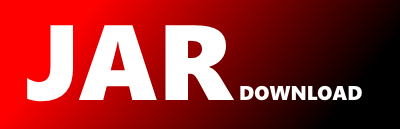
software.amazon.awssdk.services.redshift.model.CreateClusterRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateClusterRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField DB_NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("DBName")
.getter(getter(CreateClusterRequest::dbName)).setter(setter(Builder::dbName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBName").build()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(CreateClusterRequest::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterType").getter(getter(CreateClusterRequest::clusterType)).setter(setter(Builder::clusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterType").build()).build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NodeType").getter(getter(CreateClusterRequest::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField MASTER_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUsername").getter(getter(CreateClusterRequest::masterUsername))
.setter(setter(Builder::masterUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUsername").build()).build();
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(CreateClusterRequest::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField> CLUSTER_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ClusterSecurityGroups")
.getter(getter(CreateClusterRequest::clusterSecurityGroups))
.setter(setter(Builder::clusterSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("ClusterSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ClusterSecurityGroupName").build()).build()).build()).build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroupIds")
.getter(getter(CreateClusterRequest::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroupId").build()).build()).build()).build();
private static final SdkField CLUSTER_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterSubnetGroupName").getter(getter(CreateClusterRequest::clusterSubnetGroupName))
.setter(setter(Builder::clusterSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterSubnetGroupName").build())
.build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(CreateClusterRequest::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(CreateClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField CLUSTER_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterParameterGroupName").getter(getter(CreateClusterRequest::clusterParameterGroupName))
.setter(setter(Builder::clusterParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterParameterGroupName").build())
.build();
private static final SdkField AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("AutomatedSnapshotRetentionPeriod")
.getter(getter(CreateClusterRequest::automatedSnapshotRetentionPeriod))
.setter(setter(Builder::automatedSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomatedSnapshotRetentionPeriod")
.build()).build();
private static final SdkField MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("ManualSnapshotRetentionPeriod")
.getter(getter(CreateClusterRequest::manualSnapshotRetentionPeriod))
.setter(setter(Builder::manualSnapshotRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManualSnapshotRetentionPeriod")
.build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(CreateClusterRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField CLUSTER_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterVersion").getter(getter(CreateClusterRequest::clusterVersion))
.setter(setter(Builder::clusterVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterVersion").build()).build();
private static final SdkField ALLOW_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AllowVersionUpgrade").getter(getter(CreateClusterRequest::allowVersionUpgrade))
.setter(setter(Builder::allowVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowVersionUpgrade").build())
.build();
private static final SdkField NUMBER_OF_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfNodes").getter(getter(CreateClusterRequest::numberOfNodes))
.setter(setter(Builder::numberOfNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfNodes").build()).build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("PubliclyAccessible").getter(getter(CreateClusterRequest::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PubliclyAccessible").build())
.build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted").getter(getter(CreateClusterRequest::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted").build()).build();
private static final SdkField HSM_CLIENT_CERTIFICATE_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HsmClientCertificateIdentifier")
.getter(getter(CreateClusterRequest::hsmClientCertificateIdentifier))
.setter(setter(Builder::hsmClientCertificateIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HsmClientCertificateIdentifier")
.build()).build();
private static final SdkField HSM_CONFIGURATION_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("HsmConfigurationIdentifier")
.getter(getter(CreateClusterRequest::hsmConfigurationIdentifier))
.setter(setter(Builder::hsmConfigurationIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HsmConfigurationIdentifier").build())
.build();
private static final SdkField ELASTIC_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ElasticIp").getter(getter(CreateClusterRequest::elasticIp)).setter(setter(Builder::elasticIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElasticIp").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateClusterRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName("Tag")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Tag").build()).build()).build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(CreateClusterRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField ENHANCED_VPC_ROUTING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnhancedVpcRouting").getter(getter(CreateClusterRequest::enhancedVpcRouting))
.setter(setter(Builder::enhancedVpcRouting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnhancedVpcRouting").build())
.build();
private static final SdkField ADDITIONAL_INFO_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdditionalInfo").getter(getter(CreateClusterRequest::additionalInfo))
.setter(setter(Builder::additionalInfo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalInfo").build()).build();
private static final SdkField> IAM_ROLES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IamRoles")
.getter(getter(CreateClusterRequest::iamRoles))
.setter(setter(Builder::iamRoles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoles").build(),
ListTrait
.builder()
.memberLocationName("IamRoleArn")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("IamRoleArn").build()).build()).build()).build();
private static final SdkField MAINTENANCE_TRACK_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaintenanceTrackName").getter(getter(CreateClusterRequest::maintenanceTrackName))
.setter(setter(Builder::maintenanceTrackName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaintenanceTrackName").build())
.build();
private static final SdkField SNAPSHOT_SCHEDULE_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SnapshotScheduleIdentifier")
.getter(getter(CreateClusterRequest::snapshotScheduleIdentifier))
.setter(setter(Builder::snapshotScheduleIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotScheduleIdentifier").build())
.build();
private static final SdkField AVAILABILITY_ZONE_RELOCATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("AvailabilityZoneRelocation")
.getter(getter(CreateClusterRequest::availabilityZoneRelocation))
.setter(setter(Builder::availabilityZoneRelocation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZoneRelocation").build())
.build();
private static final SdkField AQUA_CONFIGURATION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AquaConfigurationStatus").getter(getter(CreateClusterRequest::aquaConfigurationStatusAsString))
.setter(setter(Builder::aquaConfigurationStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AquaConfigurationStatus").build())
.build();
private static final SdkField DEFAULT_IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DefaultIamRoleArn").getter(getter(CreateClusterRequest::defaultIamRoleArn))
.setter(setter(Builder::defaultIamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultIamRoleArn").build()).build();
private static final SdkField LOAD_SAMPLE_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LoadSampleData").getter(getter(CreateClusterRequest::loadSampleData))
.setter(setter(Builder::loadSampleData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadSampleData").build()).build();
private static final SdkField MANAGE_MASTER_PASSWORD_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ManageMasterPassword").getter(getter(CreateClusterRequest::manageMasterPassword))
.setter(setter(Builder::manageMasterPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManageMasterPassword").build())
.build();
private static final SdkField MASTER_PASSWORD_SECRET_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MasterPasswordSecretKmsKeyId")
.getter(getter(CreateClusterRequest::masterPasswordSecretKmsKeyId))
.setter(setter(Builder::masterPasswordSecretKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterPasswordSecretKmsKeyId")
.build()).build();
private static final SdkField IP_ADDRESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IpAddressType").getter(getter(CreateClusterRequest::ipAddressType))
.setter(setter(Builder::ipAddressType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpAddressType").build()).build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZ").getter(getter(CreateClusterRequest::multiAZ)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField REDSHIFT_IDC_APPLICATION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RedshiftIdcApplicationArn").getter(getter(CreateClusterRequest::redshiftIdcApplicationArn))
.setter(setter(Builder::redshiftIdcApplicationArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftIdcApplicationArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_NAME_FIELD,
CLUSTER_IDENTIFIER_FIELD, CLUSTER_TYPE_FIELD, NODE_TYPE_FIELD, MASTER_USERNAME_FIELD, MASTER_USER_PASSWORD_FIELD,
CLUSTER_SECURITY_GROUPS_FIELD, VPC_SECURITY_GROUP_IDS_FIELD, CLUSTER_SUBNET_GROUP_NAME_FIELD,
AVAILABILITY_ZONE_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, CLUSTER_PARAMETER_GROUP_NAME_FIELD,
AUTOMATED_SNAPSHOT_RETENTION_PERIOD_FIELD, MANUAL_SNAPSHOT_RETENTION_PERIOD_FIELD, PORT_FIELD, CLUSTER_VERSION_FIELD,
ALLOW_VERSION_UPGRADE_FIELD, NUMBER_OF_NODES_FIELD, PUBLICLY_ACCESSIBLE_FIELD, ENCRYPTED_FIELD,
HSM_CLIENT_CERTIFICATE_IDENTIFIER_FIELD, HSM_CONFIGURATION_IDENTIFIER_FIELD, ELASTIC_IP_FIELD, TAGS_FIELD,
KMS_KEY_ID_FIELD, ENHANCED_VPC_ROUTING_FIELD, ADDITIONAL_INFO_FIELD, IAM_ROLES_FIELD, MAINTENANCE_TRACK_NAME_FIELD,
SNAPSHOT_SCHEDULE_IDENTIFIER_FIELD, AVAILABILITY_ZONE_RELOCATION_FIELD, AQUA_CONFIGURATION_STATUS_FIELD,
DEFAULT_IAM_ROLE_ARN_FIELD, LOAD_SAMPLE_DATA_FIELD, MANAGE_MASTER_PASSWORD_FIELD,
MASTER_PASSWORD_SECRET_KMS_KEY_ID_FIELD, IP_ADDRESS_TYPE_FIELD, MULTI_AZ_FIELD, REDSHIFT_IDC_APPLICATION_ARN_FIELD));
private final String dbName;
private final String clusterIdentifier;
private final String clusterType;
private final String nodeType;
private final String masterUsername;
private final String masterUserPassword;
private final List clusterSecurityGroups;
