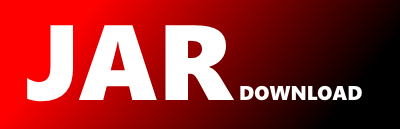
software.amazon.awssdk.services.redshift.model.TableRestoreStatus Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the status of a RestoreTableFromClusterSnapshot operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TableRestoreStatus implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TABLE_RESTORE_REQUEST_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TableRestoreRequestId").getter(getter(TableRestoreStatus::tableRestoreRequestId))
.setter(setter(Builder::tableRestoreRequestId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableRestoreRequestId").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(TableRestoreStatus::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Message")
.getter(getter(TableRestoreStatus::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField REQUEST_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("RequestTime").getter(getter(TableRestoreStatus::requestTime)).setter(setter(Builder::requestTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestTime").build()).build();
private static final SdkField PROGRESS_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ProgressInMegaBytes").getter(getter(TableRestoreStatus::progressInMegaBytes))
.setter(setter(Builder::progressInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgressInMegaBytes").build())
.build();
private static final SdkField TOTAL_DATA_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("TotalDataInMegaBytes").getter(getter(TableRestoreStatus::totalDataInMegaBytes))
.setter(setter(Builder::totalDataInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalDataInMegaBytes").build())
.build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(TableRestoreStatus::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField SNAPSHOT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotIdentifier").getter(getter(TableRestoreStatus::snapshotIdentifier))
.setter(setter(Builder::snapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotIdentifier").build())
.build();
private static final SdkField SOURCE_DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceDatabaseName").getter(getter(TableRestoreStatus::sourceDatabaseName))
.setter(setter(Builder::sourceDatabaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceDatabaseName").build())
.build();
private static final SdkField SOURCE_SCHEMA_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceSchemaName").getter(getter(TableRestoreStatus::sourceSchemaName))
.setter(setter(Builder::sourceSchemaName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceSchemaName").build()).build();
private static final SdkField SOURCE_TABLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceTableName").getter(getter(TableRestoreStatus::sourceTableName))
.setter(setter(Builder::sourceTableName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceTableName").build()).build();
private static final SdkField TARGET_DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetDatabaseName").getter(getter(TableRestoreStatus::targetDatabaseName))
.setter(setter(Builder::targetDatabaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetDatabaseName").build())
.build();
private static final SdkField TARGET_SCHEMA_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetSchemaName").getter(getter(TableRestoreStatus::targetSchemaName))
.setter(setter(Builder::targetSchemaName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetSchemaName").build()).build();
private static final SdkField NEW_TABLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewTableName").getter(getter(TableRestoreStatus::newTableName)).setter(setter(Builder::newTableName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewTableName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
TABLE_RESTORE_REQUEST_ID_FIELD, STATUS_FIELD, MESSAGE_FIELD, REQUEST_TIME_FIELD, PROGRESS_IN_MEGA_BYTES_FIELD,
TOTAL_DATA_IN_MEGA_BYTES_FIELD, CLUSTER_IDENTIFIER_FIELD, SNAPSHOT_IDENTIFIER_FIELD, SOURCE_DATABASE_NAME_FIELD,
SOURCE_SCHEMA_NAME_FIELD, SOURCE_TABLE_NAME_FIELD, TARGET_DATABASE_NAME_FIELD, TARGET_SCHEMA_NAME_FIELD,
NEW_TABLE_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final String tableRestoreRequestId;
private final String status;
private final String message;
private final Instant requestTime;
private final Long progressInMegaBytes;
private final Long totalDataInMegaBytes;
private final String clusterIdentifier;
private final String snapshotIdentifier;
private final String sourceDatabaseName;
private final String sourceSchemaName;
private final String sourceTableName;
private final String targetDatabaseName;
private final String targetSchemaName;
private final String newTableName;
private TableRestoreStatus(BuilderImpl builder) {
this.tableRestoreRequestId = builder.tableRestoreRequestId;
this.status = builder.status;
this.message = builder.message;
this.requestTime = builder.requestTime;
this.progressInMegaBytes = builder.progressInMegaBytes;
this.totalDataInMegaBytes = builder.totalDataInMegaBytes;
this.clusterIdentifier = builder.clusterIdentifier;
this.snapshotIdentifier = builder.snapshotIdentifier;
this.sourceDatabaseName = builder.sourceDatabaseName;
this.sourceSchemaName = builder.sourceSchemaName;
this.sourceTableName = builder.sourceTableName;
this.targetDatabaseName = builder.targetDatabaseName;
this.targetSchemaName = builder.targetSchemaName;
this.newTableName = builder.newTableName;
}
/**
*
* The unique identifier for the table restore request.
