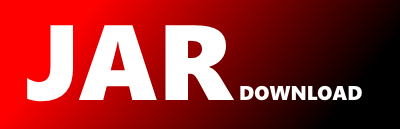
software.amazon.awssdk.services.redshift.model.ModifyClusterRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyClusterRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(ModifyClusterRequest::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterType").getter(getter(ModifyClusterRequest::clusterType)).setter(setter(Builder::clusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterType").build()).build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(ModifyClusterRequest::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfNodes").getter(getter(ModifyClusterRequest::numberOfNodes))
.setter(setter(Builder::numberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNodes").build()).build();
private static final SdkField> CLUSTER_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ClusterSecurityGroups")
.getter(getter(ModifyClusterRequest::clusterSecurityGroups))
.setter(setter(Builder::clusterSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("ClusterSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ClusterSecurityGroupName").build()).build()).build()).build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroupIds")
.getter(getter(ModifyClusterRequest::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroupId").build()).build()).build()).build();
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(ModifyClusterRequest::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField CLUSTER_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterParameterGroupName").getter(getter(ModifyClusterRequest::clusterParameterGroupName))
.setter(setter(Builder::clusterParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterParameterGroupName").build())
.build();
private static final SdkField AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AutomatedSnapshotRetentionPeriod")
.getter(getter(ModifyClusterRequest::automatedSnapshotRetentionPeriod))
.setter(setter(Builder::automatedSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomatedSnapshotRetentionPeriod")
.build()).build();
private static final SdkField MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ManualSnapshotRetentionPeriod")
.getter(getter(ModifyClusterRequest::manualSnapshotRetentionPeriod))
.setter(setter(Builder::manualSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManualSnapshotRetentionPeriod")
.build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField CLUSTER_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterVersion").getter(getter(ModifyClusterRequest::clusterVersion))
.setter(setter(Builder::clusterVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterVersion").build()).build();
private static final SdkField ALLOW_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AllowVersionUpgrade").getter(getter(ModifyClusterRequest::allowVersionUpgrade))
.setter(setter(Builder::allowVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowVersionUpgrade").build())
.build();
private static final SdkField HSM_CLIENT_CERTIFICATE_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HsmClientCertificateIdentifier")
.getter(getter(ModifyClusterRequest::hsmClientCertificateIdentifier))
.setter(setter(Builder::hsmClientCertificateIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HsmClientCertificateIdentifier")
.build()).build();
private static final SdkField HSM_CONFIGURATION_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HsmConfigurationIdentifier")
.getter(getter(ModifyClusterRequest::hsmConfigurationIdentifier))
.setter(setter(Builder::hsmConfigurationIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HsmConfigurationIdentifier").build())
.build();
private static final SdkField NEW_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewClusterIdentifier").getter(getter(ModifyClusterRequest::newClusterIdentifier))
.setter(setter(Builder::newClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewClusterIdentifier").build())
.build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("PubliclyAccessible").getter(getter(ModifyClusterRequest::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PubliclyAccessible").build())
.build();
private static final SdkField ELASTIC_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ElasticIp").getter(getter(ModifyClusterRequest::elasticIp)).setter(setter(Builder::elasticIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElasticIp").build()).build();
private static final SdkField ENHANCED_VPC_ROUTING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnhancedVpcRouting").getter(getter(ModifyClusterRequest::enhancedVpcRouting))
.setter(setter(Builder::enhancedVpcRouting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnhancedVpcRouting").build())
.build();
