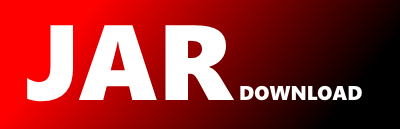
software.amazon.awssdk.services.redshift.model.Snapshot Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a snapshot.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Snapshot implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SNAPSHOT_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotIdentifier").getter(getter(Snapshot::snapshotIdentifier))
.setter(setter(Builder::snapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotIdentifier").build())
.build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(Snapshot::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField SNAPSHOT_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("SnapshotCreateTime").getter(getter(Snapshot::snapshotCreateTime))
.setter(setter(Builder::snapshotCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotCreateTime").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(Snapshot::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(Snapshot::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(Snapshot::availabilityZone)).setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ClusterCreateTime").getter(getter(Snapshot::clusterCreateTime))
.setter(setter(Builder::clusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterCreateTime").build()).build();
private static final SdkField MASTER_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUsername").getter(getter(Snapshot::masterUsername)).setter(setter(Builder::masterUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUsername").build()).build();
private static final SdkField CLUSTER_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterVersion").getter(getter(Snapshot::clusterVersion)).setter(setter(Builder::clusterVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterVersion").build()).build();
private static final SdkField ENGINE_FULL_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineFullVersion").getter(getter(Snapshot::engineFullVersion))
.setter(setter(Builder::engineFullVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineFullVersion").build()).build();
private static final SdkField SNAPSHOT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotType").getter(getter(Snapshot::snapshotType)).setter(setter(Builder::snapshotType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotType").build()).build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(Snapshot::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfNodes").getter(getter(Snapshot::numberOfNodes)).setter(setter(Builder::numberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNodes").build()).build();
private static final SdkField DB_NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DBName")
.getter(getter(Snapshot::dbName)).setter(setter(Builder::dbName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBName").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(Snapshot::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted").getter(getter(Snapshot::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(Snapshot::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField ENCRYPTED_WITH_HSM_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EncryptedWithHSM").getter(getter(Snapshot::encryptedWithHSM)).setter(setter(Builder::encryptedWithHSM))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncryptedWithHSM").build()).build();
private static final SdkField> ACCOUNTS_WITH_RESTORE_ACCESS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AccountsWithRestoreAccess")
.getter(getter(Snapshot::accountsWithRestoreAccess))
.setter(setter(Builder::accountsWithRestoreAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountsWithRestoreAccess").build(),
ListTrait
.builder()
.memberLocationName("AccountWithRestoreAccess")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AccountWithRestoreAccess::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AccountWithRestoreAccess").build()).build()).build()).build();
private static final SdkField OWNER_ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OwnerAccount").getter(getter(Snapshot::ownerAccount)).setter(setter(Builder::ownerAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerAccount").build()).build();
private static final SdkField TOTAL_BACKUP_SIZE_IN_MEGA_BYTES_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("TotalBackupSizeInMegaBytes")
.getter(getter(Snapshot::totalBackupSizeInMegaBytes))
.setter(setter(Builder::totalBackupSizeInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalBackupSizeInMegaBytes").build())
.build();
private static final SdkField ACTUAL_INCREMENTAL_BACKUP_SIZE_IN_MEGA_BYTES_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("ActualIncrementalBackupSizeInMegaBytes")
