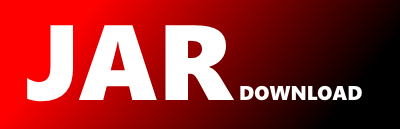
software.amazon.awssdk.services.redshift.model.DescribeResizeResponse Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the result of a cluster resize operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeResizeResponse extends RedshiftResponse implements
ToCopyableBuilder {
private static final SdkField TARGET_NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetNodeType").getter(getter(DescribeResizeResponse::targetNodeType))
.setter(setter(Builder::targetNodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetNodeType").build()).build();
private static final SdkField TARGET_NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TargetNumberOfNodes").getter(getter(DescribeResizeResponse::targetNumberOfNodes))
.setter(setter(Builder::targetNumberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetNumberOfNodes").build())
.build();
private static final SdkField TARGET_CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetClusterType").getter(getter(DescribeResizeResponse::targetClusterType))
.setter(setter(Builder::targetClusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetClusterType").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DescribeResizeResponse::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField> IMPORT_TABLES_COMPLETED_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImportTablesCompleted")
.getter(getter(DescribeResizeResponse::importTablesCompleted))
.setter(setter(Builder::importTablesCompleted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImportTablesCompleted").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> IMPORT_TABLES_IN_PROGRESS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImportTablesInProgress")
.getter(getter(DescribeResizeResponse::importTablesInProgress))
.setter(setter(Builder::importTablesInProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImportTablesInProgress").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> IMPORT_TABLES_NOT_STARTED_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImportTablesNotStarted")
.getter(getter(DescribeResizeResponse::importTablesNotStarted))
.setter(setter(Builder::importTablesNotStarted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImportTablesNotStarted").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AVG_RESIZE_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("AvgResizeRateInMegaBytesPerSecond")
.getter(getter(DescribeResizeResponse::avgResizeRateInMegaBytesPerSecond))
.setter(setter(Builder::avgResizeRateInMegaBytesPerSecond))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvgResizeRateInMegaBytesPerSecond")
.build()).build();
private static final SdkField TOTAL_RESIZE_DATA_IN_MEGA_BYTES_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("TotalResizeDataInMegaBytes")
.getter(getter(DescribeResizeResponse::totalResizeDataInMegaBytes))
.setter(setter(Builder::totalResizeDataInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalResizeDataInMegaBytes").build())
.build();
private static final SdkField PROGRESS_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ProgressInMegaBytes").getter(getter(DescribeResizeResponse::progressInMegaBytes))
.setter(setter(Builder::progressInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgressInMegaBytes").build())
.build();
private static final SdkField ELAPSED_TIME_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ElapsedTimeInSeconds").getter(getter(DescribeResizeResponse::elapsedTimeInSeconds))
.setter(setter(Builder::elapsedTimeInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElapsedTimeInSeconds").build())
.build();
private static final SdkField ESTIMATED_TIME_TO_COMPLETION_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("EstimatedTimeToCompletionInSeconds")
.getter(getter(DescribeResizeResponse::estimatedTimeToCompletionInSeconds))
.setter(setter(Builder::estimatedTimeToCompletionInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedTimeToCompletionInSeconds")
.build()).build();
private static final SdkField RESIZE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResizeType").getter(getter(DescribeResizeResponse::resizeType)).setter(setter(Builder::resizeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResizeType").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Message")
.getter(getter(DescribeResizeResponse::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField TARGET_ENCRYPTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetEncryptionType").getter(getter(DescribeResizeResponse::targetEncryptionType))
.setter(setter(Builder::targetEncryptionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetEncryptionType").build())
.build();
private static final SdkField DATA_TRANSFER_PROGRESS_PERCENT_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("DataTransferProgressPercent")
.getter(getter(DescribeResizeResponse::dataTransferProgressPercent))
