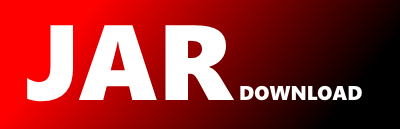
software.amazon.awssdk.services.redshift.DefaultRedshiftAsyncClient Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.redshift.internal.RedshiftServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.redshift.model.AcceptReservedNodeExchangeRequest;
import software.amazon.awssdk.services.redshift.model.AcceptReservedNodeExchangeResponse;
import software.amazon.awssdk.services.redshift.model.AccessToClusterDeniedException;
import software.amazon.awssdk.services.redshift.model.AccessToSnapshotDeniedException;
import software.amazon.awssdk.services.redshift.model.AddPartnerRequest;
import software.amazon.awssdk.services.redshift.model.AddPartnerResponse;
import software.amazon.awssdk.services.redshift.model.AssociateDataShareConsumerRequest;
import software.amazon.awssdk.services.redshift.model.AssociateDataShareConsumerResponse;
import software.amazon.awssdk.services.redshift.model.AuthenticationProfileAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.AuthenticationProfileNotFoundException;
import software.amazon.awssdk.services.redshift.model.AuthenticationProfileQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.AuthorizationAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.AuthorizationNotFoundException;
import software.amazon.awssdk.services.redshift.model.AuthorizationQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.AuthorizeClusterSecurityGroupIngressRequest;
import software.amazon.awssdk.services.redshift.model.AuthorizeClusterSecurityGroupIngressResponse;
import software.amazon.awssdk.services.redshift.model.AuthorizeDataShareRequest;
import software.amazon.awssdk.services.redshift.model.AuthorizeDataShareResponse;
import software.amazon.awssdk.services.redshift.model.AuthorizeEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.AuthorizeEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.AuthorizeSnapshotAccessRequest;
import software.amazon.awssdk.services.redshift.model.AuthorizeSnapshotAccessResponse;
import software.amazon.awssdk.services.redshift.model.BatchDeleteClusterSnapshotsRequest;
import software.amazon.awssdk.services.redshift.model.BatchDeleteClusterSnapshotsResponse;
import software.amazon.awssdk.services.redshift.model.BatchDeleteRequestSizeExceededException;
import software.amazon.awssdk.services.redshift.model.BatchModifyClusterSnapshotsLimitExceededException;
import software.amazon.awssdk.services.redshift.model.BatchModifyClusterSnapshotsRequest;
import software.amazon.awssdk.services.redshift.model.BatchModifyClusterSnapshotsResponse;
import software.amazon.awssdk.services.redshift.model.BucketNotFoundException;
import software.amazon.awssdk.services.redshift.model.CancelResizeRequest;
import software.amazon.awssdk.services.redshift.model.CancelResizeResponse;
import software.amazon.awssdk.services.redshift.model.ClusterAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ClusterNotFoundException;
import software.amazon.awssdk.services.redshift.model.ClusterOnLatestRevisionException;
import software.amazon.awssdk.services.redshift.model.ClusterParameterGroupAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ClusterParameterGroupNotFoundException;
import software.amazon.awssdk.services.redshift.model.ClusterParameterGroupQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ClusterQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ClusterSecurityGroupAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ClusterSecurityGroupNotFoundException;
import software.amazon.awssdk.services.redshift.model.ClusterSecurityGroupQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ClusterSnapshotAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ClusterSnapshotNotFoundException;
import software.amazon.awssdk.services.redshift.model.ClusterSnapshotQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ClusterSubnetGroupAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ClusterSubnetGroupNotFoundException;
import software.amazon.awssdk.services.redshift.model.ClusterSubnetGroupQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ClusterSubnetQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ConflictPolicyUpdateException;
import software.amazon.awssdk.services.redshift.model.CopyClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.CopyClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.CopyToRegionDisabledException;
import software.amazon.awssdk.services.redshift.model.CreateAuthenticationProfileRequest;
import software.amazon.awssdk.services.redshift.model.CreateAuthenticationProfileResponse;
import software.amazon.awssdk.services.redshift.model.CreateClusterParameterGroupRequest;
import software.amazon.awssdk.services.redshift.model.CreateClusterParameterGroupResponse;
import software.amazon.awssdk.services.redshift.model.CreateClusterRequest;
import software.amazon.awssdk.services.redshift.model.CreateClusterResponse;
import software.amazon.awssdk.services.redshift.model.CreateClusterSecurityGroupRequest;
import software.amazon.awssdk.services.redshift.model.CreateClusterSecurityGroupResponse;
import software.amazon.awssdk.services.redshift.model.CreateClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.CreateClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.CreateClusterSubnetGroupRequest;
import software.amazon.awssdk.services.redshift.model.CreateClusterSubnetGroupResponse;
import software.amazon.awssdk.services.redshift.model.CreateCustomDomainAssociationRequest;
import software.amazon.awssdk.services.redshift.model.CreateCustomDomainAssociationResponse;
import software.amazon.awssdk.services.redshift.model.CreateEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.CreateEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.CreateEventSubscriptionRequest;
import software.amazon.awssdk.services.redshift.model.CreateEventSubscriptionResponse;
import software.amazon.awssdk.services.redshift.model.CreateHsmClientCertificateRequest;
import software.amazon.awssdk.services.redshift.model.CreateHsmClientCertificateResponse;
import software.amazon.awssdk.services.redshift.model.CreateHsmConfigurationRequest;
import software.amazon.awssdk.services.redshift.model.CreateHsmConfigurationResponse;
import software.amazon.awssdk.services.redshift.model.CreateRedshiftIdcApplicationRequest;
import software.amazon.awssdk.services.redshift.model.CreateRedshiftIdcApplicationResponse;
import software.amazon.awssdk.services.redshift.model.CreateScheduledActionRequest;
import software.amazon.awssdk.services.redshift.model.CreateScheduledActionResponse;
import software.amazon.awssdk.services.redshift.model.CreateSnapshotCopyGrantRequest;
import software.amazon.awssdk.services.redshift.model.CreateSnapshotCopyGrantResponse;
import software.amazon.awssdk.services.redshift.model.CreateSnapshotScheduleRequest;
import software.amazon.awssdk.services.redshift.model.CreateSnapshotScheduleResponse;
import software.amazon.awssdk.services.redshift.model.CreateTagsRequest;
import software.amazon.awssdk.services.redshift.model.CreateTagsResponse;
import software.amazon.awssdk.services.redshift.model.CreateUsageLimitRequest;
import software.amazon.awssdk.services.redshift.model.CreateUsageLimitResponse;
import software.amazon.awssdk.services.redshift.model.CustomCnameAssociationException;
import software.amazon.awssdk.services.redshift.model.CustomDomainAssociationNotFoundException;
import software.amazon.awssdk.services.redshift.model.DeauthorizeDataShareRequest;
import software.amazon.awssdk.services.redshift.model.DeauthorizeDataShareResponse;
import software.amazon.awssdk.services.redshift.model.DeleteAuthenticationProfileRequest;
import software.amazon.awssdk.services.redshift.model.DeleteAuthenticationProfileResponse;
import software.amazon.awssdk.services.redshift.model.DeleteClusterParameterGroupRequest;
import software.amazon.awssdk.services.redshift.model.DeleteClusterParameterGroupResponse;
import software.amazon.awssdk.services.redshift.model.DeleteClusterRequest;
import software.amazon.awssdk.services.redshift.model.DeleteClusterResponse;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSecurityGroupRequest;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSecurityGroupResponse;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSubnetGroupRequest;
import software.amazon.awssdk.services.redshift.model.DeleteClusterSubnetGroupResponse;
import software.amazon.awssdk.services.redshift.model.DeleteCustomDomainAssociationRequest;
import software.amazon.awssdk.services.redshift.model.DeleteCustomDomainAssociationResponse;
import software.amazon.awssdk.services.redshift.model.DeleteEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.DeleteEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.DeleteEventSubscriptionRequest;
import software.amazon.awssdk.services.redshift.model.DeleteEventSubscriptionResponse;
import software.amazon.awssdk.services.redshift.model.DeleteHsmClientCertificateRequest;
import software.amazon.awssdk.services.redshift.model.DeleteHsmClientCertificateResponse;
import software.amazon.awssdk.services.redshift.model.DeleteHsmConfigurationRequest;
import software.amazon.awssdk.services.redshift.model.DeleteHsmConfigurationResponse;
import software.amazon.awssdk.services.redshift.model.DeletePartnerRequest;
import software.amazon.awssdk.services.redshift.model.DeletePartnerResponse;
import software.amazon.awssdk.services.redshift.model.DeleteRedshiftIdcApplicationRequest;
import software.amazon.awssdk.services.redshift.model.DeleteRedshiftIdcApplicationResponse;
import software.amazon.awssdk.services.redshift.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.redshift.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.redshift.model.DeleteScheduledActionRequest;
import software.amazon.awssdk.services.redshift.model.DeleteScheduledActionResponse;
import software.amazon.awssdk.services.redshift.model.DeleteSnapshotCopyGrantRequest;
import software.amazon.awssdk.services.redshift.model.DeleteSnapshotCopyGrantResponse;
import software.amazon.awssdk.services.redshift.model.DeleteSnapshotScheduleRequest;
import software.amazon.awssdk.services.redshift.model.DeleteSnapshotScheduleResponse;
import software.amazon.awssdk.services.redshift.model.DeleteTagsRequest;
import software.amazon.awssdk.services.redshift.model.DeleteTagsResponse;
import software.amazon.awssdk.services.redshift.model.DeleteUsageLimitRequest;
import software.amazon.awssdk.services.redshift.model.DeleteUsageLimitResponse;
