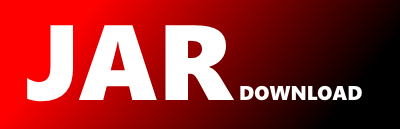
software.amazon.awssdk.services.redshift.model.Recommendation Maven / Gradle / Ivy
Show all versions of redshift Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.redshift.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An Amazon Redshift Advisor recommended action on the Amazon Redshift cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Recommendation implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Recommendation::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterIdentifier").getter(getter(Recommendation::clusterIdentifier))
.setter(setter(Builder::clusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterIdentifier").build()).build();
private static final SdkField NAMESPACE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NamespaceArn").getter(getter(Recommendation::namespaceArn)).setter(setter(Builder::namespaceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NamespaceArn").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedAt").getter(getter(Recommendation::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField RECOMMENDATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecommendationType").getter(getter(Recommendation::recommendationType))
.setter(setter(Builder::recommendationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendationType").build())
.build();
private static final SdkField TITLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Title")
.getter(getter(Recommendation::title)).setter(setter(Builder::title))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Title").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Recommendation::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField OBSERVATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Observation").getter(getter(Recommendation::observation)).setter(setter(Builder::observation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Observation").build()).build();
private static final SdkField IMPACT_RANKING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ImpactRanking").getter(getter(Recommendation::impactRankingAsString))
.setter(setter(Builder::impactRanking))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImpactRanking").build()).build();
private static final SdkField RECOMMENDATION_TEXT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RecommendationText").getter(getter(Recommendation::recommendationText))
.setter(setter(Builder::recommendationText))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendationText").build())
.build();
private static final SdkField> RECOMMENDED_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RecommendedActions")
.getter(getter(Recommendation::recommendedActions))
.setter(setter(Builder::recommendedActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecommendedActions").build(),
ListTrait
.builder()
.memberLocationName("RecommendedAction")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RecommendedAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("RecommendedAction").build()).build()).build()).build();
private static final SdkField> REFERENCE_LINKS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReferenceLinks")
.getter(getter(Recommendation::referenceLinks))
.setter(setter(Builder::referenceLinks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReferenceLinks").build(),
ListTrait
.builder()
.memberLocationName("ReferenceLink")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ReferenceLink::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReferenceLink").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD,
CLUSTER_IDENTIFIER_FIELD, NAMESPACE_ARN_FIELD, CREATED_AT_FIELD, RECOMMENDATION_TYPE_FIELD, TITLE_FIELD,
DESCRIPTION_FIELD, OBSERVATION_FIELD, IMPACT_RANKING_FIELD, RECOMMENDATION_TEXT_FIELD, RECOMMENDED_ACTIONS_FIELD,
REFERENCE_LINKS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Id", ID_FIELD);
put("ClusterIdentifier", CLUSTER_IDENTIFIER_FIELD);
put("NamespaceArn", NAMESPACE_ARN_FIELD);
put("CreatedAt", CREATED_AT_FIELD);
put("RecommendationType", RECOMMENDATION_TYPE_FIELD);
put("Title", TITLE_FIELD);
put("Description", DESCRIPTION_FIELD);
put("Observation", OBSERVATION_FIELD);
put("ImpactRanking", IMPACT_RANKING_FIELD);
put("RecommendationText", RECOMMENDATION_TEXT_FIELD);
put("RecommendedActions", RECOMMENDED_ACTIONS_FIELD);
put("ReferenceLinks", REFERENCE_LINKS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String id;
private final String clusterIdentifier;
private final String namespaceArn;
private final Instant createdAt;
private final String recommendationType;
private final String title;
private final String description;
private final String observation;
private final String impactRanking;
private final String recommendationText;
private final List recommendedActions;
private final List referenceLinks;
private Recommendation(BuilderImpl builder) {
this.id = builder.id;
this.clusterIdentifier = builder.clusterIdentifier;
this.namespaceArn = builder.namespaceArn;
this.createdAt = builder.createdAt;
this.recommendationType = builder.recommendationType;
this.title = builder.title;
this.description = builder.description;
this.observation = builder.observation;
this.impactRanking = builder.impactRanking;
this.recommendationText = builder.recommendationText;
this.recommendedActions = builder.recommendedActions;
this.referenceLinks = builder.referenceLinks;
}
/**
*
* A unique identifier of the Advisor recommendation.
*
*
* @return A unique identifier of the Advisor recommendation.
*/
public final String id() {
return id;
}
/**
*
* The unique identifier of the cluster for which the recommendation is returned.
*
*
* @return The unique identifier of the cluster for which the recommendation is returned.
*/
public final String clusterIdentifier() {
return clusterIdentifier;
}
/**
*
* The Amazon Redshift cluster namespace ARN for which the recommendations is returned.
*
*
* @return The Amazon Redshift cluster namespace ARN for which the recommendations is returned.
*/
public final String namespaceArn() {
return namespaceArn;
}
/**
*
* The date and time (UTC) that the recommendation was created.
