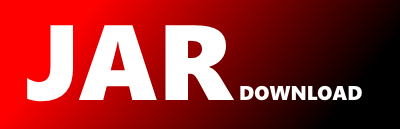
software.amazon.awssdk.services.redshift.model.CopyClusterSnapshotRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CopyClusterSnapshotRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField SOURCE_SNAPSHOT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceSnapshotIdentifier").getter(getter(CopyClusterSnapshotRequest::sourceSnapshotIdentifier))
.setter(setter(Builder::sourceSnapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceSnapshotIdentifier").build())
.build();
private static final SdkField SOURCE_SNAPSHOT_CLUSTER_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SourceSnapshotClusterIdentifier")
.getter(getter(CopyClusterSnapshotRequest::sourceSnapshotClusterIdentifier))
.setter(setter(Builder::sourceSnapshotClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceSnapshotClusterIdentifier")
.build()).build();
private static final SdkField TARGET_SNAPSHOT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetSnapshotIdentifier").getter(getter(CopyClusterSnapshotRequest::targetSnapshotIdentifier))
.setter(setter(Builder::targetSnapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetSnapshotIdentifier").build())
.build();
private static final SdkField MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ManualSnapshotRetentionPeriod")
.getter(getter(CopyClusterSnapshotRequest::manualSnapshotRetentionPeriod))
.setter(setter(Builder::manualSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManualSnapshotRetentionPeriod")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
SOURCE_SNAPSHOT_IDENTIFIER_FIELD, SOURCE_SNAPSHOT_CLUSTER_IDENTIFIER_FIELD, TARGET_SNAPSHOT_IDENTIFIER_FIELD,
MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD));
private final String sourceSnapshotIdentifier;
private final String sourceSnapshotClusterIdentifier;
private final String targetSnapshotIdentifier;
private final Integer manualSnapshotRetentionPeriod;
private CopyClusterSnapshotRequest(BuilderImpl builder) {
super(builder);
this.sourceSnapshotIdentifier = builder.sourceSnapshotIdentifier;
this.sourceSnapshotClusterIdentifier = builder.sourceSnapshotClusterIdentifier;
this.targetSnapshotIdentifier = builder.targetSnapshotIdentifier;
this.manualSnapshotRetentionPeriod = builder.manualSnapshotRetentionPeriod;
}
/**
*
* The identifier for the source snapshot.
*
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid automated snapshot whose state is available
.
*
*
*
*
* @return The identifier for the source snapshot.
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid automated snapshot whose state is available
.
*
*
*/
public final String sourceSnapshotIdentifier() {
return sourceSnapshotIdentifier;
}
/**
*
* The identifier of the cluster the source snapshot was created from. This parameter is required if your IAM user
* or role has a policy containing a snapshot resource element that specifies anything other than * for the cluster
* name.
*
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid cluster.
*
*
*
*
* @return The identifier of the cluster the source snapshot was created from. This parameter is required if your
* IAM user or role has a policy containing a snapshot resource element that specifies anything other than *
* for the cluster name.
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid cluster.
*
*
*/
public final String sourceSnapshotClusterIdentifier() {
return sourceSnapshotClusterIdentifier;
}
/**
*
* The identifier given to the new manual snapshot.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for the Amazon Web Services account that is making the request.
*
*
*
*
* @return The identifier given to the new manual snapshot.
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for the Amazon Web Services account that is making the request.
*
*
*/
public final String targetSnapshotIdentifier() {
return targetSnapshotIdentifier;
}
/**
*
* The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is retained
* indefinitely.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
*
*
* @return The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is
* retained indefinitely.