import software.amazon.awssdk.services.redshift.model.DependentServiceAccessDeniedException;
import software.amazon.awssdk.services.redshift.model.DependentServiceRequestThrottlingException;
import software.amazon.awssdk.services.redshift.model.DependentServiceUnavailableException;
import software.amazon.awssdk.services.redshift.model.DescribeAccountAttributesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeAccountAttributesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeAuthenticationProfilesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeAuthenticationProfilesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterDbRevisionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterDbRevisionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterParameterGroupsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterParameterGroupsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterParametersRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterParametersResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSecurityGroupsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSecurityGroupsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSnapshotsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSnapshotsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSubnetGroupsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterSubnetGroupsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterTracksRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterTracksResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClusterVersionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClusterVersionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeClustersRequest;
import software.amazon.awssdk.services.redshift.model.DescribeClustersResponse;
import software.amazon.awssdk.services.redshift.model.DescribeCustomDomainAssociationsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeCustomDomainAssociationsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesForConsumerRequest;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesForConsumerResponse;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesForProducerRequest;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesForProducerResponse;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeDataSharesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeDefaultClusterParametersRequest;
import software.amazon.awssdk.services.redshift.model.DescribeDefaultClusterParametersResponse;
import software.amazon.awssdk.services.redshift.model.DescribeEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.DescribeEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.DescribeEndpointAuthorizationRequest;
import software.amazon.awssdk.services.redshift.model.DescribeEndpointAuthorizationResponse;
import software.amazon.awssdk.services.redshift.model.DescribeEventCategoriesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeEventCategoriesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeEventSubscriptionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeEventSubscriptionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeEventsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeEventsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeHsmClientCertificatesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeHsmClientCertificatesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeHsmConfigurationsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeHsmConfigurationsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeInboundIntegrationsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeInboundIntegrationsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeLoggingStatusRequest;
import software.amazon.awssdk.services.redshift.model.DescribeLoggingStatusResponse;
import software.amazon.awssdk.services.redshift.model.DescribeNodeConfigurationOptionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeNodeConfigurationOptionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeOrderableClusterOptionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeOrderableClusterOptionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribePartnersRequest;
import software.amazon.awssdk.services.redshift.model.DescribePartnersResponse;
import software.amazon.awssdk.services.redshift.model.DescribeRedshiftIdcApplicationsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeRedshiftIdcApplicationsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodeExchangeStatusRequest;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodeExchangeStatusResponse;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodeOfferingsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodeOfferingsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeReservedNodesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeResizeRequest;
import software.amazon.awssdk.services.redshift.model.DescribeResizeResponse;
import software.amazon.awssdk.services.redshift.model.DescribeScheduledActionsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeScheduledActionsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeSnapshotCopyGrantsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeSnapshotCopyGrantsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeSnapshotSchedulesRequest;
import software.amazon.awssdk.services.redshift.model.DescribeSnapshotSchedulesResponse;
import software.amazon.awssdk.services.redshift.model.DescribeStorageRequest;
import software.amazon.awssdk.services.redshift.model.DescribeStorageResponse;
import software.amazon.awssdk.services.redshift.model.DescribeTableRestoreStatusRequest;
import software.amazon.awssdk.services.redshift.model.DescribeTableRestoreStatusResponse;
import software.amazon.awssdk.services.redshift.model.DescribeTagsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeTagsResponse;
import software.amazon.awssdk.services.redshift.model.DescribeUsageLimitsRequest;
import software.amazon.awssdk.services.redshift.model.DescribeUsageLimitsResponse;
import software.amazon.awssdk.services.redshift.model.DisableLoggingRequest;
import software.amazon.awssdk.services.redshift.model.DisableLoggingResponse;
import software.amazon.awssdk.services.redshift.model.DisableSnapshotCopyRequest;
import software.amazon.awssdk.services.redshift.model.DisableSnapshotCopyResponse;
import software.amazon.awssdk.services.redshift.model.DisassociateDataShareConsumerRequest;
import software.amazon.awssdk.services.redshift.model.DisassociateDataShareConsumerResponse;
import software.amazon.awssdk.services.redshift.model.EnableLoggingRequest;
import software.amazon.awssdk.services.redshift.model.EnableLoggingResponse;
import software.amazon.awssdk.services.redshift.model.EnableSnapshotCopyRequest;
import software.amazon.awssdk.services.redshift.model.EnableSnapshotCopyResponse;
import software.amazon.awssdk.services.redshift.model.EndpointAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.EndpointAuthorizationAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.EndpointAuthorizationNotFoundException;
import software.amazon.awssdk.services.redshift.model.EndpointAuthorizationsPerClusterLimitExceededException;
import software.amazon.awssdk.services.redshift.model.EndpointNotFoundException;
import software.amazon.awssdk.services.redshift.model.EndpointsPerAuthorizationLimitExceededException;
import software.amazon.awssdk.services.redshift.model.EndpointsPerClusterLimitExceededException;
import software.amazon.awssdk.services.redshift.model.EventSubscriptionQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.FailoverPrimaryComputeRequest;
import software.amazon.awssdk.services.redshift.model.FailoverPrimaryComputeResponse;
import software.amazon.awssdk.services.redshift.model.GetClusterCredentialsRequest;
import software.amazon.awssdk.services.redshift.model.GetClusterCredentialsResponse;
import software.amazon.awssdk.services.redshift.model.GetClusterCredentialsWithIamRequest;
import software.amazon.awssdk.services.redshift.model.GetClusterCredentialsWithIamResponse;
import software.amazon.awssdk.services.redshift.model.GetReservedNodeExchangeConfigurationOptionsRequest;
import software.amazon.awssdk.services.redshift.model.GetReservedNodeExchangeConfigurationOptionsResponse;
import software.amazon.awssdk.services.redshift.model.GetReservedNodeExchangeOfferingsRequest;
import software.amazon.awssdk.services.redshift.model.GetReservedNodeExchangeOfferingsResponse;
import software.amazon.awssdk.services.redshift.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.redshift.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.redshift.model.HsmClientCertificateAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.HsmClientCertificateNotFoundException;
import software.amazon.awssdk.services.redshift.model.HsmClientCertificateQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.HsmConfigurationAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.HsmConfigurationNotFoundException;
import software.amazon.awssdk.services.redshift.model.HsmConfigurationQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.InProgressTableRestoreQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.IncompatibleOrderableOptionsException;
import software.amazon.awssdk.services.redshift.model.InsufficientClusterCapacityException;
import software.amazon.awssdk.services.redshift.model.InsufficientS3BucketPolicyException;
import software.amazon.awssdk.services.redshift.model.IntegrationNotFoundException;
import software.amazon.awssdk.services.redshift.model.InvalidAuthenticationProfileRequestException;
import software.amazon.awssdk.services.redshift.model.InvalidAuthorizationStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterParameterGroupStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterSecurityGroupStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterSnapshotScheduleStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterSnapshotStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterSubnetGroupStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterSubnetStateException;
import software.amazon.awssdk.services.redshift.model.InvalidClusterTrackException;
import software.amazon.awssdk.services.redshift.model.InvalidDataShareException;
import software.amazon.awssdk.services.redshift.model.InvalidElasticIpException;
import software.amazon.awssdk.services.redshift.model.InvalidEndpointStateException;