*
*
* @return The unique identifier for the table restore request.
*/
public final String tableRestoreRequestId() {
return tableRestoreRequestId;
}
/**
*
* A value that describes the current state of the table restore request.
*
*
* Valid Values: SUCCEEDED
, FAILED
, CANCELED
, PENDING
,
* IN_PROGRESS
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TableRestoreStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return A value that describes the current state of the table restore request.
*
* Valid Values: SUCCEEDED
, FAILED
, CANCELED
, PENDING
,
* IN_PROGRESS
* @see TableRestoreStatusType
*/
public final TableRestoreStatusType status() {
return TableRestoreStatusType.fromValue(status);
}
/**
*
* A value that describes the current state of the table restore request.
*
*
* Valid Values: SUCCEEDED
, FAILED
, CANCELED
, PENDING
,
* IN_PROGRESS
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TableRestoreStatusType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return A value that describes the current state of the table restore request.
*
* Valid Values: SUCCEEDED
, FAILED
, CANCELED
, PENDING
,
* IN_PROGRESS
* @see TableRestoreStatusType
*/
public final String statusAsString() {
return status;
}
/**
*
* A description of the status of the table restore request. Status values include SUCCEEDED
,
* FAILED
, CANCELED
, PENDING
, IN_PROGRESS
.
*
*
* @return A description of the status of the table restore request. Status values include SUCCEEDED
,
* FAILED
, CANCELED
, PENDING
, IN_PROGRESS
.
*/
public final String message() {
return message;
}
/**
*
* The time that the table restore request was made, in Universal Coordinated Time (UTC).
*
*
* @return The time that the table restore request was made, in Universal Coordinated Time (UTC).
*/
public final Instant requestTime() {
return requestTime;
}
/**
*
* The amount of data restored to the new table so far, in megabytes (MB).
*
*
* @return The amount of data restored to the new table so far, in megabytes (MB).
*/
public final Long progressInMegaBytes() {
return progressInMegaBytes;
}
/**
*
* The total amount of data to restore to the new table, in megabytes (MB).
*
*
* @return The total amount of data to restore to the new table, in megabytes (MB).
*/
public final Long totalDataInMegaBytes() {
return totalDataInMegaBytes;
}
/**
*
* The identifier of the Amazon Redshift cluster that the table is being restored to.
*
*
* @return The identifier of the Amazon Redshift cluster that the table is being restored to.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The identifier of the snapshot that the table is being restored from.
*
*
* @return The identifier of the snapshot that the table is being restored from.
*/
public final String snapshotIdentifier() {
return snapshotIdentifier;
}
/**
*
* The name of the source database that contains the table being restored.
*
*
* @return The name of the source database that contains the table being restored.
*/
public final String sourceDatabaseName() {
return sourceDatabaseName;
}
/**
*
* The name of the source schema that contains the table being restored.
*
*
* @return The name of the source schema that contains the table being restored.
*/
public final String sourceSchemaName() {
return sourceSchemaName;
}
/**
*
* The name of the source table being restored.
*
*
* @return The name of the source table being restored.