.setter(setter(Builder::dataTransferProgressPercent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataTransferProgressPercent")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TARGET_NODE_TYPE_FIELD,
TARGET_NUMBER_OF_NODES_FIELD, TARGET_CLUSTER_TYPE_FIELD, STATUS_FIELD, IMPORT_TABLES_COMPLETED_FIELD,
IMPORT_TABLES_IN_PROGRESS_FIELD, IMPORT_TABLES_NOT_STARTED_FIELD, AVG_RESIZE_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD,
TOTAL_RESIZE_DATA_IN_MEGA_BYTES_FIELD, PROGRESS_IN_MEGA_BYTES_FIELD, ELAPSED_TIME_IN_SECONDS_FIELD,
ESTIMATED_TIME_TO_COMPLETION_IN_SECONDS_FIELD, RESIZE_TYPE_FIELD, MESSAGE_FIELD, TARGET_ENCRYPTION_TYPE_FIELD,
DATA_TRANSFER_PROGRESS_PERCENT_FIELD));
private final String targetNodeType;
private final Integer targetNumberOfNodes;
private final String targetClusterType;
private final String status;
private final List importTablesCompleted;
private final List importTablesInProgress;
private final List importTablesNotStarted;
private final Double avgResizeRateInMegaBytesPerSecond;
private final Long totalResizeDataInMegaBytes;
private final Long progressInMegaBytes;
private final Long elapsedTimeInSeconds;
private final Long estimatedTimeToCompletionInSeconds;
private final String resizeType;
private final String message;
private final String targetEncryptionType;
private final Double dataTransferProgressPercent;
private DescribeResizeResponse(BuilderImpl builder) {
super(builder);
this.targetNodeType = builder.targetNodeType;
this.targetNumberOfNodes = builder.targetNumberOfNodes;
this.targetClusterType = builder.targetClusterType;
this.status = builder.status;
this.importTablesCompleted = builder.importTablesCompleted;
this.importTablesInProgress = builder.importTablesInProgress;
this.importTablesNotStarted = builder.importTablesNotStarted;
this.avgResizeRateInMegaBytesPerSecond = builder.avgResizeRateInMegaBytesPerSecond;
this.totalResizeDataInMegaBytes = builder.totalResizeDataInMegaBytes;
this.progressInMegaBytes = builder.progressInMegaBytes;
this.elapsedTimeInSeconds = builder.elapsedTimeInSeconds;
this.estimatedTimeToCompletionInSeconds = builder.estimatedTimeToCompletionInSeconds;
this.resizeType = builder.resizeType;
this.message = builder.message;
this.targetEncryptionType = builder.targetEncryptionType;
this.dataTransferProgressPercent = builder.dataTransferProgressPercent;
}
/**
*
* The node type that the cluster will have after the resize operation is complete.
*
*
* @return The node type that the cluster will have after the resize operation is complete.
*/
public final String targetNodeType() {
return targetNodeType;
}
/**
*
* The number of nodes that the cluster will have after the resize operation is complete.
*
*
* @return The number of nodes that the cluster will have after the resize operation is complete.
*/
public final Integer targetNumberOfNodes() {
return targetNumberOfNodes;
}
/**
*
* The cluster type after the resize operation is complete.
*
*
* Valid Values: multi-node
| single-node
*
*
* @return The cluster type after the resize operation is complete.
*
* Valid Values: multi-node
| single-node
*/
public final String targetClusterType() {
return targetClusterType;
}
/**
*
* The status of the resize operation.
*
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
|
* CANCELLING
*
*
* @return The status of the resize operation.
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
* | CANCELLING
*/
public final String status() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the ImportTablesCompleted property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasImportTablesCompleted() {
return importTablesCompleted != null && !(importTablesCompleted instanceof SdkAutoConstructList);
}
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasImportTablesCompleted} method.
*
*
* @return The names of tables that have been completely imported .
*
* Valid Values: List of table names.
*/
public final List importTablesCompleted() {
return importTablesCompleted;
}
/**
* For responses, this returns true if the service returned a value for the ImportTablesInProgress property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasImportTablesInProgress() {
return importTablesInProgress != null && !(importTablesInProgress instanceof SdkAutoConstructList);
}
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasImportTablesInProgress} method.
*
*
* @return The names of tables that are being currently imported.
*
* Valid Values: List of table names.
*/
public final List importTablesInProgress() {
return importTablesInProgress;
}
/**
* For responses, this returns true if the service returned a value for the ImportTablesNotStarted property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasImportTablesNotStarted() {
return importTablesNotStarted != null && !(importTablesNotStarted instanceof SdkAutoConstructList);
}
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasImportTablesNotStarted} method.