import software.amazon.awssdk.services.redshift.model.InvalidHsmClientCertificateStateException;
import software.amazon.awssdk.services.redshift.model.InvalidHsmConfigurationStateException;
import software.amazon.awssdk.services.redshift.model.InvalidNamespaceException;
import software.amazon.awssdk.services.redshift.model.InvalidPolicyException;
import software.amazon.awssdk.services.redshift.model.InvalidReservedNodeStateException;
import software.amazon.awssdk.services.redshift.model.InvalidRestoreException;
import software.amazon.awssdk.services.redshift.model.InvalidRetentionPeriodException;
import software.amazon.awssdk.services.redshift.model.InvalidS3BucketNameException;
import software.amazon.awssdk.services.redshift.model.InvalidS3KeyPrefixException;
import software.amazon.awssdk.services.redshift.model.InvalidScheduleException;
import software.amazon.awssdk.services.redshift.model.InvalidScheduledActionException;
import software.amazon.awssdk.services.redshift.model.InvalidSnapshotCopyGrantStateException;
import software.amazon.awssdk.services.redshift.model.InvalidSubnetException;
import software.amazon.awssdk.services.redshift.model.InvalidSubscriptionStateException;
import software.amazon.awssdk.services.redshift.model.InvalidTableRestoreArgumentException;
import software.amazon.awssdk.services.redshift.model.InvalidTagException;
import software.amazon.awssdk.services.redshift.model.InvalidUsageLimitException;
import software.amazon.awssdk.services.redshift.model.InvalidVpcNetworkStateException;
import software.amazon.awssdk.services.redshift.model.Ipv6CidrBlockNotFoundException;
import software.amazon.awssdk.services.redshift.model.LimitExceededException;
import software.amazon.awssdk.services.redshift.model.ListRecommendationsRequest;
import software.amazon.awssdk.services.redshift.model.ListRecommendationsResponse;
import software.amazon.awssdk.services.redshift.model.ModifyAquaConfigurationRequest;
import software.amazon.awssdk.services.redshift.model.ModifyAquaConfigurationResponse;
import software.amazon.awssdk.services.redshift.model.ModifyAuthenticationProfileRequest;
import software.amazon.awssdk.services.redshift.model.ModifyAuthenticationProfileResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterDbRevisionRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterDbRevisionResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterIamRolesRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterIamRolesResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterMaintenanceRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterMaintenanceResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterParameterGroupRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterParameterGroupResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSnapshotScheduleRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSnapshotScheduleResponse;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSubnetGroupRequest;
import software.amazon.awssdk.services.redshift.model.ModifyClusterSubnetGroupResponse;
import software.amazon.awssdk.services.redshift.model.ModifyCustomDomainAssociationRequest;
import software.amazon.awssdk.services.redshift.model.ModifyCustomDomainAssociationResponse;
import software.amazon.awssdk.services.redshift.model.ModifyEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.ModifyEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.ModifyEventSubscriptionRequest;
import software.amazon.awssdk.services.redshift.model.ModifyEventSubscriptionResponse;
import software.amazon.awssdk.services.redshift.model.ModifyRedshiftIdcApplicationRequest;
import software.amazon.awssdk.services.redshift.model.ModifyRedshiftIdcApplicationResponse;
import software.amazon.awssdk.services.redshift.model.ModifyScheduledActionRequest;
import software.amazon.awssdk.services.redshift.model.ModifyScheduledActionResponse;
import software.amazon.awssdk.services.redshift.model.ModifySnapshotCopyRetentionPeriodRequest;
import software.amazon.awssdk.services.redshift.model.ModifySnapshotCopyRetentionPeriodResponse;
import software.amazon.awssdk.services.redshift.model.ModifySnapshotScheduleRequest;
import software.amazon.awssdk.services.redshift.model.ModifySnapshotScheduleResponse;
import software.amazon.awssdk.services.redshift.model.ModifyUsageLimitRequest;
import software.amazon.awssdk.services.redshift.model.ModifyUsageLimitResponse;
import software.amazon.awssdk.services.redshift.model.NumberOfNodesPerClusterLimitExceededException;
import software.amazon.awssdk.services.redshift.model.NumberOfNodesQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.PartnerNotFoundException;
import software.amazon.awssdk.services.redshift.model.PauseClusterRequest;
import software.amazon.awssdk.services.redshift.model.PauseClusterResponse;
import software.amazon.awssdk.services.redshift.model.PurchaseReservedNodeOfferingRequest;
import software.amazon.awssdk.services.redshift.model.PurchaseReservedNodeOfferingResponse;
import software.amazon.awssdk.services.redshift.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.redshift.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.redshift.model.RebootClusterRequest;
import software.amazon.awssdk.services.redshift.model.RebootClusterResponse;
import software.amazon.awssdk.services.redshift.model.RedshiftException;
import software.amazon.awssdk.services.redshift.model.RedshiftIdcApplicationAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.RedshiftIdcApplicationNotExistsException;
import software.amazon.awssdk.services.redshift.model.RedshiftIdcApplicationQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.RejectDataShareRequest;
import software.amazon.awssdk.services.redshift.model.RejectDataShareResponse;
import software.amazon.awssdk.services.redshift.model.ReservedNodeAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ReservedNodeAlreadyMigratedException;
import software.amazon.awssdk.services.redshift.model.ReservedNodeExchangeNotFoundException;
import software.amazon.awssdk.services.redshift.model.ReservedNodeNotFoundException;
import software.amazon.awssdk.services.redshift.model.ReservedNodeOfferingNotFoundException;
import software.amazon.awssdk.services.redshift.model.ReservedNodeQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ResetClusterParameterGroupRequest;
import software.amazon.awssdk.services.redshift.model.ResetClusterParameterGroupResponse;
import software.amazon.awssdk.services.redshift.model.ResizeClusterRequest;
import software.amazon.awssdk.services.redshift.model.ResizeClusterResponse;
import software.amazon.awssdk.services.redshift.model.ResizeNotFoundException;
import software.amazon.awssdk.services.redshift.model.ResourceNotFoundException;
import software.amazon.awssdk.services.redshift.model.RestoreFromClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.RestoreFromClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.RestoreTableFromClusterSnapshotRequest;
import software.amazon.awssdk.services.redshift.model.RestoreTableFromClusterSnapshotResponse;
import software.amazon.awssdk.services.redshift.model.ResumeClusterRequest;
import software.amazon.awssdk.services.redshift.model.ResumeClusterResponse;
import software.amazon.awssdk.services.redshift.model.RevokeClusterSecurityGroupIngressRequest;
import software.amazon.awssdk.services.redshift.model.RevokeClusterSecurityGroupIngressResponse;
import software.amazon.awssdk.services.redshift.model.RevokeEndpointAccessRequest;
import software.amazon.awssdk.services.redshift.model.RevokeEndpointAccessResponse;
import software.amazon.awssdk.services.redshift.model.RevokeSnapshotAccessRequest;
import software.amazon.awssdk.services.redshift.model.RevokeSnapshotAccessResponse;
import software.amazon.awssdk.services.redshift.model.RotateEncryptionKeyRequest;
import software.amazon.awssdk.services.redshift.model.RotateEncryptionKeyResponse;
import software.amazon.awssdk.services.redshift.model.ScheduleDefinitionTypeUnsupportedException;
import software.amazon.awssdk.services.redshift.model.ScheduledActionAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.ScheduledActionNotFoundException;
import software.amazon.awssdk.services.redshift.model.ScheduledActionQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.ScheduledActionTypeUnsupportedException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyAlreadyDisabledException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyAlreadyEnabledException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyDisabledException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyGrantAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyGrantNotFoundException;
import software.amazon.awssdk.services.redshift.model.SnapshotCopyGrantQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.SnapshotScheduleAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.SnapshotScheduleNotFoundException;
import software.amazon.awssdk.services.redshift.model.SnapshotScheduleQuotaExceededException;
import software.amazon.awssdk.services.redshift.model.SnapshotScheduleUpdateInProgressException;
import software.amazon.awssdk.services.redshift.model.SnsInvalidTopicException;
import software.amazon.awssdk.services.redshift.model.SnsNoAuthorizationException;
import software.amazon.awssdk.services.redshift.model.SnsTopicArnNotFoundException;
import software.amazon.awssdk.services.redshift.model.SourceNotFoundException;
import software.amazon.awssdk.services.redshift.model.SubnetAlreadyInUseException;
import software.amazon.awssdk.services.redshift.model.SubscriptionAlreadyExistException;
import software.amazon.awssdk.services.redshift.model.SubscriptionCategoryNotFoundException;
import software.amazon.awssdk.services.redshift.model.SubscriptionEventIdNotFoundException;
import software.amazon.awssdk.services.redshift.model.SubscriptionNotFoundException;
import software.amazon.awssdk.services.redshift.model.SubscriptionSeverityNotFoundException;
import software.amazon.awssdk.services.redshift.model.TableLimitExceededException;
import software.amazon.awssdk.services.redshift.model.TableRestoreNotFoundException;
import software.amazon.awssdk.services.redshift.model.TagLimitExceededException;
import software.amazon.awssdk.services.redshift.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.redshift.model.UnauthorizedPartnerIntegrationException;
import software.amazon.awssdk.services.redshift.model.UnknownSnapshotCopyRegionException;
import software.amazon.awssdk.services.redshift.model.UnsupportedOperationException;
