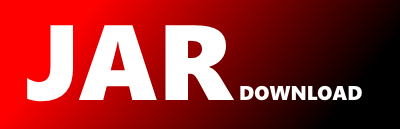
software.amazon.awssdk.services.redshift.model.CreateRedshiftIdcApplicationRequest Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateRedshiftIdcApplicationRequest extends RedshiftRequest implements
ToCopyableBuilder {
private static final SdkField IDC_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdcInstanceArn").getter(getter(CreateRedshiftIdcApplicationRequest::idcInstanceArn))
.setter(setter(Builder::idcInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdcInstanceArn").build()).build();
private static final SdkField REDSHIFT_IDC_APPLICATION_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RedshiftIdcApplicationName")
.getter(getter(CreateRedshiftIdcApplicationRequest::redshiftIdcApplicationName))
.setter(setter(Builder::redshiftIdcApplicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftIdcApplicationName").build())
.build();
private static final SdkField IDENTITY_NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityNamespace").getter(getter(CreateRedshiftIdcApplicationRequest::identityNamespace))
.setter(setter(Builder::identityNamespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityNamespace").build()).build();
private static final SdkField IDC_DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdcDisplayName").getter(getter(CreateRedshiftIdcApplicationRequest::idcDisplayName))
.setter(setter(Builder::idcDisplayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdcDisplayName").build()).build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IamRoleArn").getter(getter(CreateRedshiftIdcApplicationRequest::iamRoleArn))
.setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField> AUTHORIZED_TOKEN_ISSUER_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AuthorizedTokenIssuerList")
.getter(getter(CreateRedshiftIdcApplicationRequest::authorizedTokenIssuerList))
.setter(setter(Builder::authorizedTokenIssuerList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthorizedTokenIssuerList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AuthorizedTokenIssuer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_INTEGRATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ServiceIntegrations")
.getter(getter(CreateRedshiftIdcApplicationRequest::serviceIntegrations))
.setter(setter(Builder::serviceIntegrations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceIntegrations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceIntegrationsUnion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IDC_INSTANCE_ARN_FIELD,
REDSHIFT_IDC_APPLICATION_NAME_FIELD, IDENTITY_NAMESPACE_FIELD, IDC_DISPLAY_NAME_FIELD, IAM_ROLE_ARN_FIELD,
AUTHORIZED_TOKEN_ISSUER_LIST_FIELD, SERVICE_INTEGRATIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("IdcInstanceArn", IDC_INSTANCE_ARN_FIELD);
put("RedshiftIdcApplicationName", REDSHIFT_IDC_APPLICATION_NAME_FIELD);
put("IdentityNamespace", IDENTITY_NAMESPACE_FIELD);
put("IdcDisplayName", IDC_DISPLAY_NAME_FIELD);
put("IamRoleArn", IAM_ROLE_ARN_FIELD);
put("AuthorizedTokenIssuerList", AUTHORIZED_TOKEN_ISSUER_LIST_FIELD);
put("ServiceIntegrations", SERVICE_INTEGRATIONS_FIELD);
}
});
private final String idcInstanceArn;
private final String redshiftIdcApplicationName;
private final String identityNamespace;
private final String idcDisplayName;
private final String iamRoleArn;
private final List authorizedTokenIssuerList;
private final List serviceIntegrations;
private CreateRedshiftIdcApplicationRequest(BuilderImpl builder) {
super(builder);
this.idcInstanceArn = builder.idcInstanceArn;
this.redshiftIdcApplicationName = builder.redshiftIdcApplicationName;
this.identityNamespace = builder.identityNamespace;
this.idcDisplayName = builder.idcDisplayName;
this.iamRoleArn = builder.iamRoleArn;
this.authorizedTokenIssuerList = builder.authorizedTokenIssuerList;
this.serviceIntegrations = builder.serviceIntegrations;
}
/**
*
* The Amazon resource name (ARN) of the IAM Identity Center instance where Amazon Redshift creates a new managed
* application.
*
*
* @return The Amazon resource name (ARN) of the IAM Identity Center instance where Amazon Redshift creates a new
* managed application.
*/
public final String idcInstanceArn() {
return idcInstanceArn;
}
/**
*
* The name of the Redshift application in IAM Identity Center.
*
*
* @return The name of the Redshift application in IAM Identity Center.
*/
public final String redshiftIdcApplicationName() {
return redshiftIdcApplicationName;
}
/**
*
* The namespace for the Amazon Redshift IAM Identity Center application instance. It determines which managed
* application verifies the connection token.
*
*
* @return The namespace for the Amazon Redshift IAM Identity Center application instance. It determines which
* managed application verifies the connection token.
*/
public final String identityNamespace() {
return identityNamespace;
}
/**
*
* The display name for the Amazon Redshift IAM Identity Center application instance. It appears in the console.
*
*
* @return The display name for the Amazon Redshift IAM Identity Center application instance. It appears in the
* console.
*/
public final String idcDisplayName() {
return idcDisplayName;
}
/**
*
* The IAM role ARN for the Amazon Redshift IAM Identity Center application instance. It has the required
* permissions to be assumed and invoke the IDC Identity Center API.
*
*
* @return The IAM role ARN for the Amazon Redshift IAM Identity Center application instance. It has the required
* permissions to be assumed and invoke the IDC Identity Center API.
*/
public final String iamRoleArn() {
return iamRoleArn;
}
/**
* For responses, this returns true if the service returned a value for the AuthorizedTokenIssuerList property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAuthorizedTokenIssuerList() {
return authorizedTokenIssuerList != null && !(authorizedTokenIssuerList instanceof SdkAutoConstructList);
}
/**
*
* The token issuer list for the Amazon Redshift IAM Identity Center application instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAuthorizedTokenIssuerList} method.
*
*
* @return The token issuer list for the Amazon Redshift IAM Identity Center application instance.
*/
public final List authorizedTokenIssuerList() {
return authorizedTokenIssuerList;
}
/**
* For responses, this returns true if the service returned a value for the ServiceIntegrations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServiceIntegrations() {
return serviceIntegrations != null && !(serviceIntegrations instanceof SdkAutoConstructList);
}
/**
*
* A collection of service integrations for the Redshift IAM Identity Center application.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceIntegrations} method.
*
*
* @return A collection of service integrations for the Redshift IAM Identity Center application.
*/
public final List serviceIntegrations() {
return serviceIntegrations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(idcInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(redshiftIdcApplicationName());
hashCode = 31 * hashCode + Objects.hashCode(identityNamespace());
hashCode = 31 * hashCode + Objects.hashCode(idcDisplayName());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(hasAuthorizedTokenIssuerList() ? authorizedTokenIssuerList() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceIntegrations() ? serviceIntegrations() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateRedshiftIdcApplicationRequest)) {
return false;
}
CreateRedshiftIdcApplicationRequest other = (CreateRedshiftIdcApplicationRequest) obj;
return Objects.equals(idcInstanceArn(), other.idcInstanceArn())
&& Objects.equals(redshiftIdcApplicationName(), other.redshiftIdcApplicationName())
&& Objects.equals(identityNamespace(), other.identityNamespace())
&& Objects.equals(idcDisplayName(), other.idcDisplayName()) && Objects.equals(iamRoleArn(), other.iamRoleArn())
&& hasAuthorizedTokenIssuerList() == other.hasAuthorizedTokenIssuerList()
&& Objects.equals(authorizedTokenIssuerList(), other.authorizedTokenIssuerList())
&& hasServiceIntegrations() == other.hasServiceIntegrations()
&& Objects.equals(serviceIntegrations(), other.serviceIntegrations());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateRedshiftIdcApplicationRequest").add("IdcInstanceArn", idcInstanceArn())
.add("RedshiftIdcApplicationName", redshiftIdcApplicationName()).add("IdentityNamespace", identityNamespace())
.add("IdcDisplayName", idcDisplayName()).add("IamRoleArn", iamRoleArn())
.add("AuthorizedTokenIssuerList", hasAuthorizedTokenIssuerList() ? authorizedTokenIssuerList() : null)
.add("ServiceIntegrations", hasServiceIntegrations() ? serviceIntegrations() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IdcInstanceArn":
return Optional.ofNullable(clazz.cast(idcInstanceArn()));
case "RedshiftIdcApplicationName":
return Optional.ofNullable(clazz.cast(redshiftIdcApplicationName()));
case "IdentityNamespace":
return Optional.ofNullable(clazz.cast(identityNamespace()));
case "IdcDisplayName":
return Optional.ofNullable(clazz.cast(idcDisplayName()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "AuthorizedTokenIssuerList":
return Optional.ofNullable(clazz.cast(authorizedTokenIssuerList()));
case "ServiceIntegrations":
return Optional.ofNullable(clazz.cast(serviceIntegrations()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function