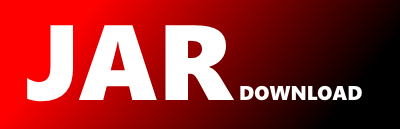
software.amazon.awssdk.services.redshift.model.DescribeClusterSnapshotsRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeClusterSnapshotsRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(DescribeClusterSnapshotsRequest::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField SNAPSHOT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotIdentifier").getter(getter(DescribeClusterSnapshotsRequest::snapshotIdentifier))
.setter(setter(Builder::snapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotIdentifier").build())
.build();
private static final SdkField SNAPSHOT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotArn").getter(getter(DescribeClusterSnapshotsRequest::snapshotArn))
.setter(setter(Builder::snapshotArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotArn").build()).build();
private static final SdkField SNAPSHOT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotType").getter(getter(DescribeClusterSnapshotsRequest::snapshotType))
.setter(setter(Builder::snapshotType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotType").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(DescribeClusterSnapshotsRequest::startTime))
.setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(DescribeClusterSnapshotsRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField MAX_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxRecords").getter(getter(DescribeClusterSnapshotsRequest::maxRecords))
.setter(setter(Builder::maxRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxRecords").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Marker")
.getter(getter(DescribeClusterSnapshotsRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final SdkField OWNER_ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OwnerAccount").getter(getter(DescribeClusterSnapshotsRequest::ownerAccount))
.setter(setter(Builder::ownerAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerAccount").build()).build();
private static final SdkField> TAG_KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagKeys")
.getter(getter(DescribeClusterSnapshotsRequest::tagKeys))
.setter(setter(Builder::tagKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagKeys").build(),
ListTrait
.builder()
.memberLocationName("TagKey")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("TagKey").build()).build()).build()).build();
private static final SdkField> TAG_VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagValues")
.getter(getter(DescribeClusterSnapshotsRequest::tagValues))
.setter(setter(Builder::tagValues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagValues").build(),
ListTrait
.builder()
.memberLocationName("TagValue")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("TagValue").build()).build()).build()).build();
private static final SdkField CLUSTER_EXISTS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ClusterExists").getter(getter(DescribeClusterSnapshotsRequest::clusterExists))
.setter(setter(Builder::clusterExists))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterExists").build()).build();
private static final SdkField> SORTING_ENTITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SortingEntities")
.getter(getter(DescribeClusterSnapshotsRequest::sortingEntities))
.setter(setter(Builder::sortingEntities))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SortingEntities").build(),
ListTrait
.builder()
.memberLocationName("SnapshotSortingEntity")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SnapshotSortingEntity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SnapshotSortingEntity").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_IDENTIFIER_FIELD,
SNAPSHOT_IDENTIFIER_FIELD, SNAPSHOT_ARN_FIELD, SNAPSHOT_TYPE_FIELD, START_TIME_FIELD, END_TIME_FIELD,
MAX_RECORDS_FIELD, MARKER_FIELD, OWNER_ACCOUNT_FIELD, TAG_KEYS_FIELD, TAG_VALUES_FIELD, CLUSTER_EXISTS_FIELD,
SORTING_ENTITIES_FIELD));
private final String clusterIdentifier;
private final String snapshotIdentifier;
private final String snapshotArn;
private final String snapshotType;
private final Instant startTime;
private final Instant endTime;
private final Integer maxRecords;
private final String marker;
private final String ownerAccount;
private final List tagKeys;
private final List tagValues;
private final Boolean clusterExists;
private final List sortingEntities;
private DescribeClusterSnapshotsRequest(BuilderImpl builder) {
super(builder);
this.clusterIdentifier = builder.clusterIdentifier;
this.snapshotIdentifier = builder.snapshotIdentifier;
this.snapshotArn = builder.snapshotArn;
this.snapshotType = builder.snapshotType;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.maxRecords = builder.maxRecords;
this.marker = builder.marker;
this.ownerAccount = builder.ownerAccount;
this.tagKeys = builder.tagKeys;
this.tagValues = builder.tagValues;
this.clusterExists = builder.clusterExists;
this.sortingEntities = builder.sortingEntities;
}
/**
*
* The identifier of the cluster which generated the requested snapshots.
*
*
* @return The identifier of the cluster which generated the requested snapshots.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The snapshot identifier of the snapshot about which to return information.
*
*
* @return The snapshot identifier of the snapshot about which to return information.
*/
public final String snapshotIdentifier() {
return snapshotIdentifier;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot associated with the message to describe cluster snapshots.
*
*
* @return The Amazon Resource Name (ARN) of the snapshot associated with the message to describe cluster snapshots.