private static final SdkField MAINTENANCE_TRACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaintenanceTrackName").getter(getter(ModifyClusterRequest::maintenanceTrackName))
.setter(setter(Builder::maintenanceTrackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaintenanceTrackName").build())
.build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted").getter(getter(ModifyClusterRequest::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(ModifyClusterRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField AVAILABILITY_ZONE_RELOCATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AvailabilityZoneRelocation")
.getter(getter(ModifyClusterRequest::availabilityZoneRelocation))
.setter(setter(Builder::availabilityZoneRelocation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZoneRelocation").build())
.build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(ModifyClusterRequest::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(ModifyClusterRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_IDENTIFIER_FIELD,
CLUSTER_TYPE_FIELD, NODE_TYPE_FIELD, NUMBER_OF_NODES_FIELD, CLUSTER_SECURITY_GROUPS_FIELD,
VPC_SECURITY_GROUP_IDS_FIELD, MASTER_USER_PASSWORD_FIELD, CLUSTER_PARAMETER_GROUP_NAME_FIELD,
AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD, MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD,
PREFERRED_MAINTENANCE_WINDOW_FIELD, CLUSTER_VERSION_FIELD, ALLOW_VERSION_UPGRADE_FIELD,
HSM_CLIENT_CERTIFICATE_IDENTIFIER_FIELD, HSM_CONFIGURATION_IDENTIFIER_FIELD, NEW_CLUSTER_IDENTIFIER_FIELD,
PUBLICLY_ACCESSIBLE_FIELD, ELASTIC_IP_FIELD, ENHANCED_VPC_ROUTING_FIELD, MAINTENANCE_TRACK_NAME_FIELD,
ENCRYPTED_FIELD, KMS_KEY_ID_FIELD, AVAILABILITY_ZONE_RELOCATION_FIELD, AVAILABILITY_ZONE_FIELD, PORT_FIELD));
private final String clusterIdentifier;
private final String clusterType;
private final String nodeType;
private final Integer numberOfNodes;
private final List clusterSecurityGroups;
private final List vpcSecurityGroupIds;
private final String masterUserPassword;
private final String clusterParameterGroupName;
private final Integer automatedSnapshotRetentionPeriod;
private final Integer manualSnapshotRetentionPeriod;
private final String preferredMaintenanceWindow;
private final String clusterVersion;
private final Boolean allowVersionUpgrade;
private final String hsmClientCertificateIdentifier;
private final String hsmConfigurationIdentifier;
private final String newClusterIdentifier;
private final Boolean publiclyAccessible;
private final String elasticIp;
private final Boolean enhancedVpcRouting;
private final String maintenanceTrackName;
private final Boolean encrypted;
private final String kmsKeyId;
private final Boolean availabilityZoneRelocation;
private final String availabilityZone;
private final Integer port;
private ModifyClusterRequest(BuilderImpl builder) {
super(builder);
this.clusterIdentifier = builder.clusterIdentifier;
this.clusterType = builder.clusterType;
this.nodeType = builder.nodeType;
this.numberOfNodes = builder.numberOfNodes;
this.clusterSecurityGroups = builder.clusterSecurityGroups;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.masterUserPassword = builder.masterUserPassword;
this.clusterParameterGroupName = builder.clusterParameterGroupName;
this.automatedSnapshotRetentionPeriod = builder.automatedSnapshotRetentionPeriod;
this.manualSnapshotRetentionPeriod = builder.manualSnapshotRetentionPeriod;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.clusterVersion = builder.clusterVersion;
this.allowVersionUpgrade = builder.allowVersionUpgrade;
this.hsmClientCertificateIdentifier = builder.hsmClientCertificateIdentifier;
this.hsmConfigurationIdentifier = builder.hsmConfigurationIdentifier;
this.newClusterIdentifier = builder.newClusterIdentifier;
this.publiclyAccessible = builder.publiclyAccessible;
this.elasticIp = builder.elasticIp;
this.enhancedVpcRouting = builder.enhancedVpcRouting;
this.maintenanceTrackName = builder.maintenanceTrackName;
this.encrypted = builder.encrypted;
this.kmsKeyId = builder.kmsKeyId;
this.availabilityZoneRelocation = builder.availabilityZoneRelocation;
this.availabilityZone = builder.availabilityZone;
this.port = builder.port;
}
/**
*
* The unique identifier of the cluster to be modified.
*
*
* Example: examplecluster
*
*
* @return The unique identifier of the cluster to be modified.
*
* Example: examplecluster
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The new cluster type.
*
*
* When you submit your cluster resize request, your existing cluster goes into a read-only mode. After Amazon
* Redshift provisions a new cluster based on your resize requirements, there will be outage for a period while the
* old cluster is deleted and your connection is switched to the new cluster. You can use DescribeResize to
* track the progress of the resize request.