import software.amazon.awssdk.services.redshift.model.UnsupportedOptionException;
import software.amazon.awssdk.services.redshift.model.UpdatePartnerStatusRequest;
import software.amazon.awssdk.services.redshift.model.UpdatePartnerStatusResponse;
import software.amazon.awssdk.services.redshift.model.UsageLimitAlreadyExistsException;
import software.amazon.awssdk.services.redshift.model.UsageLimitNotFoundException;
import software.amazon.awssdk.services.redshift.transform.AcceptReservedNodeExchangeRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AddPartnerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AssociateDataShareConsumerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AuthorizeClusterSecurityGroupIngressRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AuthorizeDataShareRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AuthorizeEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.AuthorizeSnapshotAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.BatchDeleteClusterSnapshotsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.BatchModifyClusterSnapshotsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CancelResizeRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CopyClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateAuthenticationProfileRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateClusterParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateClusterSecurityGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateClusterSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateCustomDomainAssociationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateEventSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateHsmClientCertificateRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateHsmConfigurationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateRedshiftIdcApplicationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateScheduledActionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateSnapshotCopyGrantRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateSnapshotScheduleRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateTagsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.CreateUsageLimitRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeauthorizeDataShareRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteAuthenticationProfileRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteClusterParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteClusterSecurityGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteClusterSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteCustomDomainAssociationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteEventSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteHsmClientCertificateRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteHsmConfigurationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeletePartnerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteRedshiftIdcApplicationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteScheduledActionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteSnapshotCopyGrantRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteSnapshotScheduleRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteTagsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DeleteUsageLimitRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeAccountAttributesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeAuthenticationProfilesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterDbRevisionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterParameterGroupsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterParametersRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterSecurityGroupsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterSnapshotsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterSubnetGroupsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterTracksRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClusterVersionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeClustersRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeCustomDomainAssociationsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeDataSharesForConsumerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeDataSharesForProducerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeDataSharesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeDefaultClusterParametersRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeEndpointAuthorizationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeEventCategoriesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeEventSubscriptionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeEventsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeHsmClientCertificatesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeHsmConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeInboundIntegrationsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeLoggingStatusRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeNodeConfigurationOptionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeOrderableClusterOptionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribePartnersRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeRedshiftIdcApplicationsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeReservedNodeExchangeStatusRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeReservedNodeOfferingsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeReservedNodesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeResizeRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeScheduledActionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeSnapshotCopyGrantsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeSnapshotSchedulesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeStorageRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeTableRestoreStatusRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DescribeUsageLimitsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DisableLoggingRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DisableSnapshotCopyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.DisassociateDataShareConsumerRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.EnableLoggingRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.EnableSnapshotCopyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.FailoverPrimaryComputeRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.GetClusterCredentialsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.GetClusterCredentialsWithIamRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.GetReservedNodeExchangeConfigurationOptionsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.GetReservedNodeExchangeOfferingsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.GetResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ListRecommendationsRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyAquaConfigurationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyAuthenticationProfileRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterDbRevisionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterIamRolesRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterMaintenanceRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterSnapshotScheduleRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyClusterSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyCustomDomainAssociationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyEventSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyRedshiftIdcApplicationRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyScheduledActionRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifySnapshotCopyRetentionPeriodRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifySnapshotScheduleRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ModifyUsageLimitRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.PauseClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.PurchaseReservedNodeOfferingRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.PutResourcePolicyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RebootClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RejectDataShareRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ResetClusterParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ResizeClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RestoreFromClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RestoreTableFromClusterSnapshotRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.ResumeClusterRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RevokeClusterSecurityGroupIngressRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RevokeEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RevokeSnapshotAccessRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.RotateEncryptionKeyRequestMarshaller;
import software.amazon.awssdk.services.redshift.transform.UpdatePartnerStatusRequestMarshaller;
import software.amazon.awssdk.services.redshift.waiters.RedshiftAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link RedshiftAsyncClient}.
*
* @see RedshiftAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultRedshiftAsyncClient implements RedshiftAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultRedshiftAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final AsyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultRedshiftAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Exchanges a DC1 Reserved Node for a DC2 Reserved Node with no changes to the configuration (term, payment type,
* or number of nodes) and no additional costs.
*
*
* @param acceptReservedNodeExchangeRequest
* @return A Java Future containing the result of the AcceptReservedNodeExchange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ReservedNodeNotFoundException The specified reserved compute node not found.
* - InvalidReservedNodeStateException Indicates that the Reserved Node being exchanged is not in an
* active state.
* - ReservedNodeAlreadyMigratedException Indicates that the reserved node has already been exchanged.
* - ReservedNodeOfferingNotFoundException Specified offering does not exist.
* - UnsupportedOperationException The requested operation isn't supported.
* - DependentServiceUnavailableException Your request cannot be completed because a dependent internal
* service is temporarily unavailable. Wait 30 to 60 seconds and try again.
