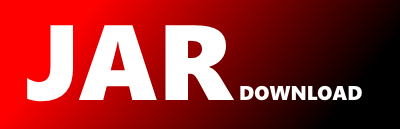
software.amazon.awssdk.services.redshift.model.ResizeClusterMessage Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a resize cluster operation. For example, a scheduled action to run the ResizeCluster
API
* operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResizeClusterMessage implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(ResizeClusterMessage::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterType").getter(getter(ResizeClusterMessage::clusterType)).setter(setter(Builder::clusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterType").build()).build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(ResizeClusterMessage::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfNodes").getter(getter(ResizeClusterMessage::numberOfNodes))
.setter(setter(Builder::numberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNodes").build()).build();
private static final SdkField CLASSIC_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Classic").getter(getter(ResizeClusterMessage::classic)).setter(setter(Builder::classic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Classic").build()).build();
private static final SdkField RESERVED_NODE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReservedNodeId").getter(getter(ResizeClusterMessage::reservedNodeId))
.setter(setter(Builder::reservedNodeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReservedNodeId").build()).build();
private static final SdkField TARGET_RESERVED_NODE_OFFERING_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TargetReservedNodeOfferingId")
.getter(getter(ResizeClusterMessage::targetReservedNodeOfferingId))
.setter(setter(Builder::targetReservedNodeOfferingId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetReservedNodeOfferingId")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_IDENTIFIER_FIELD,
CLUSTER_TYPE_FIELD, NODE_TYPE_FIELD, NUMBER_OF_NODES_FIELD, CLASSIC_FIELD, RESERVED_NODE_ID_FIELD,
TARGET_RESERVED_NODE_OFFERING_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String clusterIdentifier;
private final String clusterType;
private final String nodeType;
private final Integer numberOfNodes;
private final Boolean classic;
private final String reservedNodeId;
private final String targetReservedNodeOfferingId;
private ResizeClusterMessage(BuilderImpl builder) {
this.clusterIdentifier = builder.clusterIdentifier;
this.clusterType = builder.clusterType;
this.nodeType = builder.nodeType;
this.numberOfNodes = builder.numberOfNodes;
this.classic = builder.classic;
this.reservedNodeId = builder.reservedNodeId;
this.targetReservedNodeOfferingId = builder.targetReservedNodeOfferingId;
}
/**
*
* The unique identifier for the cluster to resize.
*
*
* @return The unique identifier for the cluster to resize.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The new cluster type for the specified cluster.
*
*
* @return The new cluster type for the specified cluster.
*/
public final String clusterType() {
return clusterType;
}
/**
*
* The new node type for the nodes you are adding. If not specified, the cluster's current node type is used.
*
*
* @return The new node type for the nodes you are adding. If not specified, the cluster's current node type is
* used.
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The new number of nodes for the cluster. If not specified, the cluster's current number of nodes is used.
*
*
* @return The new number of nodes for the cluster. If not specified, the cluster's current number of nodes is used.
*/
public final Integer numberOfNodes() {
return numberOfNodes;
}
/**
*
* A boolean value indicating whether the resize operation is using the classic resize process. If you don't provide
* this parameter or set the value to false
, the resize type is elastic.
*
*
* @return A boolean value indicating whether the resize operation is using the classic resize process. If you don't
* provide this parameter or set the value to false
, the resize type is elastic.
*/
public final Boolean classic() {
return classic;
}
/**
*
* The identifier of the reserved node.
*
*
* @return The identifier of the reserved node.
*/
public final String reservedNodeId() {
return reservedNodeId;
}
/**
*
* The identifier of the target reserved node offering.
*
*
* @return The identifier of the target reserved node offering.
*/
public final String targetReservedNodeOfferingId() {
return targetReservedNodeOfferingId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(clusterType());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(classic());
hashCode = 31 * hashCode + Objects.hashCode(reservedNodeId());
hashCode = 31 * hashCode + Objects.hashCode(targetReservedNodeOfferingId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResizeClusterMessage)) {
return false;
}
ResizeClusterMessage other = (ResizeClusterMessage) obj;
return Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(clusterType(), other.clusterType()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(numberOfNodes(), other.numberOfNodes()) && Objects.equals(classic(), other.classic())
&& Objects.equals(reservedNodeId(), other.reservedNodeId())
&& Objects.equals(targetReservedNodeOfferingId(), other.targetReservedNodeOfferingId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ResizeClusterMessage").add("ClusterIdentifier", clusterIdentifier())
.add("ClusterType", clusterType()).add("NodeType", nodeType()).add("NumberOfNodes", numberOfNodes())
.add("Classic", classic()).add("ReservedNodeId", reservedNodeId())
.add("TargetReservedNodeOfferingId", targetReservedNodeOfferingId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "ClusterType":
return Optional.ofNullable(clazz.cast(clusterType()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "NumberOfNodes":
return Optional.ofNullable(clazz.cast(numberOfNodes()));
case "Classic":
return Optional.ofNullable(clazz.cast(classic()));
case "ReservedNodeId":
return Optional.ofNullable(clazz.cast(reservedNodeId()));
case "TargetReservedNodeOfferingId":
return Optional.ofNullable(clazz.cast(targetReservedNodeOfferingId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function