.getter(getter(Snapshot::actualIncrementalBackupSizeInMegaBytes))
.setter(setter(Builder::actualIncrementalBackupSizeInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ActualIncrementalBackupSizeInMegaBytes").build()).build();
private static final SdkField BACKUP_PROGRESS_IN_MEGA_BYTES_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("BackupProgressInMegaBytes").getter(getter(Snapshot::backupProgressInMegaBytes))
.setter(setter(Builder::backupProgressInMegaBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupProgressInMegaBytes").build())
.build();
private static final SdkField CURRENT_BACKUP_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("CurrentBackupRateInMegaBytesPerSecond")
.getter(getter(Snapshot::currentBackupRateInMegaBytesPerSecond))
.setter(setter(Builder::currentBackupRateInMegaBytesPerSecond))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CurrentBackupRateInMegaBytesPerSecond").build()).build();
private static final SdkField ESTIMATED_SECONDS_TO_COMPLETION_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("EstimatedSecondsToCompletion")
.getter(getter(Snapshot::estimatedSecondsToCompletion))
.setter(setter(Builder::estimatedSecondsToCompletion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedSecondsToCompletion")
.build()).build();
private static final SdkField ELAPSED_TIME_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ElapsedTimeInSeconds").getter(getter(Snapshot::elapsedTimeInSeconds))
.setter(setter(Builder::elapsedTimeInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElapsedTimeInSeconds").build())
.build();
private static final SdkField SOURCE_REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceRegion").getter(getter(Snapshot::sourceRegion)).setter(setter(Builder::sourceRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceRegion").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Snapshot::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final SdkField> RESTORABLE_NODE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RestorableNodeTypes")
.getter(getter(Snapshot::restorableNodeTypes))
.setter(setter(Builder::restorableNodeTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RestorableNodeTypes").build(),
ListTrait
.builder()
.memberLocationName("NodeType")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("NodeType").build()).build()).build()).build();
private static final SdkField ENHANCED_VPC_ROUTING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnhancedVpcRouting").getter(getter(Snapshot::enhancedVpcRouting))
.setter(setter(Builder::enhancedVpcRouting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnhancedVpcRouting").build())
.build();
private static final SdkField MAINTENANCE_TRACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaintenanceTrackName").getter(getter(Snapshot::maintenanceTrackName))
.setter(setter(Builder::maintenanceTrackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaintenanceTrackName").build())
.build();
private static final SdkField MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ManualSnapshotRetentionPeriod")
.getter(getter(Snapshot::manualSnapshotRetentionPeriod))
.setter(setter(Builder::manualSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManualSnapshotRetentionPeriod")
.build()).build();
private static final SdkField MANUAL_SNAPSHOT_REMAINING_DAYS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ManualSnapshotRemainingDays")
.getter(getter(Snapshot::manualSnapshotRemainingDays))
.setter(setter(Builder::manualSnapshotRemainingDays))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManualSnapshotRemainingDays")
.build()).build();
private static final SdkField SNAPSHOT_RETENTION_START_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("SnapshotRetentionStartTime")
.getter(getter(Snapshot::snapshotRetentionStartTime))
.setter(setter(Builder::snapshotRetentionStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotRetentionStartTime").build())
.build();
private static final SdkField MASTER_PASSWORD_SECRET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterPasswordSecretArn").getter(getter(Snapshot::masterPasswordSecretArn))
.setter(setter(Builder::masterPasswordSecretArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterPasswordSecretArn").build())
.build();
private static final SdkField MASTER_PASSWORD_SECRET_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MasterPasswordSecretKmsKeyId")
.getter(getter(Snapshot::masterPasswordSecretKmsKeyId))
.setter(setter(Builder::masterPasswordSecretKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterPasswordSecretKmsKeyId")
.build()).build();