* - ReservedNodeAlreadyExistsException User already has a reservation with the given identifier.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AcceptReservedNodeExchange
* @see AWS API Documentation
*/
@Override
public CompletableFuture acceptReservedNodeExchange(
AcceptReservedNodeExchangeRequest acceptReservedNodeExchangeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(acceptReservedNodeExchangeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, acceptReservedNodeExchangeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AcceptReservedNodeExchange");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AcceptReservedNodeExchangeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AcceptReservedNodeExchange").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AcceptReservedNodeExchangeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(acceptReservedNodeExchangeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds a partner integration to a cluster. This operation authorizes a partner to push status updates for the
* specified database. To complete the integration, you also set up the integration on the partner website.
*
*
* @param addPartnerRequest
* @return A Java Future containing the result of the AddPartner operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - PartnerNotFoundException The name of the partner was not found.
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - UnauthorizedPartnerIntegrationException The partner integration is not authorized.
* - UnsupportedOperationException The requested operation isn't supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AddPartner
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture addPartner(AddPartnerRequest addPartnerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addPartnerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addPartnerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddPartner");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AddPartnerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("AddPartner")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddPartnerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addPartnerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* From a datashare consumer account, associates a datashare with the account (AssociateEntireAccount) or the
* specified namespace (ConsumerArn). If you make this association, the consumer can consume the datashare.
*
*
* @param associateDataShareConsumerRequest
* @return A Java Future containing the result of the AssociateDataShareConsumer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidDataShareException There is an error with the datashare.
* - InvalidNamespaceException The namespace isn't valid because the namespace doesn't exist. Provide a
* valid namespace.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AssociateDataShareConsumer
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateDataShareConsumer(
AssociateDataShareConsumerRequest associateDataShareConsumerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateDataShareConsumerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateDataShareConsumerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateDataShareConsumer");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AssociateDataShareConsumerResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateDataShareConsumer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateDataShareConsumerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateDataShareConsumerRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group. Depending on whether the application
* accessing your cluster is running on the Internet or an Amazon EC2 instance, you can authorize inbound access to
* either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP) range or to an Amazon EC2 security group.
* You can add as many as 20 ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify EC2SecurityGroupName and
* EC2SecurityGroupOwnerId. The Amazon EC2 security group and Amazon Redshift cluster must be in the same
* Amazon Web Services Region.
*
*
* If you authorize access to a CIDR/IP address range, specify CIDRIP. For an overview of CIDR blocks, see
* the Wikipedia article on Classless
* Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients running on these IP addresses or the
* EC2 instance are authorized to connect to the cluster. For information about managing security groups, go to Working with Security
* Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeClusterSecurityGroupIngress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterSecurityGroupNotFoundException The cluster security group name does not refer to an existing
* cluster security group.
* - InvalidClusterSecurityGroupStateException The state of the cluster security group is not
*
available
.
* - AuthorizationAlreadyExistsException The specified CIDR block or EC2 security group is already
* authorized for the specified cluster security group.
* - AuthorizationQuotaExceededException The authorization quota for the cluster security group has been
* reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AuthorizeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
@Override
public CompletableFuture authorizeClusterSecurityGroupIngress(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeClusterSecurityGroupIngressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
authorizeClusterSecurityGroupIngressRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeClusterSecurityGroupIngress");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AuthorizeClusterSecurityGroupIngressResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeClusterSecurityGroupIngress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AuthorizeClusterSecurityGroupIngressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(authorizeClusterSecurityGroupIngressRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* From a data producer account, authorizes the sharing of a datashare with one or more consumer accounts or
* managing entities. To authorize a datashare for a data consumer, the producer account must have the correct
* access permissions.
*
*
* @param authorizeDataShareRequest
* @return A Java Future containing the result of the AuthorizeDataShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidDataShareException There is an error with the datashare.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AuthorizeDataShare
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture authorizeDataShare(AuthorizeDataShareRequest authorizeDataShareRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeDataShareRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, authorizeDataShareRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeDataShare");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AuthorizeDataShareResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeDataShare").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AuthorizeDataShareRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(authorizeDataShareRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Grants access to a cluster.
*
*
* @param authorizeEndpointAccessRequest
* @return A Java Future containing the result of the AuthorizeEndpointAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - EndpointAuthorizationsPerClusterLimitExceededException The number of endpoint authorizations per
* cluster has exceeded its limit.
* - UnsupportedOperationException The requested operation isn't supported.
* - EndpointAuthorizationAlreadyExistsException The authorization already exists for this endpoint.
* - InvalidAuthorizationStateException The status of the authorization is not valid.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AuthorizeEndpointAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture authorizeEndpointAccess(
AuthorizeEndpointAccessRequest authorizeEndpointAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeEndpointAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, authorizeEndpointAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeEndpointAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AuthorizeEndpointAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeEndpointAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AuthorizeEndpointAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(authorizeEndpointAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Authorizes the specified Amazon Web Services account to restore the specified snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @return A Java Future containing the result of the AuthorizeSnapshotAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterSnapshotNotFoundException The snapshot identifier does not refer to an existing cluster
* snapshot.
* - AuthorizationAlreadyExistsException The specified CIDR block or EC2 security group is already
* authorized for the specified cluster security group.
* - AuthorizationQuotaExceededException The authorization quota for the cluster security group has been
* reached.
* - DependentServiceRequestThrottlingException The request cannot be completed because a dependent
* service is throttling requests made by Amazon Redshift on your behalf. Wait and retry the request.
* - InvalidClusterSnapshotStateException The specified cluster snapshot is not in the
*
available
state, or other accounts are authorized to access the snapshot.
* - LimitExceededException The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* - UnsupportedOperationException The requested operation isn't supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.AuthorizeSnapshotAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture authorizeSnapshotAccess(
AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeSnapshotAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, authorizeSnapshotAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeSnapshotAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(AuthorizeSnapshotAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeSnapshotAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AuthorizeSnapshotAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(authorizeSnapshotAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a set of cluster snapshots.
*
*
* @param batchDeleteClusterSnapshotsRequest
* @return A Java Future containing the result of the BatchDeleteClusterSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BatchDeleteRequestSizeExceededException The maximum number for a batch delete of snapshots has been
* reached. The limit is 100.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.BatchDeleteClusterSnapshots
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchDeleteClusterSnapshots(
BatchDeleteClusterSnapshotsRequest batchDeleteClusterSnapshotsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteClusterSnapshotsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteClusterSnapshotsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteClusterSnapshots");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(BatchDeleteClusterSnapshotsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteClusterSnapshots").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchDeleteClusterSnapshotsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchDeleteClusterSnapshotsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies the settings for a set of cluster snapshots.
*
*
* @param batchModifyClusterSnapshotsRequest
* @return A Java Future containing the result of the BatchModifyClusterSnapshots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidRetentionPeriodException The retention period specified is either in the past or is not a
* valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* - BatchModifyClusterSnapshotsLimitExceededException The maximum number for snapshot identifiers has
* been reached. The limit is 100.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.BatchModifyClusterSnapshots
* @see AWS API Documentation
*/
@Override
public CompletableFuture batchModifyClusterSnapshots(
BatchModifyClusterSnapshotsRequest batchModifyClusterSnapshotsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchModifyClusterSnapshotsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchModifyClusterSnapshotsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchModifyClusterSnapshots");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(BatchModifyClusterSnapshotsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("BatchModifyClusterSnapshots").withProtocolMetadata(protocolMetadata)
.withMarshaller(new BatchModifyClusterSnapshotsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(batchModifyClusterSnapshotsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a resize operation for a cluster.
*
*
* @param cancelResizeRequest
* @return A Java Future containing the result of the CancelResize operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - ResizeNotFoundException A resize operation for the specified cluster is not found.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - UnsupportedOperationException The requested operation isn't supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CancelResize
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelResize(CancelResizeRequest cancelResizeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelResizeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelResizeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelResize");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CancelResizeResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelResize").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelResizeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelResizeRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster snapshot. The source must be an automated
* snapshot and it must be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated snapshots of the cluster. Also, when the
* retention period of the snapshot expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the snapshot. Manual snapshots are retained
* until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @return A Java Future containing the result of the CopyClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - ClusterSnapshotAlreadyExistsException The value specified as a snapshot identifier is already used by
* an existing snapshot.