*
*
* Valid Values: multi-node | single-node
*
*
* @return The new cluster type.
*
* When you submit your cluster resize request, your existing cluster goes into a read-only mode. After
* Amazon Redshift provisions a new cluster based on your resize requirements, there will be outage for a
* period while the old cluster is deleted and your connection is switched to the new cluster. You can use
* DescribeResize to track the progress of the resize request.
*
*
* Valid Values: multi-node | single-node
*/
public final String clusterType() {
return clusterType;
}
/**
*
* The new node type of the cluster. If you specify a new node type, you must also specify the number of nodes
* parameter.
*
*
* For more information about resizing clusters, go to Resizing Clusters in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: ds2.xlarge
| ds2.8xlarge
| dc1.large
|
* dc1.8xlarge
| dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
*
*
* @return The new node type of the cluster. If you specify a new node type, you must also specify the number of
* nodes parameter.
*
* For more information about resizing clusters, go to Resizing Clusters in
* Amazon Redshift in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: ds2.xlarge
| ds2.8xlarge
| dc1.large
|
* dc1.8xlarge
| dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The new number of nodes of the cluster. If you specify a new number of nodes, you must also specify the node type
* parameter.
*
*
* For more information about resizing clusters, go to Resizing Clusters in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: Integer greater than 0
.
*
*
* @return The new number of nodes of the cluster. If you specify a new number of nodes, you must also specify the
* node type parameter.
*
* For more information about resizing clusters, go to Resizing Clusters in
* Amazon Redshift in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: Integer greater than 0
.
*/
public final Integer numberOfNodes() {
return numberOfNodes;
}
/**
* Returns true if the ClusterSecurityGroups property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasClusterSecurityGroups() {
return clusterSecurityGroups != null && !(clusterSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of cluster security groups to be authorized on this cluster. This change is asynchronously applied as soon
* as possible.
*
*
* Security groups currently associated with the cluster, and not in the list of groups to apply, will be revoked
* from the cluster.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasClusterSecurityGroups()} to see if a value was sent in this field.
*
*
* @return A list of cluster security groups to be authorized on this cluster. This change is asynchronously applied
* as soon as possible.
*
* Security groups currently associated with the cluster, and not in the list of groups to apply, will be
* revoked from the cluster.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*/
public final List clusterSecurityGroups() {
return clusterSecurityGroups;
}
/**
* Returns true if the VpcSecurityGroupIds property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of virtual private cloud (VPC) security groups to be associated with the cluster. This change is
* asynchronously applied as soon as possible.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasVpcSecurityGroupIds()} to see if a value was sent in this field.
*
*
* @return A list of virtual private cloud (VPC) security groups to be associated with the cluster. This change is
* asynchronously applied as soon as possible.
*/
public final List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The new password for the cluster master user. This change is asynchronously applied as soon as possible. Between
* the time of the request and the completion of the request, the MasterUserPassword
element exists in
* the PendingModifiedValues
element of the operation response.
*
*
*
* Operations never return the password, so this operation provides a way to regain access to the master user
* account for a cluster if the password is lost.
*
*
*
* Default: Uses existing setting.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33 to 126) except ' (single quote), " (double quote), \, /, @,
* or space.
*
*
*
*
* @return The new password for the cluster master user. This change is asynchronously applied as soon as possible.
* Between the time of the request and the completion of the request, the MasterUserPassword
* element exists in the PendingModifiedValues
element of the operation response.
*
* Operations never return the password, so this operation provides a way to regain access to the master
* user account for a cluster if the password is lost.
*
*
*
* Default: Uses existing setting.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33 to 126) except ' (single quote), " (double quote), \,
* /, @, or space.
*
*
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
*
* The name of the cluster parameter group to apply to this cluster. This change is applied only after the cluster
* is rebooted. To reboot a cluster use RebootCluster.
*
*
* Default: Uses existing setting.
*
*
* Constraints: The cluster parameter group must be in the same parameter group family that matches the cluster
* version.
*
*
* @return The name of the cluster parameter group to apply to this cluster. This change is applied only after the
* cluster is rebooted. To reboot a cluster use RebootCluster.
*
* Default: Uses existing setting.
*
*
* Constraints: The cluster parameter group must be in the same parameter group family that matches the
* cluster version.
*/
public final String clusterParameterGroupName() {
return clusterParameterGroupName;
}
/**
*
* The number of days that automated snapshots are retained. If the value is 0, automated snapshots are disabled.
* Even if automated snapshots are disabled, you can still create manual snapshots when you want with
* CreateClusterSnapshot.
*
*
* If you decrease the automated snapshot retention period from its current value, existing automated snapshots that
* fall outside of the new retention period will be immediately deleted.
*
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35 days.
*
*
* Default: Uses existing setting.
*
*
* Constraints: Must be a value from 0 to 35.
*
*
* @return The number of days that automated snapshots are retained. If the value is 0, automated snapshots are
* disabled. Even if automated snapshots are disabled, you can still create manual snapshots when you want
* with CreateClusterSnapshot.
*
* If you decrease the automated snapshot retention period from its current value, existing automated
* snapshots that fall outside of the new retention period will be immediately deleted.
*
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35
* days.
*
*
* Default: Uses existing setting.
*
*
* Constraints: Must be a value from 0 to 35.
*/
public final Integer automatedSnapshotRetentionPeriod() {
return automatedSnapshotRetentionPeriod;
}
/**
*
* The default for number of days that a newly created manual snapshot is retained. If the value is -1, the manual
* snapshot is retained indefinitely. This value doesn't retroactively change the retention periods of existing
* manual snapshots.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
*
*
* @return The default for number of days that a newly created manual snapshot is retained. If the value is -1, the
* manual snapshot is retained indefinitely. This value doesn't retroactively change the retention periods
* of existing manual snapshots.
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
*/
public final Integer manualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
/**
*
* The weekly time range (in UTC) during which system maintenance can occur, if necessary. If system maintenance is
* necessary during the window, it may result in an outage.
*
*
* This maintenance window change is made immediately. If the new maintenance window indicates the current time,
* there must be at least 120 minutes between the current time and end of the window in order to ensure that pending
* changes are applied.
*
*
* Default: Uses existing setting.
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi, for example wed:07:30-wed:08:00
.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes.
*
*
* @return The weekly time range (in UTC) during which system maintenance can occur, if necessary. If system
* maintenance is necessary during the window, it may result in an outage.
*
* This maintenance window change is made immediately. If the new maintenance window indicates the current
* time, there must be at least 120 minutes between the current time and end of the window in order to
* ensure that pending changes are applied.
*
*
* Default: Uses existing setting.
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi, for example wed:07:30-wed:08:00
.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes.
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The new version number of the Amazon Redshift engine to upgrade to.
*
*
* For major version upgrades, if a non-default cluster parameter group is currently in use, a new cluster parameter
* group in the cluster parameter group family for the new version must be specified. The new cluster parameter
* group can be the default for that cluster parameter group family. For more information about parameters and
* parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* Example: 1.0
*
*
* @return The new version number of the Amazon Redshift engine to upgrade to.
*
* For major version upgrades, if a non-default cluster parameter group is currently in use, a new cluster
* parameter group in the cluster parameter group family for the new version must be specified. The new
* cluster parameter group can be the default for that cluster parameter group family. For more information
* about parameters and parameter groups, go to Amazon
* Redshift Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* Example: 1.0
*/
public final String clusterVersion() {
return clusterVersion;
}
/**
*
* If true
, major version upgrades will be applied automatically to the cluster during the maintenance
* window.
*
*
* Default: false
*
*
* @return If true
, major version upgrades will be applied automatically to the cluster during the
* maintenance window.
*
* Default: false
*/
public final Boolean allowVersionUpgrade() {
return allowVersionUpgrade;
}
/**
*
* Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data encryption
* keys stored in an HSM.
*
*
* @return Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data
* encryption keys stored in an HSM.
*/
public final String hsmClientCertificateIdentifier() {
return hsmClientCertificateIdentifier;
}
/**
*
* Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can use to
* retrieve and store keys in an HSM.
*
*
* @return Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can
* use to retrieve and store keys in an HSM.
*/
public final String hsmConfigurationIdentifier() {
return hsmConfigurationIdentifier;
}
/**
*
* The new identifier for the cluster.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an AWS account.
*
*
*
*
* Example: examplecluster
*
*
* @return The new identifier for the cluster.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an AWS account.
*
*
*
*
* Example: examplecluster
*/
public final String newClusterIdentifier() {
return newClusterIdentifier;
}
/**
*
* If true
, the cluster can be accessed from a public network. Only clusters in VPCs can be set to be
* publicly available.
*
*
* @return If true
, the cluster can be accessed from a public network. Only clusters in VPCs can be set
* to be publicly available.
*/
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
*
* The Elastic IP (EIP) address for the cluster.
*
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet gateway. For
* more information about provisioning clusters in EC2-VPC, go to Supported
* Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
*
*
* @return The Elastic IP (EIP) address for the cluster.
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet
* gateway. For more information about provisioning clusters in EC2-VPC, go to Supported Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
*/
public final String elasticIp() {
return elasticIp;
}
/**
*
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster
* that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the
* Amazon Redshift Cluster Management Guide.
*
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*
*
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a
* cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC
* Routing in the Amazon Redshift Cluster Management Guide.
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*/
public final Boolean enhancedVpcRouting() {
return enhancedVpcRouting;
}
/**
*
* The name for the maintenance track that you want to assign for the cluster. This name change is asynchronous. The
* new track name stays in the PendingModifiedValues
for the cluster until the next maintenance window.
* When the maintenance track changes, the cluster is switched to the latest cluster release available for the
* maintenance track. At this point, the maintenance track name is applied.
*
*
* @return The name for the maintenance track that you want to assign for the cluster. This name change is
* asynchronous. The new track name stays in the PendingModifiedValues
for the cluster until
* the next maintenance window. When the maintenance track changes, the cluster is switched to the latest
* cluster release available for the maintenance track. At this point, the maintenance track name is
* applied.
*/
public final String maintenanceTrackName() {
return maintenanceTrackName;
}
/**
*
* Indicates whether the cluster is encrypted. If the value is encrypted (true) and you provide a value for the
* KmsKeyId
parameter, we encrypt the cluster with the provided KmsKeyId
. If you don't
* provide a KmsKeyId
, we encrypt with the default key.
*
*
* If the value is not encrypted (false), then the cluster is decrypted.
*
*
* @return Indicates whether the cluster is encrypted. If the value is encrypted (true) and you provide a value for
* the KmsKeyId
parameter, we encrypt the cluster with the provided KmsKeyId
. If
* you don't provide a KmsKeyId
, we encrypt with the default key.
*
* If the value is not encrypted (false), then the cluster is decrypted.
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* The AWS Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the
* cluster.
*
*
* @return The AWS Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in
* the cluster.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster
* modification is complete.
*
*
* @return The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the
* cluster modification is complete.
*/
public final Boolean availabilityZoneRelocation() {
return availabilityZoneRelocation;
}
/**
*
* The option to initiate relocation for an Amazon Redshift cluster to the target Availability Zone.
*
*
* @return The option to initiate relocation for an Amazon Redshift cluster to the target Availability Zone.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The option to change the port of an Amazon Redshift cluster.
*
*
* @return The option to change the port of an Amazon Redshift cluster.
*/
public final Integer port() {
return port;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(clusterType());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(hasClusterSecurityGroups() ? clusterSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(clusterParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(automatedSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(manualSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(clusterVersion());
hashCode = 31 * hashCode + Objects.hashCode(allowVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(hsmClientCertificateIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(hsmConfigurationIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(newClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(elasticIp());
hashCode = 31 * hashCode + Objects.hashCode(enhancedVpcRouting());
hashCode = 31 * hashCode + Objects.hashCode(maintenanceTrackName());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZoneRelocation());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(port());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyClusterRequest)) {
return false;
}
ModifyClusterRequest other = (ModifyClusterRequest) obj;
return Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(clusterType(), other.clusterType()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(numberOfNodes(), other.numberOfNodes())
&& hasClusterSecurityGroups() == other.hasClusterSecurityGroups()
&& Objects.equals(clusterSecurityGroups(), other.clusterSecurityGroups())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(masterUserPassword(), other.masterUserPassword())
&& Objects.equals(clusterParameterGroupName(), other.clusterParameterGroupName())
&& Objects.equals(automatedSnapshotRetentionPeriod(), other.automatedSnapshotRetentionPeriod())
&& Objects.equals(manualSnapshotRetentionPeriod(), other.manualSnapshotRetentionPeriod())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(clusterVersion(), other.clusterVersion())
&& Objects.equals(allowVersionUpgrade(), other.allowVersionUpgrade())
&& Objects.equals(hsmClientCertificateIdentifier(), other.hsmClientCertificateIdentifier())
&& Objects.equals(hsmConfigurationIdentifier(), other.hsmConfigurationIdentifier())
&& Objects.equals(newClusterIdentifier(), other.newClusterIdentifier())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible())
&& Objects.equals(elasticIp(), other.elasticIp())
&& Objects.equals(enhancedVpcRouting(), other.enhancedVpcRouting())
&& Objects.equals(maintenanceTrackName(), other.maintenanceTrackName())
&& Objects.equals(encrypted(), other.encrypted()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(availabilityZoneRelocation(), other.availabilityZoneRelocation())
&& Objects.equals(availabilityZone(), other.availabilityZone()) && Objects.equals(port(), other.port());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyClusterRequest").add("ClusterIdentifier", clusterIdentifier())
.add("ClusterType", clusterType()).add("NodeType", nodeType()).add("NumberOfNodes", numberOfNodes())
.add("ClusterSecurityGroups", hasClusterSecurityGroups() ? clusterSecurityGroups() : null)
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null)
.add("MasterUserPassword", masterUserPassword()).add("ClusterParameterGroupName", clusterParameterGroupName())
.add("AutomatedSnapshotRetentionPeriod", automatedSnapshotRetentionPeriod())
.add("ManualSnapshotRetentionPeriod", manualSnapshotRetentionPeriod())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("ClusterVersion", clusterVersion())
.add("AllowVersionUpgrade", allowVersionUpgrade())
.add("HsmClientCertificateIdentifier", hsmClientCertificateIdentifier())
.add("HsmConfigurationIdentifier", hsmConfigurationIdentifier())
.add("NewClusterIdentifier", newClusterIdentifier()).add("PubliclyAccessible", publiclyAccessible())
.add("ElasticIp", elasticIp()).add("EnhancedVpcRouting", enhancedVpcRouting())
.add("MaintenanceTrackName", maintenanceTrackName()).add("Encrypted", encrypted()).add("KmsKeyId", kmsKeyId())
.add("AvailabilityZoneRelocation", availabilityZoneRelocation()).add("AvailabilityZone", availabilityZone())
.add("Port", port()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "ClusterType":
return Optional.ofNullable(clazz.cast(clusterType()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "NumberOfNodes":
return Optional.ofNullable(clazz.cast(numberOfNodes()));
case "ClusterSecurityGroups":
return Optional.ofNullable(clazz.cast(clusterSecurityGroups()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "ClusterParameterGroupName":
return Optional.ofNullable(clazz.cast(clusterParameterGroupName()));
case "AutomatedSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(automatedSnapshotRetentionPeriod()));
case "ManualSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(manualSnapshotRetentionPeriod()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "ClusterVersion":
return Optional.ofNullable(clazz.cast(clusterVersion()));
case "AllowVersionUpgrade":
return Optional.ofNullable(clazz.cast(allowVersionUpgrade()));
case "HsmClientCertificateIdentifier":
return Optional.ofNullable(clazz.cast(hsmClientCertificateIdentifier()));
case "HsmConfigurationIdentifier":
return Optional.ofNullable(clazz.cast(hsmConfigurationIdentifier()));
case "NewClusterIdentifier":
return Optional.ofNullable(clazz.cast(newClusterIdentifier()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "ElasticIp":
return Optional.ofNullable(clazz.cast(elasticIp()));
case "EnhancedVpcRouting":
return Optional.ofNullable(clazz.cast(enhancedVpcRouting()));
case "MaintenanceTrackName":
return Optional.ofNullable(clazz.cast(maintenanceTrackName()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "AvailabilityZoneRelocation":
return Optional.ofNullable(clazz.cast(availabilityZoneRelocation()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function