private static final SdkField SNAPSHOT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotArn").getter(getter(Snapshot::snapshotArn)).setter(setter(Builder::snapshotArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SNAPSHOT_IDENTIFIER_FIELD,
CLUSTER_IDENTIFIER_FIELD, SNAPSHOT_CREATE_TIME_FIELD, STATUS_FIELD, PORT_FIELD, AVAILABILITY_ZONE_FIELD,
CLUSTER_CREATE_TIME_FIELD, MASTER_USERNAME_FIELD, CLUSTER_VERSION_FIELD, ENGINE_FULL_VERSION_FIELD,
SNAPSHOT_TYPE_FIELD, NODE_TYPE_FIELD, NUMBER_OF_NODES_FIELD, DB_NAME_FIELD, VPC_ID_FIELD, ENCRYPTED_FIELD,
KMS_KEY_ID_FIELD, ENCRYPTED_WITH_HSM_FIELD, ACCOUNTS_WITH_RESTORE_ACCESS_FIELD, OWNER_ACCOUNT_FIELD,
TOTAL_BACKUP_SIZE_IN_MEGA_BYTES_FIELD, ACTUAL_INCREMENTAL_BACKUP_SIZE_IN_MEGA_BYTES_FIELD,
BACKUP_PROGRESS_IN_MEGA_BYTES_FIELD, CURRENT_BACKUP_RATE_IN_MEGA_BYTES_PER_SECOND_FIELD,
ESTIMATED_SECONDS_TO_COMPLETION_FIELD, ELAPSED_TIME_IN_SECONDS_FIELD, SOURCE_REGION_FIELD, TAGS_FIELD,
RESTORABLE_NODE_TYPES_FIELD, ENHANCED_VPC_ROUTING_FIELD, MAINTENANCE_TRACK_NAME_FIELD,
MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD, MANUAL_SNAPSHOT_REMAINING_DAYS_FIELD, SNAPSHOT_RETENTION_START_TIME_FIELD,
MASTER_PASSWORD_SECRET_ARN_FIELD, MASTER_PASSWORD_SECRET_KMS_KEY_ID_FIELD, SNAPSHOT_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String snapshotIdentifier;
private final String clusterIdentifier;
private final Instant snapshotCreateTime;
private final String status;
private final Integer port;
private final String availabilityZone;
private final Instant clusterCreateTime;
private final String masterUsername;
private final String clusterVersion;
private final String engineFullVersion;
private final String snapshotType;
private final String nodeType;
private final Integer numberOfNodes;
private final String dbName;
private final String vpcId;
private final Boolean encrypted;
private final String kmsKeyId;
private final Boolean encryptedWithHSM;
private final List accountsWithRestoreAccess;
private final String ownerAccount;
private final Double totalBackupSizeInMegaBytes;
private final Double actualIncrementalBackupSizeInMegaBytes;
private final Double backupProgressInMegaBytes;
private final Double currentBackupRateInMegaBytesPerSecond;
private final Long estimatedSecondsToCompletion;
private final Long elapsedTimeInSeconds;
private final String sourceRegion;
private final List tags;
private final List restorableNodeTypes;
private final Boolean enhancedVpcRouting;
private final String maintenanceTrackName;
private final Integer manualSnapshotRetentionPeriod;
private final Integer manualSnapshotRemainingDays;
private final Instant snapshotRetentionStartTime;
private final String masterPasswordSecretArn;
private final String masterPasswordSecretKmsKeyId;
private final String snapshotArn;
private Snapshot(BuilderImpl builder) {
this.snapshotIdentifier = builder.snapshotIdentifier;
this.clusterIdentifier = builder.clusterIdentifier;
this.snapshotCreateTime = builder.snapshotCreateTime;
this.status = builder.status;
this.port = builder.port;
this.availabilityZone = builder.availabilityZone;
this.clusterCreateTime = builder.clusterCreateTime;
this.masterUsername = builder.masterUsername;
this.clusterVersion = builder.clusterVersion;
this.engineFullVersion = builder.engineFullVersion;
this.snapshotType = builder.snapshotType;
this.nodeType = builder.nodeType;
this.numberOfNodes = builder.numberOfNodes;
this.dbName = builder.dbName;
this.vpcId = builder.vpcId;
this.encrypted = builder.encrypted;
this.kmsKeyId = builder.kmsKeyId;
this.encryptedWithHSM = builder.encryptedWithHSM;
this.accountsWithRestoreAccess = builder.accountsWithRestoreAccess;
this.ownerAccount = builder.ownerAccount;
this.totalBackupSizeInMegaBytes = builder.totalBackupSizeInMegaBytes;
this.actualIncrementalBackupSizeInMegaBytes = builder.actualIncrementalBackupSizeInMegaBytes;
this.backupProgressInMegaBytes = builder.backupProgressInMegaBytes;
this.currentBackupRateInMegaBytesPerSecond = builder.currentBackupRateInMegaBytesPerSecond;
this.estimatedSecondsToCompletion = builder.estimatedSecondsToCompletion;
this.elapsedTimeInSeconds = builder.elapsedTimeInSeconds;
this.sourceRegion = builder.sourceRegion;
this.tags = builder.tags;
this.restorableNodeTypes = builder.restorableNodeTypes;
this.enhancedVpcRouting = builder.enhancedVpcRouting;
this.maintenanceTrackName = builder.maintenanceTrackName;
this.manualSnapshotRetentionPeriod = builder.manualSnapshotRetentionPeriod;
this.manualSnapshotRemainingDays = builder.manualSnapshotRemainingDays;
this.snapshotRetentionStartTime = builder.snapshotRetentionStartTime;
this.masterPasswordSecretArn = builder.masterPasswordSecretArn;
this.masterPasswordSecretKmsKeyId = builder.masterPasswordSecretKmsKeyId;
this.snapshotArn = builder.snapshotArn;
}
/**
*
* The snapshot identifier that is provided in the request.