*/
public final String snapshotArn() {
return snapshotArn;
}
/**
*
* The type of snapshots for which you are requesting information. By default, snapshots of all types are returned.
*
*
* Valid Values: automated
| manual
*
*
* @return The type of snapshots for which you are requesting information. By default, snapshots of all types are
* returned.
*
* Valid Values: automated
| manual
*/
public final String snapshotType() {
return snapshotType;
}
/**
*
* A value that requests only snapshots created at or after the specified time. The time value is specified in ISO
* 8601 format. For more information about ISO 8601, go to the ISO8601 Wikipedia page.
*
*
* Example: 2012-07-16T18:00:00Z
*
*
* @return A value that requests only snapshots created at or after the specified time. The time value is specified
* in ISO 8601 format. For more information about ISO 8601, go to the ISO8601 Wikipedia page.
*
* Example: 2012-07-16T18:00:00Z
*/
public final Instant startTime() {
return startTime;
}
/**
*
* A time value that requests only snapshots created at or before the specified time. The time value is specified in
* ISO 8601 format. For more information about ISO 8601, go to the ISO8601 Wikipedia page.
*
*
* Example: 2012-07-16T18:00:00Z
*
*
* @return A time value that requests only snapshots created at or before the specified time. The time value is
* specified in ISO 8601 format. For more information about ISO 8601, go to the ISO8601 Wikipedia page.
*
* Example: 2012-07-16T18:00:00Z
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of the
* response. You can retrieve the next set of records by retrying the command with the returned marker value.
*
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*
*
* @return The maximum number of response records to return in each call. If the number of remaining response
* records exceeds the specified MaxRecords
value, a value is returned in a marker
* field of the response. You can retrieve the next set of records by retrying the command with the returned
* marker value.
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*/
public final Integer maxRecords() {
return maxRecords;
}
/**
*
* An optional parameter that specifies the starting point to return a set of response records. When the results of
* a DescribeClusterSnapshots request exceed the value specified in MaxRecords
, Amazon Web
* Services returns a value in the Marker
field of the response. You can retrieve the next set of
* response records by providing the returned marker value in the Marker
parameter and retrying the
* request.
*
*
* @return An optional parameter that specifies the starting point to return a set of response records. When the
* results of a DescribeClusterSnapshots request exceed the value specified in
* MaxRecords
, Amazon Web Services returns a value in the Marker
field of the
* response. You can retrieve the next set of response records by providing the returned marker value in the
* Marker
parameter and retrying the request.
*/
public final String marker() {
return marker;
}
/**
*
* The Amazon Web Services account used to create or copy the snapshot. Use this field to filter the results to
* snapshots owned by a particular account. To describe snapshots you own, either specify your Amazon Web Services
* account, or do not specify the parameter.
*
*
* @return The Amazon Web Services account used to create or copy the snapshot. Use this field to filter the results
* to snapshots owned by a particular account. To describe snapshots you own, either specify your Amazon Web
* Services account, or do not specify the parameter.
*/
public final String ownerAccount() {
return ownerAccount;
}
/**
* For responses, this returns true if the service returned a value for the TagKeys property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTagKeys() {
return tagKeys != null && !(tagKeys instanceof SdkAutoConstructList);
}
/**
*
* A tag key or keys for which you want to return all matching cluster snapshots that are associated with the
* specified key or keys. For example, suppose that you have snapshots that are tagged with keys called
* owner
and environment
. If you specify both of these tag keys in the request, Amazon
* Redshift returns a response with the snapshots that have either or both of these tag keys associated with them.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagKeys} method.
*
*
* @return A tag key or keys for which you want to return all matching cluster snapshots that are associated with
* the specified key or keys. For example, suppose that you have snapshots that are tagged with keys called
* owner
and environment
. If you specify both of these tag keys in the request,
* Amazon Redshift returns a response with the snapshots that have either or both of these tag keys
* associated with them.
*/
public final List tagKeys() {
return tagKeys;
}
/**
* For responses, this returns true if the service returned a value for the TagValues property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTagValues() {
return tagValues != null && !(tagValues instanceof SdkAutoConstructList);
}
/**
*
* A tag value or values for which you want to return all matching cluster snapshots that are associated with the
* specified tag value or values. For example, suppose that you have snapshots that are tagged with values called
* admin
and test
. If you specify both of these tag values in the request, Amazon Redshift
* returns a response with the snapshots that have either or both of these tag values associated with them.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagValues} method.
*
*
* @return A tag value or values for which you want to return all matching cluster snapshots that are associated
* with the specified tag value or values. For example, suppose that you have snapshots that are tagged with
* values called admin
and test
. If you specify both of these tag values in the
* request, Amazon Redshift returns a response with the snapshots that have either or both of these tag
* values associated with them.
