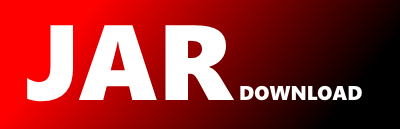
software.amazon.awssdk.services.redshift.model.ModifyEndpointAccessResponse Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyEndpointAccessResponse extends RedshiftResponse implements
ToCopyableBuilder {
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(ModifyEndpointAccessResponse::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField RESOURCE_OWNER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceOwner").getter(getter(ModifyEndpointAccessResponse::resourceOwner))
.setter(setter(Builder::resourceOwner))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceOwner").build()).build();
private static final SdkField SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetGroupName").getter(getter(ModifyEndpointAccessResponse::subnetGroupName))
.setter(setter(Builder::subnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetGroupName").build()).build();
private static final SdkField ENDPOINT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointStatus").getter(getter(ModifyEndpointAccessResponse::endpointStatus))
.setter(setter(Builder::endpointStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointStatus").build()).build();
private static final SdkField ENDPOINT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointName").getter(getter(ModifyEndpointAccessResponse::endpointName))
.setter(setter(Builder::endpointName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointName").build()).build();
private static final SdkField ENDPOINT_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndpointCreateTime").getter(getter(ModifyEndpointAccessResponse::endpointCreateTime))
.setter(setter(Builder::endpointCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointCreateTime").build())
.build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(ModifyEndpointAccessResponse::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Address")
.getter(getter(ModifyEndpointAccessResponse::address)).setter(setter(Builder::address))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Address").build()).build();
private static final SdkField> VPC_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroups")
.getter(getter(ModifyEndpointAccessResponse::vpcSecurityGroups))
.setter(setter(Builder::vpcSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(VpcSecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroup").build()).build()).build()).build();
private static final SdkField VPC_ENDPOINT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("VpcEndpoint").getter(getter(ModifyEndpointAccessResponse::vpcEndpoint))
.setter(setter(Builder::vpcEndpoint)).constructor(VpcEndpoint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcEndpoint").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_IDENTIFIER_FIELD,
RESOURCE_OWNER_FIELD, SUBNET_GROUP_NAME_FIELD, ENDPOINT_STATUS_FIELD, ENDPOINT_NAME_FIELD,
ENDPOINT_CREATE_TIME_FIELD, PORT_FIELD, ADDRESS_FIELD, VPC_SECURITY_GROUPS_FIELD, VPC_ENDPOINT_FIELD));
private final String clusterIdentifier;
private final String resourceOwner;
private final String subnetGroupName;
private final String endpointStatus;
private final String endpointName;
private final Instant endpointCreateTime;
private final Integer port;
private final String address;
private final List vpcSecurityGroups;
private final VpcEndpoint vpcEndpoint;
private ModifyEndpointAccessResponse(BuilderImpl builder) {
super(builder);
this.clusterIdentifier = builder.clusterIdentifier;
this.resourceOwner = builder.resourceOwner;
this.subnetGroupName = builder.subnetGroupName;
this.endpointStatus = builder.endpointStatus;
this.endpointName = builder.endpointName;
this.endpointCreateTime = builder.endpointCreateTime;
this.port = builder.port;
this.address = builder.address;
this.vpcSecurityGroups = builder.vpcSecurityGroups;
this.vpcEndpoint = builder.vpcEndpoint;
}
/**
*
* The cluster identifier of the cluster associated with the endpoint.
*
*
* @return The cluster identifier of the cluster associated with the endpoint.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The Amazon Web Services account ID of the owner of the cluster.
*
*
* @return The Amazon Web Services account ID of the owner of the cluster.
*/
public final String resourceOwner() {
return resourceOwner;
}
/**
*
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*
*
* @return The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*/
public final String subnetGroupName() {
return subnetGroupName;
}
/**
*
* The status of the endpoint.
*
*
* @return The status of the endpoint.
*/
public final String endpointStatus() {
return endpointStatus;
}
/**
*
* The name of the endpoint.
*
*
* @return The name of the endpoint.
*/
public final String endpointName() {
return endpointName;
}
/**
*
* The time (UTC) that the endpoint was created.
*
*
* @return The time (UTC) that the endpoint was created.
*/
public final Instant endpointCreateTime() {
return endpointCreateTime;
}
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* @return The port number on which the cluster accepts incoming connections.
*/
public final Integer port() {
return port;
}
/**
*
* The DNS address of the endpoint.
*
*
* @return The DNS address of the endpoint.
*/
public final String address() {
return address;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroups property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroups() {
return vpcSecurityGroups != null && !(vpcSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* The security groups associated with the endpoint.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroups} method.
*
*
* @return The security groups associated with the endpoint.
*/
public final List vpcSecurityGroups() {
return vpcSecurityGroups;
}
/**
* Returns the value of the VpcEndpoint property for this object.
*
* @return The value of the VpcEndpoint property for this object.
*/
public final VpcEndpoint vpcEndpoint() {
return vpcEndpoint;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(resourceOwner());
hashCode = 31 * hashCode + Objects.hashCode(subnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(endpointStatus());
hashCode = 31 * hashCode + Objects.hashCode(endpointName());
hashCode = 31 * hashCode + Objects.hashCode(endpointCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(address());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroups() ? vpcSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(vpcEndpoint());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyEndpointAccessResponse)) {
return false;
}
ModifyEndpointAccessResponse other = (ModifyEndpointAccessResponse) obj;
return Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(resourceOwner(), other.resourceOwner())
&& Objects.equals(subnetGroupName(), other.subnetGroupName())
&& Objects.equals(endpointStatus(), other.endpointStatus())
&& Objects.equals(endpointName(), other.endpointName())
&& Objects.equals(endpointCreateTime(), other.endpointCreateTime()) && Objects.equals(port(), other.port())
&& Objects.equals(address(), other.address()) && hasVpcSecurityGroups() == other.hasVpcSecurityGroups()
&& Objects.equals(vpcSecurityGroups(), other.vpcSecurityGroups())
&& Objects.equals(vpcEndpoint(), other.vpcEndpoint());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyEndpointAccessResponse").add("ClusterIdentifier", clusterIdentifier())
.add("ResourceOwner", resourceOwner()).add("SubnetGroupName", subnetGroupName())
.add("EndpointStatus", endpointStatus()).add("EndpointName", endpointName())
.add("EndpointCreateTime", endpointCreateTime()).add("Port", port()).add("Address", address())
.add("VpcSecurityGroups", hasVpcSecurityGroups() ? vpcSecurityGroups() : null).add("VpcEndpoint", vpcEndpoint())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "ResourceOwner":
return Optional.ofNullable(clazz.cast(resourceOwner()));
case "SubnetGroupName":
return Optional.ofNullable(clazz.cast(subnetGroupName()));
case "EndpointStatus":
return Optional.ofNullable(clazz.cast(endpointStatus()));
case "EndpointName":
return Optional.ofNullable(clazz.cast(endpointName()));
case "EndpointCreateTime":
return Optional.ofNullable(clazz.cast(endpointCreateTime()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "Address":
return Optional.ofNullable(clazz.cast(address()));
case "VpcSecurityGroups":
return Optional.ofNullable(clazz.cast(vpcSecurityGroups()));
case "VpcEndpoint":
return Optional.ofNullable(clazz.cast(vpcEndpoint()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function