*
*
* @return The snapshot identifier that is provided in the request.
*/
public final String snapshotIdentifier() {
return snapshotIdentifier;
}
/**
*
* The identifier of the cluster for which the snapshot was taken.
*
*
* @return The identifier of the cluster for which the snapshot was taken.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The time (in UTC format) when Amazon Redshift began the snapshot. A snapshot contains a copy of the cluster data
* as of this exact time.
*
*
* @return The time (in UTC format) when Amazon Redshift began the snapshot. A snapshot contains a copy of the
* cluster data as of this exact time.
*/
public final Instant snapshotCreateTime() {
return snapshotCreateTime;
}
/**
*
* The snapshot status. The value of the status depends on the API operation used:
*
*
* -
*
* CreateClusterSnapshot and CopyClusterSnapshot returns status as "creating".
*
*
* -
*
* DescribeClusterSnapshots returns status as "creating", "available", "final snapshot", or "failed".
*
*
* -
*
* DeleteClusterSnapshot returns status as "deleted".
*
*
*
*
* @return The snapshot status. The value of the status depends on the API operation used:
*
* -
*
* CreateClusterSnapshot and CopyClusterSnapshot returns status as "creating".
*
*
* -
*
* DescribeClusterSnapshots returns status as "creating", "available", "final snapshot", or "failed".
*
*
* -
*
* DeleteClusterSnapshot returns status as "deleted".
*
*
*/
public final String status() {
return status;
}
/**
*
* The port that the cluster is listening on.
*
*
* @return The port that the cluster is listening on.
*/
public final Integer port() {
return port;
}
/**
*
* The Availability Zone in which the cluster was created.
*
*
* @return The Availability Zone in which the cluster was created.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The time (UTC) when the cluster was originally created.
*
*
* @return The time (UTC) when the cluster was originally created.
*/
public final Instant clusterCreateTime() {
return clusterCreateTime;
}
/**
*
* The admin user name for the cluster.
*
*
* @return The admin user name for the cluster.
*/
public final String masterUsername() {
return masterUsername;
}
/**
*
* The version ID of the Amazon Redshift engine that is running on the cluster.
*
*
* @return The version ID of the Amazon Redshift engine that is running on the cluster.
*/
public final String clusterVersion() {
return clusterVersion;
}
/**
*
* The cluster version of the cluster used to create the snapshot. For example, 1.0.15503.
*
*
* @return The cluster version of the cluster used to create the snapshot. For example, 1.0.15503.
*/
public final String engineFullVersion() {
return engineFullVersion;
}
/**
*
* The snapshot type. Snapshots created using CreateClusterSnapshot and CopyClusterSnapshot are of
* type "manual".
*
*
* @return The snapshot type. Snapshots created using CreateClusterSnapshot and CopyClusterSnapshot
* are of type "manual".
*/
public final String snapshotType() {
return snapshotType;
}
/**
*
* The node type of the nodes in the cluster.
*
*
* @return The node type of the nodes in the cluster.
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The number of nodes in the cluster.
*
*
* @return The number of nodes in the cluster.
*/
public final Integer numberOfNodes() {
return numberOfNodes;
}
/**
*
* The name of the database that was created when the cluster was created.
*
*
* @return The name of the database that was created when the cluster was created.
*/
public final String dbName() {
return dbName;
}
/**
*
* The VPC identifier of the cluster if the snapshot is from a cluster in a VPC. Otherwise, this field is not in the
* output.