* - ClusterSnapshotNotFoundException The snapshot identifier does not refer to an existing cluster
* snapshot.
* - InvalidClusterSnapshotStateException The specified cluster snapshot is not in the
*
available
state, or other accounts are authorized to access the snapshot.
* - ClusterSnapshotQuotaExceededException The request would result in the user exceeding the allowed
* number of cluster snapshots.
* - InvalidRetentionPeriodException The retention period specified is either in the past or is not a
* valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CopyClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture copyClusterSnapshot(
CopyClusterSnapshotRequest copyClusterSnapshotRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(copyClusterSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, copyClusterSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CopyClusterSnapshot");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CopyClusterSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CopyClusterSnapshot").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CopyClusterSnapshotRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(copyClusterSnapshotRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an authentication profile with the specified parameters.
*
*
* @param createAuthenticationProfileRequest
* @return A Java Future containing the result of the CreateAuthenticationProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthenticationProfileAlreadyExistsException The authentication profile already exists.
* - AuthenticationProfileQuotaExceededException The size or number of authentication profiles has
* exceeded the quota. The maximum length of the JSON string and maximum number of authentication profiles
* is determined by a quota for your account.
* - InvalidAuthenticationProfileRequestException The authentication profile request is not valid. The
* profile name can't be null or empty. The authentication profile API operation must be available in the
* Amazon Web Services Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateAuthenticationProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture createAuthenticationProfile(
CreateAuthenticationProfileRequest createAuthenticationProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAuthenticationProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAuthenticationProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAuthenticationProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateAuthenticationProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAuthenticationProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAuthenticationProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAuthenticationProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new cluster with the specified parameters.
*
*
* To create a cluster in Virtual Private Cloud (VPC), you must provide a cluster subnet group name. The cluster
* subnet group identifies the subnets of your VPC that Amazon Redshift uses when creating the cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterAlreadyExistsException The account already has a cluster with the given identifier.
* - InsufficientClusterCapacityException The number of nodes specified exceeds the allotted capacity of
* the cluster.
* - ClusterParameterGroupNotFoundException The parameter group name does not refer to an existing
* parameter group.
* - ClusterSecurityGroupNotFoundException The cluster security group name does not refer to an existing
* cluster security group.
* - ClusterQuotaExceededException The request would exceed the allowed number of cluster instances for
* this account. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - NumberOfNodesQuotaExceededException The operation would exceed the number of nodes allotted to the
* account. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - NumberOfNodesPerClusterLimitExceededException The operation would exceed the number of nodes allowed
* for a cluster.
* - ClusterSubnetGroupNotFoundException The cluster subnet group name does not refer to an existing
* cluster subnet group.
* - InvalidVpcNetworkStateException The cluster subnet group does not cover all Availability Zones.
* - InvalidClusterSubnetGroupStateException The cluster subnet group cannot be deleted because it is in
* use.
* - InvalidSubnetException The requested subnet is not valid, or not all of the subnets are in the same
* VPC.
* - UnauthorizedOperationException Your account is not authorized to perform the requested operation.
* - HsmClientCertificateNotFoundException There is no Amazon Redshift HSM client certificate with the
* specified identifier.
* - HsmConfigurationNotFoundException There is no Amazon Redshift HSM configuration with the specified
* identifier.
* - InvalidElasticIpException The Elastic IP (EIP) is invalid or cannot be found.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - LimitExceededException The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* - DependentServiceRequestThrottlingException The request cannot be completed because a dependent
* service is throttling requests made by Amazon Redshift on your behalf. Wait and retry the request.
* - InvalidClusterTrackException The provided cluster track name is not valid.
* - SnapshotScheduleNotFoundException We could not find the specified snapshot schedule.
* - InvalidRetentionPeriodException The retention period specified is either in the past or is not a
* valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* - Ipv6CidrBlockNotFoundException There are no subnets in your VPC with associated IPv6 CIDR blocks. To
* use dual-stack mode, associate an IPv6 CIDR block with each subnet in your VPC.
* - UnsupportedOperationException The requested operation isn't supported.
* - RedshiftIdcApplicationNotExistsException The application you attempted to find doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createCluster(CreateClusterRequest createClusterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCluster");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCluster").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can associate a cluster with a parameter group
* when you create the cluster. You can also associate an existing cluster with a parameter group after the cluster
* is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies to the databases you create on the
* cluster. For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @return A Java Future containing the result of the CreateClusterParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterParameterGroupQuotaExceededException The request would result in the user exceeding the
* allowed number of cluster parameter groups. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - ClusterParameterGroupAlreadyExistsException A cluster parameter group with the same name already
* exists.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture createClusterParameterGroup(
CreateClusterParameterGroupRequest createClusterParameterGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateClusterParameterGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateClusterParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateClusterParameterGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterParameterGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterParameterGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new Amazon Redshift security group. You use security groups to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @return A Java Future containing the result of the CreateClusterSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterSecurityGroupAlreadyExistsException A cluster security group with the same name already
* exists.
* - ClusterSecurityGroupQuotaExceededException The request would result in the user exceeding the allowed
* number of cluster security groups. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateClusterSecurityGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture createClusterSecurityGroup(
CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterSecurityGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterSecurityGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateClusterSecurityGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateClusterSecurityGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateClusterSecurityGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterSecurityGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterSecurityGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSnapshotRequest
* @return A Java Future containing the result of the CreateClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterSnapshotAlreadyExistsException The value specified as a snapshot identifier is already used by
* an existing snapshot.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - ClusterSnapshotQuotaExceededException The request would result in the user exceeding the allowed
* number of cluster snapshots.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - InvalidRetentionPeriodException The retention period specified is either in the past or is not a
* valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateClusterSnapshot
* @see AWS API Documentation
*/
@Override
public CompletableFuture createClusterSnapshot(
CreateClusterSnapshotRequest createClusterSnapshotRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateClusterSnapshot");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateClusterSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateClusterSnapshot").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterSnapshotRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterSnapshotRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of one or more subnets in your existing
* Amazon Virtual Private Cloud (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift
* Cluster Subnet Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @return A Java Future containing the result of the CreateClusterSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterSubnetGroupAlreadyExistsException A ClusterSubnetGroupName is already used by an
* existing cluster subnet group.
* - ClusterSubnetGroupQuotaExceededException The request would result in user exceeding the allowed
* number of cluster subnet groups. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - ClusterSubnetQuotaExceededException The request would result in user exceeding the allowed number of
* subnets in a cluster subnet groups. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - InvalidSubnetException The requested subnet is not valid, or not all of the subnets are in the same
* VPC.
* - UnauthorizedOperationException Your account is not authorized to perform the requested operation.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - DependentServiceRequestThrottlingException The request cannot be completed because a dependent
* service is throttling requests made by Amazon Redshift on your behalf. Wait and retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateClusterSubnetGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture createClusterSubnetGroup(
CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterSubnetGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateClusterSubnetGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateClusterSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateClusterSubnetGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterSubnetGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterSubnetGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Used to create a custom domain name for a cluster. Properties include the custom domain name, the cluster the
* custom domain is associated with, and the certificate Amazon Resource Name (ARN).
*
*
* @param createCustomDomainAssociationRequest
* @return A Java Future containing the result of the CreateCustomDomainAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnsupportedOperationException The requested operation isn't supported.