private final List vpcSecurityGroupIds;
private final String clusterSubnetGroupName;
private final String availabilityZone;
private final String preferredMaintenanceWindow;
private final String clusterParameterGroupName;
private final Integer automatedSnapshotRetentionPeriod;
private final Integer manualSnapshotRetentionPeriod;
private final Integer port;
private final String clusterVersion;
private final Boolean allowVersionUpgrade;
private final Integer numberOfNodes;
private final Boolean publiclyAccessible;
private final Boolean encrypted;
private final String hsmClientCertificateIdentifier;
private final String hsmConfigurationIdentifier;
private final String elasticIp;
private final List tags;
private final String kmsKeyId;
private final Boolean enhancedVpcRouting;
private final String additionalInfo;
private final List iamRoles;
private final String maintenanceTrackName;
private final String snapshotScheduleIdentifier;
private final Boolean availabilityZoneRelocation;
private final String aquaConfigurationStatus;
private final String defaultIamRoleArn;
private final String loadSampleData;
private final Boolean manageMasterPassword;
private final String masterPasswordSecretKmsKeyId;
private final String ipAddressType;
private final Boolean multiAZ;
private final String redshiftIdcApplicationArn;
private CreateClusterRequest(BuilderImpl builder) {
super(builder);
this.dbName = builder.dbName;
this.clusterIdentifier = builder.clusterIdentifier;
this.clusterType = builder.clusterType;
this.nodeType = builder.nodeType;
this.masterUsername = builder.masterUsername;
this.masterUserPassword = builder.masterUserPassword;
this.clusterSecurityGroups = builder.clusterSecurityGroups;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.clusterSubnetGroupName = builder.clusterSubnetGroupName;
this.availabilityZone = builder.availabilityZone;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.clusterParameterGroupName = builder.clusterParameterGroupName;
this.automatedSnapshotRetentionPeriod = builder.automatedSnapshotRetentionPeriod;
this.manualSnapshotRetentionPeriod = builder.manualSnapshotRetentionPeriod;
this.port = builder.port;
this.clusterVersion = builder.clusterVersion;
this.allowVersionUpgrade = builder.allowVersionUpgrade;
this.numberOfNodes = builder.numberOfNodes;
this.publiclyAccessible = builder.publiclyAccessible;
this.encrypted = builder.encrypted;
this.hsmClientCertificateIdentifier = builder.hsmClientCertificateIdentifier;
this.hsmConfigurationIdentifier = builder.hsmConfigurationIdentifier;
this.elasticIp = builder.elasticIp;
this.tags = builder.tags;
this.kmsKeyId = builder.kmsKeyId;
this.enhancedVpcRouting = builder.enhancedVpcRouting;
this.additionalInfo = builder.additionalInfo;
this.iamRoles = builder.iamRoles;
this.maintenanceTrackName = builder.maintenanceTrackName;
this.snapshotScheduleIdentifier = builder.snapshotScheduleIdentifier;
this.availabilityZoneRelocation = builder.availabilityZoneRelocation;
this.aquaConfigurationStatus = builder.aquaConfigurationStatus;
this.defaultIamRoleArn = builder.defaultIamRoleArn;
this.loadSampleData = builder.loadSampleData;
this.manageMasterPassword = builder.manageMasterPassword;
this.masterPasswordSecretKmsKeyId = builder.masterPasswordSecretKmsKeyId;
this.ipAddressType = builder.ipAddressType;
this.multiAZ = builder.multiAZ;
this.redshiftIdcApplicationArn = builder.redshiftIdcApplicationArn;
}
/**
*
* The name of the first database to be created when the cluster is created.
*
*
* To create additional databases after the cluster is created, connect to the cluster with a SQL client and use SQL
* commands to create a database. For more information, go to Create a Database in the
* Amazon Redshift Database Developer Guide.
*
*
* Default: dev
*
*
* Constraints:
*
*
* -
*
* Must contain 1 to 64 alphanumeric characters.
*
*
* -
*
* Must contain only lowercase letters.
*
*
* -
*
* Cannot be a word that is reserved by the service. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*
*
* @return The name of the first database to be created when the cluster is created.
*
* To create additional databases after the cluster is created, connect to the cluster with a SQL client and
* use SQL commands to create a database. For more information, go to Create a Database in
* the Amazon Redshift Database Developer Guide.
*
*
* Default: dev
*
*
* Constraints:
*
*
* -
*
* Must contain 1 to 64 alphanumeric characters.
*
*
* -
*
* Must contain only lowercase letters.
*
*
* -
*
* Cannot be a word that is reserved by the service. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public final String dbName() {
return dbName;
}
/**
*
* A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent cluster
* operations such as deleting or modifying. The identifier also appears in the Amazon Redshift console.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an Amazon Web Services account.
*
*
*
*
* Example: myexamplecluster
*
*
* @return A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent
* cluster operations such as deleting or modifying. The identifier also appears in the Amazon Redshift
* console.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an Amazon Web Services account.
*
*
*
*
* Example: myexamplecluster
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The type of the cluster. When cluster type is specified as
*
*
* -
*
* single-node
, the NumberOfNodes parameter is not required.
*
*
* -
*
* multi-node
, the NumberOfNodes parameter is required.
*
*
*
*
* Valid Values: multi-node
| single-node
*
*
* Default: multi-node
*
*
* @return The type of the cluster. When cluster type is specified as
*
* -
*
* single-node
, the NumberOfNodes parameter is not required.
*
*
* -
*
* multi-node
, the NumberOfNodes parameter is required.
*
*
*
*
* Valid Values: multi-node
| single-node
*
*
* Default: multi-node
*/
public final String clusterType() {
return clusterType;
}
/**
*
* The node type to be provisioned for the cluster. For information about node types, go to Working with
* Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
*
*
* @return The node type to be provisioned for the cluster. For information about node types, go to
* Working with Clusters in the Amazon Redshift Cluster Management Guide.
*
* Valid Values: dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
*/
public final String nodeType() {
return nodeType;
}
/**
*
* The user name associated with the admin user account for the cluster that is being created.
*
*
* Constraints:
*
*
* -
*
* Must be 1 - 128 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Must not contain a colon (:) or a slash (/).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*
*
* @return The user name associated with the admin user account for the cluster that is being created.
*
* Constraints:
*
*
* -
*
* Must be 1 - 128 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Must not contain a colon (:) or a slash (/).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public final String masterUsername() {
return masterUsername;
}
/**
*
* The password associated with the admin user account for the cluster that is being created.
*
*
* You can't use MasterUserPassword
if ManageMasterPassword
is true
.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33-126) except '
(single quote), "
* (double quote), \
, /
, or @
.
*
*
*
*
* @return The password associated with the admin user account for the cluster that is being created.
*
* You can't use MasterUserPassword
if ManageMasterPassword
is true
.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33-126) except '
(single quote),
* "
(double quote), \
, /
, or @
.
*
*
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
* For responses, this returns true if the service returned a value for the ClusterSecurityGroups property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasClusterSecurityGroups() {
return clusterSecurityGroups != null && !(clusterSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of security groups to be associated with this cluster.
*
*
* Default: The default cluster security group for Amazon Redshift.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasClusterSecurityGroups} method.
*
*
* @return A list of security groups to be associated with this cluster.
*
* Default: The default cluster security group for Amazon Redshift.
*/
public final List clusterSecurityGroups() {
return clusterSecurityGroups;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroupIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
*
* Default: The default VPC security group is associated with the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroupIds} method.
*
*
* @return A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
* Default: The default VPC security group is associated with the cluster.
*/
public final List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The name of a cluster subnet group to be associated with this cluster.
*
*
* If this parameter is not provided the resulting cluster will be deployed outside virtual private cloud (VPC).
*
*
* @return The name of a cluster subnet group to be associated with this cluster.
*
* If this parameter is not provided the resulting cluster will be deployed outside virtual private cloud
* (VPC).
*/
public final String clusterSubnetGroupName() {
return clusterSubnetGroupName;
}
/**
*
* The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. For example, if you
* have several EC2 instances running in a specific Availability Zone, then you might want the cluster to be
* provisioned in the same zone in order to decrease network latency.
*
*
* Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint.
*
*
* Example: us-east-2d
*
*
* Constraint: The specified Availability Zone must be in the same region as the current endpoint.
*
*
* @return The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. For example,
* if you have several EC2 instances running in a specific Availability Zone, then you might want the
* cluster to be provisioned in the same zone in order to decrease network latency.
*
* Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint.
*
*
* Example: us-east-2d
*
*
* Constraint: The specified Availability Zone must be in the same region as the current endpoint.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The weekly time range (in UTC) during which automated cluster maintenance can occur.
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random day
* of the week. For more information about the time blocks for each region, see Maintenance Windows in Amazon Redshift Cluster Management Guide.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @return The weekly time range (in UTC) during which automated cluster maintenance can occur.
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a
* random day of the week. For more information about the time blocks for each region, see Maintenance Windows in Amazon Redshift Cluster Management Guide.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Minimum 30-minute window.
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The name of the parameter group to be associated with this cluster.
*
*
* Default: The default Amazon Redshift cluster parameter group. For information about the default parameter group,
* go to Working with
* Amazon Redshift Parameter Groups
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The name of the parameter group to be associated with this cluster.