*
*
* @return The VPC identifier of the cluster if the snapshot is from a cluster in a VPC. Otherwise, this field is
* not in the output.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* If true
, the data in the snapshot is encrypted at rest.
*
*
* @return If true
, the data in the snapshot is encrypted at rest.
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* The Key Management Service (KMS) key ID of the encryption key that was used to encrypt data in the cluster from
* which the snapshot was taken.
*
*
* @return The Key Management Service (KMS) key ID of the encryption key that was used to encrypt data in the
* cluster from which the snapshot was taken.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* A boolean that indicates whether the snapshot data is encrypted using the HSM keys of the source cluster.
* true
indicates that the data is encrypted using HSM keys.
*
*
* @return A boolean that indicates whether the snapshot data is encrypted using the HSM keys of the source cluster.
* true
indicates that the data is encrypted using HSM keys.
*/
public final Boolean encryptedWithHSM() {
return encryptedWithHSM;
}
/**
* For responses, this returns true if the service returned a value for the AccountsWithRestoreAccess property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAccountsWithRestoreAccess() {
return accountsWithRestoreAccess != null && !(accountsWithRestoreAccess instanceof SdkAutoConstructList);
}
/**
*
* A list of the Amazon Web Services accounts authorized to restore the snapshot. Returns null
if no
* accounts are authorized. Visible only to the snapshot owner.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAccountsWithRestoreAccess} method.
*
*
* @return A list of the Amazon Web Services accounts authorized to restore the snapshot. Returns null
* if no accounts are authorized. Visible only to the snapshot owner.
*/
public final List accountsWithRestoreAccess() {
return accountsWithRestoreAccess;
}
/**
*
* For manual snapshots, the Amazon Web Services account used to create or copy the snapshot. For automatic
* snapshots, the owner of the cluster. The owner can perform all snapshot actions, such as sharing a manual
* snapshot.
*
*
* @return For manual snapshots, the Amazon Web Services account used to create or copy the snapshot. For automatic
* snapshots, the owner of the cluster. The owner can perform all snapshot actions, such as sharing a manual
* snapshot.
*/
public final String ownerAccount() {
return ownerAccount;
}
/**
*
* The size of the complete set of backup data that would be used to restore the cluster.
*
*
* @return The size of the complete set of backup data that would be used to restore the cluster.
*/
public final Double totalBackupSizeInMegaBytes() {
return totalBackupSizeInMegaBytes;
}
/**
*
* The size of the incremental backup.
*
*
* @return The size of the incremental backup.
*/
public final Double actualIncrementalBackupSizeInMegaBytes() {
return actualIncrementalBackupSizeInMegaBytes;
}
/**
*
* The number of megabytes that have been transferred to the snapshot backup.
*
*
* @return The number of megabytes that have been transferred to the snapshot backup.
*/
public final Double backupProgressInMegaBytes() {
return backupProgressInMegaBytes;
}
/**
*
* The number of megabytes per second being transferred to the snapshot backup. Returns 0
for a
* completed backup.
*
*
* @return The number of megabytes per second being transferred to the snapshot backup. Returns 0
for a
* completed backup.
*/
public final Double currentBackupRateInMegaBytesPerSecond() {
return currentBackupRateInMegaBytesPerSecond;
}
/**
*
* The estimate of the time remaining before the snapshot backup will complete. Returns 0
for a
* completed backup.
*
*
* @return The estimate of the time remaining before the snapshot backup will complete. Returns 0
for a
* completed backup.
*/
public final Long estimatedSecondsToCompletion() {
return estimatedSecondsToCompletion;
}
/**
*
* The amount of time an in-progress snapshot backup has been running, or the amount of time it took a completed
* backup to finish.
*
*
* @return The amount of time an in-progress snapshot backup has been running, or the amount of time it took a
* completed backup to finish.
*/
public final Long elapsedTimeInSeconds() {
return elapsedTimeInSeconds;
}
/**
*
* The source region from which the snapshot was copied.
*
*
* @return The source region from which the snapshot was copied.
*/
public final String sourceRegion() {
return sourceRegion;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The list of tags for the cluster snapshot.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The list of tags for the cluster snapshot.
