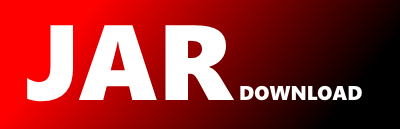
software.amazon.awssdk.services.redshift.model.ReservedNodeOffering Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a reserved node offering.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReservedNodeOffering implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField RESERVED_NODE_OFFERING_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReservedNodeOfferingId").getter(getter(ReservedNodeOffering::reservedNodeOfferingId))
.setter(setter(Builder::reservedNodeOfferingId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReservedNodeOfferingId").build())
.build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(ReservedNodeOffering::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Duration").getter(getter(ReservedNodeOffering::duration)).setter(setter(Builder::duration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Duration").build()).build();
private static final SdkField FIXED_PRICE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("FixedPrice").getter(getter(ReservedNodeOffering::fixedPrice)).setter(setter(Builder::fixedPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FixedPrice").build()).build();
private static final SdkField USAGE_PRICE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("UsagePrice").getter(getter(ReservedNodeOffering::usagePrice)).setter(setter(Builder::usagePrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UsagePrice").build()).build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CurrencyCode").getter(getter(ReservedNodeOffering::currencyCode)).setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrencyCode").build()).build();
private static final SdkField OFFERING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OfferingType").getter(getter(ReservedNodeOffering::offeringType)).setter(setter(Builder::offeringType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OfferingType").build()).build();
private static final SdkField> RECURRING_CHARGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RecurringCharges")
.getter(getter(ReservedNodeOffering::recurringCharges))
.setter(setter(Builder::recurringCharges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecurringCharges").build(),
ListTrait
.builder()
.memberLocationName("RecurringCharge")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RecurringCharge::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("RecurringCharge").build()).build()).build()).build();
private static final SdkField RESERVED_NODE_OFFERING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReservedNodeOfferingType").getter(getter(ReservedNodeOffering::reservedNodeOfferingTypeAsString))
.setter(setter(Builder::reservedNodeOfferingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReservedNodeOfferingType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
RESERVED_NODE_OFFERING_ID_FIELD, NODE_TYPE_FIELD, DURATION_FIELD, FIXED_PRICE_FIELD, USAGE_PRICE_FIELD,
CURRENCY_CODE_FIELD, OFFERING_TYPE_FIELD, RECURRING_CHARGES_FIELD, RESERVED_NODE_OFFERING_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String reservedNodeOfferingId;
private final String nodeType;
private final Integer duration;
private final Double fixedPrice;
private final Double usagePrice;
private final String currencyCode;
private final String offeringType;
private final List recurringCharges;
private final String reservedNodeOfferingType;
private ReservedNodeOffering(BuilderImpl builder) {
this.reservedNodeOfferingId = builder.reservedNodeOfferingId;
this.nodeType = builder.nodeType;
this.duration = builder.duration;
this.fixedPrice = builder.fixedPrice;
this.usagePrice = builder.usagePrice;
this.currencyCode = builder.currencyCode;
this.offeringType = builder.offeringType;
this.recurringCharges = builder.recurringCharges;
this.reservedNodeOfferingType = builder.reservedNodeOfferingType;
}
/**
*
* The offering identifier.
*
*
* @return The offering identifier.
*/
public final String reservedNodeOfferingId() {
return reservedNodeOfferingId;
}
/**
*
* The node type offered by the reserved node offering.
*
*
* @return The node type offered by the reserved node offering.
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The duration, in seconds, for which the offering will reserve the node.
*
*
* @return The duration, in seconds, for which the offering will reserve the node.
*/
public final Integer duration() {
return duration;
}
/**
*
* The upfront fixed charge you will pay to purchase the specific reserved node offering.
*
*
* @return The upfront fixed charge you will pay to purchase the specific reserved node offering.
*/
public final Double fixedPrice() {
return fixedPrice;
}
/**
*
* The rate you are charged for each hour the cluster that is using the offering is running.
*
*
* @return The rate you are charged for each hour the cluster that is using the offering is running.