*
*
* @return The date and time (UTC) that the recommendation was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The type of Advisor recommendation.
*
*
* @return The type of Advisor recommendation.
*/
public final String recommendationType() {
return recommendationType;
}
/**
*
* The title of the recommendation.
*
*
* @return The title of the recommendation.
*/
public final String title() {
return title;
}
/**
*
* The description of the recommendation.
*
*
* @return The description of the recommendation.
*/
public final String description() {
return description;
}
/**
*
* The description of what was observed about your cluster.
*
*
* @return The description of what was observed about your cluster.
*/
public final String observation() {
return observation;
}
/**
*
* The scale of the impact that the Advisor recommendation has to the performance and cost of the cluster.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #impactRanking}
* will return {@link ImpactRankingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #impactRankingAsString}.
*
*
* @return The scale of the impact that the Advisor recommendation has to the performance and cost of the cluster.
* @see ImpactRankingType
*/
public final ImpactRankingType impactRanking() {
return ImpactRankingType.fromValue(impactRanking);
}
/**
*
* The scale of the impact that the Advisor recommendation has to the performance and cost of the cluster.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #impactRanking}
* will return {@link ImpactRankingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #impactRankingAsString}.
*
*
* @return The scale of the impact that the Advisor recommendation has to the performance and cost of the cluster.
* @see ImpactRankingType
*/
public final String impactRankingAsString() {
return impactRanking;
}
/**
*
* The description of the recommendation.
*
*
* @return The description of the recommendation.
*/
public final String recommendationText() {
return recommendationText;
}
/**
* For responses, this returns true if the service returned a value for the RecommendedActions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRecommendedActions() {
return recommendedActions != null && !(recommendedActions instanceof SdkAutoConstructList);
}
/**
*
* List of Amazon Redshift recommended actions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRecommendedActions} method.
*
*
* @return List of Amazon Redshift recommended actions.
*/
public final List recommendedActions() {
return recommendedActions;
}
/**
* For responses, this returns true if the service returned a value for the ReferenceLinks property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReferenceLinks() {
return referenceLinks != null && !(referenceLinks instanceof SdkAutoConstructList);
}
/**
*
* List of helpful links for more information about the Advisor recommendation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReferenceLinks} method.
*
*
* @return List of helpful links for more information about the Advisor recommendation.
*/
public final List referenceLinks() {
return referenceLinks;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(clusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(namespaceArn());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(recommendationType());
hashCode = 31 * hashCode + Objects.hashCode(title());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(observation());
hashCode = 31 * hashCode + Objects.hashCode(impactRankingAsString());
hashCode = 31 * hashCode + Objects.hashCode(recommendationText());
hashCode = 31 * hashCode + Objects.hashCode(hasRecommendedActions() ? recommendedActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasReferenceLinks() ? referenceLinks() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Recommendation)) {
return false;
}
Recommendation other = (Recommendation) obj;
return Objects.equals(id(), other.id()) && Objects.equals(clusterIdentifier(), other.clusterIdentifier())
&& Objects.equals(namespaceArn(), other.namespaceArn()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(recommendationType(), other.recommendationType()) && Objects.equals(title(), other.title())
&& Objects.equals(description(), other.description()) && Objects.equals(observation(), other.observation())
&& Objects.equals(impactRankingAsString(), other.impactRankingAsString())
&& Objects.equals(recommendationText(), other.recommendationText())
&& hasRecommendedActions() == other.hasRecommendedActions()
&& Objects.equals(recommendedActions(), other.recommendedActions())
&& hasReferenceLinks() == other.hasReferenceLinks() && Objects.equals(referenceLinks(), other.referenceLinks());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Recommendation").add("Id", id()).add("ClusterIdentifier", clusterIdentifier())
.add("NamespaceArn", namespaceArn()).add("CreatedAt", createdAt())
.add("RecommendationType", recommendationType()).add("Title", title()).add("Description", description())
.add("Observation", observation()).add("ImpactRanking", impactRankingAsString())
.add("RecommendationText", recommendationText())
.add("RecommendedActions", hasRecommendedActions() ? recommendedActions() : null)
.add("ReferenceLinks", hasReferenceLinks() ? referenceLinks() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "ClusterIdentifier":
return Optional.ofNullable(clazz.cast(clusterIdentifier()));
case "NamespaceArn":
return Optional.ofNullable(clazz.cast(namespaceArn()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "RecommendationType":
return Optional.ofNullable(clazz.cast(recommendationType()));
case "Title":
return Optional.ofNullable(clazz.cast(title()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Observation":
return Optional.ofNullable(clazz.cast(observation()));
case "ImpactRanking":
return Optional.ofNullable(clazz.cast(impactRankingAsString()));
case "RecommendationText":
return Optional.ofNullable(clazz.cast(recommendationText()));
case "RecommendedActions":
return Optional.ofNullable(clazz.cast(recommendedActions()));
case "ReferenceLinks":
return Optional.ofNullable(clazz.cast(referenceLinks()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function