*
* Default: The default Amazon Redshift cluster parameter group. For information about the default parameter
* group, go to Working with
* Amazon Redshift Parameter Groups
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public final String clusterParameterGroupName() {
return clusterParameterGroupName;
}
/**
*
* The number of days that automated snapshots are retained. If the value is 0, automated snapshots are disabled.
* Even if automated snapshots are disabled, you can still create manual snapshots when you want with
* CreateClusterSnapshot.
*
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35 days.
*
*
* Default: 1
*
*
* Constraints: Must be a value from 0 to 35.
*
*
* @return The number of days that automated snapshots are retained. If the value is 0, automated snapshots are
* disabled. Even if automated snapshots are disabled, you can still create manual snapshots when you want
* with CreateClusterSnapshot.
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35
* days.
*
*
* Default: 1
*
*
* Constraints: Must be a value from 0 to 35.
*/
public final Integer automatedSnapshotRetentionPeriod() {
return automatedSnapshotRetentionPeriod;
}
/**
*
* The default number of days to retain a manual snapshot. If the value is -1, the snapshot is retained
* indefinitely. This setting doesn't change the retention period of existing snapshots.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* @return The default number of days to retain a manual snapshot. If the value is -1, the snapshot is retained
* indefinitely. This setting doesn't change the retention period of existing snapshots.
*
* The value must be either -1 or an integer between 1 and 3,653.
*/
public final Integer manualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* The cluster is accessible only via the JDBC and ODBC connection strings. Part of the connection string requires
* the port on which the cluster will listen for incoming connections.
*
*
* Default: 5439
*
*
* Valid Values:
*
*
* -
*
* For clusters with ra3 nodes - Select a port within the ranges 5431-5455
or 8191-8215
.
* (If you have an existing cluster with ra3 nodes, it isn't required that you change the port to these ranges.)
*
*
* -
*
* For clusters with dc2 nodes - Select a port within the range 1150-65535
.
*
*
*
*
* @return The port number on which the cluster accepts incoming connections.
*
* The cluster is accessible only via the JDBC and ODBC connection strings. Part of the connection string
* requires the port on which the cluster will listen for incoming connections.
*
*
* Default: 5439
*
*
* Valid Values:
*
*
* -
*
* For clusters with ra3 nodes - Select a port within the ranges 5431-5455
or
* 8191-8215
. (If you have an existing cluster with ra3 nodes, it isn't required that you
* change the port to these ranges.)
*
*
* -
*
* For clusters with dc2 nodes - Select a port within the range 1150-65535
.
*
*
*/
public final Integer port() {
return port;
}
/**
*
* The version of the Amazon Redshift engine software that you want to deploy on the cluster.
*
*
* The version selected runs on all the nodes in the cluster.
*
*
* Constraints: Only version 1.0 is currently available.
*
*
* Example: 1.0
*
*
* @return The version of the Amazon Redshift engine software that you want to deploy on the cluster.
*
* The version selected runs on all the nodes in the cluster.
*
*
* Constraints: Only version 1.0 is currently available.
*
*
* Example: 1.0
*/
public final String clusterVersion() {
return clusterVersion;
}
/**
*
* If true
, major version upgrades can be applied during the maintenance window to the Amazon Redshift
* engine that is running on the cluster.
*
*
* When a new major version of the Amazon Redshift engine is released, you can request that the service
* automatically apply upgrades during the maintenance window to the Amazon Redshift engine that is running on your
* cluster.
*
*
* Default: true
*
*
* @return If true
, major version upgrades can be applied during the maintenance window to the Amazon
* Redshift engine that is running on the cluster.
*
* When a new major version of the Amazon Redshift engine is released, you can request that the service
* automatically apply upgrades during the maintenance window to the Amazon Redshift engine that is running
* on your cluster.
*
*
* Default: true
*/
public final Boolean allowVersionUpgrade() {
return allowVersionUpgrade;
}
/**
*
* The number of compute nodes in the cluster. This parameter is required when the ClusterType parameter is
* specified as multi-node
.
*
*
* For information about determining how many nodes you need, go to Working with
* Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* If you don't specify this parameter, you get a single-node cluster. When requesting a multi-node cluster, you
* must specify the number of nodes that you want in the cluster.
*
*
* Default: 1
*
*
* Constraints: Value must be at least 1 and no more than 100.
*
*
* @return The number of compute nodes in the cluster. This parameter is required when the ClusterType
* parameter is specified as multi-node
.
*
* For information about determining how many nodes you need, go to
* Working with Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* If you don't specify this parameter, you get a single-node cluster. When requesting a multi-node cluster,
* you must specify the number of nodes that you want in the cluster.
*
*
* Default: 1
*
*
* Constraints: Value must be at least 1 and no more than 100.
*/
public final Integer numberOfNodes() {
return numberOfNodes;
}
/**
*
* If true
, the cluster can be accessed from a public network.
*
*
* @return If true
, the cluster can be accessed from a public network.
*/
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
*
* If true
, the data in the cluster is encrypted at rest.
*
*
* Default: false
*
*
* @return If true
, the data in the cluster is encrypted at rest.
*
* Default: false
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data encryption
* keys stored in an HSM.
*
*
* @return Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data
* encryption keys stored in an HSM.
*/
public final String hsmClientCertificateIdentifier() {
return hsmClientCertificateIdentifier;
}
/**
*
* Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can use to
* retrieve and store keys in an HSM.
*
*
* @return Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can
* use to retrieve and store keys in an HSM.
*/
public final String hsmConfigurationIdentifier() {
return hsmConfigurationIdentifier;
}
/**
*
* The Elastic IP (EIP) address for the cluster.
*
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet gateway.
* Don't specify the Elastic IP address for a publicly accessible cluster with availability zone relocation turned
* on. For more information about provisioning clusters in EC2-VPC, go to Supported
* Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
*
*
* @return The Elastic IP (EIP) address for the cluster.
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet
* gateway. Don't specify the Elastic IP address for a publicly accessible cluster with availability zone
* relocation turned on. For more information about provisioning clusters in EC2-VPC, go to Supported Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
*/
public final String elasticIp() {
return elasticIp;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tag instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tag instances.
*/
public final List tags() {
return tags;
}
/**
*
* The Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the
* cluster.
*
*
* @return The Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the
* cluster.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster
* that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the
* Amazon Redshift Cluster Management Guide.
*
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*
*
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a
* cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC
* Routing in the Amazon Redshift Cluster Management Guide.
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*/
public final Boolean enhancedVpcRouting() {
return enhancedVpcRouting;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public final String additionalInfo() {
return additionalInfo;
}
/**
* For responses, this returns true if the service returned a value for the IamRoles property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasIamRoles() {
return iamRoles != null && !(iamRoles instanceof SdkAutoConstructList);
}
/**
*
* A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other Amazon Web
* Services services. You must supply the IAM roles in their Amazon Resource Name (ARN) format.
*
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go to Quotas and limits in the
* Amazon Redshift Cluster Management Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIamRoles} method.
*
*
* @return A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other
* Amazon Web Services services. You must supply the IAM roles in their Amazon Resource Name (ARN) format.
*
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go to
* Quotas and
* limits in the Amazon Redshift Cluster Management Guide.
*/
public final List iamRoles() {
return iamRoles;
}
/**
*
* An optional parameter for the name of the maintenance track for the cluster. If you don't provide a maintenance
* track name, the cluster is assigned to the current
track.
*
*
* @return An optional parameter for the name of the maintenance track for the cluster. If you don't provide a
* maintenance track name, the cluster is assigned to the current
track.
*/
public final String maintenanceTrackName() {
return maintenanceTrackName;
}
/**
*
* A unique identifier for the snapshot schedule.
*
*
* @return A unique identifier for the snapshot schedule.
*/
public final String snapshotScheduleIdentifier() {
return snapshotScheduleIdentifier;
}
/**
*
* The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster is
* created.
*
*
* @return The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the
* cluster is created.
*/
public final Boolean availabilityZoneRelocation() {
return availabilityZoneRelocation;
}
/**
*
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #aquaConfigurationStatus} will return {@link AquaConfigurationStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #aquaConfigurationStatusAsString}.
*
*
* @return This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
* @see AquaConfigurationStatus
*/
public final AquaConfigurationStatus aquaConfigurationStatus() {
return AquaConfigurationStatus.fromValue(aquaConfigurationStatus);
}
/**
*
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #aquaConfigurationStatus} will return {@link AquaConfigurationStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #aquaConfigurationStatusAsString}.
*
*
* @return This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
* @see AquaConfigurationStatus
*/
public final String aquaConfigurationStatusAsString() {
return aquaConfigurationStatus;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM role that was set as default for the cluster when the cluster was
* created.
*
*
* @return The Amazon Resource Name (ARN) for the IAM role that was set as default for the cluster when the cluster
* was created.
*/
public final String defaultIamRoleArn() {
return defaultIamRoleArn;
}
/**
*
* A flag that specifies whether to load sample data once the cluster is created.
*
*
* @return A flag that specifies whether to load sample data once the cluster is created.