*/
public final String sourceTableName() {
return sourceTableName;
}
/**
*
* The name of the database to restore the table to.
*
*
* @return The name of the database to restore the table to.
*/
public final String targetDatabaseName() {
return targetDatabaseName;
}
/**
*
* The name of the schema to restore the table to.
*
*
* @return The name of the schema to restore the table to.
*/
public final String targetSchemaName() {
return targetSchemaName;
}
/**
*
* The name of the table to create as a result of the table restore request.
*
*
* @return The name of the table to create as a result of the table restore request.
*/
public final String newTableName() {
return newTableName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(tableRestoreRequestId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(requestTime());
hashCode = 31 * hashCode + Objects.hashCode(progressInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(totalDataInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(snapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceDatabaseName());
hashCode = 31 * hashCode + Objects.hashCode(sourceSchemaName());
hashCode = 31 * hashCode + Objects.hashCode(sourceTableName());
hashCode = 31 * hashCode + Objects.hashCode(targetDatabaseName());
hashCode = 31 * hashCode + Objects.hashCode(targetSchemaName());
hashCode = 31 * hashCode + Objects.hashCode(newTableName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TableRestoreStatus)) {
return false;
}
TableRestoreStatus other = (TableRestoreStatus) obj;
return Objects.equals(tableRestoreRequestId(), other.tableRestoreRequestId())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(message(), other.message())
&& Objects.equals(requestTime(), other.requestTime())
&& Objects.equals(progressInMegaBytes(), other.progressInMegaBytes())
&& Objects.equals(totalDataInMegaBytes(), other.totalDataInMegaBytes())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(snapshotIdentifier(), other.snapshotIdentifier())
&& Objects.equals(sourceDatabaseName(), other.sourceDatabaseName())
&& Objects.equals(sourceSchemaName(), other.sourceSchemaName())
&& Objects.equals(sourceTableName(), other.sourceTableName())
&& Objects.equals(targetDatabaseName(), other.targetDatabaseName())
&& Objects.equals(targetSchemaName(), other.targetSchemaName())
&& Objects.equals(newTableName(), other.newTableName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TableRestoreStatus").add("TableRestoreRequestId", tableRestoreRequestId())
.add("Status", statusAsString()).add("Message", message()).add("RequestTime", requestTime())
.add("ProgressInMegaBytes", progressInMegaBytes()).add("TotalDataInMegaBytes", totalDataInMegaBytes())
.add("ClusterIdentifier", clusterIdentifier()).add("SnapshotIdentifier", snapshotIdentifier())
.add("SourceDatabaseName", sourceDatabaseName()).add("SourceSchemaName", sourceSchemaName())
.add("SourceTableName", sourceTableName()).add("TargetDatabaseName", targetDatabaseName())
.add("TargetSchemaName", targetSchemaName()).add("NewTableName", newTableName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TableRestoreRequestId":
return Optional.ofNullable(clazz.cast(tableRestoreRequestId()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "RequestTime":
return Optional.ofNullable(clazz.cast(requestTime()));
case "ProgressInMegaBytes":
return Optional.ofNullable(clazz.cast(progressInMegaBytes()));
case "TotalDataInMegaBytes":
return Optional.ofNullable(clazz.cast(totalDataInMegaBytes()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "SnapshotIdentifier":
return Optional.ofNullable(clazz.cast(snapshotIdentifier()));
case "SourceDatabaseName":
return Optional.ofNullable(clazz.cast(sourceDatabaseName()));
case "SourceSchemaName":
return Optional.ofNullable(clazz.cast(sourceSchemaName()));
case "SourceTableName":
return Optional.ofNullable(clazz.cast(sourceTableName()));
case "TargetDatabaseName":
return Optional.ofNullable(clazz.cast(targetDatabaseName()));
case "TargetSchemaName":
return Optional.ofNullable(clazz.cast(targetSchemaName()));
case "NewTableName":
return Optional.ofNullable(clazz.cast(newTableName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function