*/
public final Double usagePrice() {
return usagePrice;
}
/**
*
* The currency code for the compute nodes offering.
*
*
* @return The currency code for the compute nodes offering.
*/
public final String currencyCode() {
return currencyCode;
}
/**
*
* The anticipated utilization of the reserved node, as defined in the reserved node offering.
*
*
* @return The anticipated utilization of the reserved node, as defined in the reserved node offering.
*/
public final String offeringType() {
return offeringType;
}
/**
* Returns true if the RecurringCharges property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasRecurringCharges() {
return recurringCharges != null && !(recurringCharges instanceof SdkAutoConstructList);
}
/**
*
* The charge to your account regardless of whether you are creating any clusters using the node offering. Recurring
* charges are only in effect for heavy-utilization reserved nodes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasRecurringCharges()} to see if a value was sent in this field.
*
*
* @return The charge to your account regardless of whether you are creating any clusters using the node offering.
* Recurring charges are only in effect for heavy-utilization reserved nodes.
*/
public final List recurringCharges() {
return recurringCharges;
}
/**
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #reservedNodeOfferingType} will return {@link ReservedNodeOfferingType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #reservedNodeOfferingTypeAsString}.
*
*
* @return
* @see ReservedNodeOfferingType
*/
public final ReservedNodeOfferingType reservedNodeOfferingType() {
return ReservedNodeOfferingType.fromValue(reservedNodeOfferingType);
}
/**
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #reservedNodeOfferingType} will return {@link ReservedNodeOfferingType#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #reservedNodeOfferingTypeAsString}.
*
*
* @return
* @see ReservedNodeOfferingType
*/
public final String reservedNodeOfferingTypeAsString() {
return reservedNodeOfferingType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(reservedNodeOfferingId());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(duration());
hashCode = 31 * hashCode + Objects.hashCode(fixedPrice());
hashCode = 31 * hashCode + Objects.hashCode(usagePrice());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(offeringType());
hashCode = 31 * hashCode + Objects.hashCode(hasRecurringCharges() ? recurringCharges() : null);
hashCode = 31 * hashCode + Objects.hashCode(reservedNodeOfferingTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReservedNodeOffering)) {
return false;
}
ReservedNodeOffering other = (ReservedNodeOffering) obj;
return Objects.equals(reservedNodeOfferingId(), other.reservedNodeOfferingId())
&& Objects.equals(nodeType(), other.nodeType()) && Objects.equals(duration(), other.duration())
&& Objects.equals(fixedPrice(), other.fixedPrice()) && Objects.equals(usagePrice(), other.usagePrice())
&& Objects.equals(currencyCode(), other.currencyCode()) && Objects.equals(offeringType(), other.offeringType())
&& hasRecurringCharges() == other.hasRecurringCharges()
&& Objects.equals(recurringCharges(), other.recurringCharges())
&& Objects.equals(reservedNodeOfferingTypeAsString(), other.reservedNodeOfferingTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReservedNodeOffering").add("ReservedNodeOfferingId", reservedNodeOfferingId())
.add("NodeType", nodeType()).add("Duration", duration()).add("FixedPrice", fixedPrice())
.add("UsagePrice", usagePrice()).add("CurrencyCode", currencyCode()).add("OfferingType", offeringType())
.add("RecurringCharges", hasRecurringCharges() ? recurringCharges() : null)
.add("ReservedNodeOfferingType", reservedNodeOfferingTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReservedNodeOfferingId":
return Optional.ofNullable(clazz.cast(reservedNodeOfferingId()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "Duration":
return Optional.ofNullable(clazz.cast(duration()));
case "FixedPrice":
return Optional.ofNullable(clazz.cast(fixedPrice()));
case "UsagePrice":
return Optional.ofNullable(clazz.cast(usagePrice()));
case "CurrencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "OfferingType":
return Optional.ofNullable(clazz.cast(offeringType()));
case "RecurringCharges":
return Optional.ofNullable(clazz.cast(recurringCharges()));
case "ReservedNodeOfferingType":
return Optional.ofNullable(clazz.cast(reservedNodeOfferingTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function