*/
public final List tags() {
return tags;
}
/**
* For responses, this returns true if the service returned a value for the RestorableNodeTypes property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRestorableNodeTypes() {
return restorableNodeTypes != null && !(restorableNodeTypes instanceof SdkAutoConstructList);
}
/**
*
* The list of node types that this cluster snapshot is able to restore into.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRestorableNodeTypes} method.
*
*
* @return The list of node types that this cluster snapshot is able to restore into.
*/
public final List restorableNodeTypes() {
return restorableNodeTypes;
}
/**
*
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster
* that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the
* Amazon Redshift Cluster Management Guide.
*
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*
*
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a
* cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC
* Routing in the Amazon Redshift Cluster Management Guide.
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*/
public final Boolean enhancedVpcRouting() {
return enhancedVpcRouting;
}
/**
*
* The name of the maintenance track for the snapshot.
*
*
* @return The name of the maintenance track for the snapshot.
*/
public final String maintenanceTrackName() {
return maintenanceTrackName;
}
/**
*
* The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is retained
* indefinitely.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* @return The number of days that a manual snapshot is retained. If the value is -1, the manual snapshot is
* retained indefinitely.
*
* The value must be either -1 or an integer between 1 and 3,653.
*/
public final Integer manualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
/**
*
* The number of days until a manual snapshot will pass its retention period.
*
*
* @return The number of days until a manual snapshot will pass its retention period.
*/
public final Integer manualSnapshotRemainingDays() {
return manualSnapshotRemainingDays;
}
/**
*
* A timestamp representing the start of the retention period for the snapshot.
*
*
* @return A timestamp representing the start of the retention period for the snapshot.
*/
public final Instant snapshotRetentionStartTime() {
return snapshotRetentionStartTime;
}
/**
*
* The Amazon Resource Name (ARN) for the cluster's admin user credentials secret.
*
*
* @return The Amazon Resource Name (ARN) for the cluster's admin user credentials secret.
*/
public final String masterPasswordSecretArn() {
return masterPasswordSecretArn;
}
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin credentials secret.
*
*
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin credentials
* secret.
*/
public final String masterPasswordSecretKmsKeyId() {
return masterPasswordSecretKmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot.
*
*
* @return The Amazon Resource Name (ARN) of the snapshot.
*/
public final String snapshotArn() {
return snapshotArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(snapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(snapshotCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(clusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(masterUsername());
hashCode = 31 * hashCode + Objects.hashCode(clusterVersion());
hashCode = 31 * hashCode + Objects.hashCode(engineFullVersion());
hashCode = 31 * hashCode + Objects.hashCode(snapshotType());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(dbName());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(encryptedWithHSM());
hashCode = 31 * hashCode + Objects.hashCode(hasAccountsWithRestoreAccess() ? accountsWithRestoreAccess() : null);
hashCode = 31 * hashCode + Objects.hashCode(ownerAccount());
hashCode = 31 * hashCode + Objects.hashCode(totalBackupSizeInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(actualIncrementalBackupSizeInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(backupProgressInMegaBytes());
hashCode = 31 * hashCode + Objects.hashCode(currentBackupRateInMegaBytesPerSecond());
hashCode = 31 * hashCode + Objects.hashCode(estimatedSecondsToCompletion());