*/
public final List tagValues() {
return tagValues;
}
/**
*
* A value that indicates whether to return snapshots only for an existing cluster. You can perform table-level
* restore only by using a snapshot of an existing cluster, that is, a cluster that has not been deleted. Values for
* this parameter work as follows:
*
*
* -
*
* If ClusterExists
is set to true
, ClusterIdentifier
is required.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
isn't specified,
* all snapshots associated with deleted clusters (orphaned snapshots) are returned.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
is specified for a
* deleted cluster, snapshots associated with that cluster are returned.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
is specified for an
* existing cluster, no snapshots are returned.
*
*
*
*
* @return A value that indicates whether to return snapshots only for an existing cluster. You can perform
* table-level restore only by using a snapshot of an existing cluster, that is, a cluster that has not been
* deleted. Values for this parameter work as follows:
*
* -
*
* If ClusterExists
is set to true
, ClusterIdentifier
is required.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
isn't
* specified, all snapshots associated with deleted clusters (orphaned snapshots) are returned.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
is
* specified for a deleted cluster, snapshots associated with that cluster are returned.
*
*
* -
*
* If ClusterExists
is set to false
and ClusterIdentifier
is
* specified for an existing cluster, no snapshots are returned.
*
*
*/
public final Boolean clusterExists() {
return clusterExists;
}
/**
* For responses, this returns true if the service returned a value for the SortingEntities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSortingEntities() {
return sortingEntities != null && !(sortingEntities instanceof SdkAutoConstructList);
}
/**
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSortingEntities} method.
*
*
* @return
*/
public final List sortingEntities() {
return sortingEntities;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(snapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(snapshotArn());
hashCode = 31 * hashCode + Objects.hashCode(snapshotType());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(maxRecords());
hashCode = 31 * hashCode + Objects.hashCode(marker());
hashCode = 31 * hashCode + Objects.hashCode(ownerAccount());
hashCode = 31 * hashCode + Objects.hashCode(hasTagKeys() ? tagKeys() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTagValues() ? tagValues() : null);
hashCode = 31 * hashCode + Objects.hashCode(clusterExists());
hashCode = 31 * hashCode + Objects.hashCode(hasSortingEntities() ? sortingEntities() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeClusterSnapshotsRequest)) {
return false;
}
DescribeClusterSnapshotsRequest other = (DescribeClusterSnapshotsRequest) obj;
return Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(snapshotIdentifier(), other.snapshotIdentifier())
&& Objects.equals(snapshotArn(), other.snapshotArn()) && Objects.equals(snapshotType(), other.snapshotType())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(maxRecords(), other.maxRecords()) && Objects.equals(marker(), other.marker())
&& Objects.equals(ownerAccount(), other.ownerAccount()) && hasTagKeys() == other.hasTagKeys()
&& Objects.equals(tagKeys(), other.tagKeys()) && hasTagValues() == other.hasTagValues()
&& Objects.equals(tagValues(), other.tagValues()) && Objects.equals(clusterExists(), other.clusterExists())
&& hasSortingEntities() == other.hasSortingEntities()
&& Objects.equals(sortingEntities(), other.sortingEntities());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeClusterSnapshotsRequest").add("ClusterIdentifier", clusterIdentifier())
.add("SnapshotIdentifier", snapshotIdentifier()).add("SnapshotArn", snapshotArn())
.add("SnapshotType", snapshotType()).add("StartTime", startTime()).add("EndTime", endTime())
.add("MaxRecords", maxRecords()).add("Marker", marker()).add("OwnerAccount", ownerAccount())
.add("TagKeys", hasTagKeys() ? tagKeys() : null).add("TagValues", hasTagValues() ? tagValues() : null)
.add("ClusterExists", clusterExists()).add("SortingEntities", hasSortingEntities() ? sortingEntities() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "SnapshotIdentifier":
return Optional.ofNullable(clazz.cast(snapshotIdentifier()));
case "SnapshotArn":
return Optional.ofNullable(clazz.cast(snapshotArn()));
case "SnapshotType":
return Optional.ofNullable(clazz.cast(snapshotType()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "MaxRecords":
return Optional.ofNullable(clazz.cast(maxRecords()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
case "OwnerAccount":
return Optional.ofNullable(clazz.cast(ownerAccount()));
case "TagKeys":
return Optional.ofNullable(clazz.cast(tagKeys()));
case "TagValues":
return Optional.ofNullable(clazz.cast(tagValues()));
case "ClusterExists":
return Optional.ofNullable(clazz.cast(clusterExists()));
case "SortingEntities":
return Optional.ofNullable(clazz.cast(sortingEntities()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function