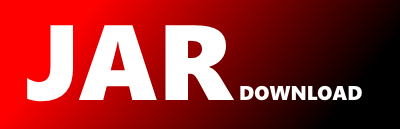
software.amazon.awssdk.services.redshift.model.ModifyUsageLimitResponse Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a usage limit object for a cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyUsageLimitResponse extends RedshiftResponse implements
ToCopyableBuilder {
private static final SdkField USAGE_LIMIT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::usageLimitId)).setter(setter(Builder::usageLimitId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UsageLimitId").build()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::clusterIdentifier)).setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField FEATURE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::featureTypeAsString)).setter(setter(Builder::featureType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FeatureType").build()).build();
private static final SdkField LIMIT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::limitTypeAsString)).setter(setter(Builder::limitType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LimitType").build()).build();
private static final SdkField AMOUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(ModifyUsageLimitResponse::amount)).setter(setter(Builder::amount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Amount").build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::periodAsString)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField BREACH_ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyUsageLimitResponse::breachActionAsString)).setter(setter(Builder::breachAction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BreachAction").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ModifyUsageLimitResponse::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USAGE_LIMIT_ID_FIELD,
CLUSTER_IDENTIFIER_FIELD, FEATURE_TYPE_FIELD, LIMIT_TYPE_FIELD, AMOUNT_FIELD, PERIOD_FIELD, BREACH_ACTION_FIELD,
TAGS_FIELD));
private final String usageLimitId;
private final String clusterIdentifier;
private final String featureType;
private final String limitType;
private final Long amount;
private final String period;
private final String breachAction;
private final List tags;
private ModifyUsageLimitResponse(BuilderImpl builder) {
super(builder);
this.usageLimitId = builder.usageLimitId;
this.clusterIdentifier = builder.clusterIdentifier;
this.featureType = builder.featureType;
this.limitType = builder.limitType;
this.amount = builder.amount;
this.period = builder.period;
this.breachAction = builder.breachAction;
this.tags = builder.tags;
}
/**
*
* The identifier of the usage limit.
*
*
* @return The identifier of the usage limit.
*/
public String usageLimitId() {
return usageLimitId;
}
/**
*
* The identifier of the cluster with a usage limit.
*
*
* @return The identifier of the cluster with a usage limit.
*/
public String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The Amazon Redshift feature to which the limit applies.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureType} will
* return {@link UsageLimitFeatureType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureTypeAsString}.
*
*
* @return The Amazon Redshift feature to which the limit applies.
* @see UsageLimitFeatureType
*/
public UsageLimitFeatureType featureType() {
return UsageLimitFeatureType.fromValue(featureType);
}
/**
*
* The Amazon Redshift feature to which the limit applies.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureType} will
* return {@link UsageLimitFeatureType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureTypeAsString}.
*
*
* @return The Amazon Redshift feature to which the limit applies.
* @see UsageLimitFeatureType
*/
public String featureTypeAsString() {
return featureType;
}
/**
*
* The type of limit. Depending on the feature type, this can be based on a time duration or data size.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #limitType} will
* return {@link UsageLimitLimitType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #limitTypeAsString}.
*
*
* @return The type of limit. Depending on the feature type, this can be based on a time duration or data size.
* @see UsageLimitLimitType
*/
public UsageLimitLimitType limitType() {
return UsageLimitLimitType.fromValue(limitType);
}
/**
*
* The type of limit. Depending on the feature type, this can be based on a time duration or data size.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #limitType} will
* return {@link UsageLimitLimitType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #limitTypeAsString}.
*
*
* @return The type of limit. Depending on the feature type, this can be based on a time duration or data size.
* @see UsageLimitLimitType
*/
public String limitTypeAsString() {
return limitType;
}
/**
*
* The limit amount. If time-based, this amount is in minutes. If data-based, this amount is in terabytes (TB).
*
*
* @return The limit amount. If time-based, this amount is in minutes. If data-based, this amount is in terabytes
* (TB).
*/
public Long amount() {
return amount;
}
/**
*
* The time period that the amount applies to. A weekly
period begins on Sunday. The default is
* monthly
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #period} will
* return {@link UsageLimitPeriod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #periodAsString}.