hashCode = 31 * hashCode + Objects.hashCode(elapsedTimeInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(sourceRegion());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasRestorableNodeTypes() ? restorableNodeTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(enhancedVpcRouting());
hashCode = 31 * hashCode + Objects.hashCode(maintenanceTrackName());
hashCode = 31 * hashCode + Objects.hashCode(manualSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(manualSnapshotRemainingDays());
hashCode = 31 * hashCode + Objects.hashCode(snapshotRetentionStartTime());
hashCode = 31 * hashCode + Objects.hashCode(masterPasswordSecretArn());
hashCode = 31 * hashCode + Objects.hashCode(masterPasswordSecretKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(snapshotArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Snapshot)) {
return false;
}
Snapshot other = (Snapshot) obj;
return Objects.equals(snapshotIdentifier(), other.snapshotIdentifier())
&& Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(snapshotCreateTime(), other.snapshotCreateTime()) && Objects.equals(status(), other.status())
&& Objects.equals(port(), other.port()) && Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(clusterCreateTime(), other.clusterCreateTime())
&& Objects.equals(masterUsername(), other.masterUsername())
&& Objects.equals(clusterVersion(), other.clusterVersion())
&& Objects.equals(engineFullVersion(), other.engineFullVersion())
&& Objects.equals(snapshotType(), other.snapshotType()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(numberOfNodes(), other.numberOfNodes()) && Objects.equals(dbName(), other.dbName())
&& Objects.equals(vpcId(), other.vpcId()) && Objects.equals(encrypted(), other.encrypted())
&& Objects.equals(kmsKeyId(), other.kmsKeyId()) && Objects.equals(encryptedWithHSM(), other.encryptedWithHSM())
&& hasAccountsWithRestoreAccess() == other.hasAccountsWithRestoreAccess()
&& Objects.equals(accountsWithRestoreAccess(), other.accountsWithRestoreAccess())
&& Objects.equals(ownerAccount(), other.ownerAccount())
&& Objects.equals(totalBackupSizeInMegaBytes(), other.totalBackupSizeInMegaBytes())
&& Objects.equals(actualIncrementalBackupSizeInMegaBytes(), other.actualIncrementalBackupSizeInMegaBytes())
&& Objects.equals(backupProgressInMegaBytes(), other.backupProgressInMegaBytes())
&& Objects.equals(currentBackupRateInMegaBytesPerSecond(), other.currentBackupRateInMegaBytesPerSecond())
&& Objects.equals(estimatedSecondsToCompletion(), other.estimatedSecondsToCompletion())
&& Objects.equals(elapsedTimeInSeconds(), other.elapsedTimeInSeconds())
&& Objects.equals(sourceRegion(), other.sourceRegion()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && hasRestorableNodeTypes() == other.hasRestorableNodeTypes()
&& Objects.equals(restorableNodeTypes(), other.restorableNodeTypes())
&& Objects.equals(enhancedVpcRouting(), other.enhancedVpcRouting())
&& Objects.equals(maintenanceTrackName(), other.maintenanceTrackName())
&& Objects.equals(manualSnapshotRetentionPeriod(), other.manualSnapshotRetentionPeriod())
&& Objects.equals(manualSnapshotRemainingDays(), other.manualSnapshotRemainingDays())
&& Objects.equals(snapshotRetentionStartTime(), other.snapshotRetentionStartTime())
&& Objects.equals(masterPasswordSecretArn(), other.masterPasswordSecretArn())
&& Objects.equals(masterPasswordSecretKmsKeyId(), other.masterPasswordSecretKmsKeyId())
&& Objects.equals(snapshotArn(), other.snapshotArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Snapshot").add("SnapshotIdentifier", snapshotIdentifier())
.add("ClusterIdentifier", clusterIdentifier()).add("SnapshotCreateTime", snapshotCreateTime())
.add("Status", status()).add("Port", port()).add("AvailabilityZone", availabilityZone())
.add("ClusterCreateTime", clusterCreateTime()).add("MasterUsername", masterUsername())
.add("ClusterVersion", clusterVersion()).add("EngineFullVersion", engineFullVersion())
.add("SnapshotType", snapshotType()).add("NodeType", nodeType()).add("NumberOfNodes", numberOfNodes())
.add("DBName", dbName()).add("VpcId", vpcId()).add("Encrypted", encrypted()).add("KmsKeyId", kmsKeyId())