*
*
* @return The names of tables that have not been yet imported.
*
* Valid Values: List of table names
*/
public final List importTablesNotStarted() {
return importTablesNotStarted;
}
/**
*
* The average rate of the resize operation over the last few minutes, measured in megabytes per second. After the
* resize operation completes, this value shows the average rate of the entire resize operation.
*
*
* @return The average rate of the resize operation over the last few minutes, measured in megabytes per second.
* After the resize operation completes, this value shows the average rate of the entire resize operation.
*/
public final Double avgResizeRateInMegaBytesPerSecond() {
return avgResizeRateInMegaBytesPerSecond;
}
/**
*
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*
*
* @return The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*/
public final Long totalResizeDataInMegaBytes() {
return totalResizeDataInMegaBytes;
}
/**
*
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that has
* been processed so far. When the resize operation is complete, this value shows the total amount of data, in
* megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the estimated total amount
* of data before resize).
*
*
* @return While the resize operation is in progress, this value shows the current amount of data, in megabytes,
* that has been processed so far. When the resize operation is complete, this value shows the total amount
* of data, in megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the
* estimated total amount of data before resize).
*/
public final Long progressInMegaBytes() {
return progressInMegaBytes;
}
/**
*
* The amount of seconds that have elapsed since the resize operation began. After the resize operation completes,
* this value shows the total actual time, in seconds, for the resize operation.
*
*
* @return The amount of seconds that have elapsed since the resize operation began. After the resize operation
* completes, this value shows the total actual time, in seconds, for the resize operation.
*/
public final Long elapsedTimeInSeconds() {
return elapsedTimeInSeconds;
}
/**
*
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated based
* on the average resize rate and the estimated amount of data remaining to be processed. Once the resize operation
* is complete, this value will be 0.
*
*
* @return The estimated time remaining, in seconds, until the resize operation is complete. This value is
* calculated based on the average resize rate and the estimated amount of data remaining to be processed.
* Once the resize operation is complete, this value will be 0.
*/
public final Long estimatedTimeToCompletionInSeconds() {
return estimatedTimeToCompletionInSeconds;
}
/**
*
* An enum with possible values of ClassicResize
and ElasticResize
. These values describe
* the type of resize operation being performed.
*
*
* @return An enum with possible values of ClassicResize
and ElasticResize
. These values
* describe the type of resize operation being performed.
*/
public final String resizeType() {
return resizeType;
}
/**
*
* An optional string to provide additional details about the resize action.
*
*
* @return An optional string to provide additional details about the resize action.
*/
public final String message() {
return message;
}
/**
*
* The type of encryption for the cluster after the resize is complete.
*
*
* Possible values are KMS
and None
.
*
*
* @return The type of encryption for the cluster after the resize is complete.
*
* Possible values are KMS
and None
.
*/
public final String targetEncryptionType() {
return targetEncryptionType;
}
/**
*
* The percent of data transferred from source cluster to target cluster.
*
*
* @return The percent of data transferred from source cluster to target cluster.
