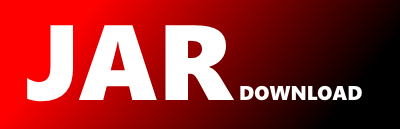
software.amazon.awssdk.services.redshift.model.PendingModifiedValues Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes cluster attributes that are in a pending state. A change to one or more the attributes was requested and is
* in progress or will be applied.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PendingModifiedValues implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(PendingModifiedValues::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(PendingModifiedValues::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfNodes").getter(getter(PendingModifiedValues::numberOfNodes))
.setter(setter(Builder::numberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNodes").build()).build();
private static final SdkField CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterType").getter(getter(PendingModifiedValues::clusterType)).setter(setter(Builder::clusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterType").build()).build();
private static final SdkField CLUSTER_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterVersion").getter(getter(PendingModifiedValues::clusterVersion))
.setter(setter(Builder::clusterVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterVersion").build()).build();
private static final SdkField AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AutomatedSnapshotRetentionPeriod")
.getter(getter(PendingModifiedValues::automatedSnapshotRetentionPeriod))
.setter(setter(Builder::automatedSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomatedSnapshotRetentionPeriod")
.build()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(PendingModifiedValues::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("PubliclyAccessible").getter(getter(PendingModifiedValues::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PubliclyAccessible").build())
.build();
private static final SdkField ENHANCED_VPC_ROUTING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnhancedVpcRouting").getter(getter(PendingModifiedValues::enhancedVpcRouting))
.setter(setter(Builder::enhancedVpcRouting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnhancedVpcRouting").build())
.build();
private static final SdkField MAINTENANCE_TRACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaintenanceTrackName").getter(getter(PendingModifiedValues::maintenanceTrackName))
.setter(setter(Builder::maintenanceTrackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaintenanceTrackName").build())
.build();
private static final SdkField ENCRYPTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EncryptionType").getter(getter(PendingModifiedValues::encryptionType))
.setter(setter(Builder::encryptionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncryptionType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MASTER_USER_PASSWORD_FIELD,
NODE_TYPE_FIELD, NUMBER_OF_NODES_FIELD, CLUSTER_TYPE_FIELD, CLUSTER_VERSION_FIELD,
AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD, CLUSTER_IDENTIFIER_FIELD, PUBLICLY_ACCESSIBLE_FIELD,
ENHANCED_VPC_ROUTING_FIELD, MAINTENANCE_TRACK_NAME_FIELD, ENCRYPTION_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String masterUserPassword;
private final String nodeType;
private final Integer numberOfNodes;
private final String clusterType;
private final String clusterVersion;
private final Integer automatedSnapshotRetentionPeriod;
private final String clusterIdentifier;
private final Boolean publiclyAccessible;
private final Boolean enhancedVpcRouting;
private final String maintenanceTrackName;
private final String encryptionType;
private PendingModifiedValues(BuilderImpl builder) {
this.masterUserPassword = builder.masterUserPassword;
this.nodeType = builder.nodeType;
this.numberOfNodes = builder.numberOfNodes;
this.clusterType = builder.clusterType;
this.clusterVersion = builder.clusterVersion;
this.automatedSnapshotRetentionPeriod = builder.automatedSnapshotRetentionPeriod;
this.clusterIdentifier = builder.clusterIdentifier;
this.publiclyAccessible = builder.publiclyAccessible;
this.enhancedVpcRouting = builder.enhancedVpcRouting;
this.maintenanceTrackName = builder.maintenanceTrackName;
this.encryptionType = builder.encryptionType;
}
/**
*
* The pending or in-progress change of the admin user password for the cluster.
*
*
* @return The pending or in-progress change of the admin user password for the cluster.
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
*
* The pending or in-progress change of the cluster's node type.
*
*
* @return The pending or in-progress change of the cluster's node type.
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The pending or in-progress change of the number of nodes in the cluster.
*
*
* @return The pending or in-progress change of the number of nodes in the cluster.
*/
public final Integer numberOfNodes() {
return numberOfNodes;
}
/**
*
* The pending or in-progress change of the cluster type.
