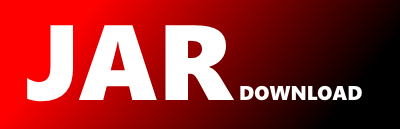
software.amazon.awssdk.services.rds.model.ScalingConfigurationInfo Maven / Gradle / Ivy
Show all versions of rds Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.rds.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The scaling configuration for an Aurora DB cluster in serverless
DB engine mode.
*
*
* For more information, see Using Amazon Aurora
* Serverless v1 in the Amazon Aurora User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScalingConfigurationInfo implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MIN_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MinCapacity").getter(getter(ScalingConfigurationInfo::minCapacity)).setter(setter(Builder::minCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinCapacity").build()).build();
private static final SdkField MAX_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxCapacity").getter(getter(ScalingConfigurationInfo::maxCapacity)).setter(setter(Builder::maxCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxCapacity").build()).build();
private static final SdkField AUTO_PAUSE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoPause").getter(getter(ScalingConfigurationInfo::autoPause)).setter(setter(Builder::autoPause))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoPause").build()).build();
private static final SdkField SECONDS_UNTIL_AUTO_PAUSE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SecondsUntilAutoPause").getter(getter(ScalingConfigurationInfo::secondsUntilAutoPause))
.setter(setter(Builder::secondsUntilAutoPause))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecondsUntilAutoPause").build())
.build();
private static final SdkField TIMEOUT_ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimeoutAction").getter(getter(ScalingConfigurationInfo::timeoutAction))
.setter(setter(Builder::timeoutAction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutAction").build()).build();
private static final SdkField SECONDS_BEFORE_TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SecondsBeforeTimeout").getter(getter(ScalingConfigurationInfo::secondsBeforeTimeout))
.setter(setter(Builder::secondsBeforeTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecondsBeforeTimeout").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MIN_CAPACITY_FIELD,
MAX_CAPACITY_FIELD, AUTO_PAUSE_FIELD, SECONDS_UNTIL_AUTO_PAUSE_FIELD, TIMEOUT_ACTION_FIELD,
SECONDS_BEFORE_TIMEOUT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Integer minCapacity;
private final Integer maxCapacity;
private final Boolean autoPause;
private final Integer secondsUntilAutoPause;
private final String timeoutAction;
private final Integer secondsBeforeTimeout;
private ScalingConfigurationInfo(BuilderImpl builder) {
this.minCapacity = builder.minCapacity;
this.maxCapacity = builder.maxCapacity;
this.autoPause = builder.autoPause;
this.secondsUntilAutoPause = builder.secondsUntilAutoPause;
this.timeoutAction = builder.timeoutAction;
this.secondsBeforeTimeout = builder.secondsBeforeTimeout;
}
/**
*
* The minimum capacity for an Aurora DB cluster in serverless
DB engine mode.
*
*
* @return The minimum capacity for an Aurora DB cluster in serverless
DB engine mode.
*/
public final Integer minCapacity() {
return minCapacity;
}
/**
*
* The maximum capacity for an Aurora DB cluster in serverless
DB engine mode.
*
*
* @return The maximum capacity for an Aurora DB cluster in serverless
DB engine mode.
*/
public final Integer maxCapacity() {
return maxCapacity;
}
/**
*
* Indicates whether automatic pause is allowed for the Aurora DB cluster in serverless
DB engine mode.
*
*
* When the value is set to false for an Aurora Serverless v1 DB cluster, the DB cluster automatically resumes.
*
*
* @return Indicates whether automatic pause is allowed for the Aurora DB cluster in serverless
DB
* engine mode.
*
* When the value is set to false for an Aurora Serverless v1 DB cluster, the DB cluster automatically
* resumes.
*/
public final Boolean autoPause() {
return autoPause;
}
/**
*
* The remaining amount of time, in seconds, before the Aurora DB cluster in serverless
mode is paused.
* A DB cluster can be paused only when it's idle (it has no connections).
