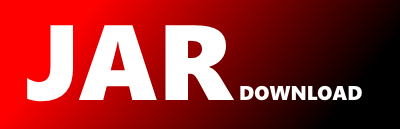
software.amazon.awssdk.services.redshift.model.GetClusterCredentialsRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The request parameters to get cluster credentials.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetClusterCredentialsRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField DB_USER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DbUser")
.getter(getter(GetClusterCredentialsRequest::dbUser)).setter(setter(Builder::dbUser))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbUser").build()).build();
private static final SdkField DB_NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DbName")
.getter(getter(GetClusterCredentialsRequest::dbName)).setter(setter(Builder::dbName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbName").build()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(GetClusterCredentialsRequest::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DurationSeconds").getter(getter(GetClusterCredentialsRequest::durationSeconds))
.setter(setter(Builder::durationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DurationSeconds").build()).build();
private static final SdkField AUTO_CREATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoCreate").getter(getter(GetClusterCredentialsRequest::autoCreate))
.setter(setter(Builder::autoCreate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoCreate").build()).build();
private static final SdkField> DB_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DbGroups")
.getter(getter(GetClusterCredentialsRequest::dbGroups))
.setter(setter(Builder::dbGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbGroups").build(),
ListTrait
.builder()
.memberLocationName("DbGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DbGroup").build()).build()).build()).build();
private static final SdkField CUSTOM_DOMAIN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomDomainName").getter(getter(GetClusterCredentialsRequest::customDomainName))
.setter(setter(Builder::customDomainName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomDomainName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_USER_FIELD, DB_NAME_FIELD,
CLUSTER_IDENTIFIER_FIELD, DURATION_SECONDS_FIELD, AUTO_CREATE_FIELD, DB_GROUPS_FIELD, CUSTOM_DOMAIN_NAME_FIELD));
private final String dbUser;
private final String dbName;
private final String clusterIdentifier;
private final Integer durationSeconds;
private final Boolean autoCreate;
private final List dbGroups;
private final String customDomainName;
private GetClusterCredentialsRequest(BuilderImpl builder) {
super(builder);
this.dbUser = builder.dbUser;
this.dbName = builder.dbName;
this.clusterIdentifier = builder.clusterIdentifier;
this.durationSeconds = builder.durationSeconds;
this.autoCreate = builder.autoCreate;
this.dbGroups = builder.dbGroups;
this.customDomainName = builder.customDomainName;
}
/**
*
* The name of a database user. If a user name matching DbUser
exists in the database, the temporary
* user credentials have the same permissions as the existing user. If DbUser
doesn't exist in the
* database and Autocreate
is True
, a new user is created using the value for
* DbUser
with PUBLIC permissions. If a database user matching the value for DbUser
* doesn't exist and Autocreate
is False
, then the command succeeds but the connection
* attempt will fail because the user doesn't exist in the database.
*
*
* For more information, see CREATE
* USER in the Amazon Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @return The name of a database user. If a user name matching DbUser
exists in the database, the
* temporary user credentials have the same permissions as the existing user. If DbUser
doesn't
* exist in the database and Autocreate
is True
, a new user is created using the
* value for DbUser
with PUBLIC permissions. If a database user matching the value for
* DbUser
doesn't exist and Autocreate
is False
, then the command
* succeeds but the connection attempt will fail because the user doesn't exist in the database.
*
* For more information, see CREATE USER in the Amazon
* Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public final String dbUser() {
return dbUser;
}
/**
*
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @return The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public final String dbName() {
return dbName;
}
/**
*
* The unique identifier of the cluster that contains the database for which you are requesting credentials. This
* parameter is case sensitive.
*
*
* @return The unique identifier of the cluster that contains the database for which you are requesting credentials.
* This parameter is case sensitive.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The number of seconds until the returned temporary password expires.
*
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*
*
* @return The number of seconds until the returned temporary password expires.
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*/
public final Integer durationSeconds() {
return durationSeconds;
}
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*
* @return Create a database user with the name specified for the user named in DbUser
if one does not
* exist.
*/
public final Boolean autoCreate() {
return autoCreate;
}
/**
* For responses, this returns true if the service returned a value for the DbGroups property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDbGroups() {
return dbGroups != null && !(dbGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDbGroups} method.
*
*
* @return A list of the names of existing database groups that the user named in DbUser
will join for
* the current session, in addition to any group memberships for an existing user. If not specified, a new
* user is added only to PUBLIC.
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public final List dbGroups() {
return dbGroups;
}
/**
*
* The custom domain name for the cluster credentials.
*
*
* @return The custom domain name for the cluster credentials.
*/
public final String customDomainName() {
return customDomainName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dbUser());
hashCode = 31 * hashCode + Objects.hashCode(dbName());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(durationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(autoCreate());
hashCode = 31 * hashCode + Objects.hashCode(hasDbGroups() ? dbGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(customDomainName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetClusterCredentialsRequest)) {
return false;
}
GetClusterCredentialsRequest other = (GetClusterCredentialsRequest) obj;
return Objects.equals(dbUser(), other.dbUser()) && Objects.equals(dbName(), other.dbName())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(durationSeconds(), other.durationSeconds()) && Objects.equals(autoCreate(), other.autoCreate())
&& hasDbGroups() == other.hasDbGroups() && Objects.equals(dbGroups(), other.dbGroups())
&& Objects.equals(customDomainName(), other.customDomainName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetClusterCredentialsRequest").add("DbUser", dbUser()).add("DbName", dbName())
.add("ClusterIdentifier", clusterIdentifier()).add("DurationSeconds", durationSeconds())
.add("AutoCreate", autoCreate()).add("DbGroups", hasDbGroups() ? dbGroups() : null)
.add("CustomDomainName", customDomainName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DbUser":
return Optional.ofNullable(clazz.cast(dbUser()));
case "DbName":
return Optional.ofNullable(clazz.cast(dbName()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "DurationSeconds":
return Optional.ofNullable(clazz.cast(durationSeconds()));
case "AutoCreate":
return Optional.ofNullable(clazz.cast(autoCreate()));
case "DbGroups":
return Optional.ofNullable(clazz.cast(dbGroups()));
case "CustomDomainName":
return Optional.ofNullable(clazz.cast(customDomainName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function