*/
public final Double dataTransferProgressPercent() {
return dataTransferProgressPercent;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(targetNodeType());
hashCode = 31 * hashCode + Objects.hashCode(targetNumberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(targetClusterType());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(hasImportTablesCompleted() ? importTablesCompleted() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasImportTablesInProgress() ? importTablesInProgress() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasImportTablesNotStarted() ? importTablesNotStarted() : null);
hashCode = 31 * hashCode + Objects.hashCode(avgResizeRateInMegaBytesPerSecond());
hashCode = 31 * hashCode + Objects.hashCode(totalResizeDataInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(progressInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(elapsedTimeInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(estimatedTimeToCompletionInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(resizeType());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(targetEncryptionType());
hashCode = 31 * hashCode + Objects.hashCode(dataTransferProgressPercent());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeResizeResponse)) {
return false;
}
DescribeResizeResponse other = (DescribeResizeResponse) obj;
return Objects.equals(targetNodeType(), other.targetNodeType())
&& Objects.equals(targetNumberOfNodes(), other.targetNumberOfNodes())
&& Objects.equals(targetClusterType(), other.targetClusterType()) && Objects.equals(status(), other.status())
&& hasImportTablesCompleted() == other.hasImportTablesCompleted()
&& Objects.equals(importTablesCompleted(), other.importTablesCompleted())
&& hasImportTablesInProgress() == other.hasImportTablesInProgress()
&& Objects.equals(importTablesInProgress(), other.importTablesInProgress())
&& hasImportTablesNotStarted() == other.hasImportTablesNotStarted()
&& Objects.equals(importTablesNotStarted(), other.importTablesNotStarted())
&& Objects.equals(avgResizeRateInMegaBytesPerSecond(), other.avgResizeRateInMegaBytesPerSecond())
&& Objects.equals(totalResizeDataInMegaBytes(), other.totalResizeDataInMegaBytes())
&& Objects.equals(progressInMegaBytes(), other.progressInMegaBytes())
&& Objects.equals(elapsedTimeInSeconds(), other.elapsedTimeInSeconds())
&& Objects.equals(estimatedTimeToCompletionInSeconds(), other.estimatedTimeToCompletionInSeconds())
&& Objects.equals(resizeType(), other.resizeType()) && Objects.equals(message(), other.message())
&& Objects.equals(targetEncryptionType(), other.targetEncryptionType())
&& Objects.equals(dataTransferProgressPercent(), other.dataTransferProgressPercent());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeResizeResponse").add("TargetNodeType", targetNodeType())
.add("TargetNumberOfNodes", targetNumberOfNodes()).add("TargetClusterType", targetClusterType())
.add("Status", status())
.add("ImportTablesCompleted", hasImportTablesCompleted() ? importTablesCompleted() : null)
.add("ImportTablesInProgress", hasImportTablesInProgress() ? importTablesInProgress() : null)
.add("ImportTablesNotStarted", hasImportTablesNotStarted() ? importTablesNotStarted() : null)
.add("AvgResizeRateInMegaBytesPerSecond", avgResizeRateInMegaBytesPerSecond())
.add("TotalResizeDataInMegaBytes", totalResizeDataInMegaBytes())
.add("ProgressInMegaBytes", progressInMegaBytes()).add("ElapsedTimeInSeconds", elapsedTimeInSeconds())
.add("EstimatedTimeToCompletionInSeconds", estimatedTimeToCompletionInSeconds()).add("ResizeType", resizeType())
.add("Message", message()).add("TargetEncryptionType", targetEncryptionType())
.add("DataTransferProgressPercent", dataTransferProgressPercent()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TargetNodeType":
return Optional.ofNullable(clazz.cast(targetNodeType()));
case "TargetNumberOfNodes":
return Optional.ofNullable(clazz.cast(targetNumberOfNodes()));
case "TargetClusterType":
return Optional.ofNullable(clazz.cast(targetClusterType()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "ImportTablesCompleted":
return Optional.ofNullable(clazz.cast(importTablesCompleted()));
case "ImportTablesInProgress":
return Optional.ofNullable(clazz.cast(importTablesInProgress()));
case "ImportTablesNotStarted":
return Optional.ofNullable(clazz.cast(importTablesNotStarted()));
case "AvgResizeRateInMegaBytesPerSecond":
return Optional.ofNullable(clazz.cast(avgResizeRateInMegaBytesPerSecond()));
case "TotalResizeDataInMegaBytes":
return Optional.ofNullable(clazz.cast(totalResizeDataInMegaBytes()));
case "ProgressInMegaBytes":
return Optional.ofNullable(clazz.cast(progressInMegaBytes()));
case "ElapsedTimeInSeconds":
return Optional.ofNullable(clazz.cast(elapsedTimeInSeconds()));
case "EstimatedTimeToCompletionInSeconds":
return Optional.ofNullable(clazz.cast(estimatedTimeToCompletionInSeconds()));
case "ResizeType":
return Optional.ofNullable(clazz.cast(resizeType()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "TargetEncryptionType":
return Optional.ofNullable(clazz.cast(targetEncryptionType()));
case "DataTransferProgressPercent":
return Optional.ofNullable(clazz.cast(dataTransferProgressPercent()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function