*/
public final String loadSampleData() {
return loadSampleData;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage this cluster's admin credentials. You can't
* use MasterUserPassword
if ManageMasterPassword
is true. If
* ManageMasterPassword
is false or not set, Amazon Redshift uses MasterUserPassword
for
* the admin user account's password.
*
*
* @return If true
, Amazon Redshift uses Secrets Manager to manage this cluster's admin credentials.
* You can't use MasterUserPassword
if ManageMasterPassword
is true. If
* ManageMasterPassword
is false or not set, Amazon Redshift uses
* MasterUserPassword
for the admin user account's password.
*/
public final Boolean manageMasterPassword() {
return manageMasterPassword;
}
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin credentials secret.
* You can only use this parameter if ManageMasterPassword
is true.
*
*
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin credentials
* secret. You can only use this parameter if ManageMasterPassword
is true.
*/
public final String masterPasswordSecretKmsKeyId() {
return masterPasswordSecretKmsKeyId;
}
/**
*
* The IP address types that the cluster supports. Possible values are ipv4
and dualstack
.
*
*
* @return The IP address types that the cluster supports. Possible values are ipv4
and
* dualstack
.
*/
public final String ipAddressType() {
return ipAddressType;
}
/**
*
* If true, Amazon Redshift will deploy the cluster in two Availability Zones (AZ).
*
*
* @return If true, Amazon Redshift will deploy the cluster in two Availability Zones (AZ).
*/
public final Boolean multiAZ() {
return multiAZ;
}
/**
*
* The Amazon resource name (ARN) of the Amazon Redshift IAM Identity Center application.
*
*
* @return The Amazon resource name (ARN) of the Amazon Redshift IAM Identity Center application.
*/
public final String redshiftIdcApplicationArn() {
return redshiftIdcApplicationArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dbName());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(clusterType());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(masterUsername());
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(hasClusterSecurityGroups() ? clusterSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(clusterSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(clusterParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(automatedSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(manualSnapshotRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(clusterVersion());
hashCode = 31 * hashCode + Objects.hashCode(allowVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(numberOfNodes());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(hsmClientCertificateIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(hsmConfigurationIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(elasticIp());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(enhancedVpcRouting());
hashCode = 31 * hashCode + Objects.hashCode(additionalInfo());
hashCode = 31 * hashCode + Objects.hashCode(hasIamRoles() ? iamRoles() : null);
hashCode = 31 * hashCode + Objects.hashCode(maintenanceTrackName());
hashCode = 31 * hashCode + Objects.hashCode(snapshotScheduleIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZoneRelocation());
hashCode = 31 * hashCode + Objects.hashCode(aquaConfigurationStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(defaultIamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(loadSampleData());
hashCode = 31 * hashCode + Objects.hashCode(manageMasterPassword());
hashCode = 31 * hashCode + Objects.hashCode(masterPasswordSecretKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(ipAddressType());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(redshiftIdcApplicationArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateClusterRequest)) {
return false;
}
CreateClusterRequest other = (CreateClusterRequest) obj;
return Objects.equals(dbName(), other.dbName()) && Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(clusterType(), other.clusterType()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(masterUsername(), other.masterUsername())
&& Objects.equals(masterUserPassword(), other.masterUserPassword())
&& hasClusterSecurityGroups() == other.hasClusterSecurityGroups()
&& Objects.equals(clusterSecurityGroups(), other.clusterSecurityGroups())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(clusterSubnetGroupName(), other.clusterSubnetGroupName())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(clusterParameterGroupName(), other.clusterParameterGroupName())
&& Objects.equals(automatedSnapshotRetentionPeriod(), other.automatedSnapshotRetentionPeriod())
&& Objects.equals(manualSnapshotRetentionPeriod(), other.manualSnapshotRetentionPeriod())
&& Objects.equals(port(), other.port()) && Objects.equals(clusterVersion(), other.clusterVersion())
&& Objects.equals(allowVersionUpgrade(), other.allowVersionUpgrade())
&& Objects.equals(numberOfNodes(), other.numberOfNodes())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible())
&& Objects.equals(encrypted(), other.encrypted())
&& Objects.equals(hsmClientCertificateIdentifier(), other.hsmClientCertificateIdentifier())
&& Objects.equals(hsmConfigurationIdentifier(), other.hsmConfigurationIdentifier())
&& Objects.equals(elasticIp(), other.elasticIp()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(enhancedVpcRouting(), other.enhancedVpcRouting())
&& Objects.equals(additionalInfo(), other.additionalInfo()) && hasIamRoles() == other.hasIamRoles()
&& Objects.equals(iamRoles(), other.iamRoles())
&& Objects.equals(maintenanceTrackName(), other.maintenanceTrackName())
&& Objects.equals(snapshotScheduleIdentifier(), other.snapshotScheduleIdentifier())
&& Objects.equals(availabilityZoneRelocation(), other.availabilityZoneRelocation())
&& Objects.equals(aquaConfigurationStatusAsString(), other.aquaConfigurationStatusAsString())
&& Objects.equals(defaultIamRoleArn(), other.defaultIamRoleArn())
&& Objects.equals(loadSampleData(), other.loadSampleData())
&& Objects.equals(manageMasterPassword(), other.manageMasterPassword())
&& Objects.equals(masterPasswordSecretKmsKeyId(), other.masterPasswordSecretKmsKeyId())
&& Objects.equals(ipAddressType(), other.ipAddressType()) && Objects.equals(multiAZ(), other.multiAZ())
&& Objects.equals(redshiftIdcApplicationArn(), other.redshiftIdcApplicationArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateClusterRequest").add("DBName", dbName()).add("ClusterIdentifier", clusterIdentifier())
.add("ClusterType", clusterType()).add("NodeType", nodeType()).add("MasterUsername", masterUsername())
.add("MasterUserPassword", masterUserPassword() == null ? null : "*** Sensitive Data Redacted ***")
.add("ClusterSecurityGroups", hasClusterSecurityGroups() ? clusterSecurityGroups() : null)
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null)
.add("ClusterSubnetGroupName", clusterSubnetGroupName()).add("AvailabilityZone", availabilityZone())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("ClusterParameterGroupName", clusterParameterGroupName())
.add("AutomatedSnapshotRetentionPeriod", automatedSnapshotRetentionPeriod())
.add("ManualSnapshotRetentionPeriod", manualSnapshotRetentionPeriod()).add("Port", port())
.add("ClusterVersion", clusterVersion()).add("AllowVersionUpgrade", allowVersionUpgrade())
.add("NumberOfNodes", numberOfNodes()).add("PubliclyAccessible", publiclyAccessible())
.add("Encrypted", encrypted()).add("HsmClientCertificateIdentifier", hsmClientCertificateIdentifier())
.add("HsmConfigurationIdentifier", hsmConfigurationIdentifier()).add("ElasticIp", elasticIp())
.add("Tags", hasTags() ? tags() : null).add("KmsKeyId", kmsKeyId())
.add("EnhancedVpcRouting", enhancedVpcRouting()).add("AdditionalInfo", additionalInfo())
.add("IamRoles", hasIamRoles() ? iamRoles() : null).add("MaintenanceTrackName", maintenanceTrackName())
.add("SnapshotScheduleIdentifier", snapshotScheduleIdentifier())
.add("AvailabilityZoneRelocation", availabilityZoneRelocation())
.add("AquaConfigurationStatus", aquaConfigurationStatusAsString()).add("DefaultIamRoleArn", defaultIamRoleArn())
.add("LoadSampleData", loadSampleData()).add("ManageMasterPassword", manageMasterPassword())
.add("MasterPasswordSecretKmsKeyId", masterPasswordSecretKmsKeyId()).add("IpAddressType", ipAddressType())
.add("MultiAZ", multiAZ()).add("RedshiftIdcApplicationArn", redshiftIdcApplicationArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBName":
return Optional.ofNullable(clazz.cast(dbName()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "ClusterType":
return Optional.ofNullable(clazz.cast(clusterType()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "MasterUsername":
return Optional.ofNullable(clazz.cast(masterUsername()));
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "ClusterSecurityGroups":
return Optional.ofNullable(clazz.cast(clusterSecurityGroups()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "ClusterSubnetGroupName":
return Optional.ofNullable(clazz.cast(clusterSubnetGroupName()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "ClusterParameterGroupName":
return Optional.ofNullable(clazz.cast(clusterParameterGroupName()));
case "AutomatedSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(automatedSnapshotRetentionPeriod()));
case "ManualSnapshotRetentionPeriod":
return Optional.ofNullable(clazz.cast(manualSnapshotRetentionPeriod()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "ClusterVersion":
return Optional.ofNullable(clazz.cast(clusterVersion()));
case "AllowVersionUpgrade":
return Optional.ofNullable(clazz.cast(allowVersionUpgrade()));
case "NumberOfNodes":
return Optional.ofNullable(clazz.cast(numberOfNodes()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "HsmClientCertificateIdentifier":
return Optional.ofNullable(clazz.cast(hsmClientCertificateIdentifier()));
case "HsmConfigurationIdentifier":
return Optional.ofNullable(clazz.cast(hsmConfigurationIdentifier()));
case "ElasticIp":
return Optional.ofNullable(clazz.cast(elasticIp()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "EnhancedVpcRouting":
return Optional.ofNullable(clazz.cast(enhancedVpcRouting()));
case "AdditionalInfo":
return Optional.ofNullable(clazz.cast(additionalInfo()));
case "IamRoles":
return Optional.ofNullable(clazz.cast(iamRoles()));
case "MaintenanceTrackName":
return Optional.ofNullable(clazz.cast(maintenanceTrackName()));
case "SnapshotScheduleIdentifier":
return Optional.ofNullable(clazz.cast(snapshotScheduleIdentifier()));
case "AvailabilityZoneRelocation":
return Optional.ofNullable(clazz.cast(availabilityZoneRelocation()));
case "AquaConfigurationStatus":
return Optional.ofNullable(clazz.cast(aquaConfigurationStatusAsString()));
case "DefaultIamRoleArn":
return Optional.ofNullable(clazz.cast(defaultIamRoleArn()));
case "LoadSampleData":
return Optional.ofNullable(clazz.cast(loadSampleData()));
case "ManageMasterPassword":
return Optional.ofNullable(clazz.cast(manageMasterPassword()));
case "MasterPasswordSecretKmsKeyId":
return Optional.ofNullable(clazz.cast(masterPasswordSecretKmsKeyId()));
case "IpAddressType":
return Optional.ofNullable(clazz.cast(ipAddressType()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "RedshiftIdcApplicationArn":
return Optional.ofNullable(clazz.cast(redshiftIdcApplicationArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* To create additional databases after the cluster is created, connect to the cluster with a SQL client
* and use SQL commands to create a database. For more information, go to Create a Database
* in the Amazon Redshift Database Developer Guide.
*
*
* Default: dev
*
*
* Constraints:
*
*
* -
*
* Must contain 1 to 64 alphanumeric characters.
*
*
* -
*
* Must contain only lowercase letters.
*
*
* -
*
* Cannot be a word that is reserved by the service. A list of reserved words can be found in Reserved Words in the
* Amazon Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dbName(String dbName);
/**
*
* A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent
* cluster operations such as deleting or modifying. The identifier also appears in the Amazon Redshift console.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an Amazon Web Services account.
*
*
*
*
* Example: myexamplecluster
*
*
* @param clusterIdentifier
* A unique identifier for the cluster. You use this identifier to refer to the cluster for any
* subsequent cluster operations such as deleting or modifying. The identifier also appears in the Amazon
* Redshift console.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* Alphabetic characters must be lowercase.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* -
*
* Must be unique for all clusters within an Amazon Web Services account.
*
*
*
*
* Example: myexamplecluster
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterIdentifier(String clusterIdentifier);
/**
*
* The type of the cluster. When cluster type is specified as
*
*
* -
*
* single-node
, the NumberOfNodes parameter is not required.
*
*
* -
*
* multi-node
, the NumberOfNodes parameter is required.
*
*
*
*
* Valid Values: multi-node
| single-node
*
*
* Default: multi-node
*
*
* @param clusterType
* The type of the cluster. When cluster type is specified as
*
* -
*
* single-node
, the NumberOfNodes parameter is not required.
*
*
* -
*
* multi-node
, the NumberOfNodes parameter is required.
*
*
*
*
* Valid Values: multi-node
| single-node
*
*
* Default: multi-node
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterType(String clusterType);
/**
*
* The node type to be provisioned for the cluster. For information about node types, go to Working
* with Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* Valid Values: dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
*
*
* @param nodeType
* The node type to be provisioned for the cluster. For information about node types, go to
* Working with Clusters in the Amazon Redshift Cluster Management Guide.
*
* Valid Values: dc2.large
| dc2.8xlarge
| ra3.xlplus
|
* ra3.4xlarge
| ra3.16xlarge
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nodeType(String nodeType);
/**
*
* The user name associated with the admin user account for the cluster that is being created.
*
*
* Constraints:
*
*
* -
*
* Must be 1 - 128 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Must not contain a colon (:) or a slash (/).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*
*
* @param masterUsername
* The user name associated with the admin user account for the cluster that is being created.
*
* Constraints:
*
*
* -
*
* Must be 1 - 128 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Must not contain a colon (:) or a slash (/).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the
* Amazon Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterUsername(String masterUsername);
/**
*
* The password associated with the admin user account for the cluster that is being created.
*
*
* You can't use MasterUserPassword
if ManageMasterPassword
is true
.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33-126) except '
(single quote), "
* (double quote), \
, /
, or @
.
*
*
*
*
* @param masterUserPassword
* The password associated with the admin user account for the cluster that is being created.
*
* You can't use MasterUserPassword
if ManageMasterPassword
is
* true
.
*
*
* Constraints:
*
*
* -
*
* Must be between 8 and 64 characters in length.
*
*
* -
*
* Must contain at least one uppercase letter.
*
*
* -
*
* Must contain at least one lowercase letter.
*
*
* -
*
* Must contain one number.
*
*
* -
*
* Can be any printable ASCII character (ASCII code 33-126) except '
(single quote),
* "
(double quote), \
, /
, or @
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterUserPassword(String masterUserPassword);
/**
*
* A list of security groups to be associated with this cluster.
*
*
* Default: The default cluster security group for Amazon Redshift.
*
*
* @param clusterSecurityGroups
* A list of security groups to be associated with this cluster.
*
* Default: The default cluster security group for Amazon Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterSecurityGroups(Collection clusterSecurityGroups);
/**
*
* A list of security groups to be associated with this cluster.
*
*
* Default: The default cluster security group for Amazon Redshift.
*
*
* @param clusterSecurityGroups
* A list of security groups to be associated with this cluster.
*
* Default: The default cluster security group for Amazon Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterSecurityGroups(String... clusterSecurityGroups);
/**
*
* A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
*
* Default: The default VPC security group is associated with the cluster.
*
*
* @param vpcSecurityGroupIds
* A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
* Default: The default VPC security group is associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcSecurityGroupIds(Collection vpcSecurityGroupIds);
/**
*
* A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
*
* Default: The default VPC security group is associated with the cluster.
*
*
* @param vpcSecurityGroupIds
* A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
* Default: The default VPC security group is associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds);
/**
*
* The name of a cluster subnet group to be associated with this cluster.
*
*
* If this parameter is not provided the resulting cluster will be deployed outside virtual private cloud (VPC).
*
*
* @param clusterSubnetGroupName
* The name of a cluster subnet group to be associated with this cluster.
*
* If this parameter is not provided the resulting cluster will be deployed outside virtual private cloud
* (VPC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterSubnetGroupName(String clusterSubnetGroupName);
/**
*
* The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. For example, if
* you have several EC2 instances running in a specific Availability Zone, then you might want the cluster to be
* provisioned in the same zone in order to decrease network latency.
*
*
* Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint.
*
*
* Example: us-east-2d
*
*
* Constraint: The specified Availability Zone must be in the same region as the current endpoint.
*
*
* @param availabilityZone
* The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. For
* example, if you have several EC2 instances running in a specific Availability Zone, then you might
* want the cluster to be provisioned in the same zone in order to decrease network latency.
*
* Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint.
*
*
* Example: us-east-2d
*
*
* Constraint: The specified Availability Zone must be in the same region as the current endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder availabilityZone(String availabilityZone);
/**
*
* The weekly time range (in UTC) during which automated cluster maintenance can occur.
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random
* day of the week. For more information about the time blocks for each region, see Maintenance Windows in Amazon Redshift Cluster Management Guide.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range (in UTC) during which automated cluster maintenance can occur.
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a
* random day of the week. For more information about the time blocks for each region, see Maintenance Windows in Amazon Redshift Cluster Management Guide.
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Minimum 30-minute window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredMaintenanceWindow(String preferredMaintenanceWindow);
/**
*
* The name of the parameter group to be associated with this cluster.
*
*
* Default: The default Amazon Redshift cluster parameter group. For information about the default parameter
* group, go to Working with
* Amazon Redshift Parameter Groups
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @param clusterParameterGroupName
* The name of the parameter group to be associated with this cluster.
*
* Default: The default Amazon Redshift cluster parameter group. For information about the default
* parameter group, go to Working
* with Amazon Redshift Parameter Groups
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterParameterGroupName(String clusterParameterGroupName);
/**
*
* The number of days that automated snapshots are retained. If the value is 0, automated snapshots are
* disabled. Even if automated snapshots are disabled, you can still create manual snapshots when you want with
* CreateClusterSnapshot.
*
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35 days.
*
*
* Default: 1
*
*
* Constraints: Must be a value from 0 to 35.
*
*
* @param automatedSnapshotRetentionPeriod
* The number of days that automated snapshots are retained. If the value is 0, automated snapshots are
* disabled. Even if automated snapshots are disabled, you can still create manual snapshots when you
* want with CreateClusterSnapshot.
*
* You can't disable automated snapshots for RA3 node types. Set the automated retention period from 1-35
* days.
*
*
* Default: 1
*
*
* Constraints: Must be a value from 0 to 35.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder automatedSnapshotRetentionPeriod(Integer automatedSnapshotRetentionPeriod);
/**
*
* The default number of days to retain a manual snapshot. If the value is -1, the snapshot is retained
* indefinitely. This setting doesn't change the retention period of existing snapshots.
*
*
* The value must be either -1 or an integer between 1 and 3,653.
*
*
* @param manualSnapshotRetentionPeriod
* The default number of days to retain a manual snapshot. If the value is -1, the snapshot is retained
* indefinitely. This setting doesn't change the retention period of existing snapshots.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder manualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod);
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* The cluster is accessible only via the JDBC and ODBC connection strings. Part of the connection string
* requires the port on which the cluster will listen for incoming connections.
*
*
* Default: 5439
*
*
* Valid Values:
*
*
* -
*
* For clusters with ra3 nodes - Select a port within the ranges 5431-5455
or
* 8191-8215
. (If you have an existing cluster with ra3 nodes, it isn't required that you change
* the port to these ranges.)
*
*
* -
*
* For clusters with dc2 nodes - Select a port within the range 1150-65535
.
*
*
*
*
* @param port
* The port number on which the cluster accepts incoming connections.
*
* The cluster is accessible only via the JDBC and ODBC connection strings. Part of the connection string
* requires the port on which the cluster will listen for incoming connections.
*
*
* Default: 5439
*
*
* Valid Values:
*
*
* -
*
* For clusters with ra3 nodes - Select a port within the ranges 5431-5455
or
* 8191-8215
. (If you have an existing cluster with ra3 nodes, it isn't required that you
* change the port to these ranges.)
*
*
* -
*
* For clusters with dc2 nodes - Select a port within the range 1150-65535
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder port(Integer port);
/**
*
* The version of the Amazon Redshift engine software that you want to deploy on the cluster.
*
*
* The version selected runs on all the nodes in the cluster.
*
*
* Constraints: Only version 1.0 is currently available.
*
*
* Example: 1.0
*
*
* @param clusterVersion
* The version of the Amazon Redshift engine software that you want to deploy on the cluster.
*
* The version selected runs on all the nodes in the cluster.
*
*
* Constraints: Only version 1.0 is currently available.
*
*
* Example: 1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clusterVersion(String clusterVersion);
/**
*
* If true
, major version upgrades can be applied during the maintenance window to the Amazon
* Redshift engine that is running on the cluster.
*
*
* When a new major version of the Amazon Redshift engine is released, you can request that the service
* automatically apply upgrades during the maintenance window to the Amazon Redshift engine that is running on
* your cluster.
*
*
* Default: true
*
*
* @param allowVersionUpgrade
* If true
, major version upgrades can be applied during the maintenance window to the
* Amazon Redshift engine that is running on the cluster.
*
* When a new major version of the Amazon Redshift engine is released, you can request that the service
* automatically apply upgrades during the maintenance window to the Amazon Redshift engine that is
* running on your cluster.
*
*
* Default: true
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder allowVersionUpgrade(Boolean allowVersionUpgrade);
/**
*
* The number of compute nodes in the cluster. This parameter is required when the ClusterType parameter
* is specified as multi-node
.
*
*
* For information about determining how many nodes you need, go to Working
* with Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* If you don't specify this parameter, you get a single-node cluster. When requesting a multi-node cluster, you
* must specify the number of nodes that you want in the cluster.
*
*
* Default: 1
*
*
* Constraints: Value must be at least 1 and no more than 100.
*
*
* @param numberOfNodes
* The number of compute nodes in the cluster. This parameter is required when the ClusterType
* parameter is specified as multi-node
.
*
* For information about determining how many nodes you need, go to
* Working with Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* If you don't specify this parameter, you get a single-node cluster. When requesting a multi-node
* cluster, you must specify the number of nodes that you want in the cluster.
*
*
* Default: 1
*
*
* Constraints: Value must be at least 1 and no more than 100.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder numberOfNodes(Integer numberOfNodes);
/**
*
* If true
, the cluster can be accessed from a public network.
*
*
* @param publiclyAccessible
* If true
, the cluster can be accessed from a public network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder publiclyAccessible(Boolean publiclyAccessible);
/**
*
* If true
, the data in the cluster is encrypted at rest.
*
*
* Default: false
*
*
* @param encrypted
* If true
, the data in the cluster is encrypted at rest.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder encrypted(Boolean encrypted);
/**
*
* Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data
* encryption keys stored in an HSM.
*
*
* @param hsmClientCertificateIdentifier
* Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data
* encryption keys stored in an HSM.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hsmClientCertificateIdentifier(String hsmClientCertificateIdentifier);
/**
*
* Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can use
* to retrieve and store keys in an HSM.
*
*
* @param hsmConfigurationIdentifier
* Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster
* can use to retrieve and store keys in an HSM.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hsmConfigurationIdentifier(String hsmConfigurationIdentifier);
/**
*
* The Elastic IP (EIP) address for the cluster.
*
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet gateway.
* Don't specify the Elastic IP address for a publicly accessible cluster with availability zone relocation
* turned on. For more information about provisioning clusters in EC2-VPC, go to Supported Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
*
*
* @param elasticIp
* The Elastic IP (EIP) address for the cluster.
*
* Constraints: The cluster must be provisioned in EC2-VPC and publicly-accessible through an Internet
* gateway. Don't specify the Elastic IP address for a publicly accessible cluster with availability zone
* relocation turned on. For more information about provisioning clusters in EC2-VPC, go to Supported Platforms to Launch Your Cluster in the Amazon Redshift Cluster Management Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder elasticIp(String elasticIp);
/**
*
* A list of tag instances.
*
*
* @param tags
* A list of tag instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* A list of tag instances.
*
*
* @param tags
* A list of tag instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A list of tag instances.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.redshift.model.Tag.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.redshift.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.redshift.model.Tag.Builder#build()} is called immediately and its
* result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.redshift.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* The Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the
* cluster.
*
*
* @param kmsKeyId
* The Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in
* the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder kmsKeyId(String kmsKeyId);
/**
*
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster
* that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in
* the Amazon Redshift Cluster Management Guide.
*
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
*
*
* @param enhancedVpcRouting
* An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a
* cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC
* Routing in the Amazon Redshift Cluster Management Guide.
*
* If this option is true
, enhanced VPC routing is enabled.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder enhancedVpcRouting(Boolean enhancedVpcRouting);
/**
*
* Reserved.
*
*
* @param additionalInfo
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder additionalInfo(String additionalInfo);
/**
*
* A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other Amazon
* Web Services services. You must supply the IAM roles in their Amazon Resource Name (ARN) format.
*
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go to Quotas and limits in
* the Amazon Redshift Cluster Management Guide.
*
*
* @param iamRoles
* A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other
* Amazon Web Services services. You must supply the IAM roles in their Amazon Resource Name (ARN)
* format.
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go
* to Quotas and
* limits in the Amazon Redshift Cluster Management Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder iamRoles(Collection iamRoles);
/**
*
* A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other Amazon
* Web Services services. You must supply the IAM roles in their Amazon Resource Name (ARN) format.
*
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go to Quotas and limits in
* the Amazon Redshift Cluster Management Guide.
*
*
* @param iamRoles
* A list of Identity and Access Management (IAM) roles that can be used by the cluster to access other
* Amazon Web Services services. You must supply the IAM roles in their Amazon Resource Name (ARN)
* format.
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go
* to Quotas and
* limits in the Amazon Redshift Cluster Management Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder iamRoles(String... iamRoles);
/**
*
* An optional parameter for the name of the maintenance track for the cluster. If you don't provide a
* maintenance track name, the cluster is assigned to the current
track.
*
*
* @param maintenanceTrackName
* An optional parameter for the name of the maintenance track for the cluster. If you don't provide a
* maintenance track name, the cluster is assigned to the current
track.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maintenanceTrackName(String maintenanceTrackName);
/**
*
* A unique identifier for the snapshot schedule.
*
*
* @param snapshotScheduleIdentifier
* A unique identifier for the snapshot schedule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotScheduleIdentifier(String snapshotScheduleIdentifier);
/**
*
* The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster
* is created.
*
*
* @param availabilityZoneRelocation
* The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the
* cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder availabilityZoneRelocation(Boolean availabilityZoneRelocation);
/**
*
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
*
*
* @param aquaConfigurationStatus
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift
* automatically determines whether to use AQUA (Advanced Query Accelerator).
* @see AquaConfigurationStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see AquaConfigurationStatus
*/
Builder aquaConfigurationStatus(String aquaConfigurationStatus);
/**
*
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift automatically
* determines whether to use AQUA (Advanced Query Accelerator).
*
*
* @param aquaConfigurationStatus
* This parameter is retired. It does not set the AQUA configuration status. Amazon Redshift
* automatically determines whether to use AQUA (Advanced Query Accelerator).
* @see AquaConfigurationStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see AquaConfigurationStatus
*/
Builder aquaConfigurationStatus(AquaConfigurationStatus aquaConfigurationStatus);
/**
*
* The Amazon Resource Name (ARN) for the IAM role that was set as default for the cluster when the cluster was
* created.
*
*
* @param defaultIamRoleArn
* The Amazon Resource Name (ARN) for the IAM role that was set as default for the cluster when the
* cluster was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultIamRoleArn(String defaultIamRoleArn);
/**
*
* A flag that specifies whether to load sample data once the cluster is created.
*
*
* @param loadSampleData
* A flag that specifies whether to load sample data once the cluster is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder loadSampleData(String loadSampleData);
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage this cluster's admin credentials. You
* can't use MasterUserPassword
if ManageMasterPassword
is true. If
* ManageMasterPassword
is false or not set, Amazon Redshift uses MasterUserPassword
* for the admin user account's password.
*
*
* @param manageMasterPassword
* If true
, Amazon Redshift uses Secrets Manager to manage this cluster's admin credentials.
* You can't use MasterUserPassword
if ManageMasterPassword
is true. If
* ManageMasterPassword
is false or not set, Amazon Redshift uses
* MasterUserPassword
for the admin user account's password.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder manageMasterPassword(Boolean manageMasterPassword);
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin credentials
* secret. You can only use this parameter if ManageMasterPassword
is true.
*
*
* @param masterPasswordSecretKmsKeyId
* The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin
* credentials secret. You can only use this parameter if ManageMasterPassword
is true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterPasswordSecretKmsKeyId(String masterPasswordSecretKmsKeyId);
/**
*
* The IP address types that the cluster supports. Possible values are ipv4
and
* dualstack
.
*
*
* @param ipAddressType
* The IP address types that the cluster supports. Possible values are ipv4
and
* dualstack
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder ipAddressType(String ipAddressType);
/**
*
* If true, Amazon Redshift will deploy the cluster in two Availability Zones (AZ).
*
*
* @param multiAZ
* If true, Amazon Redshift will deploy the cluster in two Availability Zones (AZ).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder multiAZ(Boolean multiAZ);
/**
*
* The Amazon resource name (ARN) of the Amazon Redshift IAM Identity Center application.
*
*
* @param redshiftIdcApplicationArn
* The Amazon resource name (ARN) of the Amazon Redshift IAM Identity Center application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder redshiftIdcApplicationArn(String redshiftIdcApplicationArn);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends RedshiftRequest.BuilderImpl implements Builder {
private String dbName;
private String clusterIdentifier;
private String clusterType;
private String nodeType;
private String masterUsername;
private String masterUserPassword;
private List clusterSecurityGroups = DefaultSdkAutoConstructList.getInstance();
private List vpcSecurityGroupIds = DefaultSdkAutoConstructList.getInstance();
private String clusterSubnetGroupName;
private String availabilityZone;
private String preferredMaintenanceWindow;
private String clusterParameterGroupName;
private Integer automatedSnapshotRetentionPeriod;
private Integer manualSnapshotRetentionPeriod;
private Integer port;
private String clusterVersion;
private Boolean allowVersionUpgrade;
private Integer numberOfNodes;
private Boolean publiclyAccessible;
private Boolean encrypted;
private String hsmClientCertificateIdentifier;
private String hsmConfigurationIdentifier;
private String elasticIp;
private List tags = DefaultSdkAutoConstructList.getInstance();
private String kmsKeyId;
private Boolean enhancedVpcRouting;
private String additionalInfo;
private List iamRoles = DefaultSdkAutoConstructList.getInstance();
private String maintenanceTrackName;
private String snapshotScheduleIdentifier;
private Boolean availabilityZoneRelocation;
private String aquaConfigurationStatus;
private String defaultIamRoleArn;
private String loadSampleData;
private Boolean manageMasterPassword;
private String masterPasswordSecretKmsKeyId;
private String ipAddressType;
private Boolean multiAZ;
private String redshiftIdcApplicationArn;
private BuilderImpl() {
}
private BuilderImpl(CreateClusterRequest model) {
super(model);
dbName(model.dbName);
clusterIdentifier(model.clusterIdentifier);
clusterType(model.clusterType);
nodeType(model.nodeType);
masterUsername(model.masterUsername);
masterUserPassword(model.masterUserPassword);
clusterSecurityGroups(model.clusterSecurityGroups);
vpcSecurityGroupIds(model.vpcSecurityGroupIds);
clusterSubnetGroupName(model.clusterSubnetGroupName);
availabilityZone(model.availabilityZone);
preferredMaintenanceWindow(model.preferredMaintenanceWindow);
clusterParameterGroupName(model.clusterParameterGroupName);
automatedSnapshotRetentionPeriod(model.automatedSnapshotRetentionPeriod);
manualSnapshotRetentionPeriod(model.manualSnapshotRetentionPeriod);
port(model.port);
clusterVersion(model.clusterVersion);
allowVersionUpgrade(model.allowVersionUpgrade);
numberOfNodes(model.numberOfNodes);
publiclyAccessible(model.publiclyAccessible);
encrypted(model.encrypted);
hsmClientCertificateIdentifier(model.hsmClientCertificateIdentifier);
hsmConfigurationIdentifier(model.hsmConfigurationIdentifier);
elasticIp(model.elasticIp);
tags(model.tags);
kmsKeyId(model.kmsKeyId);
enhancedVpcRouting(model.enhancedVpcRouting);
additionalInfo(model.additionalInfo);
iamRoles(model.iamRoles);
maintenanceTrackName(model.maintenanceTrackName);
snapshotScheduleIdentifier(model.snapshotScheduleIdentifier);
availabilityZoneRelocation(model.availabilityZoneRelocation);
aquaConfigurationStatus(model.aquaConfigurationStatus);
defaultIamRoleArn(model.defaultIamRoleArn);
loadSampleData(model.loadSampleData);
manageMasterPassword(model.manageMasterPassword);
masterPasswordSecretKmsKeyId(model.masterPasswordSecretKmsKeyId);
ipAddressType(model.ipAddressType);
multiAZ(model.multiAZ);
redshiftIdcApplicationArn(model.redshiftIdcApplicationArn);
}
public final String getDbName() {
return dbName;
}
public final void setDbName(String dbName) {
this.dbName = dbName;
}
@Override
public final Builder dbName(String dbName) {
this.dbName = dbName;
return this;
}
public final String getClusterIdentifier() {
return clusterIdentifier;
}
public final void setClusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
}
@Override
public final Builder clusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
return this;
}
public final String getClusterType() {
return clusterType;
}
public final void setClusterType(String clusterType) {
this.clusterType = clusterType;
}
@Override
public final Builder clusterType(String clusterType) {
this.clusterType = clusterType;
return this;
}
public final String getNodeType() {
return nodeType;
}
public final void setNodeType(String nodeType) {
this.nodeType = nodeType;
}
@Override
public final Builder nodeType(String nodeType) {
this.nodeType = nodeType;
return this;
}
public final String getMasterUsername() {
return masterUsername;
}
public final void setMasterUsername(String masterUsername) {
this.masterUsername = masterUsername;
}
@Override
public final Builder masterUsername(String masterUsername) {
this.masterUsername = masterUsername;
return this;
}
public final String getMasterUserPassword() {
return masterUserPassword;
}
public final void setMasterUserPassword(String masterUserPassword) {
this.masterUserPassword = masterUserPassword;
}
@Override
public final Builder masterUserPassword(String masterUserPassword) {
this.masterUserPassword = masterUserPassword;
return this;
}
public final Collection getClusterSecurityGroups() {
if (clusterSecurityGroups instanceof SdkAutoConstructList) {
return null;
}
return clusterSecurityGroups;
}
public final void setClusterSecurityGroups(Collection clusterSecurityGroups) {
this.clusterSecurityGroups = ClusterSecurityGroupNameListCopier.copy(clusterSecurityGroups);
}
@Override
public final Builder clusterSecurityGroups(Collection clusterSecurityGroups) {
this.clusterSecurityGroups = ClusterSecurityGroupNameListCopier.copy(clusterSecurityGroups);
return this;
}
@Override
@SafeVarargs
public final Builder clusterSecurityGroups(String... clusterSecurityGroups) {
clusterSecurityGroups(Arrays.asList(clusterSecurityGroups));
return this;
}
public final Collection getVpcSecurityGroupIds() {
if (vpcSecurityGroupIds instanceof SdkAutoConstructList) {
return null;
}
return vpcSecurityGroupIds;
}
public final void setVpcSecurityGroupIds(Collection vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = VpcSecurityGroupIdListCopier.copy(vpcSecurityGroupIds);
}
@Override
public final Builder vpcSecurityGroupIds(Collection vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = VpcSecurityGroupIdListCopier.copy(vpcSecurityGroupIds);
return this;
}
@Override
@SafeVarargs
public final Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds) {
vpcSecurityGroupIds(Arrays.asList(vpcSecurityGroupIds));
return this;
}
public final String getClusterSubnetGroupName() {
return clusterSubnetGroupName;
}
public final void setClusterSubnetGroupName(String clusterSubnetGroupName) {
this.clusterSubnetGroupName = clusterSubnetGroupName;
}
@Override
public final Builder clusterSubnetGroupName(String clusterSubnetGroupName) {
this.clusterSubnetGroupName = clusterSubnetGroupName;
return this;
}
public final String getAvailabilityZone() {
return availabilityZone;
}
public final void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
@Override
public final Builder availabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
public final String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
public final void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
@Override
public final Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
public final String getClusterParameterGroupName() {
return clusterParameterGroupName;
}
public final void setClusterParameterGroupName(String clusterParameterGroupName) {
this.clusterParameterGroupName = clusterParameterGroupName;
}
@Override
public final Builder clusterParameterGroupName(String clusterParameterGroupName) {
this.clusterParameterGroupName = clusterParameterGroupName;
return this;
}
public final Integer getAutomatedSnapshotRetentionPeriod() {
return automatedSnapshotRetentionPeriod;
}
public final void setAutomatedSnapshotRetentionPeriod(Integer automatedSnapshotRetentionPeriod) {
this.automatedSnapshotRetentionPeriod = automatedSnapshotRetentionPeriod;
}
@Override
public final Builder automatedSnapshotRetentionPeriod(Integer automatedSnapshotRetentionPeriod) {
this.automatedSnapshotRetentionPeriod = automatedSnapshotRetentionPeriod;
return this;
}
public final Integer getManualSnapshotRetentionPeriod() {
return manualSnapshotRetentionPeriod;
}
public final void setManualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod) {
this.manualSnapshotRetentionPeriod = manualSnapshotRetentionPeriod;
}
@Override
public final Builder manualSnapshotRetentionPeriod(Integer manualSnapshotRetentionPeriod) {
this.manualSnapshotRetentionPeriod = manualSnapshotRetentionPeriod;
return this;
}
public final Integer getPort() {
return port;
}
public final void setPort(Integer port) {
this.port = port;
}
@Override
public final Builder port(Integer port) {
this.port = port;
return this;
}
public final String getClusterVersion() {
return clusterVersion;
}
public final void setClusterVersion(String clusterVersion) {
this.clusterVersion = clusterVersion;
}
@Override
public final Builder clusterVersion(String clusterVersion) {
this.clusterVersion = clusterVersion;
return this;
}
public final Boolean getAllowVersionUpgrade() {
return allowVersionUpgrade;
}
public final void setAllowVersionUpgrade(Boolean allowVersionUpgrade) {
this.allowVersionUpgrade = allowVersionUpgrade;
}
@Override
public final Builder allowVersionUpgrade(Boolean allowVersionUpgrade) {
this.allowVersionUpgrade = allowVersionUpgrade;
return this;
}
public final Integer getNumberOfNodes() {
return numberOfNodes;
}
public final void setNumberOfNodes(Integer numberOfNodes) {
this.numberOfNodes = numberOfNodes;
}
@Override
public final Builder numberOfNodes(Integer numberOfNodes) {
this.numberOfNodes = numberOfNodes;
return this;
}
public final Boolean getPubliclyAccessible() {
return publiclyAccessible;
}
public final void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
@Override
public final Builder publiclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
return this;
}
public final Boolean getEncrypted() {
return encrypted;
}
public final void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
@Override
public final Builder encrypted(Boolean encrypted) {
this.encrypted = encrypted;
return this;
}
public final String getHsmClientCertificateIdentifier() {
return hsmClientCertificateIdentifier;
}
public final void setHsmClientCertificateIdentifier(String hsmClientCertificateIdentifier) {
this.hsmClientCertificateIdentifier = hsmClientCertificateIdentifier;
}
@Override
public final Builder hsmClientCertificateIdentifier(String hsmClientCertificateIdentifier) {
this.hsmClientCertificateIdentifier = hsmClientCertificateIdentifier;
return this;
}
public final String getHsmConfigurationIdentifier() {
return hsmConfigurationIdentifier;
}
public final void setHsmConfigurationIdentifier(String hsmConfigurationIdentifier) {
this.hsmConfigurationIdentifier = hsmConfigurationIdentifier;
}
@Override
public final Builder hsmConfigurationIdentifier(String hsmConfigurationIdentifier) {
this.hsmConfigurationIdentifier = hsmConfigurationIdentifier;
return this;
}
public final String getElasticIp() {
return elasticIp;
}
public final void setElasticIp(String elasticIp) {
this.elasticIp = elasticIp;
}
@Override
public final Builder elasticIp(String elasticIp) {
this.elasticIp = elasticIp;
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getKmsKeyId() {
return kmsKeyId;
}
public final void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
@Override
public final Builder kmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
public final Boolean getEnhancedVpcRouting() {
return enhancedVpcRouting;
}
public final void setEnhancedVpcRouting(Boolean enhancedVpcRouting) {
this.enhancedVpcRouting = enhancedVpcRouting;
}
@Override
public final Builder enhancedVpcRouting(Boolean enhancedVpcRouting) {
this.enhancedVpcRouting = enhancedVpcRouting;
return this;
}
public final String getAdditionalInfo() {
return additionalInfo;
}
public final void setAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
}
@Override
public final Builder additionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
return this;
}
public final Collection getIamRoles() {
if (iamRoles instanceof SdkAutoConstructList) {
return null;
}
return iamRoles;
}
public final void setIamRoles(Collection iamRoles) {
this.iamRoles = IamRoleArnListCopier.copy(iamRoles);
}
@Override
public final Builder iamRoles(Collection iamRoles) {
this.iamRoles = IamRoleArnListCopier.copy(iamRoles);
return this;
}
@Override
@SafeVarargs
public final Builder iamRoles(String... iamRoles) {
iamRoles(Arrays.asList(iamRoles));
return this;
}
public final String getMaintenanceTrackName() {
return maintenanceTrackName;
}
public final void setMaintenanceTrackName(String maintenanceTrackName) {
this.maintenanceTrackName = maintenanceTrackName;
}
@Override
public final Builder maintenanceTrackName(String maintenanceTrackName) {
this.maintenanceTrackName = maintenanceTrackName;
return this;
}
public final String getSnapshotScheduleIdentifier() {
return snapshotScheduleIdentifier;
}
public final void setSnapshotScheduleIdentifier(String snapshotScheduleIdentifier) {
this.snapshotScheduleIdentifier = snapshotScheduleIdentifier;
}
@Override
public final Builder snapshotScheduleIdentifier(String snapshotScheduleIdentifier) {
this.snapshotScheduleIdentifier = snapshotScheduleIdentifier;
return this;
}
public final Boolean getAvailabilityZoneRelocation() {
return availabilityZoneRelocation;
}
public final void setAvailabilityZoneRelocation(Boolean availabilityZoneRelocation) {
this.availabilityZoneRelocation = availabilityZoneRelocation;
}
@Override
public final Builder availabilityZoneRelocation(Boolean availabilityZoneRelocation) {
this.availabilityZoneRelocation = availabilityZoneRelocation;
return this;
}
public final String getAquaConfigurationStatus() {
return aquaConfigurationStatus;
}
public final void setAquaConfigurationStatus(String aquaConfigurationStatus) {
this.aquaConfigurationStatus = aquaConfigurationStatus;
}
@Override
public final Builder aquaConfigurationStatus(String aquaConfigurationStatus) {
this.aquaConfigurationStatus = aquaConfigurationStatus;
return this;
}
@Override
public final Builder aquaConfigurationStatus(AquaConfigurationStatus aquaConfigurationStatus) {
this.aquaConfigurationStatus(aquaConfigurationStatus == null ? null : aquaConfigurationStatus.toString());
return this;
}
public final String getDefaultIamRoleArn() {
return defaultIamRoleArn;
}
public final void setDefaultIamRoleArn(String defaultIamRoleArn) {
this.defaultIamRoleArn = defaultIamRoleArn;
}
@Override
public final Builder defaultIamRoleArn(String defaultIamRoleArn) {
this.defaultIamRoleArn = defaultIamRoleArn;
return this;
}
public final String getLoadSampleData() {
return loadSampleData;
}
public final void setLoadSampleData(String loadSampleData) {
this.loadSampleData = loadSampleData;
}
@Override
public final Builder loadSampleData(String loadSampleData) {
this.loadSampleData = loadSampleData;
return this;
}
public final Boolean getManageMasterPassword() {
return manageMasterPassword;
}
public final void setManageMasterPassword(Boolean manageMasterPassword) {
this.manageMasterPassword = manageMasterPassword;
}
@Override
public final Builder manageMasterPassword(Boolean manageMasterPassword) {
this.manageMasterPassword = manageMasterPassword;
return this;
}
public final String getMasterPasswordSecretKmsKeyId() {
return masterPasswordSecretKmsKeyId;
}
public final void setMasterPasswordSecretKmsKeyId(String masterPasswordSecretKmsKeyId) {
this.masterPasswordSecretKmsKeyId = masterPasswordSecretKmsKeyId;
}
@Override
public final Builder masterPasswordSecretKmsKeyId(String masterPasswordSecretKmsKeyId) {
this.masterPasswordSecretKmsKeyId = masterPasswordSecretKmsKeyId;
return this;
}
public final String getIpAddressType() {
return ipAddressType;
}
public final void setIpAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
}
@Override
public final Builder ipAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
return this;
}
public final Boolean getMultiAZ() {
return multiAZ;
}
public final void setMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
}
@Override
public final Builder multiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
return this;
}
public final String getRedshiftIdcApplicationArn() {
return redshiftIdcApplicationArn;
}
public final void setRedshiftIdcApplicationArn(String redshiftIdcApplicationArn) {
this.redshiftIdcApplicationArn = redshiftIdcApplicationArn;
}
@Override
public final Builder redshiftIdcApplicationArn(String redshiftIdcApplicationArn) {
this.redshiftIdcApplicationArn = redshiftIdcApplicationArn;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateClusterRequest build() {
return new CreateClusterRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}