*
*
* @return The remaining amount of time, in seconds, before the Aurora DB cluster in serverless
mode is
* paused. A DB cluster can be paused only when it's idle (it has no connections).
*/
public final Integer secondsUntilAutoPause() {
return secondsUntilAutoPause;
}
/**
*
* The action that occurs when Aurora times out while attempting to change the capacity of an Aurora Serverless v1
* cluster. The value is either ForceApplyCapacityChange
or RollbackCapacityChange
.
*
*
* ForceApplyCapacityChange
, the default, sets the capacity to the specified value as soon as possible.
*
*
* RollbackCapacityChange
ignores the capacity change if a scaling point isn't found in the timeout
* period.
*
*
* @return The action that occurs when Aurora times out while attempting to change the capacity of an Aurora
* Serverless v1 cluster. The value is either ForceApplyCapacityChange
or
* RollbackCapacityChange
.
*
* ForceApplyCapacityChange
, the default, sets the capacity to the specified value as soon as
* possible.
*
*
* RollbackCapacityChange
ignores the capacity change if a scaling point isn't found in the
* timeout period.
*/
public final String timeoutAction() {
return timeoutAction;
}
/**
*
* The number of seconds before scaling times out. What happens when an attempted scaling action times out is
* determined by the TimeoutAction
setting.
*
*
* @return The number of seconds before scaling times out. What happens when an attempted scaling action times out
* is determined by the TimeoutAction
setting.
*/
public final Integer secondsBeforeTimeout() {
return secondsBeforeTimeout;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(minCapacity());
hashCode = 31 * hashCode + Objects.hashCode(maxCapacity());
hashCode = 31 * hashCode + Objects.hashCode(autoPause());
hashCode = 31 * hashCode + Objects.hashCode(secondsUntilAutoPause());
hashCode = 31 * hashCode + Objects.hashCode(timeoutAction());
hashCode = 31 * hashCode + Objects.hashCode(secondsBeforeTimeout());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScalingConfigurationInfo)) {
return false;
}
ScalingConfigurationInfo other = (ScalingConfigurationInfo) obj;
return Objects.equals(minCapacity(), other.minCapacity()) && Objects.equals(maxCapacity(), other.maxCapacity())
&& Objects.equals(autoPause(), other.autoPause())
&& Objects.equals(secondsUntilAutoPause(), other.secondsUntilAutoPause())
&& Objects.equals(timeoutAction(), other.timeoutAction())
&& Objects.equals(secondsBeforeTimeout(), other.secondsBeforeTimeout());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ScalingConfigurationInfo").add("MinCapacity", minCapacity()).add("MaxCapacity", maxCapacity())
.add("AutoPause", autoPause()).add("SecondsUntilAutoPause", secondsUntilAutoPause())
.add("TimeoutAction", timeoutAction()).add("SecondsBeforeTimeout", secondsBeforeTimeout()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MinCapacity":
return Optional.ofNullable(clazz.cast(minCapacity()));
case "MaxCapacity":
return Optional.ofNullable(clazz.cast(maxCapacity()));
case "AutoPause":
return Optional.ofNullable(clazz.cast(autoPause()));
case "SecondsUntilAutoPause":
return Optional.ofNullable(clazz.cast(secondsUntilAutoPause()));
case "TimeoutAction":
return Optional.ofNullable(clazz.cast(timeoutAction()));
case "SecondsBeforeTimeout":
return Optional.ofNullable(clazz.cast(secondsBeforeTimeout()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("MinCapacity", MIN_CAPACITY_FIELD);
map.put("MaxCapacity", MAX_CAPACITY_FIELD);
map.put("AutoPause", AUTO_PAUSE_FIELD);
map.put("SecondsUntilAutoPause", SECONDS_UNTIL_AUTO_PAUSE_FIELD);
map.put("TimeoutAction", TIMEOUT_ACTION_FIELD);
map.put("SecondsBeforeTimeout", SECONDS_BEFORE_TIMEOUT_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function