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - CustomCnameAssociationException An error occurred when an attempt was made to change the custom
* domain association.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture createCustomDomainAssociation(
CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCustomDomainAssociationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createCustomDomainAssociationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCustomDomainAssociation");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCustomDomainAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCustomDomainAssociation").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCustomDomainAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCustomDomainAssociationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a Redshift-managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @return A Java Future containing the result of the CreateEndpointAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - AccessToClusterDeniedException You are not authorized to access the cluster.
* - EndpointsPerClusterLimitExceededException The number of Redshift-managed VPC endpoints per cluster
* has exceeded its limit.
* - EndpointsPerAuthorizationLimitExceededException The number of Redshift-managed VPC endpoints per
* authorization has exceeded its limit.
* - InvalidClusterSecurityGroupStateException The state of the cluster security group is not
*
available
.
* - ClusterSubnetGroupNotFoundException The cluster subnet group name does not refer to an existing
* cluster subnet group.
* - EndpointAlreadyExistsException The account already has a Redshift-managed VPC endpoint with the given
* identifier.
* - UnsupportedOperationException The requested operation isn't supported.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - UnauthorizedOperationException Your account is not authorized to perform the requested operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateEndpointAccess
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createEndpointAccess(
CreateEndpointAccessRequest createEndpointAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEndpointAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEndpointAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEndpointAccess");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateEndpointAccessResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEndpointAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEndpointAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEndpointAccessRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon Redshift event notification subscription. This action requires an ARN (Amazon Resource Name) of
* an Amazon SNS topic created by either the Amazon Redshift console, the Amazon SNS console, or the Amazon SNS API.
* To obtain an ARN with Amazon SNS, you must create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs, event categories, and event severities.
* Notifications will be sent for all events you want that match those criteria. For example, you can specify source
* type = cluster, source ID = my-cluster-1 and mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR events in the Availability and Backup
* categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type = cluster and source identifier =
* my-cluster-1, notifications will be sent for all the cluster events for my-cluster-1. If you specify a source
* type but do not specify a source identifier, you will receive notice of the events for the objects of that type
* in your Amazon Web Services account. If you do not specify either the SourceType nor the SourceIdentifier, you
* will be notified of events generated from all Amazon Redshift sources belonging to your Amazon Web Services
* account. You must specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - EventSubscriptionQuotaExceededException The request would exceed the allowed number of event
* subscriptions for this account. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - SubscriptionAlreadyExistException There is already an existing event notification subscription with
* the specified name.
* - SnsInvalidTopicException Amazon SNS has responded that there is a problem with the specified Amazon
* SNS topic.
* - SnsNoAuthorizationException You do not have permission to publish to the specified Amazon SNS topic.
* - SnsTopicArnNotFoundException An Amazon SNS topic with the specified Amazon Resource Name (ARN) does
* not exist.
* - SubscriptionEventIdNotFoundException An Amazon Redshift event with the specified event ID does not
* exist.
* - SubscriptionCategoryNotFoundException The value specified for the event category was not one of the
* allowed values, or it specified a category that does not apply to the specified source type. The allowed
* values are Configuration, Management, Monitoring, and Security.
* - SubscriptionSeverityNotFoundException The value specified for the event severity was not one of the
* allowed values, or it specified a severity that does not apply to the specified source type. The allowed
* values are ERROR and INFO.
* - SourceNotFoundException The specified Amazon Redshift event source could not be found.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateEventSubscription
* @see AWS API Documentation
*/
@Override
public CompletableFuture createEventSubscription(
CreateEventSubscriptionRequest createEventSubscriptionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEventSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEventSubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEventSubscription");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateEventSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEventSubscription").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEventSubscriptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEventSubscriptionRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will use to connect to the client's HSM in
* order to store and retrieve the keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In addition to creating the HSM certificate,
* you must create an Amazon Redshift HSM configuration that provides a cluster the information needed to store and
* use encryption keys in the HSM. For more information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmClientCertificateRequest
* @return A Java Future containing the result of the CreateHsmClientCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - HsmClientCertificateAlreadyExistsException There is already an existing Amazon Redshift HSM client
* certificate with the specified identifier.
* - HsmClientCertificateQuotaExceededException The quota for HSM client certificates has been reached.
* For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateHsmClientCertificate
* @see AWS API Documentation
*/
@Override
public CompletableFuture createHsmClientCertificate(
CreateHsmClientCertificateRequest createHsmClientCertificateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHsmClientCertificateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHsmClientCertificateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHsmClientCertificate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateHsmClientCertificateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHsmClientCertificate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHsmClientCertificateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createHsmClientCertificateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an HSM configuration that contains the information required by an Amazon Redshift cluster to store and
* use database encryption keys in a Hardware Security Module (HSM). After creating the HSM configuration, you can
* specify it as a parameter when creating a cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM client certificate. For more
* information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmConfigurationRequest
* @return A Java Future containing the result of the CreateHsmConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - HsmConfigurationAlreadyExistsException There is already an existing Amazon Redshift HSM configuration
* with the specified identifier.
* - HsmConfigurationQuotaExceededException The quota for HSM configurations has been reached. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateHsmConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createHsmConfiguration(
CreateHsmConfigurationRequest createHsmConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHsmConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHsmConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHsmConfiguration");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateHsmConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHsmConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHsmConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createHsmConfigurationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon Redshift application for use with IAM Identity Center.
*
*
* @param createRedshiftIdcApplicationRequest
* @return A Java Future containing the result of the CreateRedshiftIdcApplication operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RedshiftIdcApplicationAlreadyExistsException The application you attempted to add already exists.
* - DependentServiceUnavailableException Your request cannot be completed because a dependent internal
* service is temporarily unavailable. Wait 30 to 60 seconds and try again.
* - UnsupportedOperationException The requested operation isn't supported.
* - DependentServiceAccessDeniedException A dependent service denied access for the integration.
* - RedshiftIdcApplicationQuotaExceededException The maximum number of Redshift IAM Identity Center
* applications was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateRedshiftIdcApplication
* @see AWS API Documentation
*/
@Override
public CompletableFuture createRedshiftIdcApplication(
CreateRedshiftIdcApplicationRequest createRedshiftIdcApplicationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createRedshiftIdcApplicationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRedshiftIdcApplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRedshiftIdcApplication");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateRedshiftIdcApplicationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateRedshiftIdcApplication").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateRedshiftIdcApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createRedshiftIdcApplicationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the ResizeCluster
API operation.
*
*
* @param createScheduledActionRequest
* @return A Java Future containing the result of the CreateScheduledAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - ScheduledActionAlreadyExistsException The scheduled action already exists.
* - ScheduledActionQuotaExceededException The quota for scheduled actions exceeded.
* - ScheduledActionTypeUnsupportedException The action type specified for a scheduled action is not
* supported.
* - InvalidScheduleException The schedule you submitted isn't valid.
* - InvalidScheduledActionException The scheduled action is not valid.
* - UnauthorizedOperationException Your account is not authorized to perform the requested operation.
* - UnsupportedOperationException The requested operation isn't supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateScheduledAction
* @see AWS API Documentation
*/
@Override
public CompletableFuture createScheduledAction(
CreateScheduledActionRequest createScheduledActionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createScheduledActionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createScheduledActionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateScheduledAction");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateScheduledActionResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateScheduledAction").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateScheduledActionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createScheduledActionRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use an encrypted symmetric key from Key Management
* Service (KMS) to encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @return A Java Future containing the result of the CreateSnapshotCopyGrant operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotCopyGrantAlreadyExistsException The snapshot copy grant can't be created because a grant with
* the same name already exists.
* - SnapshotCopyGrantQuotaExceededException The Amazon Web Services account has exceeded the maximum
* number of snapshot copy grants in this region.
* - LimitExceededException The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - InvalidTagException The tag is invalid.
