*/
public final ExportSourceType sourceType() {
return ExportSourceType.fromValue(sourceType);
}
/**
*
* The type of source for the export.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link ExportSourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The type of source for the export.
* @see ExportSourceType
*/
public final String sourceTypeAsString() {
return sourceType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(exportTaskIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceArn());
hashCode = 31 * hashCode + Objects.hashCode(hasExportOnly() ? exportOnly() : null);
hashCode = 31 * hashCode + Objects.hashCode(snapshotTime());
hashCode = 31 * hashCode + Objects.hashCode(taskStartTime());
hashCode = 31 * hashCode + Objects.hashCode(taskEndTime());
hashCode = 31 * hashCode + Objects.hashCode(s3Bucket());
hashCode = 31 * hashCode + Objects.hashCode(s3Prefix());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(percentProgress());
hashCode = 31 * hashCode + Objects.hashCode(totalExtractedDataInGB());
hashCode = 31 * hashCode + Objects.hashCode(failureCause());
hashCode = 31 * hashCode + Objects.hashCode(warningMessage());
hashCode = 31 * hashCode + Objects.hashCode(sourceTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CancelExportTaskResponse)) {
return false;
}
CancelExportTaskResponse other = (CancelExportTaskResponse) obj;
return Objects.equals(exportTaskIdentifier(), other.exportTaskIdentifier())
&& Objects.equals(sourceArn(), other.sourceArn()) && hasExportOnly() == other.hasExportOnly()
&& Objects.equals(exportOnly(), other.exportOnly()) && Objects.equals(snapshotTime(), other.snapshotTime())
&& Objects.equals(taskStartTime(), other.taskStartTime()) && Objects.equals(taskEndTime(), other.taskEndTime())
&& Objects.equals(s3Bucket(), other.s3Bucket()) && Objects.equals(s3Prefix(), other.s3Prefix())
&& Objects.equals(iamRoleArn(), other.iamRoleArn()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(status(), other.status()) && Objects.equals(percentProgress(), other.percentProgress())
&& Objects.equals(totalExtractedDataInGB(), other.totalExtractedDataInGB())
&& Objects.equals(failureCause(), other.failureCause())
&& Objects.equals(warningMessage(), other.warningMessage())
&& Objects.equals(sourceTypeAsString(), other.sourceTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CancelExportTaskResponse").add("ExportTaskIdentifier", exportTaskIdentifier())
.add("SourceArn", sourceArn()).add("ExportOnly", hasExportOnly() ? exportOnly() : null)
.add("SnapshotTime", snapshotTime()).add("TaskStartTime", taskStartTime()).add("TaskEndTime", taskEndTime())
.add("S3Bucket", s3Bucket()).add("S3Prefix", s3Prefix()).add("IamRoleArn", iamRoleArn())
.add("KmsKeyId", kmsKeyId()).add("Status", status()).add("PercentProgress", percentProgress())
.add("TotalExtractedDataInGB", totalExtractedDataInGB()).add("FailureCause", failureCause())
.add("WarningMessage", warningMessage()).add("SourceType", sourceTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ExportTaskIdentifier":
return Optional.ofNullable(clazz.cast(exportTaskIdentifier()));
case "SourceArn":
return Optional.ofNullable(clazz.cast(sourceArn()));
case "ExportOnly":
return Optional.ofNullable(clazz.cast(exportOnly()));
case "SnapshotTime":
return Optional.ofNullable(clazz.cast(snapshotTime()));
case "TaskStartTime":
return Optional.ofNullable(clazz.cast(taskStartTime()));
case "TaskEndTime":
return Optional.ofNullable(clazz.cast(taskEndTime()));
case "S3Bucket":
return Optional.ofNullable(clazz.cast(s3Bucket()));
case "S3Prefix":
return Optional.ofNullable(clazz.cast(s3Prefix()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "PercentProgress":
return Optional.ofNullable(clazz.cast(percentProgress()));
case "TotalExtractedDataInGB":
return Optional.ofNullable(clazz.cast(totalExtractedDataInGB()));
case "FailureCause":
return Optional.ofNullable(clazz.cast(failureCause()));
case "WarningMessage":
return Optional.ofNullable(clazz.cast(warningMessage()));
case "SourceType":
return Optional.ofNullable(clazz.cast(sourceTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ExportTaskIdentifier", EXPORT_TASK_IDENTIFIER_FIELD);
map.put("SourceArn", SOURCE_ARN_FIELD);
map.put("ExportOnly", EXPORT_ONLY_FIELD);
map.put("SnapshotTime", SNAPSHOT_TIME_FIELD);
map.put("TaskStartTime", TASK_START_TIME_FIELD);
map.put("TaskEndTime", TASK_END_TIME_FIELD);
map.put("S3Bucket", S3_BUCKET_FIELD);
map.put("S3Prefix", S3_PREFIX_FIELD);
map.put("IamRoleArn", IAM_ROLE_ARN_FIELD);
map.put("KmsKeyId", KMS_KEY_ID_FIELD);
map.put("Status", STATUS_FIELD);
map.put("PercentProgress", PERCENT_PROGRESS_FIELD);
map.put("TotalExtractedDataInGB", TOTAL_EXTRACTED_DATA_IN_GB_FIELD);
map.put("FailureCause", FAILURE_CAUSE_FIELD);
map.put("WarningMessage", WARNING_MESSAGE_FIELD);
map.put("SourceType", SOURCE_TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function