*
*
* @return The time period that the amount applies to. A weekly
period begins on Sunday. The default is
* monthly
.
* @see UsageLimitPeriod
*/
public UsageLimitPeriod period() {
return UsageLimitPeriod.fromValue(period);
}
/**
*
* The time period that the amount applies to. A weekly
period begins on Sunday. The default is
* monthly
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #period} will
* return {@link UsageLimitPeriod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #periodAsString}.
*
*
* @return The time period that the amount applies to. A weekly
period begins on Sunday. The default is
* monthly
.
* @see UsageLimitPeriod
*/
public String periodAsString() {
return period;
}
/**
*
* The action that Amazon Redshift takes when the limit is reached. Possible values are:
*
*
* -
*
* log - To log an event in a system table. The default is log.
*
*
* -
*
* emit-metric - To emit CloudWatch metrics.
*
*
* -
*
* disable - To disable the feature until the next usage period begins.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #breachAction} will
* return {@link UsageLimitBreachAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #breachActionAsString}.
*
*
* @return The action that Amazon Redshift takes when the limit is reached. Possible values are:
*
* -
*
* log - To log an event in a system table. The default is log.
*
*
* -
*
* emit-metric - To emit CloudWatch metrics.
*
*
* -
*
* disable - To disable the feature until the next usage period begins.
*
*
* @see UsageLimitBreachAction
*/
public UsageLimitBreachAction breachAction() {
return UsageLimitBreachAction.fromValue(breachAction);
}
/**
*
* The action that Amazon Redshift takes when the limit is reached. Possible values are:
*
*
* -
*
* log - To log an event in a system table. The default is log.
*
*
* -
*
* emit-metric - To emit CloudWatch metrics.
*
*
* -
*
* disable - To disable the feature until the next usage period begins.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #breachAction} will
* return {@link UsageLimitBreachAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #breachActionAsString}.
*
*
* @return The action that Amazon Redshift takes when the limit is reached. Possible values are:
*
* -
*
* log - To log an event in a system table. The default is log.
*
*
* -
*
* emit-metric - To emit CloudWatch metrics.
*
*
* -
*
* disable - To disable the feature until the next usage period begins.
*
*
* @see UsageLimitBreachAction
*/
public String breachActionAsString() {
return breachAction;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tag instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return A list of tag instances.
*/
public List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(usageLimitId());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(featureTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(limitTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(amount());
hashCode = 31 * hashCode + Objects.hashCode(periodAsString());
hashCode = 31 * hashCode + Objects.hashCode(breachActionAsString());
hashCode = 31 * hashCode + Objects.hashCode(tags());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyUsageLimitResponse)) {
return false;
}
ModifyUsageLimitResponse other = (ModifyUsageLimitResponse) obj;
return Objects.equals(usageLimitId(), other.usageLimitId())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(featureTypeAsString(), other.featureTypeAsString())
&& Objects.equals(limitTypeAsString(), other.limitTypeAsString()) && Objects.equals(amount(), other.amount())
&& Objects.equals(periodAsString(), other.periodAsString())
&& Objects.equals(breachActionAsString(), other.breachActionAsString()) && Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ModifyUsageLimitResponse").add("UsageLimitId", usageLimitId())
.add("ClusterIdentifier", clusterIdentifier()).add("FeatureType", featureTypeAsString())
.add("LimitType", limitTypeAsString()).add("Amount", amount()).add("Period", periodAsString())
.add("BreachAction", breachActionAsString()).add("Tags", tags()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UsageLimitId":
return Optional.ofNullable(clazz.cast(usageLimitId()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "FeatureType":
return Optional.ofNullable(clazz.cast(featureTypeAsString()));
case "LimitType":
return Optional.ofNullable(clazz.cast(limitTypeAsString()));
case "Amount":
return Optional.ofNullable(clazz.cast(amount()));
case "Period":
return Optional.ofNullable(clazz.cast(periodAsString()));
case "BreachAction":
return Optional.ofNullable(clazz.cast(breachActionAsString()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function