.add("EncryptedWithHSM", encryptedWithHSM())
.add("AccountsWithRestoreAccess", hasAccountsWithRestoreAccess() ? accountsWithRestoreAccess() : null)
.add("OwnerAccount", ownerAccount()).add("TotalBackupSizeInMegaBytes", totalBackupSizeInMegaBytes())
.add("ActualIncrementalBackupSizeInMegaBytes", actualIncrementalBackupSizeInMegaBytes())
.add("BackupProgressInMegaBytes", backupProgressInMegaBytes())
.add("CurrentBackupRateInMegaBytesPerSecond", currentBackupRateInMegaBytesPerSecond())
.add("EstimatedSecondsToCompletion", estimatedSecondsToCompletion())
.add("ElapsedTimeInSeconds", elapsedTimeInSeconds()).add("SourceRegion", sourceRegion())
.add("Tags", hasTags() ? tags() : null)
.add("RestorableNodeTypes", hasRestorableNodeTypes() ? restorableNodeTypes() : null)
.add("EnhancedVpcRouting", enhancedVpcRouting()).add("MaintenanceTrackName", maintenanceTrackName())
.add("ManualSnapshotRetentionPeriod", manualSnapshotRetentionPeriod())
.add("ManualSnapshotRemainingDays", manualSnapshotRemainingDays())
.add("SnapshotRetentionStartTime", snapshotRetentionStartTime())
.add("MasterPasswordSecretArn", masterPasswordSecretArn())
.add("MasterPasswordSecretKmsKeyId", masterPasswordSecretKmsKeyId()).add("SnapshotArn", snapshotArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SnapshotIdentifier":
return Optional.ofNullable(clazz.cast(snapshotIdentifier()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "SnapshotCreateTime":
return Optional.ofNullable(clazz.cast(snapshotCreateTime()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "ClusterCreateTime":
return Optional.ofNullable(clazz.cast(clusterCreateTime()));
case "MasterUsername":
return Optional.ofNullable(clazz.cast(masterUsername()));
case "ClusterVersion":
return Optional.ofNullable(clazz.cast(clusterVersion()));
case "EngineFullVersion":
return Optional.ofNullable(clazz.cast(engineFullVersion()));
case "SnapshotType":
return Optional.ofNullable(clazz.cast(snapshotType()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "NumberOfNodes":
return Optional.ofNullable(clazz.cast(numberOfNodes()));
case "DBName":
return Optional.ofNullable(clazz.cast(dbName()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "EncryptedWithHSM":
return Optional.ofNullable(clazz.cast(encryptedWithHSM()));
case "AccountsWithRestoreAccess":
return Optional.ofNullable(clazz.cast(accountsWithRestoreAccess()));
case "OwnerAccount":
return Optional.ofNullable(clazz.cast(ownerAccount()));
case "TotalBackupSizeInMegaBytes":
return Optional.ofNullable(clazz.cast(totalBackupSizeInMegaBytes()));
case "ActualIncrementalBackupSizeInMegaBytes":
return Optional.ofNullable(clazz.cast(actualIncrementalBackupSizeInMegaBytes()));
case "BackupProgressInMegaBytes":
return Optional.ofNullable(clazz.cast(backupProgressInMegaBytes()));
case "CurrentBackupRateInMegaBytesPerSecond":
return Optional.ofNullable(clazz.cast(currentBackupRateInMegaBytesPerSecond()));
case "EstimatedSecondsToCompletion":
return Optional.ofNullable(clazz.cast(estimatedSecondsToCompletion()));
case "ElapsedTimeInSeconds":
return Optional.ofNullable(clazz.cast(elapsedTimeInSeconds()));
case "SourceRegion":
return Optional.ofNullable(clazz.cast(sourceRegion()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "RestorableNodeTypes":
return Optional.ofNullable(clazz.cast(restorableNodeTypes()));
case "EnhancedVpcRouting":
return Optional.ofNullable(clazz.cast(enhancedVpcRouting()));
case "MaintenanceTrackName":
return Optional.ofNullable(clazz.cast(maintenanceTrackName()));
case "ManualSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(manualSnapshotRetentionPeriod()));
case "ManualSnapshotRemainingDays":
return Optional.ofNullable(clazz.cast(manualSnapshotRemainingDays()));
case "SnapshotRetentionStartTime":
return Optional.ofNullable(clazz.cast(snapshotRetentionStartTime()));
case "MasterPasswordSecretArn":
return Optional.ofNullable(clazz.cast(masterPasswordSecretArn()));
case "MasterPasswordSecretKmsKeyId":
return Optional.ofNullable(clazz.cast(masterPasswordSecretKmsKeyId()));
case "SnapshotArn":
return Optional.ofNullable(clazz.cast(snapshotArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function