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
*/
public final Integer manualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(sourceSnapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceSnapshotClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(targetSnapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(manualSnapshotRetentionPeriod());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CopyClusterSnapshotRequest)) {
return false;
}
CopyClusterSnapshotRequest other = (CopyClusterSnapshotRequest) obj;
return Objects.equals(sourceSnapshotIdentifier(), other.sourceSnapshotIdentifier())
&& Objects.equals(sourceSnapshotClusterIdentifier(), other.sourceSnapshotClusterIdentifier())
&& Objects.equals(targetSnapshotIdentifier(), other.targetSnapshotIdentifier())
&& Objects.equals(manualSnapshotRetentionPeriod(), other.manualSnapshotRetentionPeriod());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CopyClusterSnapshotRequest").add("SourceSnapshotIdentifier", sourceSnapshotIdentifier())
.add("SourceSnapshotClusterIdentifier", sourceSnapshotClusterIdentifier())
.add("TargetSnapshotIdentifier", targetSnapshotIdentifier())
.add("ManualSnapshotRetentionPeriod", manualSnapshotRetentionPeriod()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SourceSnapshotIdentifier":
return Optional.ofNullable(clazz.cast(sourceSnapshotIdentifier()));
case "SourceSnapshotClusterIdentifier":
return Optional.ofNullable(clazz.cast(sourceSnapshotClusterIdentifier()));
case "TargetSnapshotIdentifier":
return Optional.ofNullable(clazz.cast(targetSnapshotIdentifier()));
case "ManualSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(manualSnapshotRetentionPeriod()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid automated snapshot whose state is available
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceSnapshotIdentifier(String sourceSnapshotIdentifier);
/**
*
* The identifier of the cluster the source snapshot was created from. This parameter is required if your IAM
* user or role has a policy containing a snapshot resource element that specifies anything other than * for the
* cluster name.
*
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid cluster.
*
*
*
*
* @param sourceSnapshotClusterIdentifier
* The identifier of the cluster the source snapshot was created from. This parameter is required if your
* IAM user or role has a policy containing a snapshot resource element that specifies anything other
* than * for the cluster name.
*
* Constraints:
*
*
* -
*
* Must be the identifier for a valid cluster.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceSnapshotClusterIdentifier(String sourceSnapshotClusterIdentifier);
/**
*
* The identifier given to the new manual snapshot.
*
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for the Amazon Web Services account that is making the request.
*
*
*
*
* @param targetSnapshotIdentifier
* The identifier given to the new manual snapshot.
*
* Constraints:
*
*
* -
*
* Cannot be null, empty, or blank.
*
*
* -
*
* Must contain from 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for the Amazon Web Services account that is making the request.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder targetSnapshotIdentifier(String targetSnapshotIdentifier);
/**
*
* The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is retained
* indefinitely.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
*
*
* @param manualSnapshotRetentionPeriod
* The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is
* retained indefinitely.
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* The default value is -1.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder manualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends RedshiftRequest.BuilderImpl implements Builder {
private String sourceSnapshotIdentifier;
private String sourceSnapshotClusterIdentifier;
private String targetSnapshotIdentifier;
private Integer manualSnapshotRetentionPeriod;
private BuilderImpl() {
}
private BuilderImpl(CopyClusterSnapshotRequest model) {
super(model);
sourceSnapshotIdentifier(model.sourceSnapshotIdentifier);
sourceSnapshotClusterIdentifier(model.sourceSnapshotClusterIdentifier);
targetSnapshotIdentifier(model.targetSnapshotIdentifier);
manualSnapshotRetentionPeriod(model.manualSnapshotRetentionPeriod);
}
public final String getSourceSnapshotIdentifier() {
return sourceSnapshotIdentifier;
}
public final void setSourceSnapshotIdentifier(String sourceSnapshotIdentifier) {
this.sourceSnapshotIdentifier = sourceSnapshotIdentifier;
}
@Override
public final Builder sourceSnapshotIdentifier(String sourceSnapshotIdentifier) {
this.sourceSnapshotIdentifier = sourceSnapshotIdentifier;
return this;
}
public final String getSourceSnapshotClusterIdentifier() {
return sourceSnapshotClusterIdentifier;
}
public final void setSourceSnapshotClusterIdentifier(String sourceSnapshotClusterIdentifier) {
this.sourceSnapshotClusterIdentifier = sourceSnapshotClusterIdentifier;
}
@Override
public final Builder sourceSnapshotClusterIdentifier(String sourceSnapshotClusterIdentifier) {
this.sourceSnapshotClusterIdentifier = sourceSnapshotClusterIdentifier;
return this;
}
public final String getTargetSnapshotIdentifier() {
return targetSnapshotIdentifier;
}
public final void setTargetSnapshotIdentifier(String targetSnapshotIdentifier) {
this.targetSnapshotIdentifier = targetSnapshotIdentifier;
}
@Override
public final Builder targetSnapshotIdentifier(String targetSnapshotIdentifier) {
this.targetSnapshotIdentifier = targetSnapshotIdentifier;
return this;
}
public final Integer getManualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
public final void setManualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod) {
this.manualSnapshotRetentionPeriod = manualSnapshotRetentionPeriod;
}
@Override
public final Builder manualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod) {
this.manualSnapshotRetentionPeriod = manualSnapshotRetentionPeriod;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CopyClusterSnapshotRequest build() {
return new CopyClusterSnapshotRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}