*
*
* @return The pending or in-progress change of the cluster type.
*/
public final String clusterType() {
return clusterType;
}
/**
*
* The pending or in-progress change of the service version.
*
*
* @return The pending or in-progress change of the service version.
*/
public final String clusterVersion() {
return clusterVersion;
}
/**
*
* The pending or in-progress change of the automated snapshot retention period.
*
*
* @return The pending or in-progress change of the automated snapshot retention period.
*/
public final Integer automatedSnapshotRetentionPeriod() {
return automatedSnapshotRetentionPeriod;
}
/**
*
* The pending or in-progress change of the new identifier for the cluster.
*
*
* @return The pending or in-progress change of the new identifier for the cluster.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The pending or in-progress change of the ability to connect to the cluster from the public network.
*
*
* @return The pending or in-progress change of the ability to connect to the cluster from the public network.
*/
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
*
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster
* that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the
* Amazon Redshift Cluster Management Guide.
*
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*
*
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a
* cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC
* Routing in the Amazon Redshift Cluster Management Guide.
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*/
public final Boolean enhancedVpcRouting() {
return enhancedVpcRouting;
}
/**
*
* The name of the maintenance track that the cluster will change to during the next maintenance window.
*
*
* @return The name of the maintenance track that the cluster will change to during the next maintenance window.
*/
public final String maintenanceTrackName() {
return maintenanceTrackName;
}
/**
*
* The encryption type for a cluster. Possible values are: KMS and None.
*
*
* @return The encryption type for a cluster. Possible values are: KMS and None.
*/
public final String encryptionType() {
return encryptionType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(clusterType());
hashCode = 31 * hashCode + Objects.hashCode(clusterVersion());
hashCode = 31 * hashCode + Objects.hashCode(automatedSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(enhancedVpcRouting());
hashCode = 31 * hashCode + Objects.hashCode(maintenanceTrackName());
hashCode = 31 * hashCode + Objects.hashCode(encryptionType());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PendingModifiedValues)) {
return false;
}
PendingModifiedValues other = (PendingModifiedValues) obj;
return Objects.equals(masterUserPassword(), other.masterUserPassword()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(numberOfNodes(), other.numberOfNodes()) && Objects.equals(clusterType(), other.clusterType())
&& Objects.equals(clusterVersion(), other.clusterVersion())
&& Objects.equals(automatedSnapshotRetentionPeriod(), other.automatedSnapshotRetentionPeriod())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible())
&& Objects.equals(enhancedVpcRouting(), other.enhancedVpcRouting())
&& Objects.equals(maintenanceTrackName(), other.maintenanceTrackName())
&& Objects.equals(encryptionType(), other.encryptionType());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PendingModifiedValues").add("MasterUserPassword", masterUserPassword())
.add("NodeType", nodeType()).add("NumberOfNodes", numberOfNodes()).add("ClusterType", clusterType())
.add("ClusterVersion", clusterVersion())
.add("AutomatedSnapshotRetentionPeriod", automatedSnapshotRetentionPeriod())
.add("ClusterIdentifier", clusterIdentifier()).add("PubliclyAccessible", publiclyAccessible())
.add("EnhancedVpcRouting", enhancedVpcRouting()).add("MaintenanceTrackName", maintenanceTrackName())
.add("EncryptionType", encryptionType()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "NumberOfNodes":
return Optional.ofNullable(clazz.cast(numberOfNodes()));
case "ClusterType":
return Optional.ofNullable(clazz.cast(clusterType()));
case "ClusterVersion":
return Optional.ofNullable(clazz.cast(clusterVersion()));
case "AutomatedSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(automatedSnapshotRetentionPeriod()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "EnhancedVpcRouting":
return Optional.ofNullable(clazz.cast(enhancedVpcRouting()));
case "MaintenanceTrackName":
return Optional.ofNullable(clazz.cast(maintenanceTrackName()));
case "EncryptionType":
return Optional.ofNullable(clazz.cast(encryptionType()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function