* - DependentServiceRequestThrottlingException The request cannot be completed because a dependent
* service is throttling requests made by Amazon Redshift on your behalf. Wait and retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateSnapshotCopyGrant
* @see AWS API Documentation
*/
@Override
public CompletableFuture createSnapshotCopyGrant(
CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSnapshotCopyGrantRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSnapshotCopyGrantRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSnapshotCopyGrant");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateSnapshotCopyGrantResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSnapshotCopyGrant").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSnapshotCopyGrantRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSnapshotCopyGrantRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create a snapshot schedule that can be associated to a cluster and which overrides the default system backup
* schedule.
*
*
* @param createSnapshotScheduleRequest
* @return A Java Future containing the result of the CreateSnapshotSchedule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SnapshotScheduleAlreadyExistsException The specified snapshot schedule already exists.
* - InvalidScheduleException The schedule you submitted isn't valid.
* - SnapshotScheduleQuotaExceededException You have exceeded the quota of snapshot schedules.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - ScheduleDefinitionTypeUnsupportedException The definition you submitted is not supported.
* - InvalidTagException The tag is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateSnapshotSchedule
* @see AWS API Documentation
*/
@Override
public CompletableFuture createSnapshotSchedule(
CreateSnapshotScheduleRequest createSnapshotScheduleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSnapshotScheduleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSnapshotScheduleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSnapshotSchedule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateSnapshotScheduleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSnapshotSchedule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSnapshotScheduleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSnapshotScheduleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds tags to a cluster.
*
*
* A resource can have up to 50 tags. If you try to create more than 50 tags for a resource, you will receive an
* error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for that key will be updated with the new
* value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - ResourceNotFoundException The resource could not be found.
* - InvalidTagException The tag is invalid.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateTags
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createTags(CreateTagsRequest createTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTags");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateTagsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateTags")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createTagsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a usage limit for a specified Amazon Redshift feature on a cluster. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @return A Java Future containing the result of the CreateUsageLimit operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - LimitExceededException The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* - UsageLimitAlreadyExistsException The usage limit already exists.
* - InvalidUsageLimitException The usage limit is not valid.
* - TagLimitExceededException You have exceeded the number of tags allowed.
* - UnsupportedOperationException The requested operation isn't supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.CreateUsageLimit
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUsageLimit(CreateUsageLimitRequest createUsageLimitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUsageLimitRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUsageLimitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUsageLimit");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateUsageLimitResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUsageLimit").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateUsageLimitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createUsageLimitRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* From a datashare producer account, removes authorization from the specified datashare.
*
*
* @param deauthorizeDataShareRequest
* @return A Java Future containing the result of the DeauthorizeDataShare operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidDataShareException There is an error with the datashare.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeauthorizeDataShare
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deauthorizeDataShare(
DeauthorizeDataShareRequest deauthorizeDataShareRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deauthorizeDataShareRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deauthorizeDataShareRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeauthorizeDataShare");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeauthorizeDataShareResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeauthorizeDataShare").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeauthorizeDataShareRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deauthorizeDataShareRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an authentication profile.
*
*
* @param deleteAuthenticationProfileRequest
* @return A Java Future containing the result of the DeleteAuthenticationProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AuthenticationProfileNotFoundException The authentication profile can't be found.
* - InvalidAuthenticationProfileRequestException The authentication profile request is not valid. The
* profile name can't be null or empty. The authentication profile API operation must be available in the
* Amazon Web Services Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteAuthenticationProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteAuthenticationProfile(
DeleteAuthenticationProfileRequest deleteAuthenticationProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAuthenticationProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAuthenticationProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAuthenticationProfile");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteAuthenticationProfileResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAuthenticationProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAuthenticationProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAuthenticationProfileRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a previously provisioned cluster without its final snapshot being created. A successful response from the
* web service indicates that the request was received correctly. Use DescribeClusters to monitor the status
* of the deletion. The delete operation cannot be canceled or reverted once submitted. For more information about
* managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you want to shut down the cluster and retain it for future use, set SkipFinalClusterSnapshot to
* false
and specify a name for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is requested, the status of the cluster will be
* "final-snapshot" while the snapshot is being taken, then it's "deleting" once Amazon Redshift begins deleting the
* cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - InvalidClusterStateException The specified cluster is not in the
available
state.
* - ClusterSnapshotAlreadyExistsException The value specified as a snapshot identifier is already used by
* an existing snapshot.
* - ClusterSnapshotQuotaExceededException The request would result in the user exceeding the allowed
* number of cluster snapshots.
* - InvalidRetentionPeriodException The retention period specified is either in the past or is not a
* valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteCluster(DeleteClusterRequest deleteClusterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCluster");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteClusterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCluster").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified Amazon Redshift parameter group.
*
*
*
* You cannot delete a parameter group if it is associated with a cluster.
*
*
*
* @param deleteClusterParameterGroupRequest
* @return A Java Future containing the result of the DeleteClusterParameterGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidClusterParameterGroupStateException The cluster parameter group action can not be completed
* because another task is in progress that involves the parameter group. Wait a few moments and try the
* operation again.
* - ClusterParameterGroupNotFoundException The parameter group name does not refer to an existing
* parameter group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteClusterParameterGroup(
DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterParameterGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteClusterParameterGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteClusterParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteClusterParameterGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterParameterGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterParameterGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon Redshift security group.
*
*
*
* You cannot delete a security group that is associated with any clusters. You cannot delete the default security
* group.
*
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @return A Java Future containing the result of the DeleteClusterSecurityGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidClusterSecurityGroupStateException The state of the cluster security group is not
*
available
.
* - ClusterSecurityGroupNotFoundException The cluster security group name does not refer to an existing
* cluster security group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteClusterSecurityGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteClusterSecurityGroup(
DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterSecurityGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterSecurityGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteClusterSecurityGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteClusterSecurityGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteClusterSecurityGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterSecurityGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterSecurityGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the available
state, with no other
* users authorized to access the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you delete your cluster. Amazon Redshift
* does not delete your manual snapshots. You must delete manual snapshot explicitly to avoid getting charged. If
* other accounts are authorized to access the snapshot, you must revoke all of the authorizations before you can
* delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteClusterSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidClusterSnapshotStateException The specified cluster snapshot is not in the
*
available
state, or other accounts are authorized to access the snapshot.
* - ClusterSnapshotNotFoundException The snapshot identifier does not refer to an existing cluster
* snapshot.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteClusterSnapshot
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteClusterSnapshot(
DeleteClusterSnapshotRequest deleteClusterSnapshotRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterSnapshotRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteClusterSnapshot");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteClusterSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteClusterSnapshot").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterSnapshotRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterSnapshotRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @return A Java Future containing the result of the DeleteClusterSubnetGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidClusterSubnetGroupStateException The cluster subnet group cannot be deleted because it is in
* use.
* - InvalidClusterSubnetStateException The state of the subnet is invalid.
* - ClusterSubnetGroupNotFoundException The cluster subnet group name does not refer to an existing
* cluster subnet group.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteClusterSubnetGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteClusterSubnetGroup(
DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterSubnetGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteClusterSubnetGroup");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteClusterSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteClusterSubnetGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterSubnetGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterSubnetGroupRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Contains information about deleting a custom domain association for a cluster.
*
*
* @param deleteCustomDomainAssociationRequest
* @return A Java Future containing the result of the DeleteCustomDomainAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnsupportedOperationException The requested operation isn't supported.
* - ClusterNotFoundException The
ClusterIdentifier
parameter does not refer to an existing
* cluster.
* - CustomCnameAssociationException An error occurred when an attempt was made to change the custom
* domain association.
* - CustomDomainAssociationNotFoundException An error occurred. The custom domain name couldn't be found.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - RedshiftException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample RedshiftAsyncClient.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteCustomDomainAssociation(
DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCustomDomainAssociationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteCustomDomainAssociationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Redshift");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCustomDomainAssociation");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCustomDomainAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams